/*
* Copyright 2009 ZXing authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.zxing.pdf417.decoder;
import com.google.zxing.FormatException;
import com.google.zxing.common.BitMatrix;
/**
* <p>
* This class parses the BitMatrix image into codewords.
* </p>
*
* @author SITA Lab (kevin.osullivan@sita.aero)
*/
final class BitMatrixParser {
private static final int[] NO_ERRORS = new int[0];
private static final int MAX_ROW_DIFFERENCE = 6;
private static final int MAX_ROWS = 90;
//private static final int MAX_COLUMNS = 30;
// Maximum Codewords (Data + Error)
private static final int MAX_CW_CAPACITY = 929;
private static final int MODULES_IN_SYMBOL = 17;
private final BitMatrix bitMatrix;
private int rows = 0;
//private int columns = 0;
private int leftColumnECData = 0;
private int rightColumnECData = 0;
private int eraseCount = 0;
private int[] erasures;
private int ecLevel = -1;
BitMatrixParser(BitMatrix bitMatrix) {
this.bitMatrix = bitMatrix;
}
/**
* To ensure separability of rows, codewords of consecutive rows belong to
* different subsets of all possible codewords. This routine scans the
* symbols in the barcode. When it finds a number of consecutive rows which
* are the same, it assumes that this is a row of codewords and processes
* them into a codeword array.
*
* @return an array of codewords.
*/
int[] readCodewords() throws FormatException {
int width = bitMatrix.getWidth();
int height = bitMatrix.getHeight();
erasures = new int[MAX_CW_CAPACITY];
// Get the number of pixels in a module across the X dimension
//float moduleWidth = bitMatrix.getModuleWidth();
float moduleWidth = 1.0f; // Image has been sampled and reduced
int[] rowCounters = new int[width];
int[] codewords = new int[MAX_CW_CAPACITY];
int next = 0;
int matchingConsecutiveScans = 0;
boolean rowInProgress = false;
int rowNumber = 0;
int rowHeight = 0;
for (int i = 1; i < height; i++) {
if (rowNumber >= MAX_ROWS) {
// Something is wrong, since we have exceeded
// the maximum rows in the specification.
throw FormatException.getFormatInstance();
}
int rowDifference = 0;
// Scan a line of modules and check the
// difference between this and the previous line
for (int j = 0; j < width; j++) {
// Accumulate differences between this line and the
// previous line.
if (bitMatrix.get(j, i) != bitMatrix.get(j, i - 1)) {
rowDifference++;
}
}
if (rowDifference <= moduleWidth * MAX_ROW_DIFFERENCE) {
for (int j = 0; j < width; j++) {
// Accumulate the black pixels on this line
if (bitMatrix.get(j, i)) {
rowCounters[j]++;
}
}
// Increment the number of consecutive rows of pixels
// that are more or less the same
matchingConsecutiveScans++;
// Height of a row is a multiple of the module size in pixels
// Usually at least 3 times the module size
if (matchingConsecutiveScans >= moduleWidth * 2) { // MGMG
// We have some previous matches as well as a match here
// Set processing a unique row.
rowInProgress = true;
}
} else {
if (rowInProgress) {
// Process Row
next = processRow(rowCounters, rowNumber, rowHeight, codewords, next);
if (next == -1) {
// Something is wrong, since we have exceeded
// the maximum columns in the specification.
throw FormatException.getFormatInstance();
}
// Reinitialize the row counters.
for (int j = 0; j < rowCounters.length; j++) {
rowCounters[j] = 0;
}
rowNumber++;
rowHeight = 0;
}
matchingConsecutiveScans = 0;
rowInProgress = false;
}
rowHeight++;
}
// Check for a row that was in progress before we exited above.
if (rowInProgress) {
// Process Row
if (rowNumber >= MAX_ROWS) {
// Something is wrong, since we have exceeded
// the maximum rows in the specification.
throw FormatException.getFormatInstance();
}
next = processRow(rowCounters, rowNumber, rowHeight, codewords, next);
rowNumber++;
rows = rowNumber;
}
erasures = trimArray(erasures, eraseCount);
return trimArray(codewords, next);
}
/**
* Trim the array to the required size.
*
* @param array the array
* @param size the size to trim it to
* @return the new trimmed array
*/
private static int[] trimArray(int[] array, int size) {
if (size < 0) {
throw new IllegalArgumentException();
}
if (size == 0) {
return NO_ERRORS;
}
int[] a = new int[size];
System.arraycopy(array, 0, a, 0, size);
return a;
}
/**
* Convert the symbols in the row to codewords.
* Each PDF417 symbol character consists of four bar elements and four space
* elements, each of which can be one to six modules wide. The four bar and
* four space elements shall measure 17 modules in total.
*
* @param rowCounters an array containing the counts of black pixels for each column
* in the row.
* @param rowNumber the current row number of codewords.
* @param rowHeight the height of this row in pixels.
* @param codewords the codeword array to save codewords into.
* @param next the next available index into the codewords array.
* @return the next available index into the codeword array after processing
* this row.
*/
int processRow(int[] rowCounters, int rowNumber, int rowHeight, int[] codewords, int next)
throws FormatException {
int width = bitMatrix.getWidth();
int columnNumber = 0;
long symbol = 0;
for (int i = 0; i < width; i += MODULES_IN_SYMBOL) {
// This happens in real life and is almost surely a rare misdecode
if (i + MODULES_IN_SYMBOL > rowCounters.length) {
throw FormatException.getFormatInstance();
}
for (int mask = MODULES_IN_SYMBOL - 1; mask >= 0; mask--) {
if (rowCounters[i + (MODULES_IN_SYMBOL - 1 - mask)] >= rowHeight >>> 1) {
symbol |= 1L << mask;
}
}
if (columnNumber > 0) {
int cw = getCodeword(symbol);
if (cw < 0 && i < width - MODULES_IN_SYMBOL) {
// Skip errors on the Right row indicator column
if (eraseCount >= erasures.length) {
throw FormatException.getFormatInstance();
}
erasures[eraseCount] = next;
next++;
eraseCount++;
} else {
codewords[next++] = cw;
}
} else {
// Left row indicator column
int cw = getCodeword(symbol);
if (ecLevel < 0) {
switch (rowNumber % 3) {
case 0:
break;
case 1:
leftColumnECData = cw;
break;
case 2:
break;
}
}
}
symbol = 0;
//columns = columnNumber;
columnNumber++;
}
if (col
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
由于自身需要以及网上查阅了些资料都不怎么满足,就自己试着下载了zxing源码,然后试着整合出了android版本的zxing扫描器。该项目为zxing android版本扫描器全部源码实现,导入eclipse可直接运行,能快速扫描二维码和条形码。最主要的是可以学习了,嘎嘎,赶紧下下来,不下别后悔!
资源推荐
资源详情
资源评论
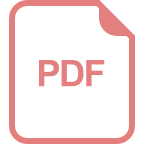
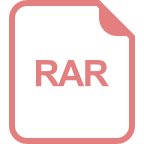
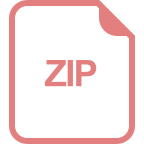
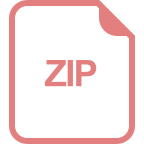
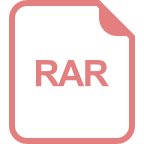
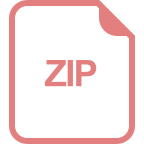
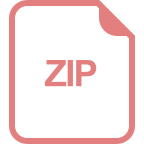
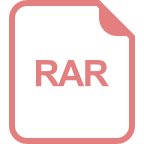
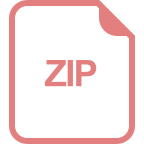
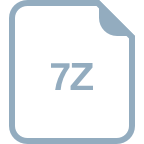
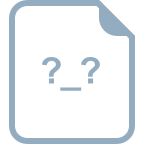
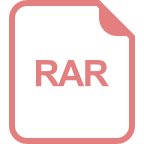
收起资源包目录

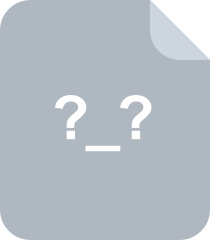
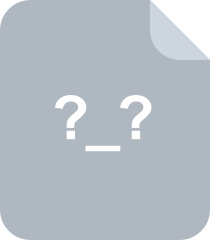
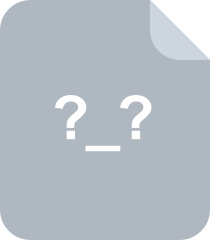
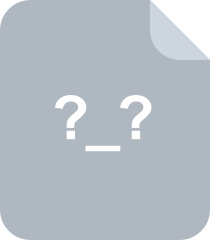
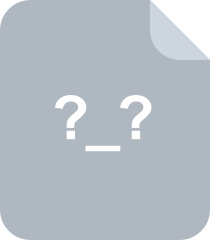
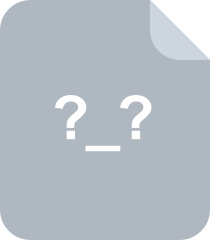
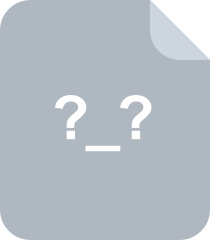
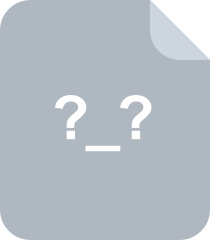
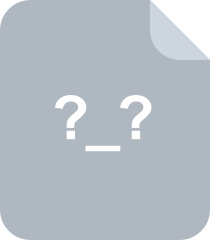
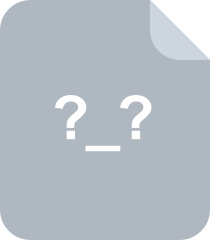
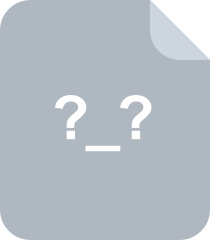
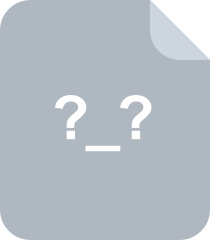
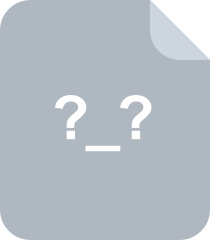
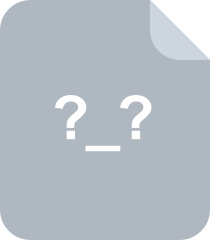
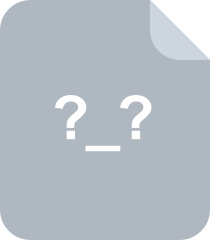
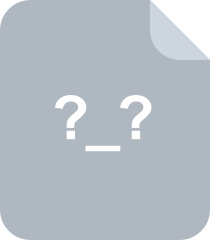
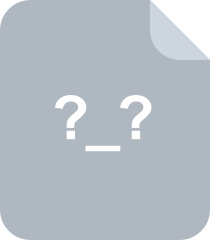
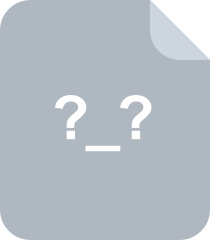
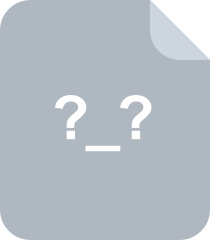
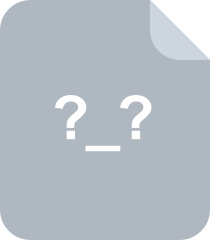
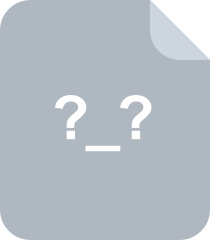
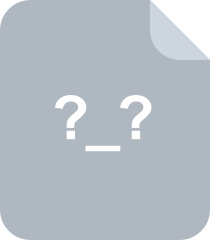
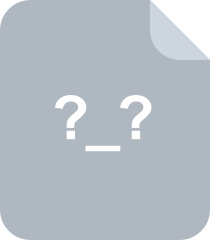
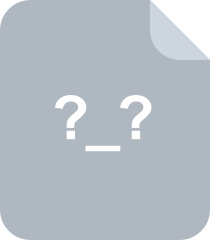
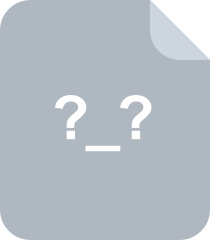
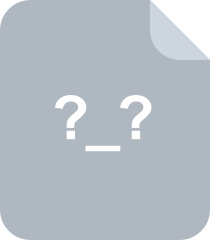
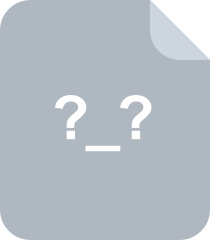
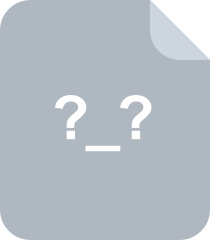
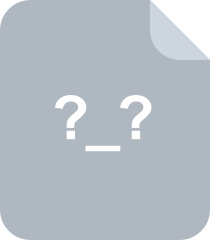
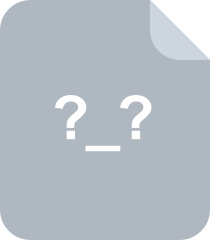
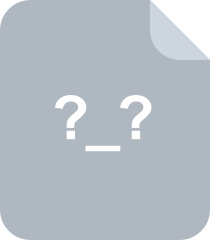
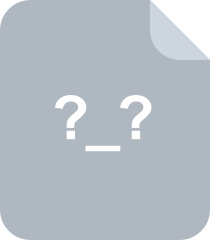
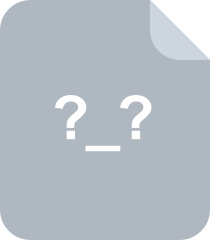
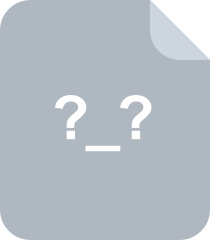
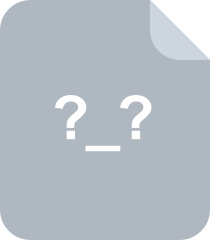
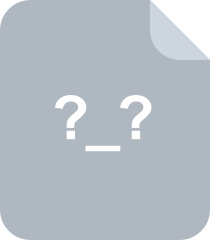
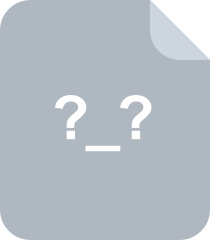
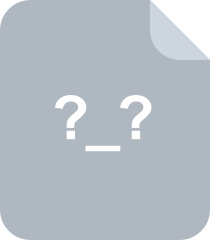
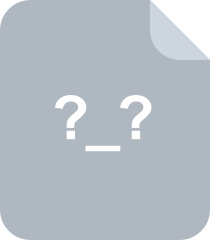
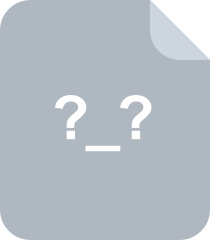
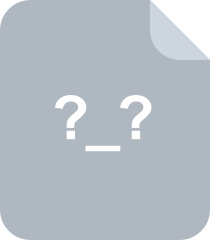
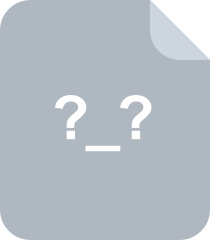
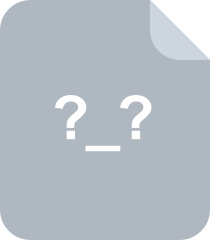
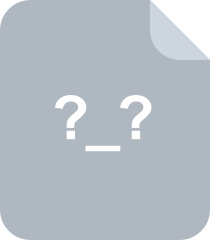
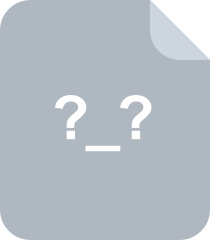
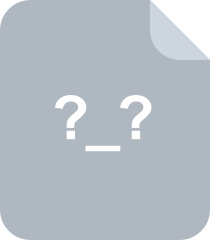
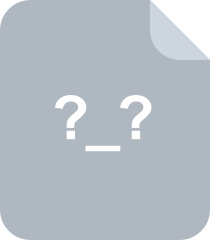
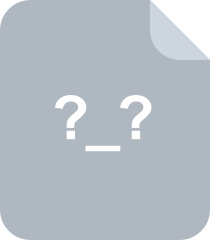
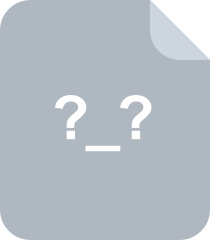
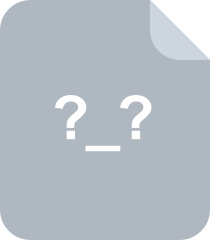
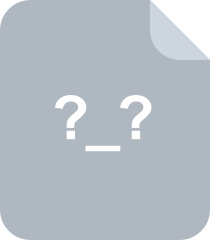
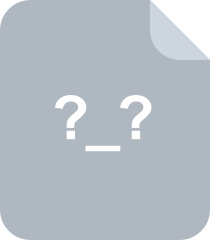
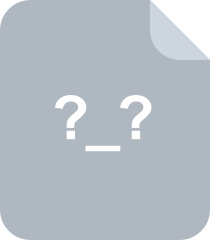
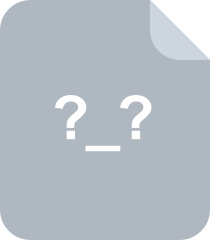
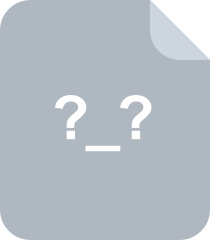
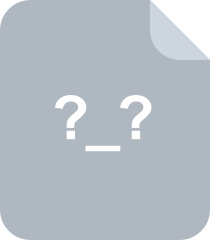
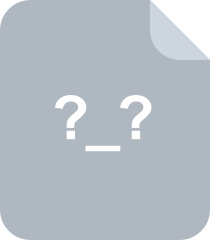
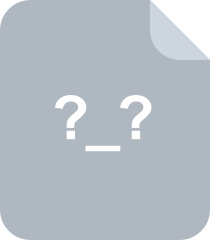
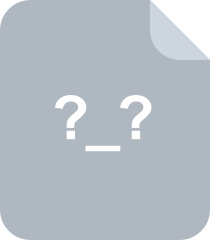
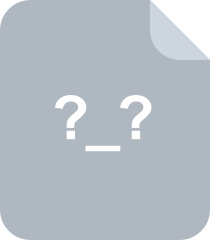
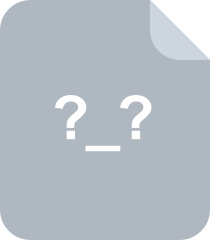
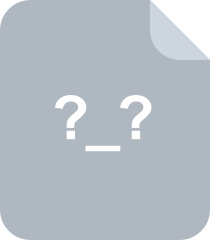
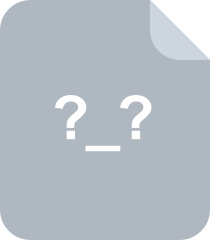
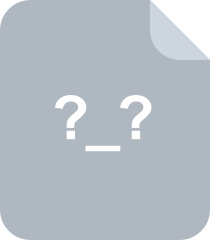
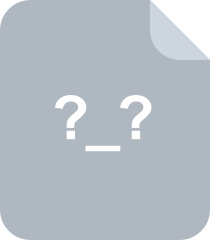
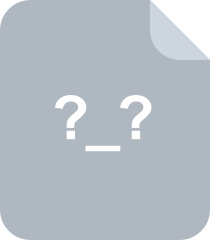
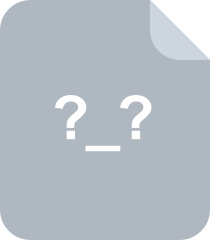
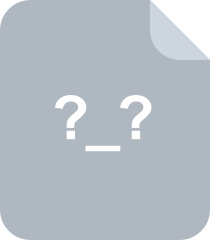
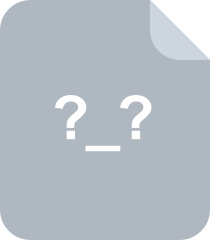
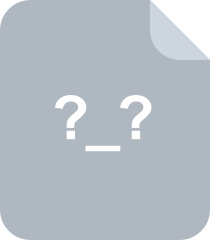
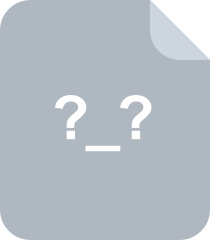
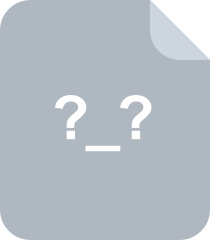
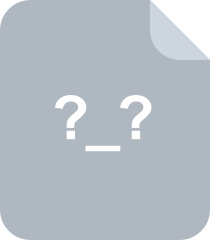
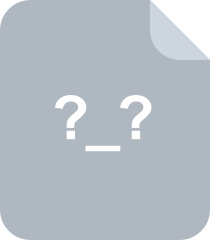
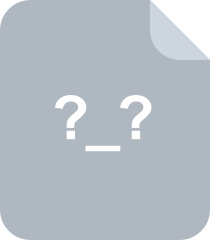
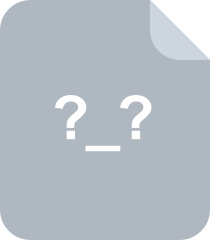
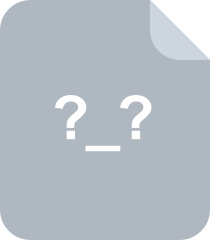
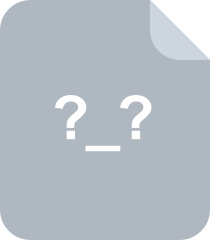
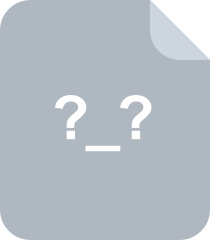
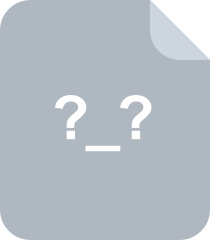
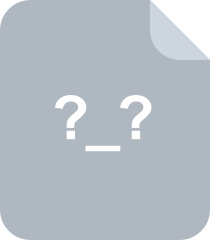
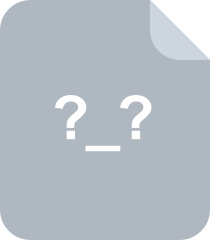
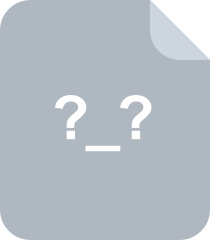
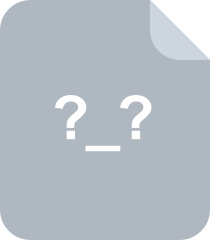
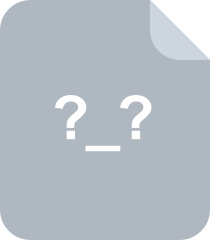
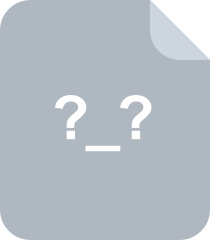
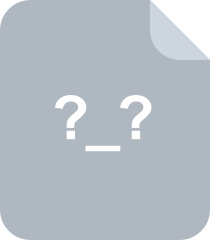
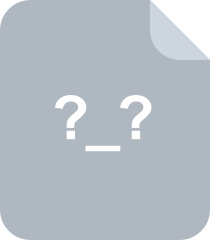
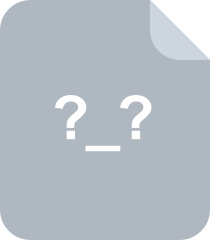
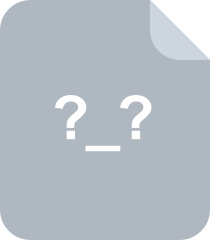
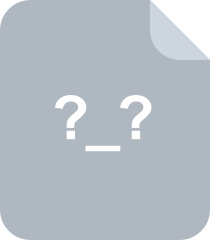
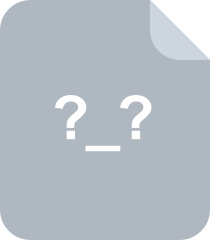
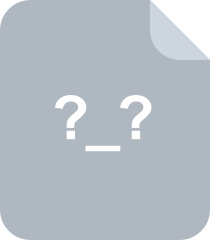
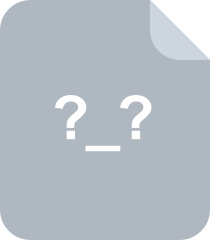
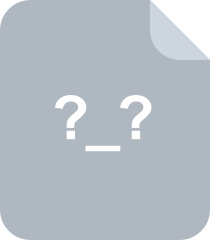
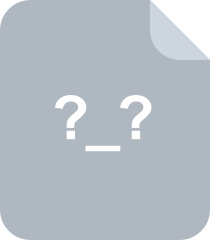
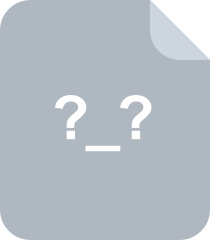
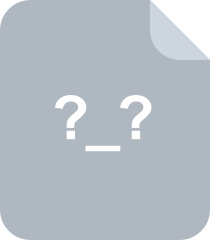
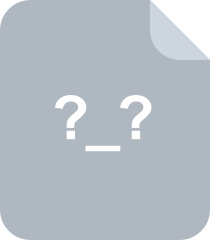
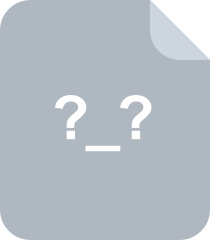
共 645 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7

zqwblog
- 粉丝: 2
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

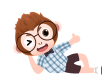
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


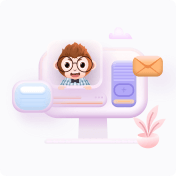
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页