package com.syntax.analysis;
import static org.junit.Assert.*;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.util.Scanner;
import org.junit.Test;
public class SyntaxAnalysisTest {
private static Stacks stack = new Stacks();
private static char s[] = null;
private static String msg = "";
// + * m ( ) #
static PreAnalysisTable S = new PreAnalysisTable("E", "E", "TA", "TA", "E","E");
static PreAnalysisTable A = new PreAnalysisTable("E", "E", "UB", "UB", "E","E");
static PreAnalysisTable B = new PreAnalysisTable("E", "E", "m", ")s(", "E","E");
static PreAnalysisTable T = new PreAnalysisTable("TA+", "E", "E", "E", "","");
static PreAnalysisTable U = new PreAnalysisTable("", "UB*", "TA", "TA", "","");
// public static String readFile(String filename) {
// String inputStr = "";
// int end = 0;
// try {
// File file = new File(filename);
// if (file.isFile() && new File(filename).exists()) {
// BufferedReader reader = new BufferedReader(
// new InputStreamReader(new FileInputStream(new File(
// filename)), "UTF-8"));
// while ((end = reader.read()) != -1) {
// inputStr += (char) end;
// }
// reader.close();
// return inputStr;
// } else {
// System.out.println("file is not exist!");
// }
// } catch (FileNotFoundException e) {
// e.printStackTrace();
// } catch (IOException e) {
// e.printStackTrace();
// }
// return null;
// }
public static char[] subS(char s[]){
String str="";
for(int i=0;i<s.length;i++){
str+=s[i];
}
str=str.substring(1, str.length());
return str.toCharArray();
}
public static boolean compare(int b) {
String ttt = stack.getPop().toString();
char d = ttt.charAt(0);//栈顶符号
// System.out.println(d);
if (d == '+' || d == '*' || d == 'm' || d == '(' || d == ')') {
if (s[b] == d) {
msg =""+ s[b];
msg += "匹配";
b++;
stack.pop();
s=subS(s);
return true;
} else {
System.out.println("error");
return false;
}
}
// -----------------------------------
else if (d == 'S') {
if (s[b] == 'm') {
msg = "S->AT";
stack.pop();
for (int i = 0; i < S.getForm().length(); i++) {
stack.push(S.getForm().toCharArray()[i]);
}
return true;
} else if (s[b] == '(') {
msg = "S->AT";
stack.pop();
for (int i = 0; i < S.getForlbracket().length(); i++) {
stack.push(S.getForlbracket().toCharArray()[i]);
}
return true;
} else {
System.out.println("error");
return false;
}
}
// --------------------------------------
else if (d == 'A') {
if (s[b] == 'm') {
msg = "A->BU";
stack.pop();
for (int i = 0; i < A.getForm().length(); i++) {
stack.push(A.getForm().toCharArray()[i]);
}
return true;
} else if (s[b] == '(') {
msg = "A->BU";
stack.pop();
for (int i = 0; i < A.getForlbracket().length(); i++) {
stack.push(A.getForlbracket().toCharArray()[i]);
}
return true;
} else {
System.out.println("error");
return false;
}
}
// --------------------------------------
else if (d == 'B') {
if (s[b] == 'm') {
stack.pop();
msg = "B->m";
for (int i = 0; i < B.getForm().length(); i++) {
stack.push(B.getForm().toCharArray()[i]);
}
return true;
} else if (s[b] == '(') {
stack.pop();
for (int i = 0; i < B.getForlbracket().length(); i++) {
stack.push(B.getForlbracket().toCharArray()[i]);
}
msg = "B->(S)";
return true;
} else {
System.out.println("error");
return false;
}
}
// ---------------------------------------
else if (d == 'U') {
if (s[b] == '+') {
stack.pop();
msg = "U->$";
return true;
} else if (s[b] == '*') {
stack.pop();
for (int i = 0; i < U.getForstar().length(); i++) {
stack.push(U.getForstar().toCharArray()[i]);
}
msg = "U->*BU";
return true;
} else if (s[b] == '#') {
stack.pop();
msg = "U->$";
return true;
} else if (s[b] == ')') {
stack.pop();
msg = "U->$";
return true;
} else {
System.out.println("error");
return false;
}
}
// ------------------------------------
else if (d == 'T') {
if (s[b] == '+') {
stack.pop();
for (int i = 0; i < T.getForadd().length(); i++) {
stack.push(T.getForadd().toCharArray()[i]);
}
msg = "T->+AT";
return true;
} else if (s[b] == ')') {
stack.pop();
msg = "T->$";
return true;
} else if (s[b] == '#') {
stack.pop();
msg = "T->$";
return true;
} else {
System.out.println("error");
return false;
}
} else if (d == '#') {
msg = "接受";
System.out.print("接受");
return false;
} else {
System.out.println("error");
return false;
}
}
//打印字符数组
public static String printArray(char b[]){
String str="";
for(int i=0;i<b.length;i++){
str+=b[i];
}
return str;
}
public static void main(String args[]) throws IOException {
// Stacks stack = new Stacks();
Scanner scan;
String inStr = "";// 输入串
int slength = 0;
int b = 0;
while (true) {
// 清空栈
stack.clear();
System.out.println("\r\n请输入待分析的字符串:");
scan = new Scanner(System.in);
inStr = scan.nextLine();
inStr+="#";
s=inStr.toCharArray();//输入串数组
// 输入exit代表退出
if (inStr.equals("exit")) {
break;
}
// 取得输入穿的长度
slength =inStr.length();
// 先把#和文法的开始符号入栈
stack.push("#");
stack.push("S");
System.out.println("用预测分析法分析符号串的过程:\r\n");
System.out.println("step\tStack\tString\tRule");
int st = 1;// st用于记录分析步骤
System.out.print(st+"\t");//打印步骤号
stack.print();//打印栈中元素
System.out.print(printArray(s)+"\t");
//System.out.println("S->AT");
while(b<slength&&compare(b)){
System.out.println(msg);
st++;//步骤号
System.out.print(st+"\t");
stack.print();
System.out.print(printArray(s)+"\t");//剩余输入串
}
}
}
}

zouhao9010053325
- 粉丝: 0
- 资源: 3
最新资源
- 基于GRCNN网络的机械臂视觉平面抓取(python开发源码+说明文档+设计报告).zip
- 个人课设基于Springboot+vue+mybatisplus+mysql的Tom在线影院购票系统开发项目.zip
- 基于SpringBoot-Vue-Android的社区管理系统源码+设计报告(前后端分离架构).zip
- 工业仪表(指针表与数字表盘)智能监控与预警系统源码(比赛获奖项目).zip
- 基于深度学习的图像分类模型训练(人工智能课程大作业,含源码与报告).zip
- 人工智能毕设基于深度学习的电力领域应用实战项目(含源码与项目说明).zip
- 基于深度学习的计算机视觉一体化平台(数据标注、训练、部署,含源码).zip
- 微信机器人搜图与ChatGPT文本自动总结项目源码(适用于chatgpt-on-wechat).zip
- 机器学习大作业基于机器学习的恶意URL检测算法(含项目说明,含源码).zip
- 基于深度学习python开发的课堂专注度行为识别系统(含项目说明与源码).zip
- 基于机器学习的二手车交易价格预测算法及项目说明(含源码+说明文档).zip
- 人工智能课设基于卷积神经网络的果蔬图像识别与分类系统(源码及说明).zip
- 安徽省机器人大赛-单片机与嵌入式赛道竞赛C赛道作品(含全部参赛资料).zip
- 毕业设计声源定位跟踪系统-最新开发(含全新源码+设计报告+全部资料).zip
- 新课设基于Springboot和MySQL的鲜花在线销售商城系统源码+报告及全部资料.zip
- 东南大学物联网竞赛参赛作品-基于EPS32的儿童用可定位通信信箱设计方案.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


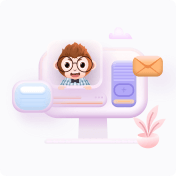