c++复数类设计
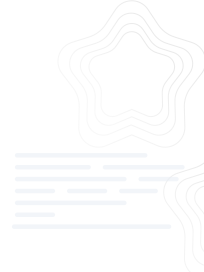


在C++编程语言中,设计一个复数类是学习面向对象编程的重要实践。复数类是一种自定义数据类型,用于表示数学中的复数,由实部和虚部组成。在这个简单的设计中,我们将探讨如何创建一个包含基本操作的C++复数类,如构造函数、赋值运算符、加减乘除等操作。 让我们从类的定义开始。在C++中,我们可以通过`class`关键字来定义一个类。复数类通常包含两个私有成员变量,一个是`real`表示实部,另一个是`imag`表示虚部: ```cpp class Complex { private: double real; double imag; }; ``` 私有成员变量不允许外部直接访问,为了提供对外部的接口,我们需要定义公共成员函数(也称为方法)。首先是构造函数,用于初始化复数的实部和虚部: ```cpp Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {} ``` 这里我们定义了一个带有默认参数的构造函数,允许用户创建任意复数或默认为零复数。 接下来是赋值运算符`=`,用于将一个复数对象的值赋给另一个复数对象: ```cpp Complex& operator=(const Complex& other) { if (this != &other) { // 防止自我赋值 real = other.real; imag = other.imag; } return *this; } ``` 为了支持复数的算术运算,我们需要重载基本的算术运算符。例如,我们可以定义加法、减法、乘法和除法: ```cpp // 加法 Complex operator+(const Complex& other) const { return Complex(real + other.real, imag + other.imag); } // 减法 Complex operator-(const Complex& other) const { return Complex(real - other.real, imag - other.imag); } // 乘法 Complex operator*(const Complex& other) const { return Complex(real * other.real - imag * other.imag, real * other.imag + imag * other.real); } // 除法 Complex operator/(const Complex& other) const { double denominator = other.real * other.real + other.imag * other.imag; return Complex((real * other.real + imag * other.imag) / denominator, (imag * other.real - real * other.imag) / denominator); } ``` 以上代码中,每个运算符都返回一个新的`Complex`对象,表示运算结果。注意,除法运算需要处理分母为零的情况,这在实际应用中可能需要额外的错误处理。 此外,为了提高代码的可读性,可以提供友元函数来实现输入输出流操作。声明`std::ostream`和`std::istream`为类的友元,然后定义相应的`<<`和`>>`运算符: ```cpp friend std::ostream& operator<<(std::ostream& os, const Complex& c); friend std::istream& operator>>(std::istream& is, Complex& c); // 输出复数 std::ostream& operator<<(std::ostream& os, const Complex& c) { os << c.real << " + " << c.imag << "i"; return os; } // 输入复数 std::istream& operator>>(std::istream& is, Complex& c) { double r, i; is >> r >> std::ws >> i; if (i == 'i') { c = Complex(r, 0); } else if (i == '+') { is >> std::ws >> c.imag; c = Complex(r, c.imag); } else { is.setstate(std::ios_base::failbit); } return is; } ``` 这样,我们就可以方便地使用`cout`和`cin`与复数对象进行交互了。 总结一下,设计一个C++复数类涉及以下几个关键点: 1. 定义私有成员变量:实部和虚部。 2. 创建构造函数:初始化复数。 3. 重载赋值运算符:实现赋值功能。 4. 重载算术运算符:支持加减乘除。 5. 使用友元函数:实现I/O操作。 这个简单的复数类设计对于C++初学者来说是一个很好的起点,它涵盖了面向对象编程的基础概念,包括类、构造函数、赋值运算符、运算符重载以及友元函数。通过实践这个项目,你可以更好地理解和掌握C++的面向对象特性。
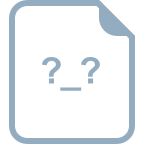
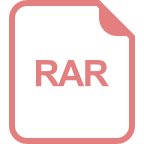
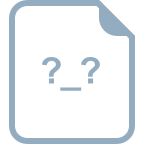
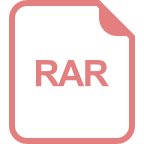
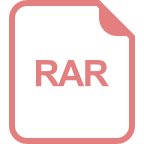
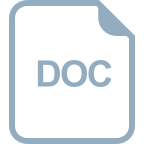
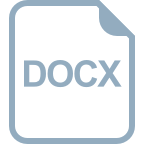
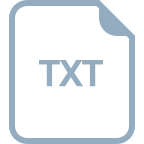
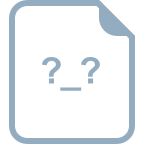
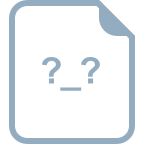
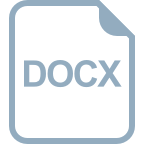
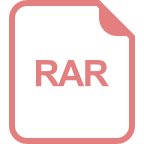
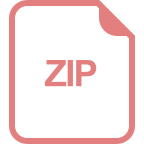
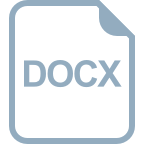
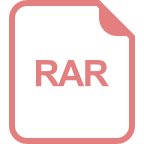



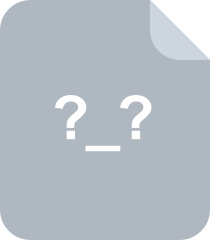

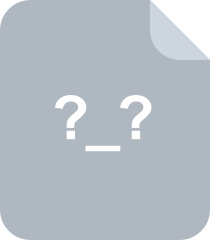
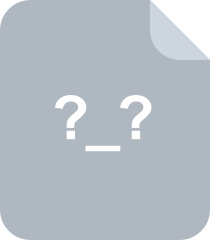


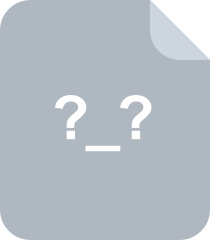

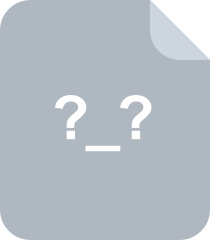
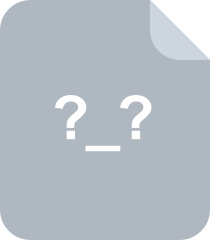
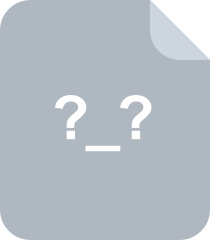
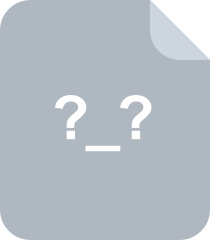

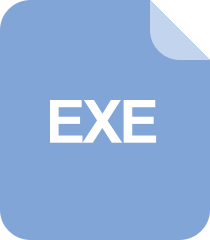
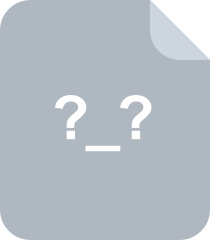
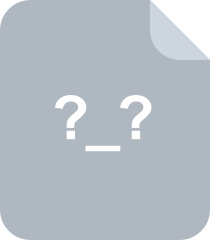
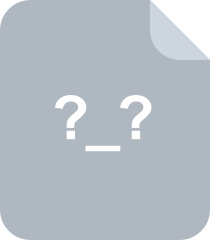
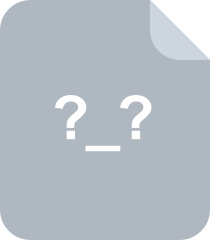
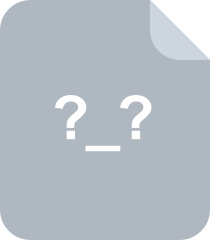
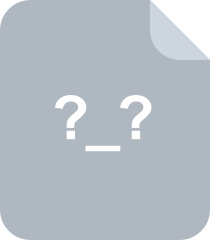
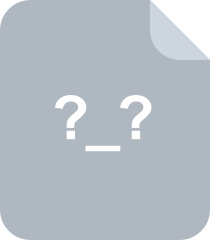
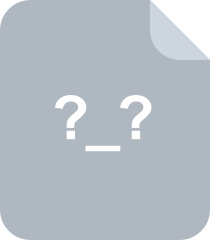
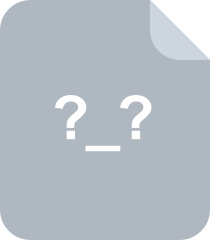
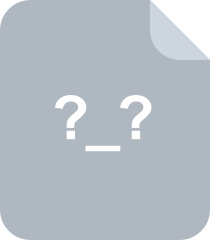
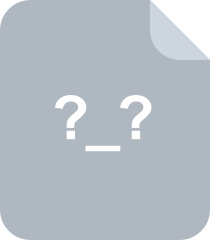
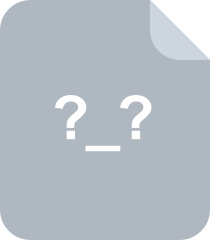
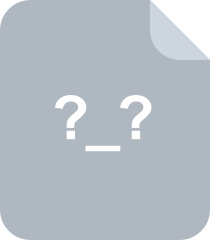
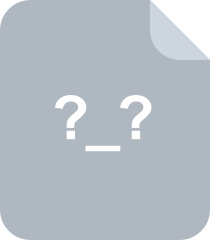
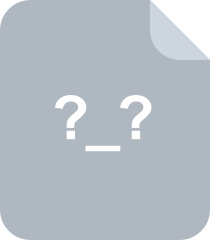
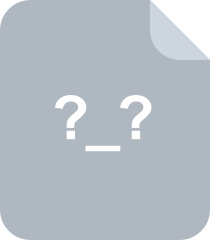
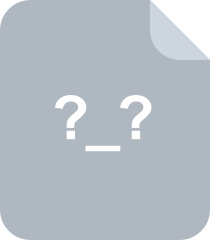
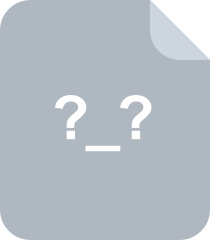
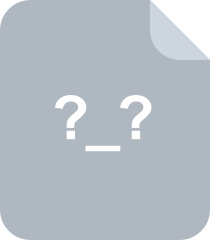
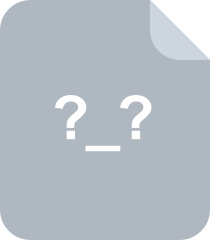
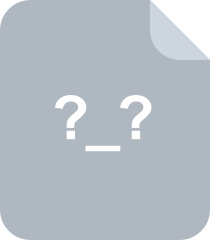
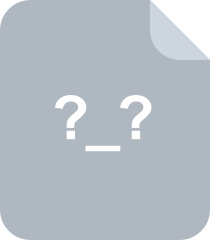
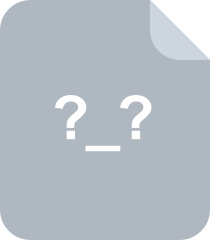
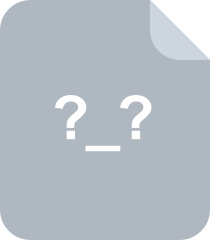
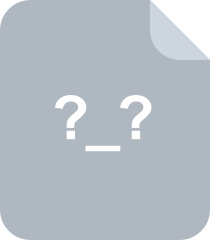
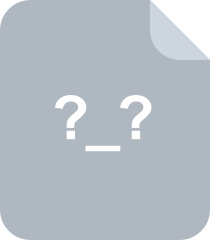
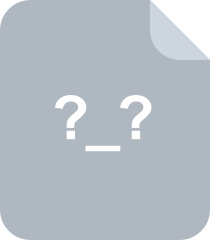
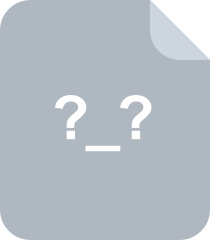
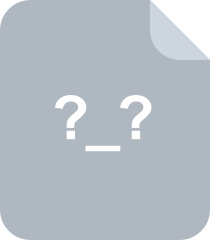
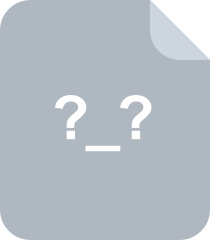
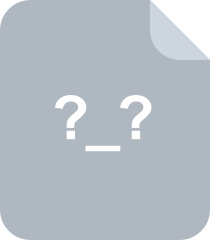
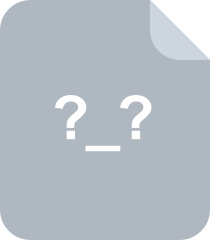
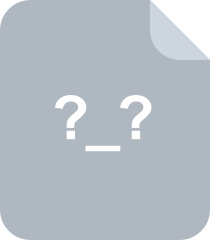
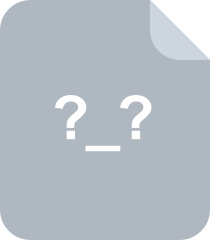
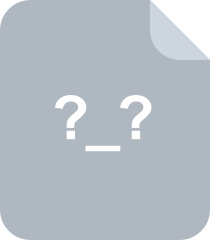
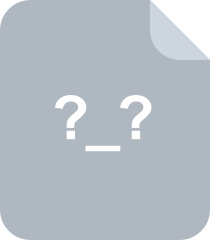
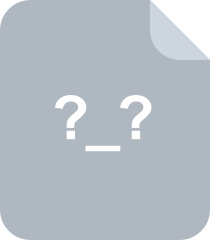
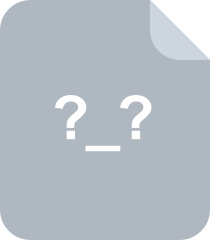
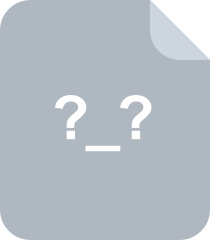
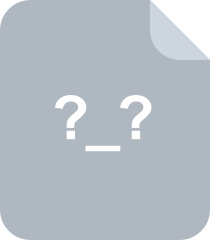
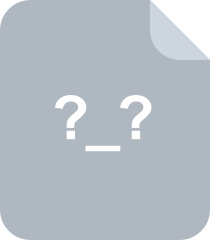
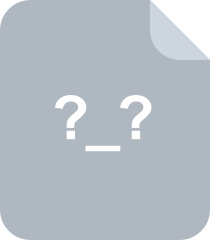
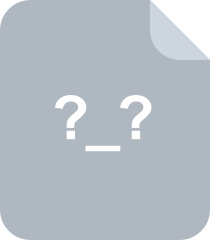
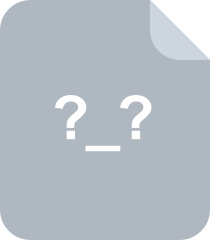
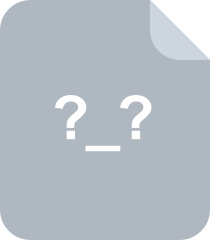
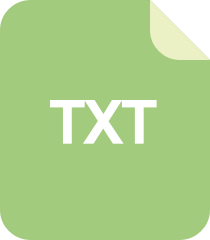
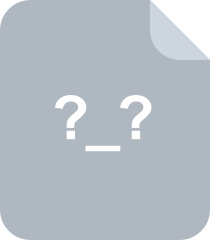
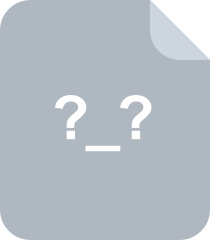
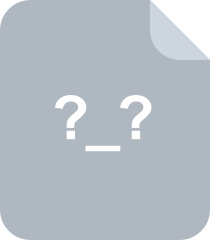
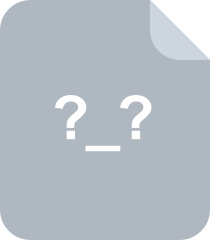
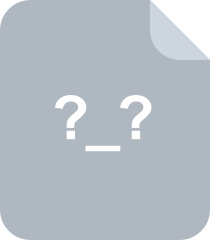
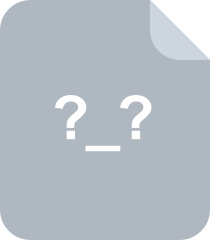
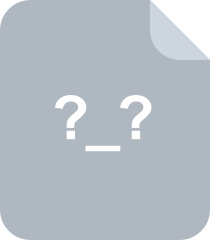
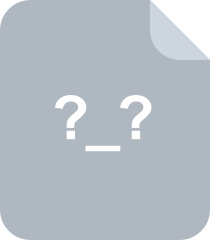
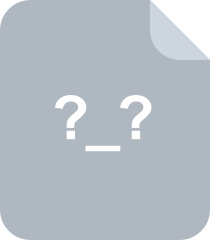
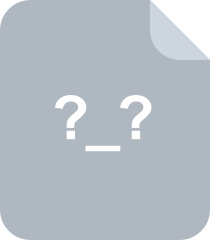
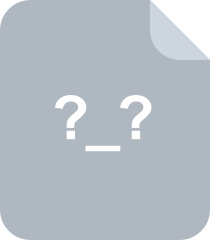
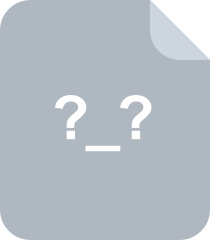
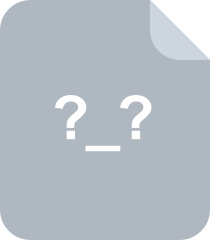
- 1
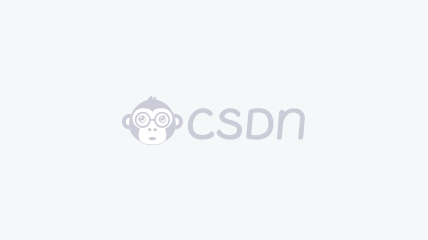
- feix1272014-10-13程序文件。

- 粉丝: 0
- 资源: 12
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

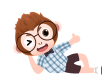
最新资源

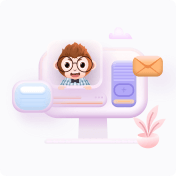
