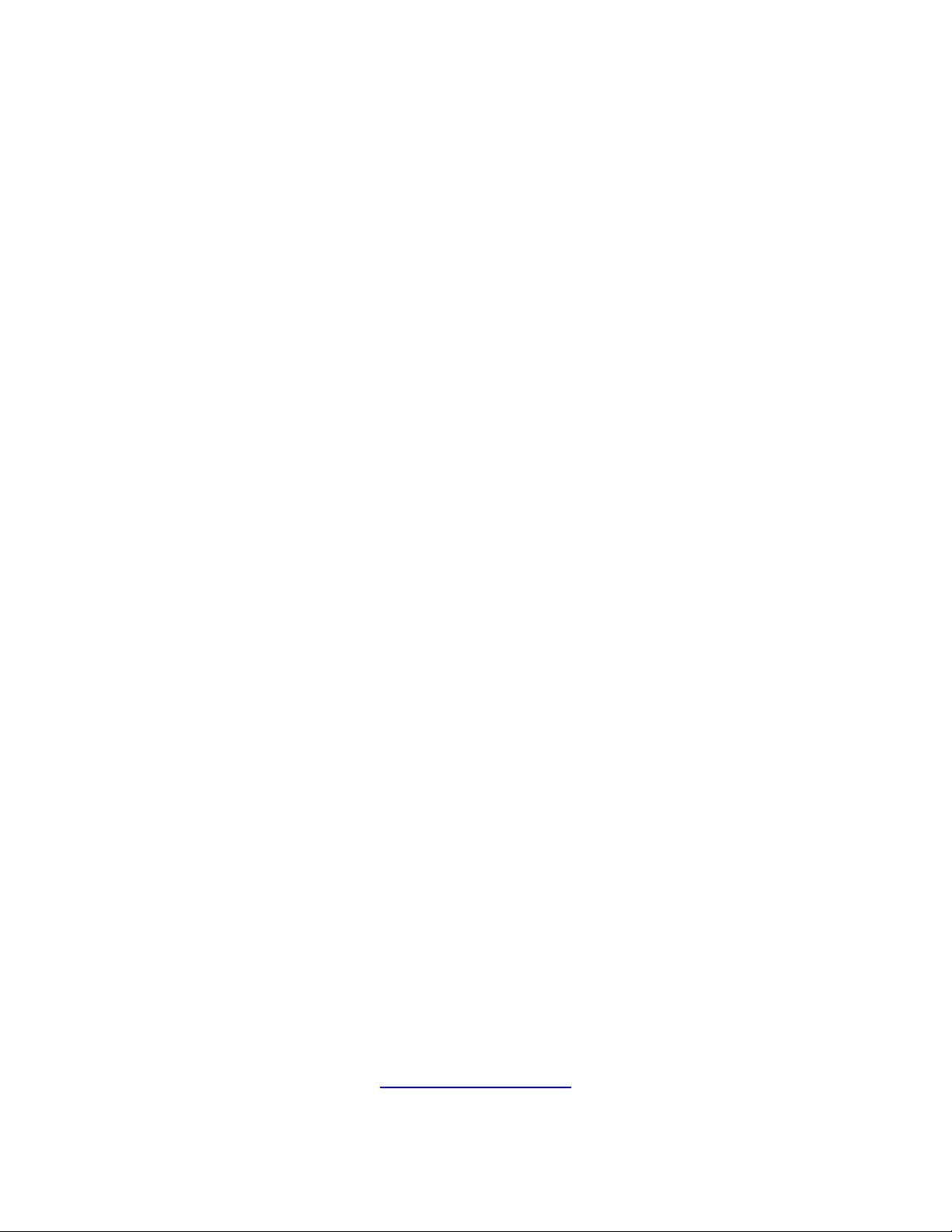
User’s Manual
V1.36.00
μC/
LIB
TM
Embedded Library
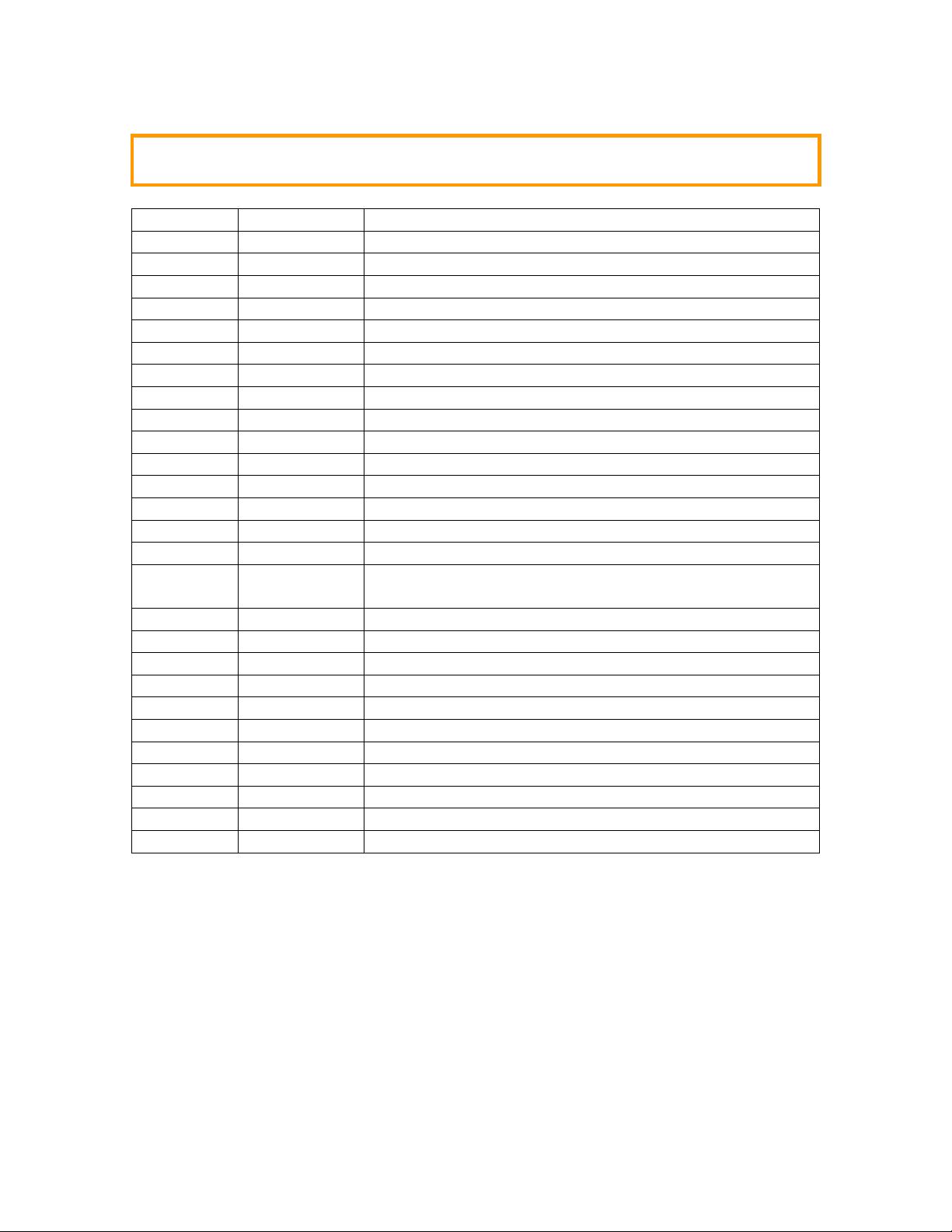
Micriμm
1290 Weston Road, Suite 306
Weston, FL 33326
USA
www.Micrium.com
Designations used by companies to distinguish their products are often claimed as
trademarks. In all instances where Micriμm Press is aware of a trademark claim, the product
name appears in initial capital letters, in all capital letters, or in accordance with the
vendor’s capatilization preference. Readers should contact the appropriate companies for
more complete information on trademarks and trademark registrations. All trademarks and
registerd trademarks in this book are the property of their respective holders.
Copyright © 2011 by Micriμm except where noted otherwise. All rights reserved. Printed in
the United States of America. No part of this publication may be reproduced or distributed
in any form or by any means, or stored in a database or retrieval system, without the prior
written permission of the publisher; with the exception that the program listings may be
entered, stored, and executed in a computer system, but they may not be reproduced for
publication.
The programs and code examples in this book are presented for instructional value. The
programs and examples have been carefully tested, but are not guaranteed to any particular
purpose. The publisher does not offer any warranties and does not guarantee the accuracy,
adequacy, or completeness of any information herein and is not responsible for any errors
and ommissions. The publisher assumes no liability for damages resulting from the use of
the information in this book or for any infringement of the intellectual property rights of
third parties that would result from the use of this information.
600-uC-LIB-004
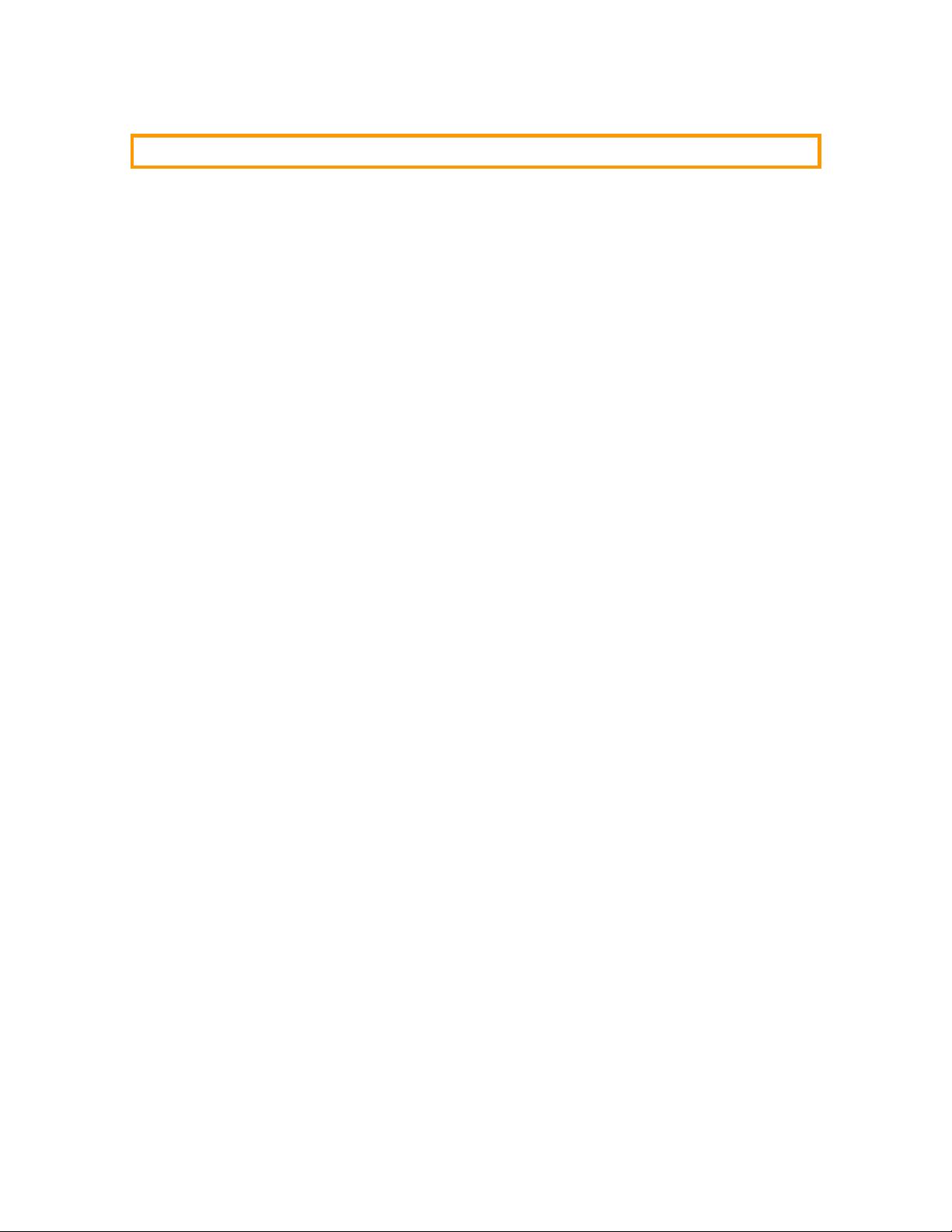
3
USER’S MANUAL VERSIONS
If you find any errors in this document, please inform us and we will make the appropriate
corrections for future releases.
Manual Version Date By Description
V1.18 2005/08/30 ITJ Manual Created
V1.19 2006/04/25 JJL Updated Manual
V1.20 2006/06/01 ITJ Updated Manual
V1.21 2006/08/09 ITJ Added memory macros
V1.22 2006/09/20 ITJ Updated Manual
V1.23 2007/02/27 SR Updated Manual
V1.24 2007/05/04 ITJ Updated Manual
V1.25 2008/06/20 ITJ Added memory management
Added ASCII module
V1.26 2008/12/01 ITJ Added heap memory
Added string parse functions
V1.27 2009/01/05 ITJ Updated Manual
V1.28 2009/02/26 EHS Updated Manual
V1.29 2009/04/28 ITJ Updated Manual
V1.30 2009/05/05 BAN Added math module with pseudo-random number generator
V1.31 2009/09/28 ITJ Updated Manual
V1.32 2010/04/02 ITJ Updated Manual
V1.33 2010/07/28 ITJ Updated Manual
V1.33 2010/09/03 ITJ Converted document from Word to FrameMaker
V1.34 2010/12/17 ITJ Updated Manual
V1.35.00 2011/06/13 ITJ Added endian memory conversion macros
V1.36.00 2011/08/15 ITJ Added memory remaining functions
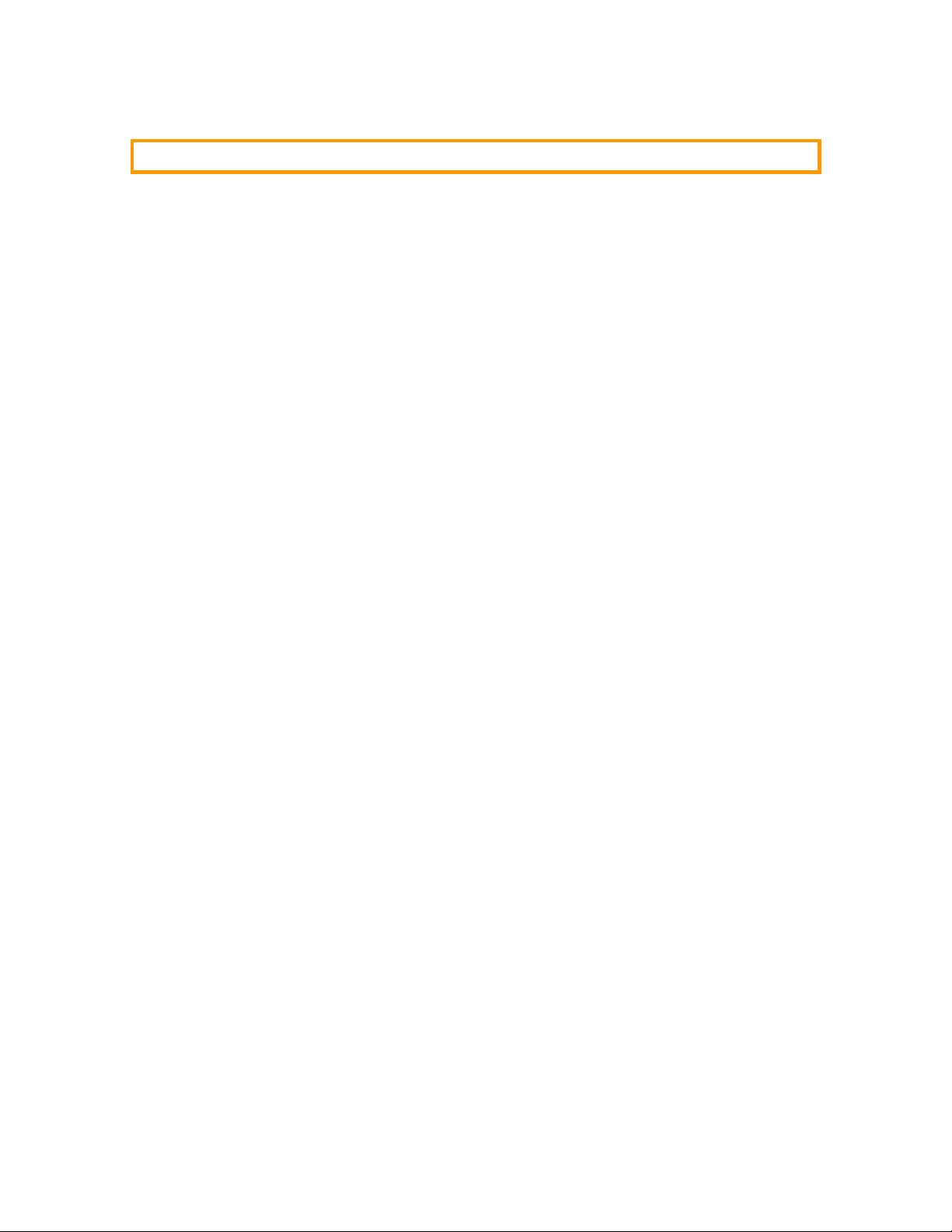
4
Table of Contents
0-1 User’s Manual Versions ......................................................................... 3
Chapter 1 Introduction ............................................................................................ 8
1-1 Portable .................................................................................................. 8
1-2 Scalable .................................................................................................. 8
1-3 Coding Standards .................................................................................. 8
1-4 MISRA C ................................................................................................. 9
1-5 Safety Critical Certification .................................................................... 9
1-6 μC/LIB Limitations ................................................................................. 9
Chapter 2 Directories and Files ............................................................................ 10
Chapter 3 μC/LIB Constant and Macro Library ................................................... 12
3-1 Library Constants ................................................................................. 12
3-1-1 Boolean Constants .............................................................................. 12
3-1-2 Bit Constants ........................................................................................ 12
3-1-3 Octet Constants ................................................................................... 12
3-1-4 Number Base Constants ...................................................................... 13
3-1-5 Integer Constants ................................................................................. 13
3-1-6 Time Constants .................................................................................... 13
3-2 Common Library Macros ..................................................................... 14
3-2-1 DEF_BITxx() .......................................................................................... 14
3-2-2 DEF_BIT_MASK_xx() ............................................................................ 15
3-2-3 DEF_BIT_FIELD_xx() ............................................................................ 16
3-2-4 DEF_BIT_SET() ..................................................................................... 18
3-2-5 DEF_BIT_CLR() ..................................................................................... 19
3-2-6 DEF_BIT_IS_SET() ................................................................................ 20
3-2-7 DEF_BIT_IS_CLR() ................................................................................ 21
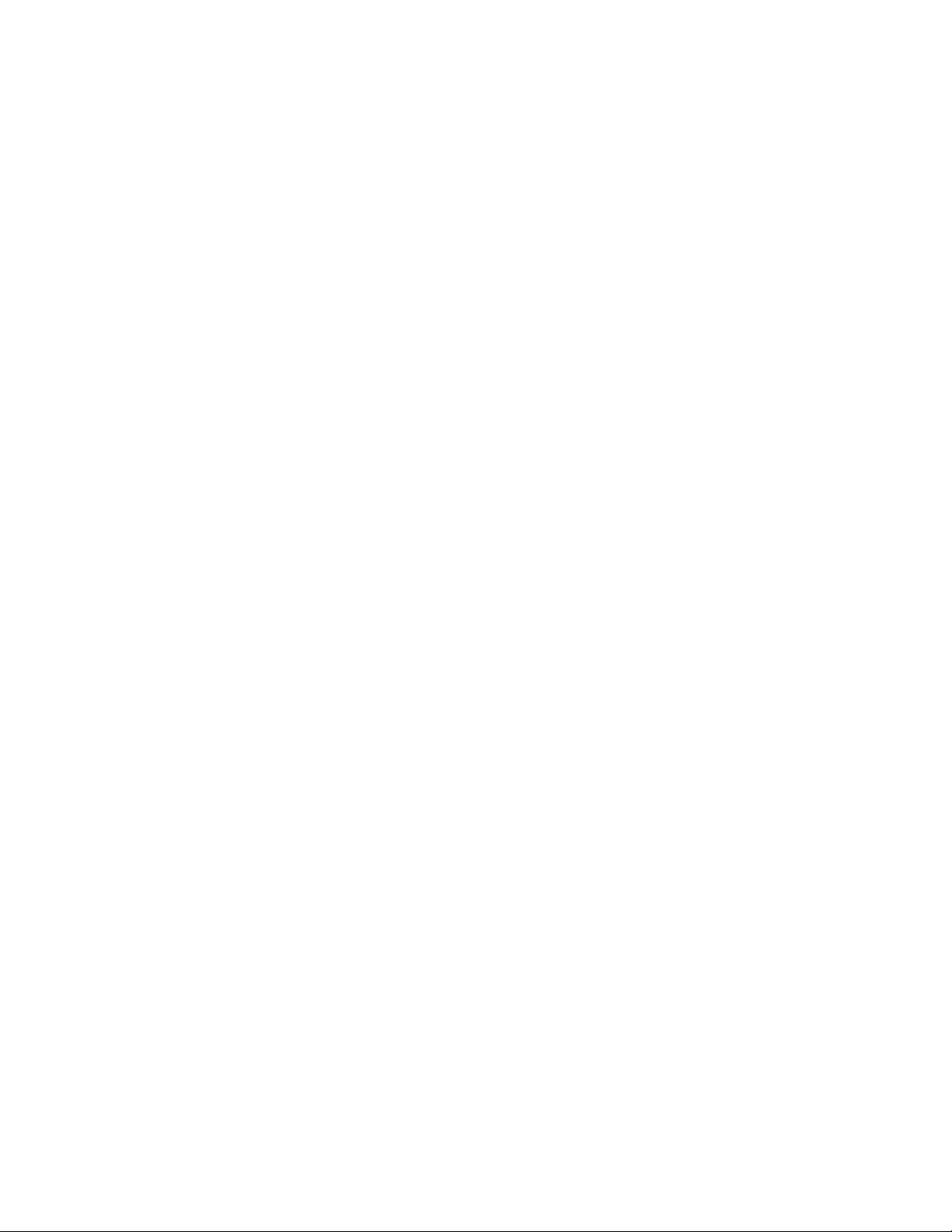
5
3-2-8 DEF_BIT_IS_SET_ANY() ....................................................................... 22
3-2-9 DEF_BIT_IS_CLR_ANY() ...................................................................... 23
3-2-10 DEF_CHK_VAL_MIN() ........................................................................... 25
3-2-11 DEF_CHK_VAL_MAX() .......................................................................... 26
3-2-12 DEF_CHK_VAL() ................................................................................... 28
3-2-13 DEF_GET_U_MAX_VAL() ...................................................................... 30
3-2-14 DEF_MIN() ............................................................................................. 31
3-2-15 DEF_MAX() ........................................................................................... 32
3-2-16 DEF_ABS() ............................................................................................ 33
Chapter 4 μC/LIB Memory Library ........................................................................ 35
4-1 Memory Library Configuration ............................................................. 35
4-2 Memory Library Macros ....................................................................... 36
4-2-1 MEM_VAL_BIG_TO_LITTLE_xx() / MEM_VAL_LITTLE_TO_BIG_xx() . 36
4-2-2 MEM_VAL_BIG_TO_HOST_xx() / MEM_VAL_HOST_TO_BIG_xx() ..... 37
4-2-3
MEM_VAL_LITTLE_TO_HOST_xx() / MEM_VAL_HOST_TO_LITTLE_xx()
39
4-2-4 MEM_VAL_GET_xxx() ........................................................................... 40
4-2-5 MEM_VAL_SET_xxx() ........................................................................... 42
4-2-6 MEM_VAL_COPY_GET_xxx() ............................................................... 44
4-2-7 MEM_VAL_COPY_SET_xxx() ............................................................... 46
4-2-8 MEM_VAL_COPY_xxx() ........................................................................ 49
4-3 Memory Library Functions ................................................................... 51
4-3-1 Mem_Clr() ............................................................................................. 51
4-3-2 Mem_Set() ............................................................................................ 52
4-3-3 Mem_Copy() ......................................................................................... 53
4-3-4 Mem_Cmp() .......................................................................................... 54
4-4 Memory Allocation Functions .............................................................. 56
4-4-1 Mem_Init() ............................................................................................. 57
4-4-2 Mem_HeapAlloc() ................................................................................. 58
4-4-3 Mem_HeapGetSizeRem() ..................................................................... 59
4-4-4 Mem_PoolClr() ...................................................................................... 61
4-4-5 Mem_PoolCreate() ............................................................................... 62
4-4-6 Mem_PoolGetSizeRem() ...................................................................... 65
4-4-7 Mem_PoolBlkGet() ............................................................................... 67
4-4-8 Mem_PoolBlkFree() .............................................................................. 69
4-5 Memory Library Optimization .............................................................. 71