二叉树的c#实现 包括添加删除
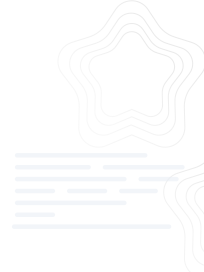

在IT领域,二叉树是一种基础且重要的数据结构,它在很多算法和程序设计中扮演着核心角色。本文将深入探讨如何使用C#语言来实现二叉树,并着重讲解二叉树的添加和删除操作。此外,我们还将讨论在实际应用中如何结合登录界面和前序、中序遍历来动态实现二叉树的功能。 二叉树由节点构成,每个节点包含一个值以及指向其左子节点和右子节点的引用。在C#中,我们可以定义一个`Node`类来表示二叉树的节点: ```csharp public class Node { public int Value; public Node LeftChild; public Node RightChild; public Node(int value) { this.Value = value; this.LeftChild = null; this.RightChild = null; } } ``` 接着,我们需要创建一个`BinaryTree`类来管理整个二叉树。在这个类中,我们可以定义添加节点的方法: ```csharp public class BinaryTree { private Node root; public void AddNode(int value) { if (root == null) { root = new Node(value); } else { AddNodeHelper(root, value); } } private void AddNodeHelper(Node currentNode, int value) { if (value < currentNode.Value) { if (currentNode.LeftChild == null) { currentNode.LeftChild = new Node(value); } else { AddNodeHelper(currentNode.LeftChild, value); } } else { if (currentNode.RightChild == null) { currentNode.RightChild = new Node(value); } else { AddNodeHelper(currentNode.RightChild, value); } } } } ``` 删除节点通常更复杂,因为它可能涉及替换、平衡树等操作。以下是一个简单的删除节点的实现: ```csharp public void RemoveNode(int value) { root = RemoveNodeHelper(root, value); } private Node RemoveNodeHelper(Node currentNode, int value) { if (currentNode == null) return null; if (value < currentNode.Value) { currentNode.LeftChild = RemoveNodeHelper(currentNode.LeftChild, value); } else if (value > currentNode.Value) { currentNode.RightChild = RemoveNodeHelper(currentNode.RightChild, value); } else { if (currentNode.LeftChild == null && currentNode.RightChild == null) { return null; // 节点是叶子节点,直接删除 } else if (currentNode.LeftChild == null) { return currentNode.RightChild; // 节点只有一个右孩子,用右孩子替换 } else if (currentNode.RightChild == null) { return currentNode.LeftChild; // 节点只有一个左孩子,用左孩子替换 } else { // 节点有两个孩子,找到右子树中的最小节点作为替代 var minNode = FindMin(currentNode.RightChild); currentNode.Value = minNode.Value; currentNode.RightChild = RemoveNodeHelper(currentNode.RightChild, minNode.Value); } } return currentNode; } private Node FindMin(Node node) { while (node.LeftChild != null) { node = node.LeftChild; } return node; } ``` 在实际应用中,如登录界面的实现,我们可以创建一个用户类,将用户信息(如用户名和密码)存储在一个二叉搜索树中,便于快速查找和管理用户。登录时,根据用户输入的用户名,通过二叉树的查找功能判断用户是否存在。同时,可以结合前序和中序遍历来展示二叉树的结构,帮助用户理解系统中存储的数据。 前序遍历(根-左-右)的C#实现: ```csharp public void PreOrderTraversal(Node node) { if (node != null) { Console.Write(node.Value + " "); PreOrderTraversal(node.LeftChild); PreOrderTraversal(node.RightChild); } } ``` 中序遍历(左-根-右)的C#实现: ```csharp public void InOrderTraversal(Node node) { if (node != null) { InOrderTraversal(node.LeftChild); Console.Write(node.Value + " "); InOrderTraversal(node.RightChild); } } ``` 通过结合以上代码,我们可以构建一个完整的二叉树系统,包括添加、删除用户,以及通过登录界面进行交互。这个系统不仅可以应用于用户管理,还可以扩展到其他需要高效查找和操作数据的场景,例如数据库索引、文件系统等。在实际开发中,还需要考虑错误处理、性能优化等因素,以提供稳定可靠的用户体验。
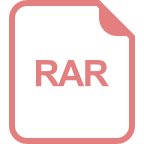
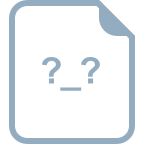
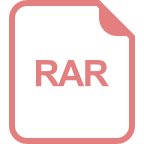
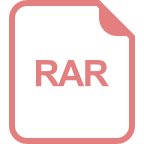
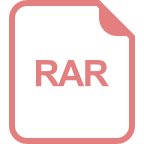
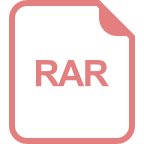
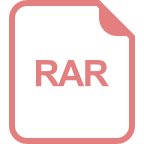
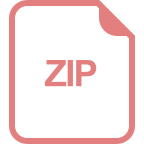
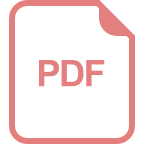
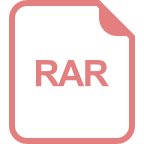
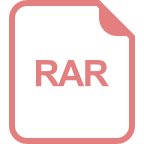
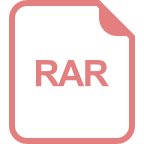
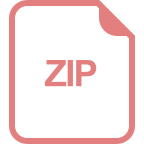
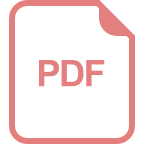


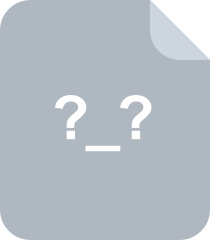



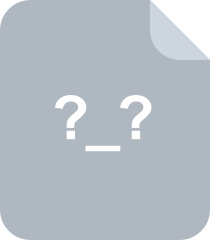
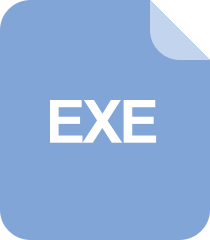
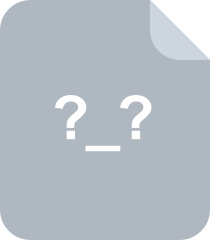
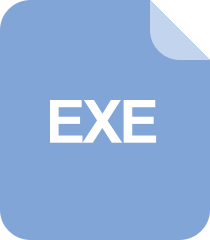
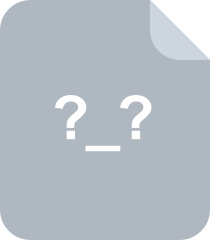
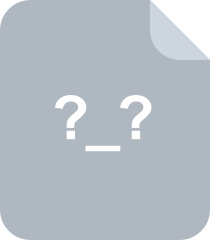
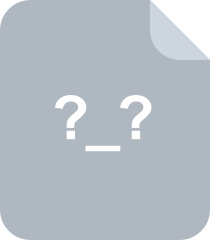
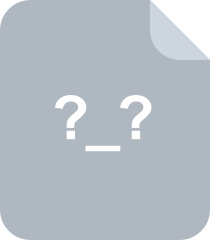
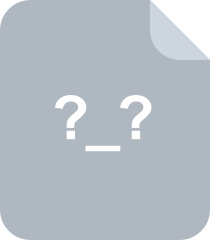
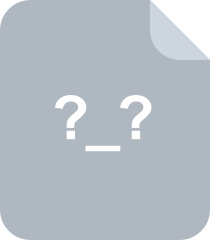
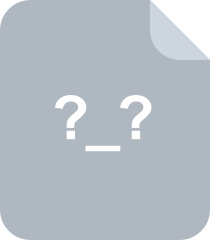
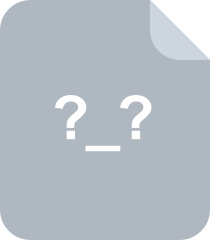


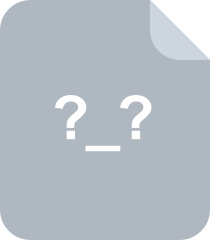
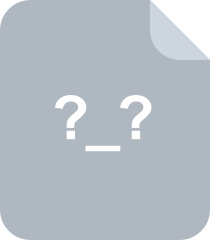
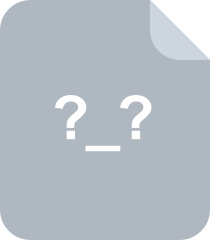
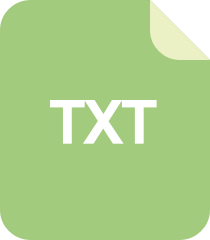
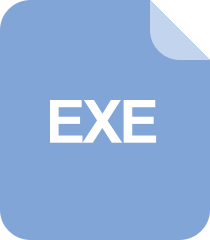
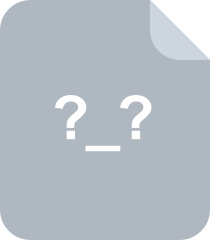
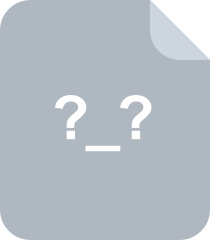
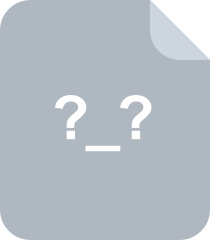
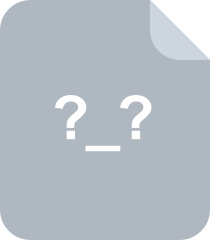
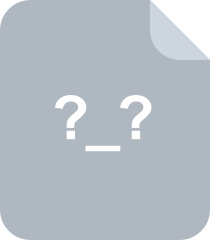
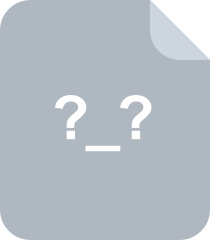
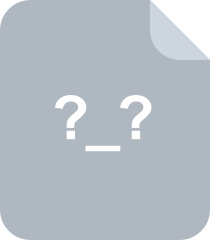

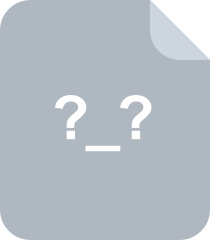
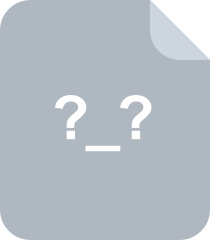
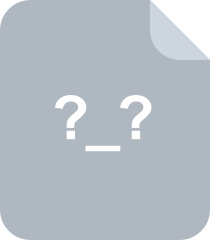
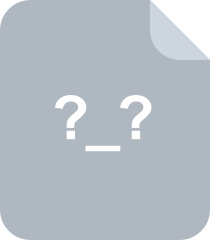
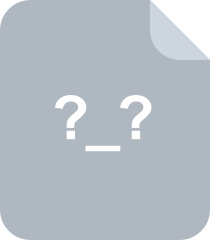
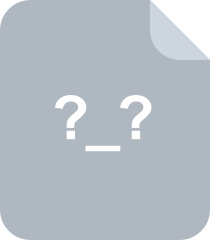
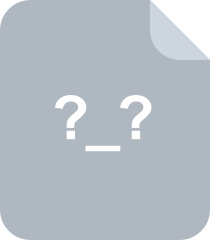
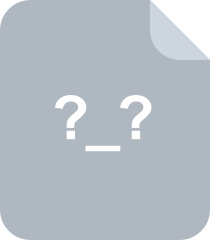

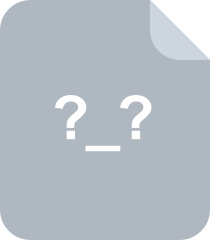
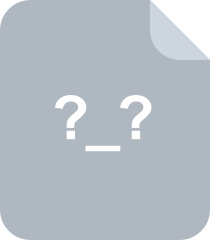
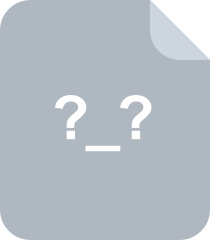
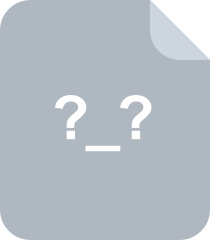
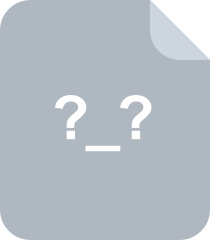
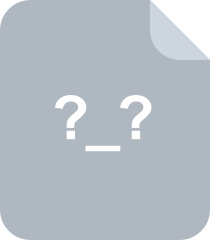
- 1
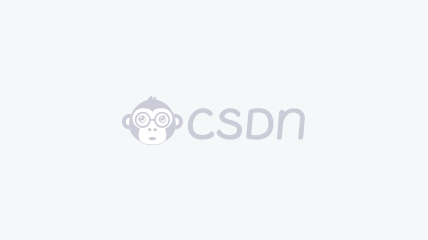

- 粉丝: 0
- 资源: 6
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

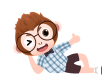
最新资源

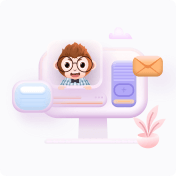
