Python 编程中,理解和掌握基础语法至关重要,其中包括运算符和表达式。运算符是用来执行特定操作的符号,而表达式则是由运算符和操作数组成的代码片段,用于计算和赋值。 3、运算符与表达式 Python 支持多种类型的运算符,包括算术运算符、比较运算符、逻辑运算符、赋值运算符和成员运算符。 3.1 算术运算符 算术运算符包括加法(+)、减法(-)、乘法(*)、除法(/)、整除(//)、取余(%)和幂运算(**)。例如: ```python a = 5 b = 2 print(a + b) # 输出 7 print(a - b) # 输出 3 print(a * b) # 输出 10 print(a / b) # 输出 2.5 print(a // b) # 输出 2 print(a % b) # 输出 1 print(a ** b) # 输出 25 ``` 3.2 比较运算符 比较运算符用于比较两个值,如等于(==)、不等于(!=)、小于(<)、大于(>)、小于等于(<=)和大于等于(>=)。例如: ```python print(3 == 3) # 输出 True print(3 != 2) # 输出 True print(5 < 10) # 输出 True print(10 > 5) # 输出 True print(7 <= 7) # 输出 True print(2 >= 2) # 输出 True ``` 3.3 逻辑运算符 逻辑运算符包括与(and)、或(or)和非(not)。它们用于组合或否定比较表达式。例如: ```python x = 5 y = 0 print(x > 0 and y > 0) # 输出 False print(x > 0 or y > 0) # 输出 True print(not (x > 0)) # 输出 False ``` 3.4 赋值运算符 Python 的赋值运算符有基本赋值(=)、增加赋值(+=、-=、*=、/=、%=、**=)和复合赋值(&=、|=、^=、>>=、<<=)。例如: ```python c = 10 d = 5 c += d # 等价于 c = c + d; 输出 15 c -= d # 等价于 c = c - d; 输出 10 c *= d # 等价于 c = c * d; 输出 50 c /= d # 等价于 c = c / d; 输出 10.0 c %= d # 等价于 c = c % d; 输出 0 c **= d # 等价于 c = c ** d; 输出 1 ``` 3.5 成员运算符 成员运算符检查一个值是否存在于序列(列表、元组、字符串)中,如 in 和 not in。例如: ```python fruits = ['apple', 'banana', 'orange'] print('apple' in fruits) # 输出 True print('grape' not in fruits) # 输出 True ``` 4、变量与类型 在 Python 中,变量无需预先声明类型,而是根据赋值自动确定。常见的类型有整型(int)、浮点型(float)、字符串(str)、布尔型(bool)和列表(list)、元组(tuple)、字典(dict)等。例如: ```python age = 25 # 整型 height = 1.78 # 浮点型 name = "Alice" # 字符串 is_student = True # 布尔型 fruits_list = ['apple', 'banana'] # 列表 tup = ('red', 'blue') # 元组 dict_info = {'name': 'Alice', 'age': 25} # 字典 ``` 5、控制流与函数 Python 中的控制流语句包括条件语句(if-else)、循环语句(for、while)和函数定义。例如: ```python for i in range(5): print(i) def greet(name): print(f"Hello, {name}!") greet("Alice") ``` 6、异常处理 Python 使用 try-except 语句处理异常。例如: ```python try: result = 10 / 0 except ZeroDivisionError: print("Cannot divide by zero!") ``` 通过以上内容,我们可以了解到 Python 作为一门易学且功能强大的编程语言,不仅语法简洁,还拥有丰富的库支持。无论是初学者还是专业开发者,都能在 Python 的世界里找到适合自己的应用场景。随着技术的发展,Python 在软件开发、数据科学和人工智能领域的地位只会越来越稳固。
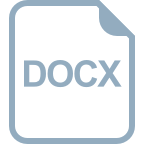
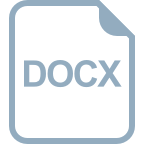
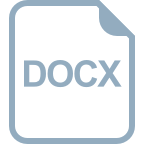
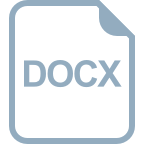
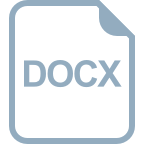
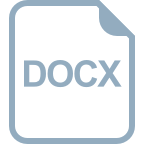
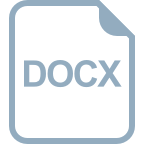
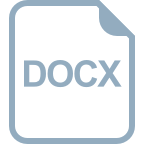
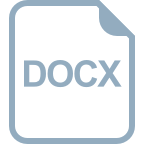
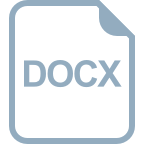
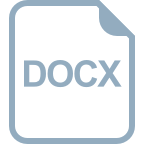
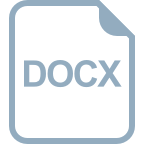
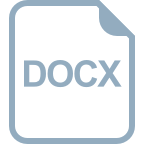
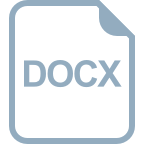
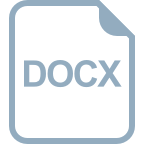
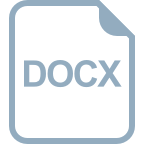
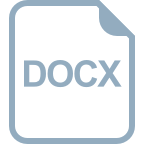
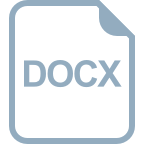
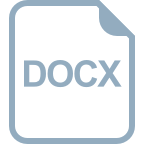
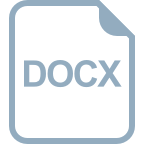
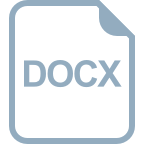
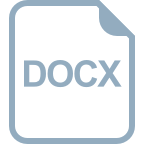
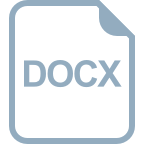
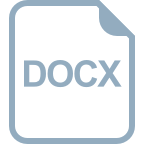
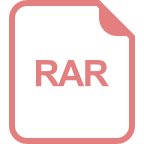
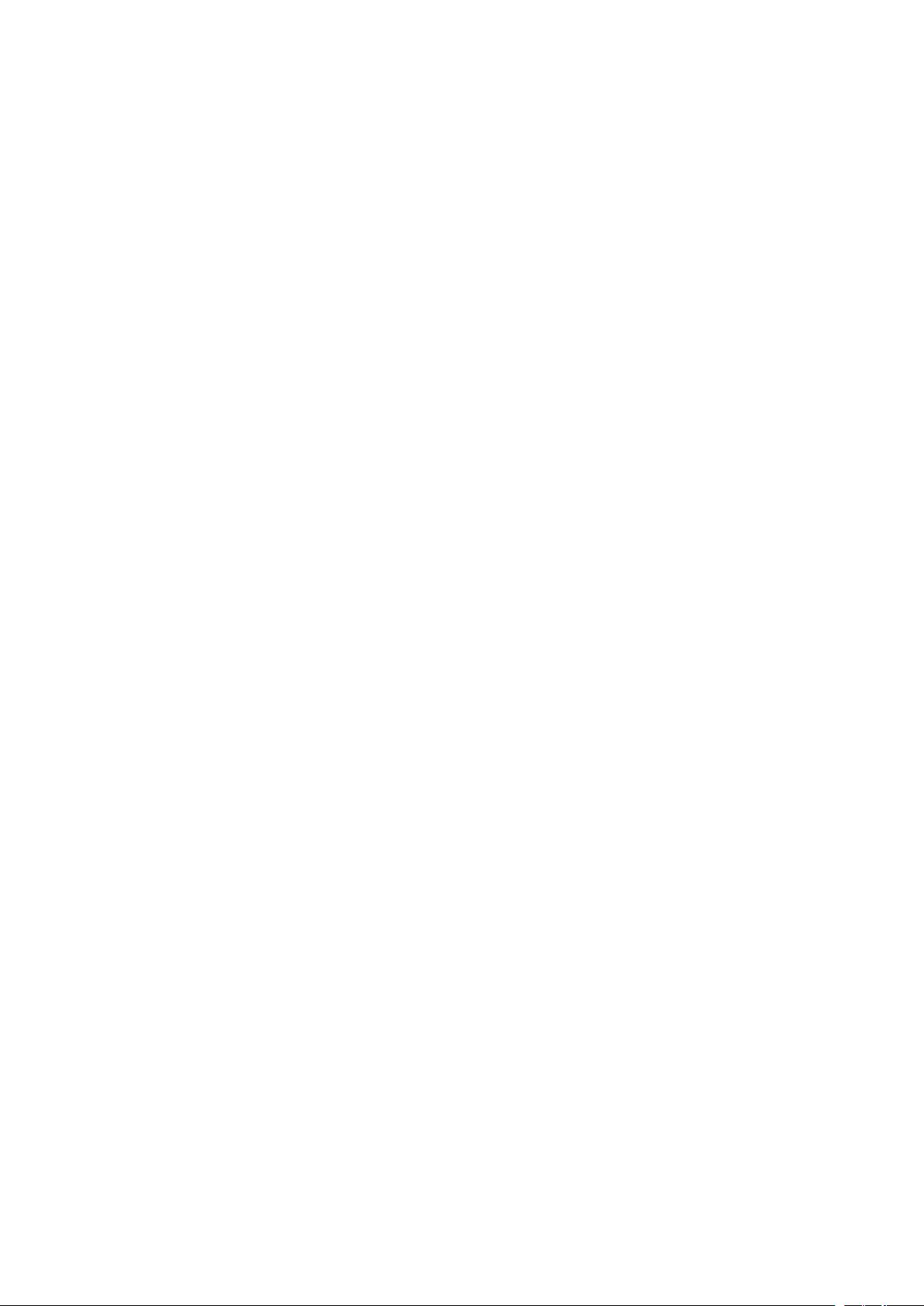
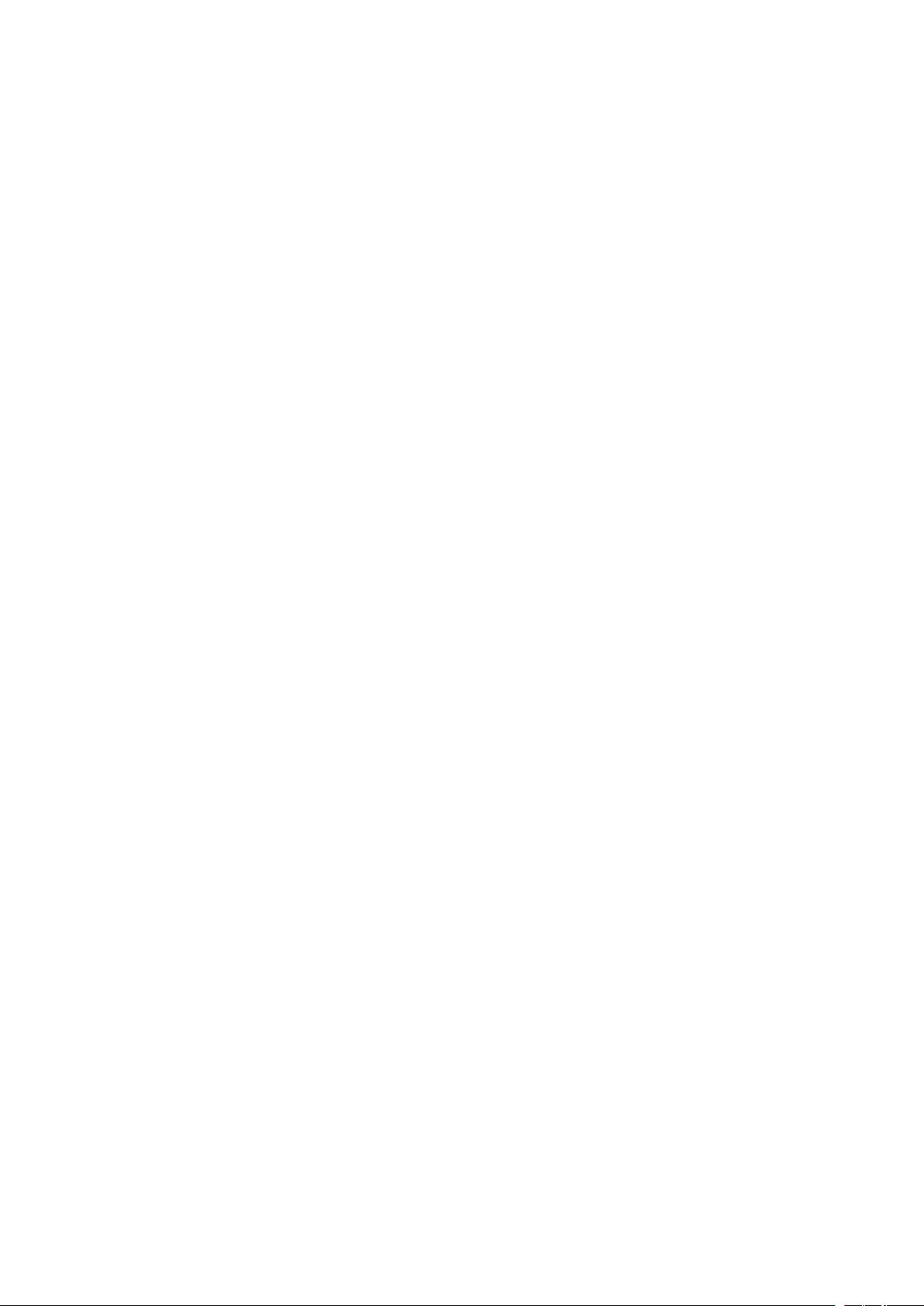
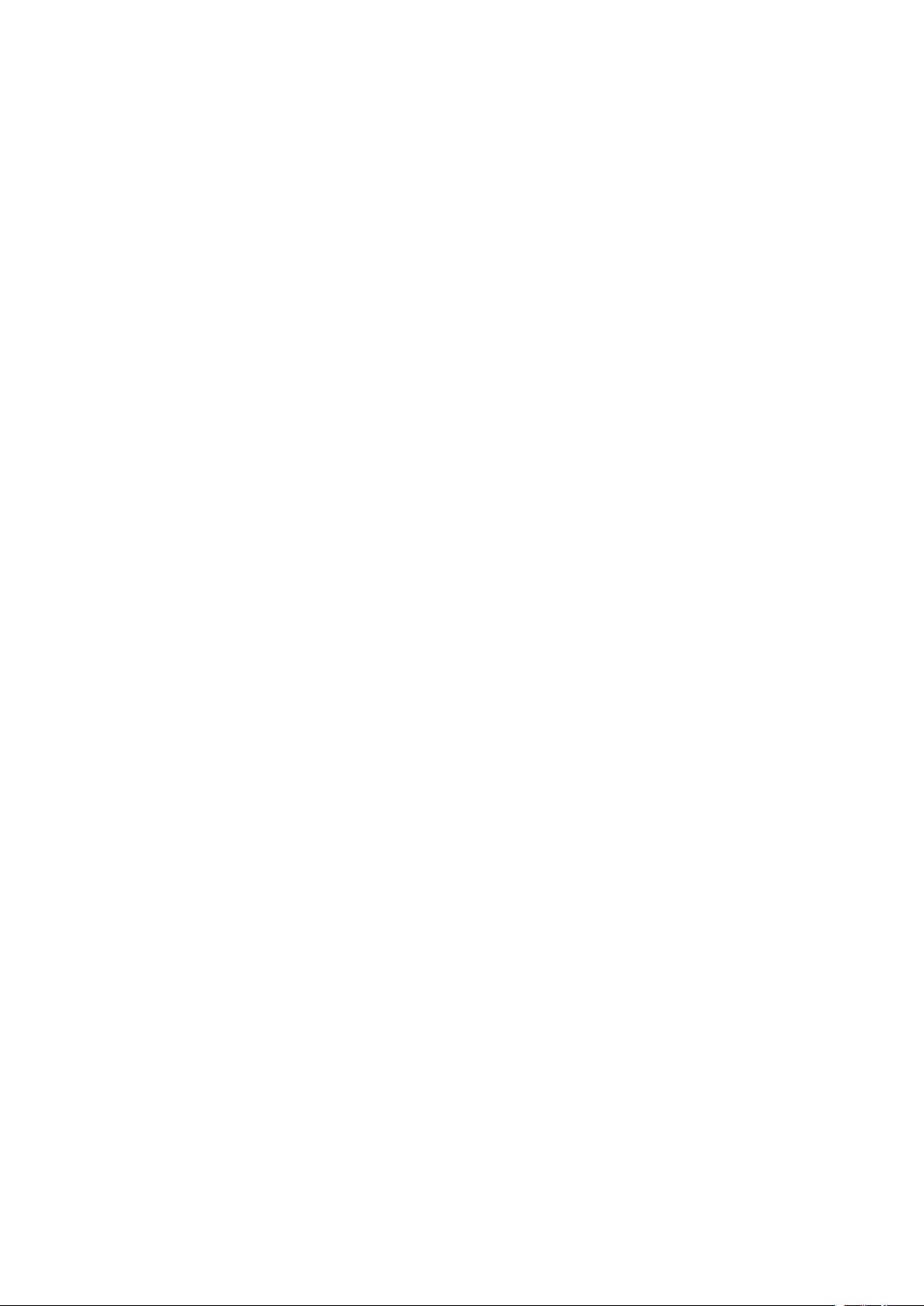
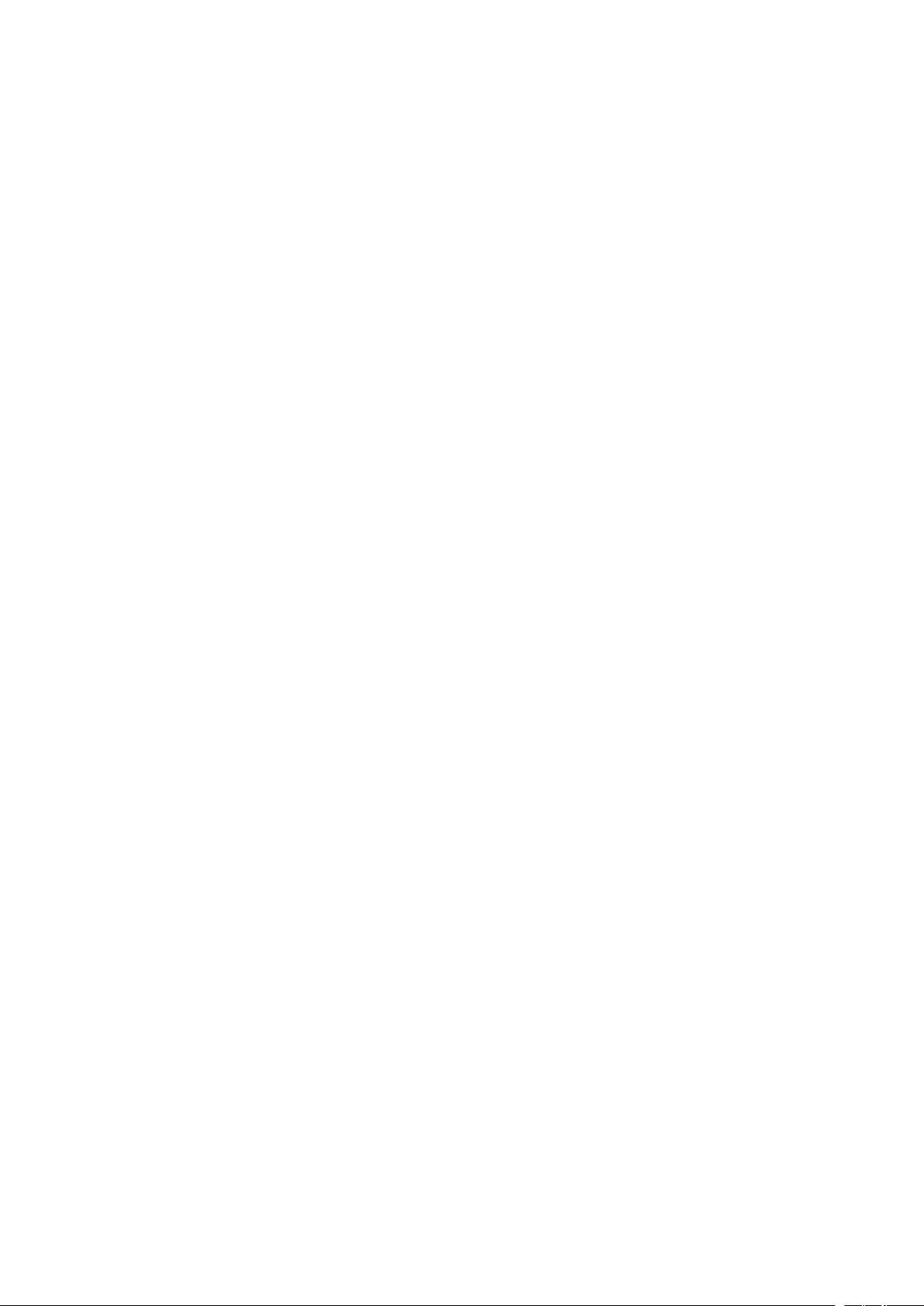
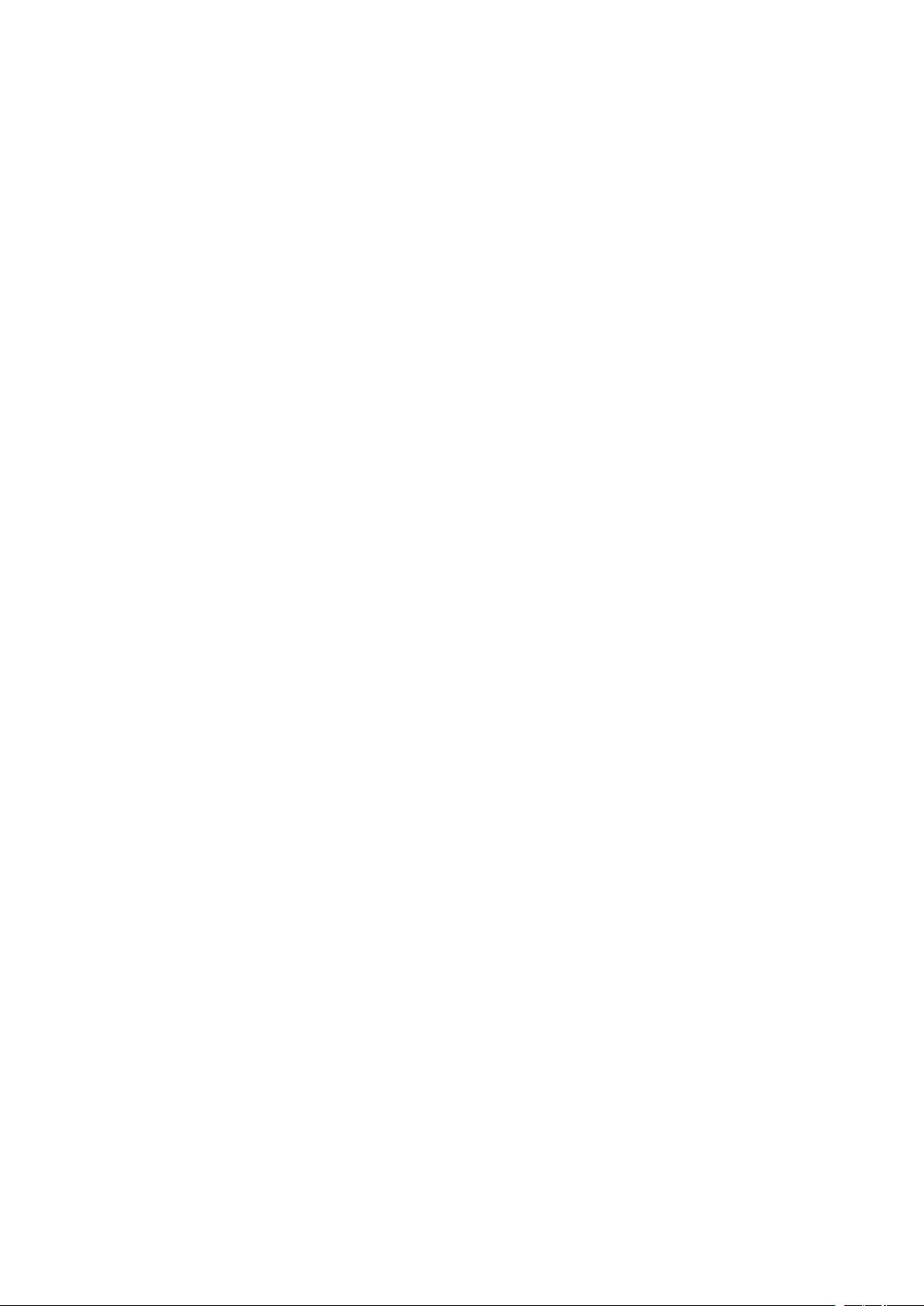
剩余65页未读,继续阅读
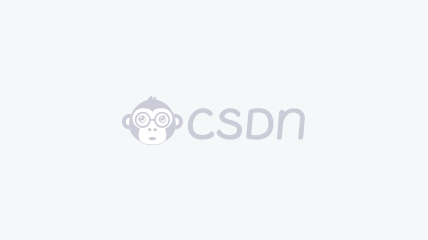

- 粉丝: 30
- 资源: 6877
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

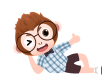
