<?php
/* $Id: setup.php 213 2009-06-19 12:28:18Z divagater $ */
/*
+-------------------------------------------------------------------------+
| Nagios Plugin for Cacti |
| |
| Copyright (C) 2007 Billy Gunn (billy@gunn.org) |
| |
| This program is free software; you can redistribute it and/or |
| modify it under the terms of the GNU General Public License |
| as published by the Free Software Foundation; either version 2 |
| of the License, or (at your option) any later version. |
| |
| This program is distributed in the hope that it will be useful, |
| but WITHOUT ANY WARRANTY; without even the implied warranty of |
| MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
| GNU General Public License for more details. |
+-------------------------------------------------------------------------+
| Cacti and Nagios are the copyright of their respective owners. |
+-------------------------------------------------------------------------+
*/
/**
* Called after install
*
* if return true, plugin will be installed but disabled
* if return false, plugin will be waiting configuration
*
* @return bool
*/
function plugin_npc_check_config() {
return true;
}
/**
* compatibility for plugin update
*/
function npc_version() {
return plugin_npc_version();
}
/**
* Version information
*/
function plugin_npc_version() {
return array( 'name' => 'npc',
'version' => '2.0.4',
'longname' => 'Nagios plugin for Cacti',
'author' => 'Billy Gunn',
'homepage' => 'http://trac2.assembla.com/npc',
'email' => 'billy@gunn.org',
'url' => 'http://trac2.assembla.com/npc'
);
}
function plugin_npc_install() {
npc_setup_tables();
api_plugin_register_realm ('npc', 'npc.php', 'NPC', 1);
api_plugin_register_realm ('npc', 'npc1.php', 'NPC Global Commands', 1);
// setup all arrays needed for npc
api_plugin_register_hook ('npc', 'config_arrays', 'npc_config_arrays', 'setup.php');
// Add the npc tab
api_plugin_register_hook ('npc', 'top_header_tabs', 'npc_show_tab', 'setup.php');
api_plugin_register_hook ('npc', 'top_graph_header_tabs', 'npc_show_tab', 'setup.php');
// Provide navigation texts
api_plugin_register_hook ('npc', 'draw_navigation_text', 'npc_draw_navigation_text', 'setup.php');
// Add Nagios host mapping select box
api_plugin_register_hook ('npc', 'config_form', 'npc_config_form', 'setup.php');
// Saves the selection from the host mapping select box
api_plugin_register_hook ('npc', 'api_device_save', 'npc_api_device_save', 'setup.php');
// Add a npc tab to the settings page
api_plugin_register_hook ('npc', 'config_settings', 'npc_config_settings', 'setup.php');
}
/**
* Remove all NPC database changes
*/
function plugin_npc_uninstall () {
// Drop all npc tables
db_execute("DROP TABLE `npc_acknowledgements`");
db_execute("DROP TABLE `npc_commands`");
db_execute("DROP TABLE `npc_commenthistory`");
db_execute("DROP TABLE `npc_comments`");
db_execute("DROP TABLE `npc_configfiles`");
db_execute("DROP TABLE `npc_configfilevariables`");
db_execute("DROP TABLE `npc_conninfo`");
db_execute("DROP TABLE `npc_contact_addresses`");
db_execute("DROP TABLE `npc_contact_notificationcommands`");
db_execute("DROP TABLE `npc_contactgroup_members`");
db_execute("DROP TABLE `npc_contactgroups`");
db_execute("DROP TABLE `npc_contactnotificationmethods`");
db_execute("DROP TABLE `npc_contactnotifications`");
db_execute("DROP TABLE `npc_contacts`");
db_execute("DROP TABLE `npc_contactstatus`");
db_execute("DROP TABLE `npc_customvariables`");
db_execute("DROP TABLE `npc_customvariablestatus`");
db_execute("DROP TABLE `npc_dbversion`");
db_execute("DROP TABLE `npc_downtimehistory`");
db_execute("DROP TABLE `npc_eventhandlers`");
db_execute("DROP TABLE `npc_externalcommands`");
db_execute("DROP TABLE `npc_flappinghistory`");
db_execute("DROP TABLE `npc_host_contactgroups`");
db_execute("DROP TABLE `npc_host_contacts`");
db_execute("DROP TABLE `npc_host_graphs`");
db_execute("DROP TABLE `npc_host_parenthosts`");
db_execute("DROP TABLE `npc_hostchecks`");
db_execute("DROP TABLE `npc_hostdependencies`");
db_execute("DROP TABLE `npc_hostescalation_contactgroups`");
db_execute("DROP TABLE `npc_hostescalation_contacts`");
db_execute("DROP TABLE `npc_hostescalations`");
db_execute("DROP TABLE `npc_hostgroup_members`");
db_execute("DROP TABLE `npc_hostgroups`");
db_execute("DROP TABLE `npc_hosts`");
db_execute("DROP TABLE `npc_hoststatus`");
db_execute("DROP TABLE `npc_instances`");
db_execute("DROP TABLE `npc_logentries`");
db_execute("DROP TABLE `npc_notifications`");
db_execute("DROP TABLE `npc_objects`");
db_execute("DROP TABLE `npc_processevents`");
db_execute("DROP TABLE `npc_programstatus`");
db_execute("DROP TABLE `npc_runtimevariables`");
db_execute("DROP TABLE `npc_scheduleddowntime`");
db_execute("DROP TABLE `npc_service_contactgroups`");
db_execute("DROP TABLE `npc_service_contacts`");
db_execute("DROP TABLE `npc_service_graphs`");
db_execute("DROP TABLE `npc_servicechecks`");
db_execute("DROP TABLE `npc_servicedependencies`");
db_execute("DROP TABLE `npc_serviceescalation_contactgroups`");
db_execute("DROP TABLE `npc_serviceescalation_contacts`");
db_execute("DROP TABLE `npc_serviceescalations`");
db_execute("DROP TABLE `npc_servicegroup_members`");
db_execute("DROP TABLE `npc_servicegroups`");
db_execute("DROP TABLE `npc_services`");
db_execute("DROP TABLE `npc_servicestatus`");
db_execute("DROP TABLE `npc_settings`");
db_execute("DROP TABLE `npc_statehistory`");
db_execute("DROP TABLE `npc_systemcommands`");
db_execute("DROP TABLE `npc_timedeventqueue`");
db_execute("DROP TABLE `npc_timedevents`");
db_execute("DROP TABLE `npc_timeperiod_timeranges`");
db_execute("DROP TABLE `npc_timeperiods`");
db_execute("ALTER TABLE `host` DROP `npc_host_object_id`");
db_execute("DELETE FROM `settings` WHERE `name` like 'npc\_%'");
api_plugin_remove_realms ('npc');
}
function npc_config_arrays () {
global $user_auth_realms, $user_auth_realm_filenames, $npc_date_format, $npc_time_format;
global $npc_default_settings, $npc_log_level, $npc_config_type;
if (isset($_SESSION["sess_user_id"])) {
$user_id=$_SESSION["sess_user_id"];
$npc_realm = db_fetch_cell("SELECT id FROM plugin_config WHERE directory = 'npc'");
$npc_enabled = db_fetch_cell("SELECT status FROM plugin_config WHERE directory = 'npc'");
if ($npc_enabled == "1") {
$user_auth_realm_filenames['npc.php'] = 9000 + $npc_realm;
$npc_log_level = array(
"0" => "None",
"1" => "ERROR - Log errors only",
"2" => "WARN - Log errors and warnings",
"3" => "INFO - Log errors, warnings, and info messages",
"4" => "DEBUG - Log everything"
);
$npc_config_type = array(
"0" => "0",
"1" => "1"
);
$npc_date_format = array(
"Y-m-d" => "2007-12-27",
"m-d-Y" => "12-27-2007",
"d-m-Y" => "27-12-2007",
"Y/m/d" => "2007/12/27",

紫颖
- 粉丝: 927
- 资源: 15
最新资源
- 毕设和企业适用springboot企业数据智能分析平台类及企业创新研发平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及企业财务管理系统源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及企业管理智能化平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及汽车管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及商业数据管理系统源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及社交媒体平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及市场营销自动化平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及数据智能化平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及数据可视化平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及投票平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及实时通信平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及视频分析平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及网络安全防护平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及招聘管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及云计算资源管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及资产管理平台源码+论文+视频.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


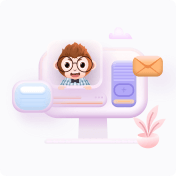
- 1
- 2
- 3
- 4
前往页