java_IO操作_(读写、追加、删除、移动、复制、修改)

### Java IO操作详解 在Java编程语言中,IO(Input/Output)操作是处理文件读写、网络数据传输等场景的基础。本文将详细介绍Java中进行文件基础操作的方法,包括读取、追加、删除、移动、复制以及修改文件的具体实现。 #### 一、读取文件内容 文件读取是文件操作中最常见的需求之一。Java提供了多种方式来读取文件内容,主要包括按字节读取、按字符读取、按行读取和随机读取等。 ##### 1. 按字节读取文件内容 按字节读取文件通常用于处理二进制文件,如图片、音频或视频等。主要使用`FileInputStream`类来实现: ```java public static void readFileByBytes(String fileName) { File file = new File(fileName); InputStream in = null; try { System.out.println("以字节为单位读取文件内容,一次读一个字节:"); // 一次读一个字节 in = new FileInputStream(file); int tempByte; while ((tempByte = in.read()) != -1) { System.out.write(tempByte); } System.out.println("以字节为单位读取文件内容,一次读多个字节:"); // 一次读多个字节 byte[] tempBytes = new byte[100]; int bytesRead = 0; in = new FileInputStream(fileName); ReadFromFile.showAvailableBytes(in); while ((bytesRead = in.read(tempBytes)) != -1) { System.out.write(tempBytes, 0, bytesRead); } } catch (IOException e) { e.printStackTrace(); } finally { if (in != null) { try { in.close(); } catch (IOException e) { e.printStackTrace(); } } } } ``` ##### 2. 按字符读取文件内容 按字符读取文件主要用于处理文本文件,如纯文本、XML或JSON等格式的文件。主要使用`InputStreamReader`和`FileReader`类来实现: ```java public static void readFileByChars(String fileName) { File file = new File(fileName); Reader reader = null; try { System.out.println("以字符为单位读取文件内容,一次读一个字符:"); // 一次读一个字符 reader = new InputStreamReader(new FileInputStream(file)); int tempChar; while ((tempChar = reader.read()) != -1) { // 对于Windows下的换行符处理 if (((char) tempChar) != '\r' && ((char) tempChar) != '\n') { System.out.print((char) tempChar); } } System.out.println("以字符为单位读取文件内容,一次读多个字符:"); // 一次读多个字符 char[] tempChars = new char[30]; int charsRead = 0; reader = new InputStreamReader(new FileInputStream(fileName)); while ((charsRead = reader.read(tempChars)) != -1) { // 处理换行符 if (charsRead == tempChars.length && tempChars[tempChars.length - 1] != '\r' && tempChars[tempChars.length - 1] != '\n') { System.out.print(tempChars); } } } catch (IOException e) { e.printStackTrace(); } finally { if (reader != null) { try { reader.close(); } catch (IOException e) { e.printStackTrace(); } } } } ``` ##### 3. 按行读取文件内容 按行读取文件内容适用于处理文本文件中的每一行数据。主要使用`BufferedReader`类来实现: ```java public static void readFileByLines(String fileName) { File file = new File(fileName); BufferedReader bufferedReader = null; try { bufferedReader = new BufferedReader(new FileReader(file)); String line; while ((line = bufferedReader.readLine()) != null) { System.out.println(line); } } catch (IOException e) { e.printStackTrace(); } finally { if (bufferedReader != null) { try { bufferedReader.close(); } catch (IOException e) { e.printStackTrace(); } } } } ``` ##### 4. 随机读取文件内容 随机读取文件内容是指可以在文件的任意位置进行读取操作,主要用于需要随机访问文件内容的场景。主要使用`RandomAccessFile`类来实现: ```java public static void readFileRandomly(String fileName) { File file = new File(fileName); RandomAccessFile randomAccessFile = null; try { randomAccessFile = new RandomAccessFile(file, "r"); long fileLength = randomAccessFile.length(); for (long curPosition = 0; curPosition < fileLength; curPosition++) { randomAccessFile.seek(curPosition); System.out.print((char)randomAccessFile.readUnsignedByte()); } } catch (IOException e) { e.printStackTrace(); } finally { if (randomAccessFile != null) { try { randomAccessFile.close(); } catch (IOException e) { e.printStackTrace(); } } } } ``` 通过以上介绍可以看出,Java提供了丰富的API来支持文件的读取操作。开发者可以根据实际需求选择合适的方式来处理文件。接下来,我们还将继续探讨文件的追加、删除、移动、复制及修改等操作。
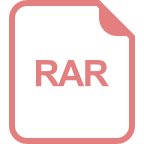
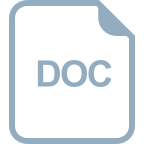
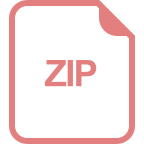
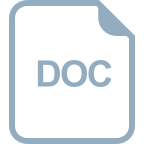
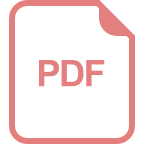
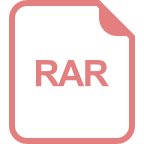
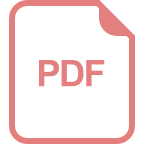
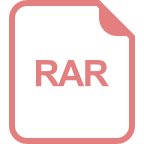
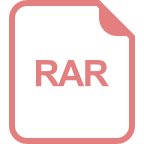
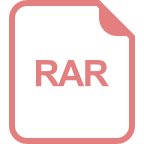
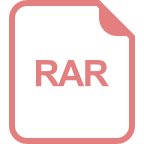
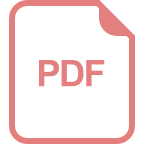
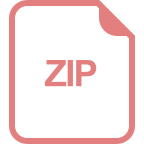
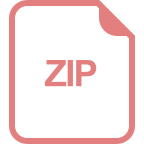
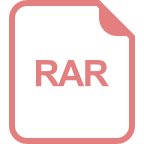
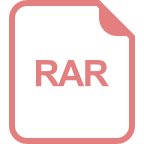
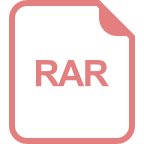
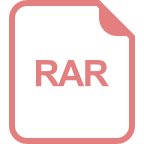
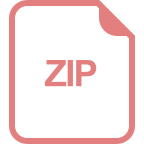
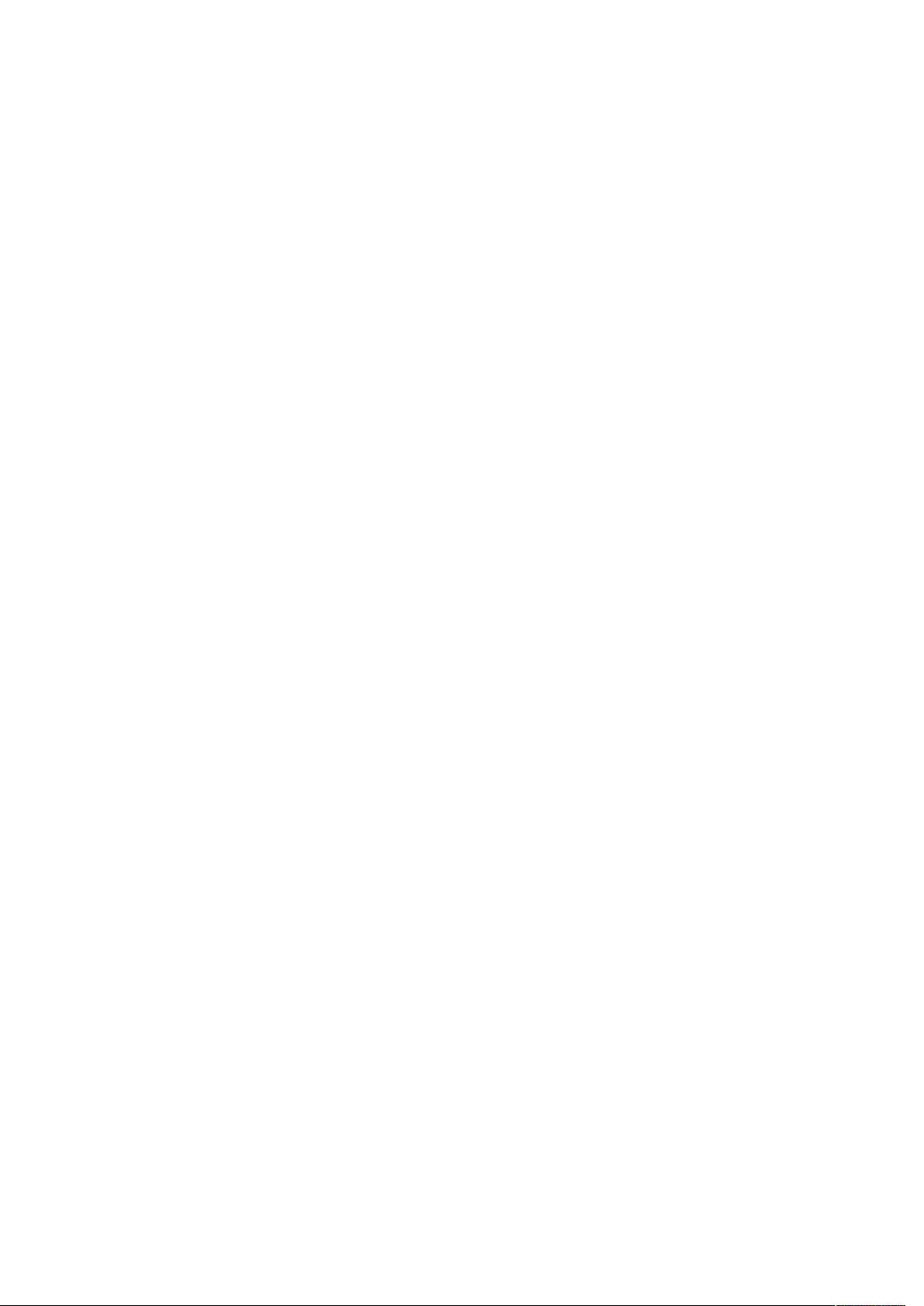
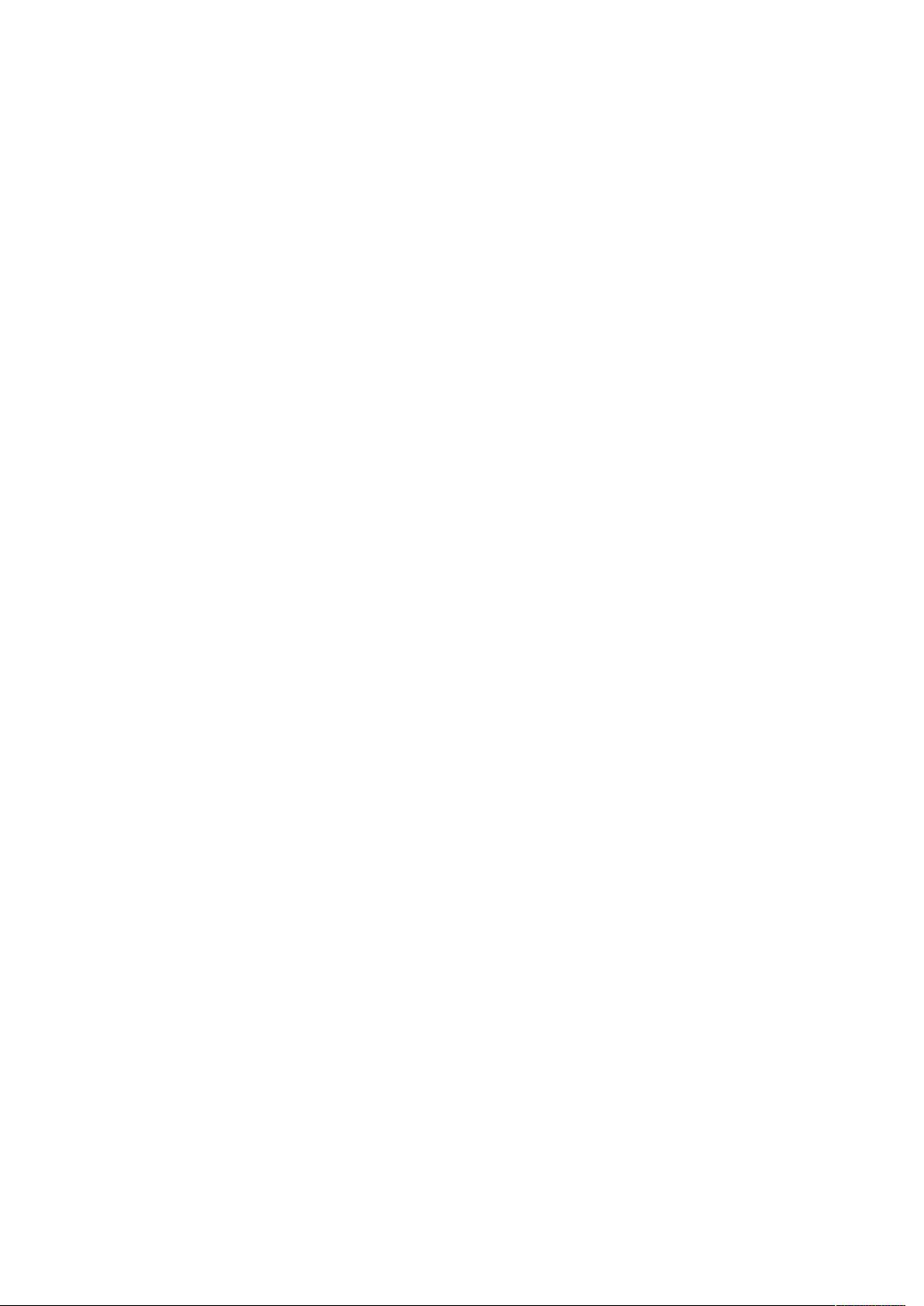
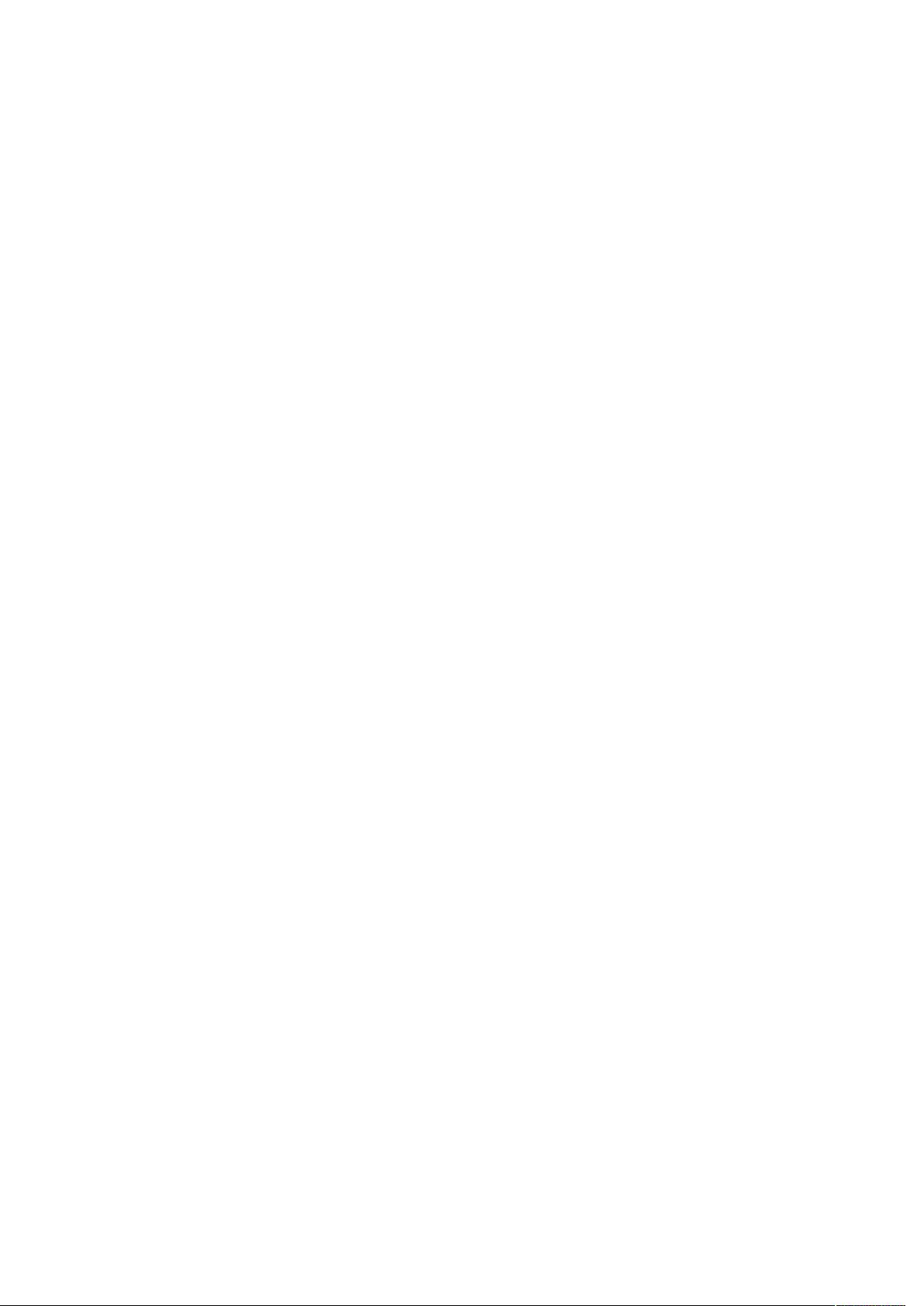
剩余14页未读,继续阅读
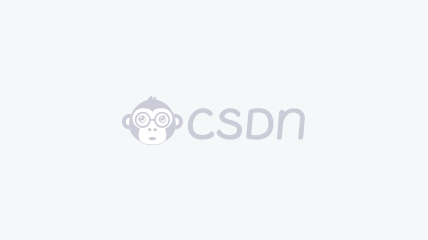
- YYKKYY135792015-12-02不是特别好
- qq_307214652015-10-27修改的注释没有啊 看着费劲啊
- jonayyyy2015-11-09修改的注释没有啊 看着费劲

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

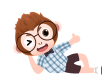
最新资源

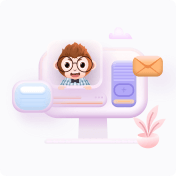
