在Android开发中,TextView是用于显示单行或多行文本的常用组件。在设计用户界面时,我们有时希望实现一种伸缩效果,使得当文本内容超过一定行数时,TextView显示一个伸缩图标,用户点击后可以展开查看全部内容。这种功能可以提升用户体验,避免长文本在有限的空间内压缩导致阅读困难。本文将详细讲解如何实现“TextView的伸缩效果,可多行才显示伸缩否则隐藏伸缩”。 我们需要自定义一个TextView,继承自Android原生的TextView类。在这个自定义的TextView中,我们可以添加额外的逻辑来判断何时显示伸缩图标。以下是一个简单的自定义TextView类的示例: ```java public class ExpandableTextView extends androidx.appcompat.widget.AppCompatTextView { private int maxLines; private boolean isExpanded = false; public ExpandableTextView(Context context) { super(context); } public ExpandableTextView(Context context, AttributeSet attrs) { super(context, attrs); init(attrs); } public ExpandableTextView(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); init(attrs); } private void init(AttributeSet attrs) { TypedArray a = getContext().obtainStyledAttributes(attrs, R.styleable.ExpandableTextView); maxLines = a.getInt(R.styleable.ExpandableTextView_maxLines, 2); a.recycle(); } @Override protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) { super.onMeasure(widthMeasureSpec, heightMeasureSpec); if (getLineCount() > maxLines && !isExpanded) { setMeasuredDimension(getMeasuredWidth(), getMeasuredHeight() + getLineHeight() * (getLineCount() - maxLines)); } } public void toggleExpansion() { isExpanded = !isExpanded; requestLayout(); } // 添加点击事件监听器,以便在点击时切换展开/折叠状态 public void setOnClickListener(OnClickListener listener) { super.setOnClickListener(listener); } } ``` 接下来,我们需要在布局XML文件中使用这个自定义的TextView,并设置`maxLines`属性,以限制默认显示的行数。同时,添加一个ImageView作为伸缩图标,并为其设置点击事件,触发TextView的展开和折叠。 ```xml <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical"> <com.example.yourpackage.ExpandableTextView android:id="@+id/expandable_text_view" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="@string/your_long_text" android:maxLines="2" app:layout_constraintTop_toTopOf="parent" android:ellipsize="end" /> <ImageView android:id="@+id/expand_collapse_icon" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/ic_expand_more" android:layout_gravity="end" android:onClick="onExpandCollapseIconClick" /> </LinearLayout> ``` 在对应的Activity或Fragment中,处理点击事件并调用TextView的`toggleExpansion()`方法: ```java public void onExpandCollapseIconClick(View view) { ExpandableTextView textView = findViewById(R.id.expandable_text_view); textView.toggleExpansion(); // 更新伸缩图标的方向(展开/折叠) ImageView icon = findViewById(R.id.expand_collapse_icon); if (textView.isExpanded()) { icon.setImageDrawable(getDrawable(R.drawable.ic_expand_less)); } else { icon.setImageDrawable(getDrawable(R.drawable.ic_expand_more)); } } ``` 在上述代码中,我们使用了`app:layout_constraintTop_toTopOf="parent"`来约束TextView的顶部,这在使用ConstraintLayout时很重要,确保布局正确。同时,`android:ellipsize="end"`属性用于在达到最大行数时在末尾添加省略号。 至于伸缩图标的资源文件(`ic_expand_more`, `ic_expand_less`),通常可以在Material Design图标库中找到,或者自行设计。它们分别表示折叠和展开的状态。 通过这种方式,我们可以创建一个具有伸缩效果的TextView,当文本内容超过设定的最大行数时,显示一个伸缩图标,用户点击后可以展开或折叠文本内容。这个功能在显示长文本但又需要保持界面简洁的情况下非常实用。在实际项目中,可以根据需求调整maxLines值、伸缩图标的样式以及交互逻辑,以适应不同的场景。
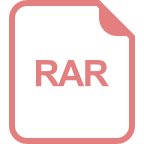
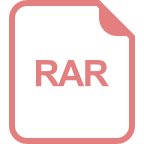
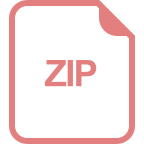
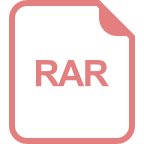
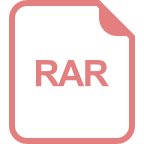
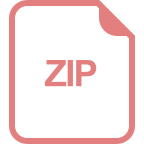
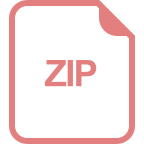
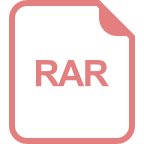
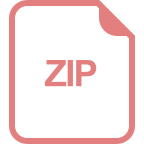
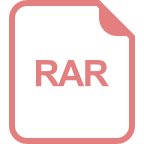
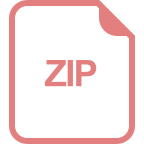
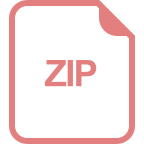
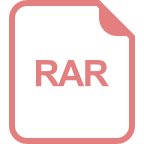
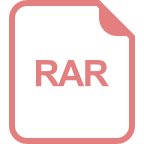
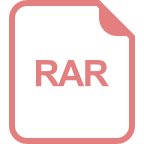



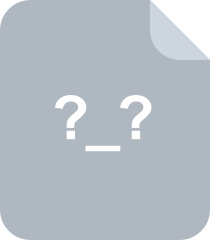
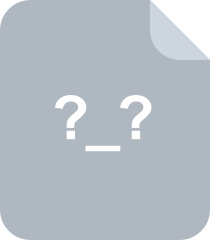


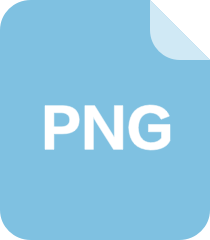
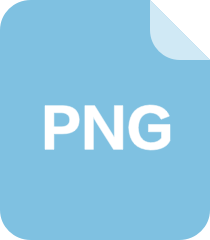
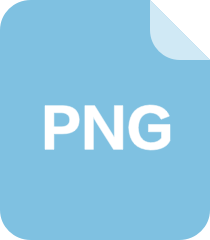

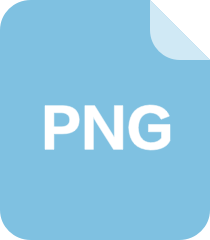

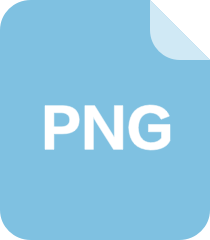

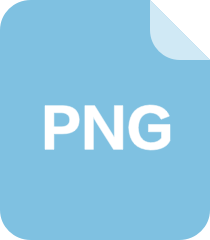

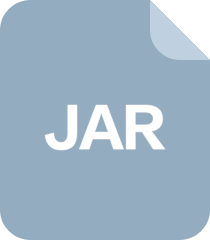
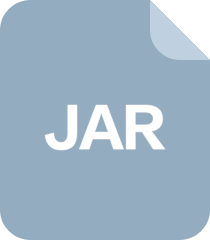




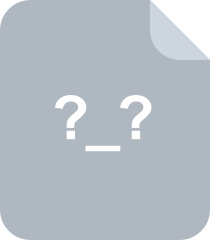
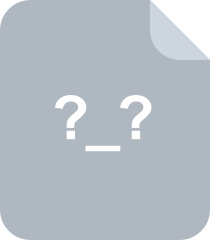
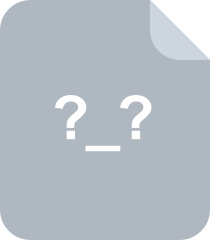
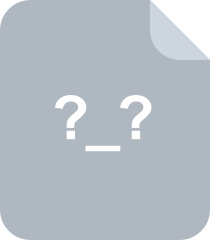
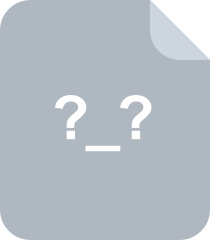
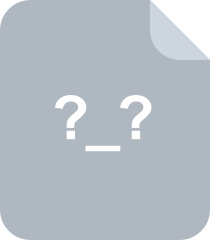
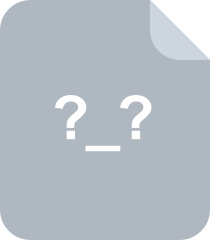
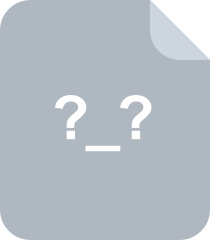
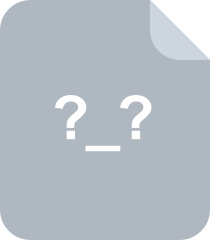
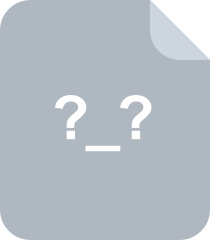
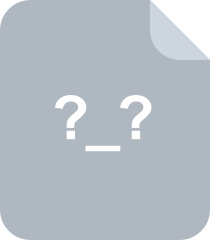
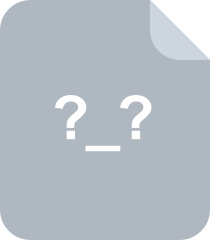
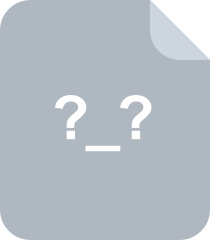
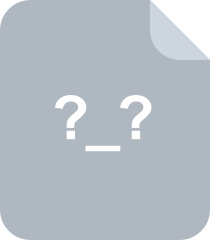



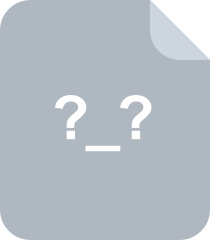

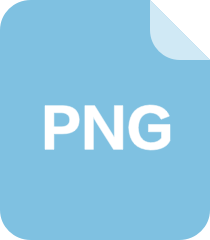
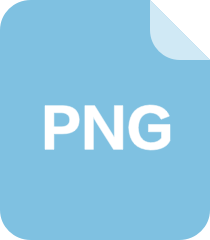
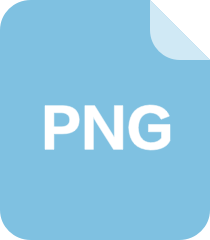

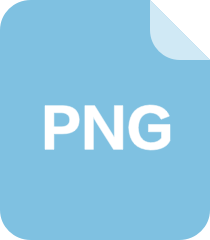

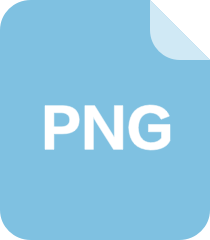

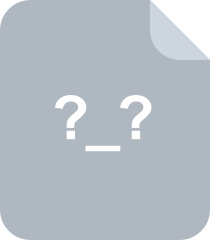
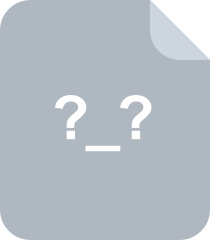
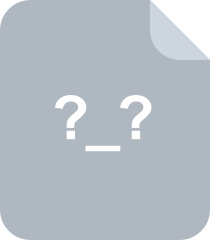

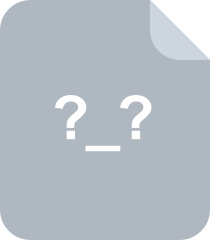

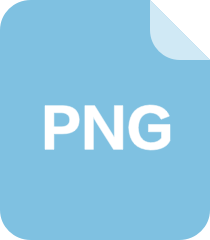

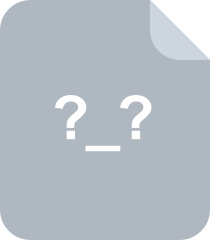

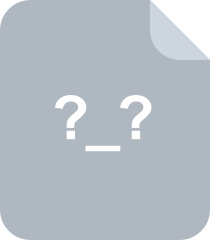
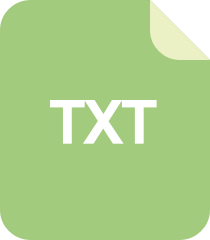
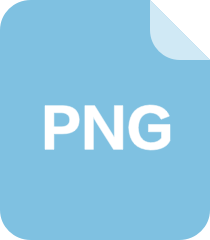





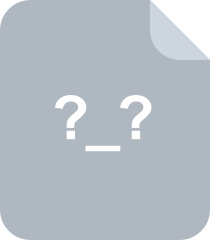
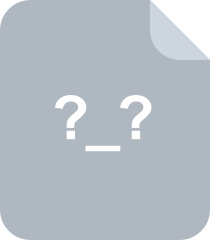




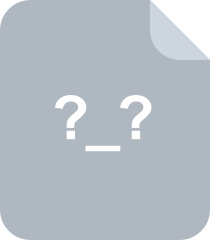
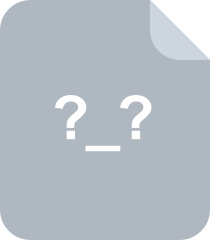
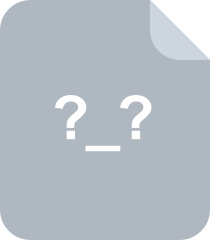
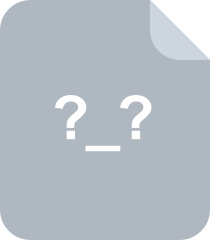
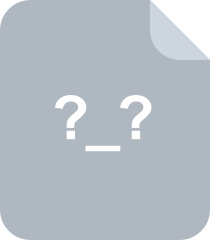

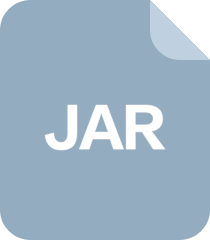
- 1
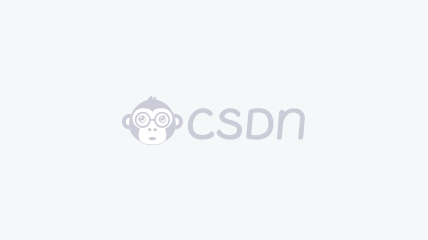
- lwg2552016-04-22效果还可以
- 詹森波恩2016-11-29多谢分享,折叠其实可以有多种样式的

- 粉丝: 47
- 资源: 50
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

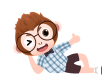
最新资源
- C语言-leetcode题解之70-climbing-stairs.c
- C语言-leetcode题解之68-text-justification.c
- C语言-leetcode题解之66-plus-one.c
- C语言-leetcode题解之64-minimum-path-sum.c
- C语言-leetcode题解之63-unique-paths-ii.c
- C语言-leetcode题解之62-unique-paths.c
- C语言-leetcode题解之61-rotate-list.c
- C语言-leetcode题解之59-spiral-matrix-ii.c
- C语言-leetcode题解之58-length-of-last-word.c
- 计算机编程课程设计基础教程

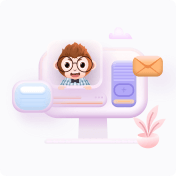
