package hibernate3.object;
import java.util.List;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.hibernate.LockMode;
import org.hibernate.Query;
import org.hibernate.criterion.Example;
/**
* A data access object (DAO) providing persistence and search support for
* Employee entities. Transaction control of the save(), update() and delete()
* operations can directly support Spring container-managed transactions or they
* can be augmented to handle user-managed Spring transactions. Each of these
* methods provides additional information for how to configure it for the
* desired type of transaction control.
*
* @see hibernate3.object.Employee
* @author MyEclipse Persistence Tools
*/
public class EmployeeDAO extends BaseHibernateDAO {
private static final Log log = LogFactory.getLog(EmployeeDAO.class);
// property constants
public static final String NAME = "name";
public static final String ADDRESS = "address";
public static final String TITLE = "title";
public static final String SALARY = "salary";
public void save(Employee transientInstance) {
log.debug("saving Employee instance");
try {
getSession().save(transientInstance);
log.debug("save successful");
} catch (RuntimeException re) {
log.error("save failed", re);
throw re;
}
}
public void delete(Employee persistentInstance) {
log.debug("deleting Employee instance");
try {
getSession().delete(persistentInstance);
log.debug("delete successful");
} catch (RuntimeException re) {
log.error("delete failed", re);
throw re;
}
}
public Employee findById(java.lang.Integer id) {
log.debug("getting Employee instance with id: " + id);
try {
Employee instance = (Employee) getSession().get(
"hibernate3.object.Employee", id);
return instance;
} catch (RuntimeException re) {
log.error("get failed", re);
throw re;
}
}
public List findByExample(Employee instance) {
log.debug("finding Employee instance by example");
try {
List results = getSession().createCriteria(
"hibernate3.object.Employee").add(Example.create(instance))
.list();
log.debug("find by example successful, result size: "
+ results.size());
return results;
} catch (RuntimeException re) {
log.error("find by example failed", re);
throw re;
}
}
public List findByProperty(String propertyName, Object value) {
log.debug("finding Employee instance with property: " + propertyName
+ ", value: " + value);
try {
String queryString = "from Employee as model where model."
+ propertyName + "= ?";
Query queryObject = getSession().createQuery(queryString);
queryObject.setParameter(0, value);
return queryObject.list();
} catch (RuntimeException re) {
log.error("find by property name failed", re);
throw re;
}
}
public List findByName(Object name) {
return findByProperty(NAME, name);
}
public List findByAddress(Object address) {
return findByProperty(ADDRESS, address);
}
public List findByTitle(Object title) {
return findByProperty(TITLE, title);
}
public List findBySalary(Object salary) {
return findByProperty(SALARY, salary);
}
public List findAll() {
log.debug("finding all Employee instances");
try {
String queryString = "from Employee";
Query queryObject = getSession().createQuery(queryString);
return queryObject.list();
} catch (RuntimeException re) {
log.error("find all failed", re);
throw re;
}
}
public Employee merge(Employee detachedInstance) {
log.debug("merging Employee instance");
try {
Employee result = (Employee) getSession().merge(detachedInstance);
log.debug("merge successful");
return result;
} catch (RuntimeException re) {
log.error("merge failed", re);
throw re;
}
}
public void attachDirty(Employee instance) {
log.debug("attaching dirty Employee instance");
try {
getSession().saveOrUpdate(instance);
log.debug("attach successful");
} catch (RuntimeException re) {
log.error("attach failed", re);
throw re;
}
}
public void attachClean(Employee instance) {
log.debug("attaching clean Employee instance");
try {
getSession().lock(instance, LockMode.NONE);
log.debug("attach successful");
} catch (RuntimeException re) {
log.error("attach failed", re);
throw re;
}
}
}

zhi070202021016
- 粉丝: 6
- 资源: 58
最新资源
- 形状分类31-YOLO(v5至v11)、COCO、CreateML、Darknet、Paligemma、VOC数据集合集.rar
- 常见排序算法概述及其性能比较
- 前端开发中的JS快速排序算法原理及实现方法
- 基于Java的环境保护与宣传网站论文.doc
- 基于8086的电子琴程序Proteus仿真
- 基于java的二手车交易网站的设计和实现论文.doc
- 纯真IP库,用于ip查询地址使用的数据库文件
- 基于javaweb的动漫网站管理系统毕业设计论文.doc
- 废物垃圾检测28-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 探索CSDN博客数据:使用Python爬虫技术
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


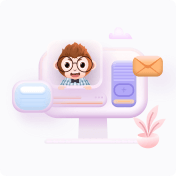