# JStructure
JavaScript 版本的数据结构,提供常用的数据结构封装,基于清华大学邓俊辉老师的数据结构课程
[](https://travis-ci.org/open-node/jstructures)
[](https://codecov.io/gh/open-node/jstructures)
# 进度
* [x] List (linked-list)
* [x] Vector
* [x] Stack
* [x] Queue
* [x] SegmentTree
* [x] UnionFind
* [x] BinTree
* [x] BST (BinanySearchTree)
* [ ] BTree
* [x] Trie
* [ ] Graph
* [x] Heap
* [ ] LeftHeap
* [ ] Priority Queue
<!-- Generated by documentation.js. Update this documentation by updating the source code. -->
### Table of Contents
<!-- Generated by documentation.js. Update this documentation by updating the source code. -->
### Table of Contents
- [AVL][1]
- [insert][2]
- [Parameters][3]
- [remove][4]
- [Parameters][5]
- [balanced][6]
- [Parameters][7]
- [AVLBalanced][8]
- [Parameters][9]
- [balFac][10]
- [Parameters][11]
- [height][12]
- [Parameters][13]
- [BST][14]
- [search][15]
- [Parameters][16]
- [insert][17]
- [Parameters][18]
- [remove][19]
- [Parameters][20]
- [removeAt][21]
- [Parameters][22]
- [searchIn][23]
- [Parameters][24]
- [BinNode][25]
- [Parameters][26]
- [data][27]
- [parent][28]
- [lc][29]
- [rc][30]
- [height][31]
- [size][32]
- [isRoot][33]
- [isLChild][34]
- [isRChild][35]
- [hasParent][36]
- [hasLChild][37]
- [hasRChild][38]
- [hasChild][39]
- [hasBothChild][40]
- [isLeaf][41]
- [sibling][42]
- [uncle][43]
- [fromParentTo][44]
- [pred][45]
- [succ][46]
- [insertAsLC][47]
- [Parameters][48]
- [insertAsRC][49]
- [Parameters][50]
- [travLevel][51]
- [Parameters][52]
- [travPre][53]
- [Parameters][54]
- [travIn][55]
- [Parameters][56]
- [travPost][57]
- [Parameters][58]
- [swap][59]
- [Parameters][60]
- [BinTree][61]
- [size][62]
- [size][63]
- [Parameters][64]
- [empty][65]
- [root][66]
- [root][67]
- [Parameters][68]
- [updateHeightAbove][69]
- [Parameters][70]
- [insertAsRoot][71]
- [Parameters][72]
- [insertAsLC][73]
- [Parameters][74]
- [insertAsRC][75]
- [Parameters][76]
- [attachAsLC][77]
- [Parameters][78]
- [attachAsRC][79]
- [Parameters][80]
- [remove][81]
- [Parameters][82]
- [secede][83]
- [Parameters][84]
- [travLevel][85]
- [Parameters][86]
- [travPre][87]
- [Parameters][88]
- [travIn][89]
- [Parameters][90]
- [travPost][91]
- [Parameters][92]
- [Heap][93]
- [Parameters][94]
- [size][95]
- [percolateUp][96]
- [Parameters][97]
- [percolateDown][98]
- [Parameters][99]
- [insert][100]
- [Parameters][101]
- [getMax][102]
- [delMax][103]
- [Parameters][104]
- [properParent][105]
- [Parameters][106]
- [ListNode][107]
- [Parameters][108]
- [insertAsSucc][109]
- [Parameters][110]
- [insertAsPred][111]
- [Parameters][112]
- [List][113]
- [Parameters][114]
- [size][115]
- [first][116]
- [last][117]
- [insertAsFirst][118]
- [Parameters][119]
- [insertAsLast][120]
- [Parameters][121]
- [insertA][122]
- [Parameters][123]
- [insertB][124]
- [Parameters][125]
- [remove][126]
- [Parameters][127]
- [disordered][128]
- [findElem][129]
- [Parameters][130]
- [search][131]
- [Parameters][132]
- [deduplicate][133]
- [uniquify][134]
- [traverse][135]
- [Parameters][136]
- [valid][137]
- [Parameters][138]
- [selectMax][139]
- [Parameters][140]
- [insertionSort][141]
- [Parameters][142]
- [selectionSort][143]
- [Parameters][144]
- [merge][145]
- [Parameters][146]
- [mergeSort][147]
- [Parameters][148]
- [Queue][149]
- [enqueue][150]
- [Parameters][151]
- [dequeue][152]
- [front][153]
- [empty][154]
- [size][155]
- [SegmentTree][156]
- [Parameters][157]
- [size][158]
- [leftChild][159]
- [Parameters][160]
- [rightChild][161]
- [Parameters][162]
- [build][163]
- [Parameters][164]
- [query][165]
- [Parameters][166]
- [update][167]
- [Parameters][168]
- [Stack][169]
- [push][170]
- [Parameters][171]
- [pop][172]
- [top][173]
- [empty][174]
- [size][175]
- [Trie][176]
- [size][177]
- [root][178]
- [insert][179]
- [Parameters][180]
- [find][181]
- [Parameters][182]
- [UnionFind][183]
- [Parameters][184]
- [find][185]
- [Parameters][186]
- [union][187]
- [Parameters][188]
- [isConnected][189]
- [Parameters][190]
- [toString][191]
- [Vector][192]
- [Parameters][193]
- [size][194]
- [insert][195]
- [Parameters][196]
- [removeRange][197]
- [Parameters][198]
- [remove][199]
- [Parameters][200]
- [disordered][201]
- [findElem][202]
- [Parameters][203]
- [search][204]
- [Parameters][205]
- [deduplicate][206]
- [uniquify][207]
- [traverse][208]
- [Parameters][209]
- [binSearch][210]
- [Parameters][211]
- [bubbleSort][212]
- [Parameters][213]
- [merge][214]
- [Parameters][215]
- [mergeSort][216]
- [Parameters][217]
## AVL
**Extends BST**
AVL 类(AVL 树, 继承自 BST)
Returns **[AVL][218]** Instance
### insert
插入元素
#### Parameters
- `e` **Anyone** 要插入的数据元素
Returns **[BinNode][219]**
### remove
删除元素, 注意是删除节点,非节点为根的子树
#### Parameters
- `e` **Anyone** 要插入的数据元素
Returns **[Boolean][220]** 是否成功删除
### balanced
判断某个节点是否理想平衡即:左右高度相等
#### Parameters
- `x`
- `要判断的节点` **[BinNode][219]**
Returns **[Boolean][220]**
### AVLBalanced
判断某个节点是否AVL平衡即:-2<左高度-右高度<2
#### Parameters
- `x`
- `要判断的节点` **[BinNode][219]**
Returns **[Boolean][220]**
### balFac
获取节点的平衡因子 x.lc.height - x.rc.height;
#### Parameters
- `x`
- `要判断的节点` **[BinNode][219]**
Returns **[number][221]** 左子树高度和右子树高度的差值
### height
获取节点的高度
#### Parameters
- `x`
- `要判断的节点` **[BinNode][219]**
Returns **[number][221]** 节点高度,空树高 -1, 叶子高 0
## BST
**Extends BinTree**
BST 类(二叉搜索树类, 继承自 BinTree)
Returns **[BST][222]** Instance
### search
查找元素 e 所在的节点
#### Parameters
- `e` **Anyone** 要搜索的元素
Returns **\[[BinNode][219]]** 返回两项,第一项是搜索命中的节点,第二项是命中节点的父节点
### insert
插入元素
#### Parameters
- `e` **Anyone** 要插入的数据元素
Returns **[BinNode][219]**
### remove
删除元素, 注意是删除节点,非节点为根的子树
#### Parameters
- `e` **Anyone** 要插入的数据元素
Retur
没有合适的资源?快使用搜索试试~ 我知道了~
JavaScript 版本的数据结构,提供常用的数据结构封装,基于清华大学邓俊辉老师的数据结构课程.zip
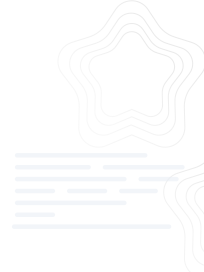
共62个文件
js:27个
css:6个
woff2:4个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 142 浏览量
2025-01-02
21:02:50
上传
评论
收藏 1.24MB ZIP 举报
温馨提示
J结构JavaScript 版本的数据结构,提供常用的数据结构封装,基于清华大学邓俊辉老师的数据结构课程 进度列表(链接列表)向量堆队列线段树联合查找二叉树BST(二叉搜索树)B树字典树图形堆左堆优先队列目录目录AVL插入参数消除参数均衡参数AVL平衡参数巴尔法克参数高度参数BST搜索参数插入参数消除参数移除参数搜索参数二进制节点参数数据父母液晶rc高度尺寸是Root是LChild是RChild有父级有孩子hasRCild 属性有孩子hasBothChild是叶子兄弟叔叔fromParentTo前成功插入AsLC参数插入AsRC参数旅行级别参数旅行预参数旅行参数旅行邮报参数交换参数二叉树尺寸尺寸参数空的根根参数更新高度参数插入根目录参数插入AsLC参数插入AsRC参数连接AsLC参数附加到RC参数消除参数脱离参数旅行级别参数旅行预参数旅行参数旅行邮报参数堆参
资源推荐
资源详情
资源评论
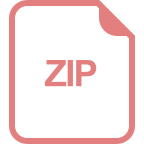
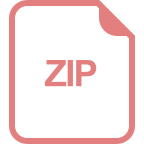
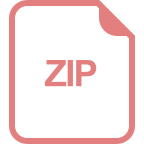
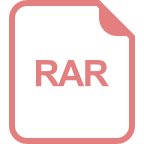
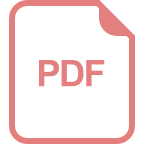
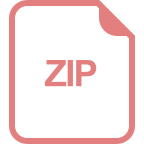
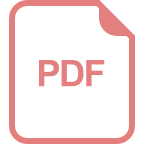
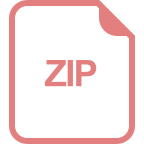
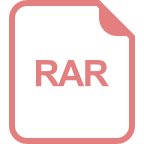
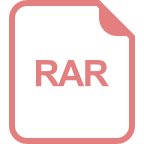
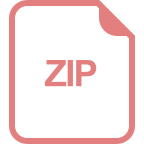
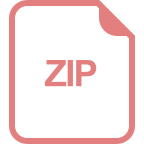
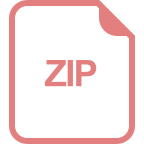
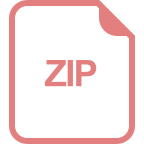
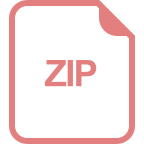
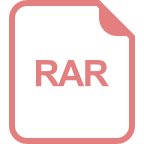
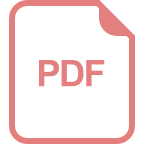
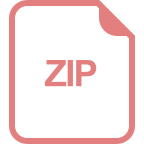
收起资源包目录

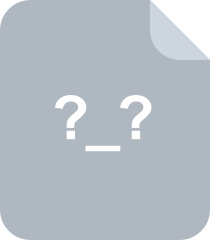
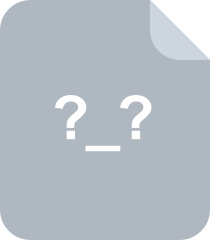
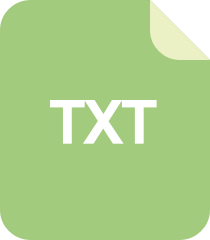



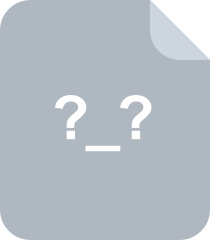
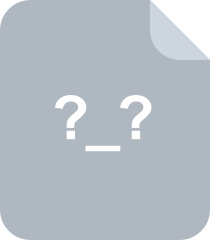


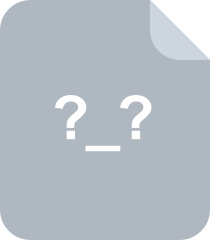
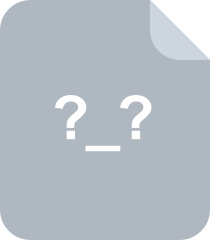


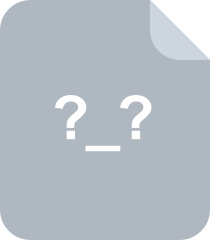
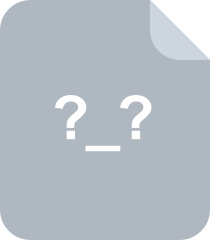


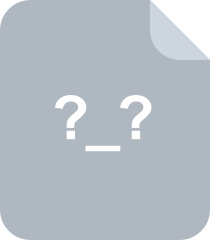
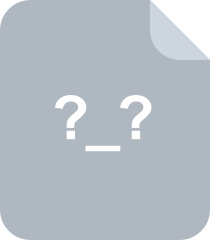
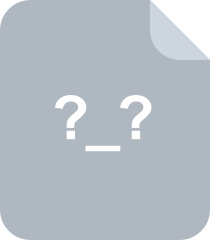


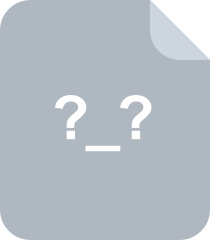
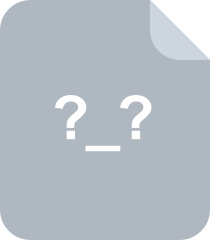


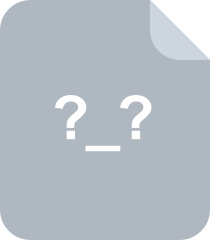
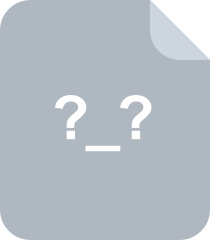


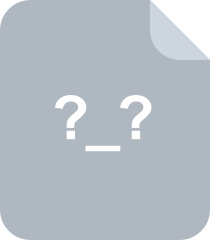
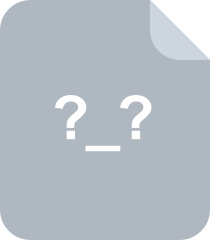


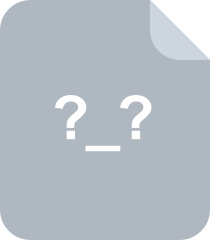
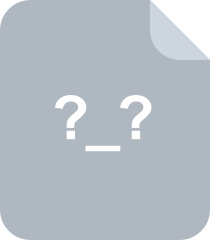
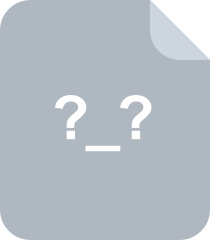
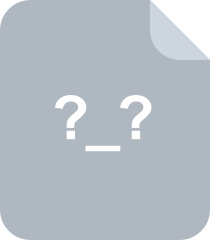

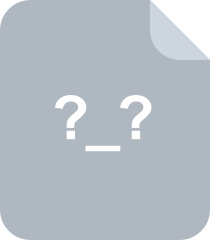


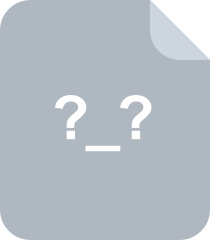
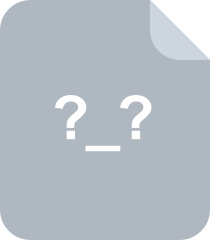


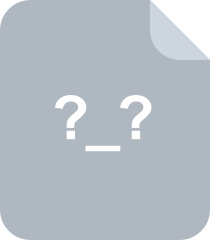
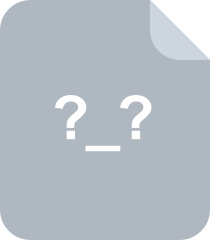
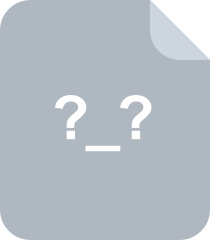


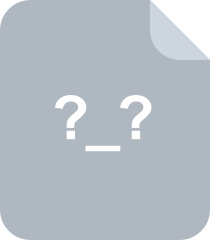
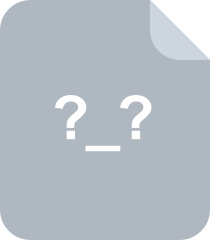
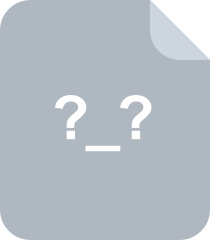
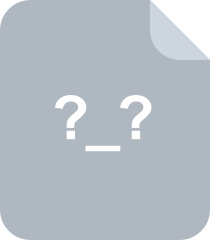
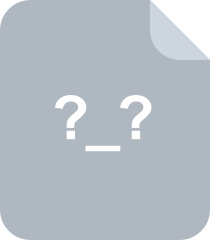
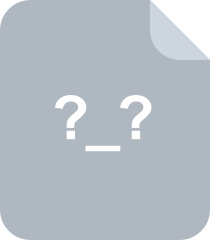
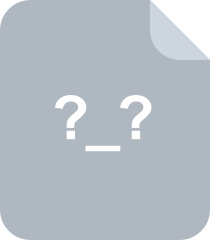
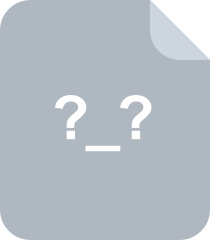


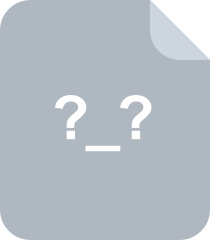
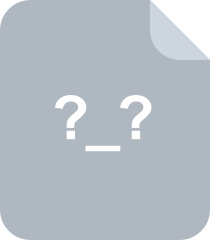
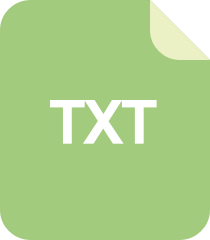
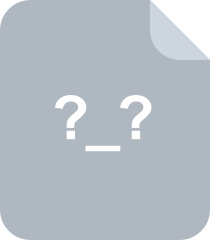

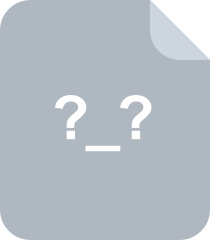
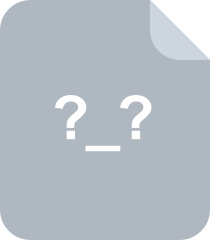


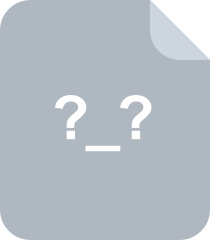
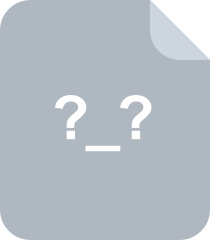

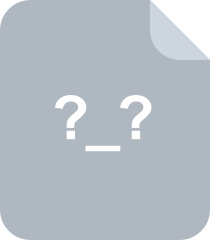
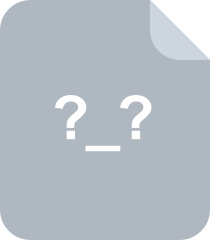


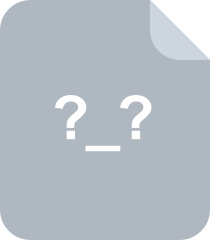
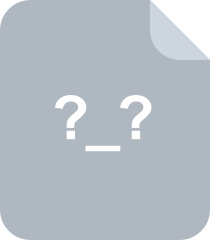

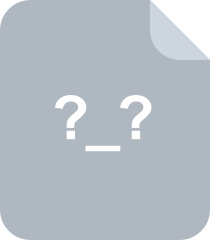
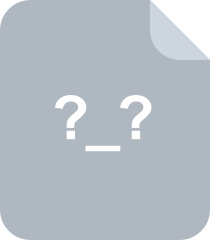

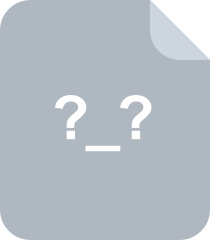
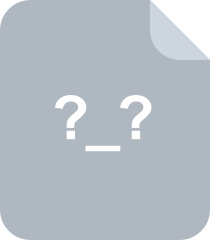
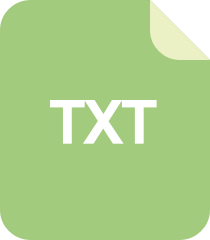
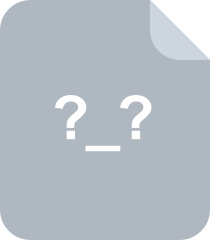
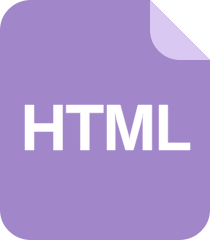
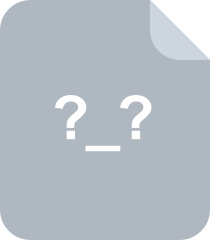
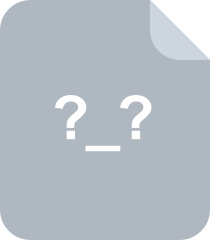
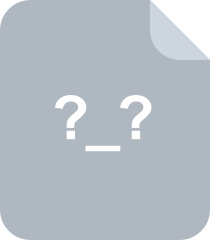
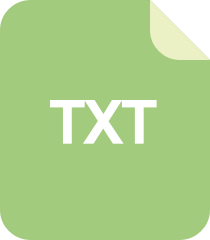
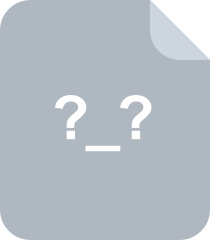
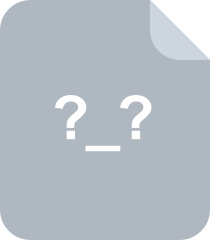
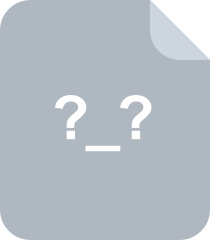
共 62 条
- 1
资源评论
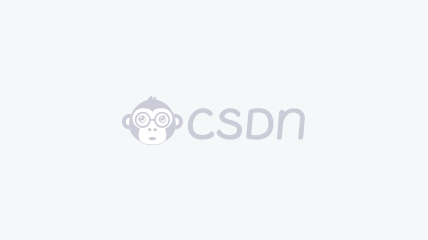

赵闪闪168
- 粉丝: 1726
- 资源: 6932
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

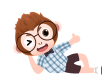
最新资源
- 【岗位说明】绩效专员岗位职责02.doc
- 【岗位说明】人力资源部部门经理人事专员培训专员绩效薪酬专员绩效考核专员岗位职责.doc
- 【岗位说明】人力资源岗位说明书02.doc
- 【岗位说明】人力资源部岗位设置岗位职责.doc
- 【岗位说明】人力资源部岗位职责说明书.doc
- 【岗位说明】人力资源经理岗位职责说明书.doc
- 【岗位说明】人力资源部岗位说明书.doc
- 【岗位说明】人力资源部门职责及部门岗位说明书.doc
- 【岗位说明】人力资源部经理岗位职责.doc
- 【岗位说明】薪酬绩效专员岗位职责说明书.doc
- 【岗位说明】社保专员岗位说明书.doc
- 【岗位说明】人力资源总监岗位职责02.doc
- 【岗位说明】招聘专员岗位职责说明书.doc
- 【岗位说明】员工关系专员职位说明书.xls
- 【岗位说明】广告公司岗位职责02.doc
- 【岗位说明】广告公司岗位职责01.doc
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


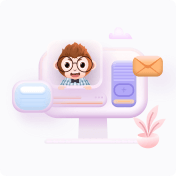
安全验证
文档复制为VIP权益,开通VIP直接复制
