[](https://github.com/redis/ioredis)
[](https://github.com/redis/ioredis/actions/workflows/release.yml?query=branch%3Amain)
[](https://coveralls.io/github/luin/ioredis?branch=main)
[](http://commitizen.github.io/cz-cli/)
[](https://github.com/semantic-release/semantic-release)
[](https://discord.gg/redis)
[](https://www.twitch.tv/redisinc)
[](https://www.youtube.com/redisinc)
[](https://twitter.com/redisinc)
A robust, performance-focused and full-featured [Redis](http://redis.io) client for [Node.js](https://nodejs.org).
Supports Redis >= 2.6.12. Completely compatible with Redis 7.x.
# Features
ioredis is a robust, full-featured Redis client that is
used in the world's biggest online commerce company [Alibaba](http://www.alibaba.com/) and many other awesome companies.
0. Full-featured. It supports [Cluster](http://redis.io/topics/cluster-tutorial), [Sentinel](https://redis.io/docs/reference/sentinel-clients), [Streams](https://redis.io/topics/streams-intro), [Pipelining](http://redis.io/topics/pipelining), and of course [Lua scripting](http://redis.io/commands/eval), [Redis Functions](https://redis.io/topics/functions-intro), [Pub/Sub](http://redis.io/topics/pubsub) (with the support of binary messages).
1. High performance ð.
2. Delightful API ð. It works with Node callbacks and Native promises.
3. Transformation of command arguments and replies.
4. Transparent key prefixing.
5. Abstraction for Lua scripting, allowing you to [define custom commands](https://github.com/redis/ioredis#lua-scripting).
6. Supports [binary data](https://github.com/redis/ioredis#handle-binary-data).
7. Supports [TLS](https://github.com/redis/ioredis#tls-options) ð.
8. Supports offline queue and ready checking.
9. Supports ES6 types, such as `Map` and `Set`.
10. Supports GEO commands ð.
11. Supports Redis ACL.
12. Sophisticated error handling strategy.
13. Supports NAT mapping.
14. Supports autopipelining.
**100% written in TypeScript and official declarations are provided:**
<img width="837" src="resources/ts-screenshot.png" alt="TypeScript Screenshot" />
# Versions
| Version | Branch | Node.js Version | Redis Version |
| -------------- | ------ | --------------- | --------------- |
| 5.x.x (latest) | main | >= 12 | 2.6.12 ~ latest |
| 4.x.x | v4 | >= 6 | 2.6.12 ~ 7 |
Refer to [CHANGELOG.md](CHANGELOG.md) for features and bug fixes introduced in v5.
ð [Upgrading from v4 to v5](https://github.com/redis/ioredis/wiki/Upgrading-from-v4-to-v5)
# Links
- [API Documentation](https://redis.github.io/ioredis/) ([Redis](https://redis.github.io/ioredis/classes/Redis.html), [Cluster](https://redis.github.io/ioredis/classes/Cluster.html))
- [Changelog](CHANGELOG.md)
<hr>
# Quick Start
## Install
```shell
npm install ioredis
```
In a TypeScript project, you may want to add TypeScript declarations for Node.js:
```shell
npm install --save-dev @types/node
```
## Basic Usage
```javascript
// Import ioredis.
// You can also use `import { Redis } from "ioredis"`
// if your project is a TypeScript project,
// Note that `import Redis from "ioredis"` is still supported,
// but will be deprecated in the next major version.
const Redis = require("ioredis");
// Create a Redis instance.
// By default, it will connect to localhost:6379.
// We are going to cover how to specify connection options soon.
const redis = new Redis();
redis.set("mykey", "value"); // Returns a promise which resolves to "OK" when the command succeeds.
// ioredis supports the node.js callback style
redis.get("mykey", (err, result) => {
if (err) {
console.error(err);
} else {
console.log(result); // Prints "value"
}
});
// Or ioredis returns a promise if the last argument isn't a function
redis.get("mykey").then((result) => {
console.log(result); // Prints "value"
});
redis.zadd("sortedSet", 1, "one", 2, "dos", 4, "quatro", 3, "three");
redis.zrange("sortedSet", 0, 2, "WITHSCORES").then((elements) => {
// ["one", "1", "dos", "2", "three", "3"] as if the command was `redis> ZRANGE sortedSet 0 2 WITHSCORES`
console.log(elements);
});
// All arguments are passed directly to the redis server,
// so technically ioredis supports all Redis commands.
// The format is: redis[SOME_REDIS_COMMAND_IN_LOWERCASE](ARGUMENTS_ARE_JOINED_INTO_COMMAND_STRING)
// so the following statement is equivalent to the CLI: `redis> SET mykey hello EX 10`
redis.set("mykey", "hello", "EX", 10);
```
See the `examples/` folder for more examples. For example:
- [TTL](examples/ttl.js)
- [Strings](examples/string.js)
- [Hashes](examples/hash.js)
- [Lists](examples/list.js)
- [Sets](examples/set.js)
- [Sorted Sets](examples/zset.js)
- [Streams](examples/stream.js)
- [Redis Modules](examples/module.js) e.g. RedisJSON
All Redis commands are supported. See [the documentation](https://redis.github.io/ioredis/classes/Redis.html) for details.
## Connect to Redis
When a new `Redis` instance is created,
a connection to Redis will be created at the same time.
You can specify which Redis to connect to by:
```javascript
new Redis(); // Connect to 127.0.0.1:6379
new Redis(6380); // 127.0.0.1:6380
new Redis(6379, "192.168.1.1"); // 192.168.1.1:6379
new Redis("/tmp/redis.sock");
new Redis({
port: 6379, // Redis port
host: "127.0.0.1", // Redis host
username: "default", // needs Redis >= 6
password: "my-top-secret",
db: 0, // Defaults to 0
});
```
You can also specify connection options as a [`redis://` URL](http://www.iana.org/assignments/uri-schemes/prov/redis) or [`rediss://` URL](https://www.iana.org/assignments/uri-schemes/prov/rediss) when using [TLS encryption](#tls-options):
```javascript
// Connect to 127.0.0.1:6380, db 4, using password "authpassword":
new Redis("redis://:authpassword@127.0.0.1:6380/4");
// Username can also be passed via URI.
new Redis("redis://username:authpassword@127.0.0.1:6380/4");
```
See [API Documentation](https://redis.github.io/ioredis/index.html#RedisOptions) for all available options.
## Pub/Sub
Redis provides several commands for developers to implement the [Publishâsubscribe pattern](https://en.wikipedia.org/wiki/Publish%E2%80%93subscribe_pattern). There are two roles in this pattern: publisher and subscriber. Publishers are not programmed to send their messages to specific subscribers. Rather, published messages are characterized into channels, without knowledge of what (if any) subscribers there may be.
By leveraging Node.js's built-in events module, ioredis makes pub/sub very straightforward to use. Below is a simple example that consists of two files, one is publisher.js that publishes messages to a channel, the other is subscriber.js that listens for messages on specific channels.
```javascript
// publisher.js
const Redis = require("ioredis");
const redis = new Redis();
setInterval(() => {
const message = { foo: Math.random() };
// Publish to my-channel-1 or my-channel-2 randomly.
const channel = `my-channel-${1 + Math.round(Math.random())}`;
// Message can be either a string or a buffer
redis.publish(channel, JSON.stringify(message));
console.log("Published %s to %s", message, channel);
}, 1000);
```
```javascript
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
适用于Node.js的强大、注重性能且功能齐全的Redis客户端。支持 Redis >= 2.6.12。与 Redis 7.x 完全兼容。特征ioredis 是一个强大且功能齐全的 Redis 客户端,被全球最大的在线商务公司阿里巴巴和许多其他优秀公司使用。功能齐全。它支持Cluster、Sentinel、Streams、Pipelining,当然还有Lua 脚本、Redis Functions、Pub/Sub(支持二进制消息)。高性能。令人愉悦的 API 。它适用于 Node 回调和 Native 承诺。命令参数和答复的转换。透明密钥前缀。Lua 脚本的抽象,允许您定义自定义命令。支持二进制数据。支持TLS 。支持离线队列和就绪检查。支持 ES6 类型,例如Map和Set。支持 GEO 命令。支持Redis ACL。复杂的错误处理策略。支持NAT映射。支持自动流水线。100%用 TypeScript 编写,并提供官方声明版本版本
资源推荐
资源详情
资源评论
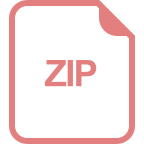
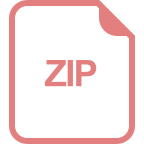
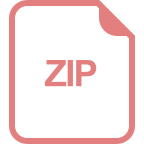
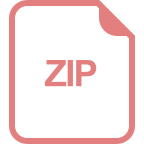
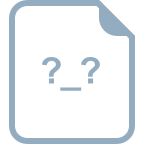
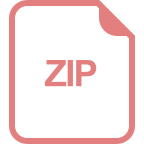
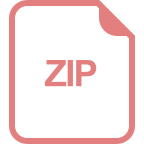
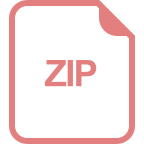
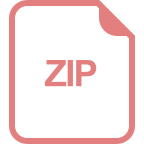
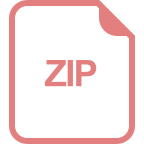
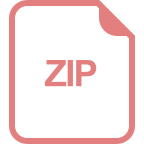
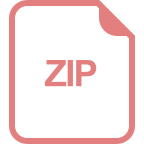
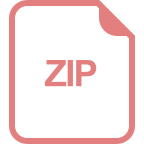
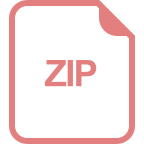
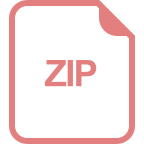
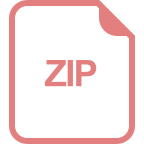
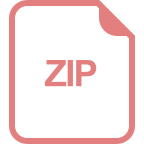
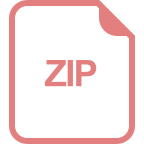
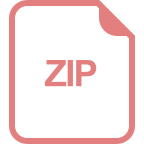
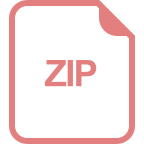
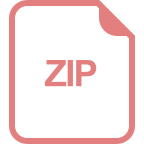
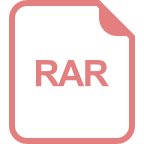
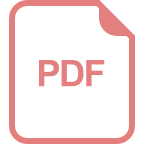
收起资源包目录

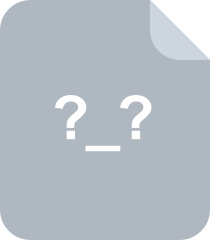
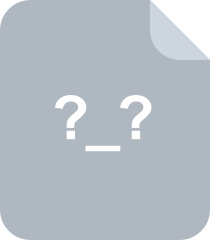
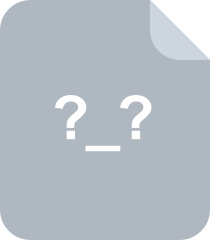
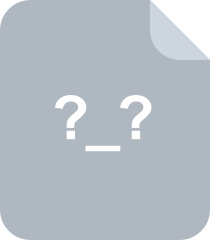
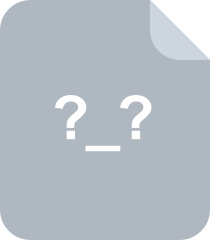
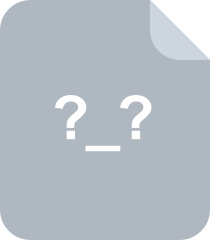
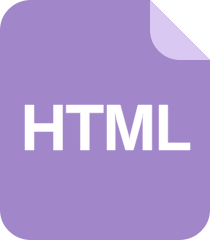
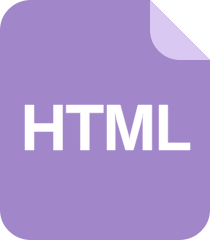
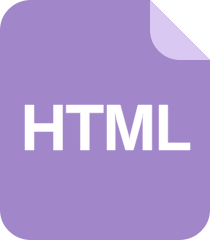
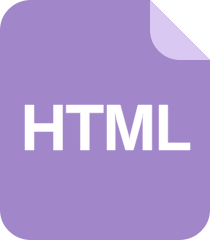
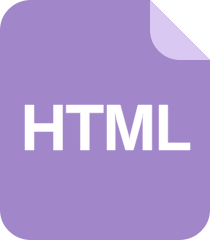
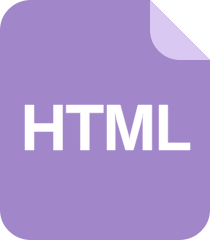
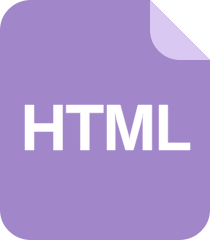
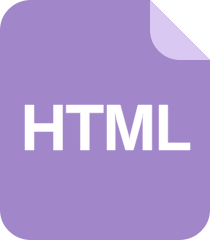
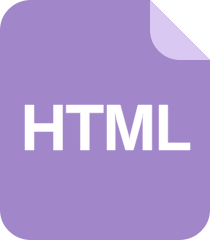
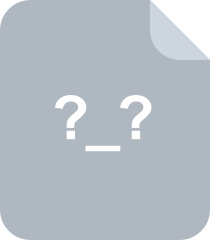
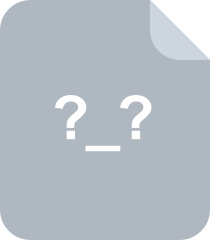
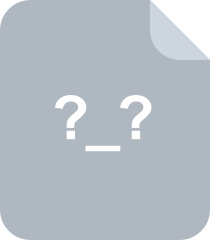
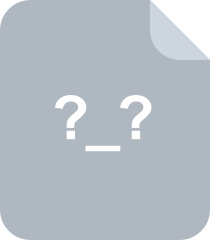
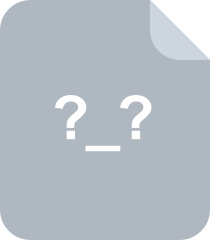
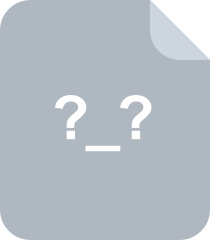
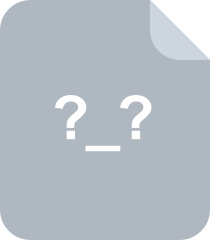
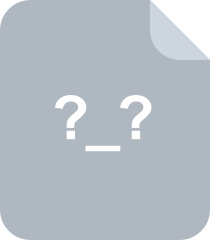
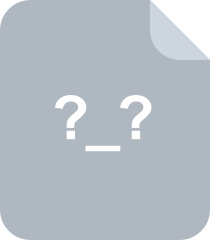
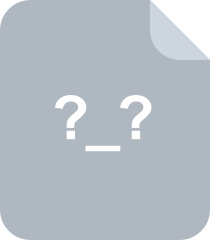
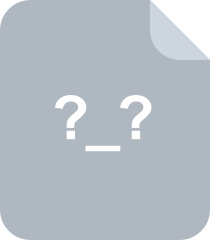
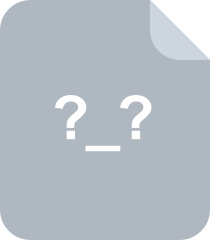
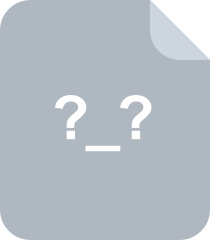
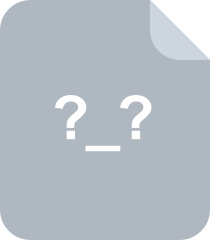
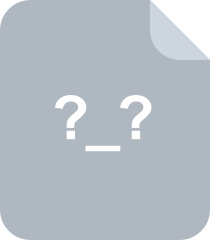
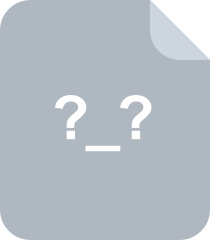
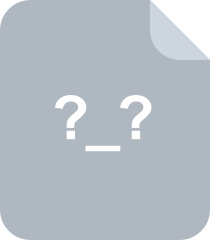
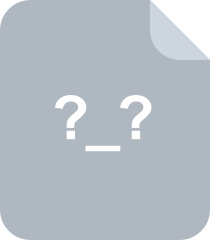
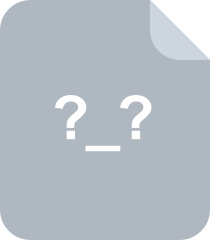
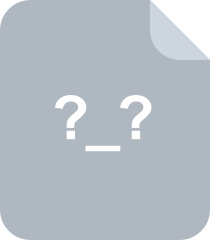
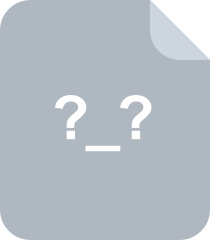
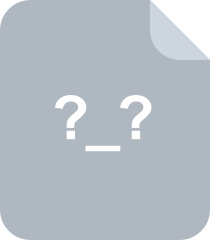
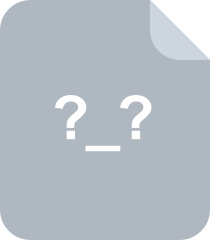
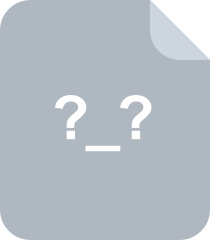
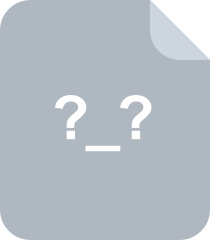
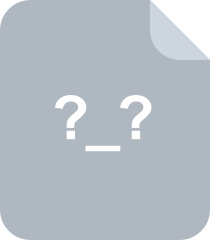
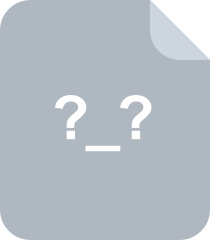
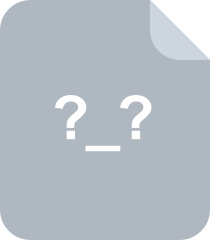
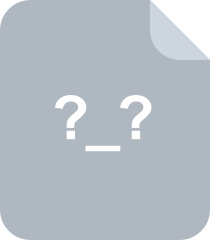
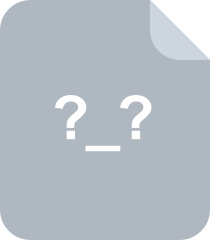
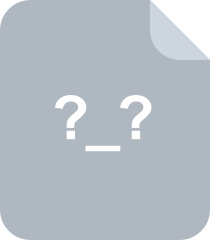
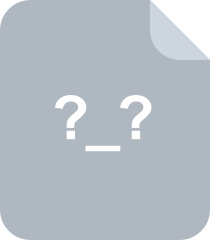
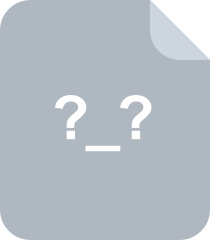
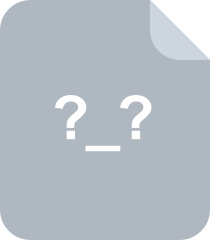
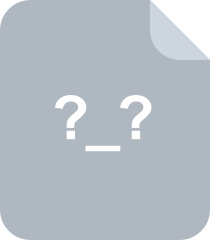
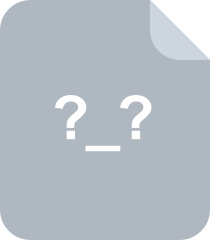
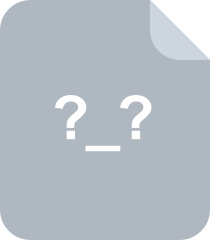
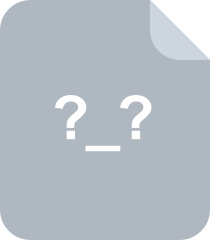
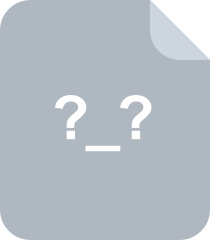
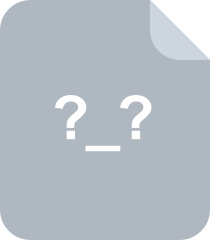
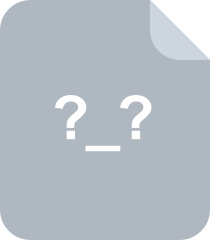
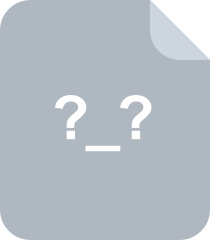
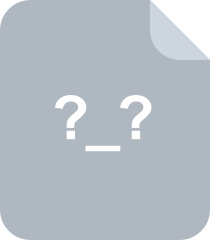
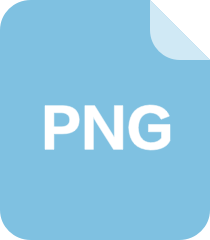
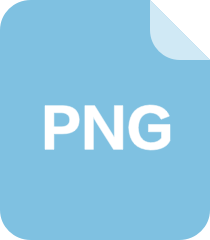
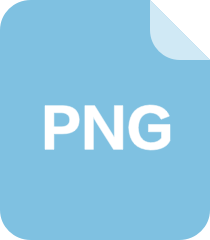
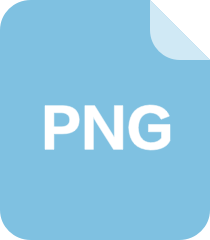
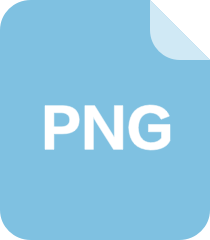
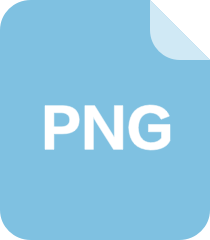
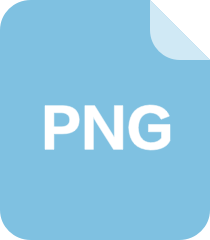
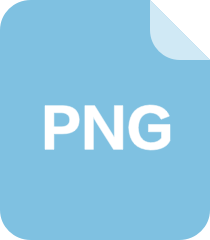
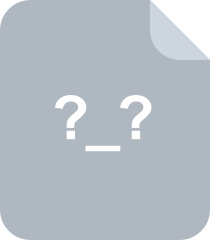
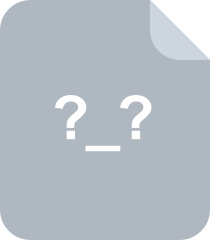
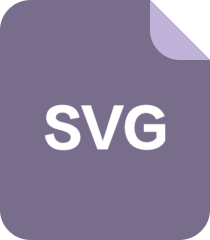
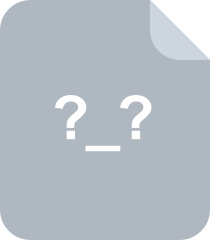
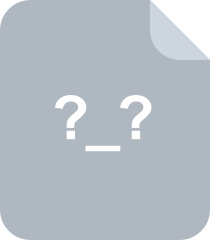
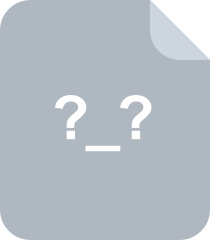
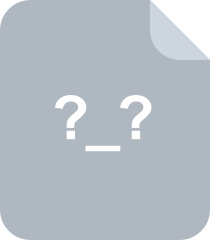
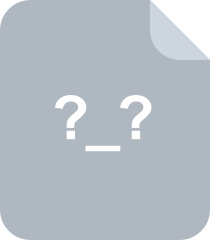
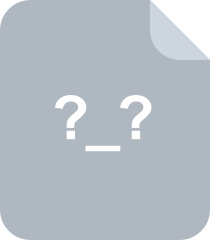
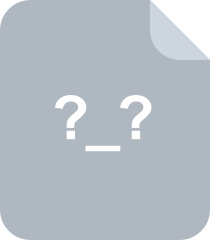
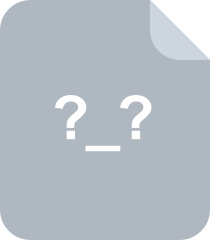
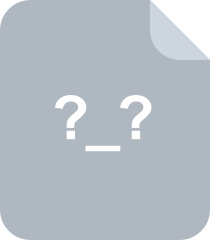
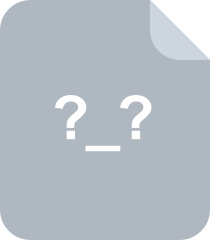
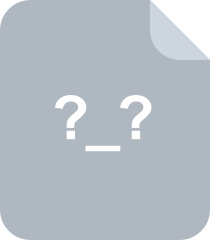
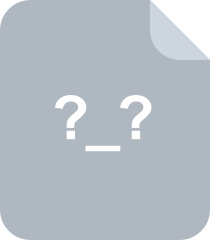
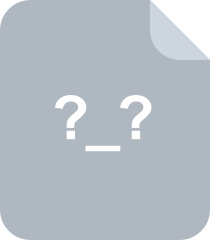
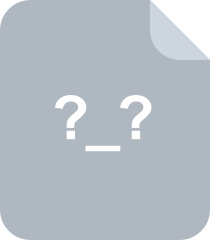
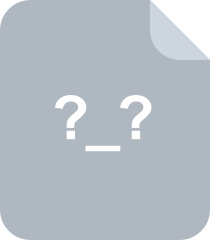
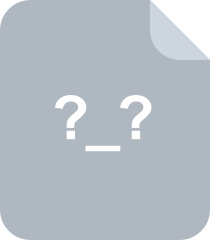
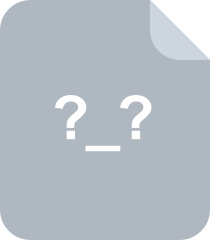
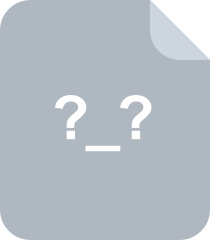
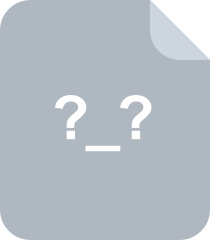
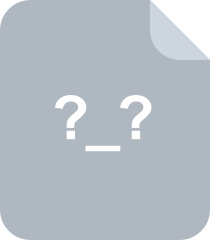
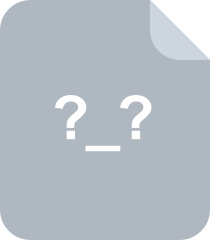
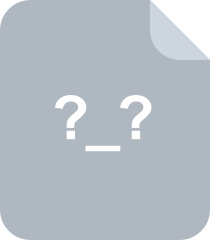
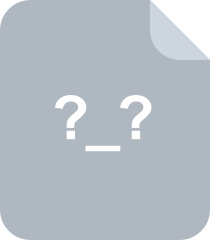
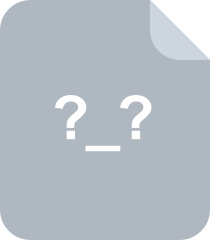
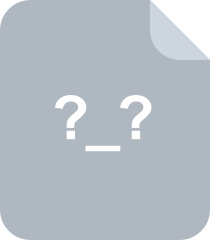
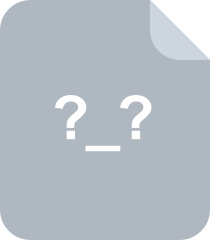
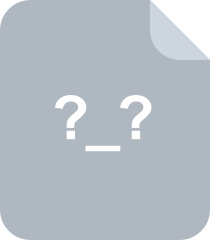
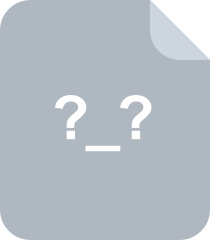
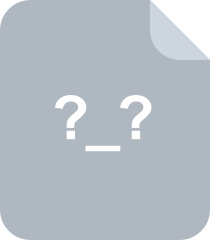
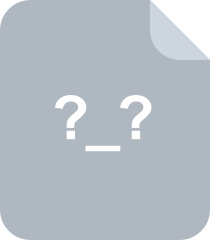
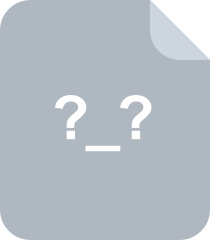
共 194 条
- 1
- 2
资源评论
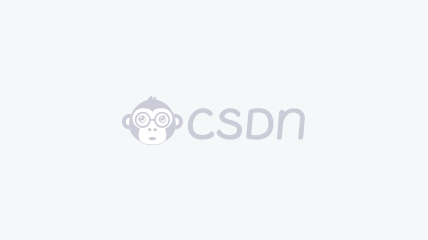

赵闪闪168
- 粉丝: 1679
- 资源: 5392
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

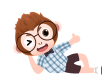
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


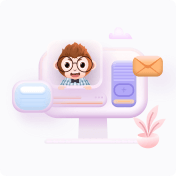
安全验证
文档复制为VIP权益,开通VIP直接复制
