# vuex-persistedstate
Persist and rehydrate your [Vuex](http://vuex.vuejs.org/) state between page reloads.
<hr />
> ð¨ Not maintained anymore! As I don't use Vue in my day to day work, it becomes very hard to stay up to date with any changes with things like Vuex, Nuxt.js and other tools used by the community. That's why I decided to stop spending my spare time to this repository. Feel free to reach out if you would like to take over ownership of the package on NPM. Thank you for any contribution any of you had made to this project ð.
<hr />
[](https://github.com/robinvdvleuten/vuex-persistedstate/actions?query=workflow%3Atest)
[](https://www.npmjs.com/package/vuex-persistedstate)
[](https://www.npmjs.com/package/vuex-persistedstate)
[](https://github.com/prettier/prettier)
[](https://github.com/robinvdvleuten/vuex-persistedstate/blob/master/LICENSE)
[](http://makeapullrequest.com)
[](https://github.com/robinvdvleuten/vuex-persistedstate/blob/master/.github/CODE_OF_CONDUCT.md)
<a href="https://webstronauts.com/">
<img src="https://webstronauts.com/badges/sponsored-by-webstronauts.svg" alt="Sponsored by The Webstronauts" width="200" height="65">
</a>
## Install
```bash
npm install --save vuex-persistedstate
```
The [UMD](https://github.com/umdjs/umd) build is also available on [unpkg](https://unpkg.com):
```html
<script src="https://unpkg.com/vuex-persistedstate/dist/vuex-persistedstate.umd.js"></script>
```
You can find the library on `window.createPersistedState`.
## Usage
```js
import { createStore } from "vuex";
import createPersistedState from "vuex-persistedstate";
const store = createStore({
// ...
plugins: [createPersistedState()],
});
```
For usage with for Vuex 3 and Vue 2, please see [3.x.x branch](https://github.com/robinvdvleuten/vuex-persistedstate/tree/3.x.x).
## Examples
Check out a basic example on [CodeSandbox](https://codesandbox.io).
[](https://codesandbox.io/s/80k4m2598)
Or configured to use with [js-cookie](https://github.com/js-cookie/js-cookie).
[](https://codesandbox.io/s/xl356qvvkz)
Or configured to use with [secure-ls](https://github.com/softvar/secure-ls)
[](https://codesandbox.io/s/vuex-persistedstate-with-secure-ls-encrypted-data-7l9wb?fontsize=14)
### Example with Vuex modules
New plugin instances can be created in separate files, but must be imported and added to plugins object in the main Vuex file.
```js
/* module.js */
export const dataStore = {
state: {
data: []
}
}
/* store.js */
import { dataStore } from './module'
const dataState = createPersistedState({
paths: ['data']
})
export new Vuex.Store({
modules: {
dataStore
},
plugins: [dataState]
})
```
### Example with Nuxt.js
It is possible to use vuex-persistedstate with Nuxt.js. It must be included as a NuxtJS plugin:
#### With local storage (client-side only)
```javascript
// nuxt.config.js
...
/*
* Naming your plugin 'xxx.client.js' will make it execute only on the client-side.
* https://nuxtjs.org/guide/plugins/#name-conventional-plugin
*/
plugins: [{ src: '~/plugins/persistedState.client.js' }]
...
```
```javascript
// ~/plugins/persistedState.client.js
import createPersistedState from 'vuex-persistedstate'
export default ({store}) => {
createPersistedState({
key: 'yourkey',
paths: [...]
...
})(store)
}
```
#### Using cookies (universal client + server-side)
Add `cookie` and `js-cookie`:
`npm install --save cookie js-cookie`
or `yarn add cookie js-cookie`
```javascript
// nuxt.config.js
...
plugins: [{ src: '~/plugins/persistedState.js'}]
...
```
```javascript
// ~/plugins/persistedState.js
import createPersistedState from 'vuex-persistedstate';
import * as Cookies from 'js-cookie';
import cookie from 'cookie';
export default ({ store, req }) => {
createPersistedState({
paths: [...],
storage: {
getItem: (key) => {
// See https://nuxtjs.org/guide/plugins/#using-process-flags
if (process.server) {
const parsedCookies = cookie.parse(req.headers.cookie);
return parsedCookies[key];
} else {
return Cookies.get(key);
}
},
// Please see https://github.com/js-cookie/js-cookie#json, on how to handle JSON.
setItem: (key, value) =>
Cookies.set(key, value, { expires: 365, secure: false }),
removeItem: key => Cookies.remove(key)
}
})(store);
};
```
## API
### `createPersistedState([options])`
Creates a new instance of the plugin with the given options. The following options
can be provided to configure the plugin for your specific needs:
- `key <String>`: The key to store the persisted state under. Defaults to `vuex`.
- `paths <Array>`: An array of any paths to partially persist the state. If no paths are given, the complete state is persisted. If an empty array is given, no state is persisted. Paths must be specified using dot notation. If using modules, include the module name. eg: "auth.user" Defaults to `undefined`.
- `reducer <Function>`: A function that will be called to reduce the state to persist based on the given paths. Defaults to include the values.
- `subscriber <Function>`: A function called to setup mutation subscription. Defaults to `store => handler => store.subscribe(handler)`.
- `storage <Object>`: Instead of (or in combination with) `getState` and `setState`. Defaults to localStorage.
- `getState <Function>`: A function that will be called to rehydrate a previously persisted state. Defaults to using `storage`.
- `setState <Function>`: A function that will be called to persist the given state. Defaults to using `storage`.
- `filter <Function>`: A function that will be called to filter any mutations which will trigger `setState` on storage eventually. Defaults to `() => true`.
- `overwrite <Boolean>`: When rehydrating, whether to overwrite the existing state with the output from `getState` directly, instead of merging the two objects with `deepmerge`. Defaults to `false`.
- `arrayMerger <Function>`: A function for merging arrays when rehydrating state. Defaults to `function (store, saved) { return saved }` (saved state replaces supplied state).
- `rehydrated <Function>`: A function that will be called when the rehydration is finished. Useful when you are using Nuxt.js, which the rehydration of the persisted state happens asynchronously. Defaults to `store => {}`
- `fetchBeforeUse <Boolean>`: A boolean indicating if the state should be fetched from storage before the plugin is used. Defaults to `false`.
- `assertStorage <Function>`: An overridable function to ensure storage is available, fired on plugins's initialization. Default one is performing a Write-Delete operation on the given Storage instance. Note, default behaviour could throw an error (like `DOMException: QuotaExceededError`).
## Customize Storage
If it's not ideal to have the state of the Vuex store inside localstorage. One can easily implement the functionality to use [cookies](https://github.com/js-cookie/js-cookie) for that
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
vuex-持久状态在页面重新加载之间保留并补充Vuex状态。 不再维护!由于我日常工作中不使用 Vue,因此很难及时了解 Vuex、Nuxt.js 和社区使用的其他工具的任何变化。这就是为什么我决定不再将业余时间花在这个存储库上。如果您想接管 NPM 上的软件包的所有权,请随时与我们联系。感谢您对这个项目做出的任何贡献。 安装npm install --save vuex-persistedstateUMD版本也可以通过unpkg获得<script src="https://unpkg.com/vuex-persistedstate/dist/vuex-persistedstate.umd.js"></script>您可以在 上找到该图书馆window.createPersistedState。用法import { createStore } from "vuex";import createPersistedState from "vuex-persistedstate";const store = createStore({
资源推荐
资源详情
资源评论
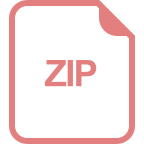
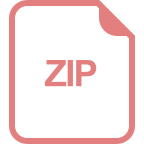
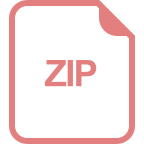
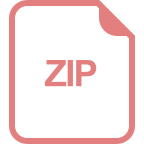
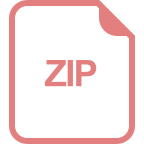
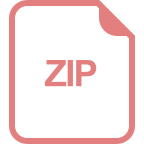
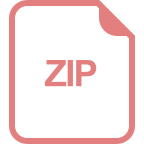
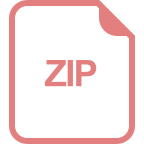
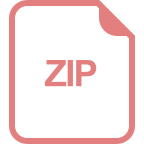
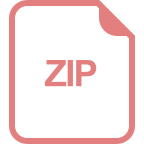
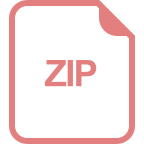
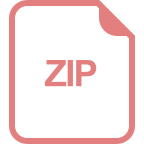
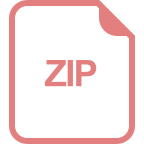
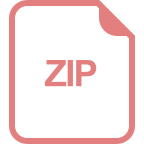
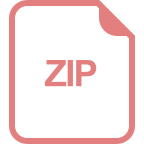
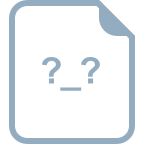
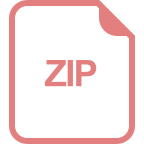
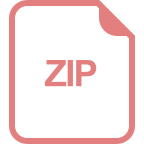
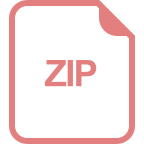
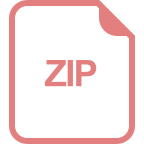
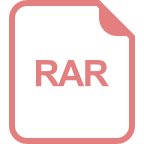
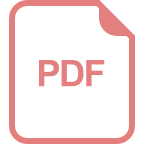
收起资源包目录


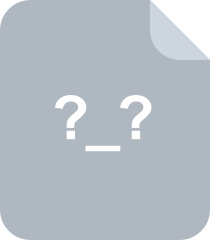
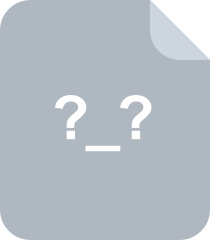
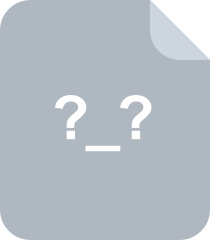

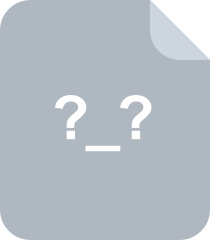
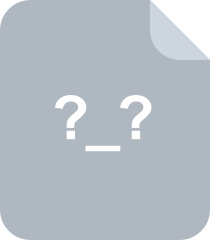
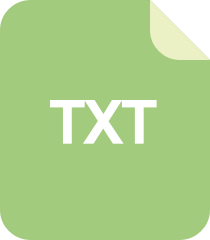

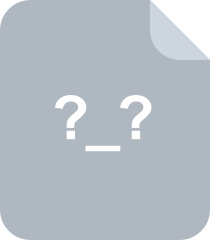
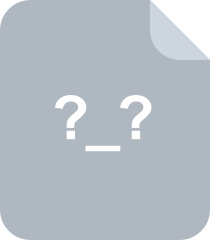
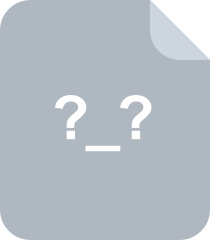
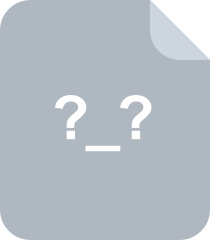
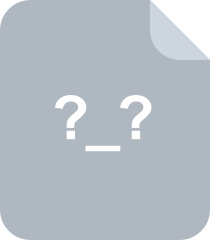
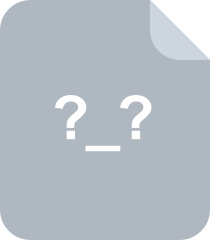
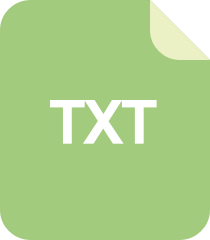
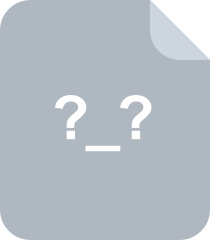
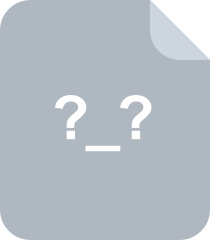
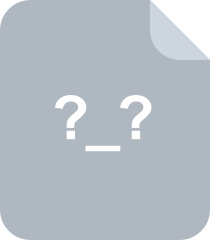
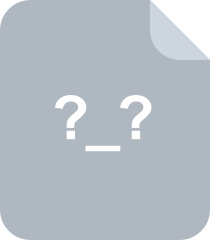
共 17 条
- 1
资源评论
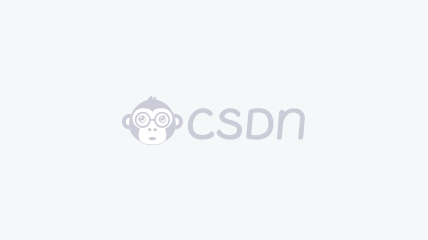

赵闪闪168
- 粉丝: 1635
- 资源: 4856
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

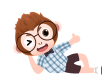
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


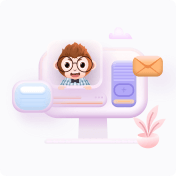
安全验证
文档复制为VIP权益,开通VIP直接复制
