This repo is deprecated, it was created in the days before Vue shipped with TypeScript out of the box. Now the
best path to get started is through [the official CLI](https://cli.vuejs.org). We'll keep this repo around as
a useful archive.
---
# TypeScript Vue Starter
This quick start guide will teach you how to get [TypeScript](http://www.typescriptlang.org/) and [Vue](https://vuejs.org) working together.
This guide is flexible enough that any steps here can be used to integrate TypeScript into an existing Vue project.
# Before you begin
If you're new to Typescript and Vue, here are few resources to get you up and running:
## TypeScript
* [Up and Running with TypeScript](https://egghead.io/courses/up-and-running-with-typescript)
* [TypeScript 5 Minute Tutorial](https://www.typescriptlang.org/docs/handbook/typescript-in-5-minutes.html)
* [Documentation](http://www.typescriptlang.org/docs/home.html)
* [TypeScript GitHub](https://github.com/Microsoft/TypeScript)
## Vue
* [Vuejs Guide](https://vuejs.org/v2/guide/)
* [Vuejs Tutorial](https://www.youtube.com/playlist?list=PL4cUxeGkcC9gQcYgjhBoeQH7wiAyZNrYa)
* [Build an App with Vue.js](https://scotch.io/tutorials/build-an-app-with-vue-js-a-lightweight-alternative-to-angularjs)
# Initialize your project
Let's create a new package.
```sh
mkdir typescript-vue-tutorial
cd typescript-vue-tutorial
```
Next, we'll scaffold our project in the following way:
```txt
typescript-vue-tutorial/
├─ dist/
└─ src/
└─ components/
```
TypeScript files will start out in your `src` folder, run through the TypeScript compiler, then webpack, and end up in a `bundle.js` file in `dist`.
Any components that we write will go in the `src/components` folder.
Let's scaffold this out:
```shell
mkdir src
cd src
mkdir components
cd ..
```
Webpack will eventually generate the `dist` directory for us.
# Initialize the project
Now we'll turn this folder into an npm package.
```shell
npm init
```
You'll be given a series of prompts.
You can use the defaults except for your entry point.
You can always go back and change these in the `package.json` file that's been generated for you.
# Install our dependencies
Ensure TypeScript, Webpack, Vue and the necessary loaders are installed.
```sh
npm install --save-dev typescript webpack webpack-cli ts-loader css-loader vue vue-loader vue-template-compiler
```
Webpack is a tool that will bundle your code and optionally all of its dependencies into a single `.js` file.
While you don't need to use a bundler like Webpack or Browserify, these tools will allow us to use `.vue` files which we'll cover in a bit.
We didn't need to [add `.d.ts` files](https://www.typescriptlang.org/docs/handbook/declaration-files/consumption.html), but if we were using a package which didn't ship declaration files, we'd need to install the appropriate `@types/` package.
[Read more about using definition files in our documentation](https://www.typescriptlang.org/docs/handbook/declaration-files/consumption.html).
# Add a TypeScript configuration file
You'll want to bring your TypeScript files together - both the code you'll be writing as well as any necessary declaration files.
To do this, you'll need to create a `tsconfig.json` which contains a list of your input files as well as all your compilation settings.
Simply create a new file in your project root named `tsconfig.json` and fill it with the following contents:
You can easily create `tsconfig.json` this command.
```
tsc --init
```
```json
{
"compilerOptions": {
"outDir": "./built/",
"sourceMap": true,
"strict": true,
"noImplicitReturns": true,
"module": "es2015",
"moduleResolution": "node",
"target": "es5"
},
"include": [
"./src/**/*"
]
}
```
Notice the `strict` flag is set to true.
At the very least, TypeScript's `noImplicitThis` flag will need to be turned on to leverage Vue's declaration files, but `strict` gives us that and more (like `noImplicitAny` and `strictNullChecks`).
We strongly recommend using TypeScript's stricter options for a better experience.
# Adding Webpack
We'll need to add a `webpack.config.js` to bundle our app.
```js
var path = require('path')
var webpack = require('webpack')
const VueLoaderPlugin = require('vue-loader/lib/plugin')
module.exports = {
entry: './src/index.ts',
output: {
path: path.resolve(__dirname, './dist'),
publicPath: '/dist/',
filename: 'build.js'
},
module: {
rules: [
{
test: /\.vue$/,
loader: 'vue-loader',
options: {
loaders: {
// Since sass-loader (weirdly) has SCSS as its default parse mode, we map
// the "scss" and "sass" values for the lang attribute to the right configs here.
// other preprocessors should work out of the box, no loader config like this necessary.
'scss': 'vue-style-loader!css-loader!sass-loader',
'sass': 'vue-style-loader!css-loader!sass-loader?indentedSyntax',
}
// other vue-loader options go here
}
},
{
test: /\.tsx?$/,
loader: 'ts-loader',
exclude: /node_modules/,
options: {
appendTsSuffixTo: [/\.vue$/],
}
},
{
test: /\.(png|jpg|gif|svg)$/,
loader: 'file-loader',
options: {
name: '[name].[ext]?[hash]'
}
},
{
test: /\.css$/,
use: [
'vue-style-loader',
'css-loader'
]
}
]
},
resolve: {
extensions: ['.ts', '.js', '.vue', '.json'],
alias: {
'vue$': 'vue/dist/vue.esm.js'
}
},
devServer: {
historyApiFallback: true,
noInfo: true
},
performance: {
hints: false
},
devtool: '#eval-source-map',
plugins: [
// make sure to include the plugin for the magic
new VueLoaderPlugin()
]
}
if (process.env.NODE_ENV === 'production') {
module.exports.devtool = '#source-map'
// http://vue-loader.vuejs.org/en/workflow/production.html
module.exports.plugins = (module.exports.plugins || []).concat([
new webpack.DefinePlugin({
'process.env': {
NODE_ENV: '"production"'
}
}),
new webpack.optimize.UglifyJsPlugin({
sourceMap: true,
compress: {
warnings: false
}
}),
new webpack.LoaderOptionsPlugin({
minimize: true
})
])
}
```
# Add a build script
Open up your `package.json` and add a script named `build` to run Webpack.
Your `"scripts"` field should look something like this:
```json
"scripts": {
"build": "webpack",
"test": "echo \"Error: no test specified\" && exit 1"
},
```
Once we add an entry point, we'll be able to build by running
```sh
npm run build
```
and have builds get triggered on changes by running
```sh
npm run build -- --watch
```
# Create a basic project
Let's create the most bare-bones Vue & TypeScript example that we can try out.
First, create the file `./src/index.ts`:
```ts
// src/index.ts
import Vue from "vue";
let v = new Vue({
el: "#app",
template: `
<div>
<div>Hello {{name}}!</div>
Name: <input v-model="name" type="text">
</div>`,
data: {
name: "World"
}
});
```
Let's check to see if everything is wired up correctly.
Create an `index.html` with the following content at your root:
```html
<!doctype html>
<html>
<head></head>
<body>
<div id="app"></div>
</body>
<script src="./dist/build.js"></script>
</html>
```
Now run `npm run build` and open up your `index.html` file in a browser.
You should see some text that says `Hello World!`.
Below that,
没有合适的资源?快使用搜索试试~ 我知道了~
TypeScript 和 Vue 的入门模板,带有详细的 README,描述了如何将两者结合使用 .zip
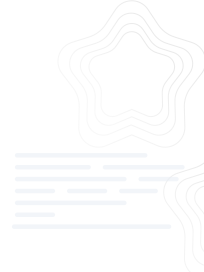
共17个文件
json:4个
ts:3个
vue:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 46 浏览量
2024-12-01
08:52:03
上传
评论
收藏 95KB ZIP 举报
温馨提示
TypeScript 和 Vue 的入门模板,带有详细的 README,描述了如何将两者结合使用。此 repo 已弃用,它是在 Vue 附带 TypeScript 之前创建的。现在开始的最佳途径是通过官方 CLI。我们将保留此 repo 作为有用的存档。TypeScript Vue Starter本快速入门指南将教您如何让TypeScript和Vue协同工作。本指南非常灵活,可以使用此处的任何步骤将 TypeScript 集成到现有的 Vue 项目中。开始之前如果你是 Typescript 和 Vue 的新手,以下是一些可帮助你入门和运行的资源TypeScript使用 TypeScript 启动并运行TypeScript 5 分钟教程文档TypeScript GitHubVueVuejs 指南Vuejs 教程使用 Vue.js 构建应用程序初始化项目让我们创建一个新的包。mkdir typescript-vue-tutorialcd typescript-vue-tutorial接下来,我们将按照以下方式搭建我们的项目types
资源推荐
资源详情
资源评论
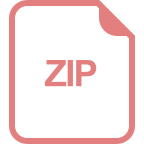
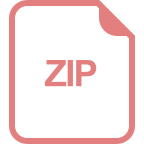
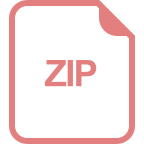
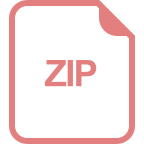
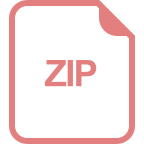
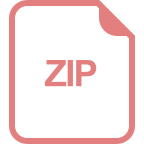
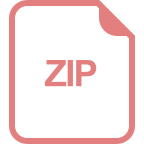
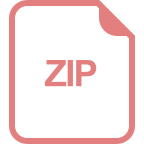
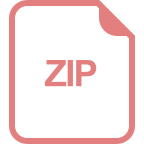
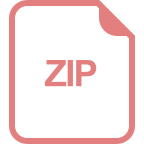
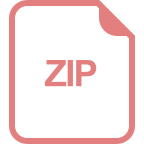
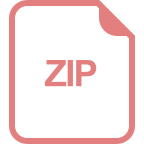
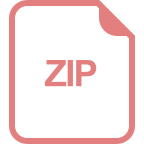
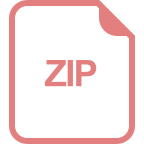
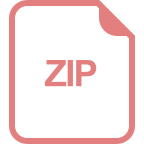
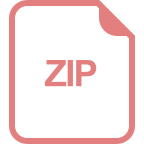
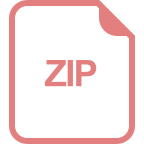
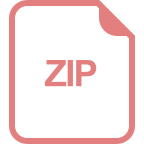
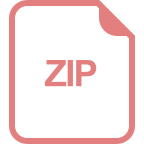
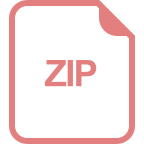
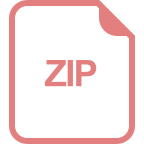
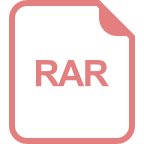
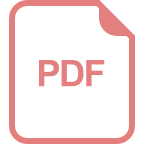
收起资源包目录

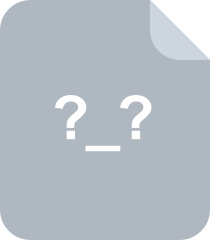

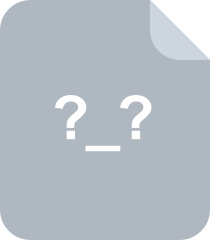
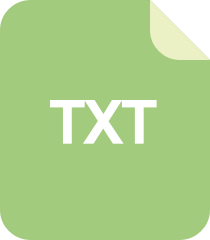
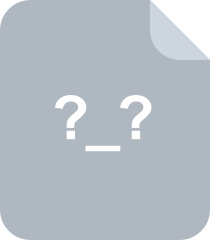


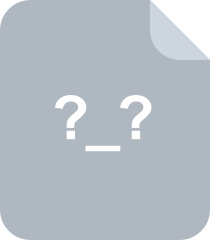
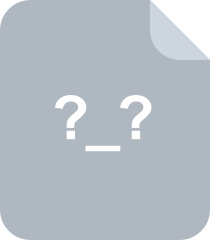
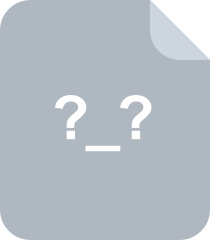
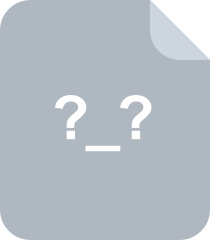
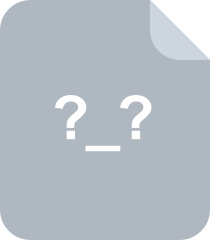
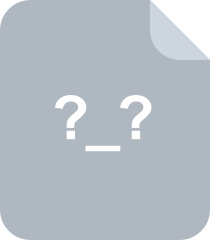
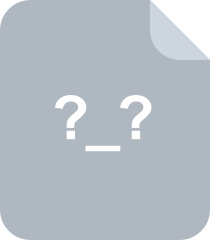
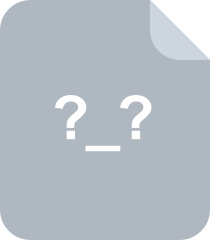
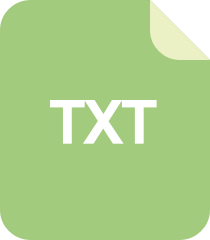
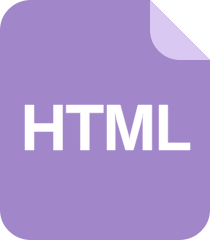
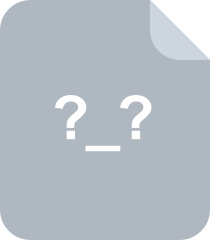
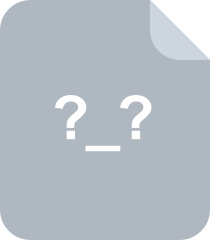
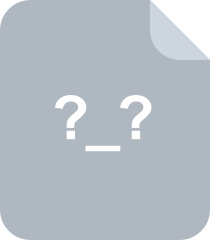
共 17 条
- 1
资源评论
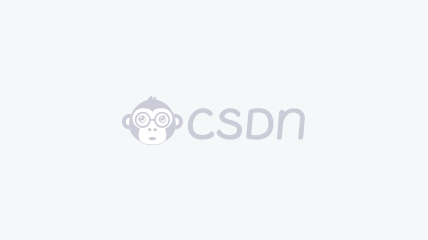

赵闪闪168
- 粉丝: 1633
- 资源: 4239
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

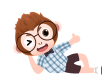
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


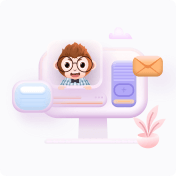
安全验证
文档复制为VIP权益,开通VIP直接复制
