# PPE Detection using yolo3 and DeepSORT
## Introduction
In Industry, specially manufacturing industry, Personal Protective Equipment (PPE) like helmet (hard-hat), safety-harness, goggles etc play a very important role in ensuring the safety of workers. However, many accidents still occur, due to the negligence of the workers as well as their supervisors. Supervisors can make mistakes due to the fact that such tasks are monotonous and they may not be able to monitor consistently. This project aims to utilize existing CCTV camera infrastructure to assist supervisors to monitor workers effectively by providing them with real time alerts.
## Functioning
* Input is taken from CCTV cameras
* YOLO is used for detecting persons with proper PPE and those without PPE.
* Deep_SORT allocates unique ids to detected persons and tracks them through consecutive frames of the video.
* An alert is raised if a person is found to be without proper PPE for more than some set duration, say 5 seconds.

It detects persons without helmet and displays the number of persons with helmet and
those without helmet. It sends notification in the message box for each camera. There is global
message box, where alerts from all cameras are displayed.

It detects that the same person about which it had warned earlier has now worn a
helmet and notifies that also.

Please note that this is still a work under progress and new ideas and contributions are welcome.
* Currently, the model is trained to detect helmets (hard-hat) only. I have plans to train the model for other PPEs as well.
* Currently, only usb cameras are supported. Support for other cameras needs to be added.
* The tracker needs to be made robust.
* Integrate service (via mobile app or SMS) to send real-time notifications to supervisors present on the field.
## Quick Start
Using conda environment is recommended. Follow these steps to get the code running:
1. First, download the zip file.
2. Download the following files into the project directory:
[mars-small128.pb](https://1drv.ms/u/c/024d7625f12b47b2/QbJHK_Eldk0ggAL4AQAAAAAA8rfjUd8TxK6_-Q)
[full_yolo3_helmet_and_person.h5](https://1drv.ms/u/c/024d7625f12b47b2/QbJHK_Eldk0ggAL3AQAAAAAAExKZFaGcssUM5Q)
3. Run the following command to create a conda environmnet:
```bash
conda env create -f environment.yml
```
Alternatively,
```bash
conda create --name helmet-detection --file requirements.txt
```
4. Activate the conda environment:
```bash
conda activate helmet-detection
```
5. To run the code with gui :
```bash
python predict_gui.py -c config.json -n <number of cameras>
```
Note that the gui supports only upto 2 cameras.
To run the code without gui :
```bash
python predict.py -c config.json -n <number of cameras>
```
Here you can enter any number of cameras you want to use.
## Training the model
### 1. Data preparation
**Data Collection**
The dataset containing images of people wearing helmets and people without helmets were collected mostly from google search. Some images have people applauding, those were collected from Stanford 40 Action Dataset. Download images for training from [train_image_folder](https://drive.google.com/drive/folders/1b5ocFK8Z_plni0JL4gVhs3383V7Q9EYH?usp=sharing).
**Annotations**
Annotaion of each image was done in Pascal VOC format using the awesome lightweight annotation tool [LabelImg](https://github.com/tzutalin/labelImg) for object-detection. Download annotations from [train_annot_folder](https://drive.google.com/drive/folders/1u_s_kxq0x_fqtqgJn9nKC92ikrThMDru?usp=sharing).
**Organize the dataset into 4 folders:**
* train_image_folder <= the folder that contains the train images.
* train_annot_folder <= the folder that contains the train annotations in VOC format.
* valid_image_folder <= the folder that contains the validation images.
* valid_annot_folder <= the folder that contains the validation annotations in VOC format.
There is a one-to-one correspondence by file name between images and annotations. If the validation set is empty, the training set will be automatically splitted into the training set and validation set using the ratio of 0.8.
### 2. Edit the configuration file
The configuration file is a json file, which looks like this:
```
{
"model" : {
"min_input_size": 288,
"max_input_size": 448,
"anchors": [33,34, 52,218, 55,67, 92,306, 96,88, 118,158, 153,347, 209,182, 266,359],
"labels": ["helmet","person with helmet","person without helmet"]
},
"train": {
"train_image_folder": "train_image_folder/",
"train_annot_folder": "train_annot_folder/",
"cache_name": "helmet_train.pkl",
"train_times": 8,
"batch_size": 8,
"learning_rate": 1e-4,
"nb_epochs": 100,
"warmup_epochs": 3,
"ignore_thresh": 0.5,
"gpus": "0,1",
"grid_scales": [1,1,1],
"obj_scale": 5,
"noobj_scale": 1,
"xywh_scale": 1,
"tensorboard_dir": "logs",
"saved_weights_name": "full_yolo3_helmet_and_person.h5",
"debug": true
},
"valid": {
"valid_image_folder": "",
"valid_annot_folder": "",
"cache_name": "",
"valid_times": 1
}
}
```
The model section defines the type of the model to construct as well as other parameters of the model such as the input image size and the list of anchors. The `labels` setting lists the labels to be trained on. Only images, which has labels being listed, are fed to the network. The rest images are simply ignored. By this way, a Dog Detector can easily be trained using VOC or COCO dataset by setting `labels` to `['dog']`.
Download pretrained weights for backend at:
[backend.h5](https://1drv.ms/u/c/024d7625f12b47b2/QbJHK_Eldk0ggAL5AQAAAAAA1JJB2XEu27RBmw)
**These weights must be put in the root folder of the repository. They are the pretrained weights for the backend only and will be loaded during model creation. The code does not work without these weights.**
### 3. Generate anchors for your dataset (optional)
`python gen_anchors.py -c config.json`
Copy the generated anchors printed on the terminal to the `anchors` setting in `config.json`.
### 4. Start the training process
`python train.py -c config.json`
By the end of this process, the code will write the weights of the best model to file best_weights.h5 (or whatever name specified in the setting "saved_weights_name" in the config.json file). The training process stops when the loss on the validation set is not improved in 3 consecutive epoches.
### 5. Perform detection using trained weights on live feed from webcam
To run the code with gui :
```bash
python predict_gui.py -c config.json -n <number of cameras>
```
Note that the gui supports only upto 2 cameras.
To run the code without gui :
```bash
python predict.py -c config.json -n <number of cameras>
```
Here you can enter any number of cameras you want to use.
## Acknowledgements
* [rekon/keras-yolo2](https://github.com/rekon/keras-yolo2) for training data.
* [experiencor/keras-yolo3](https://github.com/experiencor/keras-yolo3) for YOLO v3 implementation.
* [nwojke/deep_sort](https://github.com/nwojke/deep_sort) for Deep_SORT implementation.

赵闪闪168
- 粉丝: 1726
- 资源: 6943
最新资源
- 信息融合与状态估计 主要是针对多传感器多时滞(包括状态之后和观测滞后)系统,带有色噪声多重时滞传感网络系统的序列协方差交叉融合Kalman滤波器 将带有色噪声的系统转化为带相关噪声的系统,然后再进行
- EMplanner注释版本matlab代码,可刀 该算法使用dp动态规划进行了轨迹规划,过程中未向apollo的EM规划器一样还使用了QP进行了轨迹规划,整个大包
- qt tcp udp socket 通信 实现文字、图片、文件、语音和实时对讲 包括客户端和服务器端,带有报告,所有功能支持客户端和服务器端双向通信 自己开发,非转卖 物品可复制,联系不 ,介
- 240201118王辰辰实验4.pdf
- 永磁同步电机的全速度范围无传感器矢量控制:脉振高频注入(方波注入)切到改进SMO 低速段采用HFI脉振高频注入启动,中高速段采用基于转子磁链模型的SMO,切方法为加权系数 改进的SMO不使用低通滤
- 240201118王辰辰实验6.pdf
- PWM控制半桥全桥LLC谐振变器 仿真包括开环和闭环,可实现软开关,波形如下 matlab simulink模型
- LoRA原理详解,深入理解Lora原理,以及案例实战
- 汇川中型plc+纯ST语言双轴同步设备,程序中没有使用任何库文件,纯原生codesys功能块 非常适合初学入门者,三个驱动模拟虚主轴和两个伺服从轴,只要手里有汇川AM400,600,AC700,80
- 数据结构(c实现--vs2013).rar
- win11打印机共享修复签名认证
- 一个项目计划+每日工作任务记录的模板:
- 中间顶升流道输送机(sw16可编辑+工程图+bom)全套技术资料100%好用.zip
- 载具自动翻转机(sw16可编辑+cad+bom)全套技术资料100%好用.zip
- 自动称重AI码垛机x_t全套技术资料100%好用.zip
- 自动滚轮柑橘剥皮机sw16可编辑全套技术资料100%好用.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


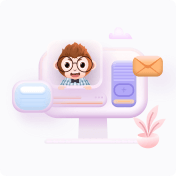