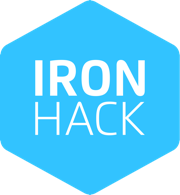
# LAB | JS Clue (mixing objects and arrays)
<details>
<summary>
<h2>Learning Goals</h2>
</summary>
This exercise allows you to practice and apply the concepts and techniques taught in class.
Upon completion of this exercise, you will be able to:
- Run predefined tests in Jasmine to verify that the program meets the technical requirements.
- Identify expected code behavior by reading and understanding test results and errors.
- Create objects using the object literal syntax
- Declare and invoke functions
- Use the `return` keyword to return a value from a function.
- Pass arrays to functions as arguments.
- Access objects stored in arrays,
- Access object properties using the dot notation and bracket notation
- Iterate over arrays using the `for` and `forEach` loops.
<br>
<hr>
</details>
## Introduction
Do you remember the classic detective board game Clue? It was a very popular game in the 90s.
The plot starts with the death of Mr. Boddy, the very wealthy owner of Tudor Manor. He was killed under _highly suspicious circumstances_, and the player's mission is to uncover which **suspect** murdered him, what **weapon** was used, and in which **room** of the mansion the crime took place.
When playing Clue, these three details are represented by a **suspect** card, a **weapon** card and a **room** card that are kept hidden in an envelope for the duration of the game. By progressively revealing the remaining cards, the players can infer which are the three cards that are hidden. When a player is confident that they know the solution to the mystery, they will try to reveal it. If a player guesses correctly, they win the game.
<p align="center"><img width="400" src="https://i.imgur.com/AZWieq9.jpg=300"/></p>
## Requirements
- Fork this repo
- Clone this repo
<br>
## Submission
- Upon completion, run the following commands:
```sh
git add .
git commit -m "Solved lab"
git push origin master
```
- Create a Pull Request so that your TAs can check your work.
<br>
## Test Your Code
This LAB is equipped with unit tests to provide automated feedback on your lab progress. If you want to check the tests, they are in the `tests/clue.spec.js` file.
To run the tests and your JavaScript code, open the `SpecRunner.html` file using the [Live Server](https://marketplace.visualstudio.com/items?itemName=ritwickdey.LiveServer) VSCode extension.
To see the outputs of the `console.log` in your JavaScript code, open the [Console in the Developer Tools](https://developer.chrome.com/docs/devtools/open/#console).
<br>
## Instructions
You will work in the `src/clue.js` file, already loaded in the `SpecRunner.html` file.
To run the tests and your JavaScript code, open the `SpecRunner.html` file using the [Live Server](https://marketplace.visualstudio.com/items?itemName=ritwickdey.LiveServer) VSCode extension.
<br>
### Iteration 1: Create the cards
Clue has three different _card types_: **suspects**, **rooms**, and **weapons**.
Let's create an `array` for every _card type_. These arrays should be named `suspectsArray`, `weaponsArray`, `roomsArray`.
Each array will contain _objects_, where each _object_ represents one card.
All of the information you need about **suspects**, **rooms**, and **weapons** can be found on the `DATA.md` file.
_Suggestion_: Copy this data into the `clue.js` file and model it into objects nested inside your cards' arrays. This is a great chance for you to experiment with IDE shortcuts, multiple-line selection, find and replace, and other great features that VS Code provides.
<br>
#### Suspects
All of six possible _suspects_ in Clue have a **first name**, **last name**, **occupation**, **age**, **description**, **image** and **color**.
To complete this iteration, you should have an array that looks something like the following:
```javascript
const suspectsArray = [
{
firstName: 'Jacob',
lastName: 'Green',
occupation: 'Entrepreneur'
// ...
}
// ...
];
```
<br>
#### Weapons
There are a total of nine _weapons_. Each of them has a different **name** and **weight**.
<br>
#### Rooms
The game board represents the blueprints of the mansion and features fifteen different _rooms_. Each room will only have a **name**.
<br>
### Iteration 2: Create the mystery
At the beginning of the game, players shuffle each of the card stacks to create a combination of _suspect_, _weapon_ and _room_. This will be the mystery to solve.
<br>
#### Random selector
Declare a function named `selectRandom` to select one element from a card stack randomly. The function should expect an `array` as an argument and should return a random element from the array.
<br>
#### Create the mystery
Declare a function named `pickMystery` that takes no arguments and returns an object with three properties: _suspect_, _weapon_ and _room_, each holding as a value a card of that specific type. You can get a random card of each type by calling `selectRandom` on each card stack.
<br>
### Iteration 3: Reveal the mystery
At last, we are ready to reveal the mystery.
Declare a function named `revealMystery` that receives an _envelope_ `object` (with the shape of the object returned by `pickMystery`) as the single argument and returns a revealing message in the following format:
**\<FIRST NAME\> \<LAST NAME\> killed Mr. Boddy using the \<WEAPON\> in the \<ROOM\>!**
<br>
## Extra Resources
- [Data Structures: Objects and Arrays](http://eloquentjavascript.net/04_data.html)
- [20 Mind-blowing facts about Cluedo](http://whatculture.com/offbeat/20-mind-blowing-facts-you-didnt-know-about-cluedo)
- [Cluedo - Wikipedia](https://en.wikipedia.org/wiki/Cluedo)
<br>
**Happy coding!** :heart:
<br>
## FAQs
<br>
<details>
<summary>I am stuck in the exercise and don't know how to solve the problem or where to start.</summary>
<br>
If you are stuck in your code and don't know how to solve the problem or where to start, you should take a step back and try to form a clear question about the specific issue you are facing. This will help you narrow down the problem and come up with potential solutions.
For example, is it a concept that you don't understand, or are you receiving an error message that you don't know how to fix? It is usually helpful to try to state the problem as clearly as possible, including any error messages you are receiving. This can help you communicate the issue to others and potentially get help from classmates or online resources.
Once you have a clear understanding of the problem, you will be able to start working toward the solution.
[Back to top](#faqs)
</details>
<details>
<summary>How do I loop over an array?</summary>
<br>
Loops allow you to repeat a block of code a certain number of times. There are several ways to loop over an array in JavaScript:
#### For loop
The `for` loop is the most traditional way to loop through an array in JavaScript. It consists of three parts: the *initialization*, the *condition*, and the *increment/decrement*:
```js
const animals = ['cat', 'dog', 'bird'];
// initialize counter variable (let i = 0)
// set condition (i < animals.length)
// increment counter (i++)
for (let i = 0; i < animals.length; i++) {
console.log(animals[i]);
}
```
The initialization is where you declare a counter variable and set its initial value.
The condition is a boolean expression that is evaluated before each iteration of the loop. If the condition is `true`, the loop will continue. Once the condition turns `false`, the loop will terminate.
The increment/decrement is where you update the counter variable and it happens at the end of each iteration.
The block of code inside the loop is repeated during each iteration.
<br>
#### While loop
The `while` loop is another way to loop through an ar

赵闪闪168
- 粉丝: 1726
- 资源: 6172
最新资源
- 中国水系线(1-5级很细致)
- 基于Golang的高并发三方支付系统设计源码,TypeScript+Vue+HTML全栈实现
- 基于Babylon.js的HTML交互式Web设计源码学习教程
- Pyside6简单进销存教程,有开发书和使用书
- 基于HTML/CSS的大学期末静态网页答辩设计源码
- 基于微信小程序的便捷小区业主决策投票小程序设计源码
- 基于Vue框架的农业电商平台后台管理系统设计源码
- 基于Vue和JavaScript的流动治超管理平台前端设计源码
- 基于Vue和JavaScript的百度地图集成展示设计源码
- 基于Vue 3和TypeScript的B2C电商平台优选集设计源码
- XAPK Installer
- 基于Qt5.14.2的简易Qt天气预报设计源码,新手练手利器
- 基于Docker/Qemu/Bochs的Linux 0.11内核开发环境源码设计
- 无标题重生之我竟然要准备信息检索考试
- 11111111145367451111111
- 人工智能视频数据集crowed-people4
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


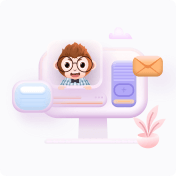