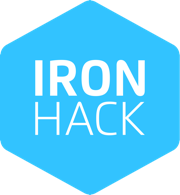
# LAB | JS Functions & Arrays
<details>
<summary>
<h2>Learning Goals</h2>
</summary>
This exercise allows you to practice and apply the concepts and techniques taught in class.
Upon completion of this exercise, you will be able to:
- Run predefined tests in Jasmine to verify that the program meets the technical requirements.
- Identify expected code behavior by reading and understanding test results and errors.
- Declare and invoke functions using function declaration, function expression, and arrow function syntax.
- Use the `return` keyword to return a value from a function.
- Pass primitive values as arguments to functions.
- Pass arrays to functions as arguments.
- Access items stored in arrays using the indexes,
- Add, remove and check for items in an array using the index and array methods (`unshift`, `push`, `splice`, `shift`, `pop`, `indexOf`, and `includes`).
- Iterate over arrays using the `for` and `forEach` loops.
<br>
<hr>
</details>
## Introduction
Array manipulation is a common task in programming. Whether you are calculating a total for a shopping cart, grabbing only the first names from a list of people, or moving a piece on a chessboard, you are probably modifying or manipulating an array somewhere in the code.
<br>
## Requirements
- Fork this repo
- Clone it to your machine
## Submission
- Upon completion, run the following commands:
```bash
git add .
git commit -m "Solved lab"
git push origin master
```
- Create a Pull Request so that your TAs can check your work.
## Automated Testing Introduction
### What is automated testing?
Automated software testing is the process of programmatically executing an application to validate and verify that it meets the business needs, as well as the technical requirements, and that it behaves as expected.
Testing should be viewed as a continuous process, not a discrete operation or single activity in the development lifecycle. Designing tests at the beginning of the product lifecycle can mitigate common issues that arise when developing complex code bases.
Having a strong *test suite* can provide you the ease of mind since you will be able to confidently improve upon your work while knowing that your not breaking a previously developed feature.
<br>
### Testing labs
This LAB and some labs you will work on during the bootcamp are equipped with unit tests to provide automated feedback on your lab progress.
<br>
### Testing with Jasmine
Jasmine is an automated testing framework for JavaScript. It is designed to be used in Behavior-driven Development (**BDD**) programming, focusing more on the business value than the technical details.
We have already included Jasmine in the project you just forked, so let's see how to use it to implement our code.
<br>
### Usage
Before starting coding, we will explain the project structure we have provided you:
```
lab-js-functions-and-arrays
├── README.md
├── SpecRunner.html
├── jasmine
│ └── ...
├── src
│ └── functions-and-arrays.js
└── tests
└── functions-and-arrays.spec.js
```
We will be working with the `src/functions-and-arrays.js`. You can find all the files in the `jasmine` folder needed to use Jasmine. All these files are already linked with the `SpecRunner.html` file.
If you want to check the tests, they are in the `tests/functions-and-arrays.spec.js` file.
#### Run tests
Running automated tests with Jasmine is super easy. All you need to do is open the `SpecRunner.html` file in your browser. You will find something similar to this:
[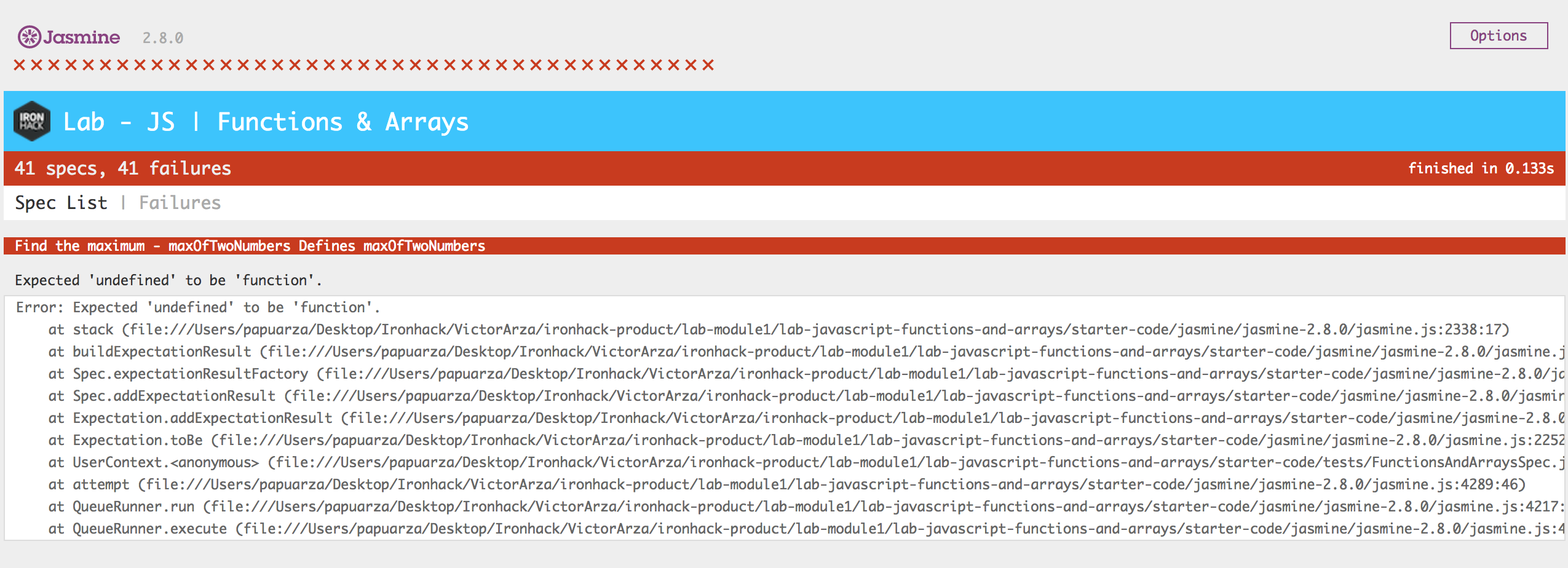](https://user-images.githubusercontent.com/23629340/33389609-c2f3965c-d533-11e7-9a03-e0a89314dd98.png)
#### Pass the tests
You should write your code on the `src/functions-and-arrays.js` file. While following the instructions for each iteration, you should check every test and ensure it's *passing*, before moving on.
Do not rush. You should take your time to read every iteration carefully and address the *breaking* tests as you progress through the exercise.
When coding with tests, it is super important that you carefully read and understand the errors you are getting. This way, you will know what's expected from your code.
To see the output of your JavaScript code, open the [Console in the Developer Tools](https://developer.chrome.com/docs/devtools/open/#console).
**Important:** Note that **you don't need to execute the functions yourself**; the tests will automatically load and execute the functions on each test run. All you need to do is declare the functions, ensure they handle the parameters passed and return what is indicated in the iteration instructions and the test description. We provide you with a sample array for some iterations, so you can do some **manual** testing if you wish.
## Instructions
While following the instructions for each iteration, carefully read the instructions and test descriptions to understand the task requirements fully. Do not rush. It would be best if you took your time to read every iteration carefully.
<br>
### Iteration #1: Find the maximum
Implement the function `maxOfTwoNumbers` that takes two numbers as arguments and returns the bigger number.
<br>
### Iteration #2: Find the longest word
Implement the function `findLongestWord` that takes as an argument an array of words and returns the longest one. If there are 2 with the same length, it should return the first occurrence.
You can use the following array to test your solution:
```javascript
const words = ['mystery', 'brother', 'aviator', 'crocodile', 'pearl', 'orchard', 'crackpot'];
```
<br>
### Iteration #3: Calculate the sum
#### Iteration #3.1: Sum numbers
Calculating a sum can be as simple as iterating over an array and adding each of the elements together.
Implement the function named `sumNumbers` that takes an array of numbers as an argument and returns the sum of all the numbers in the array. Later in the course, we will learn how to do this using the `reduce` array method, making your work significantly easier. For now, let's practice _the "declarative"_ way of adding values using loops.
You can use the following array to test your solution:
```javascript
const numbers = [6, 12, 1, 18, 13, 16, 2, 1, 8, 10];
```
<br>
#### Bonus - Iteration #3.2: A generic `sum()` function
In iteration 3, you created a function that returns the sum of an array of numbers. But what if we want to calculate the sum of the length of words in an array? What if it also includes _boolean_ values? To achieve this, we must create a function allowing this flexibility.
You should implement the function `sum()` in this iteration. The function should take an array of mixed values - numbers, strings, and booleans. The function should add all the string lengths, numeric values, and numeric values of booleans to the total sum and return the sum.
You can use the following array to test your solution:
```javascript
const mixedArr = [6, 12, 'miami', 1, true, 'barca', '200', 'lisboa', 8, 10];
// should return: 57
```
Note: Your function should only accept an array with numbers, strings, or booleans. If the array contains any other data type, such as an object, you should [throw an error](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/throw). In JavaScript, the syntax for throwing an error is as follows:
```javascript
throw new Error("Error message goes here");
```
When specifying the error message, you should be specific and descriptive in explaining the error.
<br>
### Iteration #4: Calculate the average
Calculating an average is a prevalent task. So let's practice it a bit.
**The logic behind this:**
1. Find the sum
没有合适的资源?快使用搜索试试~ 我知道了~
应用数组迭代和数组操作技术的练习.zip
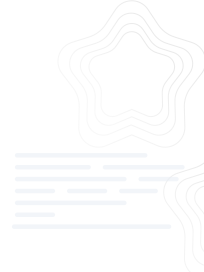
共15个文件
js:6个
txt:2个
png:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 29 浏览量
2024-11-25
03:41:59
上传
评论
收藏 64KB ZIP 举报
温馨提示
应用数组迭代和数组操作技术的练习LAB | JS 函数和数组学习目标介绍数组操作是编程中的常见任务。无论是计算购物车总额、从人员列表中仅获取名字,还是移动棋盘上的棋子,您都可能在代码中的某个位置修改或操作数组。要求Fork 这个 repo将其克隆到你的机器上提交完成后运行以下命令git add .git commit -m "Solved lab"git push origin master创建一个 Pull Request,以便你的助教可以检查你的工作。自动化测试简介什么是自动化测试?自动化软件测试是通过编程执行应用程序来验证其是否满足业务需求和技术要求并且按预期运行的过程。测试应被视为一个连续的过程,而不是开发生命周期中的一项离散操作或单一活动。在产品生命周期开始时设计测试可以缓解开发复杂代码库时出现的常见问题。拥有强大的测试套件可以让您高枕无忧,因为您可以自信地改进您的工作,同时知道您不会破坏以前开发的功能。检测实验室该实验室和您在训练营期间将进行的一些实验室都配备了单元测试,以便对您的实验室进度提供自动反馈。使用
资源推荐
资源详情
资源评论
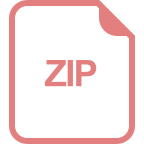
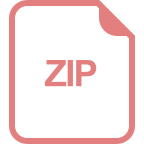
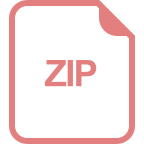
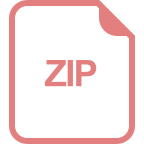
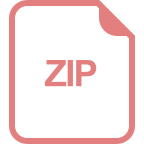
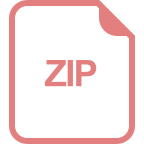
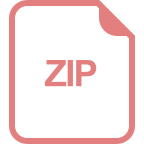
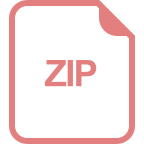
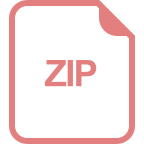
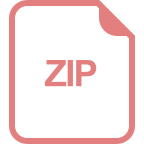
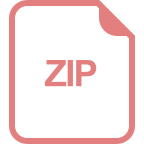
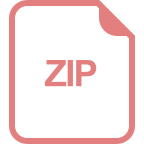
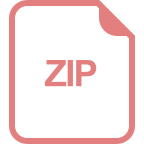
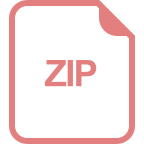
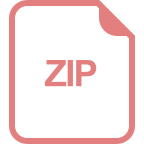
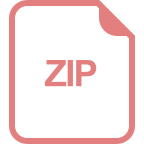
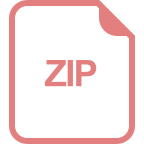
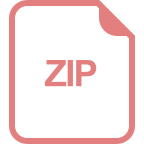
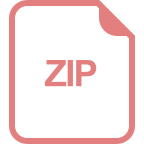
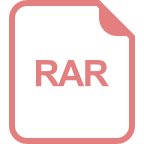
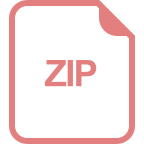
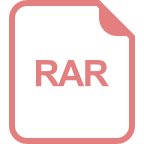
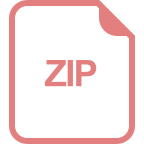
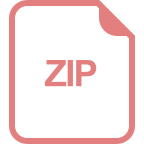
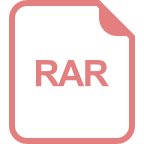
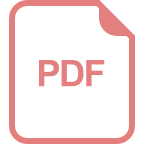
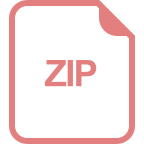
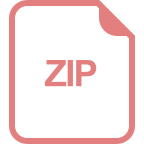
收起资源包目录


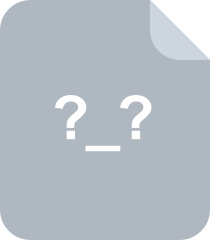
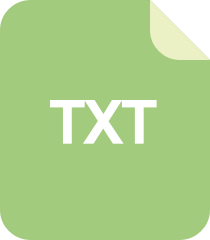

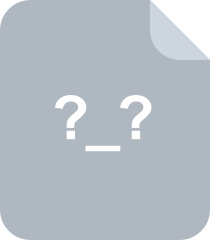


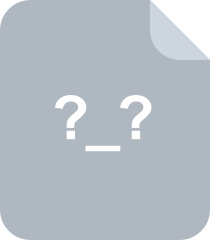
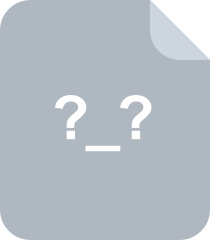
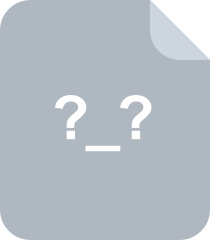
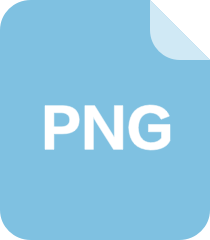
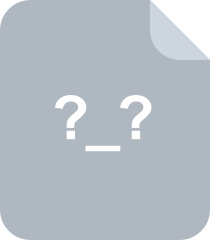
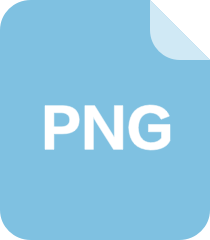
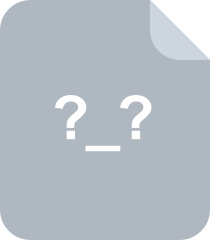

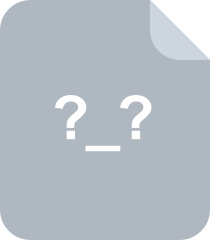
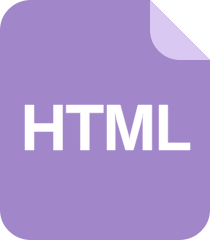
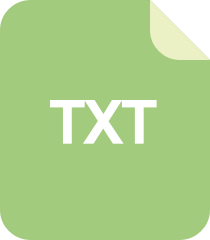
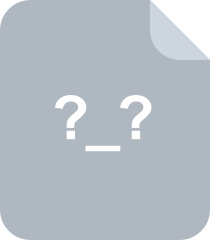
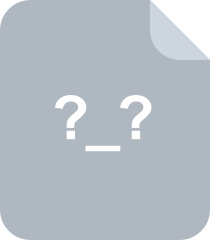
共 15 条
- 1
资源评论
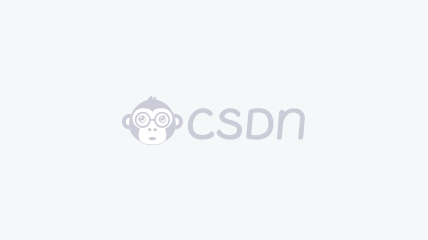

赵闪闪168
- 粉丝: 1564
- 资源: 3077
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

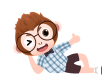
最新资源
- EngineUtilities 是一个专为自定义游戏引擎设计的数学资源和数据结构库 .zip
- egui 的 D3D9 后端 .zip
- Egui DirectX9、DirectX10、DirectX11 渲染器和 Win32 输入处理程序.zip
- DXQuake3,由 Richard Geary 在 DirectX 中开发的 Quake III 引擎.zip
- idea插件开发的第七天-开发一款数据格式化插件
- DXGL DirectX 到 OpenGL 包装器源代码.zip
- DXGI、D3D11 和 D3DCompiler 的 Haxe,hxcpp 绑定.zip
- idea插件开发的第七天-开发一款数据格式化插件
- ntfs-3g的2个rpm包
- causal-conv1d-1.1.1-cp310-cp310-win-amd64.whl.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


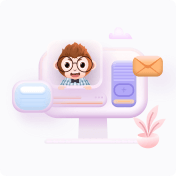
安全验证
文档复制为VIP权益,开通VIP直接复制
