/*
* Copyright 2023 The Error Prone Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.errorprone.bugpatterns;
import com.google.common.collect.ImmutableList;
import com.google.errorprone.BugCheckerRefactoringTestHelper;
import com.google.errorprone.CompilationTestHelper;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.JUnit4;
/** Tests for {@link StatementSwitchToExpressionSwitch}. */
@RunWith(JUnit4.class)
public final class StatementSwitchToExpressionSwitchTest {
private final CompilationTestHelper helper =
CompilationTestHelper.newInstance(StatementSwitchToExpressionSwitch.class, getClass());
private final BugCheckerRefactoringTestHelper refactoringHelper =
BugCheckerRefactoringTestHelper.newInstance(
StatementSwitchToExpressionSwitch.class, getClass());
@Test
public void switchByEnum_removesRedundantBreak_error() {
helper
.addSourceLines(
"Test.java",
"""
class Test {
enum Side {
OBVERSE,
REVERSE
};
public Test(int foo) {}
public void foo(Side side) {
// BUG: Diagnostic contains: [StatementSwitchToExpressionSwitch]
switch (side) {
case OBVERSE:
// Explanatory comment
System.out.println("the front is called the");
// Middle comment
System.out.println("obverse");
// Break comment
break;
// End comment
case REVERSE:
System.out.println("reverse");
}
}
}
""")
.setArgs(
ImmutableList.of("-XepOpt:StatementSwitchToExpressionSwitch:EnableDirectConversion"))
.doTest();
// Check correct generated code
refactoringHelper
.addInputLines(
"Test.java",
"""
class Test {
enum Side {
OBVERSE,
REVERSE
};
public Test(int foo) {}
public void foo(Side side) {
switch (side) {
case OBVERSE /* left comment */ /* and there is more: */ // to end of line
:
// Explanatory comment
System.out.println("the front is called the");
// Middle comment
System.out.println("obverse");
// Break comment
break;
// End comment
case REVERSE:
System.out.println("reverse");
}
}
}
""")
.addOutputLines(
"Test.java",
"""
class Test {
enum Side {
OBVERSE,
REVERSE
};
public Test(int foo) {}
public void foo(Side side) {
switch (side) {
case OBVERSE -> {
/* left comment */
/* and there is more: */
// to end of line
// Explanatory comment
System.out.println("the front is called the");
// Middle comment
System.out.println("obverse");
// Break comment
// End comment
}
case REVERSE -> System.out.println("reverse");
}
}
}
""")
.setArgs(
ImmutableList.of("-XepOpt:StatementSwitchToExpressionSwitch:EnableDirectConversion"))
.doTest(BugCheckerRefactoringTestHelper.TestMode.TEXT_MATCH);
}
@Test
public void switchByEnumWithCompletionAnalsis_removesRedundantBreak_error() {
helper
.addSourceLines(
"Test.java",
"""
class Test {
enum Side {
OBVERSE,
REVERSE
};
public Test(int foo) {}
public void foo(Side side) {
// BUG: Diagnostic contains: [StatementSwitchToExpressionSwitch]
switch (side) {
// Comment before first case
case OBVERSE:
// Explanatory comment
System.out.println("this block cannot complete normally");
{
throw new NullPointerException();
}
case REVERSE:
System.out.println("reverse");
}
}
}
""")
.setArgs(
ImmutableList.of("-XepOpt:StatementSwitchToExpressionSwitch:EnableDirectConversion"))
.doTest();
// Check correct generated code
refactoringHelper
.addInputLines(
"Test.java",
"""
class Test {
enum Side {
OBVERSE,
REVERSE
};
public Test(int foo) {}
public void foo(Side side) {
switch (side) {
// Comment before first case
case OBVERSE:
// Explanatory comment
System.out.println("this block cannot complete normally");
{
throw new NullPointerException();
}
case REVERSE:
System.out.println("reverse");
}
}
}
""")
.addOutputLines(
"Test.java",
"""
class Test {
enum Side {
OBVERSE,
REVERSE
};
public Test(int foo) {}
public void foo(Side side) {
switch (side) {
case OBVERSE -> {
// Comment before first case
// Explanatory comment
System.out.println("this block cannot complete normally");
{
throw new NullPointerException();
}
}
case REVERSE -> System.out.println("reverse");
}
}
}
""")
.setArgs(
ImmutableList.of("-XepOpt:StatementSwitchToExpressionSwitch:EnableDirectConversion"))
.doTest(BugCheckerRefactoringTestHelper.TestMode.TEXT_MATCH);
}
@Test
public void switchByEnumCard_combinesCaseComments_error() {
helper
.addSourceLines(
"Test.java",
"""
class Test {
enum Side {
HEART,
SPADE,
DIAMOND,
CLUB
};
public Test(int foo) {}
public void foo(Side side) {
// BUG: Diagnostic contains: [StatementSwitchToExpressionSwitch]
switch (side) {
case HEART:
System.out.println("heart2");
break;
case /* sparkly */ DIAMOND /* Sparkly */:
// Empty block comment 1
// Fall through
ca
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
容易出错Error Prone 是 Java 的静态分析工具,可以在编译时捕获常见的编程错误。public class ShortSet { public static void main (String[] args) { Set<Short> s = new HashSet<>(); for (short i = 0; i < 100; i++) { s.add(i); s.remove(i - 1); } System.out.println(s.size()); }}error: [CollectionIncompatibleType] Argument 'i - 1' should not be passed to this method;its type int is not compatible with its collection's type argument Short s.remove(i - 1); ^ (see https://err
资源推荐
资源详情
资源评论
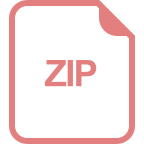
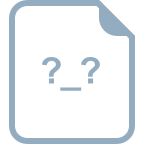
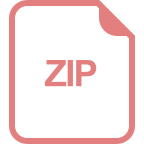
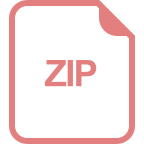
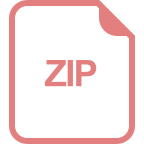
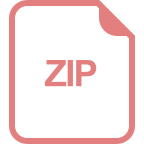
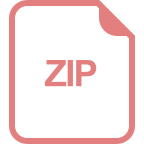
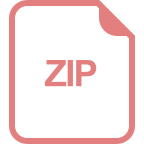
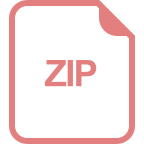
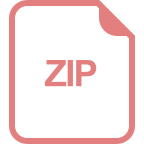
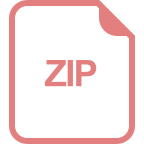
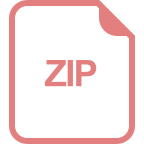
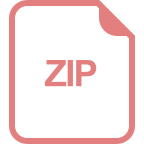
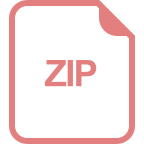
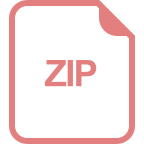
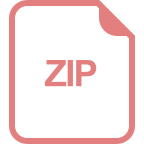
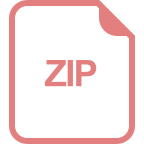
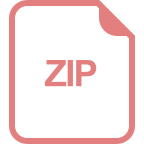
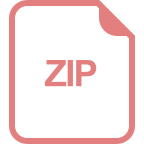
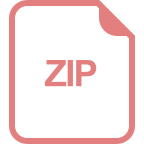
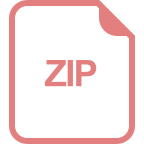
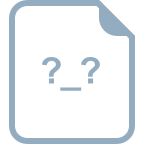
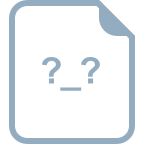
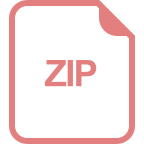
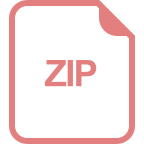
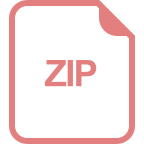
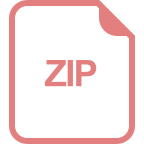
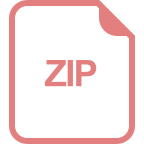
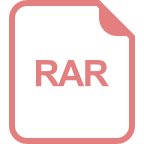
收起资源包目录

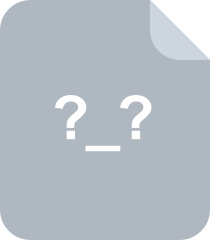
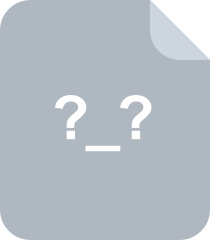
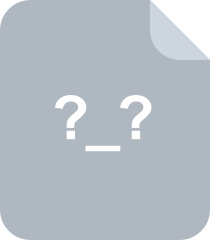
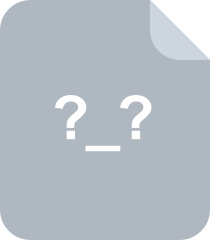
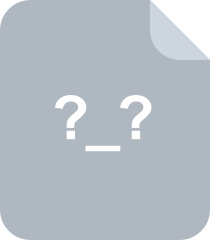
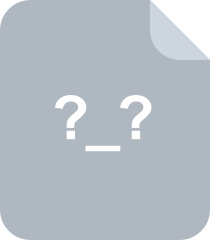
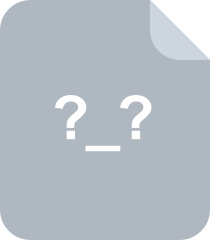
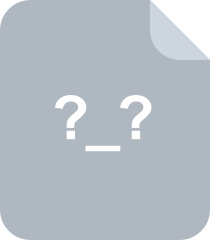
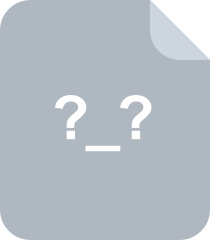
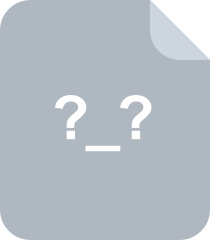
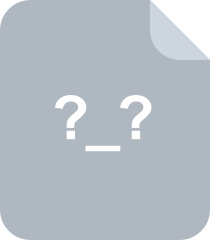
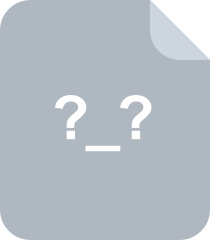
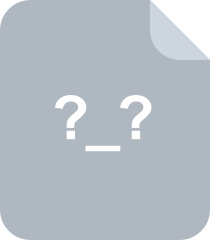
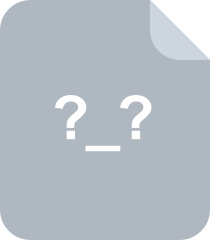
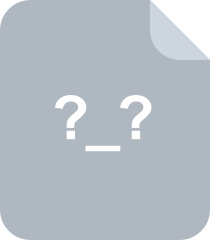
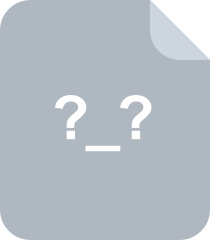
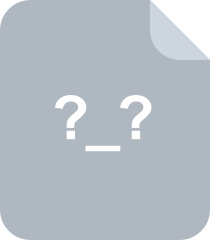
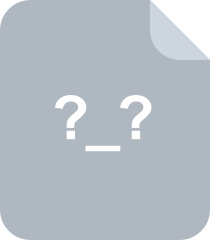
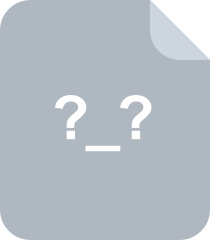
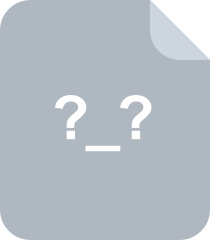
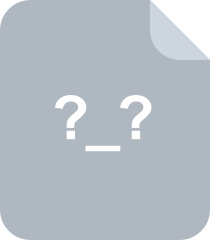
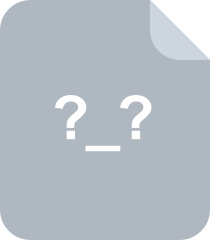
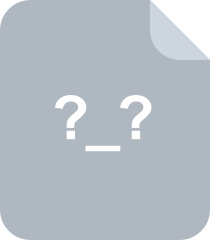
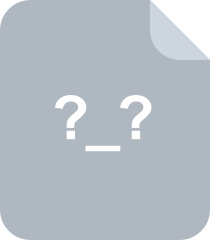
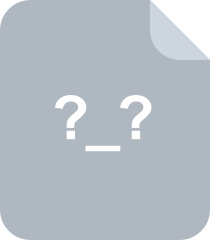
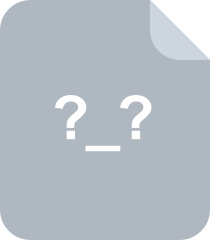
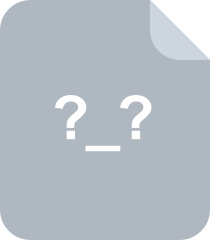
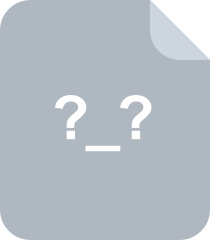
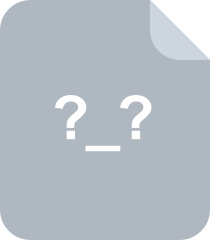
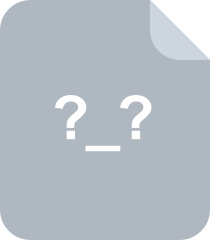
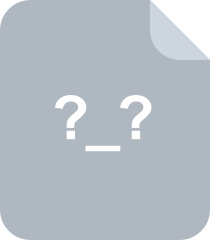
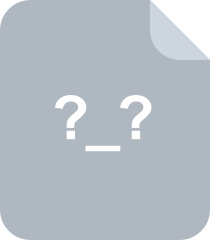
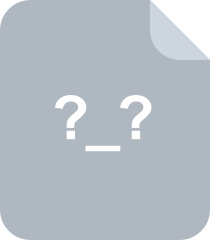
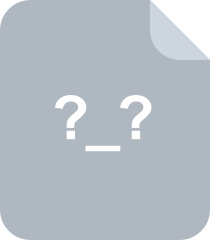
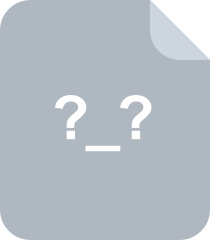
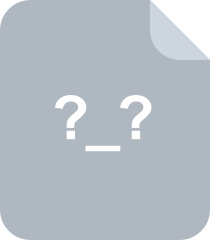
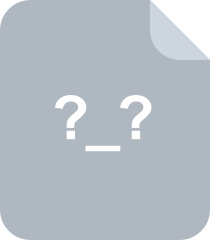
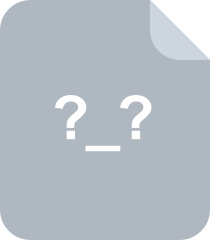
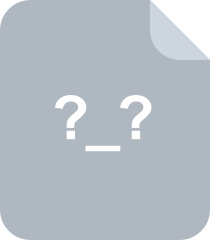
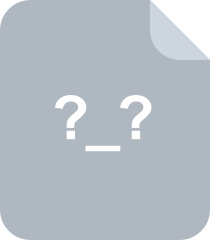
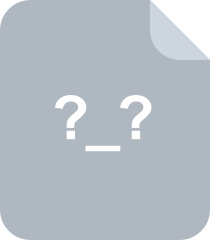
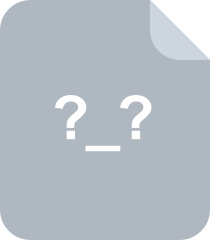
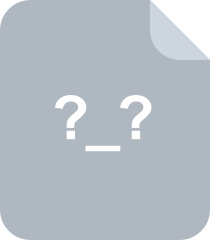
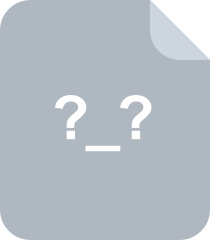
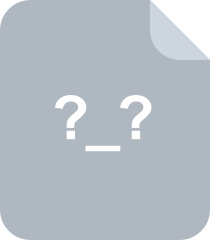
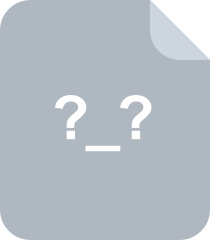
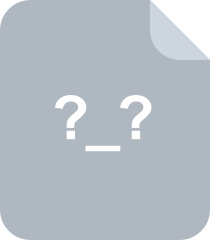
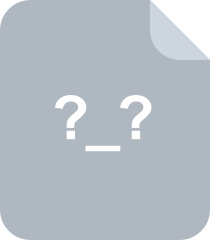
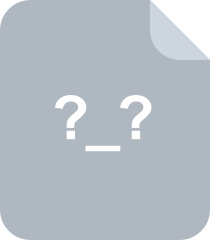
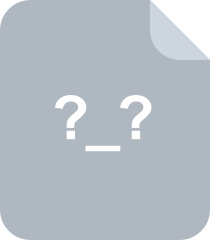
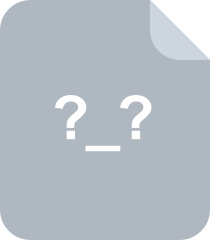
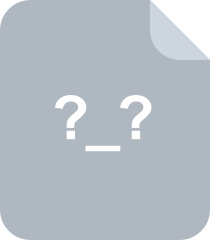
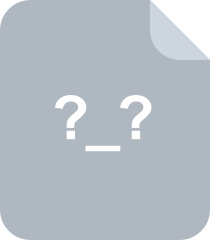
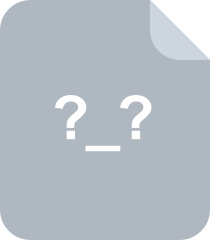
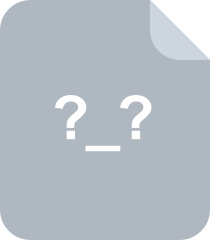
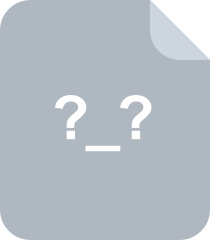
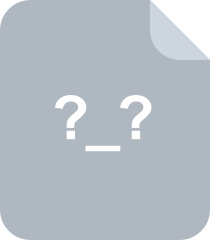
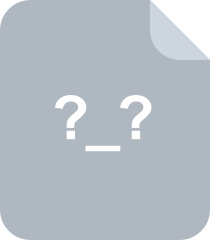
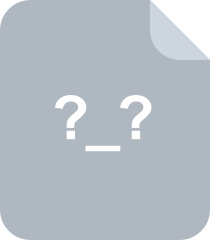
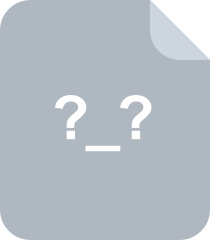
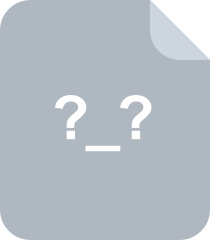
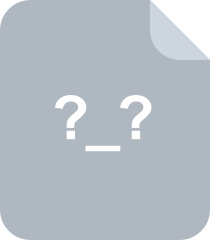
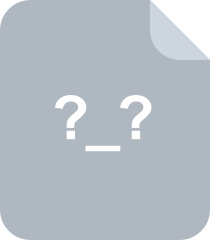
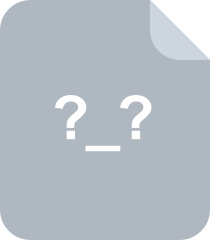
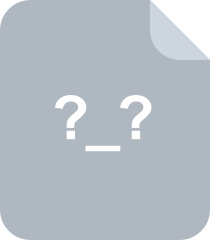
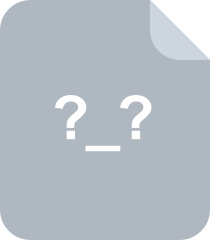
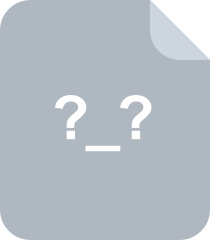
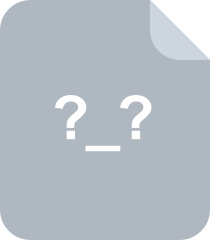
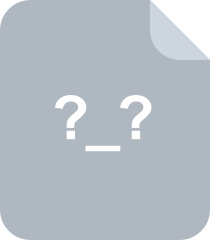
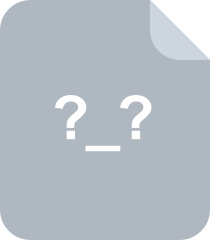
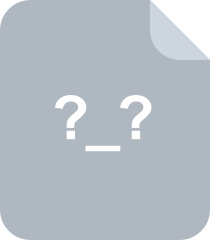
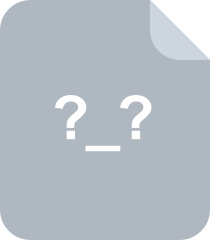
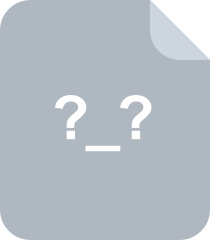
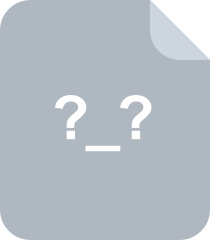
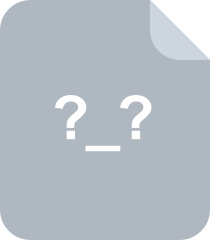
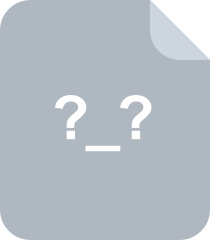
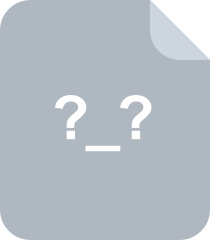
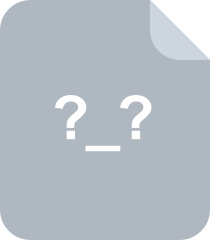
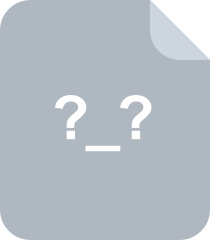
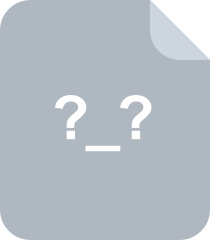
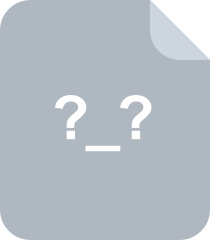
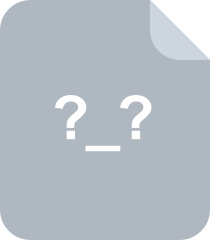
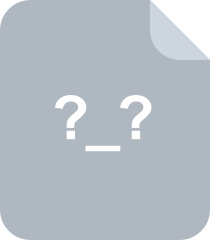
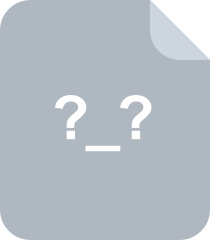
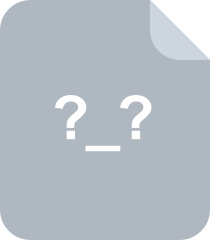
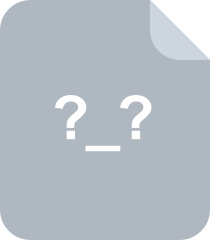
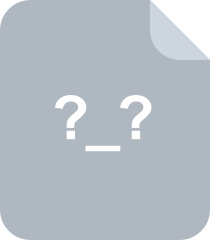
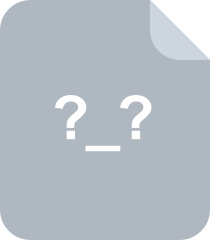
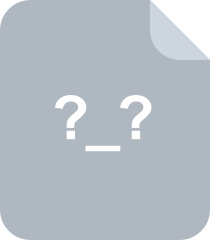
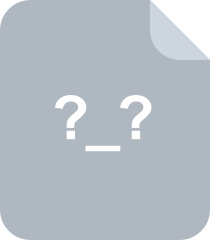
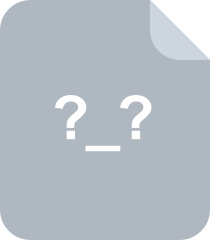
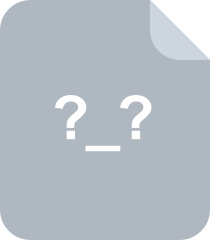
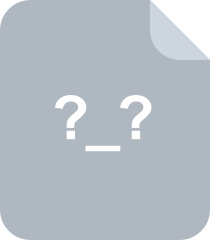
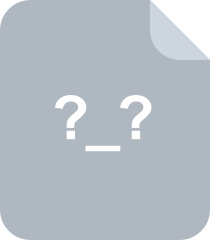
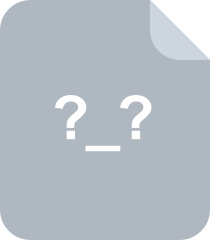
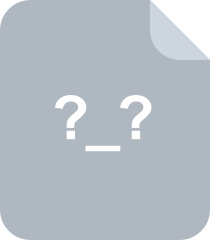
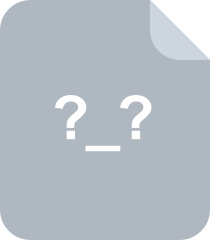
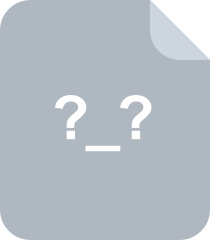
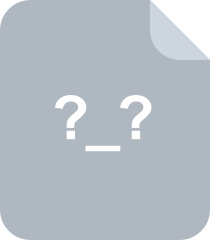
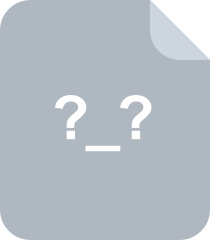
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
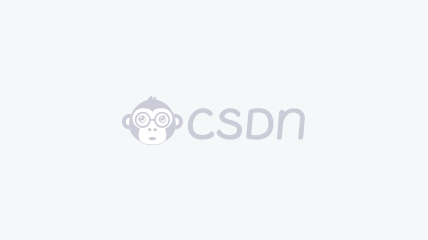

赵闪闪168
- 粉丝: 1619
- 资源: 4236
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

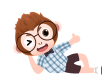
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


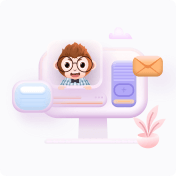
安全验证
文档复制为VIP权益,开通VIP直接复制
