// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/protobuf/struct.proto
package org.tensorflow.proto;
public final class Struct {
private Struct() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface StructuredValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.StructuredValue)
com.google.protobuf.MessageOrBuilder {
/**
* <pre>
* Represents None.
* </pre>
*
* <code>.tensorflow.NoneValue none_value = 1;</code>
* @return Whether the noneValue field is set.
*/
boolean hasNoneValue();
/**
* <pre>
* Represents None.
* </pre>
*
* <code>.tensorflow.NoneValue none_value = 1;</code>
* @return The noneValue.
*/
org.tensorflow.proto.Struct.NoneValue getNoneValue();
/**
* <pre>
* Represents None.
* </pre>
*
* <code>.tensorflow.NoneValue none_value = 1;</code>
*/
org.tensorflow.proto.Struct.NoneValueOrBuilder getNoneValueOrBuilder();
/**
* <pre>
* Represents a double-precision floating-point value (a Python `float`).
* </pre>
*
* <code>double float64_value = 11;</code>
* @return Whether the float64Value field is set.
*/
boolean hasFloat64Value();
/**
* <pre>
* Represents a double-precision floating-point value (a Python `float`).
* </pre>
*
* <code>double float64_value = 11;</code>
* @return The float64Value.
*/
double getFloat64Value();
/**
* <pre>
* Represents a signed integer value, limited to 64 bits.
* Larger values from Python's arbitrary-precision integers are unsupported.
* </pre>
*
* <code>sint64 int64_value = 12;</code>
* @return Whether the int64Value field is set.
*/
boolean hasInt64Value();
/**
* <pre>
* Represents a signed integer value, limited to 64 bits.
* Larger values from Python's arbitrary-precision integers are unsupported.
* </pre>
*
* <code>sint64 int64_value = 12;</code>
* @return The int64Value.
*/
long getInt64Value();
/**
* <pre>
* Represents a string of Unicode characters stored in a Python `str`.
* In Python 3, this is exactly what type `str` is.
* In Python 2, this is the UTF-8 encoding of the characters.
* For strings with ASCII characters only (as often used in TensorFlow code)
* there is effectively no difference between the language versions.
* The obsolescent `unicode` type of Python 2 is not supported here.
* </pre>
*
* <code>string string_value = 13;</code>
* @return Whether the stringValue field is set.
*/
boolean hasStringValue();
/**
* <pre>
* Represents a string of Unicode characters stored in a Python `str`.
* In Python 3, this is exactly what type `str` is.
* In Python 2, this is the UTF-8 encoding of the characters.
* For strings with ASCII characters only (as often used in TensorFlow code)
* there is effectively no difference between the language versions.
* The obsolescent `unicode` type of Python 2 is not supported here.
* </pre>
*
* <code>string string_value = 13;</code>
* @return The stringValue.
*/
java.lang.String getStringValue();
/**
* <pre>
* Represents a string of Unicode characters stored in a Python `str`.
* In Python 3, this is exactly what type `str` is.
* In Python 2, this is the UTF-8 encoding of the characters.
* For strings with ASCII characters only (as often used in TensorFlow code)
* there is effectively no difference between the language versions.
* The obsolescent `unicode` type of Python 2 is not supported here.
* </pre>
*
* <code>string string_value = 13;</code>
* @return The bytes for stringValue.
*/
com.google.protobuf.ByteString
getStringValueBytes();
/**
* <pre>
* Represents a boolean value.
* </pre>
*
* <code>bool bool_value = 14;</code>
* @return Whether the boolValue field is set.
*/
boolean hasBoolValue();
/**
* <pre>
* Represents a boolean value.
* </pre>
*
* <code>bool bool_value = 14;</code>
* @return The boolValue.
*/
boolean getBoolValue();
/**
* <pre>
* Represents a TensorShape.
* </pre>
*
* <code>.tensorflow.TensorShapeProto tensor_shape_value = 31;</code>
* @return Whether the tensorShapeValue field is set.
*/
boolean hasTensorShapeValue();
/**
* <pre>
* Represents a TensorShape.
* </pre>
*
* <code>.tensorflow.TensorShapeProto tensor_shape_value = 31;</code>
* @return The tensorShapeValue.
*/
org.tensorflow.proto.TensorShapeProto getTensorShapeValue();
/**
* <pre>
* Represents a TensorShape.
* </pre>
*
* <code>.tensorflow.TensorShapeProto tensor_shape_value = 31;</code>
*/
org.tensorflow.proto.TensorShapeProtoOrBuilder getTensorShapeValueOrBuilder();
/**
* <pre>
* Represents an enum value for dtype.
* </pre>
*
* <code>.tensorflow.DataType tensor_dtype_value = 32;</code>
* @return Whether the tensorDtypeValue field is set.
*/
boolean hasTensorDtypeValue();
/**
* <pre>
* Represents an enum value for dtype.
* </pre>
*
* <code>.tensorflow.DataType tensor_dtype_value = 32;</code>
* @return The enum numeric value on the wire for tensorDtypeValue.
*/
int getTensorDtypeValueValue();
/**
* <pre>
* Represents an enum value for dtype.
* </pre>
*
* <code>.tensorflow.DataType tensor_dtype_value = 32;</code>
* @return The tensorDtypeValue.
*/
org.tensorflow.proto.DataType getTensorDtypeValue();
/**
* <pre>
* Represents a value for tf.TensorSpec.
* </pre>
*
* <code>.tensorflow.TensorSpecProto tensor_spec_value = 33;</code>
* @return Whether the tensorSpecValue field is set.
*/
boolean hasTensorSpecValue();
/**
* <pre>
* Represents a value for tf.TensorSpec.
* </pre>
*
* <code>.tensorflow.TensorSpecProto tensor_spec_value = 33;</code>
* @return The tensorSpecValue.
*/
org.tensorflow.proto.Struct.TensorSpecProto getTensorSpecValue();
/**
* <pre>
* Represents a value for tf.TensorSpec.
* </pre>
*
* <code>.tensorflow.TensorSpecProto tensor_spec_value = 33;</code>
*/
org.tensorflow.proto.Struct.TensorSpecProtoOrBuilder getTensorSpecValueOrBuilder();
/**
* <pre>
* Represents a value for tf.TypeSpec.
* </pre>
*
* <code>.tensorflow.TypeSpecProto type_spec_value = 34;</code>
* @return Whether the typeSpecValue field is set.
*/
boolean hasTypeSpecValue();
/**
* <pre>
* Represents a value for tf.TypeSpec.
* </pre>
*
* <code>.tensorflow.TypeSpecProto type_spec_value = 34;</code>
* @return The typeSpecValue.
*/
org.tensorflow.proto.Struct.TypeSpecProto getTypeSpecValue();
/**
* <pre>
* Represents a value for tf.TypeSpec.
* </pre>
*
* <code>.tensorflow.TypeSpecProto type_spec_value = 34;</code>
*/
org.tensorflow.proto.Struct.TypeSpecProtoOrBuilder getTypeSpecValueOrBuilder();
/**
* <pre>
* Represents a value for tf.BoundedTensorSpec.
* </pre>
*
* <code>.tensorflow.BoundedTensorSpecProto bounded_tensor_spec_value = 35;</code>
* @return Whether the boundedTensorSpecValue field is set.
*/
boolean hasBoundedTensorSpecValue();
没有合适的资源?快使用搜索试试~ 我知道了~
TensorFlow 的 Java 绑定.zip
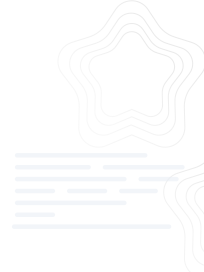
共2000个文件
java:1970个
md:13个
xml:6个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 81 浏览量
2024-11-25
01:34:48
上传
评论
收藏 6.86MB ZIP 举报
温馨提示
TensorFlow 的 Java 绑定适用于 Java 的 TensorFlow欢迎来到 TensorFlow 的 Java 世界!TensorFlow 可以在任何 JVM 上运行,用于构建、训练和运行机器学习模型。它附带一系列实用程序和框架,可帮助完成数据科学家和开发人员在此领域工作的大多数常见任务。Java 和其他 JVM 语言(例如 Scala 或 Kotlin)在世界各地的中小型企业中被广泛使用,这使得 TensorFlow 成为大规模采用机器学习的战略选择。此存储库早期,TensorFlow 的 Java 语言绑定托管在主存储库中 ,只有当核心库的新版本准备好发布时才会发布,这种情况每年只发生几次。现在,所有与 Java 相关的代码都已移至此存储库,以便它可以独立于官方 TensorFlow 版本进行发展和发布。此外,大多数构建任务已从 Bazel 迁移到 Maven,这对大多数 Java 开发人员来说更为熟悉。下面描述了存储库及其不同工件的布局tensorflow-core构建 TensorFlow for Java 核心语言绑定的所有工件目
资源推荐
资源详情
资源评论
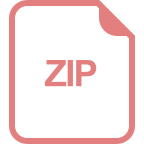
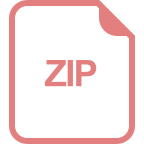
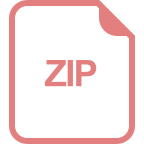
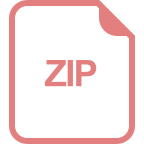
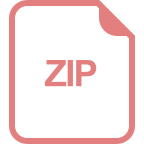
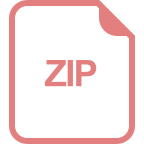
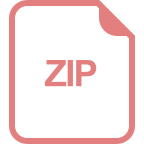
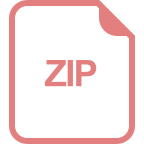
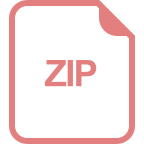
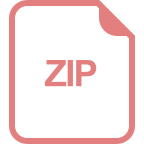
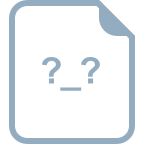
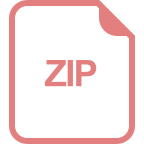
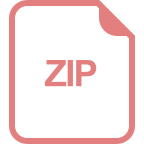
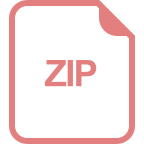
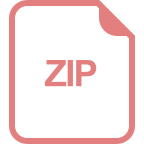
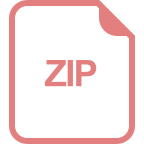
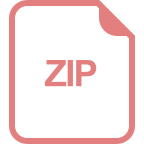
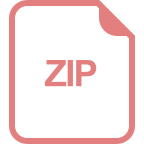
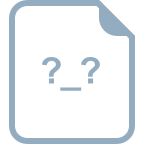
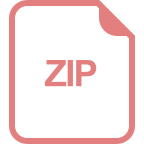
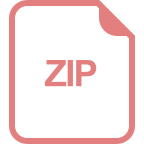
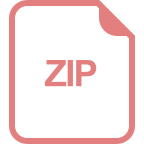
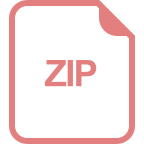
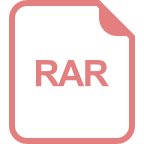
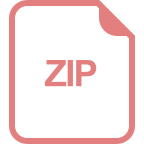
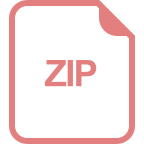
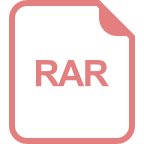
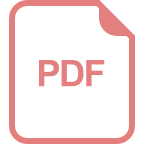
收起资源包目录

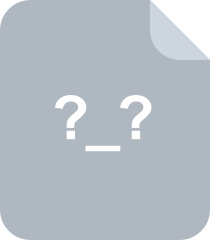
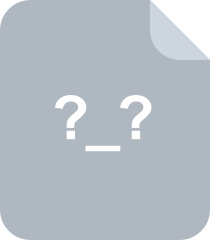
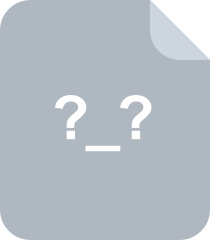
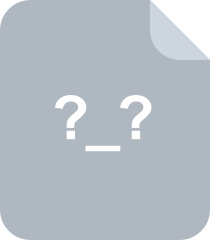
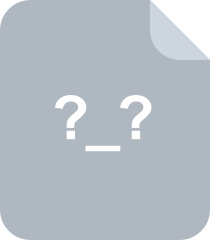
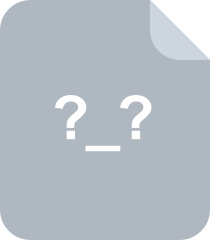
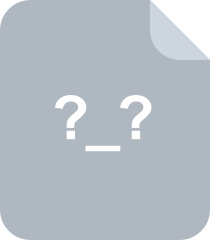
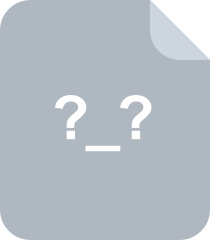
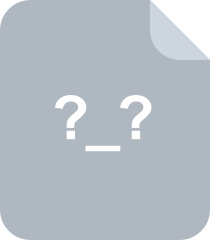
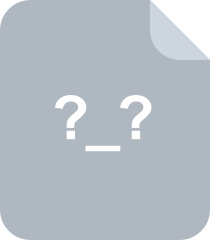
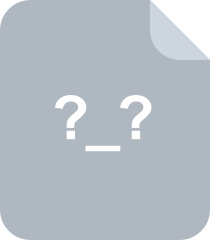
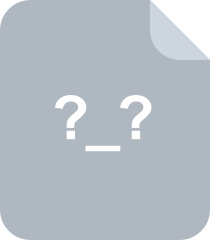
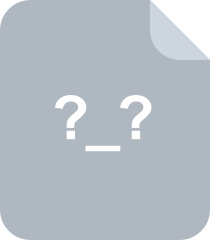
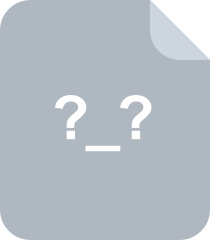
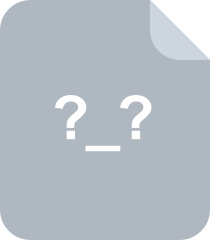
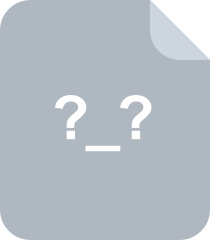
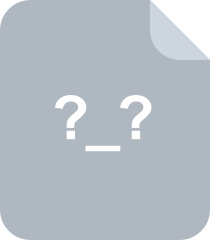
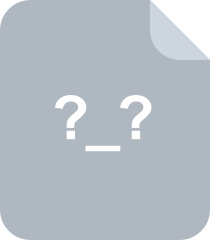
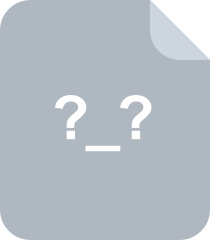
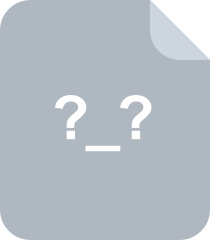
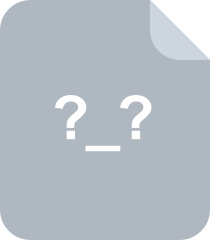
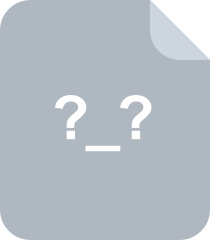
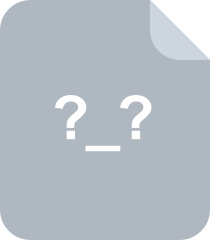
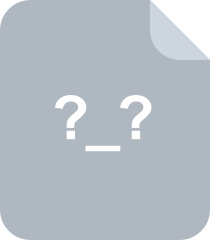
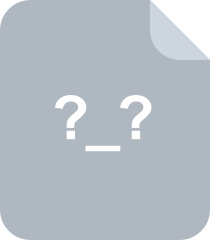
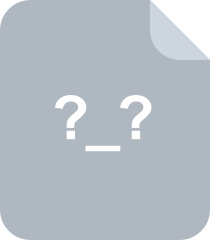
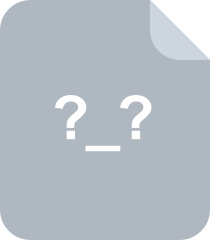
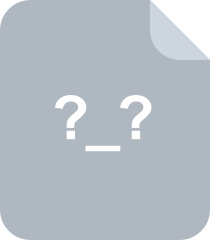
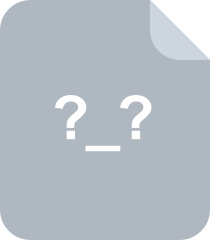
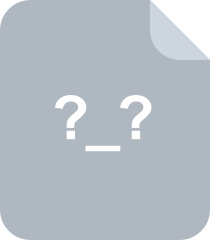
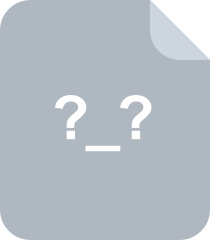
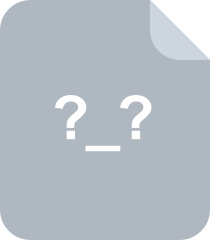
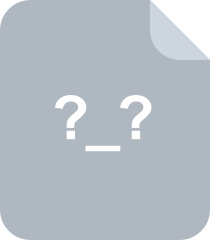
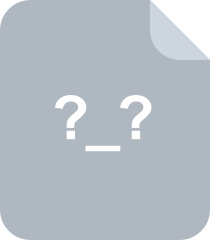
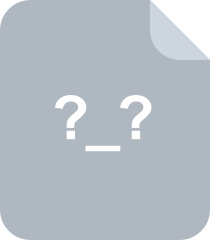
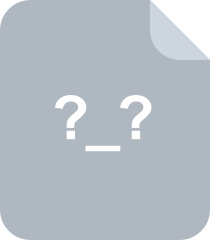
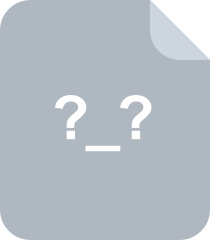
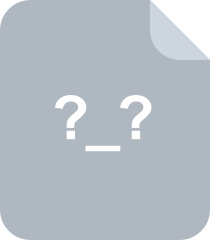
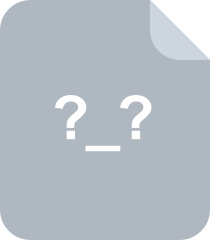
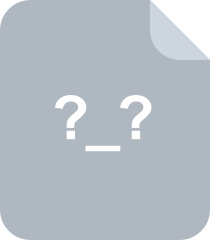
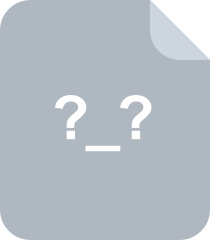
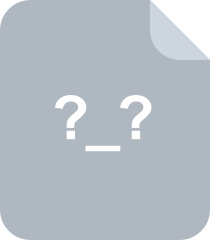
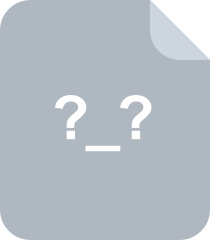
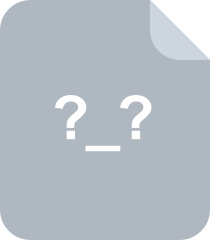
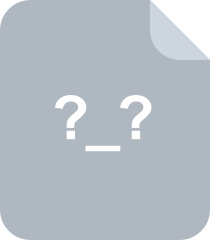
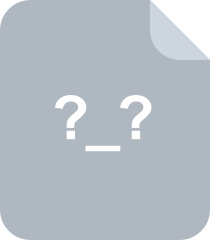
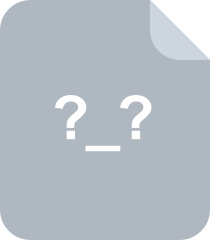
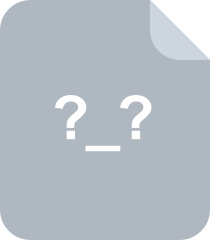
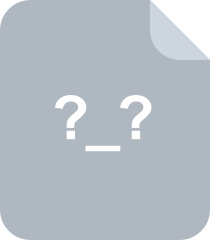
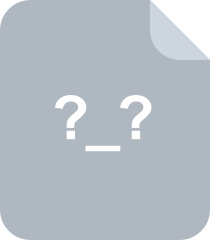
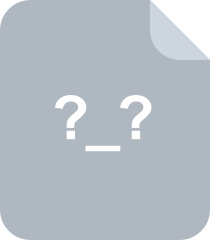
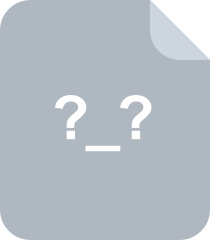
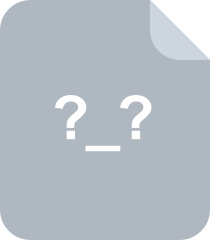
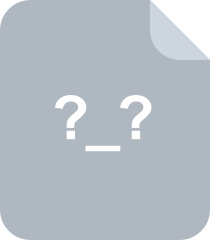
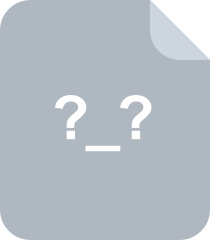
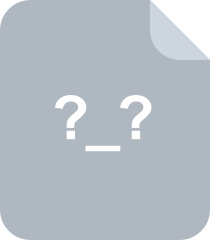
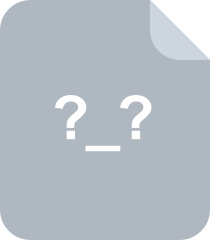
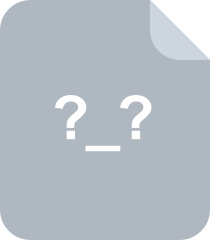
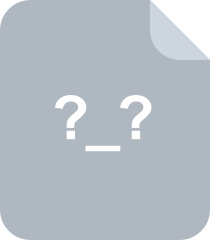
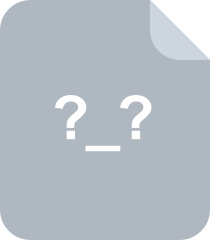
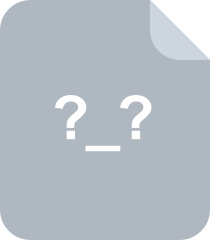
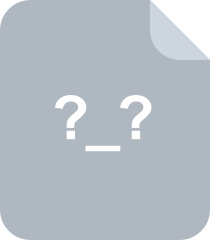
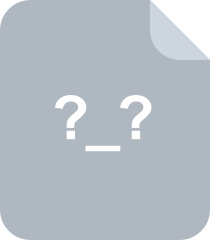
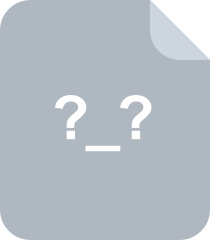
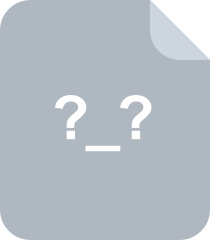
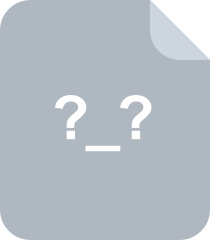
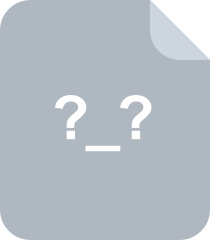
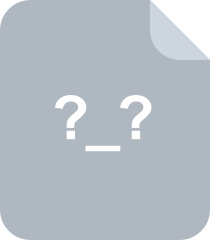
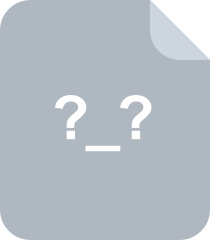
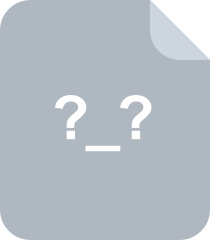
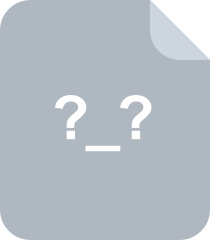
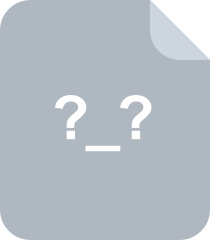
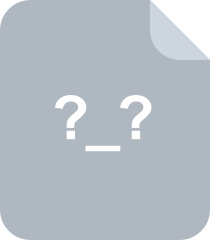
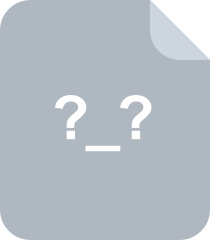
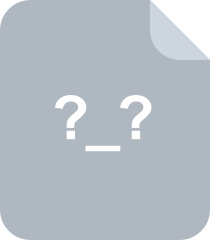
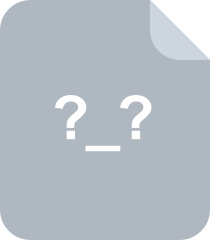
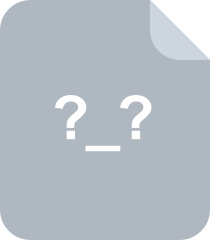
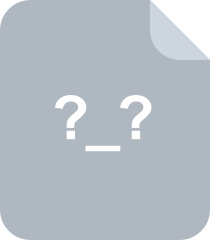
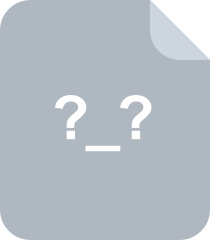
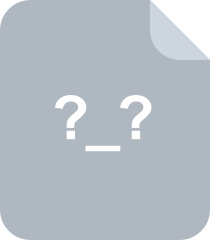
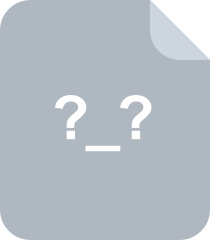
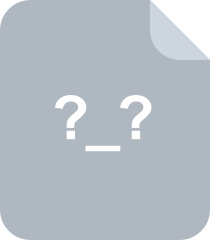
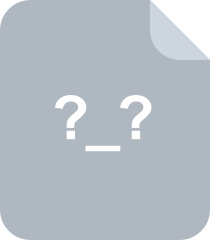
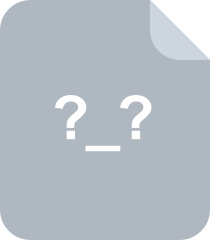
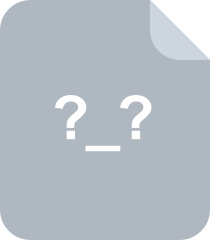
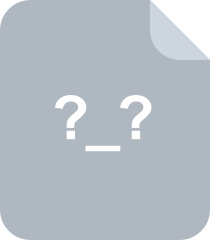
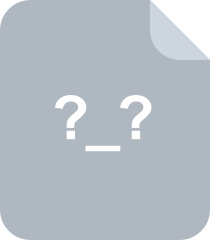
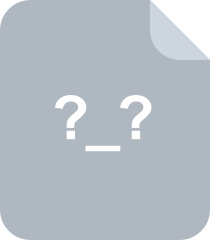
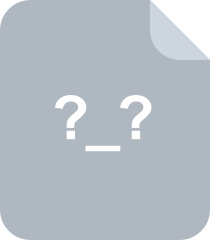
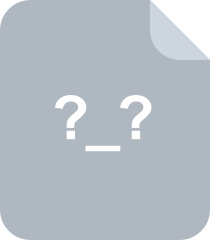
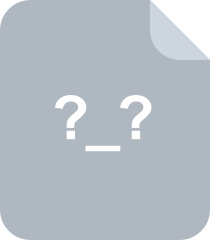
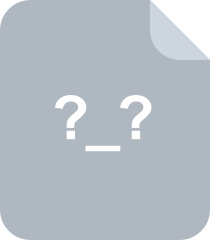
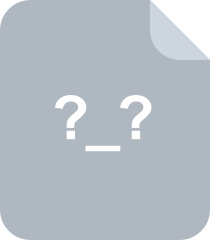
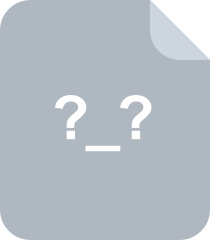
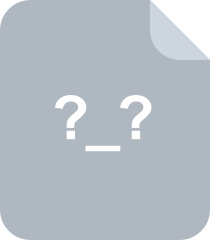
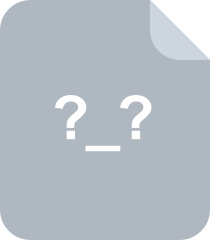
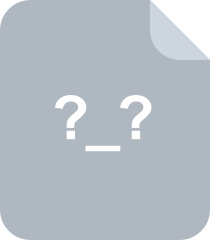
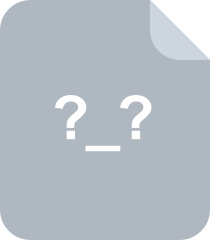
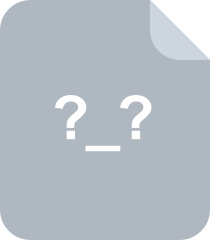
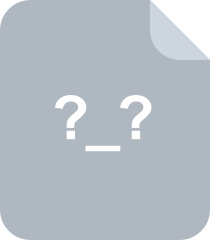
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
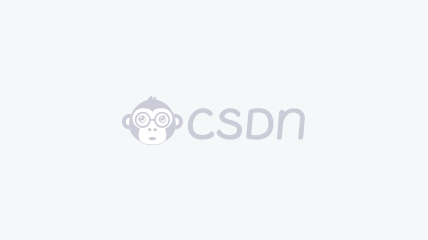

赵闪闪168
- 粉丝: 1726
- 资源: 6171
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

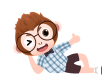
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


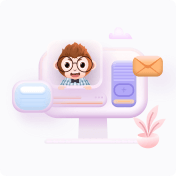
安全验证
文档复制为VIP权益,开通VIP直接复制
