// File generated from our OpenAPI spec
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import com.stripe.param.common.EmptyParam;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import lombok.Getter;
@Getter
public class PaymentIntentCreateParams extends ApiRequestParams {
/**
* <strong>Required.</strong> Amount intended to be collected by this PaymentIntent. A positive
* integer representing how much to charge in the <a
* href="https://stripe.com/docs/currencies#zero-decimal">smallest currency unit</a> (e.g., 100
* cents to charge $1.00 or 100 to charge ¥100, a zero-decimal currency). The minimum amount is
* $0.50 US or <a
* href="https://stripe.com/docs/currencies#minimum-and-maximum-charge-amounts">equivalent in
* charge currency</a>. The amount value supports up to eight digits (e.g., a value of 99999999
* for a USD charge of $999,999.99).
*/
@SerializedName("amount")
Long amount;
/**
* The amount of the application fee (if any) that will be requested to be applied to the payment
* and transferred to the application owner's Stripe account. The amount of the application fee
* collected will be capped at the total payment amount. For more information, see the
* PaymentIntents <a href="https://stripe.com/docs/payments/connected-accounts">use case for
* connected accounts</a>.
*/
@SerializedName("application_fee_amount")
Long applicationFeeAmount;
/**
* When you enable this parameter, this PaymentIntent accepts payment methods that you enable in
* the Dashboard and that are compatible with this PaymentIntent's other parameters.
*/
@SerializedName("automatic_payment_methods")
AutomaticPaymentMethods automaticPaymentMethods;
/** Controls when the funds will be captured from the customer's account. */
@SerializedName("capture_method")
CaptureMethod captureMethod;
/**
* Set to {@code true} to attempt to <a
* href="https://stripe.com/docs/api/payment_intents/confirm">confirm this PaymentIntent</a>
* immediately. This parameter defaults to {@code false}. When creating and confirming a
* PaymentIntent at the same time, you can also provide the parameters available in the <a
* href="https://stripe.com/docs/api/payment_intents/confirm">Confirm API</a>.
*/
@SerializedName("confirm")
Boolean confirm;
/**
* Describes whether we can confirm this PaymentIntent automatically, or if it requires customer
* action to confirm the payment.
*/
@SerializedName("confirmation_method")
ConfirmationMethod confirmationMethod;
/**
* ID of the ConfirmationToken used to confirm this PaymentIntent.
*
* <p>If the provided ConfirmationToken contains properties that are also being provided in this
* request, such as {@code payment_method}, then the values in this request will take precedence.
*/
@SerializedName("confirmation_token")
String confirmationToken;
/**
* <strong>Required.</strong> Three-letter <a
* href="https://www.iso.org/iso-4217-currency-codes.html">ISO currency code</a>, in lowercase.
* Must be a <a href="https://stripe.com/docs/currencies">supported currency</a>.
*/
@SerializedName("currency")
String currency;
/**
* ID of the Customer this PaymentIntent belongs to, if one exists.
*
* <p>Payment methods attached to other Customers cannot be used with this PaymentIntent.
*
* <p>If <a
* href="https://stripe.com/docs/api#payment_intent_object-setup_future_usage">setup_future_usage</a>
* is set and this PaymentIntent's payment method is not {@code card_present}, then the payment
* method attaches to the Customer after the PaymentIntent has been confirmed and any required
* actions from the user are complete. If the payment method is {@code card_present} and isn't a
* digital wallet, then a <a
* href="https://docs.stripe.com/api/charges/object#charge_object-payment_method_details-card_present-generated_card">generated_card</a>
* payment method representing the card is created and attached to the Customer instead.
*/
@SerializedName("customer")
String customer;
/** An arbitrary string attached to the object. Often useful for displaying to users. */
@SerializedName("description")
String description;
/**
* Set to {@code true} to fail the payment attempt if the PaymentIntent transitions into {@code
* requires_action}. Use this parameter for simpler integrations that don't handle customer
* actions, such as <a
* href="https://stripe.com/docs/payments/save-card-without-authentication">saving cards without
* authentication</a>. This parameter can only be used with <a
* href="https://stripe.com/docs/api/payment_intents/create#create_payment_intent-confirm">{@code
* confirm=true}</a>.
*/
@SerializedName("error_on_requires_action")
Boolean errorOnRequiresAction;
/** Specifies which fields in the response should be expanded. */
@SerializedName("expand")
List<String> expand;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map<String, Object> extraParams;
/**
* ID of the mandate that's used for this payment. This parameter can only be used with <a
* href="https://stripe.com/docs/api/payment_intents/create#create_payment_intent-confirm">{@code
* confirm=true}</a>.
*/
@SerializedName("mandate")
String mandate;
/**
* This hash contains details about the Mandate to create. This parameter can only be used with <a
* href="https://stripe.com/docs/api/payment_intents/create#create_payment_intent-confirm">{@code
* confirm=true}</a>.
*/
@SerializedName("mandate_data")
Object mandateData;
/**
* Set of <a href="https://stripe.com/docs/api/metadata">key-value pairs</a> that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format. Individual keys can be unset by posting an empty value to them. All keys can
* be unset by posting an empty value to {@code metadata}.
*/
@SerializedName("metadata")
Map<String, String> metadata;
/**
* Set to {@code true} to indicate that the customer isn't in your checkout flow during this
* payment attempt and can't authenticate. Use this parameter in scenarios where you collect card
* details and <a href="https://stripe.com/docs/payments/cards/charging-saved-cards">charge them
* later</a>. This parameter can only be used with <a
* href="https://stripe.com/docs/api/payment_intents/create#create_payment_intent-confirm">{@code
* confirm=true}</a>.
*/
@SerializedName("off_session")
Object offSession;
/**
* The Stripe account ID that these funds are intended for. Learn more about the <a
* href="https://stripe.com/docs/payments/connected-accounts">use case for connected accounts</a>.
*/
@SerializedName("on_behalf_of")
String onBehalfOf;
/**
* ID of the payment method (a PaymentMethod, Card, or <a
* href="https://stripe.com/docs/payments/payment-methods#compatibility">compatible Source</a>
* object) to attach to this PaymentIntent.
*
* <p>If you don't provide the {@code payment_method} parameter or the {@code source} parameter
* with {@code confirm=true}, {@code source} automatically populates with {@code
* customer.default_source} to improve migration for users of the Charges API. We recommend that
* you explicitly provide the {@code payment_method} moving forward.
*/
@SerializedName("payment_method")
String payment
没有合适的资源?快使用搜索试试~ 我知道了~
Stripe API 的 Java 库 .zip
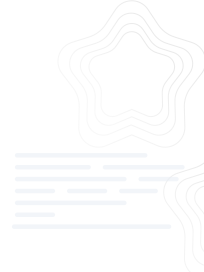
共1373个文件
java:1295个
json:51个
md:4个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 147 浏览量
2024-11-25
01:32:58
上传
评论
收藏 3.13MB ZIP 举报
温馨提示
Stripe Java 客户端库 官方Stripe Java 客户端库。安装要求Java 1.8 或更高版本Gradle 用户将此依赖项添加到项目的构建文件中implementation "com.stripe:stripe-java:28.1.0"Maven 用户将此依赖项添加到项目的 POM 中<dependency> <groupId>com.stripe</groupId> <artifactId>stripe-java</artifactId> <version>28.1.0</version></dependency>其他的您需要手动安装以下 JAR条纹罐来自https://repo1.maven.org/maven2/com/google/code/gson/gson/2.10.1/gson-2.10.1.jar的Google Gson。混淆器如果您打算使用 ProGuard,请确保排除 Stripe 客户端库。您可以通过将以下内容添加到proguard.cfg 文件来执行此操作-keep cla
资源推荐
资源详情
资源评论
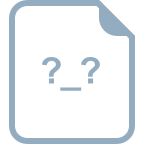
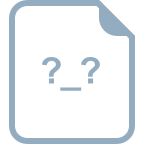
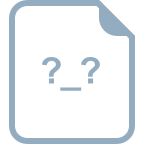
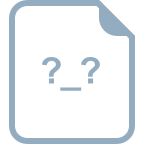
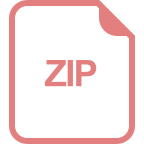
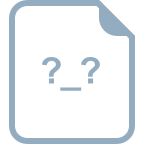
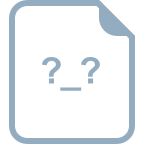
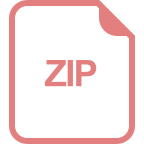
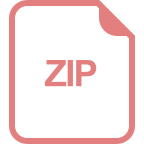
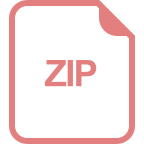
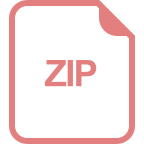
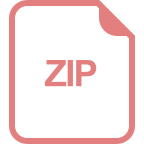
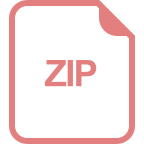
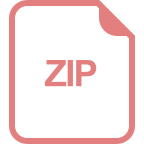
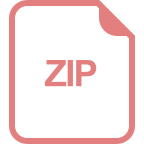
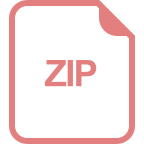
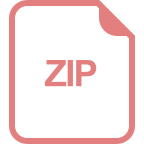
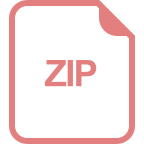
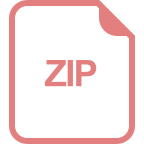
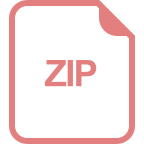
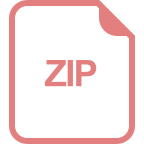
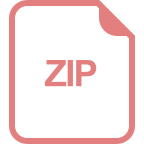
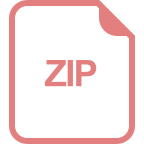
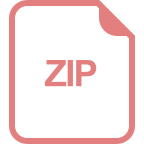
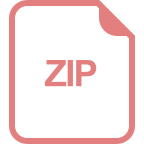
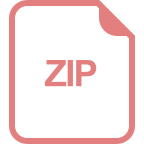
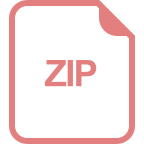
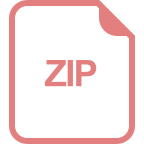
收起资源包目录

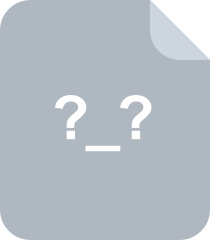
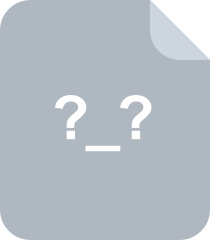
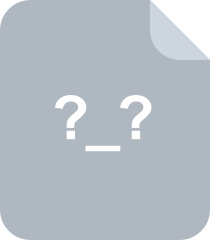
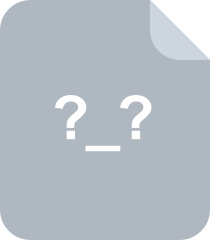
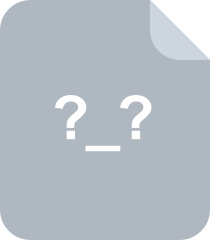
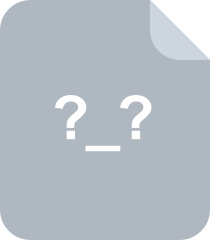
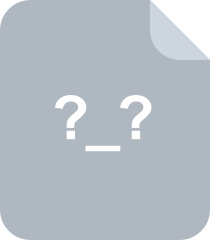
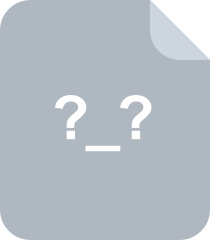
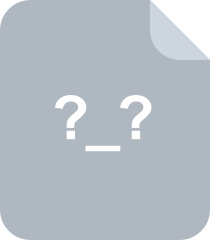
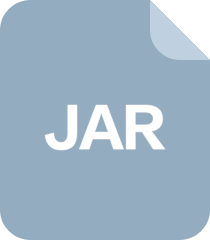
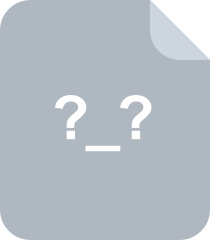
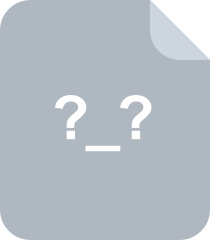
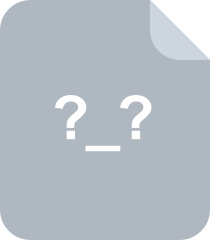
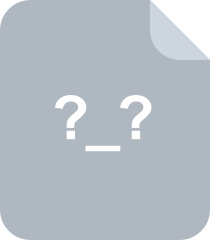
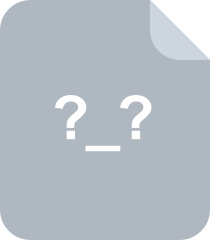
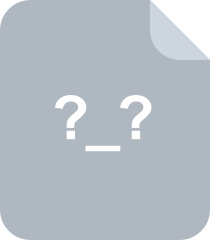
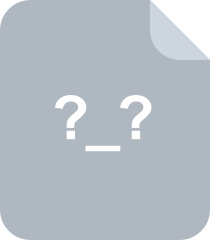
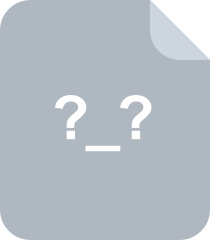
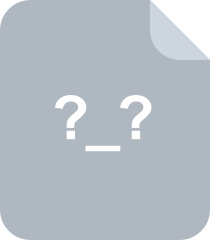
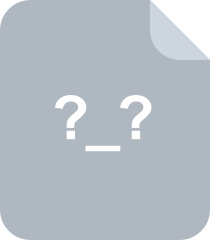
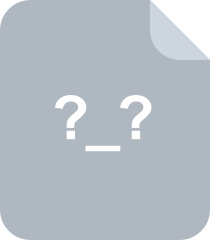
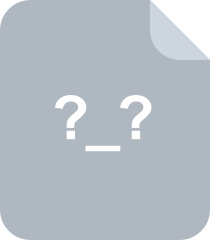
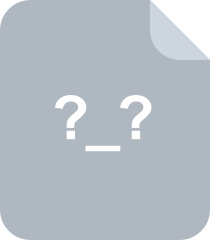
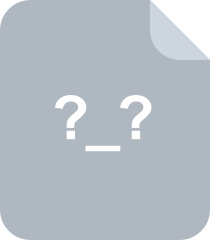
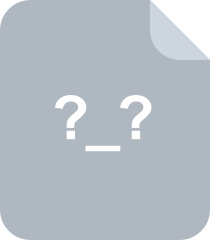
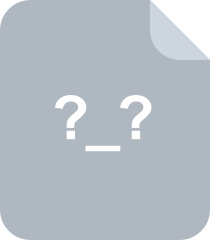
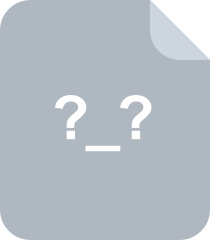
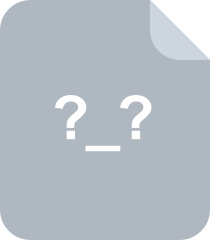
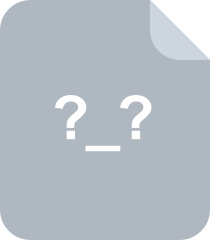
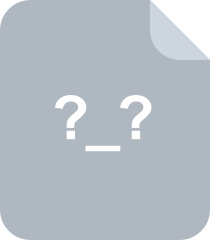
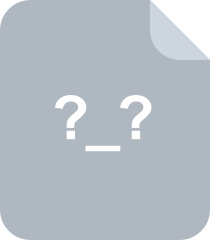
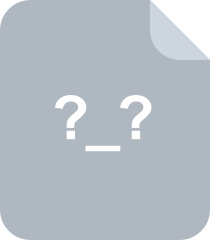
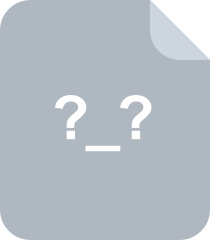
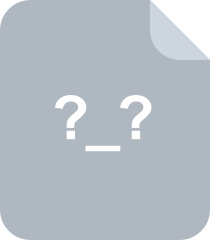
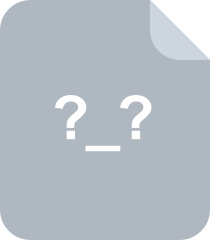
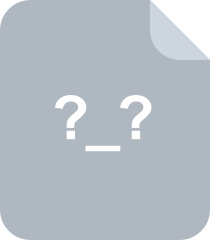
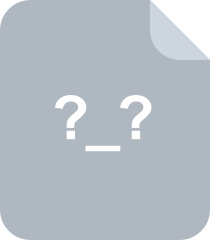
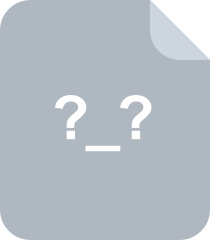
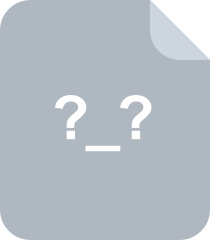
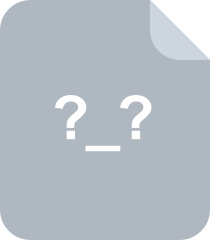
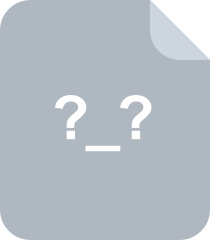
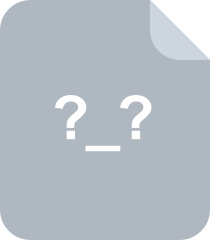
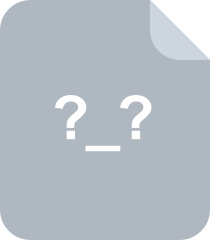
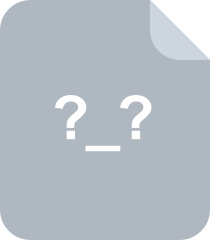
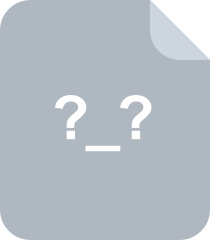
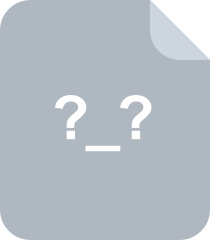
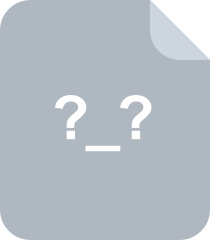
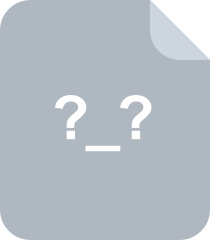
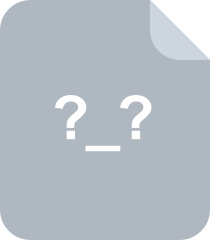
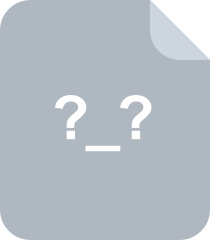
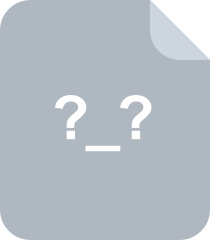
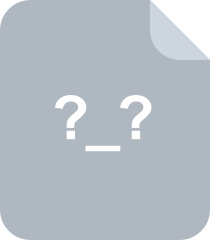
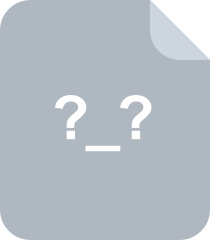
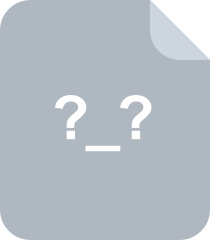
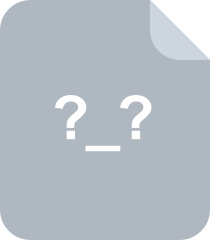
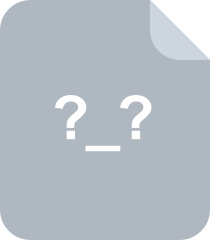
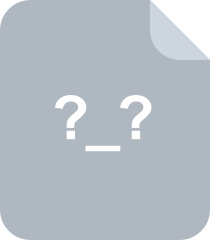
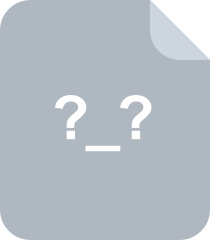
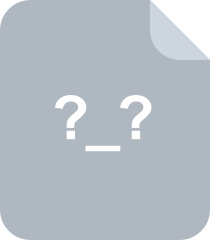
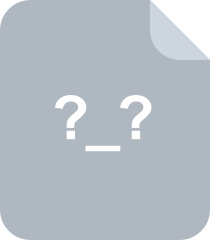
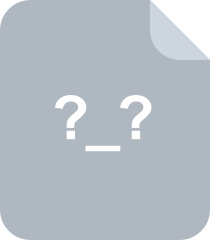
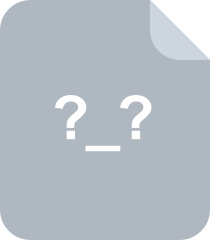
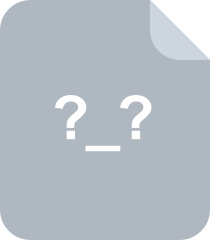
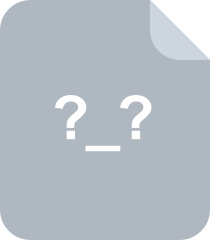
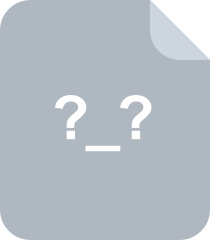
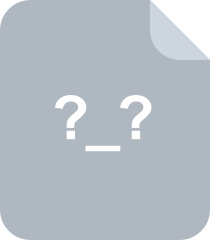
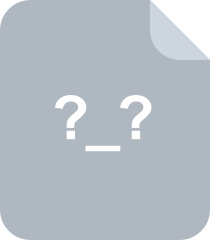
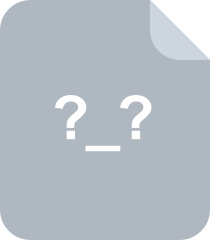
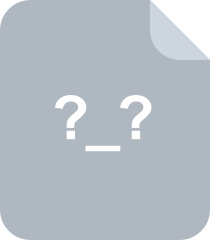
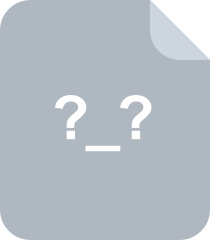
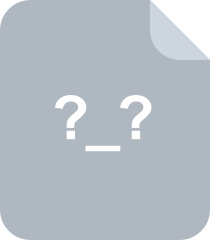
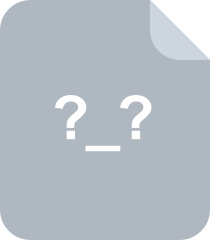
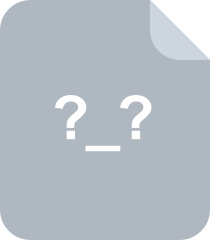
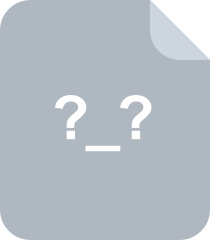
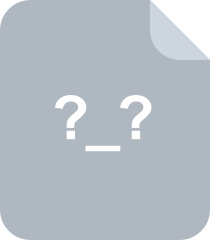
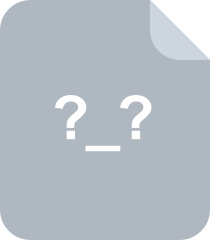
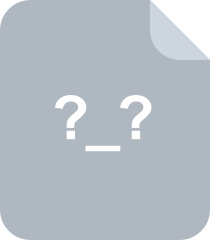
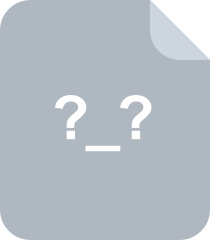
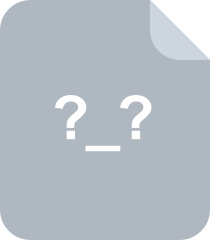
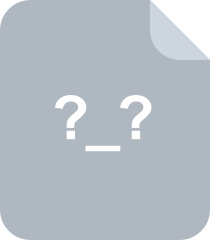
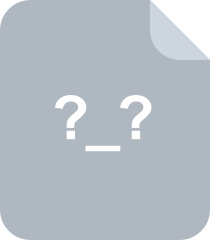
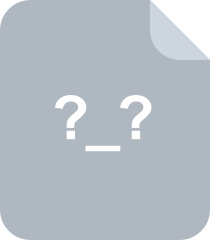
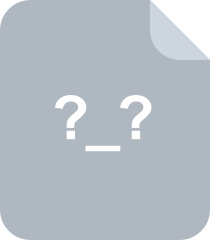
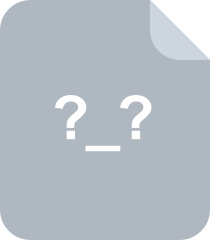
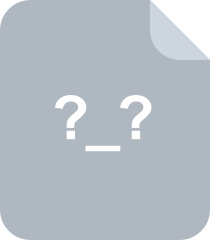
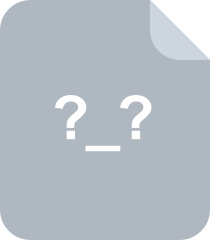
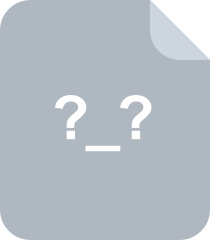
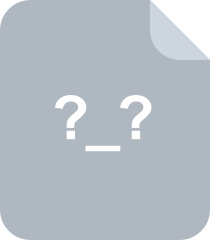
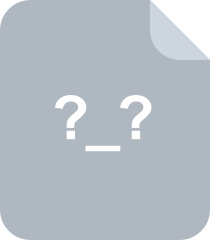
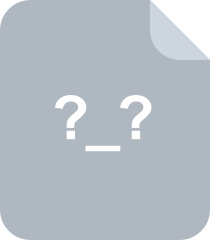
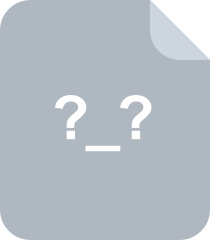
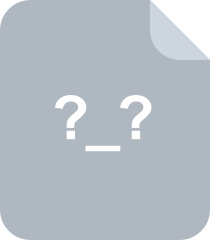
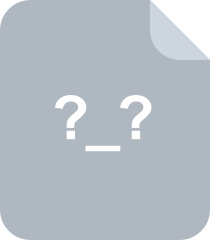
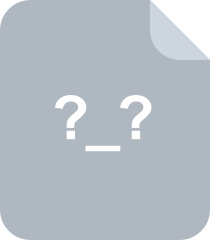
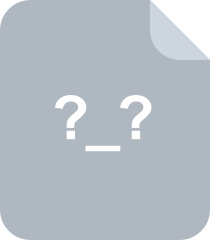
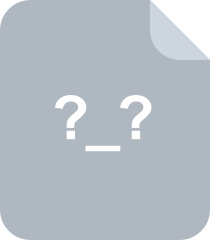
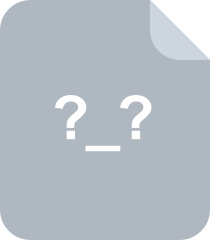
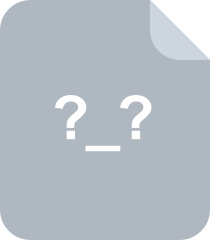
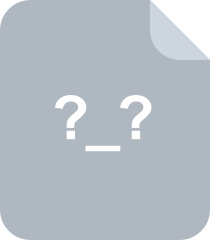
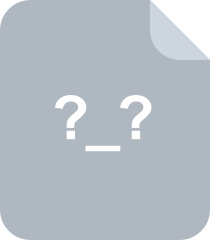
共 1373 条
- 1
- 2
- 3
- 4
- 5
- 6
- 14
资源评论
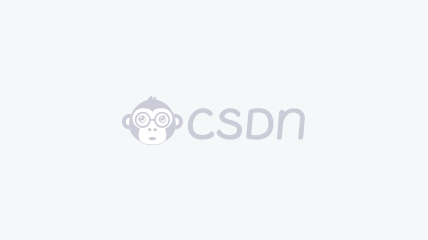

赵闪闪168
- 粉丝: 1525
- 资源: 2758
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

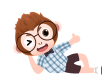
最新资源
- (源码)基于Spark的实时用户行为分析系统.zip
- (源码)基于Spring Boot和Vue的个人博客后台管理系统.zip
- 将流行的 ruby faker gem 引入 Java.zip
- (源码)基于C#和ArcGIS Engine的房屋管理系统.zip
- (源码)基于C语言的Haribote操作系统项目.zip
- (源码)基于Spring Boot框架的秒杀系统.zip
- (源码)基于Qt框架的待办事项管理系统.zip
- 将 Java 8 的 lambda 表达式反向移植到 Java 7、6 和 5.zip
- (源码)基于JavaWeb的学生管理系统.zip
- (源码)基于C++和Google Test框架的数独游戏生成与求解系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


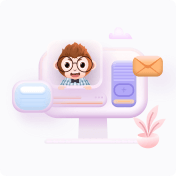
安全验证
文档复制为VIP权益,开通VIP直接复制
