# Airbnb JavaScript Style Guide() {
*A mostly reasonable approach to JavaScript*
> **Note**: this guide assumes you are using [Babel](https://babeljs.io), and requires that you use [babel-preset-airbnb](https://npmjs.com/babel-preset-airbnb) or the equivalent. It also assumes you are installing shims/polyfills in your app, with [airbnb-browser-shims](https://npmjs.com/airbnb-browser-shims) or the equivalent.
[](https://www.npmjs.com/package/eslint-config-airbnb)
[](https://www.npmjs.com/package/eslint-config-airbnb-base)
[](https://gitter.im/airbnb/javascript?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge)
This guide is available in other languages too. See [Translation](#translation)
Other Style Guides
- [ES5 (Deprecated)](https://github.com/airbnb/javascript/tree/es5-deprecated/es5)
- [React](react/)
- [CSS-in-JavaScript](css-in-javascript/)
- [CSS & Sass](https://github.com/airbnb/css)
- [Ruby](https://github.com/airbnb/ruby)
## Table of Contents
1. [Types](#types)
1. [References](#references)
1. [Objects](#objects)
1. [Arrays](#arrays)
1. [Destructuring](#destructuring)
1. [Strings](#strings)
1. [Functions](#functions)
1. [Arrow Functions](#arrow-functions)
1. [Classes & Constructors](#classes--constructors)
1. [Modules](#modules)
1. [Iterators and Generators](#iterators-and-generators)
1. [Properties](#properties)
1. [Variables](#variables)
1. [Hoisting](#hoisting)
1. [Comparison Operators & Equality](#comparison-operators--equality)
1. [Blocks](#blocks)
1. [Control Statements](#control-statements)
1. [Comments](#comments)
1. [Whitespace](#whitespace)
1. [Commas](#commas)
1. [Semicolons](#semicolons)
1. [Type Casting & Coercion](#type-casting--coercion)
1. [Naming Conventions](#naming-conventions)
1. [Accessors](#accessors)
1. [Events](#events)
1. [jQuery](#jquery)
1. [ECMAScript 5 Compatibility](#ecmascript-5-compatibility)
1. [ECMAScript 6+ (ES 2015+) Styles](#ecmascript-6-es-2015-styles)
1. [Standard Library](#standard-library)
1. [Testing](#testing)
1. [Performance](#performance)
1. [Resources](#resources)
1. [In the Wild](#in-the-wild)
1. [Translation](#translation)
1. [The JavaScript Style Guide Guide](#the-javascript-style-guide-guide)
1. [Chat With Us About JavaScript](#chat-with-us-about-javascript)
1. [Contributors](#contributors)
1. [License](#license)
1. [Amendments](#amendments)
## Types
<a name="types--primitives"></a><a name="1.1"></a>
- [1.1](#types--primitives) **Primitives**: When you access a primitive type you work directly on its value.
- `string`
- `number`
- `boolean`
- `null`
- `undefined`
- `symbol`
- `bigint`
<br />
```javascript
const foo = 1;
let bar = foo;
bar = 9;
console.log(foo, bar); // => 1, 9
```
- Symbols and BigInts cannot be faithfully polyfilled, so they should not be used when targeting browsers/environments that don’t support them natively.
<a name="types--complex"></a><a name="1.2"></a>
- [1.2](#types--complex) **Complex**: When you access a complex type you work on a reference to its value.
- `object`
- `array`
- `function`
<br />
```javascript
const foo = [1, 2];
const bar = foo;
bar[0] = 9;
console.log(foo[0], bar[0]); // => 9, 9
```
**[⬆ back to top](#table-of-contents)**
## References
<a name="references--prefer-const"></a><a name="2.1"></a>
- [2.1](#references--prefer-const) Use `const` for all of your references; avoid using `var`. eslint: [`prefer-const`](https://eslint.org/docs/rules/prefer-const), [`no-const-assign`](https://eslint.org/docs/rules/no-const-assign)
> Why? This ensures that you can’t reassign your references, which can lead to bugs and difficult to comprehend code.
```javascript
// bad
var a = 1;
var b = 2;
// good
const a = 1;
const b = 2;
```
<a name="references--disallow-var"></a><a name="2.2"></a>
- [2.2](#references--disallow-var) If you must reassign references, use `let` instead of `var`. eslint: [`no-var`](https://eslint.org/docs/rules/no-var)
> Why? `let` is block-scoped rather than function-scoped like `var`.
```javascript
// bad
var count = 1;
if (true) {
count += 1;
}
// good, use the let.
let count = 1;
if (true) {
count += 1;
}
```
<a name="references--block-scope"></a><a name="2.3"></a>
- [2.3](#references--block-scope) Note that both `let` and `const` are block-scoped, whereas `var` is function-scoped.
```javascript
// const and let only exist in the blocks they are defined in.
{
let a = 1;
const b = 1;
var c = 1;
}
console.log(a); // ReferenceError
console.log(b); // ReferenceError
console.log(c); // Prints 1
```
In the above code, you can see that referencing `a` and `b` will produce a ReferenceError, while `c` contains the number. This is because `a` and `b` are block scoped, while `c` is scoped to the containing function.
**[⬆ back to top](#table-of-contents)**
## Objects
<a name="objects--no-new"></a><a name="3.1"></a>
- [3.1](#objects--no-new) Use the literal syntax for object creation. eslint: [`no-new-object`](https://eslint.org/docs/rules/no-new-object)
```javascript
// bad
const item = new Object();
// good
const item = {};
```
<a name="es6-computed-properties"></a><a name="3.4"></a>
- [3.2](#es6-computed-properties) Use computed property names when creating objects with dynamic property names.
> Why? They allow you to define all the properties of an object in one place.
```javascript
function getKey(k) {
return `a key named ${k}`;
}
// bad
const obj = {
id: 5,
name: 'San Francisco',
};
obj[getKey('enabled')] = true;
// good
const obj = {
id: 5,
name: 'San Francisco',
[getKey('enabled')]: true,
};
```
<a name="es6-object-shorthand"></a><a name="3.5"></a>
- [3.3](#es6-object-shorthand) Use object method shorthand. eslint: [`object-shorthand`](https://eslint.org/docs/rules/object-shorthand)
```javascript
// bad
const atom = {
value: 1,
addValue: function (value) {
return atom.value + value;
},
};
// good
const atom = {
value: 1,
addValue(value) {
return atom.value + value;
},
};
```
<a name="es6-object-concise"></a><a name="3.6"></a>
- [3.4](#es6-object-concise) Use property value shorthand. eslint: [`object-shorthand`](https://eslint.org/docs/rules/object-shorthand)
> Why? It is shorter and descriptive.
```javascript
const lukeSkywalker = 'Luke Skywalker';
// bad
const obj = {
lukeSkywalker: lukeSkywalker,
};
// good
const obj = {
lukeSkywalker,
};
```
<a name="objects--grouped-shorthand"></a><a name="3.7"></a>
- [3.5](#objects--grouped-shorthand) Group your shorthand properties at the beginning of your object declaration.
> Why? It’s easier to tell which properties are using the shorthand.
```javascript
const anakinSkywalker = 'Anakin Skywalker';
const lukeSkywalker = 'Luke Skywalker';
// bad
const obj = {
episodeOne: 1,
twoJediWalkIntoACantina: 2,
lukeSkywalker,
episodeThree: 3,
mayTheFourth: 4,
anakinSkywalker,
};
// good
const obj = {
lukeSkywalker,
anakinSkywalker,
episodeOne: 1,
twoJediWalkIntoACantina: 2,
episodeThree: 3,
mayTheFourth: 4,
};
```
<a name="objects--quoted-props"></a><a name="3.8"></a>
- [3.6](#objects--quoted-props) Only quote properties tha
没有合适的资源?快使用搜索试试~ 我知道了~
JavaScript 样式指南.zip
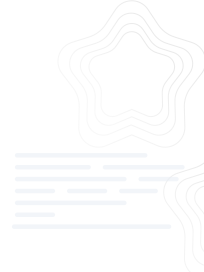
共64个文件
js:28个
md:10个
eslintrc:5个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 137 浏览量
2024-11-24
23:52:18
上传
评论
收藏 108KB ZIP 举报
温馨提示
Airbnb JavaScript 样式指南() {一种最合理的 JavaScript 方法注意本指南假设您正在使用Babel,并要求您使用babel-preset-airbnb或等效程序。它还假设您正在使用airbnb-browser-shims或等效程序在应用中安装 shims/polyfills 。 本指南还提供其他语言版本。请参阅翻译其他风格指南ES5(已弃用)反应JavaScript 中的 CSSCSS 和 Sass红宝石目录类型参考对象数组解构字符串功能箭头函数类和构造函数模块迭代器和生成器特性变量提升比较运算符和相等性区块控制语句评论空格逗号分号类型转换和强制命名约定访问器活动jQueryECMAScript 5 兼容性ECMAScript 6+ (ES 2015+) 样式标准库测试表现资源在野外翻译JavaScript 风格指南与我们讨论 JavaScript贡献者执照修订类型1.1 原始类型当您访问原始类型时,您直接对其值进行操作。string
资源推荐
资源详情
资源评论
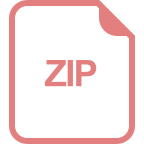
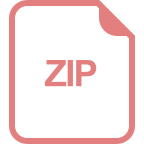
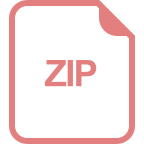
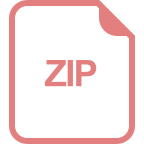
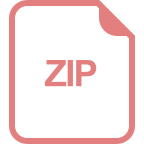
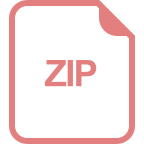
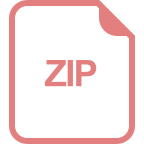
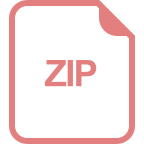
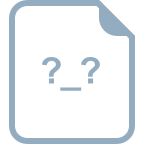
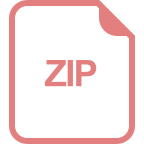
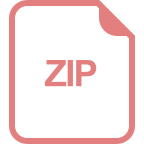
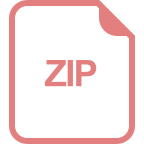
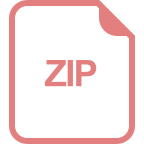
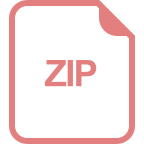
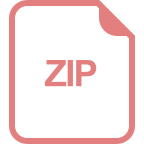
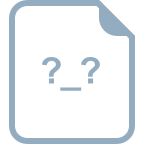
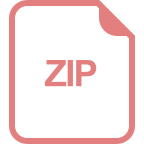
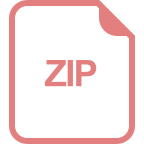
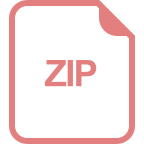
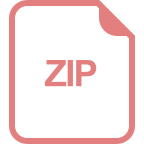
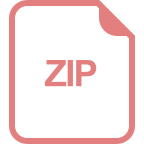
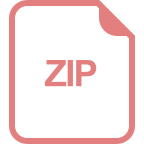
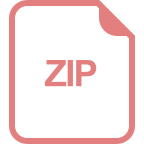
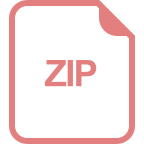
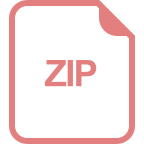
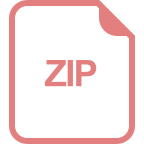
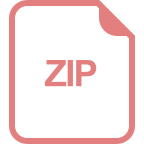
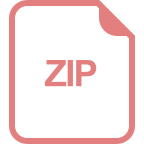
收起资源包目录

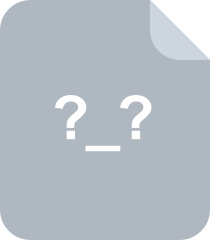
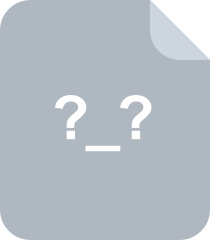


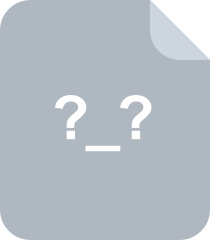
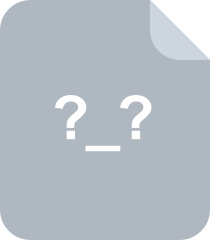
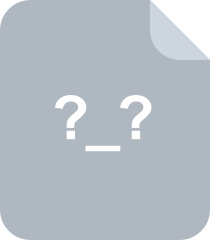
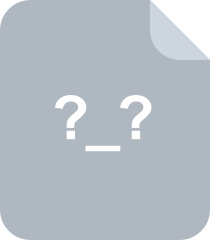
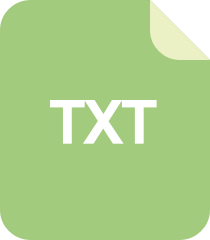

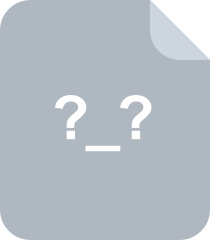
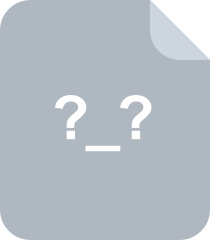
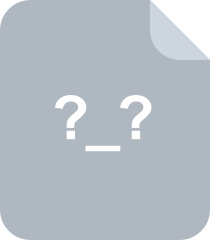

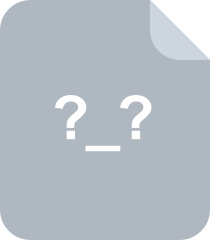
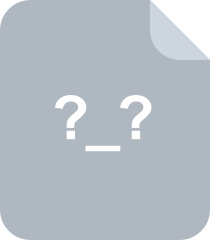
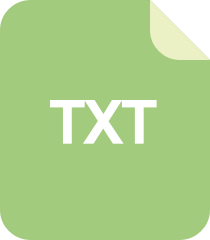

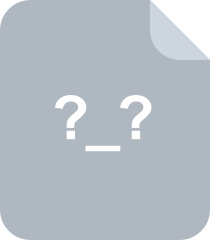
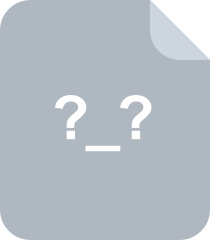
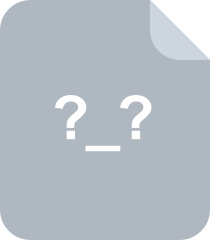
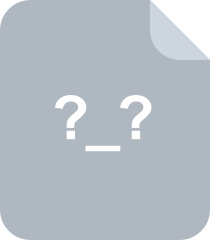

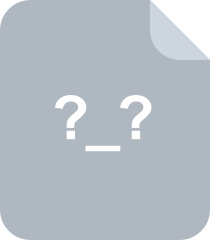


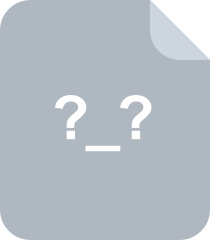
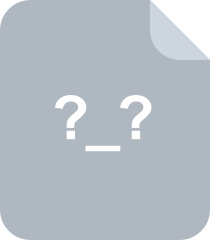
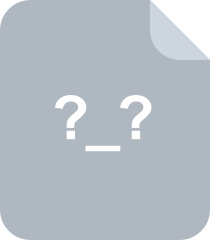
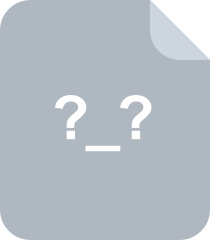
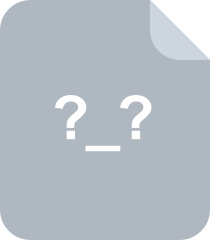

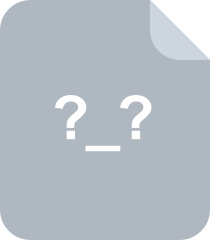
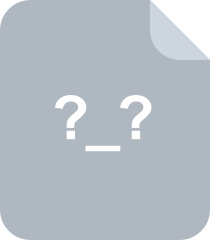
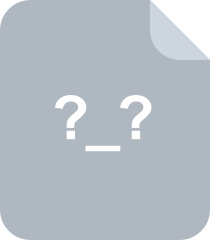
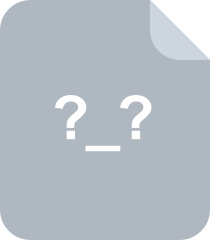
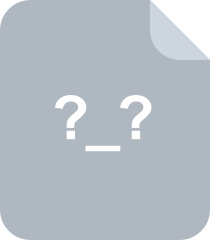
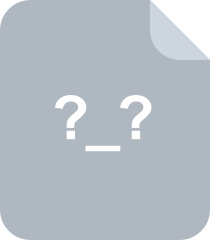
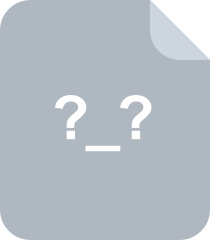
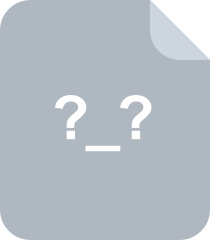
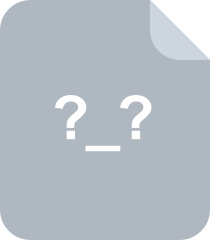
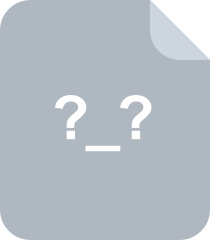
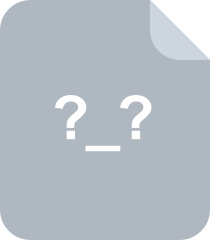
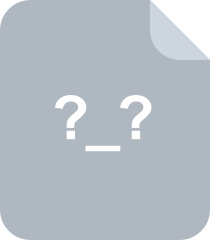
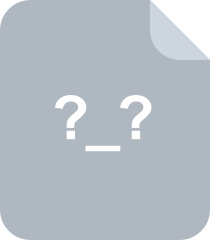

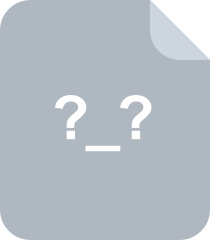
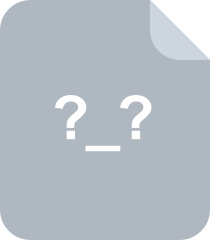
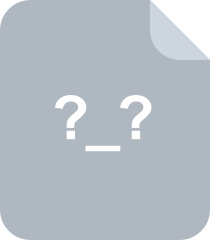
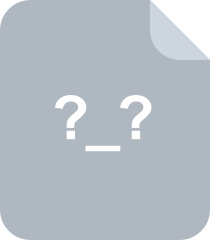
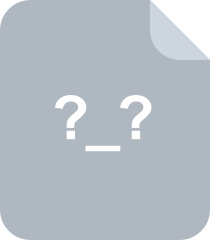
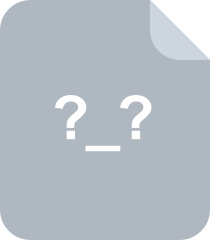

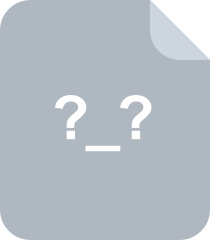
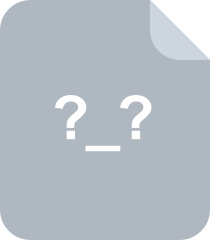
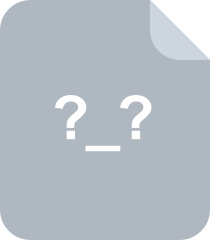
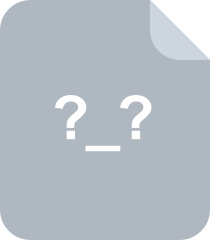
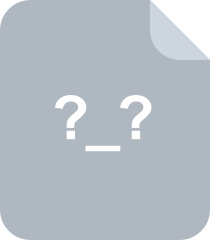
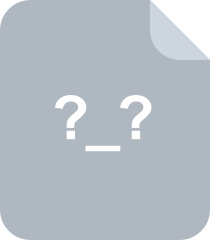

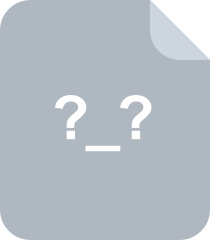
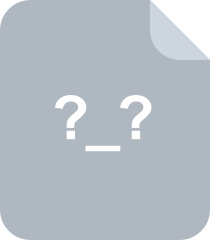
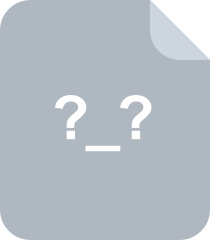
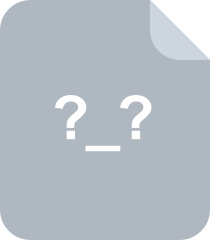
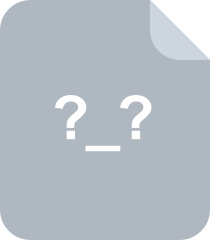
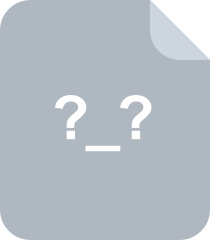
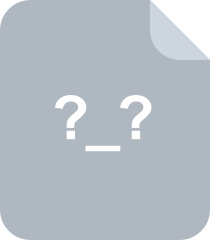
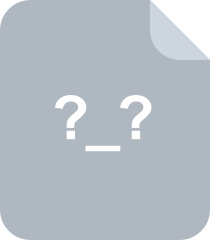

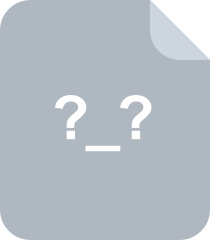
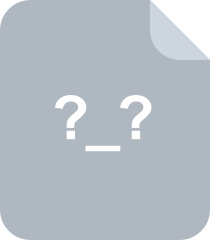
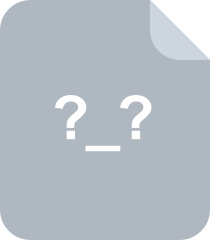
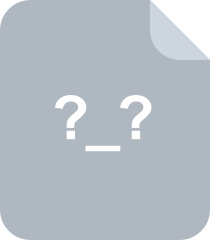
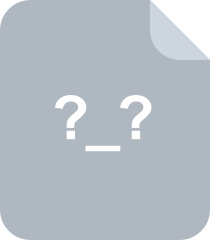
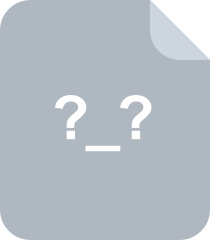
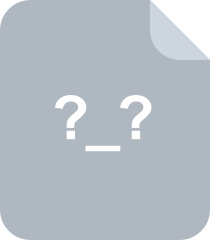
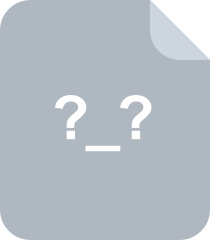
共 64 条
- 1
资源评论
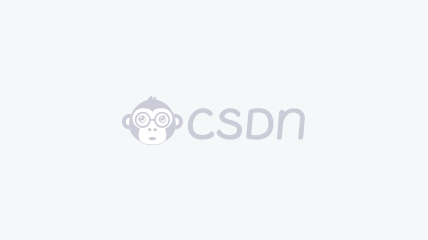

赵闪闪168
- 粉丝: 1533
- 资源: 2758
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

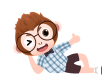
最新资源
- 从 Python 访问 Java 类.zip
- 交互式 JavaScript 沙箱.zip
- 交互式 JavaScript API 参考.zip
- 使用SSM框架的Java Web项目-电商后台管理.zip
- 与 FrontendMasters 课程 JavaScript 和 React 模式相关的 repo.zip
- win11系统有ie浏览器,打开ie浏览器自动跳转edge浏览器解决方案
- 基于Spark的新闻推荐系统源码+文档说明(高分项目)
- 27个常用分布函数详细汇总-名称+类别+用途+概率密度曲线+公式-PPT版本
- Python毕业设计基于时空图卷积ST-GCN的骨骼动作识别项目源码+文档说明(高分项目)
- 一个易于使用的多线程库,用于用 Java 创建 Discord 机器人 .zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


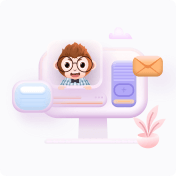
安全验证
文档复制为VIP权益,开通VIP直接复制
