根据提供的文件信息,我们可以归纳出一系列与C语言相关的知识点,主要涉及不同的函数及其用途。下面将详细介绍这些函数的功能、参数及使用示例。 ### C语言函数大全 #### 1. `abort` - **功能**:该函数用于异常终止程序。 - **原型**:`void abort(void);` - **说明**:`abort`函数会立即终止调用它的进程,并且不执行任何清理操作(如关闭打开的文件)。通常用于处理不可恢复的错误情况。 - **示例**: ```c #include <stdio.h> #include <stdlib.h> int main(void) { printf("Calling abort()\n"); abort(); return 0; /* This line will never be reached */ } ``` #### 2. `abs` - **功能**:计算整数的绝对值。 - **原型**:`int abs(int i);` - **说明**:返回传入整数的绝对值。 - **示例**: ```c #include <stdio.h> #include <math.h> int main(void) { int number = -1234; printf("Number: %d Absolute Value: %d\n", number, abs(number)); return 0; } ``` #### 3. `absread`, `abswrite` - **功能**:这两个函数用于磁盘扇区级别的读写操作。 - **原型**:`int absread(int drive, int nsects, int sectno, void *buffer);` 和 `int abswrite(int drive, int nsects, int sectno, void *buffer);` - **说明**:`absread`用于从指定磁盘驱动器读取一个或多个扇区的数据到缓冲区;`abswrite`用于将缓冲区中的数据写入指定磁盘驱动器的一个或多个扇区。 - **示例**: ```c #include <stdio.h> #include <conio.h> #include <process.h> #include <dos.h> int main(void) { int i, strt, ch_out, sector; char buf[512]; printf("Insert a diskette into drive A and press any key\n"); getch(); sector = 0; if (absread(0, 1, sector, &buf) != 0) { perror("Disk problem"); exit(1); } printf("Read OK\n"); strt = 3; for (i = 0; i < 80; i++) { ch_out = buf[strt + i]; putchar(ch_out); } printf("\n"); return 0; } ``` #### 4. `access` - **功能**:检查文件的访问权限。 - **原型**:`int access(const char *filename, int amode);` - **说明**:该函数用于确定当前进程是否能够对指定文件执行特定类型的访问。`amode`参数可以是以下常量之一或它们的按位或组合:`F_OK`、`R_OK`、`W_OK`或`X_OK`,分别表示文件存在、可读、可写和可执行。 - **示例**: ```c #include <stdio.h> #include <io.h> int file_exists(char *filename); int main(void) { printf("Does NOTEXISTS.FIL exist: %s\n", file_exists("NOTEXISTS.FIL") ? "YES" : "NO"); return 0; } int file_exists(char *filename) { return (access(filename, 0) == 0); } ``` #### 5. `acos` - **功能**:计算反余弦值。 - **原型**:`double acos(double x);` - **说明**:返回以弧度表示的参数`x`的反余弦值。`x`必须在-1到1之间。 - **示例**: ```c #include <stdio.h> #include <math.h> int main(void) { double result; double x = 0.5; result = acos(x); printf("The arccosine of %lf is %lf\n", x, result); return 0; } ``` #### 6. `allocmem` - **功能**:分配内存段。 - **原型**:`int allocmem(unsigned size, unsigned *seg);` - **说明**:此函数用于在DOS环境下分配内存。`size`参数指定要分配的内存段大小(以段为单位),`seg`参数是指向变量的指针,该变量接收分配内存的段地址。 - **示例**: ```c #include <dos.h> #include <alloc.h> #include <stdio.h> int main(void) { unsigned int size, segp; int stat; size = 64; /* (64x16) = 1024 bytes */ stat = allocmem(size, &segp); if (stat == -1) printf("Allocated memory at segment: %x\n", segp); else printf("Failed: maximum number of paragraphs available is %u\n", stat); return 0; } ``` #### 7. `arc` - **功能**:绘制圆弧。 - **原型**:`void far arc(int x, int y, int stangle, int endangle, int radius);` - **说明**:该函数用于在图形模式下绘制一个圆弧。`x`和`y`参数指定圆心位置,`stangle`和`endangle`分别指定起始角度和结束角度(以度为单位),`radius`参数指定圆的半径。 - **示例**: ```c #include <graphics.h> #include <stdlib.h> #include <stdio.h> #include <conio.h> int main(void) { int gdriver = DETECT, gmode, errorcode; int midx, midy; int stangle = 45, endangle = 135; int radius = 100; /* Initialize graphics and local variables */ initgraph(&gdriver ``` 以上列举了C语言中常见的几个函数,包括异常终止(`abort`)、绝对值计算(`abs`)、磁盘扇区读写(`absread`, `abswrite`)、文件访问权限检查(`access`)、反余弦计算(`acos`)、内存分配(`allocmem`)以及图形绘制(`arc`)等。这些函数在实际编程中非常实用,能够帮助开发者实现各种功能需求。
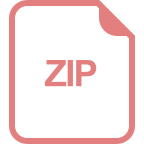
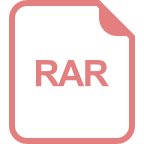
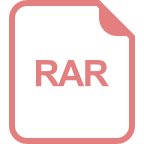
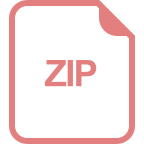
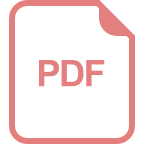
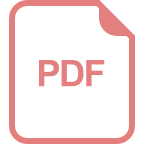
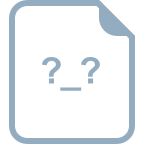
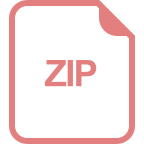
函数名: abort
功 能: 异常终止一个进程
用 法: void abort(void);
程序例:
#include <stdio.h>
#include <stdlib.h>
int main(void)
{
printf("Calling abort()\n");
abort();
return 0; /* This is never reached */
}
函数名: abs
功 能: 求整数的绝对值
用 法: int abs(int i);
程序例:
#include <stdio.h>
#include <math.h>
int main(void)
{
int number = -1234;
printf("number: %d absolute value: %d\n", number, abs(number));
}
函数名: absread, abswirte
功 能: 绝对磁盘扇区读、写数据
用 法: int absread(int drive, int nsects, int sectno, void *buffer);
int abswrite(int drive, int nsects, in tsectno, void *buffer);
程序例:
/* absread example */
#include <stdio.h>
#include <conio.h>
#include <process.h>
#include <dos.h>
int main(void)
{
int i, strt, ch_out, sector;
char buf[512];
printf("Insert a diskette into drive A and press any key\n");
getch();
sector = 0;
if (absread(0, 1, sector, &buf) != 0)
{
perror("Disk problem");
exit(1);
}
剩余13页未读,继续阅读
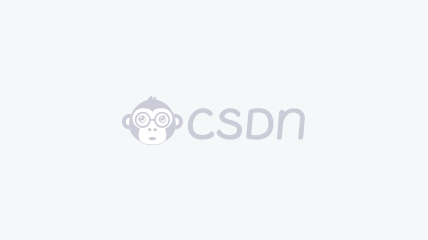

- 粉丝: 0
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

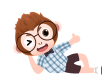
最新资源
- json的合法基色来自红包东i请各位
- 项目采用YOLO V4算法模型进行目标检测,使用Deep SORT目标跟踪算法 .zip
- 针对实时视频流和静态图像实现的对象检测和跟踪算法 .zip
- 部署 yolox 算法使用 deepstream.zip
- 基于webmagic、springboot和mybatis的MagicToe Java爬虫设计源码
- 通过实时流协议 (RTSP) 使用 Yolo、OpenCV 和 Python 进行深度学习的对象检测.zip
- 基于Python和HTML的tb商品列表查询分析设计源码
- 基于国民技术RT-THREAD的MULTInstrument多功能电子测量仪器设计源码
- 基于Java技术的网络报修平台后端设计源码
- 基于Python的美食杰中华菜系数据挖掘与分析设计源码

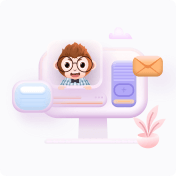
