// validat0.cpp - originally written and placed in the public domain by Wei Dai and Jeffrey Walton
// Routines in this source file are only tested in Debug builds
#include "pch.h"
#include "secblock.h"
#include "integer.h"
#include "nbtheory.h"
#include "zdeflate.h"
#include "filters.h"
#include "stdcpp.h"
#include "default.h"
#include "zinflate.h"
#include "channels.h"
#include "files.h"
#include "gf2n.h"
#include "gzip.h"
#include "zlib.h"
#include "ida.h"
#include "hex.h"
#include "asn.h"
#include <iostream>
#include <iomanip>
#include <sstream>
#include "validate.h"
// Aggressive stack checking with VS2005 SP1 and above.
#if (_MSC_FULL_VER >= 140050727)
# pragma strict_gs_check (on)
#endif
#if CRYPTOPP_MSC_VERSION
# pragma warning(disable: 4505 4355)
#endif
NAMESPACE_BEGIN(CryptoPP)
NAMESPACE_BEGIN(Test)
#if defined(CRYPTOPP_EXTENDED_VALIDATION)
// Issue 64: "PolynomialMod2::operator<<=", http://github.com/weidai11/cryptopp/issues/64
bool TestPolynomialMod2()
{
bool pass1 = true, pass2 = true, pass3 = true;
std::cout << "\nTesting PolynomialMod2 bit operations...\n\n";
static const unsigned int start = 0;
static const unsigned int stop = 4 * WORD_BITS + 1;
for (unsigned int i = start; i < stop; i++)
{
PolynomialMod2 p(1);
p <<= i;
Integer n(Integer::One());
n <<= i;
std::ostringstream oss1;
oss1 << p;
std::string str1, str2;
// str1 needs the commas removed used for grouping
str1 = oss1.str();
str1.erase(std::remove(str1.begin(), str1.end(), ','), str1.end());
// str1 needs the trailing 'b' removed
str1.erase(str1.end() - 1);
// str2 is fine as-is
str2 = IntToString(n, 2);
pass1 &= (str1 == str2);
}
for (unsigned int i = start; i < stop; i++)
{
const word w((word)SIZE_MAX);
PolynomialMod2 p(w);
p <<= i;
Integer n(Integer::POSITIVE, static_cast<lword>(w));
n <<= i;
std::ostringstream oss1;
oss1 << p;
std::string str1, str2;
// str1 needs the commas removed used for grouping
str1 = oss1.str();
str1.erase(std::remove(str1.begin(), str1.end(), ','), str1.end());
// str1 needs the trailing 'b' removed
str1.erase(str1.end() - 1);
// str2 is fine as-is
str2 = IntToString(n, 2);
pass2 &= (str1 == str2);
}
RandomNumberGenerator& prng = GlobalRNG();
for (unsigned int i = start; i < stop; i++)
{
word w; // Cast to lword due to Visual Studio
prng.GenerateBlock((byte*)&w, sizeof(w));
PolynomialMod2 p(w);
p <<= i;
Integer n(Integer::POSITIVE, static_cast<lword>(w));
n <<= i;
std::ostringstream oss1;
oss1 << p;
std::string str1, str2;
// str1 needs the commas removed used for grouping
str1 = oss1.str();
str1.erase(std::remove(str1.begin(), str1.end(), ','), str1.end());
// str1 needs the trailing 'b' removed
str1.erase(str1.end() - 1);
// str2 is fine as-is
str2 = IntToString(n, 2);
if (str1 != str2)
{
std::cout << " Oops..." << "\n";
std::cout << " random: " << std::hex << n << std::dec << "\n";
std::cout << " str1: " << str1 << "\n";
std::cout << " str2: " << str2 << "\n";
}
pass3 &= (str1 == str2);
}
std::cout << (!pass1 ? "FAILED" : "passed") << ": " << "1 shifted over range [" << std::dec << start << "," << stop << "]" << "\n";
std::cout << (!pass2 ? "FAILED" : "passed") << ": " << "0x" << std::hex << word(SIZE_MAX) << std::dec << " shifted over range [" << start << "," << stop << "]" << "\n";
std::cout << (!pass3 ? "FAILED" : "passed") << ": " << "random values shifted over range [" << std::dec << start << "," << stop << "]" << "\n";
return pass1 && pass2 && pass3;
}
#endif
#if defined(CRYPTOPP_EXTENDED_VALIDATION)
bool TestCompressors()
{
std::cout << "\nTesting Compressors and Decompressors...\n\n";
bool fail1 = false, fail2 = false, fail3 = false;
try
{
// Gzip uses Adler32 checksums. We expect a failure to to happen on occasion.
// If we see more than 2 failures in a run of 128, then we need to investigate.
unsigned int truncatedCount=0;
for (unsigned int i = 0; i<128; ++i)
{
std::string src, dest, rec;
unsigned int len = GlobalRNG().GenerateWord32(4, 0xfff);
RandomNumberSource(GlobalRNG(), len, true, new StringSink(src));
StringSource(src, true, new Gzip(new StringSink(dest)));
StringSource(dest, true, new Gunzip(new StringSink(rec)));
if (src != rec)
throw Exception(Exception::OTHER_ERROR, "Gzip failed to decompress stream");
// Tamper
try {
StringSource(dest.substr(0, len - 2), true, new Gunzip(new StringSink(rec)));
if (truncatedCount++ >= 2)
{
std::cout << "FAILED: Gzip failed to detect a truncated stream\n";
fail1 = true;
}
}
catch (const Exception&) {}
}
}
catch (const Exception& ex)
{
std::cout << "FAILED: " << ex.what() << "\n";
fail1 = true;
}
// **************************************************************
// Gzip Filename, Filetime and Comment
try
{
std::string filename = "test.txt";
std::string comment = "This is a test";
word32 filetime = GlobalRNG().GenerateWord32(4, 0xffffff);
AlgorithmParameters params = MakeParameters(Name::FileTime(), (int)filetime)
(Name::FileName(), ConstByteArrayParameter(filename.c_str(), false))
(Name::Comment(), ConstByteArrayParameter(comment.c_str(), false));
std::string src, dest;
unsigned int len = GlobalRNG().GenerateWord32(4, 0xfff);
RandomNumberSource(GlobalRNG(), len, true, new StringSink(src));
Gunzip unzip(new StringSink(dest));
StringSource(src, true, new Gzip(params, new Redirector(unzip)));
if (filename != unzip.GetFilename())
throw Exception(Exception::OTHER_ERROR, "Failed to retrieve filename");
if (filetime != unzip.GetFiletime())
throw Exception(Exception::OTHER_ERROR, "Failed to retrieve filetime");
if (comment != unzip.GetComment())
throw Exception(Exception::OTHER_ERROR, "Failed to retrieve comment");
std::cout << "passed: filenames, filetimes and comments\n";
}
catch (const Exception& ex)
{
std::cout << "FAILED: " << ex.what() << "\n";
}
// Unzip random data. See if we can induce a crash
for (unsigned int i = 0; i<128; i++)
{
SecByteBlock src;
unsigned int len = GlobalRNG().GenerateWord32(4, 0xfff);
RandomNumberSource(GlobalRNG(), len, true, new ArraySink(src, src.size()));
try {
ArraySource(src.data(), src.size(), true, new Gunzip(new Redirector(TheBitBucket())));
}
catch (const Exception&) {}
}
// Unzip random data. See if we can induce a crash
for (unsigned int i = 0; i<128; i++)
{
SecByteBlock src;
unsigned int len = GlobalRNG().GenerateWord32(4, 0xfff);
src.resize(len);
RandomNumberSource(GlobalRNG(), len, true, new ArraySink(src, src.size()));
src[0] = (byte)0x1f; // magic header
src[1] = (byte)0x8b;
src[2] = 0x00; // extra flags
src[3] = sr
没有合适的资源?快使用搜索试试~ 我知道了~
cryptopp 源码
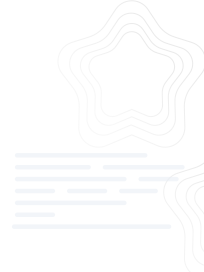
共357个文件
cpp:165个
h:163个
filters:4个

需积分: 22 9 下载量 194 浏览量
2019-01-23
14:17:44
上传
评论
收藏 1.17MB ZIP 举报
温馨提示
在cryptopp700 版本中 引入CryptoPPLib.pro 在qt 中直接依赖使用 减少编译带来的问题 https://github.com/weidai11/cryptopp https://github.com/AlexFdv/qt_cryptopp_pro
资源推荐
资源详情
资源评论
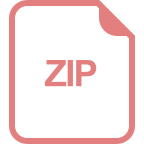
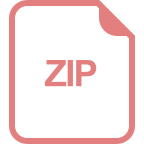
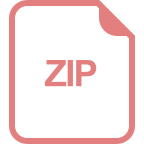
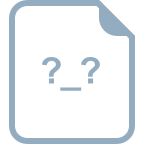
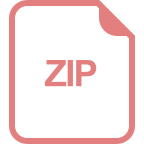
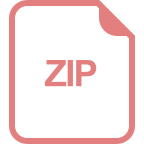
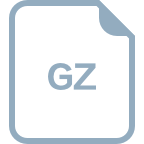
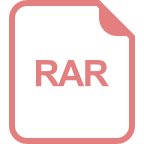
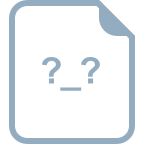
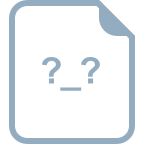
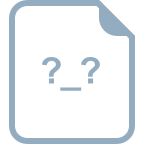
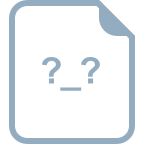
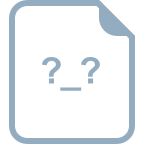
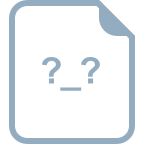
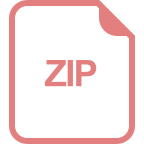
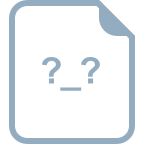
收起资源包目录

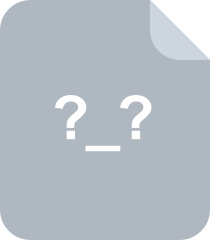
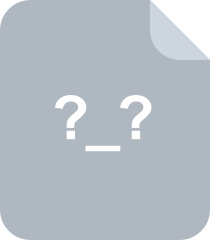
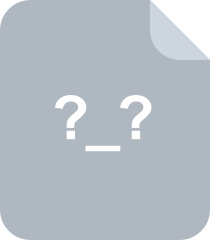
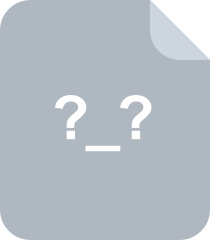
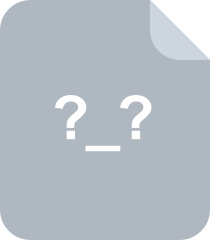
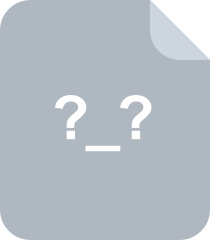
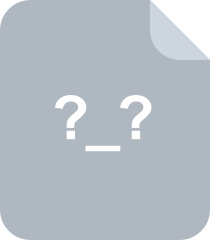
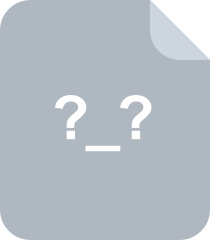
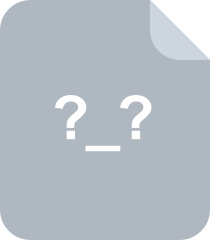
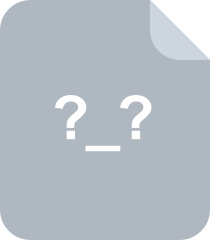
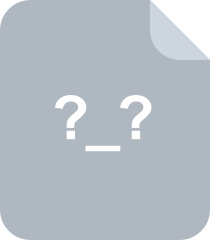
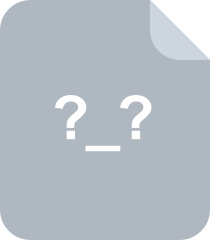
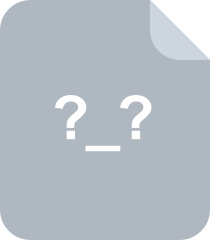
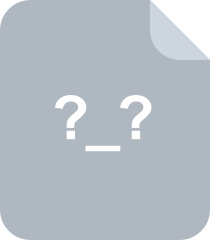
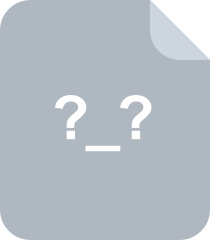
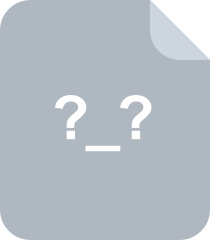
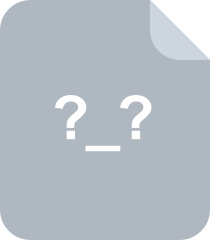
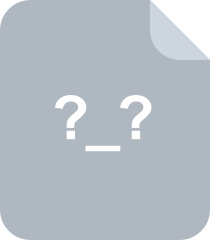
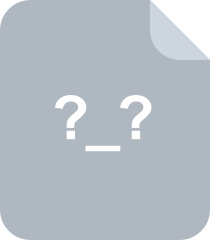
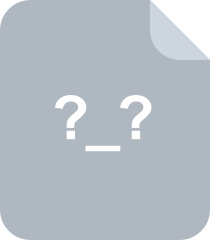
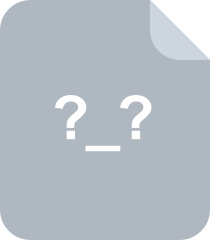
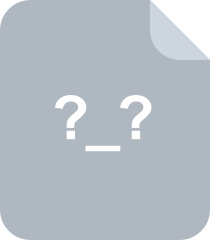
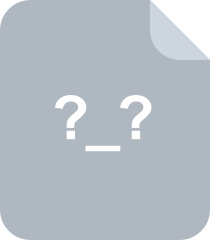
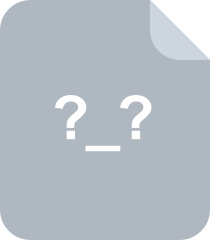
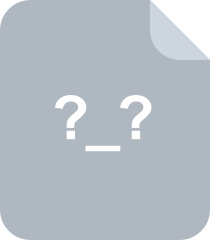
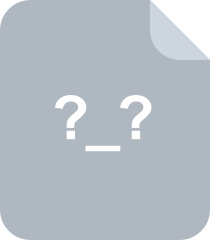
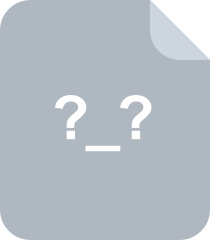
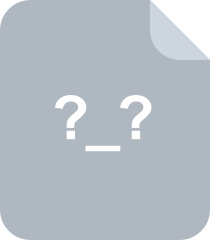
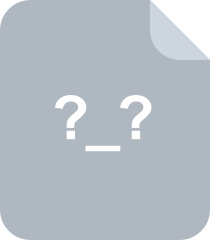
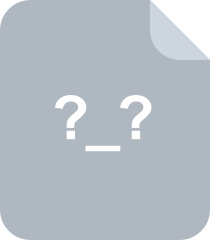
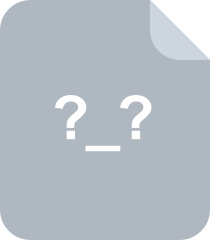
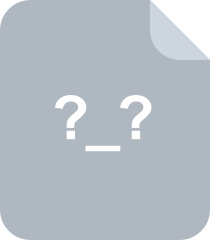
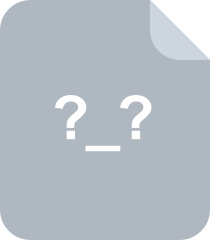
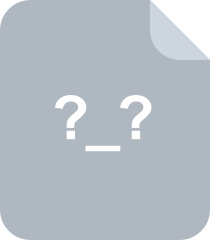
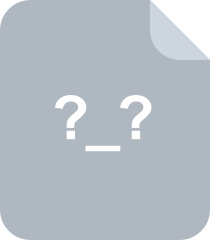
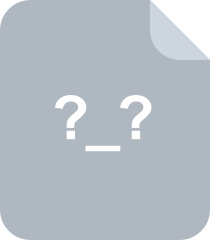
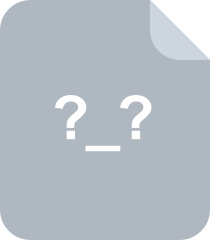
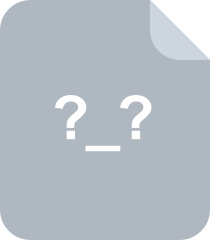
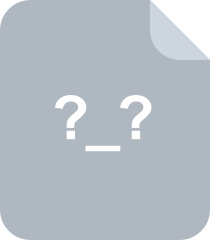
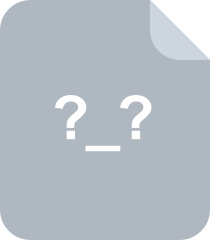
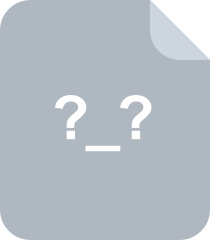
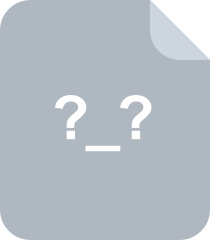
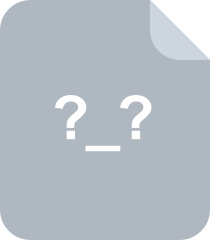
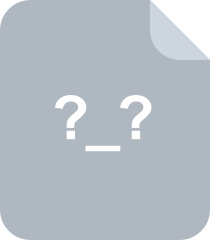
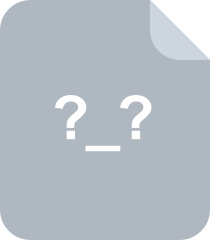
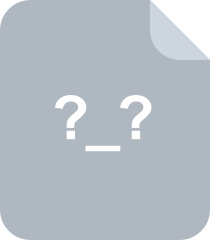
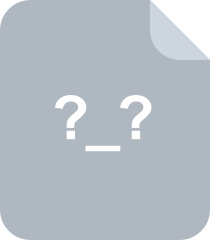
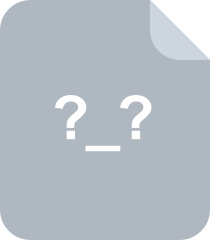
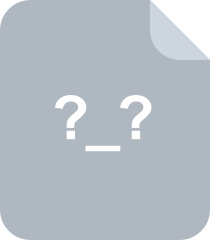
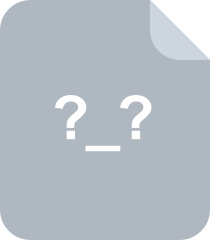
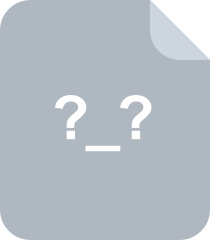
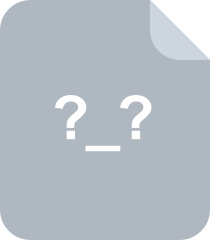
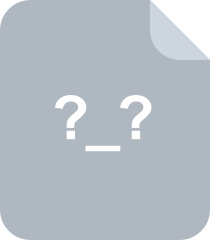
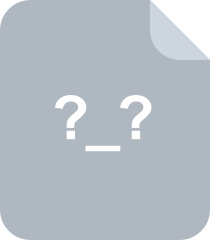
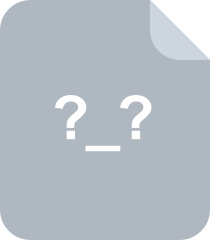
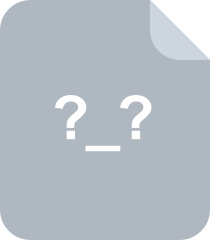
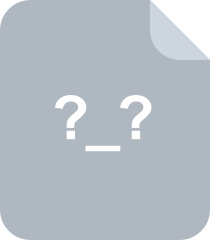
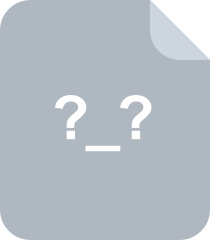
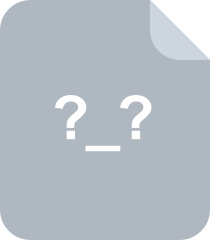
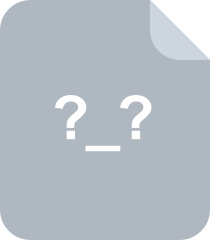
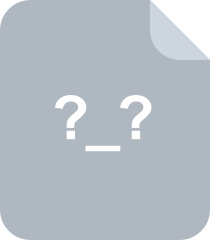
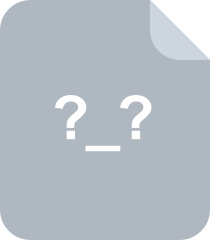
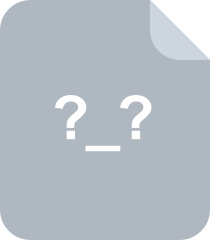
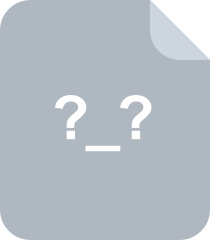
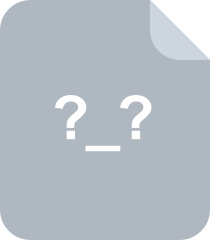
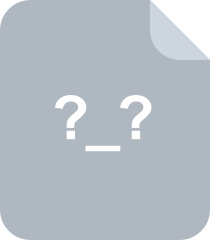
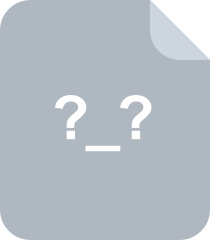
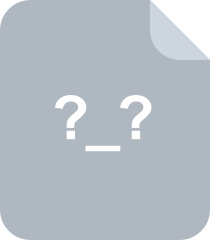
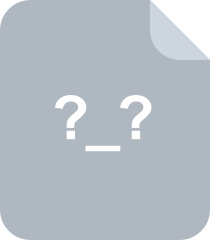
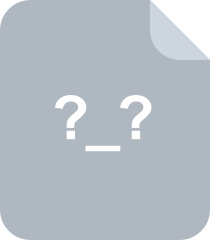
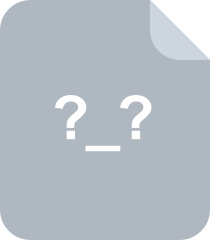
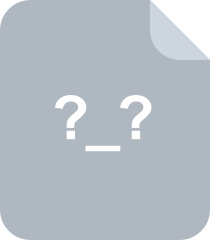
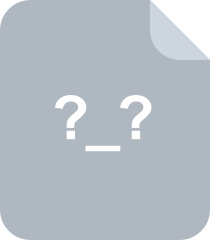
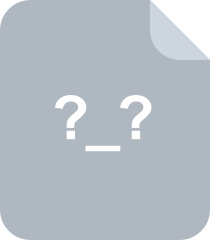
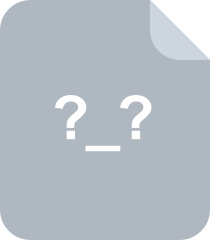
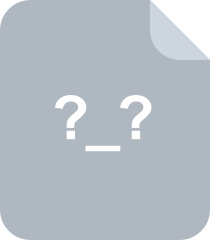
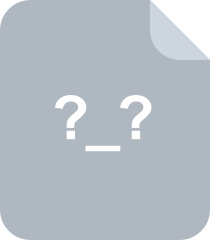
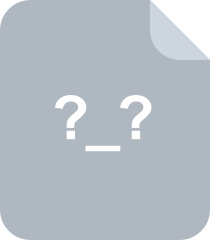
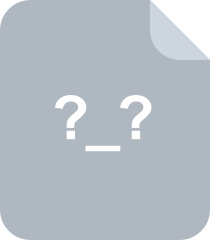
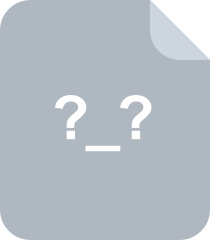
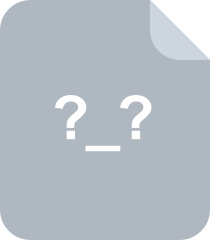
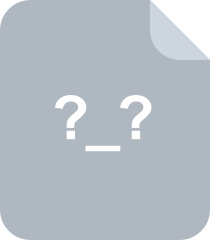
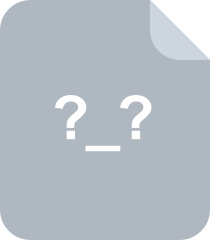
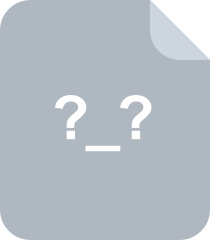
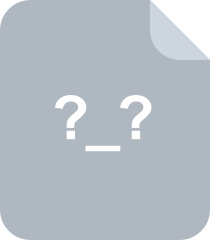
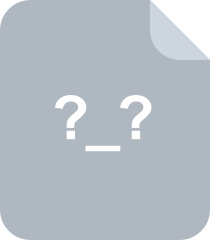
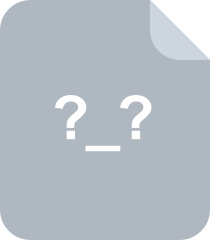
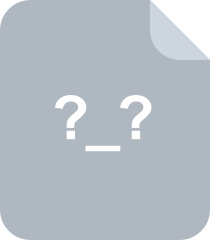
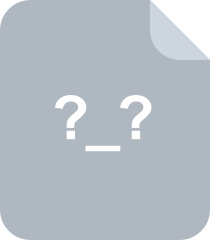
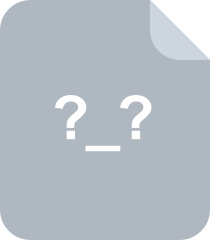
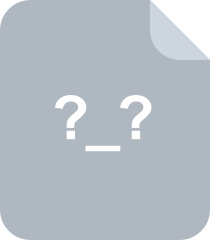
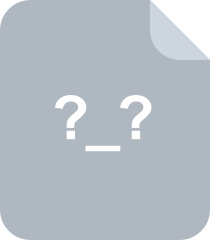
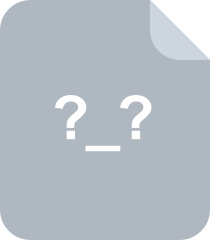
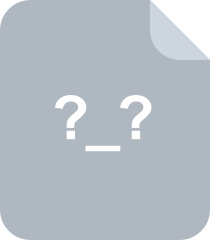
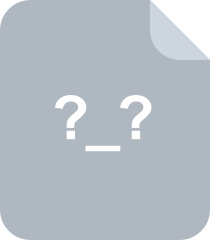
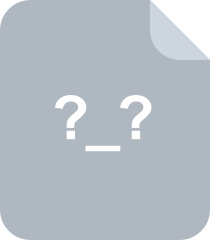
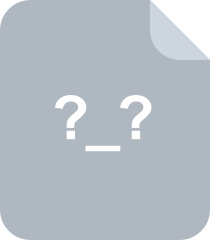
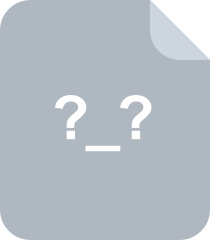
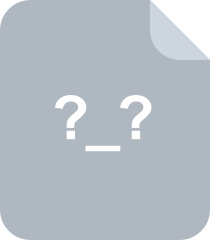
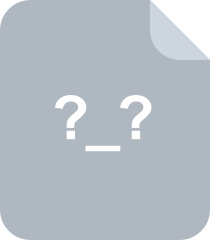
共 357 条
- 1
- 2
- 3
- 4
资源评论
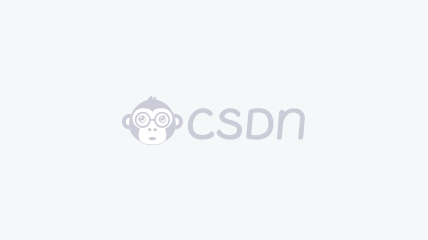

zhangshu7573256
- 粉丝: 0
- 资源: 10
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

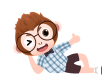
最新资源
- spark实验所需要的资料
- 414.基于SpringBoot的高校心理教育辅导系统(含报告).zip
- 多线程知乎用户爬虫,基于python3
- 412.基于SpringBoot的高校危化试剂仓储系统(含报告).zip
- Logic-2.4.9-windows-x64
- android TV 开发框架: 包含 移动的边框,键盘,标题栏
- 411.基于SpringBoot的高校实习管理系统(含报告).zip
- 410.基于SpringBoot的高校科研信息管理系统(含报告).zip
- 附件1.植物健康状态的影响指标数据.xlsx
- Windows 10 1507-x86 .NET Framework 3.5(包括.NET 2.0和3.0)安装包
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


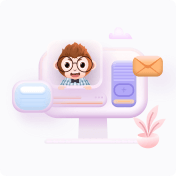
安全验证
文档复制为VIP权益,开通VIP直接复制
