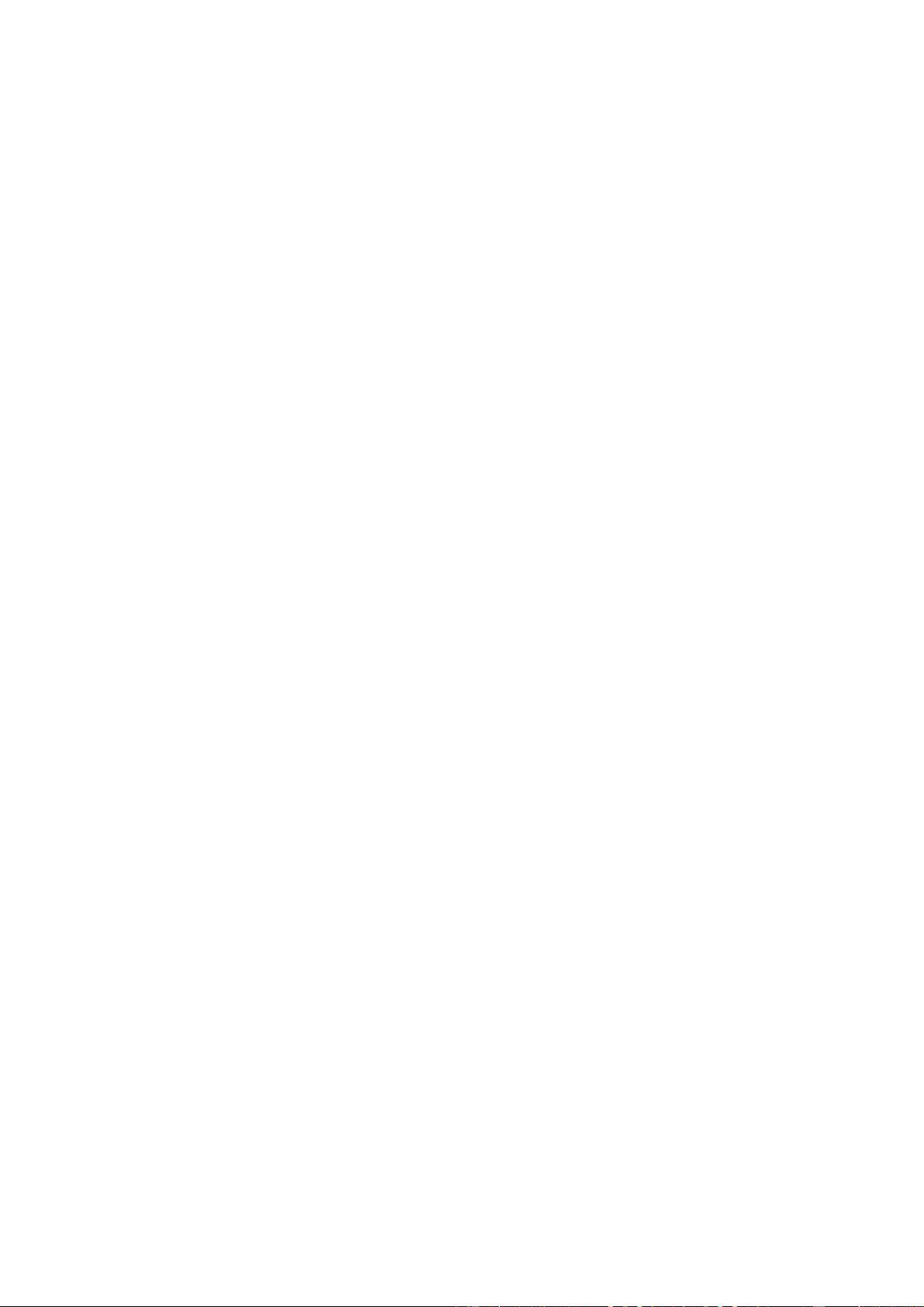
HttpClient Tutorial
Oleg Kalnichevski
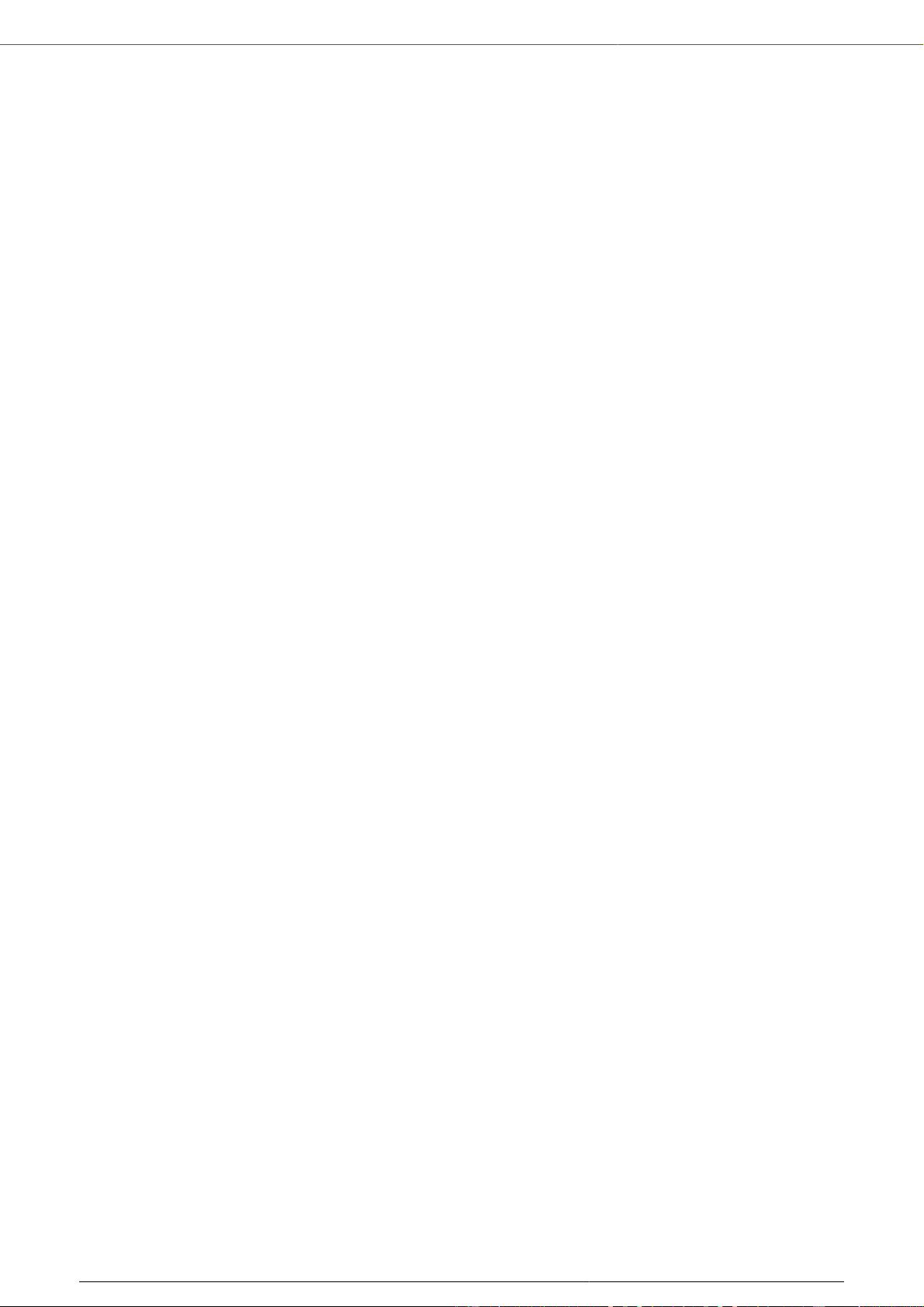
ii
Preface ................................................................................................................................... iv
1. HttpClient scope ......................................................................................................... iv
2. What HttpClient is NOT ............................................................................................. iv
1. Fundamentals ....................................................................................................................... 1
1.1. Request execution ..................................................................................................... 1
1.1.1. HTTP request ................................................................................................. 1
1.1.2. HTTP response ............................................................................................... 2
1.1.3. Working with message headers ........................................................................ 2
1.1.4. HTTP entity ................................................................................................... 4
1.1.5. Ensuring release of low level resources ............................................................ 5
1.1.6. Consuming entity content ................................................................................ 5
1.1.7. Producing entity content .................................................................................. 6
1.1.8. Response handlers .......................................................................................... 7
1.2. HTTP execution context ............................................................................................ 8
1.3. Exception handling .................................................................................................... 9
1.3.1. HTTP transport safety ..................................................................................... 9
1.3.2. Idempotent methods ........................................................................................ 9
1.3.3. Automatic exception recovery ........................................................................ 10
1.3.4. Request retry handler .................................................................................... 10
1.4. Aborting requests .................................................................................................... 11
1.5. HTTP protocol interceptors ...................................................................................... 11
1.6. HTTP parameters .................................................................................................... 12
1.6.1. Parameter hierarchies .................................................................................... 12
1.6.2. HTTP parameters beans ................................................................................ 13
1.7. HTTP request execution parameters .......................................................................... 13
2. Connection management ..................................................................................................... 15
2.1. Connection parameters ............................................................................................. 15
2.2. Connection persistence ............................................................................................. 16
2.3. HTTP connection routing ......................................................................................... 16
2.3.1. Route computation ........................................................................................ 16
2.3.2. Secure HTTP connections ............................................................................. 17
2.4. HTTP route parameters ............................................................................................ 17
2.5. Socket factories ....................................................................................................... 17
2.5.1. Secure socket layering ................................................................................... 18
2.5.2. SSL/TLS customization ................................................................................. 18
2.5.3. Hostname verification ................................................................................... 18
2.6. Protocol schemes ..................................................................................................... 19
2.7. HttpClient proxy configuration ................................................................................. 19
2.8. HTTP connection managers ..................................................................................... 20
2.8.1. Connection operators ..................................................................................... 20
2.8.2. Managed connections and connection managers .............................................. 20
2.8.3. Simple connection manager ........................................................................... 22
2.8.4. Pooling connection manager .......................................................................... 22
2.8.5. Connection manager shutdown ...................................................................... 23
2.9. Connection management parameters ......................................................................... 23
2.10. Multithreaded request execution ............................................................................. 23
2.11. Connection eviction policy ..................................................................................... 24
2.12. Connection keep alive strategy ............................................................................... 25
3. HTTP state management ..................................................................................................... 27
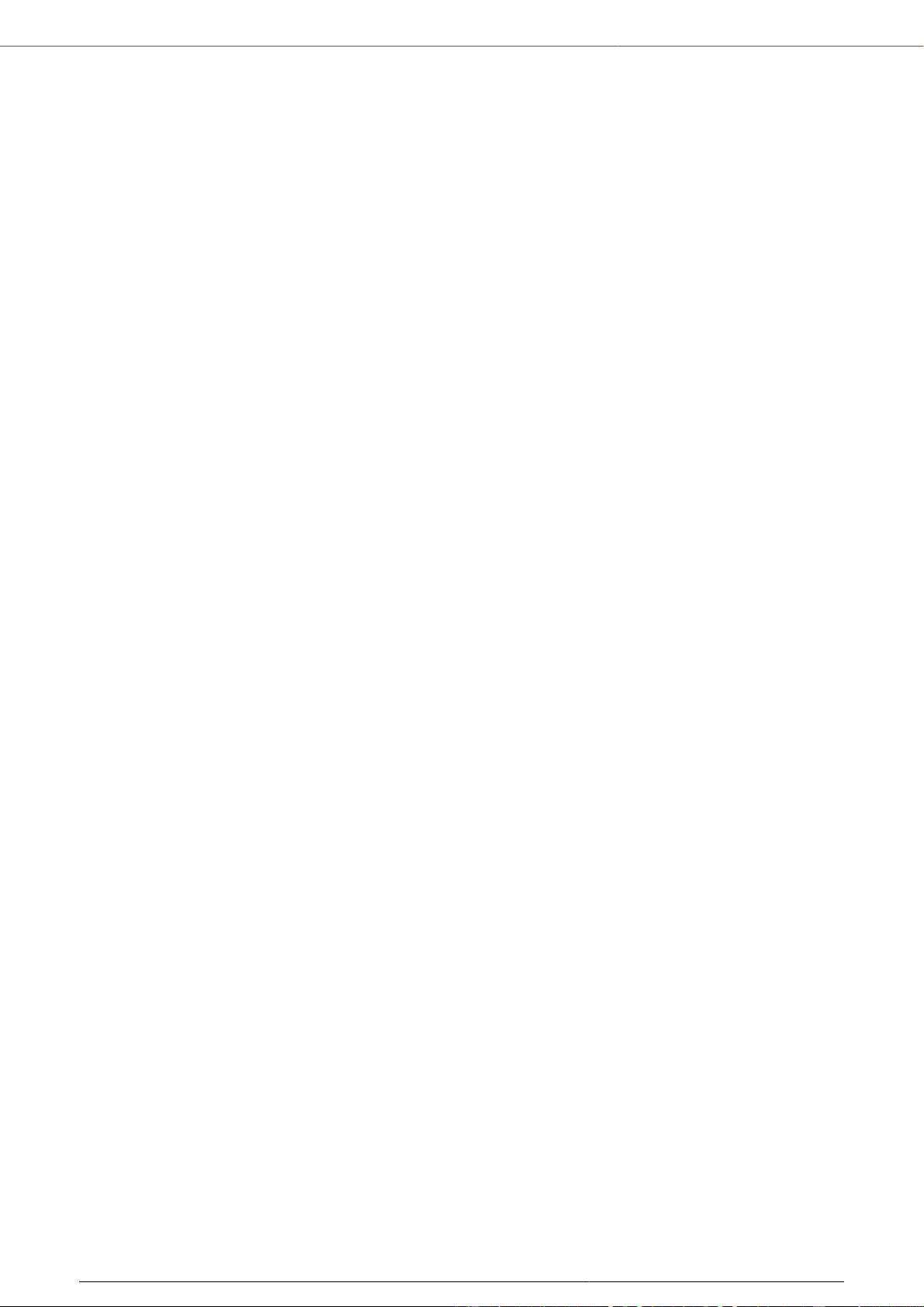
HttpClient Tutorial
iii
3.1. HTTP cookies ......................................................................................................... 27
3.1.1. Cookie versions ............................................................................................ 27
3.2. Cookie specifications ............................................................................................... 28
3.3. HTTP cookie and state management parameters ........................................................ 29
3.4. Cookie specification registry .................................................................................... 29
3.5. Choosing cookie policy ............................................................................................ 29
3.6. Custom cookie policy .............................................................................................. 30
3.7. Cookie persistence ................................................................................................... 30
3.8. HTTP state management and execution context ......................................................... 30
3.9. Per user / thread state management ........................................................................... 31
4. HTTP authentication .......................................................................................................... 32
4.1. User credentials ....................................................................................................... 32
4.2. Authentication schemes ............................................................................................ 32
4.3. HTTP authentication parameters ............................................................................... 33
4.4. Authentication scheme registry ................................................................................. 33
4.5. Credentials provider ................................................................................................. 33
4.6. HTTP authentication and execution context ............................................................... 34
4.7. Preemptive authentication ........................................................................................ 35
5. HTTP client service ........................................................................................................... 37
5.1. HttpClient facade ..................................................................................................... 37
5.2. HttpClient parameters .............................................................................................. 38
5.3. Automcatic redirect handling .................................................................................... 38
5.4. HTTP client and execution context ........................................................................... 39
6. Advanced topics ................................................................................................................. 40
6.1. Custom client connections ........................................................................................ 40
6.2. Stateful HTTP connections ....................................................................................... 41
6.2.1. User token handler ........................................................................................ 41
6.2.2. User token and execution context .................................................................. 42
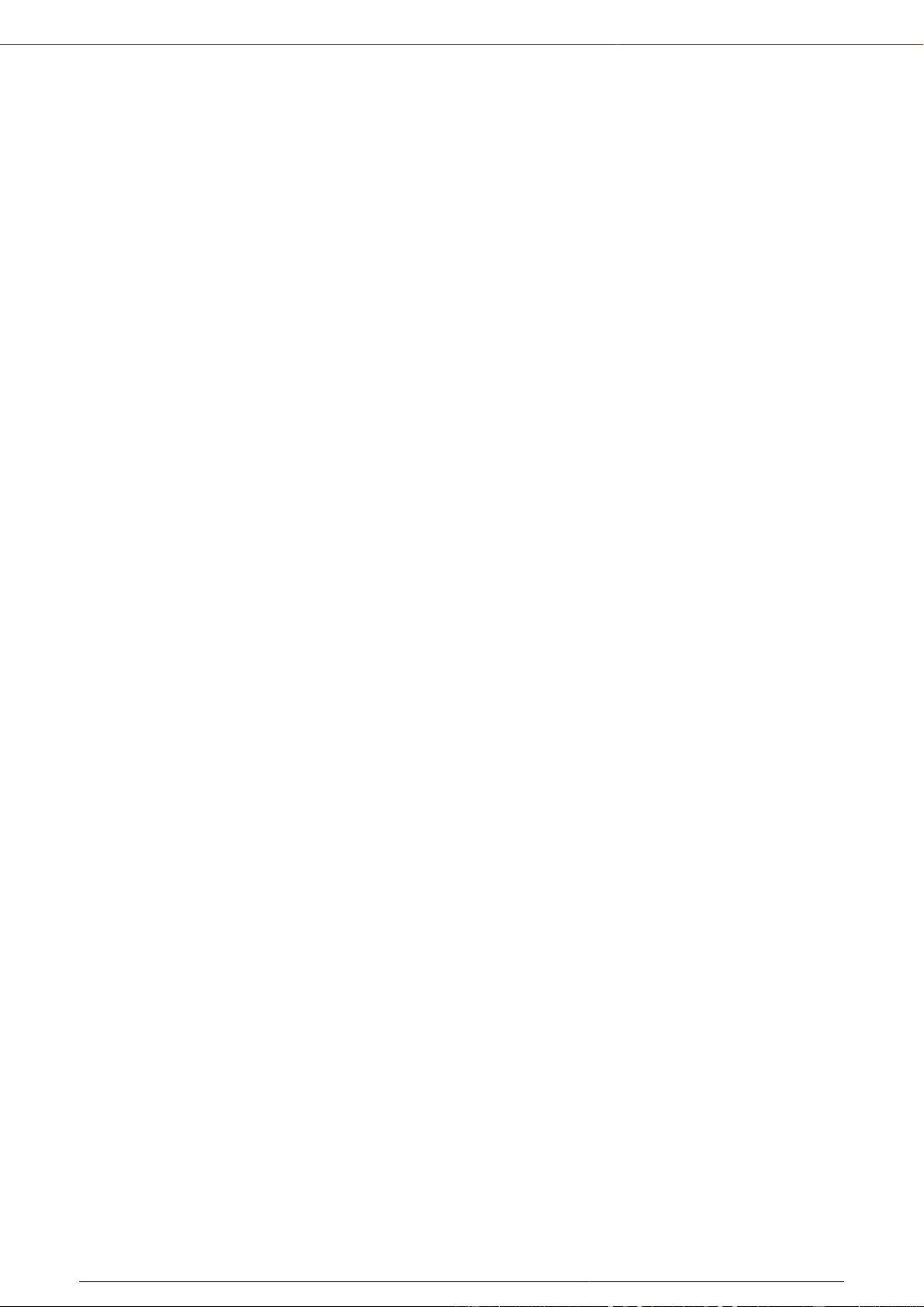
iv
Preface
The Hyper-Text Transfer Protocol (HTTP) is perhaps the most significant protocol used on the Internet
today. Web services, network-enabled appliances and the growth of network computing continue to
expand the role of the HTTP protocol beyond user-driven web browsers, while increasing the number
of applications that require HTTP support.
Although the java.net package provides basic functionality for accessing resources via HTTP, it doesn't
provide the full flexibility or functionality needed by many applications. HttpClient seeks to fill this
void by providing an efficient, up-to-date, and feature-rich package implementing the client side of
the most recent HTTP standards and recommendations.
Designed for extension while providing robust support for the base HTTP protocol, HttpClient may
be of interest to anyone building HTTP-aware client applications such as web browsers, web service
clients, or systems that leverage or extend the HTTP protocol for distributed communication.
1. HttpClient scope
• Client-side HTTP transport library based on HttpCore [http://hc.apache.org/httpcomponents-core/
index.html]
• Based on classic (blocking) I/O
• Content agnostic
2. What HttpClient is NOT
• HttpClient is NOT a browser. It is a client side HTTP transport library. HttpClient's purpose is
to transmit and receive HTTP messages. HttpClient will not attempt to cache content, execute
javascript embedded in HTML pages, try to guess content type, or reformat request / redirect location
URIs, or other functionality unrelated to the HTTP transport.
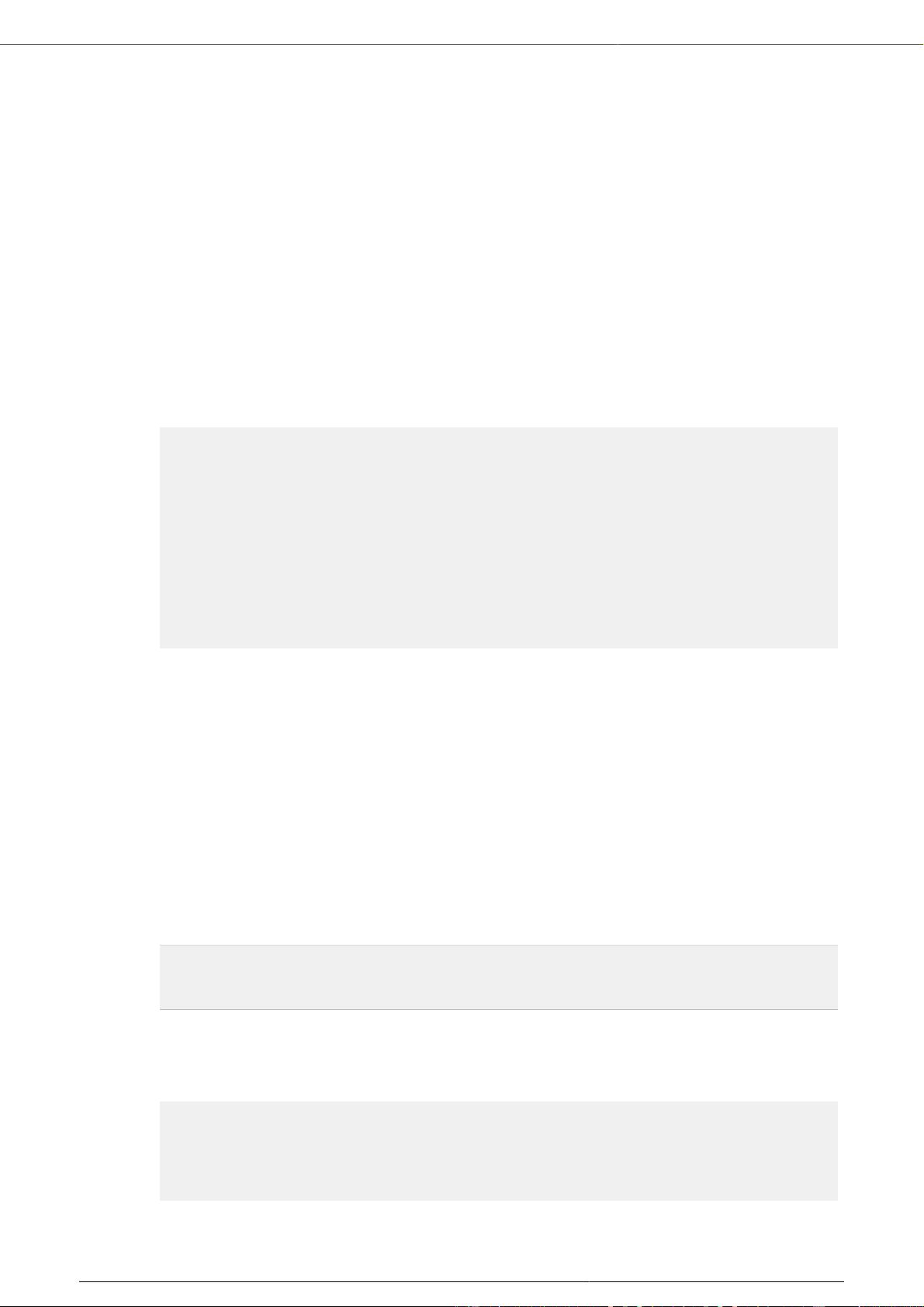
1
Chapter 1. Fundamentals
1.1. Request execution
The most essential function of HttpClient is to execute HTTP methods. Execution of an HTTP method
involves one or several HTTP request / HTTP response exchanges, usually handled internally by
HttpClient. The user is expected to provide a request object to execute and HttpClient is expected to
transmit the request to the target server return a corresponding response object, or throw an exception
if execution was unsuccessful.
Quite naturally, the main entry point of the HttpClient API is the HttpClient interface that defines the
contract described above.
Here is an example of request execution process in its simplest form:
HttpClient httpclient = new DefaultHttpClient();
HttpGet httpget = new HttpGet("http://localhost/");
HttpResponse response = httpclient.execute(httpget);
HttpEntity entity = response.getEntity();
if (entity != null) {
InputStream instream = entity.getContent();
int l;
byte[] tmp = new byte[2048];
while ((l = instream.read(tmp)) != -1) {
}
}
1.1.1. HTTP request
All HTTP requests have a request line consisting a method name, a request URI and a HTTP protocol
version.
HttpClient supports out of the box all HTTP methods defined in the HTTP/1.1 specification: GET,
HEAD, POST, PUT, DELETE, TRACE and OPTIONS. There is a special class for each method type.: HttpGet,
HttpHead, HttpPost, HttpPut, HttpDelete, HttpTrace, and HttpOptions.
The Request-URI is a Uniform Resource Identifier that identifies the resource upon which to apply
the request. HTTP request URIs consist of a protocol scheme, host name, optional port, resource path,
optional query, and optional fragment.
HttpGet httpget = new HttpGet(
"http://www.google.com/search?hl=en&q=httpclient&btnG=Google+Search&aq=f&oq=");
HttpClient provides a number of utility methods to simplify creation and modification of request URIs.
URI can be assembled programmatically:
URI uri = URIUtils.createURI("http", "www.google.com", -1, "/search",
"q=httpclient&btnG=Google+Search&aq=f&oq=", null);
HttpGet httpget = new HttpGet(uri);
System.out.println(httpget.getURI());
stdout >
评论0