/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.feilong.lib.validator;
import java.io.Serializable;
import java.net.IDN;
import java.util.Arrays;
import java.util.Locale;
/**
* <p>
* <b>Domain name</b> validation routines.
* </p>
*
* <p>
* This validator provides methods for validating Internet domain names
* and top-level domains.
* </p>
*
* <p>
* Domain names are evaluated according
* to the standards <a href="http://www.ietf.org/rfc/rfc1034.txt">RFC1034</a>,
* section 3, and <a href="http://www.ietf.org/rfc/rfc1123.txt">RFC1123</a>,
* section 2.1. No accommodation is provided for the specialized needs of
* other applications; if the domain name has been URL-encoded, for example,
* validation will fail even though the equivalent plaintext version of the
* same name would have passed.
* </p>
*
* <p>
* Validation is also provided for top-level domains (TLDs) as defined and
* maintained by the Internet Assigned Numbers Authority (IANA):
* </p>
*
* <ul>
* <li>{@link #isValidInfrastructureTld} - validates infrastructure TLDs
* (<code>.arpa</code>, etc.)</li>
* <li>{@link #isValidGenericTld} - validates generic TLDs
* (<code>.com, .org</code>, etc.)</li>
* <li>{@link #isValidCountryCodeTld} - validates country code TLDs
* (<code>.us, .uk, .cn</code>, etc.)</li>
* </ul>
*
* <p>
* (<b>NOTE</b>: This class does not provide IP address lookup for domain names or
* methods to ensure that a given domain name matches a specific IP; see
* {@link java.net.InetAddress} for that functionality.)
* </p>
*
* @version $Revision: 1781829 $
* @since Validator 1.4
*/
public class DomainValidator implements Serializable{
private static final int MAX_DOMAIN_LENGTH = 253;
private static final String[] EMPTY_STRING_ARRAY = new String[0];
private static final long serialVersionUID = -4407125112880174009L;
// Regular expression strings for hostnames (derived from RFC2396 and RFC 1123)
// RFC2396: domainlabel = alphanum | alphanum *( alphanum | "-" ) alphanum
// Max 63 characters
private static final String DOMAIN_LABEL_REGEX = "\\p{Alnum}(?>[\\p{Alnum}-]{0,61}\\p{Alnum})?";
// RFC2396 toplabel = alpha | alpha *( alphanum | "-" ) alphanum
// Max 63 characters
private static final String TOP_LABEL_REGEX = "\\p{Alpha}(?>[\\p{Alnum}-]{0,61}\\p{Alnum})?";
// RFC2396 hostname = *( domainlabel "." ) toplabel [ "." ]
// Note that the regex currently requires both a domain label and a top level label, whereas
// the RFC does not. This is because the regex is used to detect if a TLD is present.
// If the match fails, input is checked against DOMAIN_LABEL_REGEX (hostnameRegex)
// RFC1123 sec 2.1 allows hostnames to start with a digit
private static final String DOMAIN_NAME_REGEX = "^(?:" + DOMAIN_LABEL_REGEX + "\\.)+" + "(" + TOP_LABEL_REGEX
+ ")\\.?$";
private final boolean allowLocal;
/**
* Singleton instance of this validator, which
* doesn't consider local addresses as valid.
*/
private static final DomainValidator DOMAIN_VALIDATOR = new DomainValidator(false);
/**
* Singleton instance of this validator, which does
* consider local addresses valid.
*/
private static final DomainValidator DOMAIN_VALIDATOR_WITH_LOCAL = new DomainValidator(true);
/**
* RegexValidator for matching domains.
*/
private final RegexValidator domainRegex = new RegexValidator(DOMAIN_NAME_REGEX);
/**
* RegexValidator for matching a local hostname
*/
// RFC1123 sec 2.1 allows hostnames to start with a digit
private final RegexValidator hostnameRegex = new RegexValidator(DOMAIN_LABEL_REGEX);
/**
* Returns the singleton instance of this validator. It
* will not consider local addresses as valid.
*
* @return the singleton instance of this validator
*/
public static synchronized DomainValidator getInstance(){
inUse = true;
return DOMAIN_VALIDATOR;
}
/**
* Returns the singleton instance of this validator,
* with local validation as required.
*
* @param allowLocal
* Should local addresses be considered valid?
* @return the singleton instance of this validator
*/
public static synchronized DomainValidator getInstance(boolean allowLocal){
inUse = true;
if (allowLocal){
return DOMAIN_VALIDATOR_WITH_LOCAL;
}
return DOMAIN_VALIDATOR;
}
/** Private constructor. */
private DomainValidator(boolean allowLocal){
this.allowLocal = allowLocal;
}
/**
* Returns true if the specified <code>String</code> parses
* as a valid domain name with a recognized top-level domain.
* The parsing is case-insensitive.
*
* @param domain
* the parameter to check for domain name syntax
* @return true if the parameter is a valid domain name
*/
public boolean isValid(String domain){
if (domain == null){
return false;
}
domain = unicodeToASCII(domain);
// hosts must be equally reachable via punycode and Unicode;
// Unicode is never shorter than punycode, so check punycode
// if domain did not convert, then it will be caught by ASCII
// checks in the regexes below
if (domain.length() > MAX_DOMAIN_LENGTH){
return false;
}
String[] groups = domainRegex.match(domain);
if (groups != null && groups.length > 0){
return isValidTld(groups[0]);
}
return allowLocal && hostnameRegex.isValid(domain);
}
// package protected for unit test access
// must agree with isValid() above
final boolean isValidDomainSyntax(String domain){
if (domain == null){
return false;
}
domain = unicodeToASCII(domain);
// hosts must be equally reachable via punycode and Unicode;
// Unicode is never shorter than punycode, so check punycode
// if domain did not convert, then it will be caught by ASCII
// checks in the regexes below
if (domain.length() > MAX_DOMAIN_LENGTH){
return false;
}
String[] groups = domainRegex.match(domain);
return (groups != null && groups.length > 0) || hostnameRegex.isValid(domain);
}
/**
* Returns true if the specified <code>String</code> matches any
* IANA-defined top-level domain. Leading dots are ignored if present.
* The search is case-insensitive.
*
* @param tld
* the parameter to check for TLD status, not null
* @return true if the parameter is a TLD
*/
public boolean isValidTld(String tld){
tld = unicodeToASCII(tld);
if (allowLocal && isValidLocalTld(tld)){
return true;
}
return isValidInfrastructureTld(tld) || isValidGenericTld(tld) || isValidCountryCodeTld(tld);
}
/**
没有合适的资源?快使用搜索试试~ 我知道了~
feilong开发工具库 v4.1.2
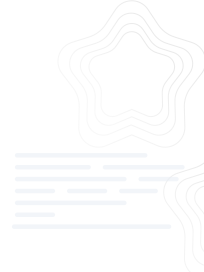
共2000个文件
java:1917个
xml:55个
html:15个

0 下载量 57 浏览量
2024-10-18
15:19:35
上传
评论
收藏 27.53MB ZIP 举报
温馨提示
feilong是一个可以让Java开发更加简单的工具库。feilong特点:1、有常用的工具类 (如处理日期的DateUtil,处理集合的CollectionsUtil等)2、有常用的JAVA常量类 (如日期格式DatePattern,时间间隔TimeInterval等)3、不必要的Exception 转成了
资源推荐
资源详情
资源评论
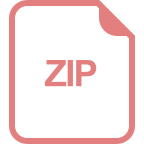
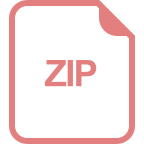
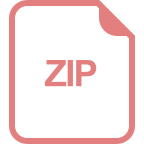
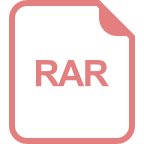
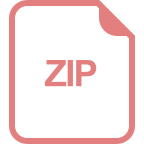
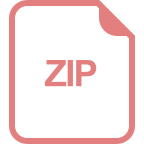
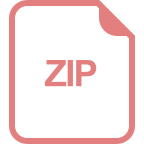
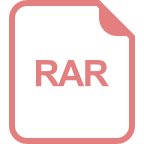
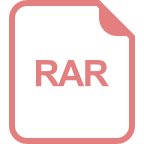
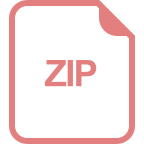
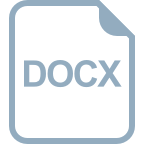
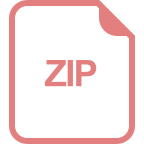
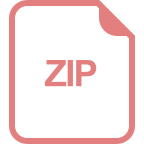
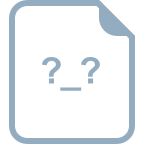
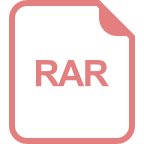
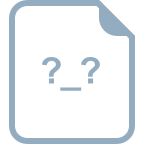
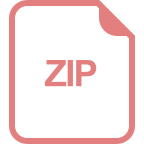
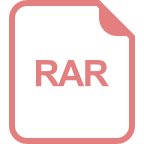
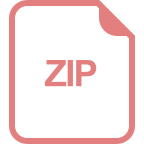
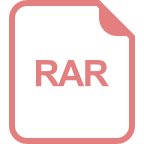
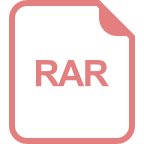
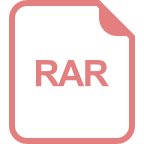
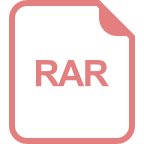
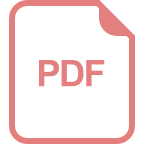
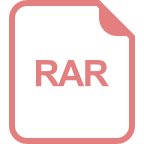
收起资源包目录

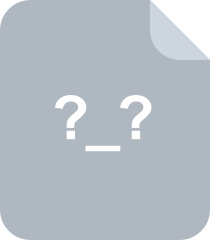
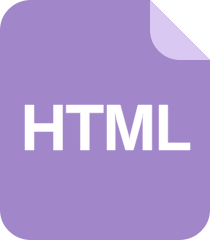
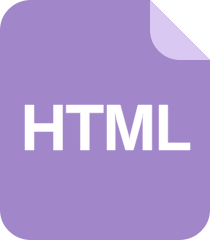
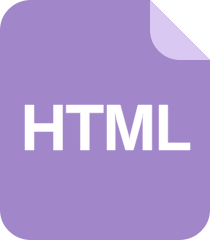
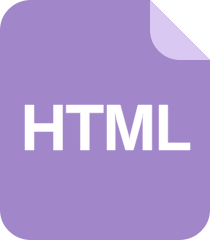
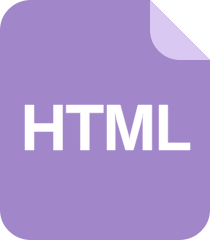
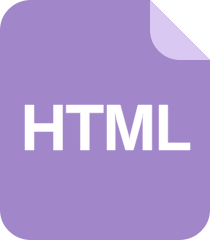
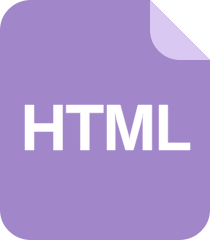
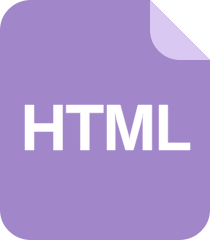
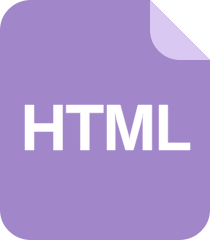
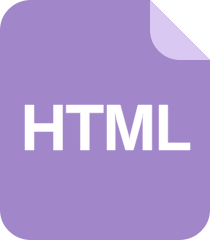
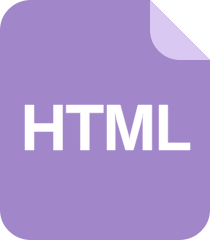
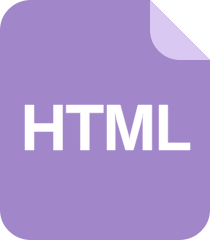
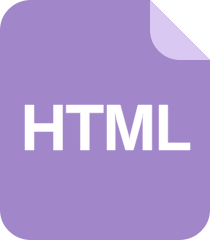
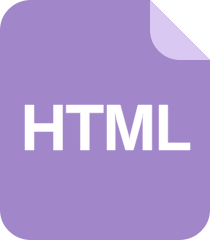
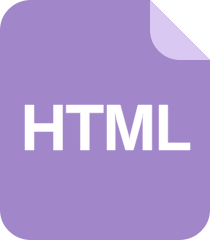
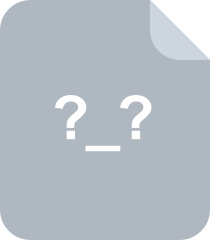
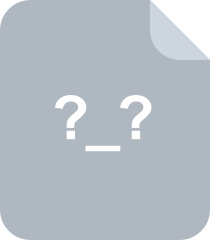
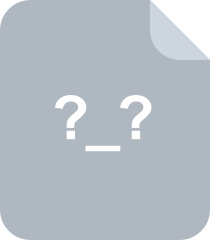
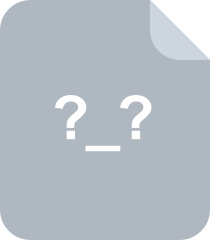
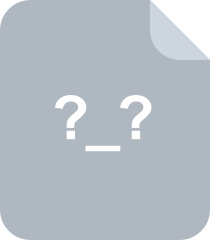
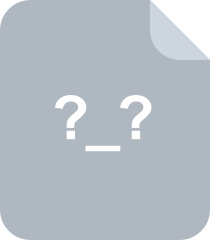
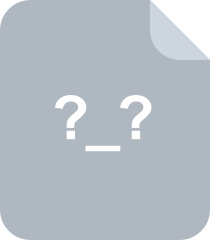
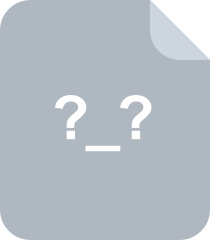
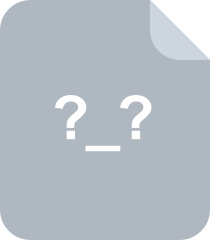
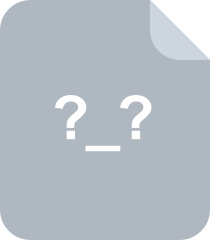
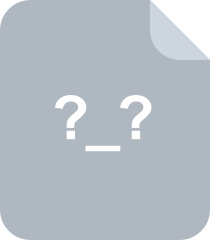
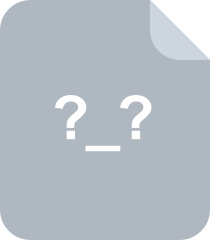
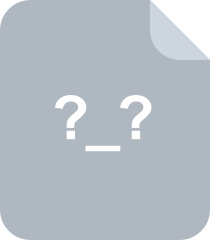
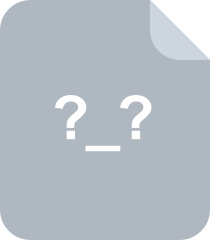
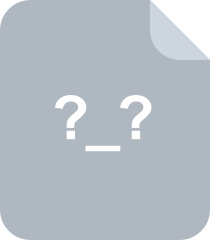
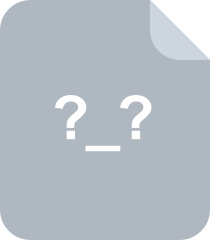
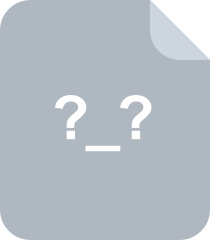
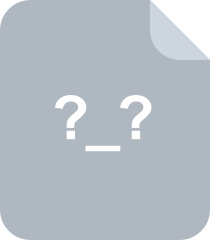
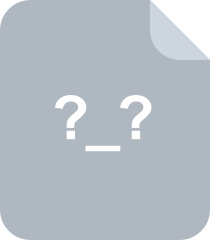
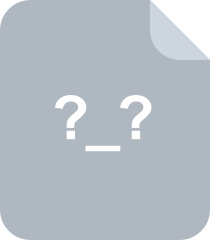
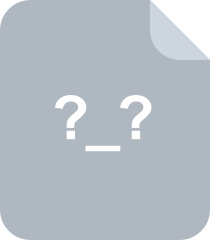
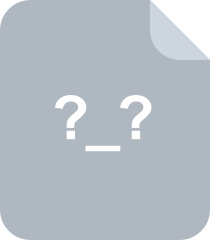
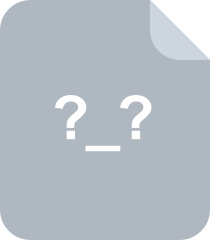
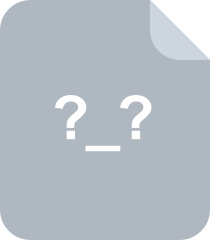
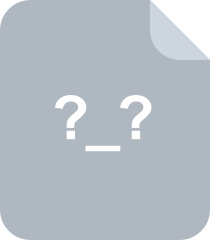
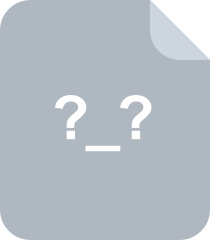
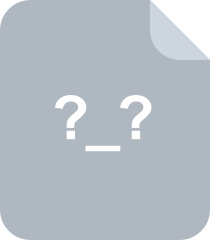
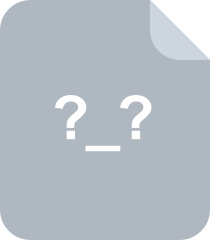
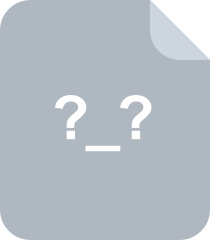
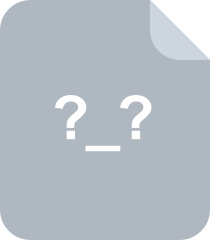
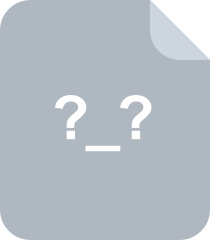
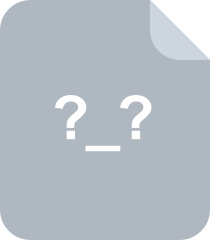
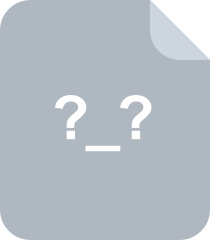
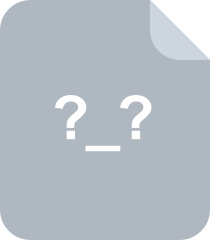
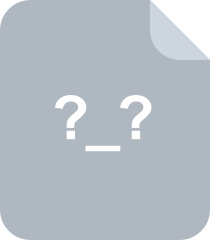
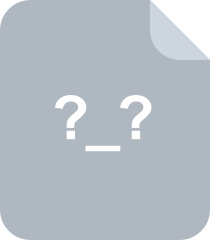
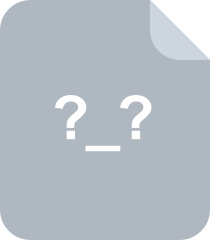
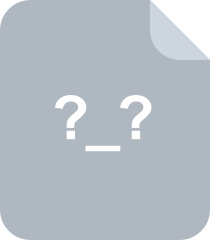
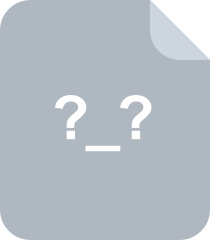
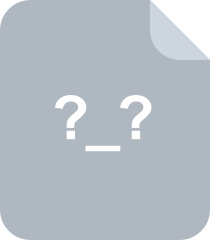
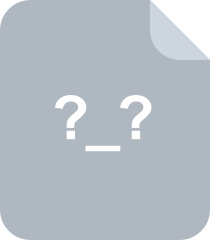
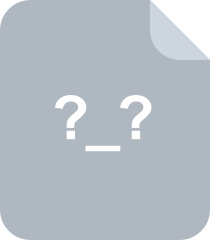
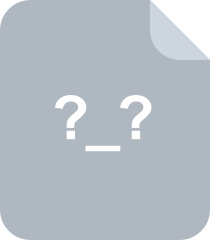
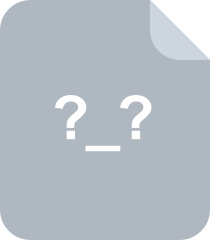
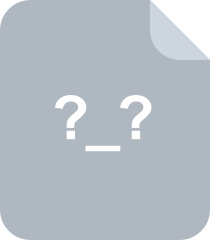
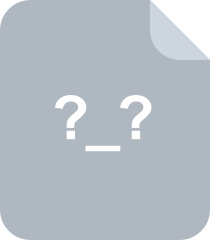
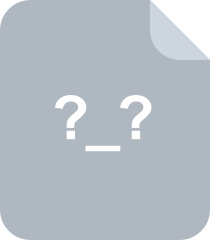
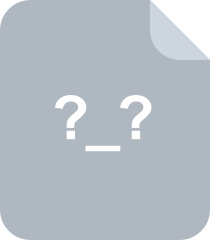
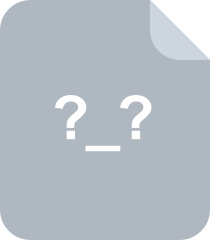
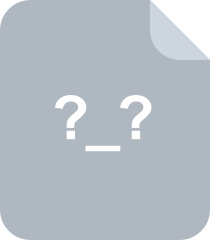
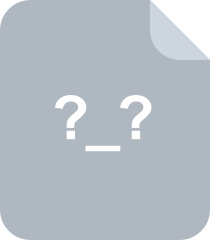
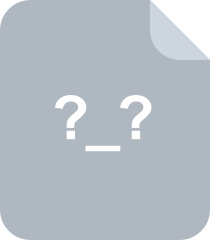
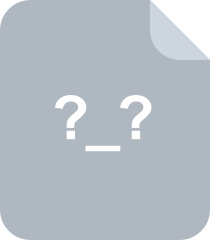
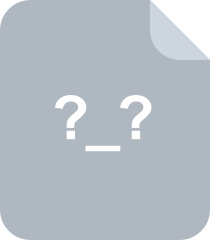
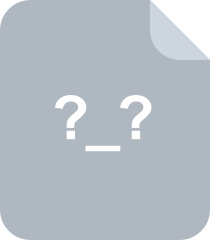
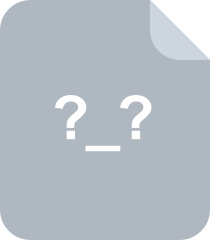
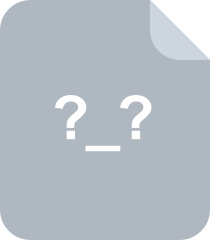
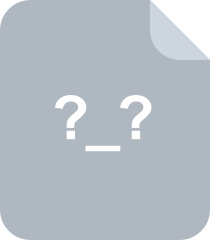
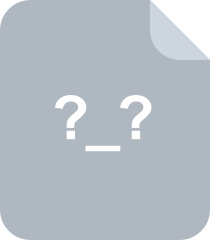
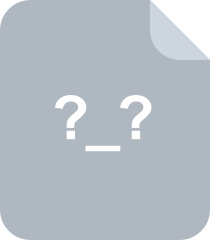
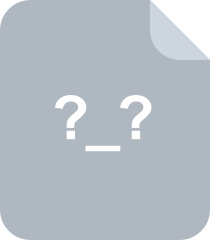
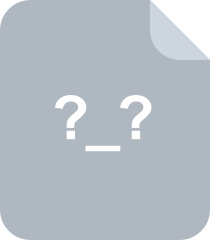
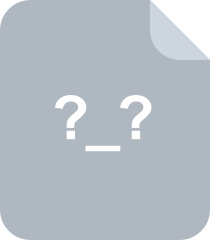
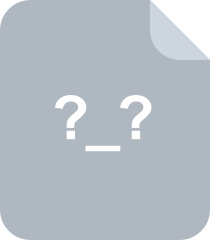
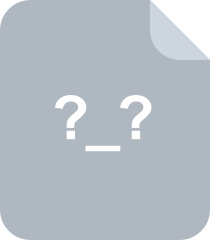
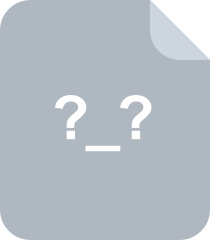
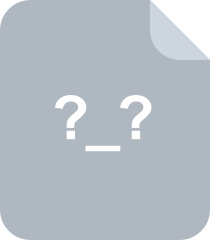
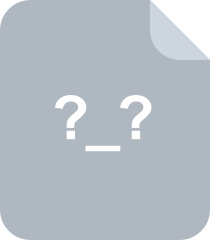
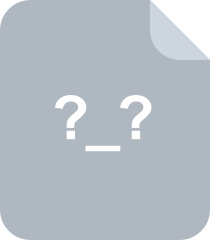
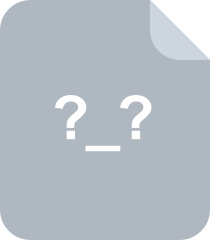
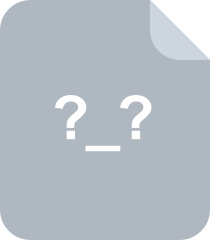
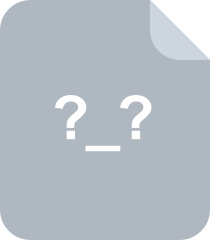
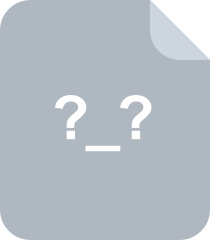
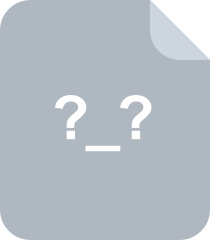
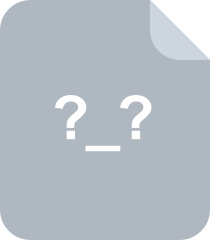
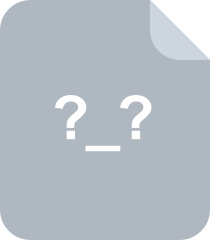
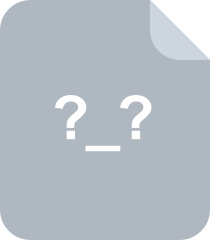
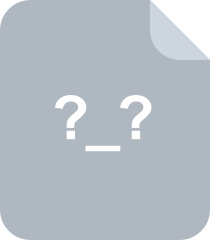
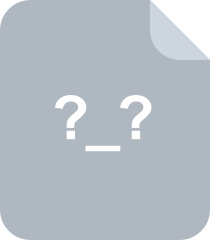
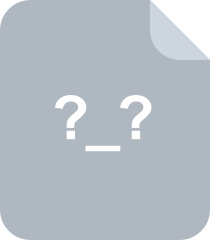
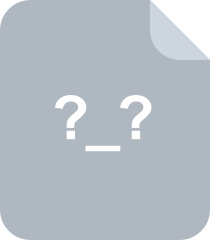
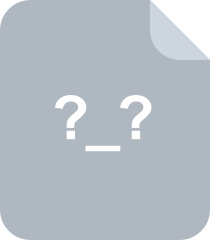
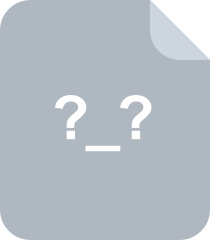
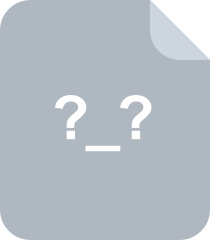
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
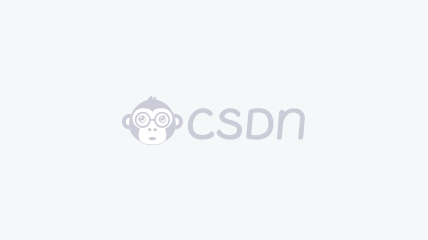

稀稀落落987
- 粉丝: 1293
- 资源: 5652
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

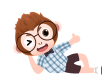
最新资源
- C语言-leetcode题解之70-climbing-stairs.c
- C语言-leetcode题解之68-text-justification.c
- C语言-leetcode题解之66-plus-one.c
- C语言-leetcode题解之64-minimum-path-sum.c
- C语言-leetcode题解之63-unique-paths-ii.c
- C语言-leetcode题解之62-unique-paths.c
- C语言-leetcode题解之61-rotate-list.c
- C语言-leetcode题解之59-spiral-matrix-ii.c
- C语言-leetcode题解之58-length-of-last-word.c
- 计算机编程课程设计基础教程
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


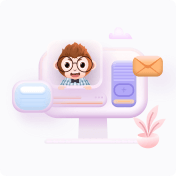
安全验证
文档复制为VIP权益,开通VIP直接复制
