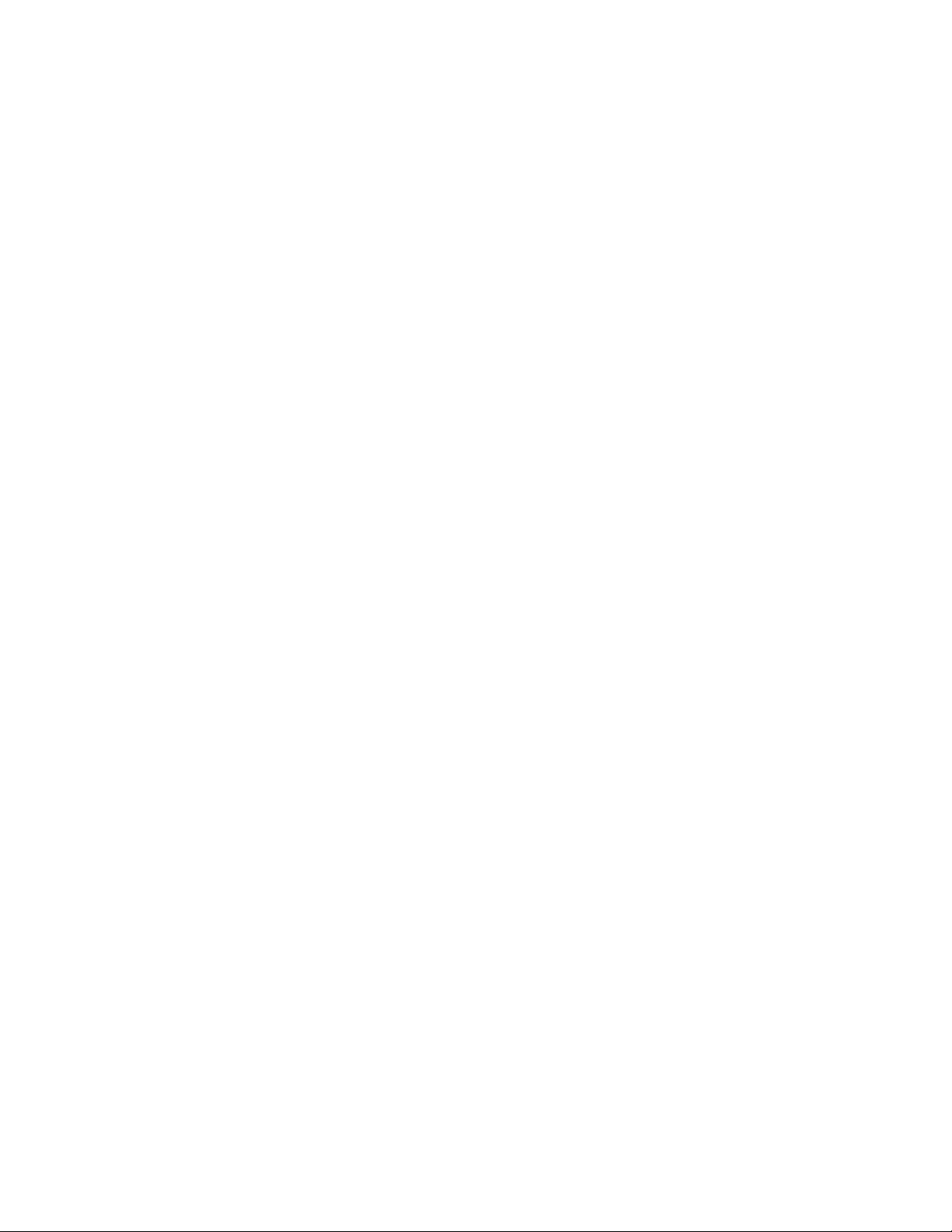
C# Language Reference
Owners: Anders Hejlsberg and Scott Wiltamuth
File: raw.doc
Last saved: 6/27/2000
Last printed: 6/8/2000
Version 0.17b0.17b
Copyright
Ó
Microsoft Corporation 1999-20001998. All Rights Reserved.
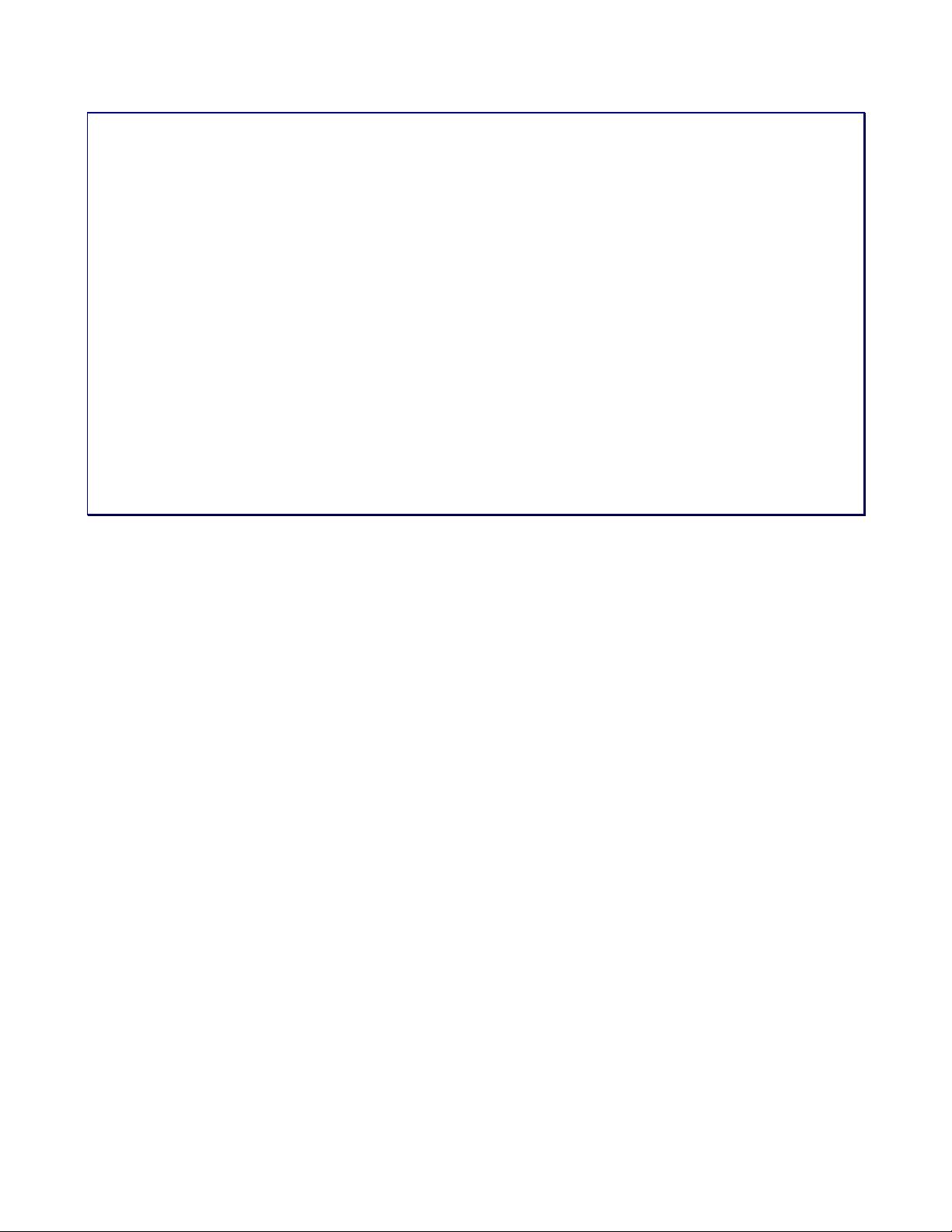
Notice
This documentation is an early release of the final documentation, which may be changed substantially prior to final
commercial release, and is information of Microsoft Corporation.
This document is provided for informational purposes only and Microsoft makes no warranties, either express or implied,
in this document. Information in this document is subject to change without notice.
The entire risk of the use or the results of the use of this document remains with the user. Complying with all applicable
copyright laws is the responsibility of the user.
Without limiting the rights under copyright, no part of this document may be reproduced, stored in or introduced into a
retrieval system, or transmitted in any form or by any means (electronic, mechanical, photocopying, recording, or
otherwise), or for any purpose, without the express written permission of Microsoft Corporation.
Microsoft may have patents, patent applications, trademarks, copyrights, or other intellectual property rights covering
subject matter in this document. Except as expressly provided in any written license agreement from Microsoft, the
furnishing of this document does not give you any license to these patents, trademarks, copyrights, or other intellectual
property.
Unpublished work. © 1999-2000 Microsoft Corporation. All rights reserved.
Microsoft, Windows, Visual Basic, and Visual C++ are either registered trademarks or trademarks of Microsoft
Corporation in the U.S.A. and/or other countries.
Other product and company names mentioned herein may be the trademarks of their respective owners.
Copyright
Ó
Microsoft Corporation 1999-20001998. All Rights Reserved.
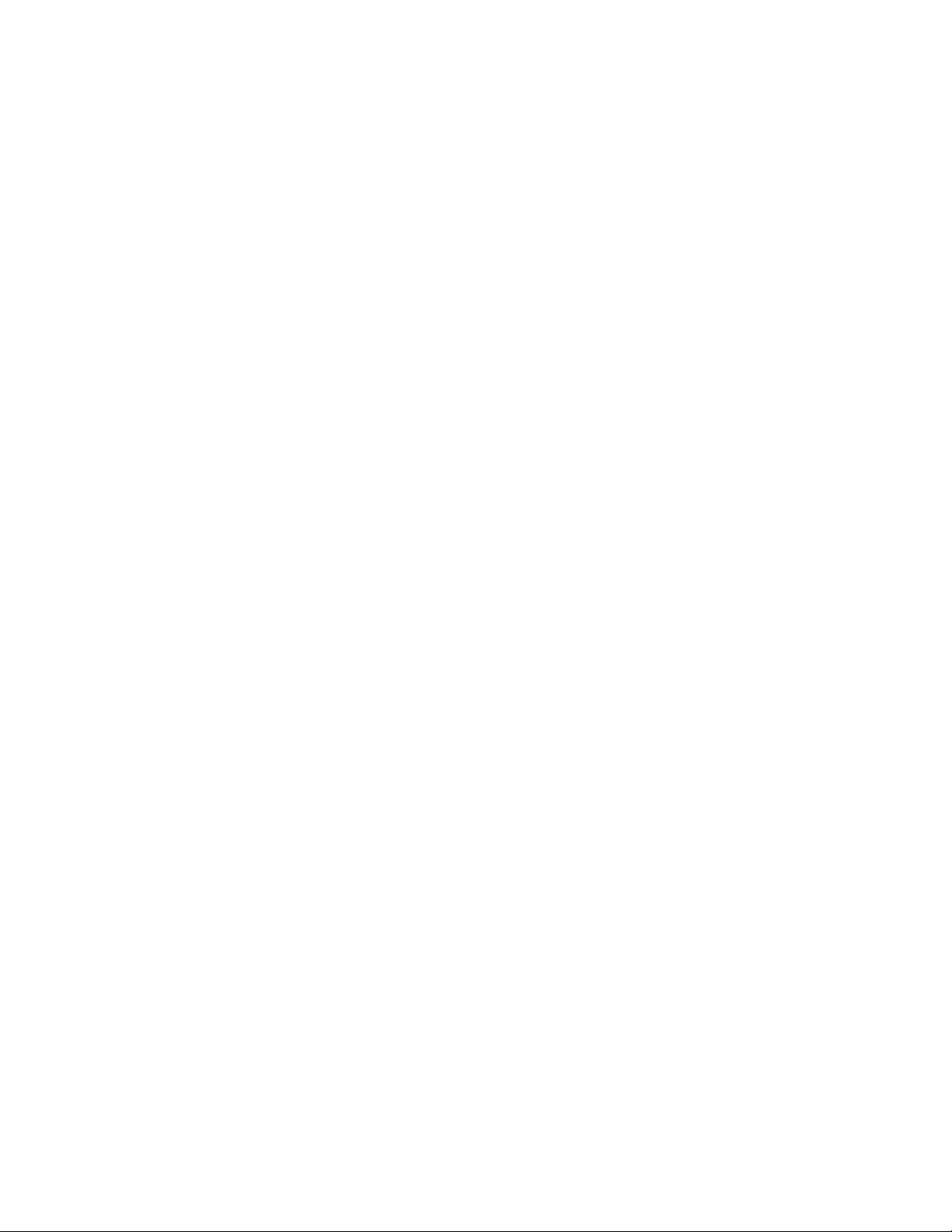
Table of Contents
Table of Contents
1. Introduction 1
1.1 Hello, world 1
1.2 Automatic memory management 2
1.3 Types 4
1.4 Predefined types 6
1.5 Array types 8
1.6 Type system unification 10
1.7 Statements 11
1.7.1 Statement lists and blocks...................................................................................................................11
1.7.2 Labeled statements and goto statements..............................................................................................12
1.7.3 Local declarations of constants and variables.....................................................................................12
1.7.4 Expression statements.........................................................................................................................12
1.7.5 The if statement..................................................................................................................................13
1.7.6 The switch statement...........................................................................................................................13
1.7.7 The while statement............................................................................................................................14
1.7.8 The do statement.................................................................................................................................14
1.7.9 The for statement................................................................................................................................14
1.7.10 The foreach statement.......................................................................................................................15
1.7.11 The break statement and the continue statement...............................................................................15
1.7.12 The return statement..........................................................................................................................15
1.7.13 The throw statement..........................................................................................................................15
1.7.14 The try statement...............................................................................................................................16
1.7.15 The checked and unchecked statements............................................................................................16
1.7.16 The lock statement............................................................................................................................16
1.8 Classes 16
1.9 Structs 16
1.10 Interfaces 17
1.11 Delegates 18
1.12 Enums 19
1.13 Namespaces20
1.14 Properties 21
1.15 Indexers 22
1.16 Events 23
1.17 Versioning 24
1.18 Attributes 27
2. Lexical structure 29
2.1 Phases of translation 29
2.2 Grammar notation 29
2.3 Pre-processing 30
2.3.1 Pre-processing declarations.................................................................................................................30
2.3.2 #if, #elif, #else, #endif........................................................................................................................32
2.3.3 Pre-processing control lines................................................................................................................33
2.3.4 #line....................................................................................................................................................33
2.3.5 Pre-processing identifiers....................................................................................................................33
2.3.6 Pre-processing expressions.................................................................................................................34
2.3.7 Interaction with white space................................................................................................................34
2.4 Lexical analysis 35
2.4.1 Input.................................................................................................................................................... 35
Copyright
Ó
Microsoft Corporation1998 1999-2000. All Rights Reserved. iii
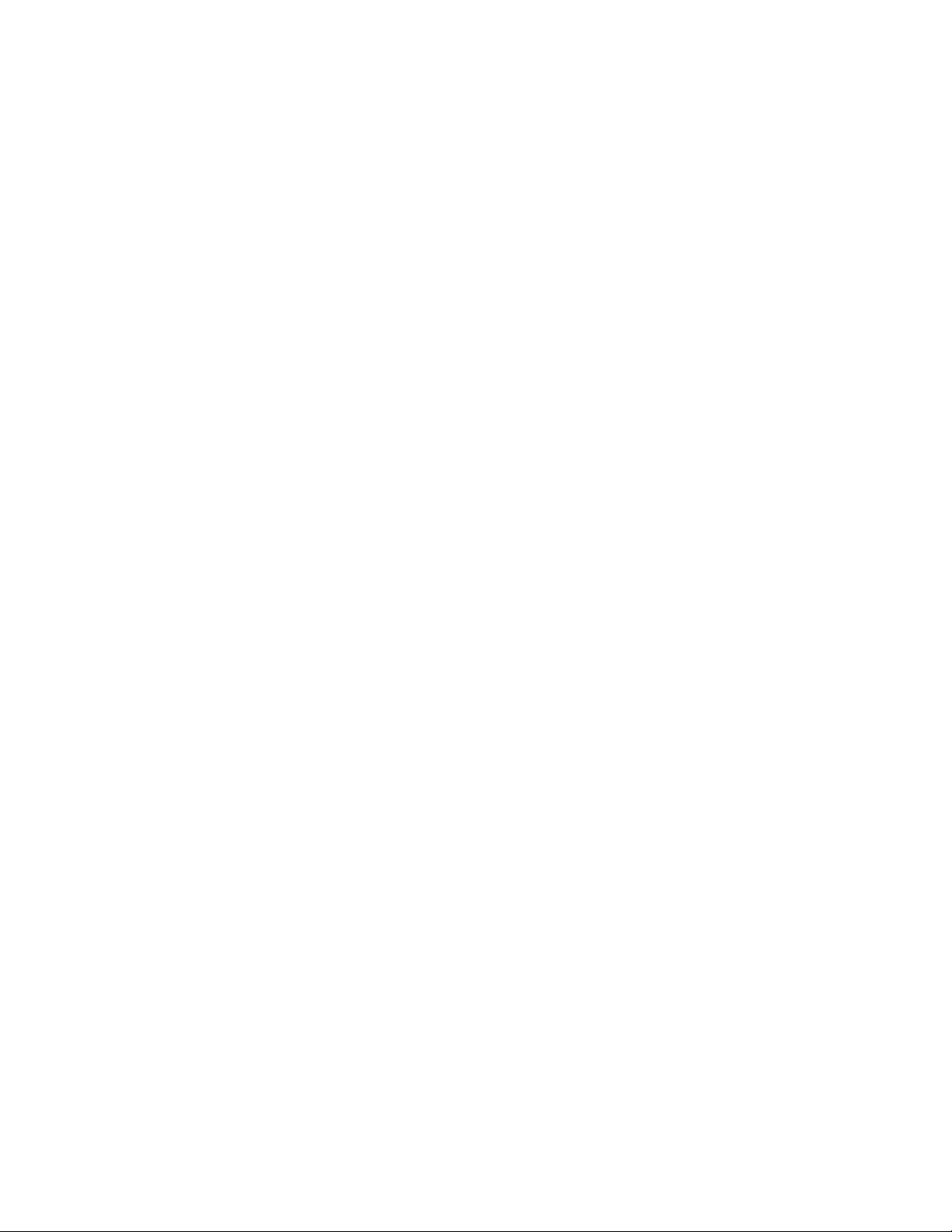
C# Language Reference
2.4.2 Input characters...................................................................................................................................35
2.4.3 Line terminators..................................................................................................................................35
2.4.4 Comments...........................................................................................................................................35
2.4.5 White space.........................................................................................................................................36
2.4.6 Tokens................................................................................................................................................36
2.5 Processing of Unicode character escape sequences36
2.5.1 Identifiers............................................................................................................................................37
2.5.2 Keywords............................................................................................................................................38
2.5.3 Literals................................................................................................................................................38
2.5.3.1 Boolean literals.............................................................................................................................38
2.5.3.2 Integer literals...............................................................................................................................39
2.5.3.3 Real literals...................................................................................................................................40
2.5.3.4 Character literals..........................................................................................................................40
2.5.3.5 String literals................................................................................................................................41
2.5.3.6 The null literal..............................................................................................................................42
2.5.4 Operators and punctuators...................................................................................................................42
3. Basic concepts 43
3.1 Declarations 43
3.2 Members45
3.2.1 Namespace members..........................................................................................................................45
3.2.2 Struct members...................................................................................................................................45
3.2.3 Enumeration members........................................................................................................................46
3.2.4 Class members....................................................................................................................................46
3.2.5 Interface members...............................................................................................................................46
3.2.6 Array members...................................................................................................................................46
3.2.7 Delegate members...............................................................................................................................46
3.3 Member access 46
3.3.1 Declared accessibility.........................................................................................................................47
3.3.2 Accessibility domains.........................................................................................................................47
3.3.3 Protected access..................................................................................................................................50
3.3.4 Accessibility constraints.....................................................................................................................50
3.4 Signatures and overloading 51
3.5 Scopes 52
3.5.1 Name hiding........................................................................................................................................54
3.5.1.1 Hiding through nesting.................................................................................................................54
3.5.1.2 Hiding through inheritance...........................................................................................................55
3.6 Namespace and type names 56
3.6.1 Fully qualified names..........................................................................................................................57
4. Types 59
4.1 Value types 59
4.1.1 Default constructors............................................................................................................................60
4.1.2 Struct types.........................................................................................................................................61
4.1.3 Simple types........................................................................................................................................61
4.1.4 Integral types......................................................................................................................................63
4.1.5 Floating point types.............................................................................................................................64
4.1.6 The decimal type.................................................................................................................................65
4.1.7 The bool type......................................................................................................................................65
4.1.8 Enumeration types..............................................................................................................................65
4.2 Reference types 65
iv Copyright
Ó
Microsoft Corporation 1999-20001998. All Rights Reserved.
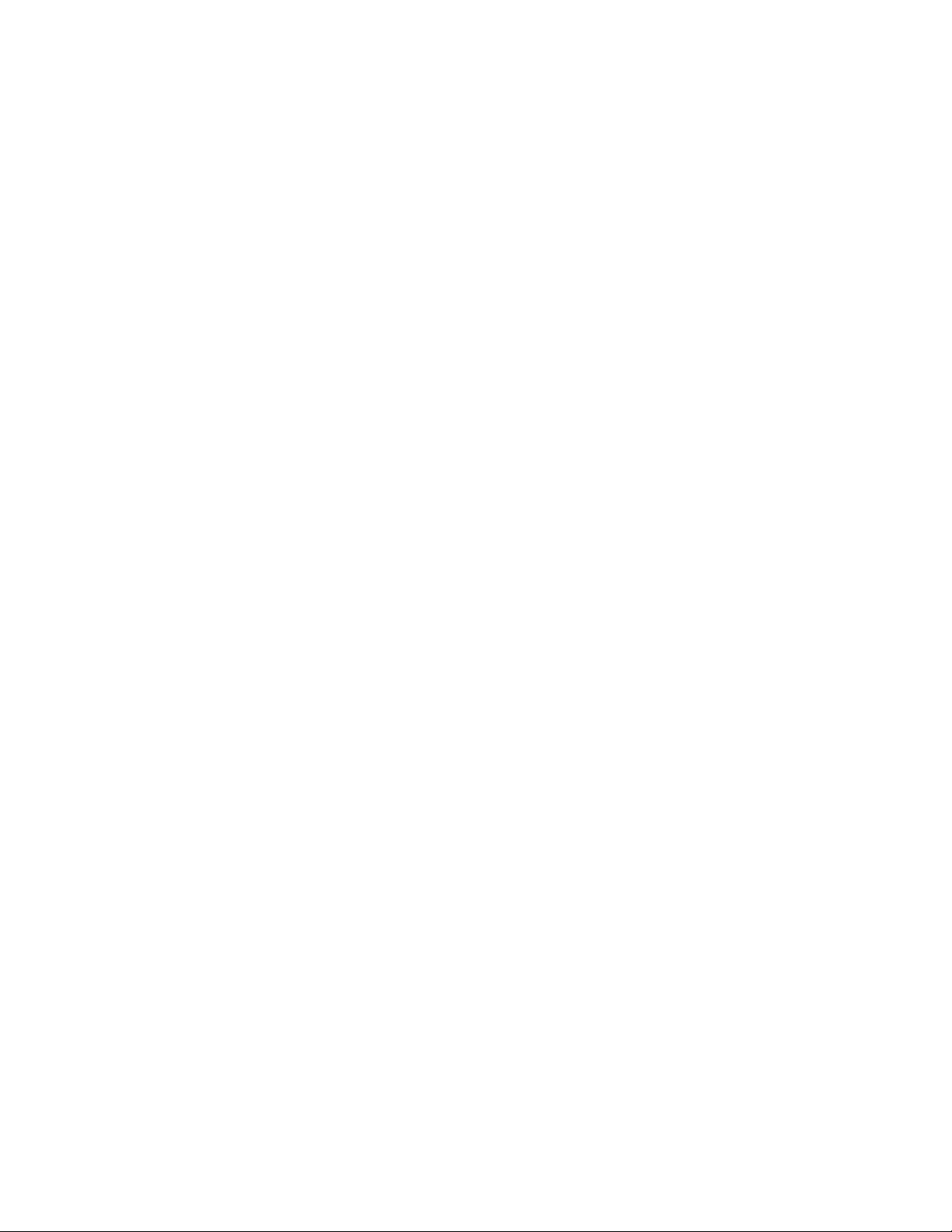
Table of Contents
4.2.1 Class types..........................................................................................................................................66
4.2.2 The object type....................................................................................................................................66
4.2.3 The string type....................................................................................................................................66
4.2.4 Interface types.....................................................................................................................................67
4.2.5 Array types..........................................................................................................................................67
4.2.6 Delegate types.....................................................................................................................................67
4.3 Boxing and unboxing 67
4.3.1 Boxing conversions.............................................................................................................................67
4.3.2 Unboxing conversions.........................................................................................................................68
5. Variables 71
5.1 Variable categories 71
5.1.1 Static variables....................................................................................................................................71
5.1.2 Instance variables................................................................................................................................71
5.1.2.1 Instance variables in classes.........................................................................................................71
5.1.2.2 Instance variables in structs..........................................................................................................72
5.1.3 Array elements....................................................................................................................................72
5.1.4 Value parameters................................................................................................................................72
5.1.5 Reference parameters..........................................................................................................................72
5.1.6 Output parameters...............................................................................................................................72
5.1.7 Local variables....................................................................................................................................73
5.2 Default values 73
5.3 Definite assignment 73
5.3.1 Initially assigned variables..................................................................................................................76
5.3.2 Initially unassigned variables..............................................................................................................76
5.4 Variable references 76
6. Conversions77
6.1 Implicit conversions 77
6.1.1 Identity conversion..............................................................................................................................77
6.1.2 Implicit numeric conversions..............................................................................................................77
6.1.3 Implicit enumeration conversions.......................................................................................................78
6.1.4 Implicit reference conversions............................................................................................................78
6.1.5 Boxing conversions.............................................................................................................................78
6.1.6 Implicit constant expression conversions............................................................................................78
6.1.7 User-defined implicit conversions.......................................................................................................78
6.2 Explicit conversions 79
6.2.1 Explicit numeric conversions..............................................................................................................79
6.2.2 Explicit enumeration conversions.......................................................................................................80
6.2.3 Explicit reference conversions............................................................................................................80
6.2.4 Unboxing conversions.........................................................................................................................81
6.2.5 User-defined explicit conversions.......................................................................................................81
6.3 Standard conversions 81
6.3.1 Standard implicit conversions.............................................................................................................81
6.3.2 Standard explicit conversions..............................................................................................................82
6.4 User-defined conversions 82
6.4.1 Permitted user-defined conversions....................................................................................................82
6.4.2 Evaluation of user-defined conversions..............................................................................................82
6.4.3 User-defined implicit conversions.......................................................................................................83
6.4.4 User-defined explicit conversions.......................................................................................................83
7. Expressions 85
Copyright
Ó
Microsoft Corporation1998 1999-2000. All Rights Reserved. v
评论0