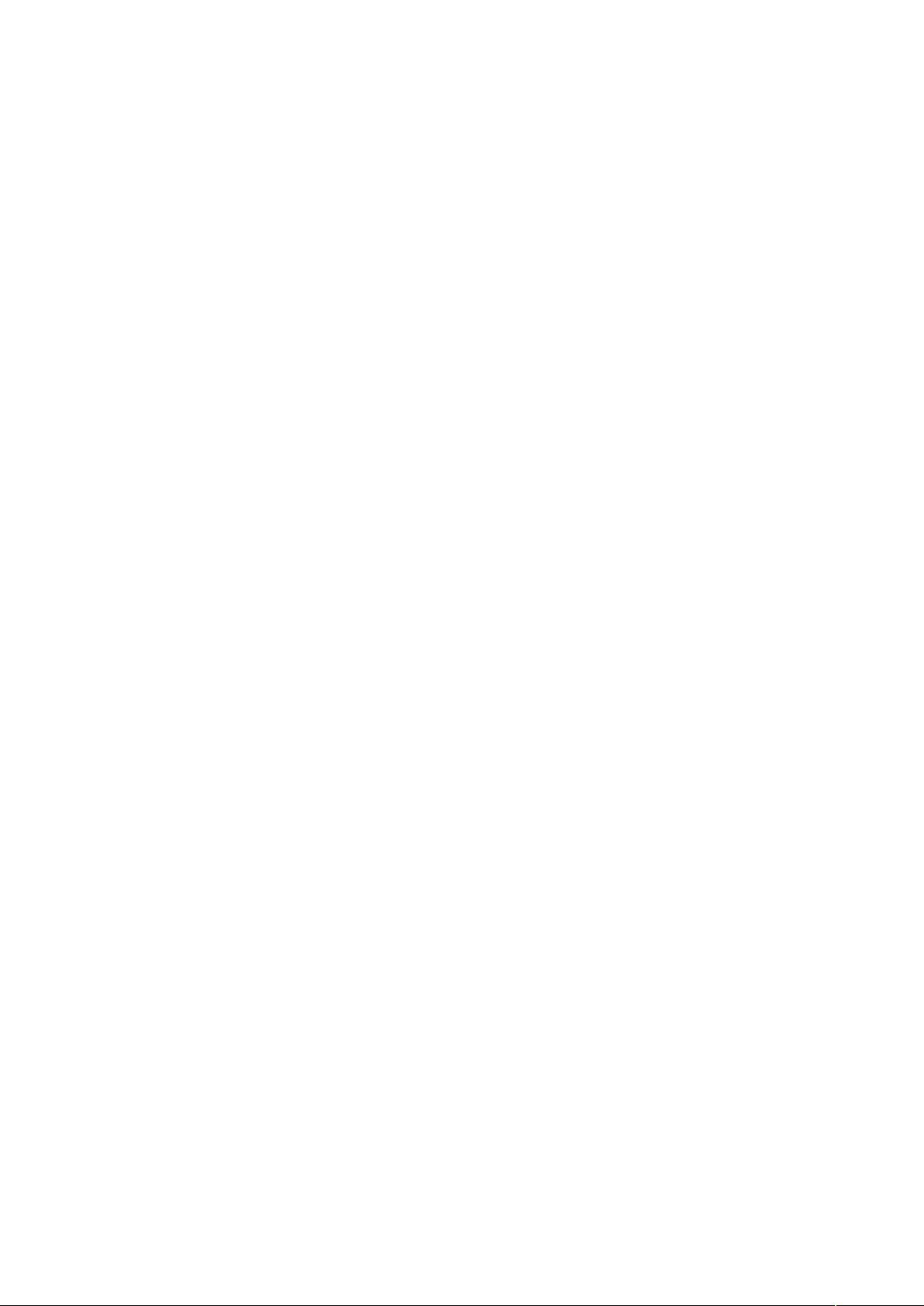
Java 课程设计报告
Java 简易计算器
姓名:
班级:
学号:
专业:
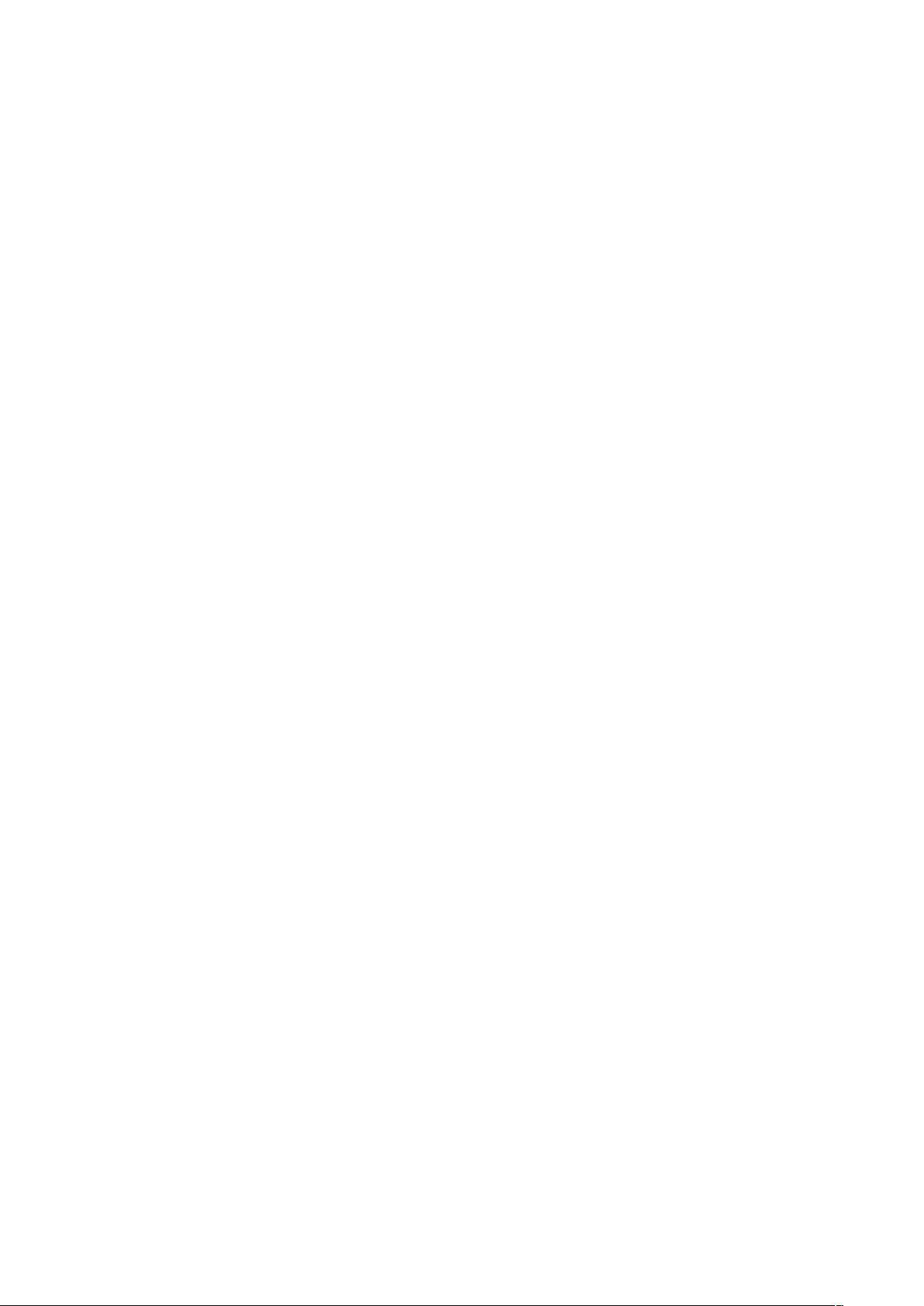
指导老师:
一、设计目的
本课程设计的目的就是要通过一次集中的强化训练,使学生能及时巩固已学的知识,补
充未学的但又必要的内容。进行课程设计目的在于加深对程序设计基础中基础理论和基本知
识的理解,促进理论与实践的结合,进一步提高程序设计的能力。具体目的如下:
1.使学生更深入地理解和掌握该课程中的有关基本概念,程序设计思想和方法。
2.培养学生综合运用所学知识独立完成课题的能力。
3.培养学生勇于探索、严谨推理、实事求是、有错必改,用实践来检验理论,全方位
考虑问题等科学技术人员应具有的素质。
4.提高学生对工作认真负责、一丝不苟,对同学团结友爱,协作攻关的基本素质。
5.培养学生从资料文献、科学实验中获得知识的能力,提高学生从别人经验中找到解
决问题的新途径的悟性,初步培养工程意识和创新能力。
6.对学生掌握知识的深度、运用理论去处理问题的能力、实验能力、课程设计能力、
书面及口头表达能力进行考核。
二、设计题目及要求
1、设计题目
简易计算器
2、设计要求
1)独自一人完成课程设计。
2)应用自己所学课程知识完成对计算器的基本任务。
3)查阅相关资料,学习和掌握项目中涉及的新知识,提高自学能力。
4)通过应用 java 程序编写计算器来提升自己对简单的图形界面有一定的掌握和了解。
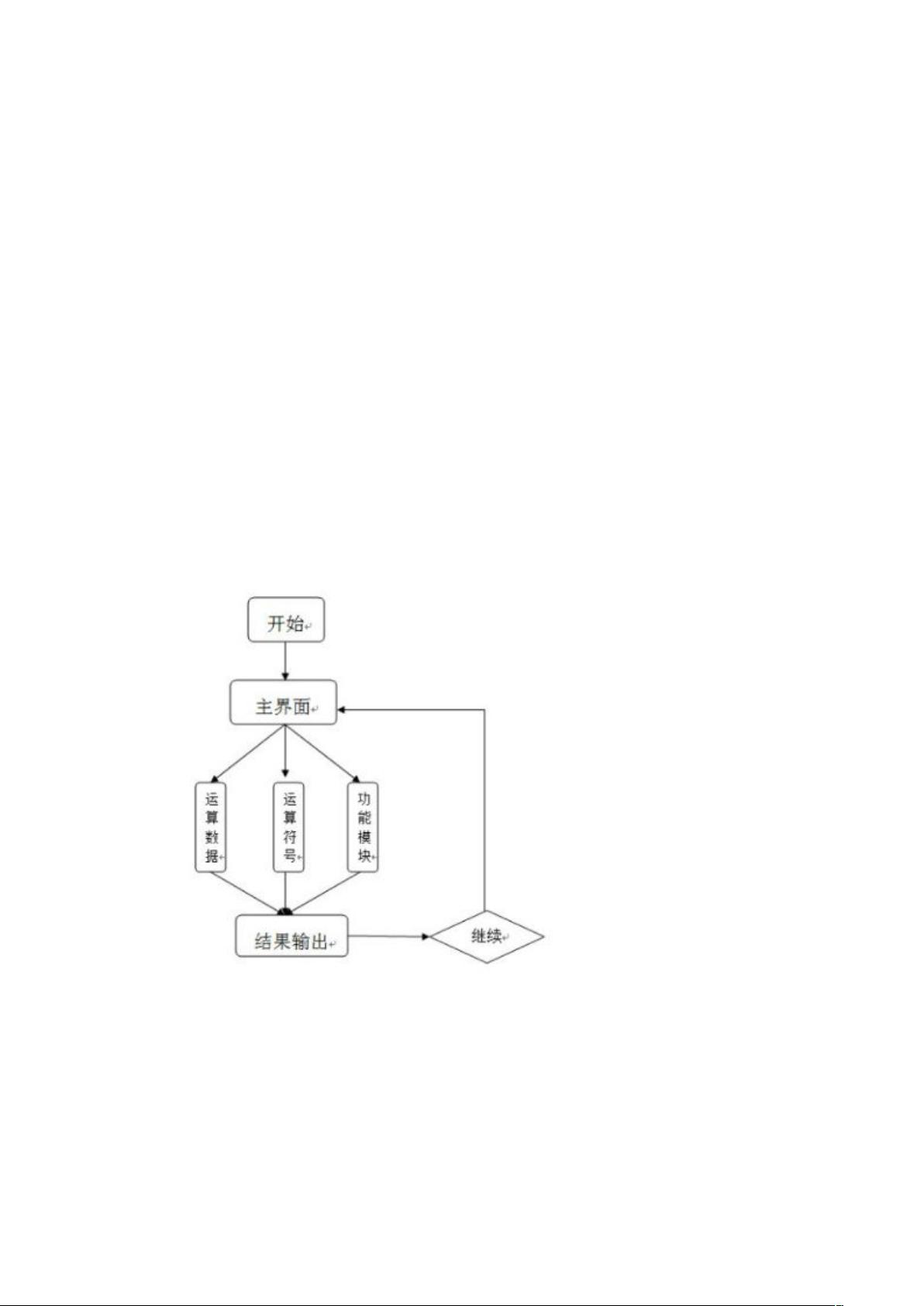
5)设计的简易计算器应能够实现+,-,×,÷,求倒数等基本运算功能,类似于 Windows
中的计算器。
6)设计一个简单,亲和图形界面(GUI)的计算机应用程序
三、总体设计
该计算器是模仿 windows 里的计算器做的,基本功能都已实现,清零,正负号,求倒数,
退格功能都能很好的实现,总体能完成一个计算器的基本功能,但仍有许多地方需要改进。
可能里面的有些算法不是最佳的,仍需改进。
四、具体设计
1,程序流程图
2,源代码(主要代码)
2..1 Calculator 类,程序的主入口
public class Calculator {
public static void main(String[] args) {
new CalculatorUI().init();
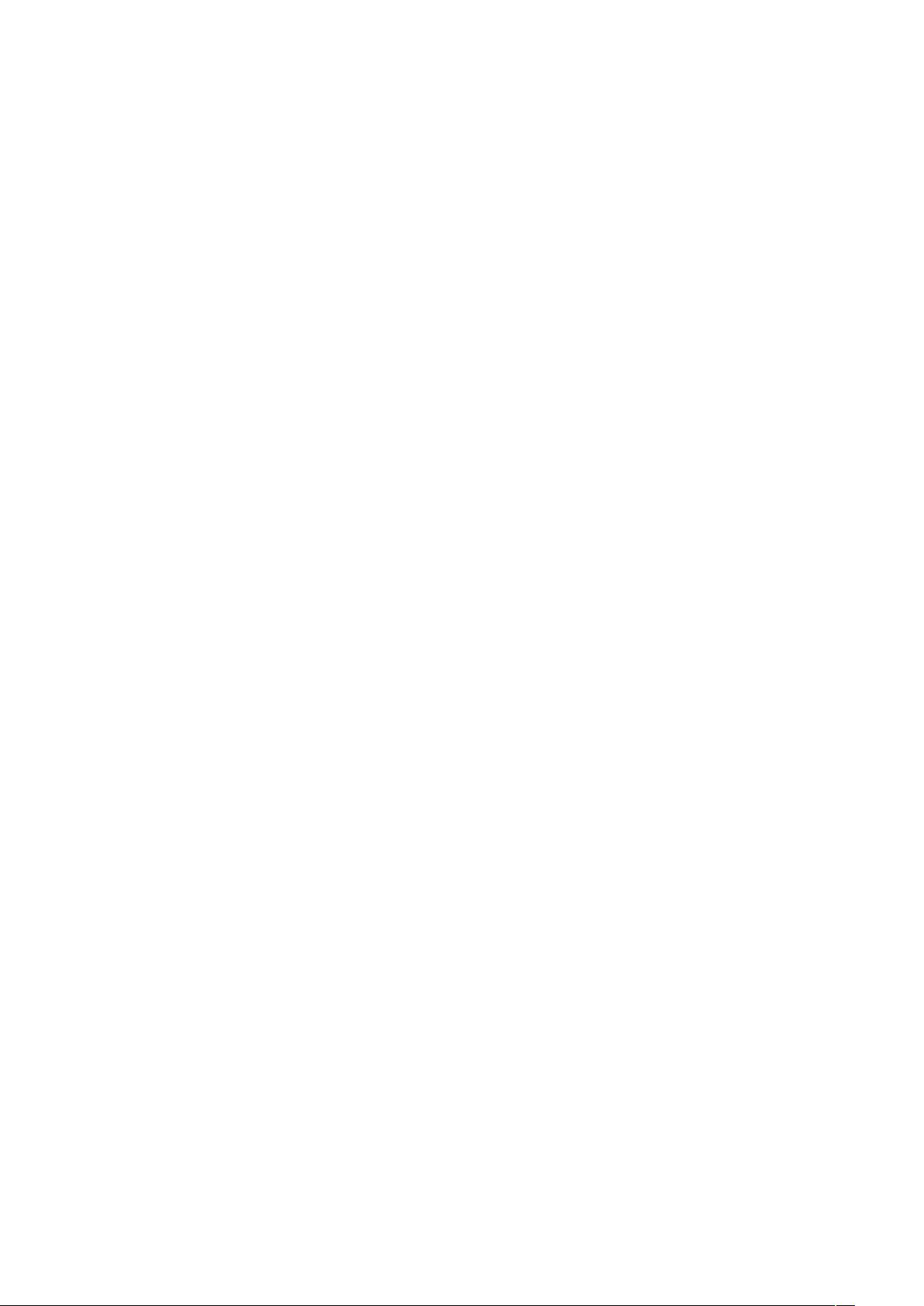
}
}
2.2 CalculatorUI 类,图形化界面,和功能
public class CalculatorUI extends JFrame {
//初始化界面
public void init() {
// 创建组件
bar = new JMenuBar();
bar.setBackground(new Color(217, 223, 240));
menuView = new JMenu("查看(V)");
standard = new JRadioButtonMenuItem("标准型(T)");
standard.setSelected(true);
science = new JRadioButtonMenuItem("科学型(S)");
ButtonGroup group = new ButtonGroup();
group.add(standard);
group.add(science);
menuEdit = new JMenu("编辑(E)");
ctrlc = new JMenuItem("复制(C)");
ctrlv = new JMenuItem("粘贴(P)");
menuHelp = new JMenu("帮助(H)");
viewHelp = new JMenuItem("查看帮助(V)");
aboutCalculator = new JMenuItem("关于计算器(A)");
panel = new JPanel(new BorderLayout(10, 3));
// panel.setBackground(Color.red);
text = new JTextField("0");
text.setEditable(false);
text.setBackground(Color.WHITE);
// Insets(int top, int left, int bottom, int right)
// 创建并初始化具有指定顶部、左边、底部、右边 inset 的新 Insets 对象。
text.setMargin(new Insets(0, 6, 0, 8));
text.setHorizontalAlignment(JTextField.RIGHT);
panelBtn = new JPanel();
panelBtn.setBackground(new Color(217, 223, 240));
panelBtn.setLayout(null);
btnMC = new JLabel(new ImageIcon("image/mc1.png"));
btnMC.setBounds(10, 5, 34, 27);
btnMR = new JLabel(new ImageIcon("image/mr1.png"));
btnMR.setBounds(50, 5, 34, 27);
btnMS = new JLabel(new ImageIcon("image/ms1.png"));
btnMS.setBounds(90, 5, 34, 27);
btnMAdd = new JLabel(new ImageIcon("image/m+1.png"));
btnMAdd.setBounds(130, 5, 34, 27);
btnMReduce = new JLabel(new ImageIcon("image/m-1.png"));
btnMReduce.setBounds(170, 5, 34, 27);
btnBack = new JLabel(new ImageIcon("image/b1.png"));
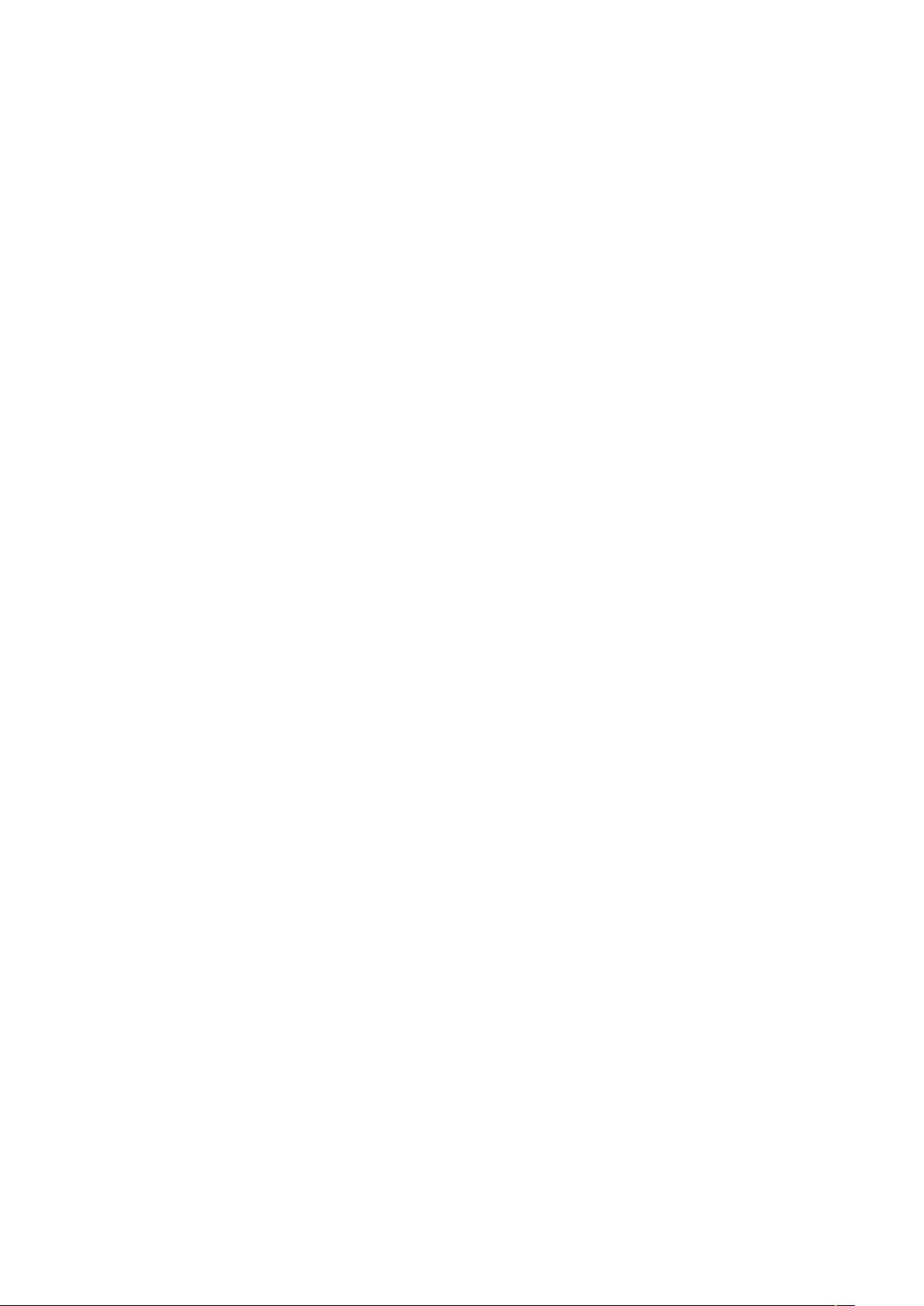
btnBack.setBounds(10, 40, 34, 27);
btnCE = new JLabel(new ImageIcon("image/ce1.png"));
btnCE.setBounds(50, 40, 34, 27);
btnC = new JLabel(new ImageIcon("image/c1.png"));
btnC.setBounds(90, 40, 34, 27);
btnAR = new JLabel(new ImageIcon("image/ar1.png"));
btnAR.setBounds(130, 40, 34, 27);
btnSqrt = new JLabel(new ImageIcon("image/s1.png"));
btnSqrt.setBounds(170, 40, 34, 27);
btn7 = new JLabel(new ImageIcon("image/71.png"));
btn7.setBounds(10, 75, 34, 27);
btn8 = new JLabel(new ImageIcon("image/81.png"));
btn8.setBounds(50, 75, 34, 27);
btn9 = new JLabel(new ImageIcon("image/91.png"));
btn9.setBounds(90, 75, 34, 27);
btnDivide = new JLabel(new ImageIcon("image/d1.png"));
btnDivide.setBounds(130, 75, 34, 27);
btnMod = new JLabel(new ImageIcon("image/mod1.png"));
btnMod.setBounds(170, 75, 34, 27);
btn4 = new JLabel(new ImageIcon("image/41.png"));
btn4.setBounds(10, 110, 34, 27);
btn5 = new JLabel(new ImageIcon("image/51.png"));
btn5.setBounds(50, 110, 34, 27);
btn6 = new JLabel(new ImageIcon("image/61.png"));
btn6.setBounds(90, 110, 34, 27);
btnMultiply = new JLabel(new ImageIcon("image/m1.png"));
btnMultiply.setBounds(130, 110, 34, 27);
btnBottom = new JLabel(new ImageIcon("image/bt1.png"));
btnBottom.setBounds(170, 110, 34, 27);
btn1 = new JLabel(new ImageIcon("image/11.png"));
btn1.setBounds(10, 145, 34, 27);
btn2 = new JLabel(new ImageIcon("image/21.png"));
btn2.setBounds(50, 145, 34, 27);
btn3 = new JLabel(new ImageIcon("image/31.png"));
btn3.setBounds(90, 145, 34, 27);
btnReduce = new JLabel(new ImageIcon("image/r1.png"));
btnReduce.setBounds(130, 145, 34, 27);
btnEqual = new JLabel(new ImageIcon("image/e1.png"));
btnEqual.setBounds(170, 145, 34, 62);
btn0 = new JLabel(new ImageIcon("image/01.png"));
btn0.setBounds(10, 180, 73, 27);
btnPoint = new JLabel(new ImageIcon("image/p1.png"));
btnPoint.setBounds(90, 180, 34, 27);
btnAdd = new JLabel(new ImageIcon("image/a1.png"));
btnAdd.setBounds(130, 180, 34, 27);
// 布局