package org.libsdl.app;
import java.util.Arrays;
import android.app.*;
import android.content.*;
import android.view.*;
import android.view.inputmethod.BaseInputConnection;
import android.view.inputmethod.EditorInfo;
import android.view.inputmethod.InputConnection;
import android.view.inputmethod.InputMethodManager;
import android.widget.AbsoluteLayout;
import android.os.*;
import android.util.Log;
import android.graphics.*;
import android.media.*;
import android.hardware.*;
/**
SDL Activity
*/
public class SDLActivity extends Activity {
private static final String TAG = "SDL";
// Keep track of the paused state
public static boolean mIsPaused = false, mIsSurfaceReady = false, mHasFocus = true;
// Main components
protected static SDLActivity mSingleton;
protected static SDLSurface mSurface;
protected static View mTextEdit;
protected static ViewGroup mLayout;
// This is what SDL runs in. It invokes SDL_main(), eventually
protected static Thread mSDLThread;
// Audio
protected static Thread mAudioThread;
protected static AudioTrack mAudioTrack;
// Load the .so
static {
System.loadLibrary("ffmpeg");
System.loadLibrary("SDL2");
//System.loadLibrary("SDL2_image");
//System.loadLibrary("SDL2_mixer");
//System.loadLibrary("SDL2_net");
//System.loadLibrary("SDL2_ttf");
System.loadLibrary("main");
}
// Setup
@Override
protected void onCreate(Bundle savedInstanceState) {
//Log.v("SDL", "onCreate()");
super.onCreate(savedInstanceState);
// So we can call stuff from static callbacks
mSingleton = this;
// Set up the surface
mSurface = new SDLSurface(getApplication());
mLayout = new AbsoluteLayout(this);
mLayout.addView(mSurface);
setContentView(mLayout);
}
// Events
@Override
protected void onPause() {
Log.v("SDL", "onPause()");
super.onPause();
SDLActivity.handlePause();
}
@Override
protected void onResume() {
Log.v("SDL", "onResume()");
super.onResume();
SDLActivity.handleResume();
}
@Override
public void onWindowFocusChanged(boolean hasFocus) {
super.onWindowFocusChanged(hasFocus);
Log.v("SDL", "onWindowFocusChanged(): " + hasFocus);
SDLActivity.mHasFocus = hasFocus;
if (hasFocus) {
SDLActivity.handleResume();
}
}
@Override
public void onLowMemory() {
Log.v("SDL", "onLowMemory()");
super.onLowMemory();
SDLActivity.nativeLowMemory();
}
@Override
protected void onDestroy() {
super.onDestroy();
Log.v("SDL", "onDestroy()");
// Send a quit message to the application
SDLActivity.nativeQuit();
// Now wait for the SDL thread to quit
if (mSDLThread != null) {
try {
mSDLThread.join();
} catch(Exception e) {
Log.v("SDL", "Problem stopping thread: " + e);
}
mSDLThread = null;
//Log.v("SDL", "Finished waiting for SDL thread");
}
}
@Override
public boolean dispatchKeyEvent(KeyEvent event) {
int keyCode = event.getKeyCode();
// Ignore certain special keys so they're handled by Android
if (keyCode == KeyEvent.KEYCODE_VOLUME_DOWN ||
keyCode == KeyEvent.KEYCODE_VOLUME_UP ||
keyCode == KeyEvent.KEYCODE_CAMERA ||
keyCode == 168 || /* API 11: KeyEvent.KEYCODE_ZOOM_IN */
keyCode == 169 /* API 11: KeyEvent.KEYCODE_ZOOM_OUT */
) {
return false;
}
return super.dispatchKeyEvent(event);
}
/** Called by onPause or surfaceDestroyed. Even if surfaceDestroyed
* is the first to be called, mIsSurfaceReady should still be set
* to 'true' during the call to onPause (in a usual scenario).
*/
public static void handlePause() {
if (!SDLActivity.mIsPaused && SDLActivity.mIsSurfaceReady) {
SDLActivity.mIsPaused = true;
SDLActivity.nativePause();
mSurface.enableSensor(Sensor.TYPE_ACCELEROMETER, false);
}
}
/** Called by onResume or surfaceCreated. An actual resume should be done only when the surface is ready.
* Note: Some Android variants may send multiple surfaceChanged events, so we don't need to resume
* every time we get one of those events, only if it comes after surfaceDestroyed
*/
public static void handleResume() {
if (SDLActivity.mIsPaused && SDLActivity.mIsSurfaceReady && SDLActivity.mHasFocus) {
SDLActivity.mIsPaused = false;
SDLActivity.nativeResume();
mSurface.enableSensor(Sensor.TYPE_ACCELEROMETER, true);
}
}
// Messages from the SDLMain thread
static final int COMMAND_CHANGE_TITLE = 1;
static final int COMMAND_UNUSED = 2;
static final int COMMAND_TEXTEDIT_HIDE = 3;
protected static final int COMMAND_USER = 0x8000;
/**
* This method is called by SDL if SDL did not handle a message itself.
* This happens if a received message contains an unsupported command.
* Method can be overwritten to handle Messages in a different class.
* @param command the command of the message.
* @param param the parameter of the message. May be null.
* @return if the message was handled in overridden method.
*/
protected boolean onUnhandledMessage(int command, Object param) {
return false;
}
/**
* A Handler class for Messages from native SDL applications.
* It uses current Activities as target (e.g. for the title).
* static to prevent implicit references to enclosing object.
*/
protected static class SDLCommandHandler extends Handler {
@Override
public void handleMessage(Message msg) {
Context context = getContext();
if (context == null) {
Log.e(TAG, "error handling message, getContext() returned null");
return;
}
switch (msg.arg1) {
case COMMAND_CHANGE_TITLE:
if (context instanceof Activity) {
((Activity) context).setTitle((String)msg.obj);
} else {
Log.e(TAG, "error handling message, getContext() returned no Activity");
}
break;
case COMMAND_TEXTEDIT_HIDE:
if (mTextEdit != null) {
mTextEdit.setVisibility(View.GONE);
InputMethodManager imm = (InputMethodManager) context.getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(mTextEdit.getWindowToken(), 0);
}
break;
default:
if ((context instanceof SDLActivity) && !((SDLActivity) context).onUnhandledMessage(msg.arg1, msg.obj)) {
Log.e(TAG, "error handling message, command is " + msg.arg1);
}
}
}
}
// Handler for the messages
Handler commandHandler = new SDLCommandHandler();
// Send a message from the SDLMain thread
boolean sendCommand(int command, Object data) {
Message msg = commandHandler.obtainMessage();
msg.arg1 = command;
msg.obj = data;
return commandHandler.sendMessage(msg);
}
// C functions we call
public static native void nativeInit();
public static native void nativeLowMemory();
public static native void nativeQuit();
public static native void nativePause();
public static native void nativeResume();
public static native void onNativeResize(int x, int y, int format);
public static native void onNativeKeyDown(int keycode);
public static native void onNativeKeyUp(int keycode)
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
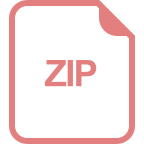
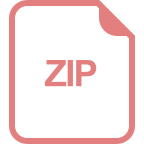
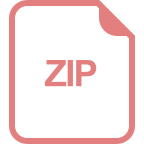
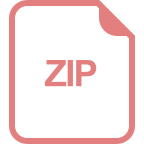
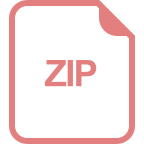
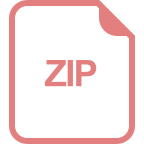
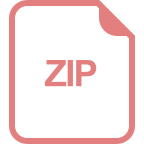
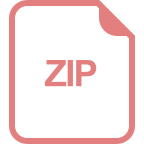
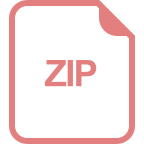
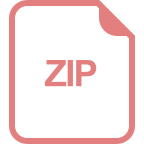
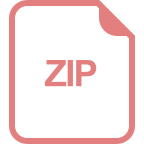
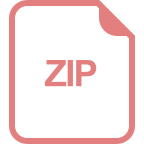
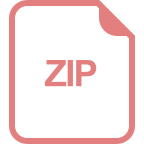
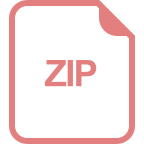
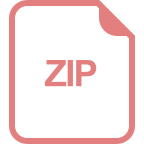
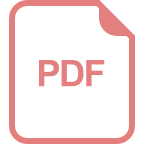
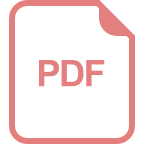
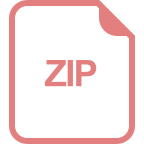
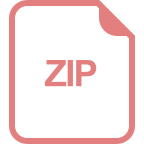
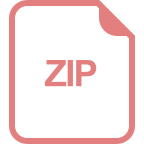
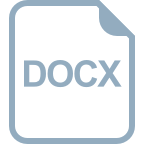
收起资源包目录

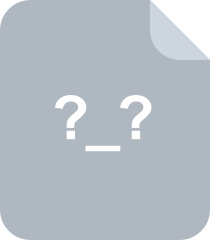
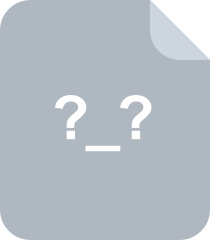
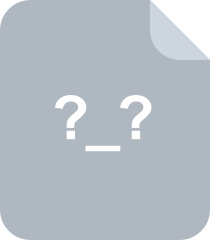
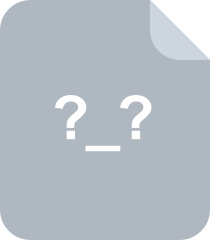
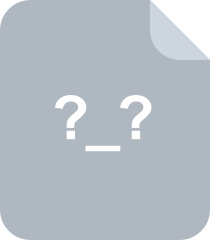
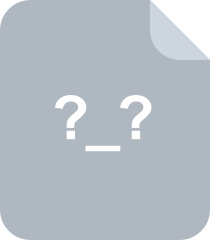
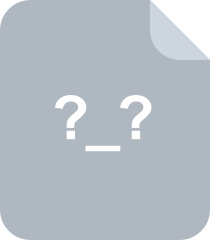
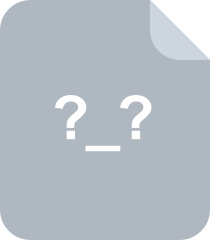
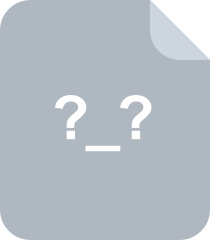
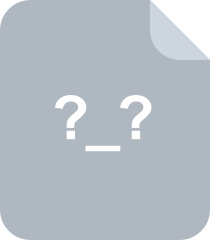
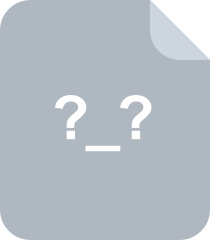
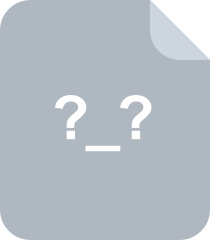
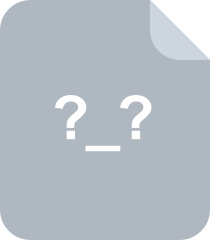
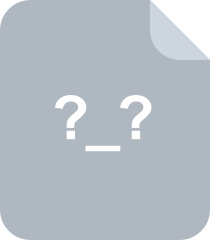
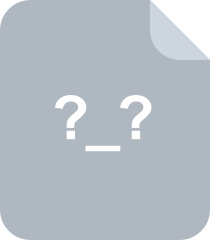
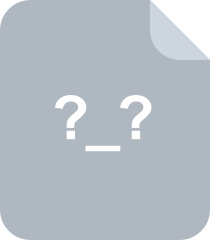
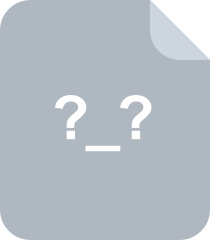
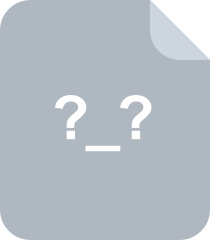
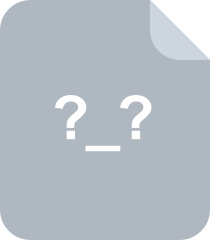
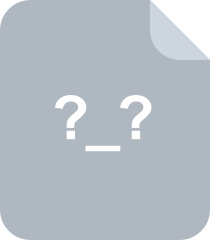
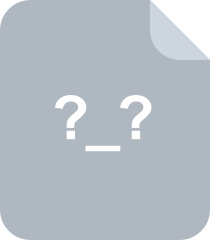
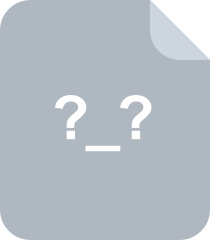
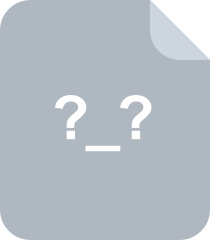
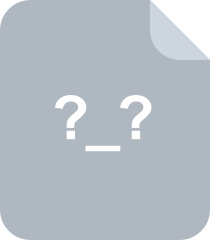
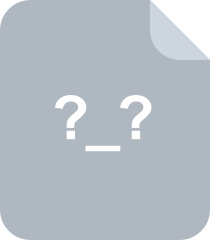
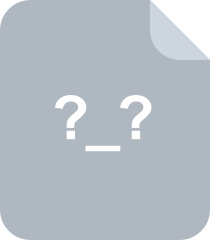
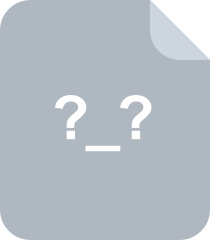
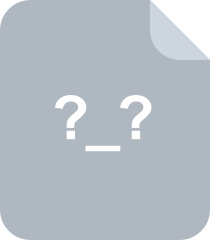
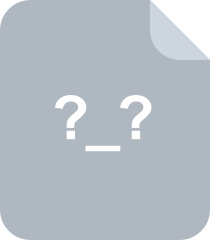
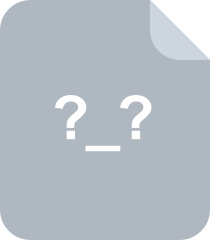
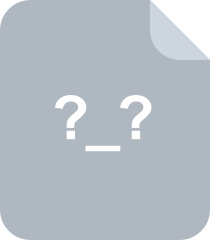
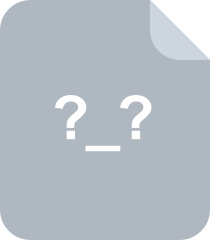
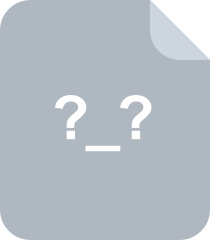
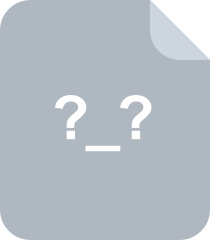
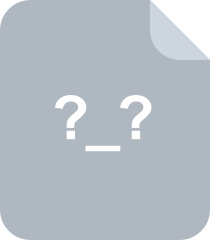
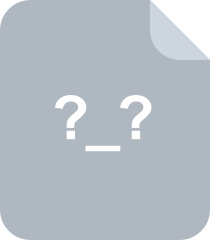
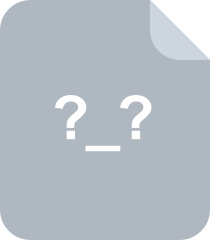
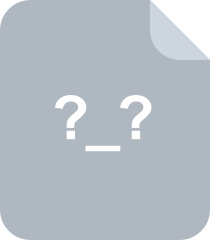
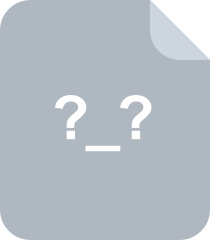
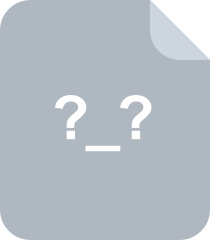
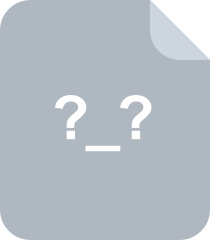
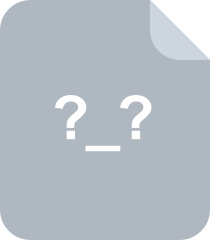
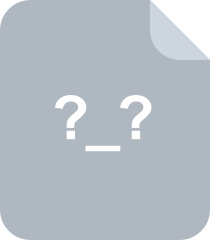
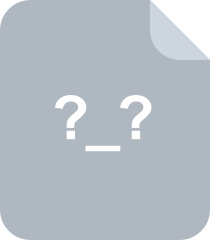
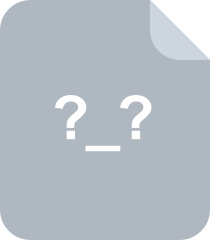
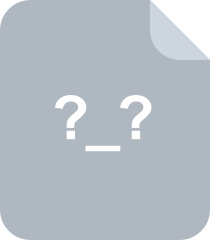
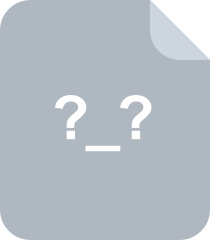
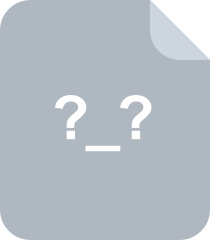
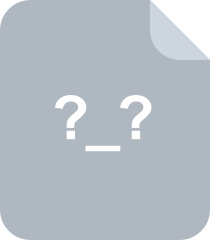
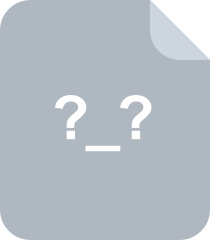
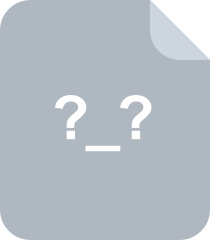
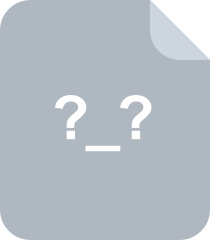
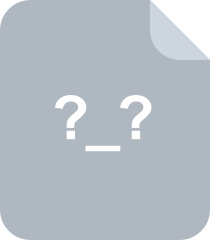
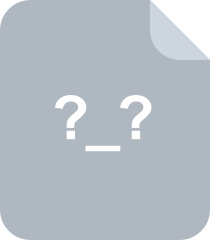
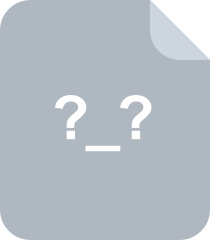
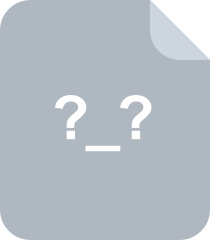
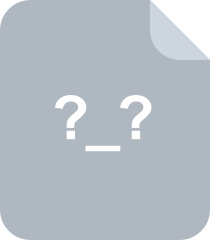
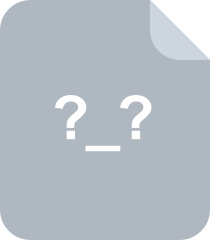
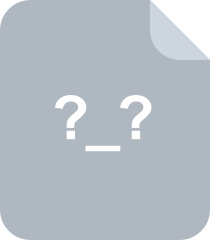
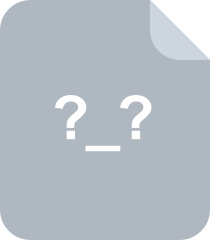
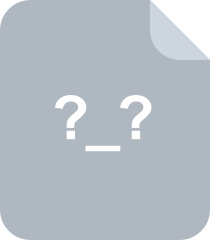
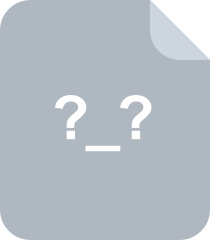
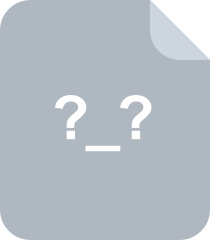
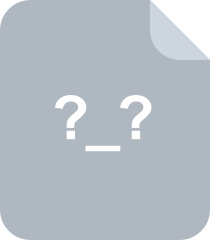
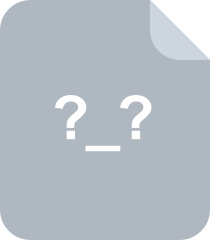
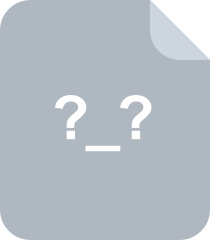
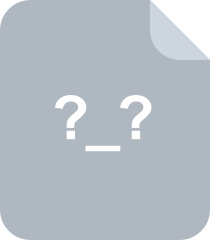
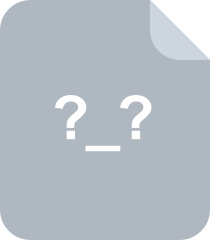
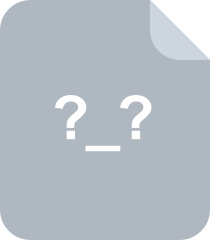
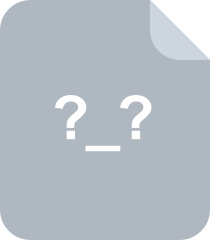
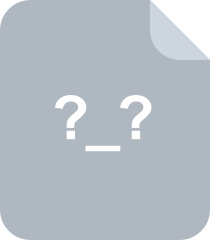
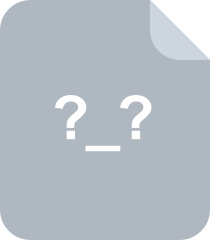
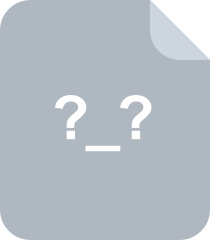
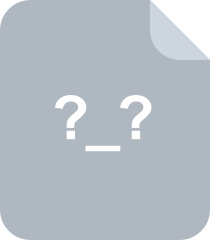
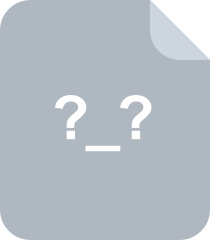
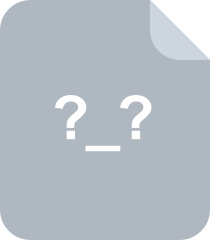
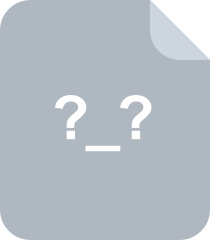
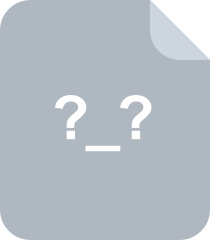
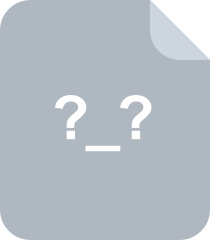
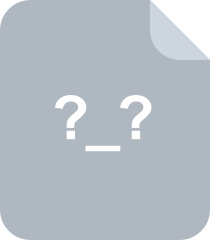
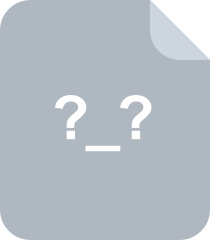
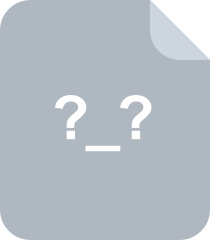
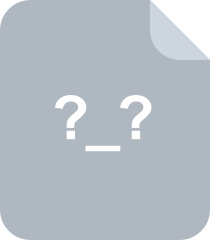
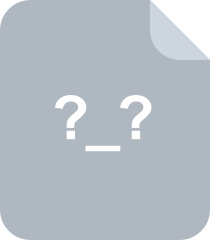
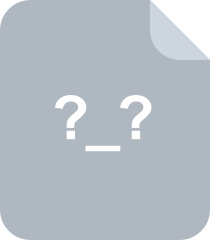
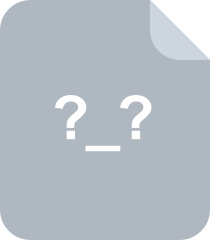
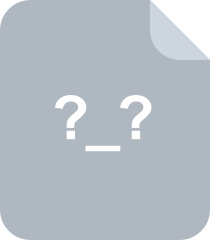
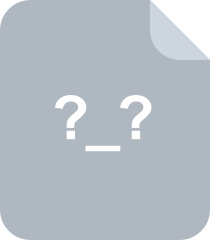
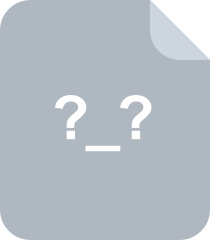
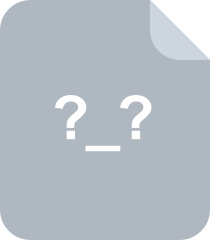
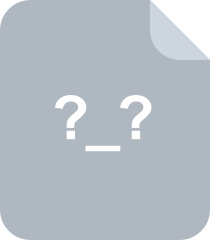
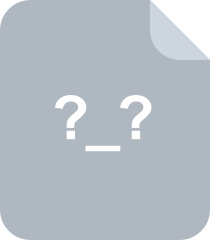
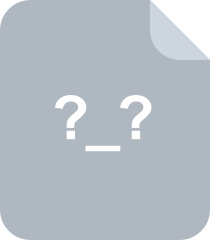
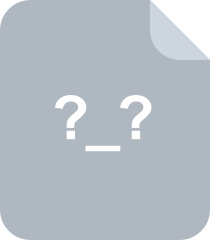
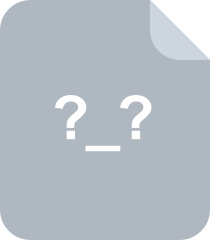
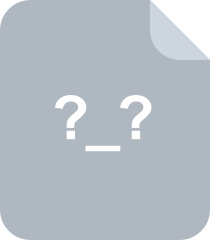
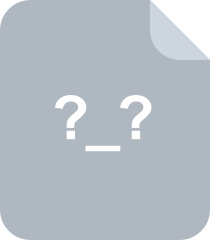
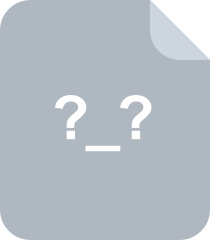
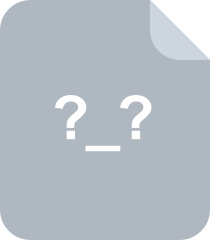
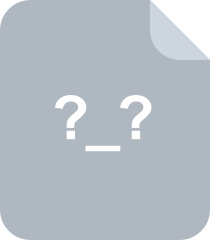
共 655 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
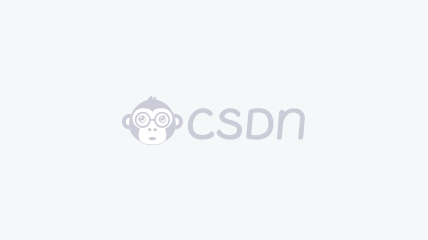

Soft_Leader
- 粉丝: 1506
- 资源: 2850

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

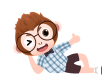
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


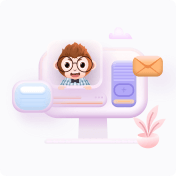
安全验证
文档复制为VIP权益,开通VIP直接复制
