《Android应用源码解析——基于"shop.zip"的深度学习》 在移动开发领域,Android以其开源、灵活的特性,成为开发者们的首选平台之一。尤其是对于初学者和毕业设计的学生,通过研究源码来提升技能是极为有效的学习方法。本文将深入探讨"应用源码之shop.zip",它是一个典型的Android应用程序,包含了JAVA ANDROID相关的代码,适用于代码学习和毕业设计。 我们关注"应用源码之shop.zip"的结构。一个标准的Android项目通常包含以下几个主要部分:`AndroidManifest.xml`(应用配置文件)、`res`(资源文件夹)、`src`(源代码文件夹)、`build.gradle`(构建脚本)以及`libs`(库文件)。在这个压缩包中,我们可以看到开发者如何组织这些元素,理解Android项目的构建逻辑。 在`src`目录下,Java代码主要位于`com.example.shop`(或者根据具体项目名有所不同)包内,这里包含了各个Activity、Service、BroadcastReceiver等组件的实现。Activity是Android应用的核心,它是用户界面的主要载体。通过阅读`MainActivity.java`,我们可以了解应用程序的启动流程,以及如何处理UI事件。例如,`onCreate()`方法是Activity生命周期的起点,用于初始化界面元素;`onOptionsItemSelected()`则处理菜单项的选择事件。 `AndroidManifest.xml`是应用的配置文件,它定义了应用的基本属性、权限、组件声明等。通过这个文件,我们可以看到应用需要哪些权限(如读写存储、访问网络等),以及哪些Activity、Service等可以被其他应用调用。这对于理解和优化应用的安全性和性能至关重要。 在`res`目录下,我们能找到应用程序的各种资源,如布局文件(`layout`)、图标的资源(`drawable`)、字符串资源(`values`)等。布局文件(如`activity_main.xml`)定义了UI的结构,而图标的资源则用于美化应用界面。字符串资源则集中管理应用中的文本,便于国际化和本地化。 `build.gradle`文件则控制了项目的构建过程,包括依赖管理、编译设置等。通过修改Gradle脚本,我们可以定制构建过程,引入第三方库,调整编译选项等。 至于`libs`目录,如果存在,通常存放的是第三方库的JAR或AAR文件,它们为应用提供了额外的功能支持。学习源码时,了解这些库的作用和使用方式,有助于扩展我们的知识面。 "应用源码之shop.zip"为Android开发者提供了一个实战的学习平台。通过分析和实践这个源码,可以深入了解Android应用的设计原则、组件交互、资源管理等多个方面。无论是对于毕业设计还是日常的代码学习,这都是一份宝贵的学习材料。希望每一个热爱编程的你,都能从中受益,不断提升自己的技能,创造出更多优秀的应用。
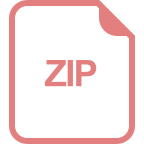
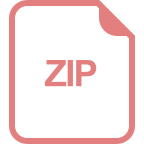
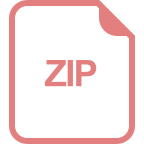
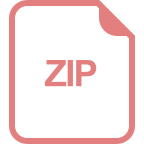
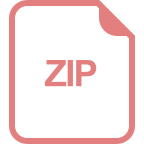
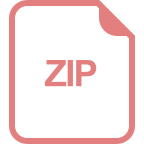
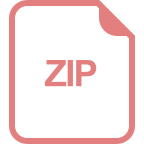
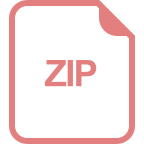
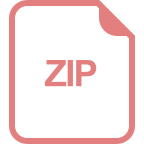
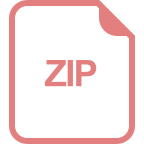
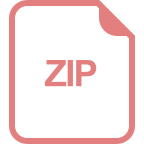
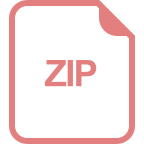
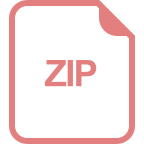
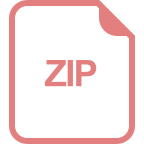
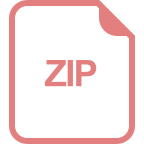
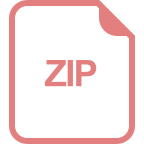
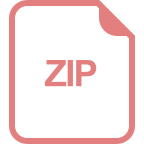
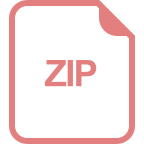
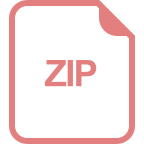
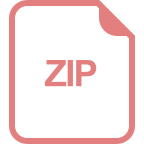
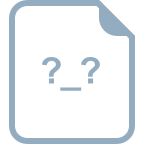
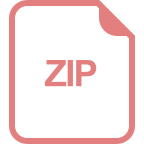
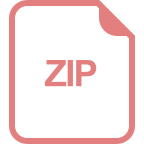
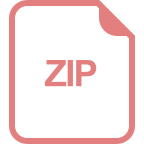
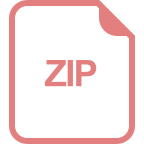




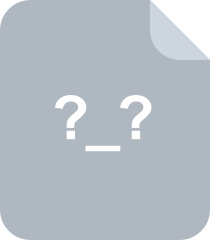




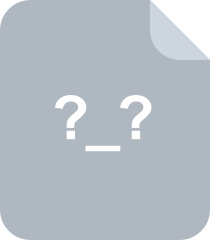
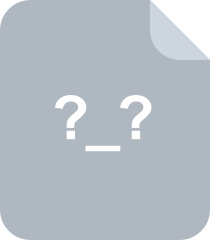
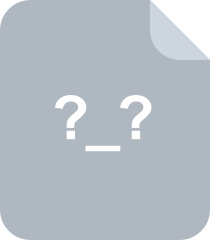
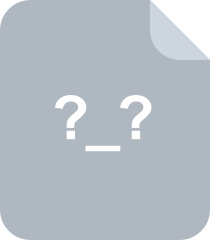


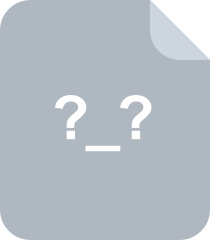

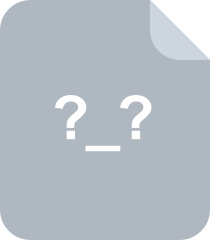
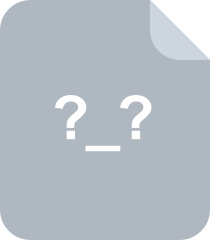
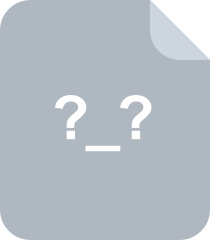
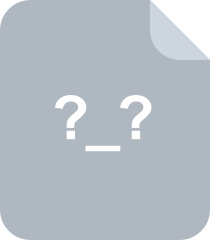

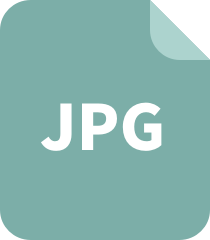
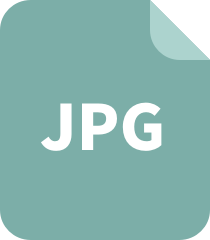
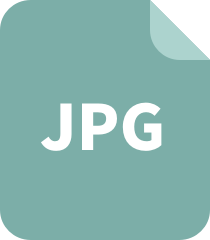
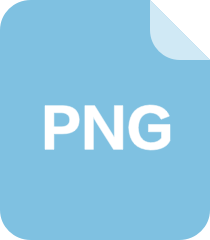

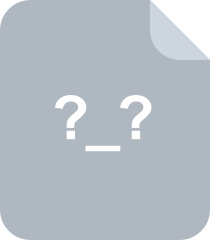
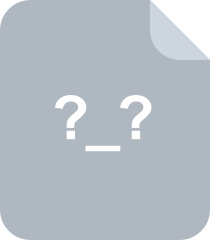
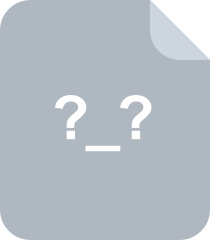



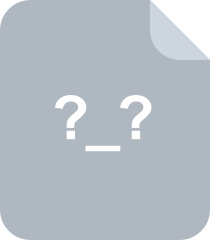
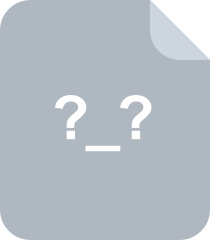
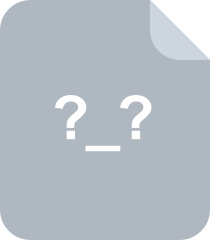
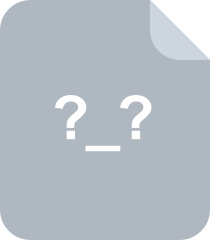
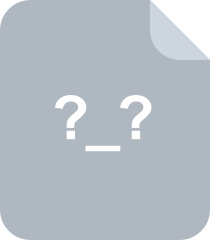
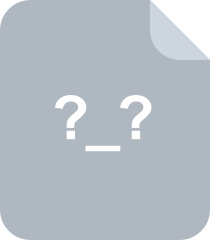
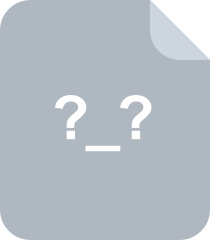
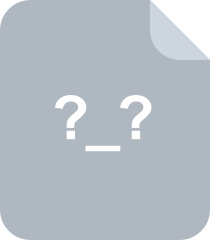
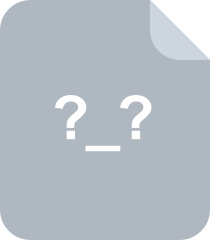
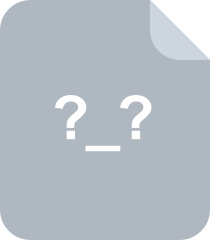
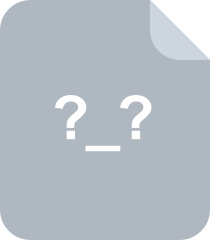
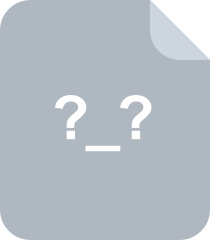
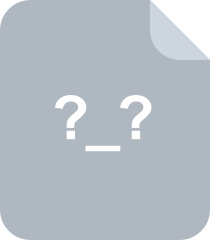
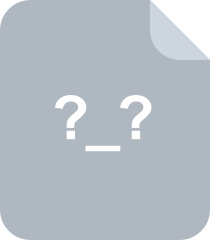
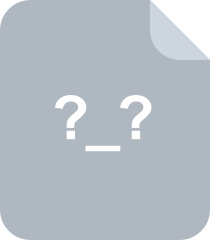
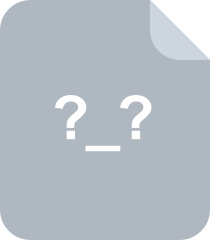




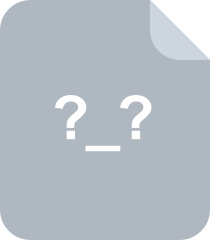

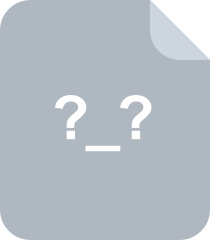




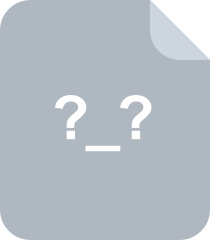
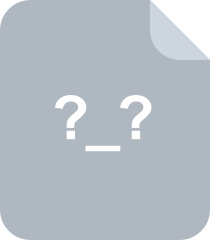
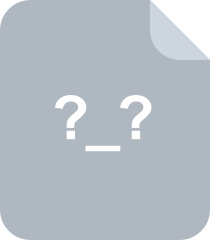
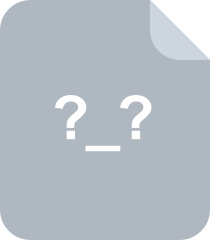


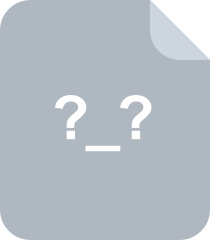

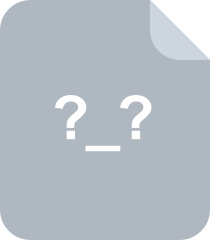
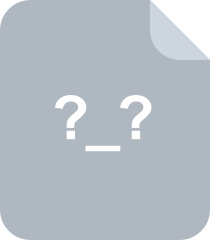
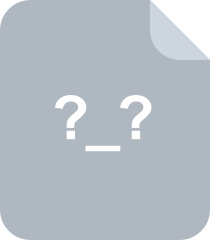
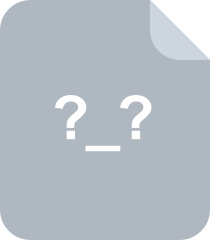

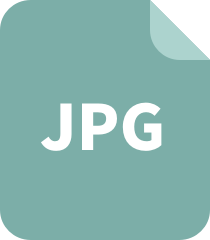
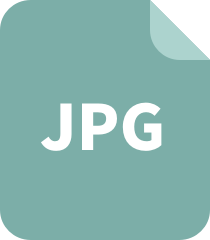
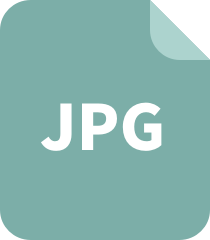
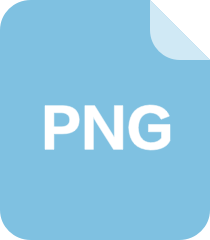

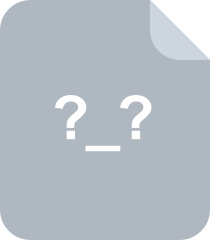
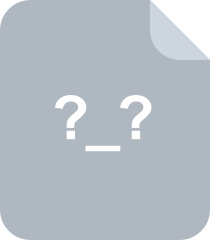
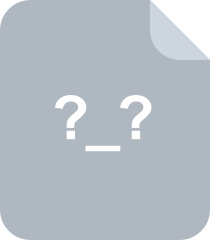



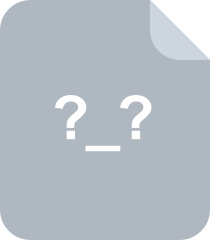
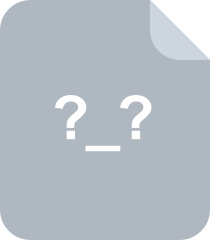
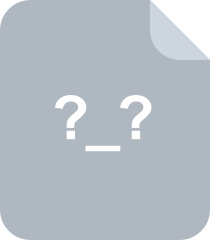
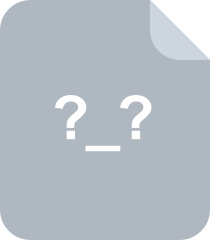
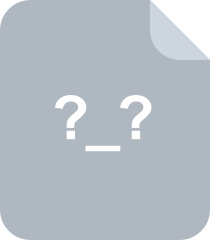
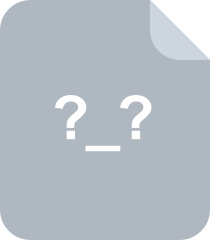
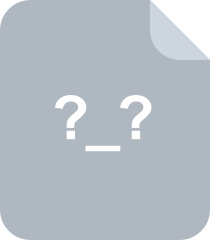
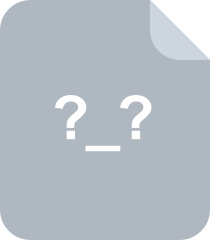
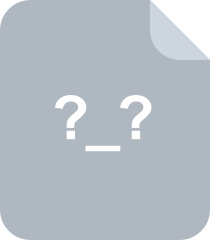
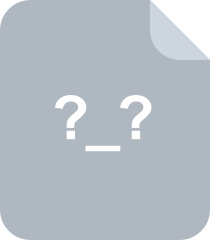
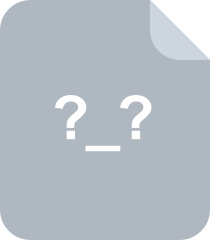
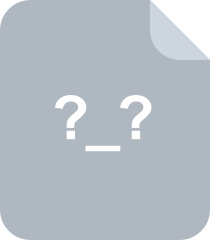
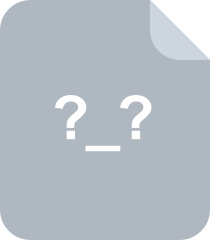
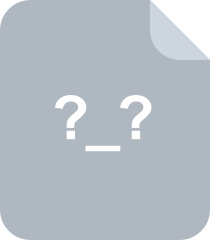
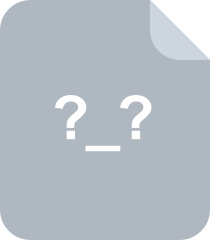
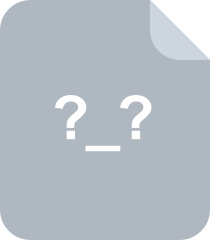




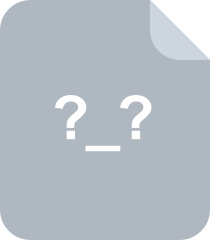
- 1
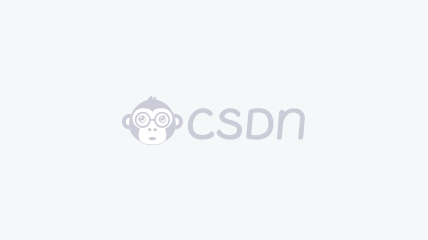

- 粉丝: 1510
- 资源: 2850
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

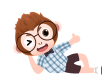
最新资源
- 白色大气风格的孤儿院慈善网站模板.zip
- 白色大气风格的红唇少女女性类网站模板.zip
- 白色大气风格的户外景点旅游公司模板下载.zip
- 白色大气风格的豪车经销商模板下载.zip
- 白色大气风格的户外摄影工作室模板下载.zip
- 白色大气风格的户外旅游公司模板下载.zip
- 白色大气风格的户外旅行装备商城网站源码下载.zip
- 白色大气风格的婚礼布置现场企业网站模板下载.zip
- 白色大气风格的婚礼现场倒计时模板下载.zip
- 白色大气风格的婚礼网站模板下载.zip
- 白色大气风格的建筑商业网站模板下载.rar
- 白色大气风格的建筑设计公司模板下载.zip
- 白色大气风格的家用电器商城整站网站源码下载.zip
- 白色大气风格的健身私人教练模板下载.zip
- 白色大气风格的金融综合服务平台模板下载.zip
- 白色大气风格的景观设计HTML网站模板.zip

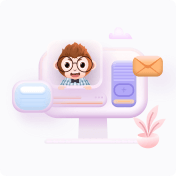
