.net 图片的二进制数据库存储和显示

根据提供的文件信息,我们可以深入探讨如何在.NET框架中处理图片的二进制数据存储与显示。这是一项在Web开发中非常实用的技术,特别是在处理大量图片数据时,通过将图片存储为二进制数据而非传统的文件系统路径,可以提高数据处理效率和减少服务器资源占用。 ### 1. 图片的二进制数据存储 #### C# 实现 在C#中,我们可以利用`FileStream`类来读取文件,并将其转换为二进制数组,然后再将这些二进制数据存储到数据库中。下面是一个具体的实现示例: ```csharp // 图片存储 protected void Button1_Click(object sender, EventArgs e) { // 图片路径 string strPath = "~/photo/03.JPG"; string strPhotoPath = Server.MapPath(strPath); // 获取图片 FileStream fs = new System.IO.FileStream(strPhotoPath, FileMode.Open, FileAccess.Read); BinaryReader br = new BinaryReader(fs); byte[] photo = br.ReadBytes((int)fs.Length); br.Close(); fs.Close(); // 连接数据库 SqlConnection myConn = new SqlConnection("DataSource=127.0.0.1;InitialCatalog=TestDB;UserID=sa;Password=sa"); // SQL语句 string strComm = "INSERT INTO personPhoto (personName, personPhotoPath, personPhoto) VALUES (@name, @path, @photo)"; SqlCommand myComm = new SqlCommand(strComm, myConn); myComm.Parameters.AddWithValue("@name", "wangwu"); myComm.Parameters.AddWithValue("@path", strPath); myComm.Parameters.AddWithValue("@photo", SqlDbType.Binary).Value = photo; // 执行SQL myConn.Open(); myComm.ExecuteNonQuery(); myConn.Close(); } ``` 在这个示例中,我们首先定义了一个文件路径,并使用`FileStream`和`BinaryReader`来读取文件内容并转换为字节数组`photo`。然后,通过一个`SqlConnection`对象连接到数据库,并构建一个插入语句,其中`@photo`参数就是之前获取到的二进制数据。 #### VB.NET 实现 在VB.NET中,实现逻辑与C#相似,主要区别在于语法的不同。下面是一个VB.NET版本的示例: ```vbnet Protected Sub Button1_Click(ByVal sender As Object, ByVal e As EventArgs) ' 图片路径 Dim strPath As String = "~/photo/03.JPG" Dim strPhotoPath As String = Server.MapPath(strPath) ' 获取图片 Dim fs As FileStream = New System.IO.FileStream(strPhotoPath, FileMode.Open, FileAccess.Read) Dim br As BinaryReader = New BinaryReader(fs) Dim photo() As Byte = br.ReadBytes(CType(fs.Length, Integer)) br.Close() fs.Close() ' 连接数据库 Dim myConn As New SqlConnection("DataSource=127.0.0.1;InitialCatalog=TestDB;UserID=sa;Password=sa") ' SQL语句 Dim strComm As String = "INSERT INTO personPhoto (personName, personPhotoPath, personPhoto) VALUES (@name, @path, @photo)" Dim myComm As New SqlCommand(strComm, myConn) myComm.Parameters.AddWithValue("@name", "wangwu") myComm.Parameters.AddWithValue("@path", strPath) myComm.Parameters.AddWithValue("@photo", SqlDbType.Binary).Value = photo ' 执行SQL myConn.Open() myComm.ExecuteNonQuery() myConn.Close() End Sub ``` ### 2. 图片的页面显示 接下来,我们来看看如何从数据库中读取这些二进制数据并在网页上显示图片。 #### 使用`Image`控件显示 一种简单的方法是使用ASP.NET中的`Image`控件来显示图片。可以通过以下步骤实现: 1. **从数据库读取二进制数据**:使用`SqlCommand`执行查询语句,获取存储在数据库中的二进制数据。 2. **将二进制数据转换为图片**:使用`MemoryStream`和`Image.FromStream()`方法将二进制数据转换为图片。 ```csharp // 假设从数据库中获取到了图片的二进制数据 imgByte byte[] imgByte = (byte[])rd.GetValue(0); MemoryStream ms = new MemoryStream(imgByte); Image img = Image.FromStream(ms); ``` #### 在GridView中显示图片 另一种常见的应用场景是在`GridView`中显示图片。这里可以使用`ImageField`或`BoundField`结合模板字段来显示图片。 1. **设置`ImageField`**:在`GridView`中添加一个`ImageField`控件,并设置其属性以从数据库中获取图片的二进制数据。 2. **绑定数据源**:将`GridView`的数据源设置为包含图片二进制数据的数据表。 ```csharp // 假设已有一个名为 GridView1 的 GridView 控件 GridView1.DataSource = yourDataSource; GridView1.DataBind(); ``` 以上就是关于如何在.NET中处理图片的二进制数据存储与显示的基本介绍。通过这种方式,不仅可以有效地管理图片资源,还能提高应用程序的整体性能。
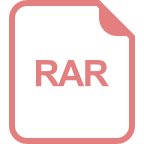
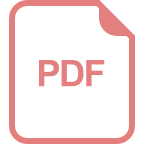
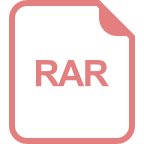
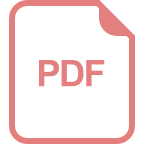
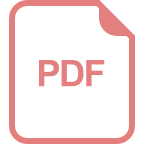
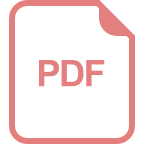
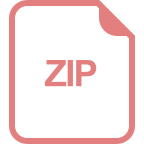
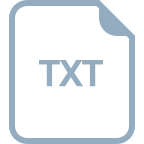
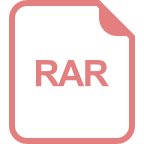
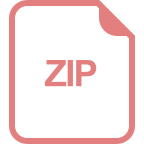
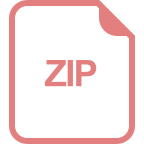
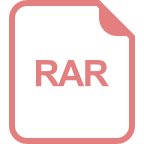
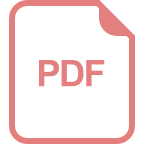
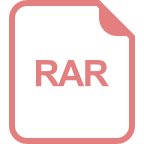
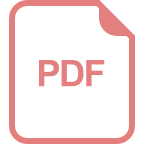
1.将图片以二进制存入数据库
2.读取二进制图片在页面显示
3.设置Image控件显示从数据库中读出的二进制图片
4.GridView中ImageField以URL方式显示图片
5.GridView显示读出的二进制图片
====================
用到的知识点:
FileSteam fs=new FileSteam(fileName,FileMode.Open,FileAccess.Read);
BinaryReader binaryReader=new BinaryReader(fs);
byte[] myByte=new byte[fs.length];
binaryReader.Read(myByte,0,Convet.ToInt32(fs.Length));
fs.close()
FileInfo fl=new FileInfo(fileName);
string imgName=fl.name;
Memorystream ms=new MemoryStream();
byte[] imgByte=(byte[])rd.GetValue(0);
Stream imgStream=new MemoryStream(imgByte);
pb.Image=Image.FromStream(imgStream);
DataView dv=(DataView)cmb.SelectedItem;
string imgId=dv.Row["id"].ToString();
============================
1.将图片以二进制存入数据库
C#
--------------------------
//保存图片到数据库
protected void Button1_Click(object sender, EventArgs e)
{
//图片路径
string strPath = "~/photo/03.JPG";
string strPhotoPath = Server.MapPath(strPath);
//读取图片
FileStream fs = new System.IO.FileStream(strPhotoPath, FileMode.Open, FileAccess.Read);
BinaryReader br = new BinaryReader(fs);
byte[] photo = br.ReadBytes((int)fs.Length);
br.Close();
fs.Close();
//存入
SqlConnection myConn = new SqlConnection("Data Source=127.0.0.1;Initial Catalog=TestDB;User ID=sa;Password=sa");
string strComm = " INSERT INTO personPhoto(personName, personPhotoPath, personPhoto) ";
strComm += " VALUES('wangwu', '" + strPath + "', @photoBinary )";
SqlCommand myComm = new SqlCommand(strComm, myConn);
myComm.Parameters.Add("@photoBinary", SqlDbType.Binary,photo.Length);
myComm.Parameters["@photoBinary"].Value = photo;
myConn.Open();
myComm.ExecuteNonQuery();
myConn.Close();
}
VB.NET
剩余8页未读,继续阅读
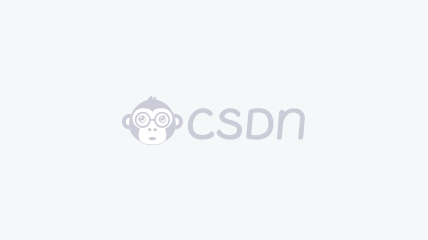
- 喏喏_2019-01-11相当不错,学习了
- hujianjoy2013-02-26找了好久,这个真的解决了我越到的难题啊!

- 粉丝: 72
- 资源: 17
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

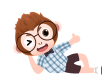
最新资源
- (源码)基于Java和MySQL的学生信息管理系统.zip
- (源码)基于ASP.NET Core的零售供应链管理系统.zip
- (源码)基于PythonSpleeter的戏曲音频处理系统.zip
- (源码)基于Spring Boot的监控与日志管理系统.zip
- (源码)基于C++的Unix V6++二级文件系统.zip
- (源码)基于Spring Boot和JPA的皮皮虾图片收集系统.zip
- (源码)基于Arduino和Python的实时歌曲信息液晶显示屏展示系统.zip
- (源码)基于C++和C混合模式的操作系统开发项目.zip
- (源码)基于Arduino的全球天气监控系统.zip
- OpenCVForUnity2.6.0.unitypackage

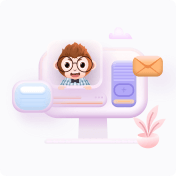
