# ShellJS - Unix shell commands for Node.js [](http://travis-ci.org/arturadib/shelljs)
ShellJS is a portable **(Windows/Linux/OS X)** implementation of Unix shell commands on top of the Node.js API. You can use it to eliminate your shell script's dependency on Unix while still keeping its familiar and powerful commands. You can also install it globally so you can run it from outside Node projects - say goodbye to those gnarly Bash scripts!
The project is [unit-tested](http://travis-ci.org/arturadib/shelljs) and battled-tested in projects like:
+ [PDF.js](http://github.com/mozilla/pdf.js) - Firefox's next-gen PDF reader
+ [Firebug](http://getfirebug.com/) - Firefox's infamous debugger
+ [JSHint](http://jshint.com) - Most popular JavaScript linter
+ [Yeoman](http://yeoman.io/) - Web application stack and development tool
+ [Deployd.com](http://deployd.com) - Open source PaaS for quick API backend generation
and [many more](https://npmjs.org/browse/depended/shelljs).
## Installing
Via npm:
```bash
$ npm install [-g] shelljs
```
If the global option `-g` is specified, the binary `shjs` will be installed. This makes it possible to
run ShellJS scripts much like any shell script from the command line, i.e. without requiring a `node_modules` folder:
```bash
$ shjs my_script
```
You can also just copy `shell.js` into your project's directory, and `require()` accordingly.
## Examples
### JavaScript
```javascript
require('shelljs/global');
if (!which('git')) {
echo('Sorry, this script requires git');
exit(1);
}
// Copy files to release dir
mkdir('-p', 'out/Release');
cp('-R', 'stuff/*', 'out/Release');
// Replace macros in each .js file
cd('lib');
ls('*.js').forEach(function(file) {
sed('-i', 'BUILD_VERSION', 'v0.1.2', file);
sed('-i', /.*REMOVE_THIS_LINE.*\n/, '', file);
sed('-i', /.*REPLACE_LINE_WITH_MACRO.*\n/, cat('macro.js'), file);
});
cd('..');
// Run external tool synchronously
if (exec('git commit -am "Auto-commit"').code !== 0) {
echo('Error: Git commit failed');
exit(1);
}
```
### CoffeeScript
```coffeescript
require 'shelljs/global'
if not which 'git'
echo 'Sorry, this script requires git'
exit 1
# Copy files to release dir
mkdir '-p', 'out/Release'
cp '-R', 'stuff/*', 'out/Release'
# Replace macros in each .js file
cd 'lib'
for file in ls '*.js'
sed '-i', 'BUILD_VERSION', 'v0.1.2', file
sed '-i', /.*REMOVE_THIS_LINE.*\n/, '', file
sed '-i', /.*REPLACE_LINE_WITH_MACRO.*\n/, cat 'macro.js', file
cd '..'
# Run external tool synchronously
if (exec 'git commit -am "Auto-commit"').code != 0
echo 'Error: Git commit failed'
exit 1
```
## Global vs. Local
The example above uses the convenience script `shelljs/global` to reduce verbosity. If polluting your global namespace is not desirable, simply require `shelljs`.
Example:
```javascript
var shell = require('shelljs');
shell.echo('hello world');
```
## Make tool
A convenience script `shelljs/make` is also provided to mimic the behavior of a Unix Makefile. In this case all shell objects are global, and command line arguments will cause the script to execute only the corresponding function in the global `target` object. To avoid redundant calls, target functions are executed only once per script.
Example (CoffeeScript):
```coffeescript
require 'shelljs/make'
target.all = ->
target.bundle()
target.docs()
target.bundle = ->
cd __dirname
mkdir 'build'
cd 'lib'
(cat '*.js').to '../build/output.js'
target.docs = ->
cd __dirname
mkdir 'docs'
cd 'lib'
for file in ls '*.js'
text = grep '//@', file # extract special comments
text.replace '//@', '' # remove comment tags
text.to 'docs/my_docs.md'
```
To run the target `all`, call the above script without arguments: `$ node make`. To run the target `docs`: `$ node make docs`, and so on.
<!--
DO NOT MODIFY BEYOND THIS POINT - IT'S AUTOMATICALLY GENERATED
-->
## Command reference
All commands run synchronously, unless otherwise stated.
### cd('dir')
Changes to directory `dir` for the duration of the script
### pwd()
Returns the current directory.
### ls([options ,] path [,path ...])
### ls([options ,] path_array)
Available options:
+ `-R`: recursive
+ `-A`: all files (include files beginning with `.`, except for `.` and `..`)
Examples:
```javascript
ls('projs/*.js');
ls('-R', '/users/me', '/tmp');
ls('-R', ['/users/me', '/tmp']); // same as above
```
Returns array of files in the given path, or in current directory if no path provided.
### find(path [,path ...])
### find(path_array)
Examples:
```javascript
find('src', 'lib');
find(['src', 'lib']); // same as above
find('.').filter(function(file) { return file.match(/\.js$/); });
```
Returns array of all files (however deep) in the given paths.
The main difference from `ls('-R', path)` is that the resulting file names
include the base directories, e.g. `lib/resources/file1` instead of just `file1`.
### cp([options ,] source [,source ...], dest)
### cp([options ,] source_array, dest)
Available options:
+ `-f`: force
+ `-r, -R`: recursive
Examples:
```javascript
cp('file1', 'dir1');
cp('-Rf', '/tmp/*', '/usr/local/*', '/home/tmp');
cp('-Rf', ['/tmp/*', '/usr/local/*'], '/home/tmp'); // same as above
```
Copies files. The wildcard `*` is accepted.
### rm([options ,] file [, file ...])
### rm([options ,] file_array)
Available options:
+ `-f`: force
+ `-r, -R`: recursive
Examples:
```javascript
rm('-rf', '/tmp/*');
rm('some_file.txt', 'another_file.txt');
rm(['some_file.txt', 'another_file.txt']); // same as above
```
Removes files. The wildcard `*` is accepted.
### mv(source [, source ...], dest')
### mv(source_array, dest')
Available options:
+ `f`: force
Examples:
```javascript
mv('-f', 'file', 'dir/');
mv('file1', 'file2', 'dir/');
mv(['file1', 'file2'], 'dir/'); // same as above
```
Moves files. The wildcard `*` is accepted.
### mkdir([options ,] dir [, dir ...])
### mkdir([options ,] dir_array)
Available options:
+ `p`: full path (will create intermediate dirs if necessary)
Examples:
```javascript
mkdir('-p', '/tmp/a/b/c/d', '/tmp/e/f/g');
mkdir('-p', ['/tmp/a/b/c/d', '/tmp/e/f/g']); // same as above
```
Creates directories.
### test(expression)
Available expression primaries:
+ `'-b', 'path'`: true if path is a block device
+ `'-c', 'path'`: true if path is a character device
+ `'-d', 'path'`: true if path is a directory
+ `'-e', 'path'`: true if path exists
+ `'-f', 'path'`: true if path is a regular file
+ `'-L', 'path'`: true if path is a symboilc link
+ `'-p', 'path'`: true if path is a pipe (FIFO)
+ `'-S', 'path'`: true if path is a socket
Examples:
```javascript
if (test('-d', path)) { /* do something with dir */ };
if (!test('-f', path)) continue; // skip if it's a regular file
```
Evaluates expression using the available primaries and returns corresponding value.
### cat(file [, file ...])
### cat(file_array)
Examples:
```javascript
var str = cat('file*.txt');
var str = cat('file1', 'file2');
var str = cat(['file1', 'file2']); // same as above
```
Returns a string containing the given file, or a concatenated string
containing the files if more than one file is given (a new line character is
introduced between each file). Wildcard `*` accepted.
### 'string'.to(file)
Examples:
```javascript
cat('input.txt').to('output.txt');
```
Analogous to the redirection operator `>` in Unix, but works with JavaScript strings (such as
those returned by `cat`, `grep`, etc). _Like Unix redirections, `to()` will overwrite any existing file!_
### sed([options ,] search_regex, replace_str, file)
Available options:
+ `-i`: Replace contents of 'file' in-place. _Note that no backups will be created!_
Examples:
```javascript
sed('-i', 'PROGRAM_VERSION', 'v0.1.3', 'source.js');
sed(/.*DELETE_THIS_LINE.*\n/, '', 'source.js');
```
Reads an input string from `file` and performs a JavaScript `replace()` on the in
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
pdf.js 是一个技术原型主要用于在 HTML5 平台上展示 PDF 文档,无需任何本地技术支持。pdf.js是一个HTML5技术的实验,探索实现一个没有本地代码支持,有效率的PDF渲染。 pdf.js是社区驱动和Mozilla Labs的支持。我们的目标是创建一个通用的,基于标准的网络平台解析和渲染PDF,并最终释放出的PDF阅读器的扩展。 示例地址: http://mozilla.github.com/pdf.js/web/viewer.html
资源推荐
资源详情
资源评论
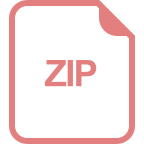
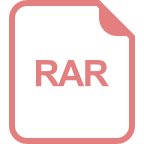
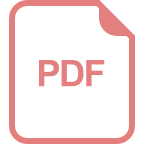
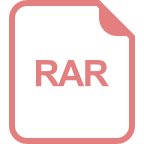
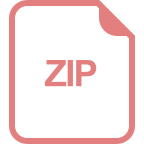
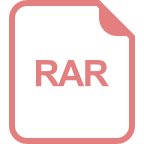
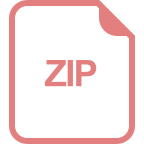
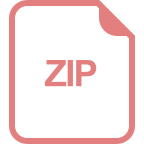
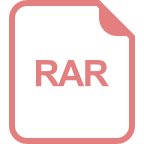
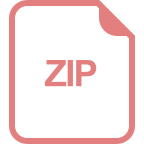
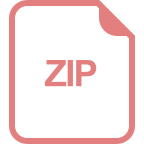
收起资源包目录

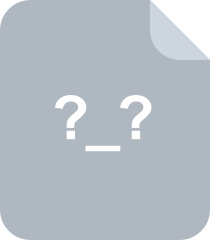
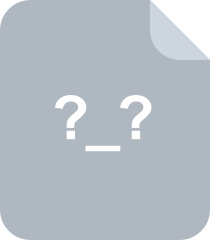
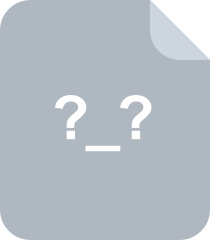
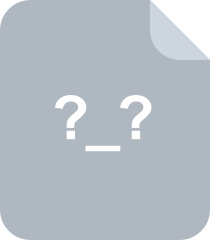
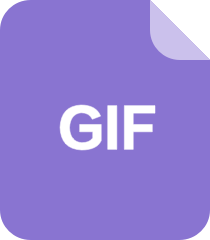
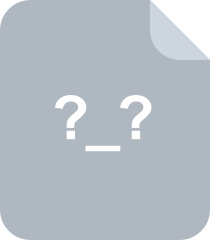
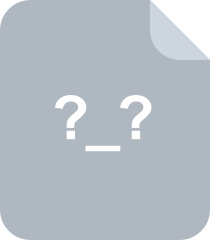
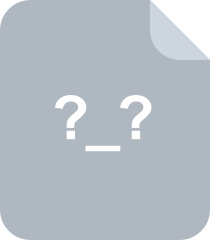
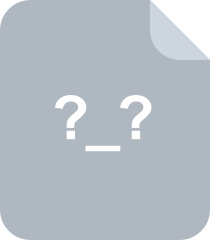
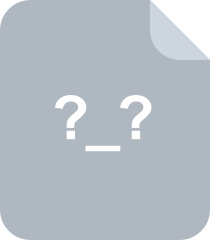
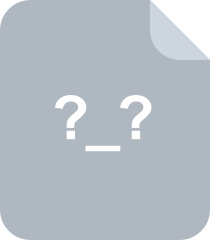
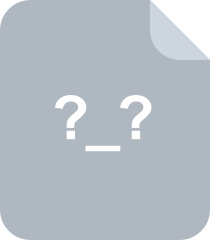
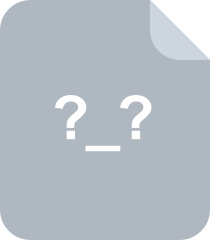
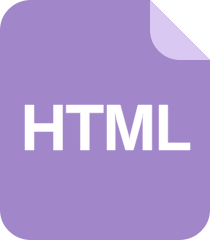
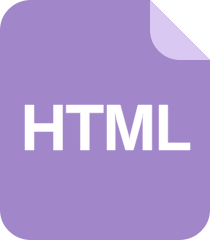
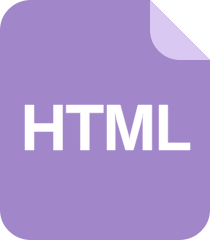
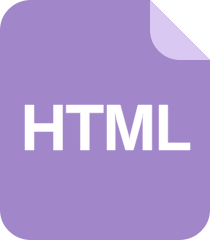
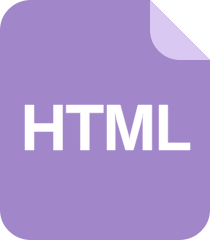
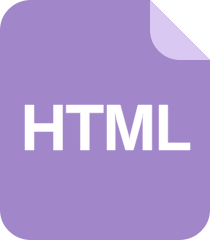
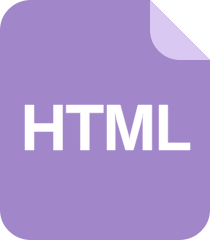
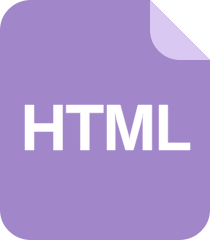
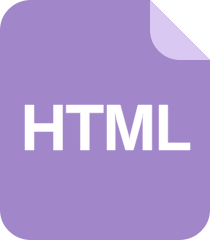
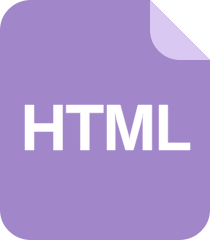
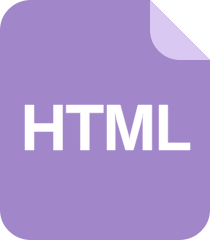
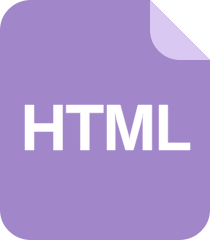
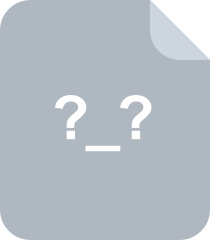
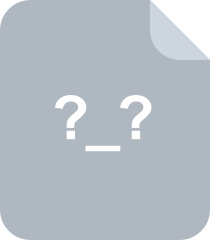
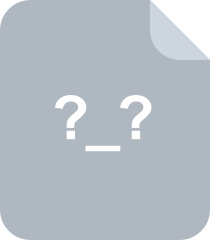
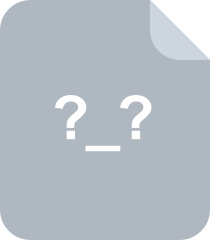
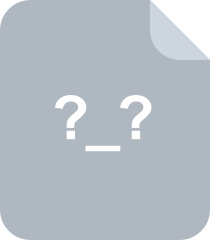
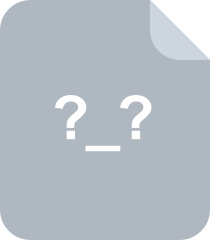
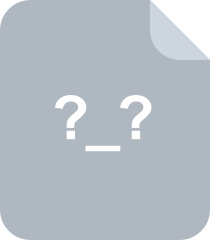
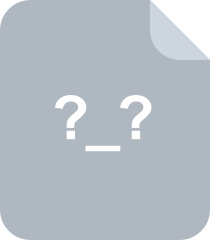
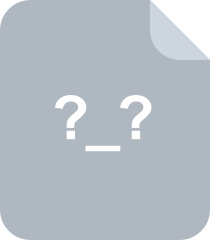
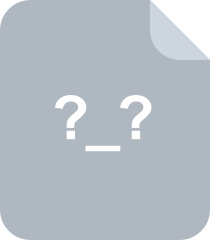
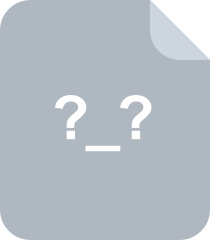
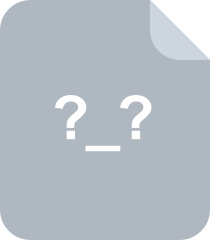
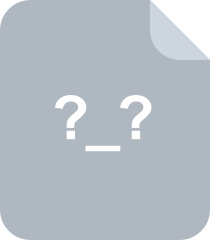
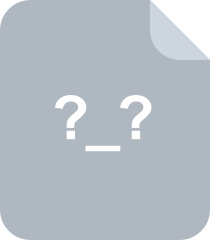
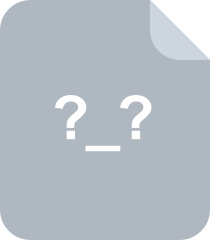
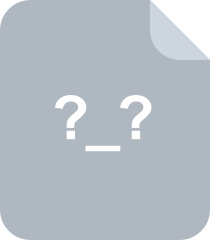
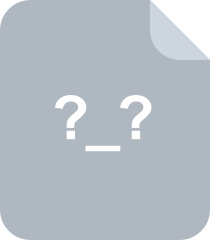
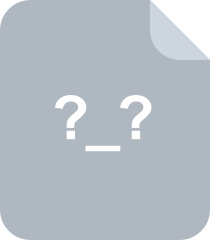
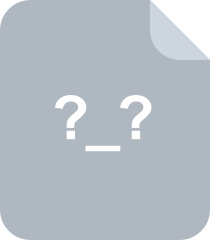
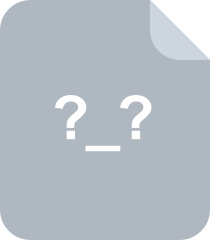
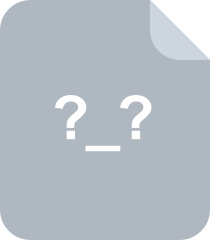
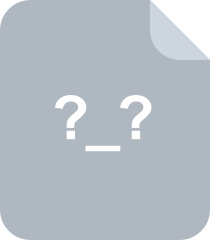
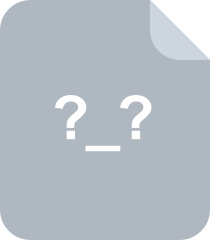
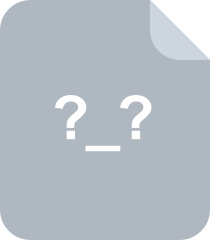
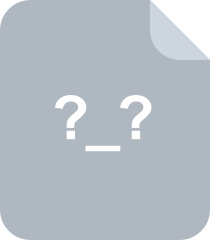
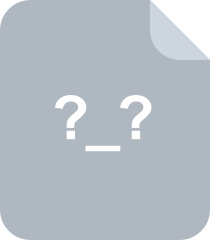
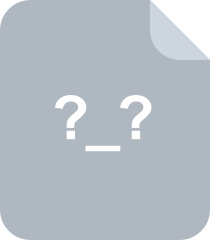
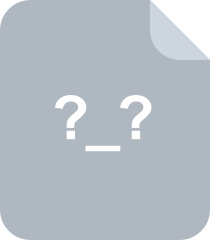
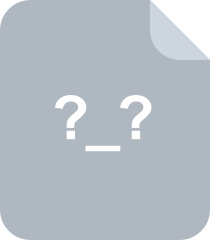
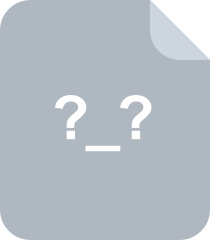
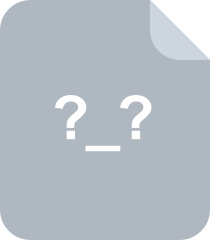
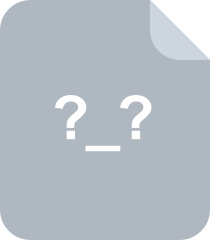
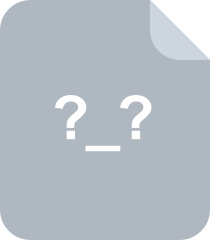
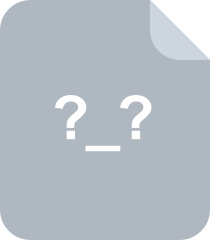
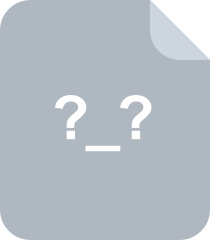
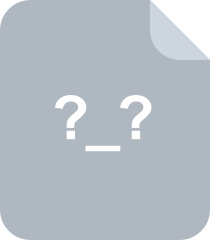
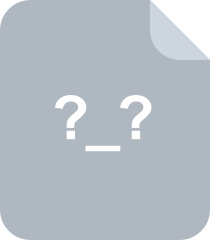
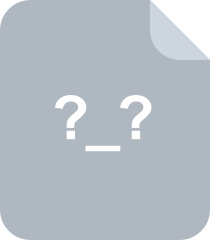
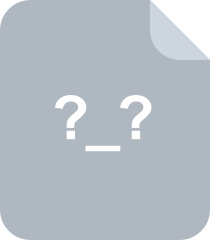
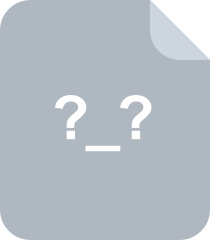
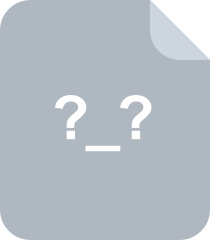
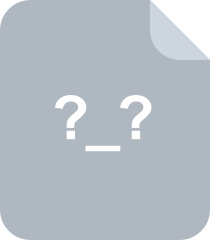
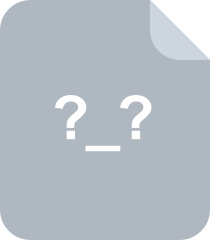
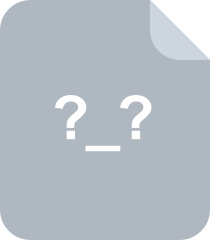
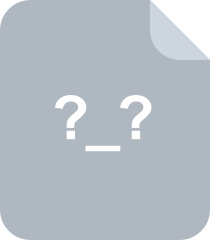
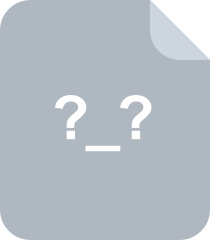
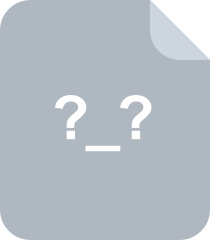
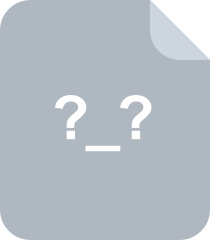
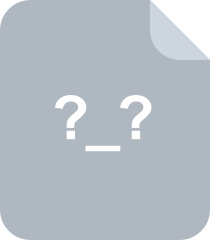
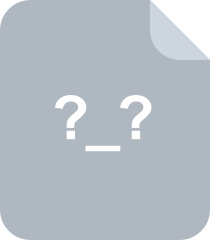
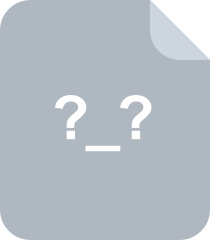
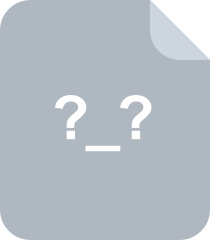
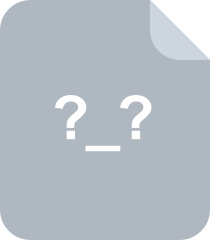
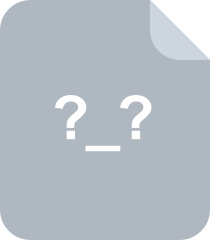
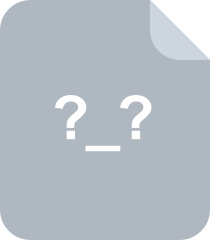
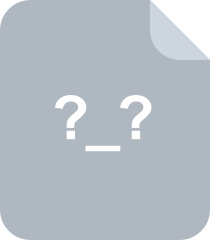
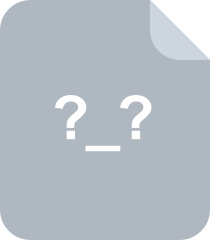
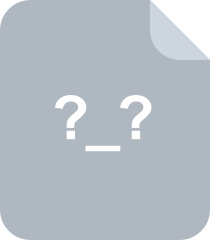
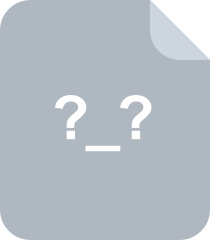
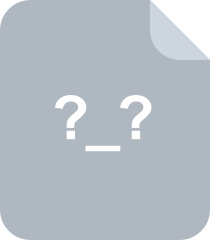
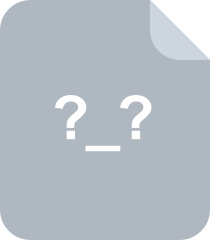
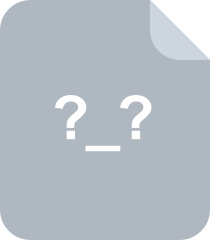
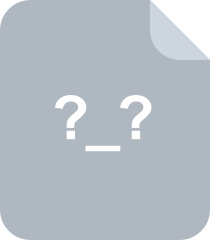
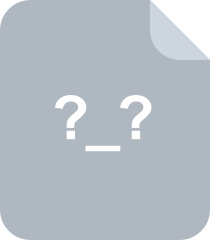
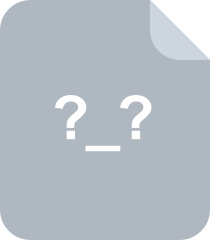
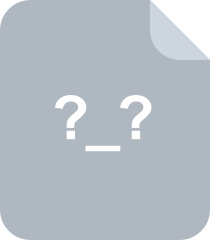
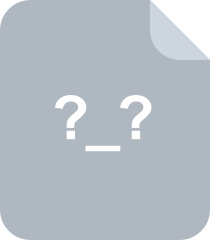
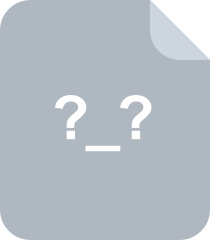
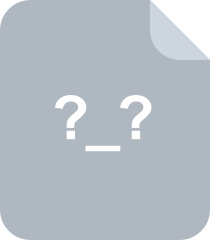
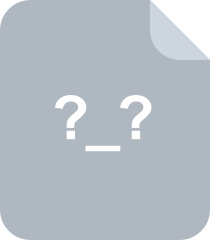
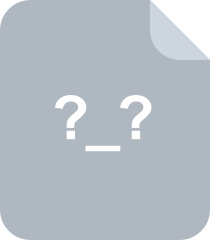
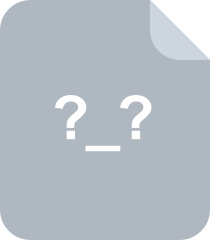
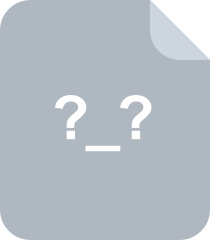
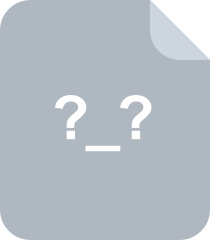
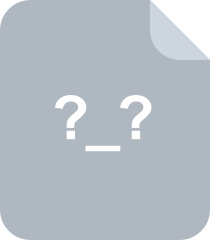
共 392 条
- 1
- 2
- 3
- 4

yannchao
- 粉丝: 0
- 资源: 6
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

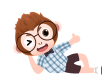
最新资源
- 遥感影像共享-JAVA-基于SpringBoot的遥感影像共享系统设计与实现
- 自习室系统-JAVA-基于springboot的自习室座位预约系统设计与实现
- 人工智能大作业-人脸识别
- 小区停车场-JAVA基于springBoot的小区停车场管理系统设计与实现
- STM32+ESP8266(ESP32)+MQTT+阿里云物联网平台
- 机械自动化与机器人控制中的速度与雅克比矩阵计算
- springboot社区医院信息平台(代码+数据库+LW)
- STM32+ESP8266(ESP32)+MQTT+阿里云物联网平台
- 宠物管理-JAVA-基于springBoot宠物管理系统设计与实现
- X230安装Sonoma成功 博通BCM94352HMB网卡 扩展坞引线改屏1080P
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


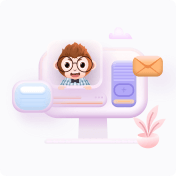
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页