/******************************************************************************
*
* package: Log4Qt
* file: patternformatter.cpp
* created: September 2007
* author: Martin Heinrich
*
*
* changes Feb 2009, Martin Heinrich
* - Fixed VS 2008 unreferenced formal parameter warning by using
* Q_UNUSED in LiteralPatternConverter::convert.
*
*
* Copyright 2007 - 2009 Martin Heinrich
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
******************************************************************************/
/******************************************************************************
* Dependencies
******************************************************************************/
#include "log4qt/helpers/patternformatter.h"
#include <QtCore/QString>
#include <QtCore/QDebug>
#include <limits.h>
#include "log4qt/helpers/datetime.h"
#include "log4qt/helpers/logerror.h"
#include "log4qt/layout.h"
#include "log4qt/logger.h"
#include "log4qt/loggingevent.h"
namespace Log4Qt
{
/**************************************************************************
*Declarations
**************************************************************************/
/*!
* \brief The class FormattingInfo stores the formatting modifier for a
* pattern converter.
*
* \sa PatternConverter
*/
class FormattingInfo
{
public:
FormattingInfo()
{ clear(); }
// FormattingInfo(const FormattingInfo &rOther); // Use compiler default
// virtual ~FormattingInfo(); // Use compiler default
// FormattingInfo &operator=(const FormattingInfo &rOther); // Use compiler default
void clear();
static QString intToString(int i);
public:
int mMinLength;
int mMaxLength;
bool mLeftAligned;
};
/*!
* \brief The class PatternConverter is the abstract base class for all
* pattern converters.
*
* PatternConverter handles the minimum and maximum modifier for a
* conversion character. The actual conversion is by calling the
* convert() member function of the derived class.
*
* \sa PatternLayout::format()
*/
class PatternConverter
{
public:
PatternConverter(const FormattingInfo &rFormattingInfo = FormattingInfo()) :
mFormattingInfo(rFormattingInfo)
{};
virtual ~PatternConverter()
{};
private:
PatternConverter(const PatternConverter &rOther); // Not implemented
PatternConverter &operator=(const PatternConverter &rOther); // Not implemented
public:
void format(QString &rFormat, const LoggingEvent &rLoggingEvent) const;
protected:
virtual QString convert(const LoggingEvent &rLoggingEvent) const = 0;
#ifndef QT_NO_DEBUG_STREAM
virtual QDebug debug(QDebug &rDebug) const = 0;
friend QDebug operator<<(QDebug, const PatternConverter &rPatternConverter);
#endif
protected:
FormattingInfo mFormattingInfo;
};
/*!
* \brief The class BasicPatternConverter converts several members of a
* LoggingEvent to a string.
*
* BasicPatternConverter is used by PatternLayout to convert members that
* do not reuquire additional formatting to a string as part of formatting
* the LoggingEvent. It handles the following conversion characters:
* 'm', 'p', 't', 'x'
*
* \sa PatternLayout::format()
* \sa PatternConverter::format()
*/
class BasicPatternConverter : public PatternConverter
{
public:
enum Type {
MESSAGE_CONVERTER,
NDC_CONVERTER,
LEVEL_CONVERTER,
THREAD_CONVERTER,
};
public:
BasicPatternConverter(const FormattingInfo &rFormattingInfo,
Type type) :
PatternConverter(rFormattingInfo),
mType(type)
{};
// virtual ~BasicPatternConverter(); // Use compiler default
private:
BasicPatternConverter(const BasicPatternConverter &rOther); // Not implemented
BasicPatternConverter &operator=(const BasicPatternConverter &rOther); // Not implemented
protected:
virtual QString convert(const LoggingEvent &rLoggingEvent) const;
#ifndef QT_NO_DEBUG_STREAM
virtual QDebug debug(QDebug &rDebug) const;
#endif
private:
Type mType;
};
/*!
* \brief The class DatePatternConverter converts the time stamp of a
* LoggingEvent to a string.
*
* DatePatternConverter is used by PatternLayout to convert the time stamp
* of a LoggingEvent to a string as part of formatting the LoggingEvent.
* It handles the 'd' and 'r' conversion character.
*
* \sa PatternLayout::format()
* \sa PatternConverter::format()
*/
class DatePatternConverter : public PatternConverter
{
public:
DatePatternConverter(const FormattingInfo &rFormattingInfo,
const QString &rFormat) :
PatternConverter(rFormattingInfo),
mFormat(rFormat)
{};
// virtual ~DatePatternConverter(); // Use compiler default
private:
DatePatternConverter(const DatePatternConverter &rOther); // Not implemented
DatePatternConverter &operator=(const DatePatternConverter &rOther); // Not implemented
protected:
virtual QString convert(const LoggingEvent &rLoggingEvent) const;
#ifndef QT_NO_DEBUG_STREAM
virtual QDebug debug(QDebug &rDebug) const;
#endif
private:
QString mFormat;
};
/*!
* \brief The class LiteralPatternConverter provides string literals.
*
* LiteralPatternConverter is used by PatternLayout to embed string
* literals as part of formatting the LoggingEvent. It handles string
* literals and the 'n' conversion character.
*
* \sa PatternLayout::format()
* \sa PatternConverter::format()
*/
class LiteralPatternConverter : public PatternConverter
{
public:
LiteralPatternConverter(const QString &rLiteral) :
PatternConverter(),
mLiteral(rLiteral)
{};
// virtual ~LiteralPatternConverter(); // Use compiler default
private:
LiteralPatternConverter(const LiteralPatternConverter &rOther); // Not implemented
LiteralPatternConverter &operator=(const LiteralPatternConverter &rOther); // Not implemented
protected:
virtual QString convert(const LoggingEvent &rLoggingEvent) const;
#ifndef QT_NO_DEBUG_STREAM
virtual QDebug debug(QDebug &rDebug) const;
#endif
private:
QString mLiteral;
};
/*!
* \brief The class LoggerPatternConverter converts the Logger name of a
* LoggingEvent to a string.
*
* LoggerPatternConverter is used by PatternLayout to convert the Logger
* name of a LoggingEvent to a string as part of formatting the
* LoggingEvent. It handles the 'c' conversion character.
*
* \sa PatternLayout::format()
* \sa PatternConverter::format()
*/
class LoggerPatternConverter : public PatternConverter
{
public:
LoggerPatternConverter(const FormattingInfo &rFormattingInfo,
int precision) :
PatternConverter(rFormattingInfo),
mPrecision(precision)
{};
// virtual ~LoggerPatternConverter(); // Use compiler default
private:
LoggerPatternConverter(const LoggerPatternConverter &rOther); // Not implemented
LoggerPatternConverter &operator=(const LoggerPatternConverter &rOther); // Not implemented
protected:
virtual QString convert(const LoggingEvent &rLoggingEvent) const;
#ifndef QT_NO_DE
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
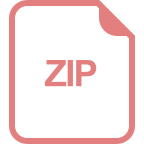
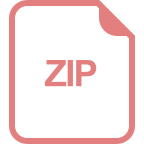
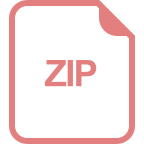
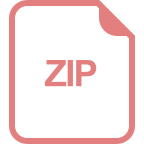
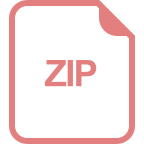
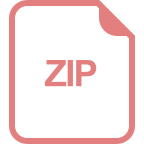
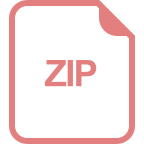
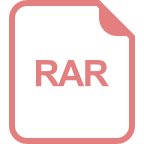
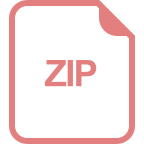
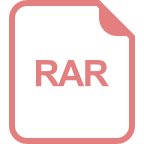
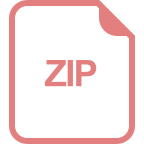
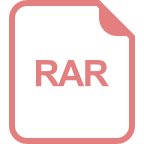
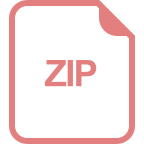
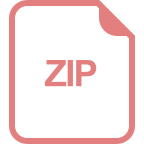
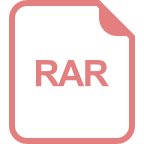
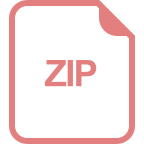
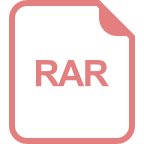
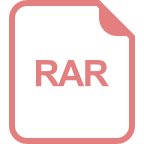
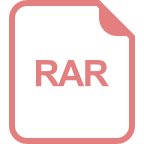
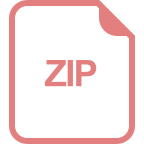
收起资源包目录


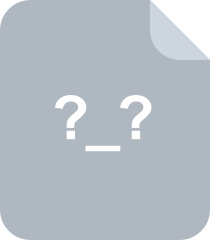
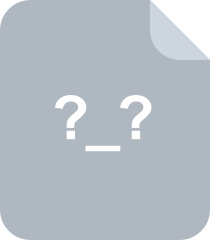
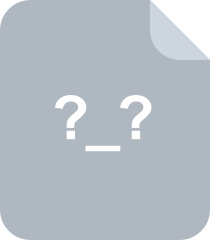
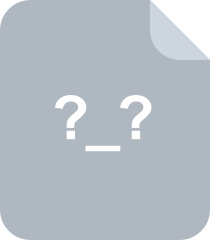

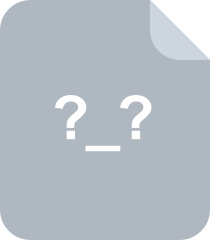
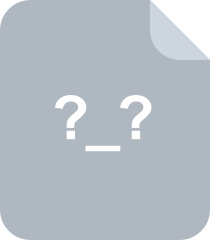
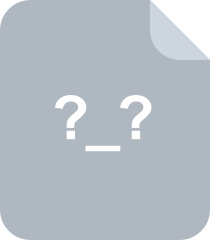
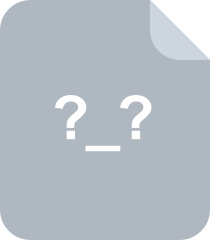
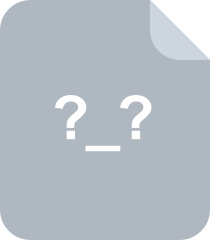
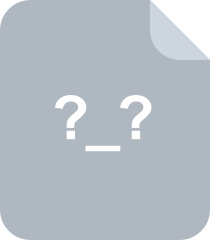
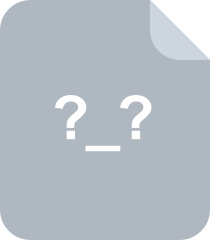
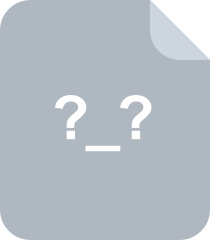
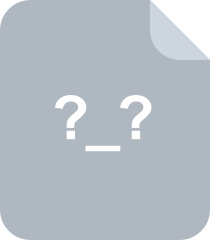
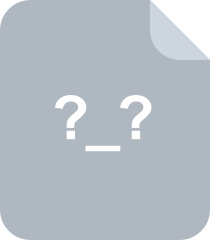
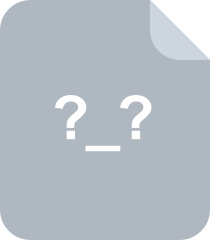
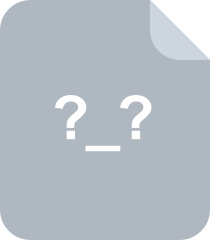
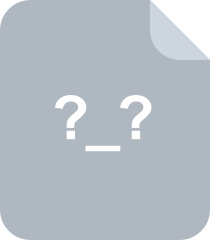
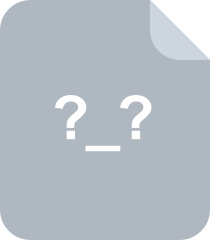
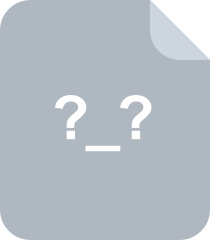
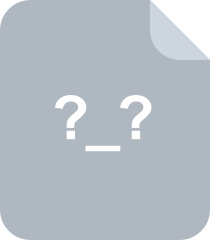
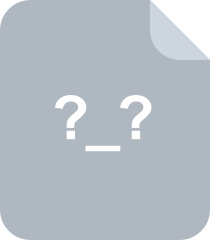
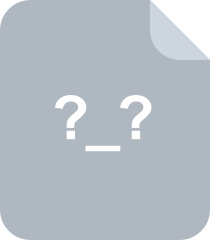
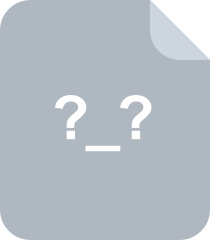
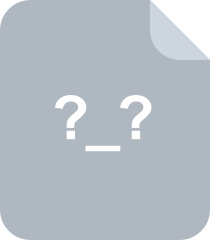
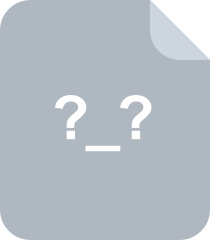
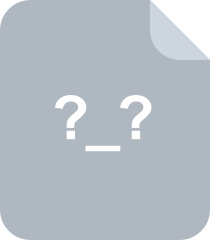
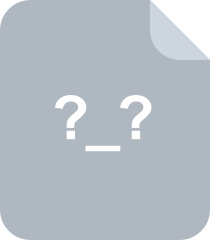
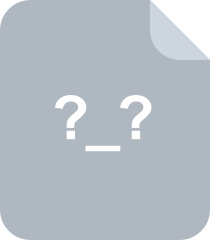
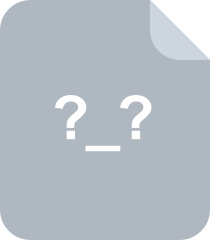
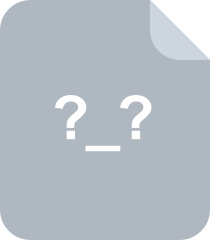
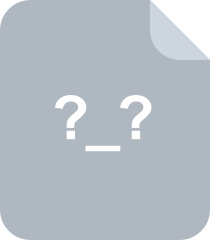
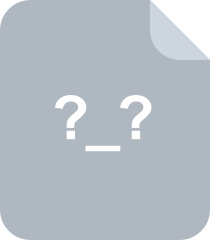
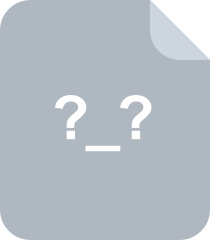
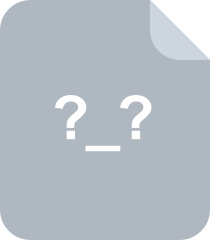

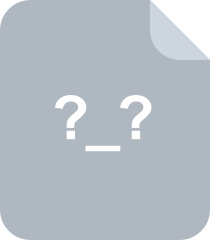
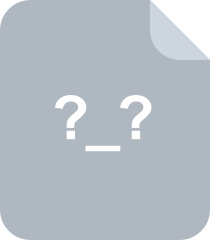
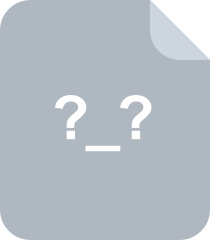
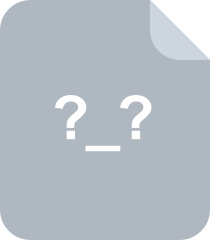
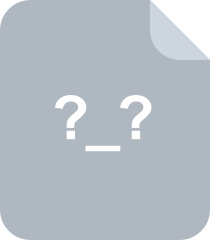
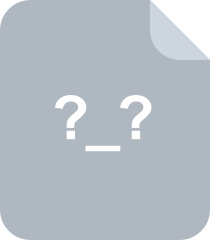
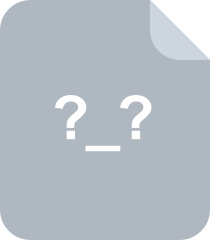
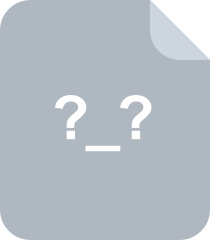
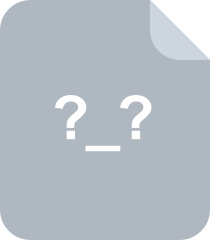
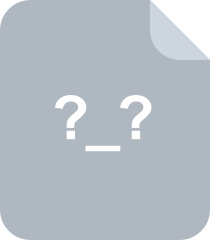
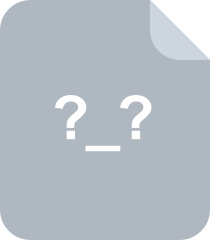
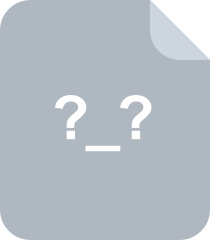
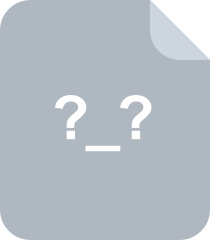
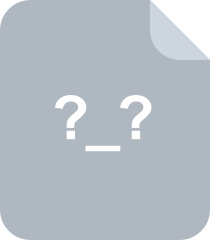

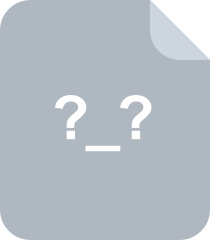
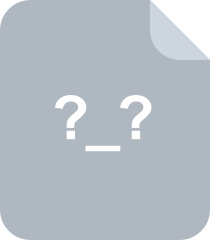
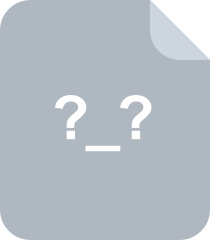
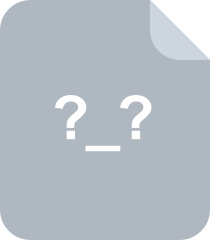
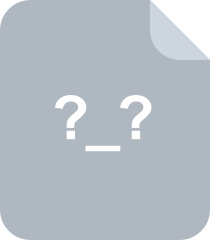
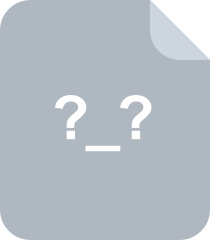
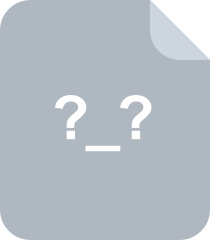
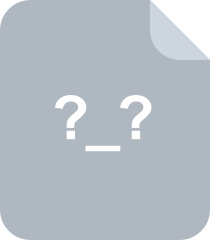
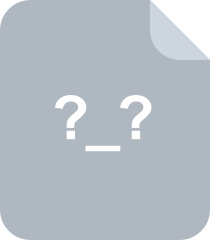
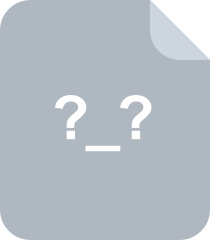
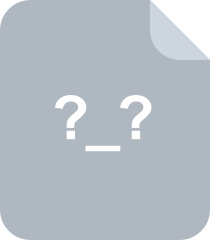
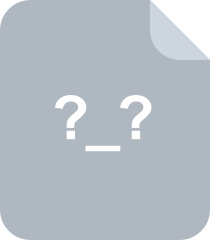
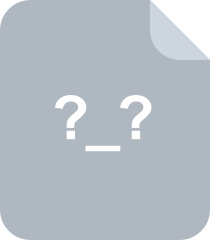
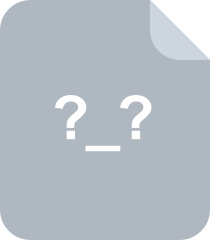

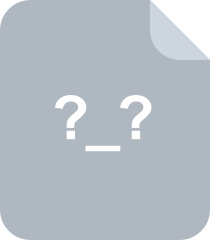
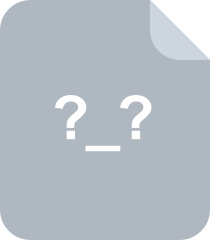
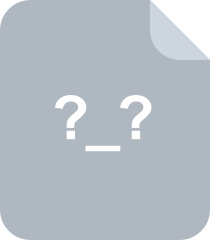
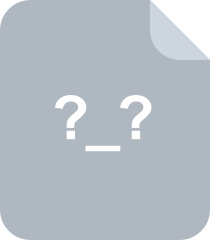
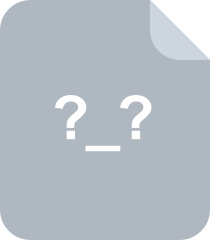
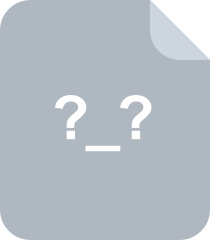
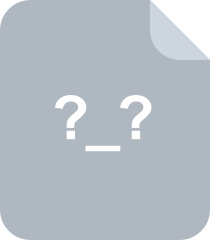
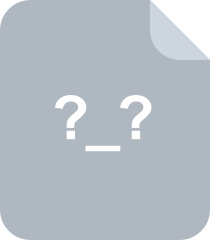
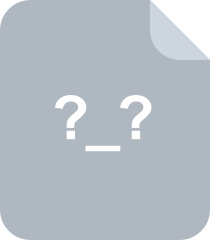
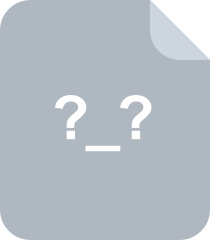
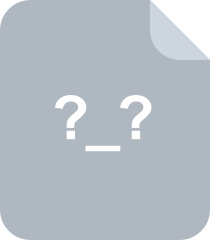
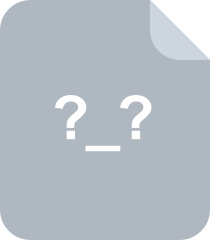
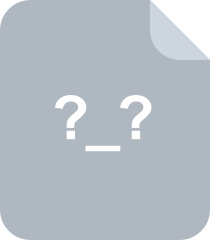
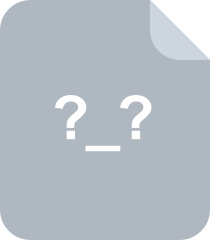
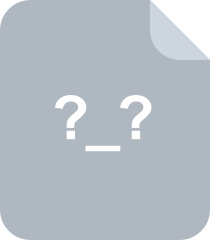
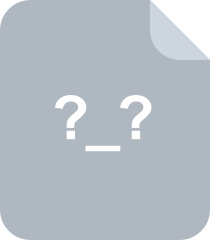
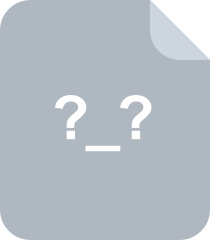
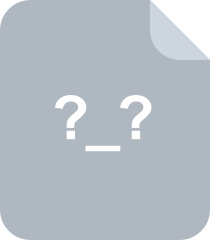
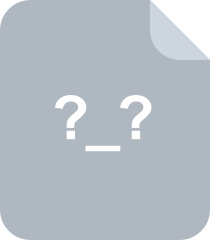
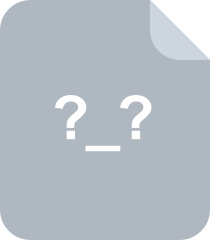
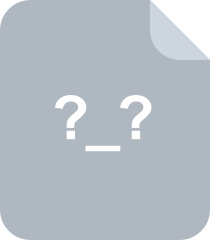
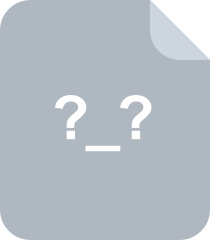
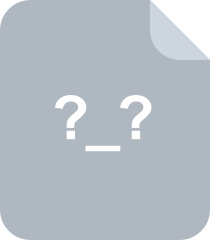
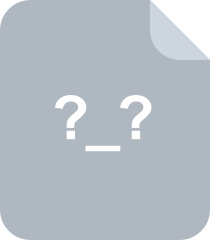
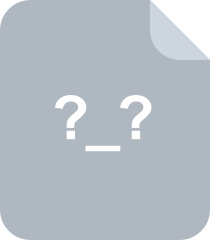
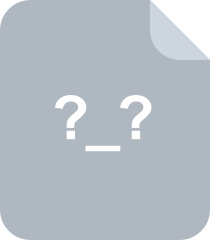
共 88 条
- 1
资源评论
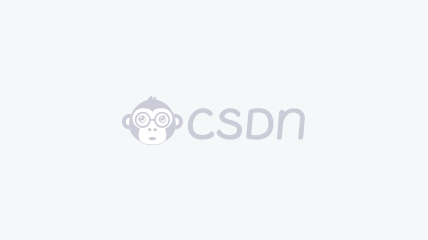


DreamLife.
- 粉丝: 3w+
- 资源: 75
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

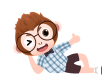
安全验证
文档复制为VIP权益,开通VIP直接复制
