SQL Server Uploader
=============================
This file contains some notes about how this plug-in to NeatUpload works. To understand this plug-in you probably should have tried NeatUpload first.
This plug-in can either generate SQL itself and use against the server or use stored procedures on the server for all tasks. An example of how those stored procedures
could look is supplied at the end of this document.
This plugin is written by Joakim Wennergren (jokedst@gmail.com). Feel free to contact me with any questions, or post them at the NeatUpload forum (www.bretle.com).
This plugin is release under the GNU Lesser General Public License. See the disclaimer in the source code files for more information.
===============
USAGE
===============
To specify whether this plug-in should generate SQL queries or use stored procedures, you configure the "provider" in web.config. If you specify procedure names
(createProcedure, openProcedure, readProcedure, writeProcedure, deleteProcedure, cleanupProcedure, renameProcedure, storeHashProcedure) the code uses the procedures.
If you instead specify table and column names (tableName, dataColumnName, partialFlagColumnName, fileNameColumnName, mimeTypeColumnName, hashColumnName) the code
will build its own SQL Queries. You can also combine both methods, if procedures are named they have precedence.
There is also the setting "hashAlgorithm". If specified, the uploader will calculate a hash value of the uploaded file while it is uploaded. The code is mainly
copied from Dean's HashingFilesystemUploadStorageProvider plug-in. The Hash algorithm may be any supported by the .NET framework, including the common MD5, SHA1 and SHA256.
Note that to specify connectionstring this plug-in supports using the ConectionStrings-section in web.config under .NET v2.0. To use a named connectionstring, enter the
name of the connection string instead of the string itself (see included web.config).
The examples in this project use a database table that looks like this:
CREATE TABLE [FileTable] (
[Id] [int] IDENTITY (1, 1) PRIMARY KEY NOT NULL ,
[FileName] [nvarchar] (50) NULL,
[DataField] [image] NOT NULL ,
[Partial] [tinyint] NOT NULL ,
[MimeType] [nvarchar] (50) NULL ,
[Created] [datetime] NOT NULL CONSTRAINT [DF_FileTable_Created] DEFAULT (getdate()),
[FileHash] [nvarchar] (50) NULL ,
)
The only necessary fields for the uploader to work are an IDENTITY field and a Image/varbinary field for the data, the others are optional.
Change the connection strings in the web.config to point to your database and the right table
Note that the version that uses generated SQL (as opposed to stored procedures) only works on SQL 2005 because it makes heavy use of the "$IDENTITY" object
to reference the IDENTITY column of the table. To fix this I should add a "IdentityColumnName"-setting, but since I only use SQL 2005 (and stored procedures)
it has never been an issue... Use stored procedures to get around this problem if you don't want to rewrite these classes and must use SQL Server 2000.
When using NeatUpload you get a bunch of warnings about the web.config file since the NeatUpload-addons to the system.web section isn't in Microsoft's .xsd-schema for
web.config. This is nothing to worry about, but if you find the warnings annoying, add this to C:\Program Files\Microsoft Visual Studio 8\Xml\Schemas\DotNetConfig.xsd
to get rid of them. It should be placed in the "choice" section under the element "configuration/system.web"
<xs:element name="neatUpload" vs:help="configuration/system.web/neatUpload">
<xs:complexType>
<xs:choice minOccurs="0" maxOccurs="1">
<xs:element name="providers" vs:help="configuration/system.web/neatUpload/providers">
<xs:complexType>
<xs:choice minOccurs="0" maxOccurs="unbounded">
<xs:element name="add" vs:help="configuration/system.web/httpHandlers/add">
<xs:complexType>
<xs:attribute name="name" type="xs:string" use="required" />
<xs:attribute name="type" type="xs:string" use="required" />
<xs:attribute name="tempDirectory" type="xs:string" use="optional" />
<!-- HashingFilesystemUploadStorageProvider specific -->
<xs:attribute name="algorithm" type="xs:string" use="optional" />
<!-- SqlServerUploader specific -->
<xs:attribute name="connectionString" type="xs:string" use="optional" />
<xs:attribute name="connectionName" type="xs:string" use="optional" />
<xs:attribute name="tableName" type="xs:string" use="optional" />
<xs:attribute name="dataColumnName" type="xs:string" use="optional" />
<xs:attribute name="partialFlagColumnName" type="xs:string" use="optional" />
<xs:attribute name="fileNameColumnName" type="xs:string" use="optional" />
<xs:attribute name="mimeTypeColumnName" type="xs:string" use="optional" />
<xs:attribute name="hashAlgorithm" type="xs:string" use="optional" />
<xs:attribute name="hashColumnName" type="xs:string" use="optional" />
<xs:attribute name="createProcedure" type="xs:string" use="optional" />
<xs:attribute name="openProcedure" type="xs:string" use="optional" />
<xs:attribute name="writeProcedure" type="xs:string" use="optional" />
<xs:attribute name="readProcedure" type="xs:string" use="optional" />
<xs:attribute name="cleanupProcedure" type="xs:string" use="optional" />
<xs:attribute name="renameProcedure" type="xs:string" use="optional" />
<xs:attribute name="storeHashProcedure" type="xs:string" use="optional" />
<xs:attribute name="deleteProcedure" type="xs:string" use="optional" />
</xs:complexType>
</xs:element>
</xs:choice>
</xs:complexType>
</xs:element>
</xs:choice>
<xs:attribute name="defaultProvider" type="xs:string" use="optional" />
<xs:attribute name="useHttpModule" type="small_boolean_Type" use="optional" />
<xs:attribute name="maxNormalRequestLength" type="xs:int" use="optional" />
<xs:attribute name="maxRequestLength" type="xs:int" use="optional" />
<xs:attribute name="postBackIDQueryParam" type="xs:string" use="optional" />
</xs:complexType>
</xs:element>
=====================================================
Stored Procedures example
SQL Server 2005:
=====================================================
CREATE TABLE [FileTable] (
[Id] [int] IDENTITY (1, 1) PRIMARY KEY NOT NULL ,
[FileName] [nvarchar] (50) NOT NULL ,
[DataField] [image] NOT NULL ,
[Partial] [tinyint] NOT NULL ,
[MimeType] [nvarchar] (50) NOT NULL ,
[Created] [datetime] NOT NULL CONSTRAINT [DF_FileTable_Created] DEFAULT (getdate()),
[FileHash] [nvarchar] (50) NULL ,
)
GO
Alter Procedure CreateBlob
@Identity Numeric Output,
@Pointer Binary(16) Output,
@FileName VarChar(250) = null,
@MIMEType VarChar(250) = null
As Begin Set NoCount ON;
Insert Into VerboseTable2 (Datafield,FileName,MimeType,PartiallyUploaded) Values ('',@FileName,@MimeType,1)
Select @Identity = SCOPE_IDENTITY()
Select @Pointer = TEXTPTR(DataField) From VerboseTable2 Where $IDENTITY = @Identity
End
Go
Alter Procedure OpenBlob
@Identity Numeric,
@Pointer VarBinary(max) Output,
@Size Int Output,
@FileName VarChar(250) Output,
@MIMEType VarChar(250) Output
As Begin Set NoCount On
Select @Pointer = TEXTPTR(DataField),
@Size = DATALENGTH(DataField),
@FileName = [FileName],
@MIMEType = MIMEType
From VerboseTable2 Where $IDENTITY = @Identity
End
Go
Alter Procedure ReadBlob
@Identity Numeric, --ignored in this implementation, here for reference
@Pointer Binary(16),
@Offset Int,
@Size Int
As Begin Set NoCount On
ReadText VerboseTable2.DataField @Pointer @Offset @Size
End
Go
Alter Procedure WriteBlob
@Identity Numeric, --ignored in this
没有合适的资源?快使用搜索试试~ 我知道了~
Asp.Net开发的某政府门户网源码
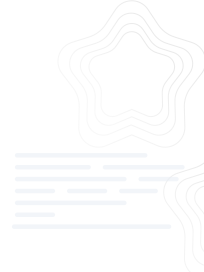
共1463个文件
html:341个
gif:297个
js:204个

需积分: 5 0 下载量 162 浏览量
2022-05-27
16:25:15
上传
评论
收藏 5.21MB RAR 举报
温馨提示
源码描述: 前台功能:网站主页 企业概况 党的建设 中心简介 企业动态 科技简讯 政策法规 项目申报 联系我们等 后台管理:后台管理采用全动态菜单,可根据自已需求自行拟定 后台主要功能包括:顶部图片管理,菜单列表管理,专家库管理,项目库管理,中心公告管理,图片信息管理,首页管理,友情链接管理,系统备份等 注意: 开发环境为Visual Studio 2013,数据库为SQL SERVER2008R2
资源详情
资源评论
资源推荐
收起资源包目录

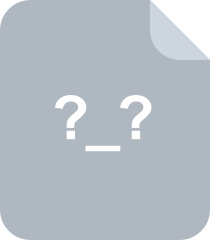
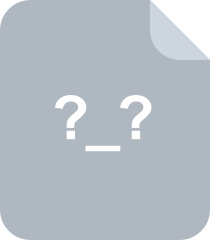
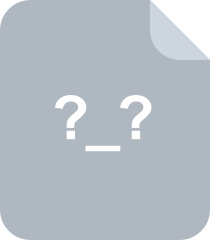
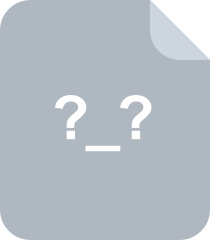
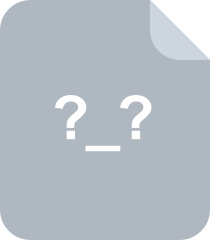
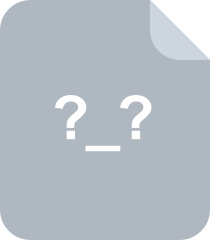
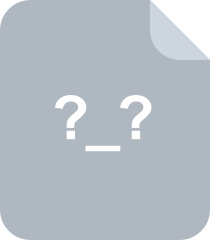
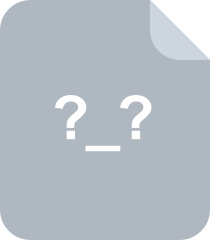
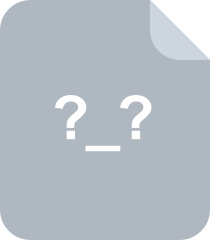
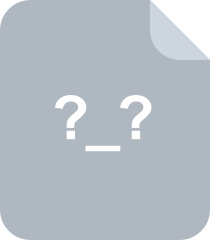
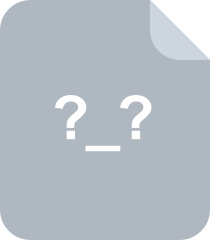
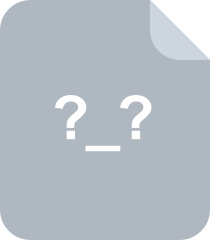
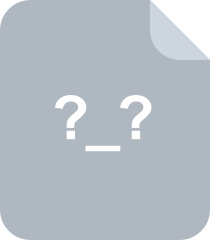
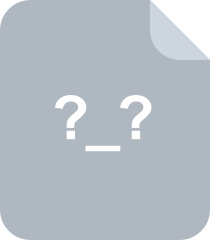
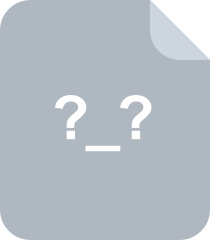
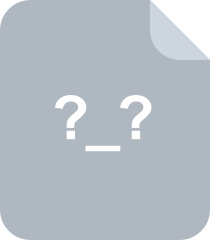
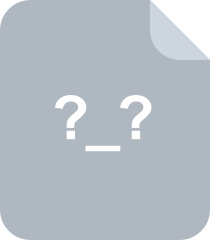
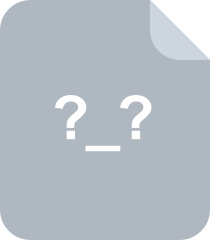
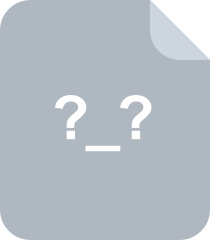
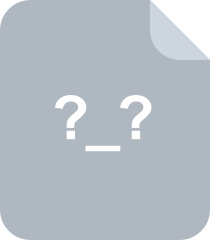
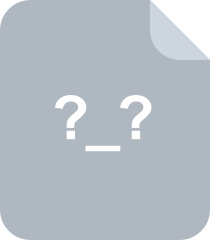
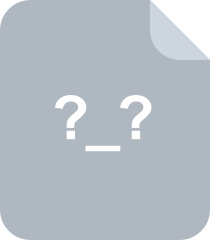
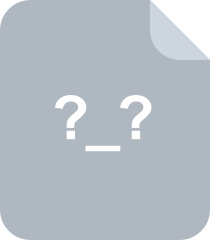
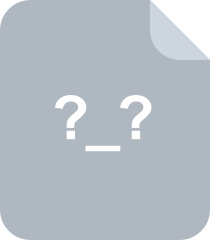
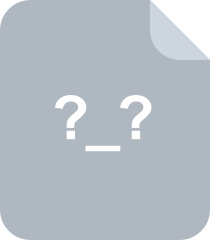
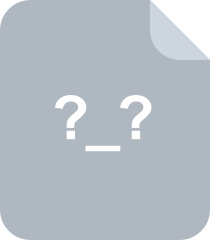
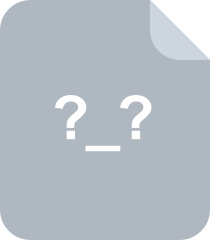
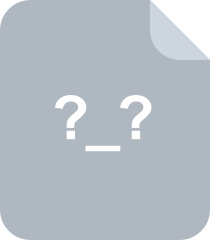
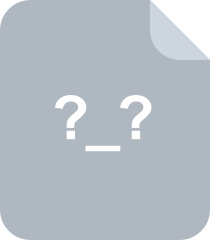
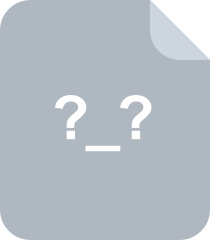
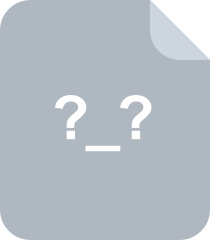
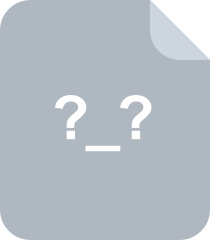
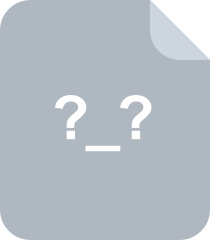
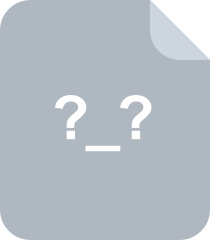
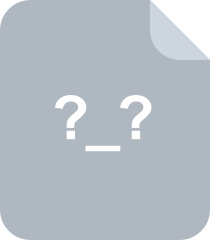
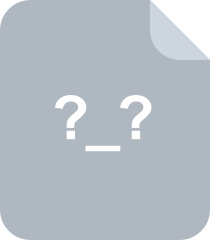
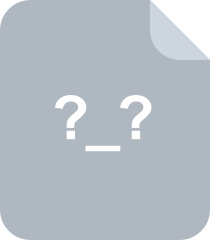
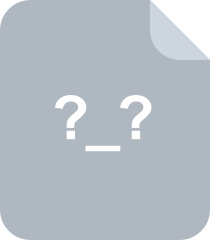
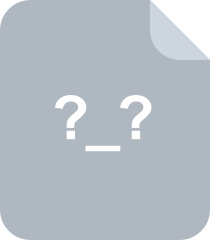
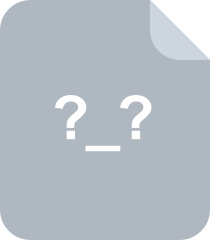
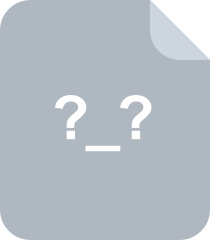
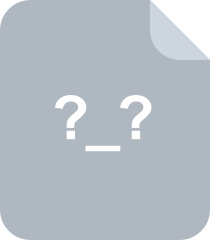
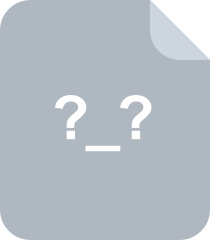
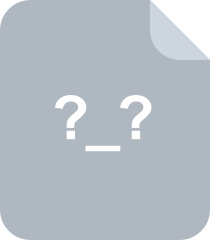
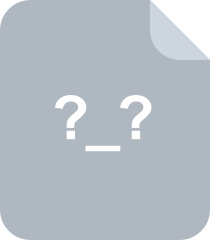
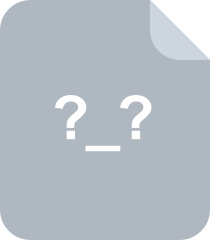
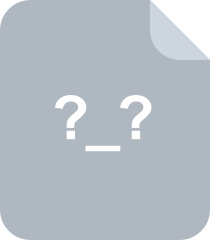
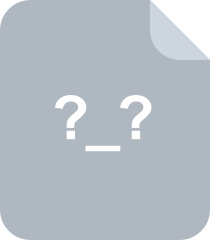
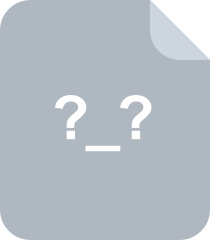
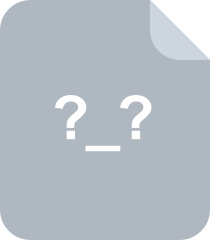
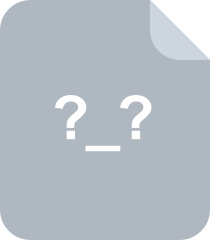
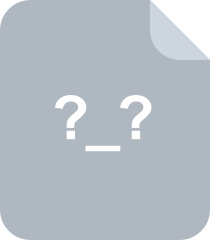
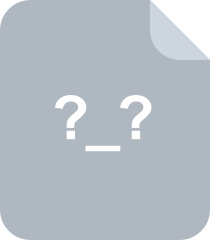
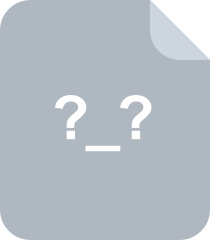
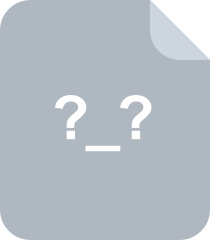
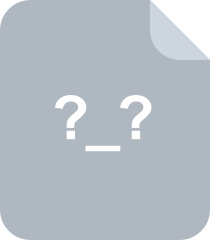
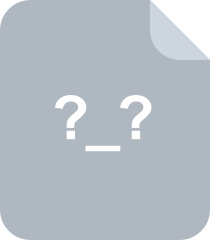
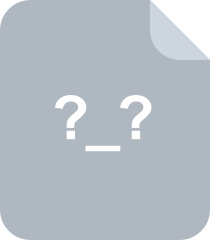
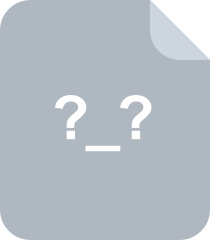
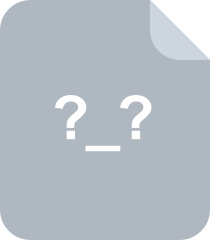
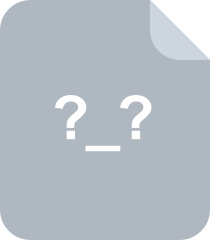
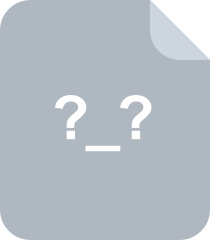
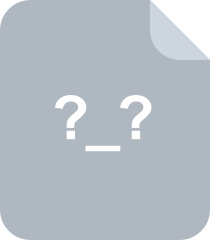
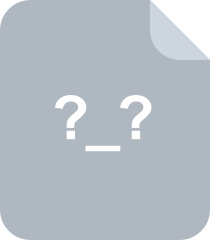
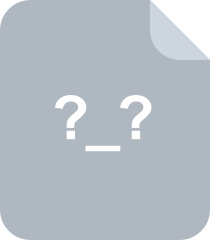
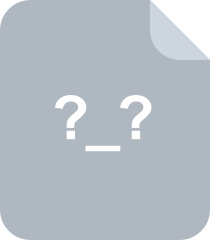
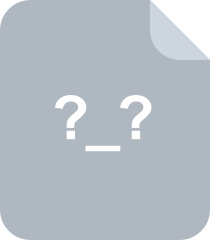
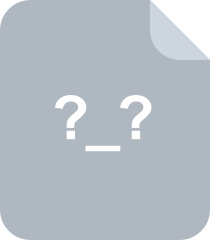
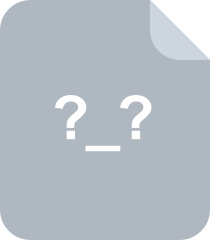
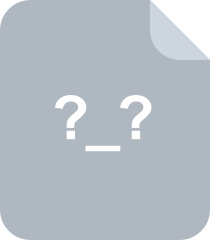
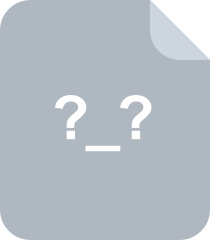
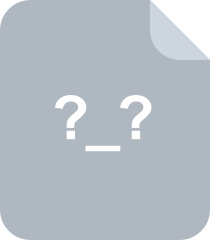
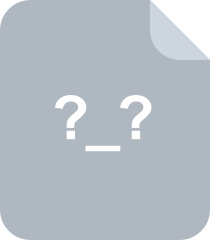
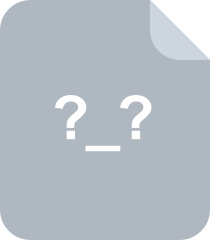
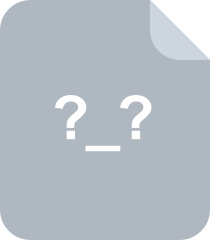
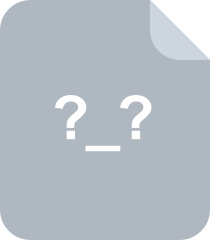
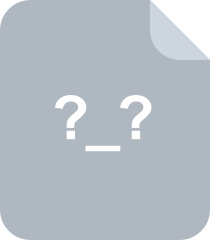
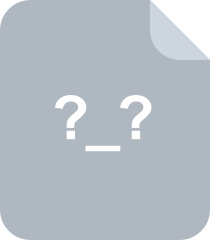
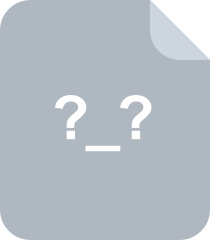
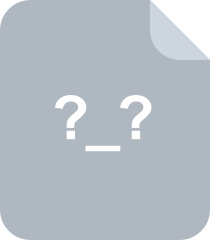
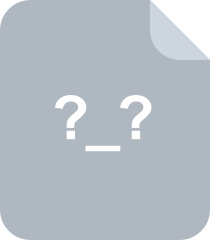
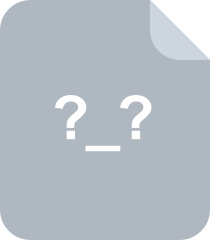
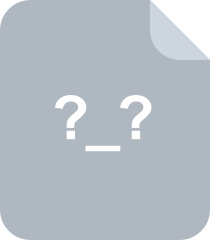
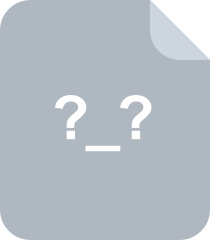
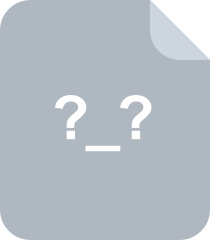
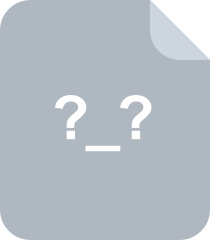
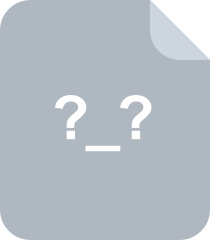
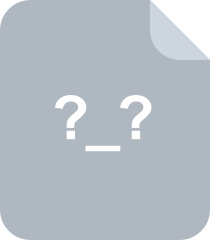
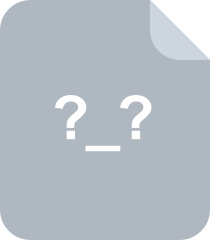
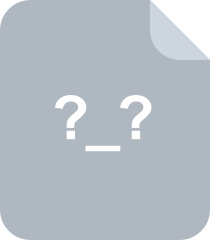
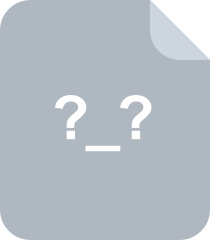
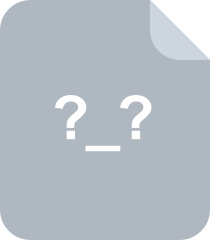
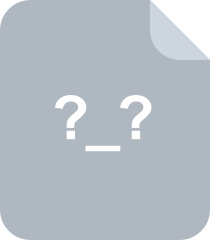
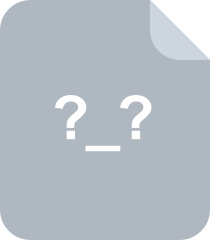
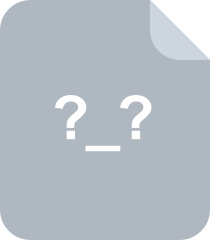
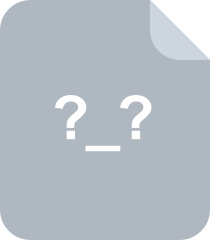
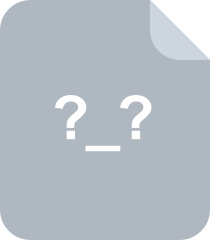
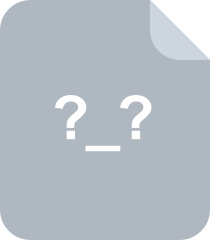
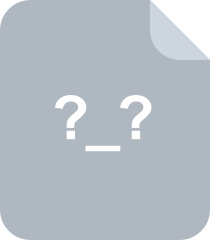
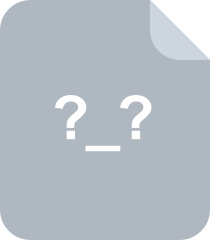
共 1463 条
- 1
- 2
- 3
- 4
- 5
- 6
- 15
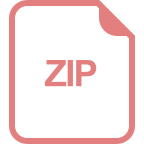
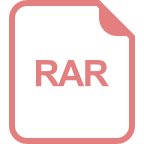
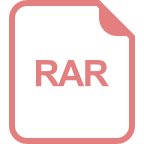
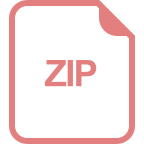
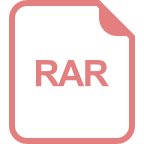
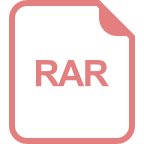
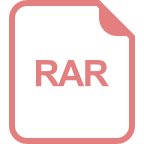
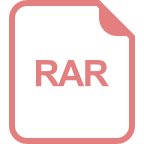
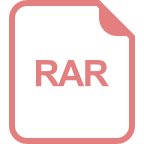
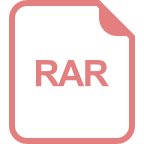
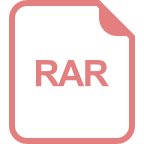
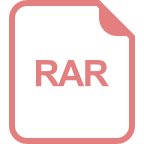
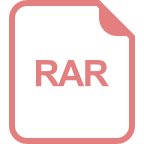
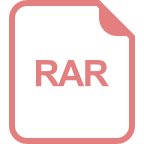
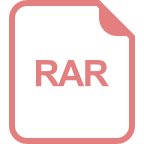
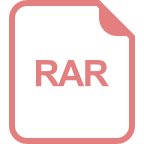
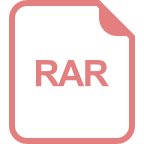
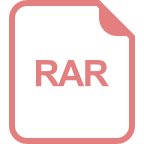
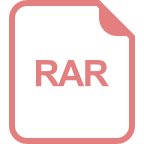
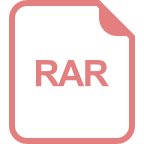

z0d1a3soft
- 粉丝: 0
- 资源: 46
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

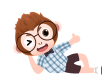
最新资源
- 基于Java语言的尚庭公寓2设计源码学习与实践
- 基于C语言为主的opensbi开源设计源码分析与优化
- JavaScript常用函数库,提升前端开发效率.zip
- Brunch前端框架(一键部署到云开发平台).zip
- 基于Java项目的常用有价值设计源码模板
- 基于Spring Boot 2.x的Elasticsearch High Level REST Client API设计源码大全
- 基于Python的链家、京东、淘宝、携程爬虫与数据可视化学习源码
- 基于OAuth2原理的Java QQ、微信、微博第三方登录封装与实现设计源码
- 基于Vue框架的租车管理系统设计源码
- fe-start-kit使用的模板,前端各种框架的快速开发模板.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


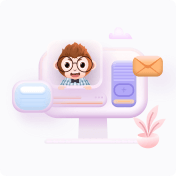
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0