在编程领域,特别是C语言中,数据结构是一个重要的概念,它允许我们有效地组织和操作数据。本主题将探讨如何利用栈这种数据结构来检测一个字符串是否为回文,即正读反读都相同的字符串。在描述中提到的例子"abc&cba@"就是一个回文串,其中"&"作为间隔符,"@"作为结束符。 栈是一种后进先出(LIFO,Last In First Out)的数据结构,类似于现实生活中的堆叠物品。在栈中,最后入栈的元素会最先出栈,这对于处理诸如括号匹配、表达式求值等问题非常有效。检测回文串时,我们可以利用栈的这一特性,逐个检查字符串中的字符。 我们需要创建一个空栈,然后遍历输入的字符串。对于每个字符,我们有以下步骤: 1. 如果遇到非间隔符字符,将其压入栈中。 2. 如果遇到结束符,或者当前字符是间隔符,跳过不处理。 3. 当遍历到字符串末尾时,如果栈不为空,我们需要进行回文验证。此时,栈顶的字符可能是未处理的非间隔符。逐个弹出栈顶字符并与当前遍历到的字符比较: - 如果它们相同,继续弹出下一个栈顶字符并比较,直到栈为空或发现不匹配的字符。 - 如果在弹出过程中发现不匹配的字符,则该字符串不是回文。 在实现这个算法时,可以使用数组或链表来模拟栈。数组作为栈的实现,通常限制了栈的大小,而链表则允许动态增长。在C语言中,我们可以使用`malloc`和`free`来动态分配和释放内存,以适应不同长度的字符串。 在提供的`回文字.cpp`源代码文件中,很可能包含了实现这个算法的具体细节。代码通常会包含以下部分: 1. 定义栈的数据结构,可能是一个结构体,包含一个字符数组和一个指示栈顶位置的指针。 2. 初始化栈的函数,通常用于设置栈顶指针为初始值(例如,指向数组的第一个元素)。 3. 压栈和弹栈的函数,分别用于将字符放入栈和从栈中取出字符。 4. 主函数,负责读取字符串,处理每个字符,并调用栈操作函数进行回文判断。 通过阅读和理解`回文字.cpp`的源代码,你可以深入学习如何在C语言中使用数据结构解决实际问题。同时,这也是一个很好的练习,可以帮助你提高对C语言栈操作的理解,以及对字符串处理和条件判断的技巧。
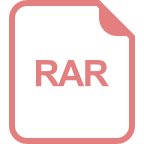
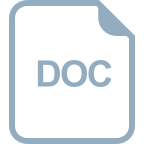
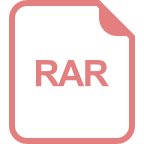
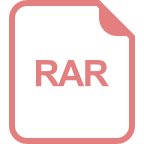
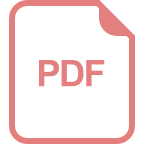
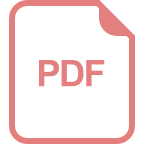
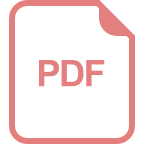
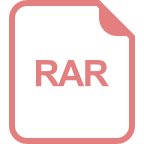
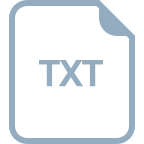
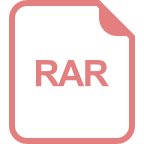
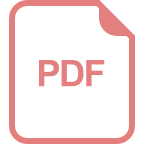
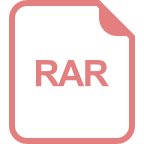
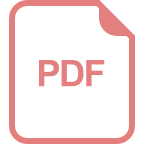
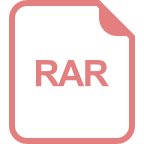
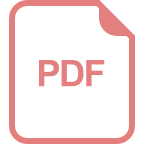
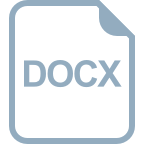

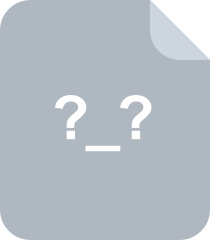
- 1
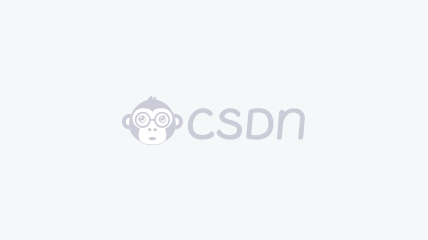
- 玩酷酷2012-12-27还好吧!不过有点小毛病。。

- 粉丝: 2
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

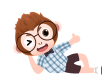
最新资源

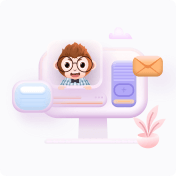
