### C#窗体动态生成小方格 #### 知识点概述 本文将详细介绍如何使用C#在Windows Forms应用程序中动态生成小方格,并通过鼠标点击事件改变这些方格的状态。这种方法利用了重载的方式获取窗体上的坐标,并在相应坐标处绘制方格。 #### 代码解析与知识点详解 ##### 1. 基本类结构定义 在给定的代码中,首先定义了一个`Form1`类,该类继承自`Form`,这是创建Windows Forms应用程序的基础。`Form1`类包含了所有必要的成员变量和方法来实现动态生成方格的功能。 ```csharp public partial class Form1 : Form { int m_GridX, m_GridY; bool[,] cells; public Form1() : this(18, 18) { InitializeComponent(); } public Form1(int gridX, int gridY) { m_GridX = gridX; m_GridY = gridY; cells = new bool[gridY, gridX]; } } ``` 这里的关键点包括: - **成员变量**:`m_GridX` 和 `m_GridY` 定义了网格的大小。 - **二维数组**:`cells` 是一个布尔类型的二维数组,用来存储每个方格的状态(是否被选中)。 - **构造函数**:提供了两个构造函数,一个默认构造函数初始化为18x18的网格,另一个允许用户自定义网格大小。 ##### 2. 绘制方格 在`OnPaint`方法中实现了方格的绘制逻辑。这里使用了双缓冲技术来提高绘图性能,避免闪烁现象。 ```csharp protected override void OnPaint(PaintEventArgs e) { float cellWidth = (float)(ClientRectangle.Width - 1) / m_GridX; float cellHeight = (float)(ClientRectangle.Height - 1) / m_GridY; Bitmap memoryBmp = new Bitmap(ClientRectangle.Width, ClientRectangle.Height); using (Graphics g = Graphics.FromImage(memoryBmp)) { // 清除背景 g.Clear(SystemColors.Control); // 绘制网格线 for (int y = 0; y <= m_GridY; y++) { for (int x = 0; x <= m_GridX; x++) { g.DrawLine(Pens.LightBlue, 0, y * cellHeight, ClientRectangle.Width, y * cellHeight); g.DrawLine(Pens.LightBlue, x * cellWidth, 0, x * cellWidth, ClientRectangle.Height); } } // 绘制已选择的方格 for (int y = 0; y < cells.GetLength(0); y++) { for (int x = 0; x < cells.GetLength(1); x++) { if (cells[y, x]) { g.FillRectangle(Brushes.LightBlue, x * cellWidth, y * cellHeight, cellWidth, cellHeight); } } } } // 将内存中的图像绘制到窗体上 e.Graphics.DrawImageUnscaled(memoryBmp, 0, 0); memoryBmp.Dispose(); } ``` 关键点包括: - **计算单元格大小**:根据窗体的宽度和高度计算每个单元格的宽度和高度。 - **绘制网格线**:使用`DrawLine`方法绘制网格线。 - **填充已选中方格**:如果`cells`数组中对应的元素为`true`,则使用`FillRectangle`方法填充颜色。 ##### 3. 鼠标点击事件处理 当用户点击窗体时,会触发`OnMouseClick`事件处理程序。此方法根据点击的位置确定所选中的方格,并切换其状态。 ```csharp protected override void OnMouseClick(MouseEventArgs e) { float cellWidth = (float)(ClientRectangle.Width - 1) / m_GridX; float cellHeight = (float)(ClientRectangle.Height - 1) / m_GridY; int x = (int)(e.X / cellWidth); int y = (int)(e.Y / cellHeight); cells[y, x] = !cells[y, x]; Invalidate(); } ``` 关键点包括: - **确定点击位置**:根据鼠标点击的位置计算出所在的方格坐标。 - **切换方格状态**:翻转对应位置的`cells`数组值。 - **刷新界面**:调用`Invalidate()`方法重新绘制窗体,以显示更新后的状态。 #### 总结 本文详细介绍了如何使用C#在Windows Forms应用程序中动态生成小方格并响应鼠标点击事件。通过上述代码和解释,你可以了解到如何实现这样的功能,包括如何设置窗体的基本属性、如何绘制图形以及如何处理用户输入。这对于学习Windows Forms编程非常有帮助。
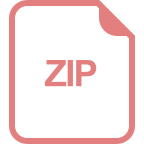
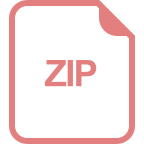
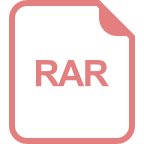
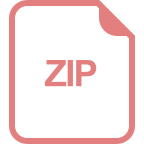
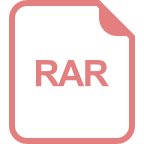
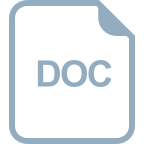
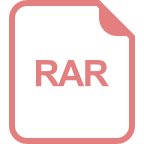
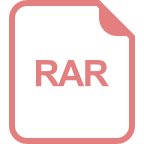
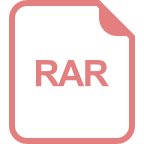
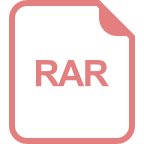
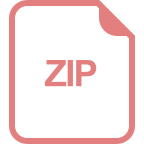
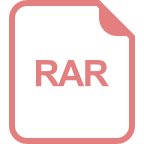
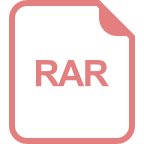
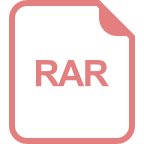
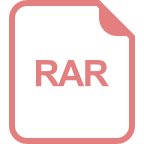
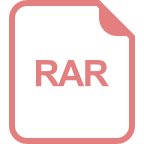
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace SweepDemo
{
public partial class Form1 :Form
{
int m_GridX, m_GridY;
bool[,] cells;
public Form1() : this(18,18)
{
InitializeComponent();
}
public Form1(int gridX, int gridY)
{
m_GridX = gridX;
m_GridY = gridY;
cells = new bool[ gridY, gridX ];
}
protected override void OnPaintBackground(PaintEventArgs e)
{
}
protected override void OnPaint(PaintEventArgs e)
{
float cellWidth = (float)(ClientRectangle.Width-1) / m_GridX;
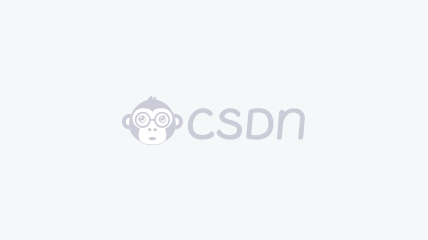

- 粉丝: 1
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

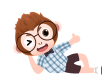
最新资源

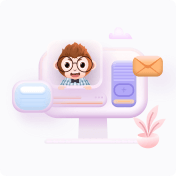
