<?xml version="1.0" encoding="utf-8" standalone="yes"?>
<doc>
<members>
<assembly>
<name>UnityEngine</name>
</assembly>
<member name="T:UnityEngine.AccelerationEvent">
<summary>
<para>Structure describing acceleration status of the device.</para>
</summary>
</member>
<member name="P:UnityEngine.AccelerationEvent.acceleration">
<summary>
<para>Value of acceleration.</para>
</summary>
</member>
<member name="P:UnityEngine.AccelerationEvent.deltaTime">
<summary>
<para>Amount of time passed since last accelerometer measurement.</para>
</summary>
</member>
<member name="T:UnityEngine.AddComponentMenu">
<summary>
<para>The AddComponentMenu attribute allows you to place a script anywhere in the "Component" menu, instead of just the "Component->Scripts" menu.</para>
</summary>
</member>
<member name="P:UnityEngine.AddComponentMenu.componentOrder">
<summary>
<para>The order of the component in the component menu (lower is higher to the top).</para>
</summary>
</member>
<member name="M:UnityEngine.AddComponentMenu.#ctor(System.String)">
<summary>
<para>Add an item in the Component menu.</para>
</summary>
<param name="menuName">The path to the component.</param>
<param name="order">Where in the component menu to add the new item.</param>
</member>
<member name="M:UnityEngine.AddComponentMenu.#ctor(System.String,System.Int32)">
<summary>
<para>Add an item in the Component menu.</para>
</summary>
<param name="menuName">The path to the component.</param>
<param name="order">Where in the component menu to add the new item.</param>
</member>
<member name="T:UnityEngine.AnchoredJoint2D">
<summary>
<para>Parent class for all joints that have anchor points.</para>
</summary>
</member>
<member name="P:UnityEngine.AnchoredJoint2D.anchor">
<summary>
<para>The joint's anchor point on the object that has the joint component.</para>
</summary>
</member>
<member name="P:UnityEngine.AnchoredJoint2D.connectedAnchor">
<summary>
<para>The joint's anchor point on the second object (ie, the one which doesn't have the joint component).</para>
</summary>
</member>
<member name="T:UnityEngine.Animation">
<summary>
<para>The animation component is used to play back animations.</para>
</summary>
</member>
<member name="P:UnityEngine.Animation.animateOnlyIfVisible">
<summary>
<para>When turned on, Unity might stop animating if it thinks that the results of the animation won't be visible to the user.</para>
</summary>
</member>
<member name="P:UnityEngine.Animation.animatePhysics">
<summary>
<para>When turned on, animations will be executed in the physics loop. This is only useful in conjunction with kinematic rigidbodies.</para>
</summary>
</member>
<member name="P:UnityEngine.Animation.clip">
<summary>
<para>The default animation.</para>
</summary>
</member>
<member name="P:UnityEngine.Animation.cullingType">
<summary>
<para>Controls culling of this Animation component.</para>
</summary>
</member>
<member name="P:UnityEngine.Animation.isPlaying">
<summary>
<para>Are we playing any animations?</para>
</summary>
</member>
<member name="P:UnityEngine.Animation.localBounds">
<summary>
<para>AABB of this Animation animation component in local space.</para>
</summary>
</member>
<member name="P:UnityEngine.Animation.playAutomatically">
<summary>
<para>Should the default animation clip (the [[Animation.clip]] property) automatically start playing on startup?</para>
</summary>
</member>
<member name="P:UnityEngine.Animation.wrapMode">
<summary>
<para>How should time beyond the playback range of the clip be treated?</para>
</summary>
</member>
<member name="M:UnityEngine.Animation.AddClip(UnityEngine.AnimationClip,System.String)">
<summary>
<para>Adds a /clip/ to the animation with name /newName/.</para>
</summary>
<param name="clip"></param>
<param name="newName"></param>
</member>
<member name="M:UnityEngine.Animation.AddClip">
<summary>
<para>Adds /clip/ to the only play between /firstFrame/ and /lastFrame/. The new clip will also be added to the animation with name /newName/.</para>
</summary>
<param name="addLoopFrame">Should an extra frame be inserted at the end that matches the first frame? Turn this on if you are making a looping animation.</param>
<param name="clip"></param>
<param name="newName"></param>
<param name="firstFrame"></param>
<param name="lastFrame"></param>
</member>
<member name="M:UnityEngine.Animation.AddClip(UnityEngine.AnimationClip,System.String,System.Int32,System.Int32,System.Boolean)">
<summary>
<para>Adds /clip/ to the only play between /firstFrame/ and /lastFrame/. The new clip will also be added to the animation with name /newName/.</para>
</summary>
<param name="addLoopFrame">Should an extra frame be inserted at the end that matches the first frame? Turn this on if you are making a looping animation.</param>
<param name="clip"></param>
<param name="newName"></param>
<param name="firstFrame"></param>
<param name="lastFrame"></param>
</member>
<member name="M:UnityEngine.Animation.Blend">
<summary>
<para>Blends the animation named /animation/ towards /targetWeight/ over the next /time/ seconds.</para>
</summary>
<param name="animation"></param>
<param name="targetWeight"></param>
<param name="fadeLength"></param>
</member>
<member name="M:UnityEngine.Animation.Blend">
<summary>
<para>Blends the animation named /animation/ towards /targetWeight/ over the next /time/ seconds.</para>
</summary>
<param name="animation"></param>
<param name="targetWeight"></param>
<param name="fadeLength"></param>
</member>
<member name="M:UnityEngine.Animation.Blend(System.String,System.Single,System.Single)">
<summary>
<para>Blends the animation named /animation/ towards /targetWeight/ over the next /time/ seconds.</para>
</summary>
<param name="animation"></param>
<param name="targetWeight"></param>
<param name="fadeLength"></param>
</member>
<member name="M:UnityEngine.Animation.CrossFade">
<summary>
<para>Fades the animation with name /animation/ in over a period of /time/ seconds and fades other animations out.</para>
</summary>
<param name="animation"></param>
<param name="fadeLength"></param>
<param name="mode"></param>
</member>
<member name="M:UnityEngine.Animation.CrossFade">
<summary>
<para>Fades the animation with name /animation/ in over a period of /time/ seconds and fades other animations out.</para>
</summary>
<param name="animation"></param>
<param name="fadeLength"></param>
<param name="mode"></param>
</member>
<member name="M:UnityEngine.Animation.CrossFade(System.String,System.Single,UnityEngine.PlayMode)">
<summary>
<para>Fades the animation with name /animation/ in over a period of /time/ seconds and fades other animations out.</para>
</summary>
<param name="animation"></param>
<param name="fadeLength"></param>
<param name="mode"></param>
</member>
<member name="M:UnityEngine.Animation.CrossFadeQueued">
<summary>
<para>Cross fades an animation after previous animations has finished playing.</para>
</summary>
<param name="animation"></param>
没有合适的资源?快使用搜索试试~ 我知道了~
unity 3D第一人称射击游戏
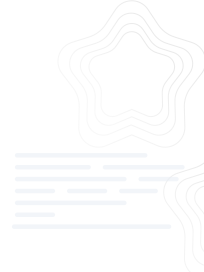
共28个文件
dll:9个
config:4个
pdb:2个


温馨提示
这是用unity 开发第一人称射击游戏,是根据教程做的,供初学者使用
资源推荐
资源详情
资源评论
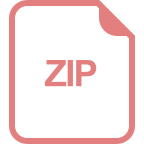
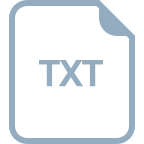
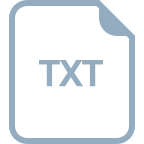
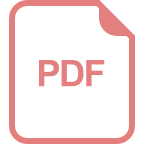
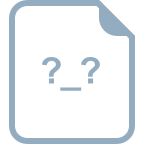
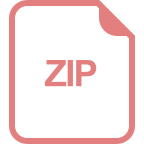
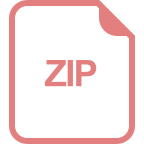
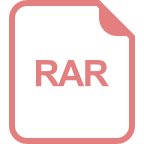
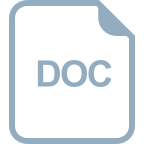
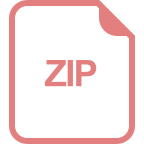
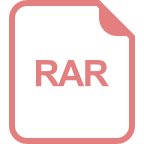
收起资源包目录


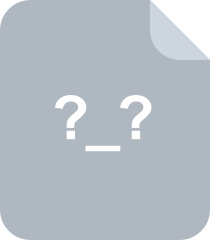

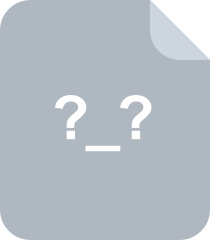





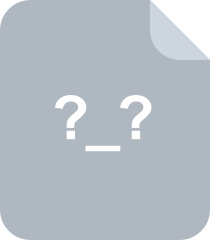
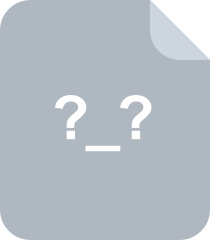
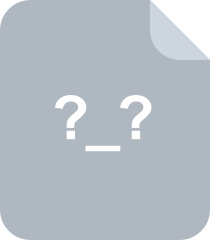
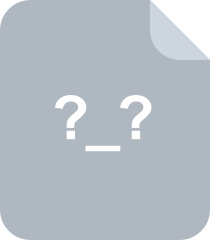
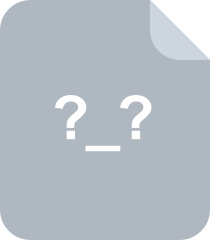
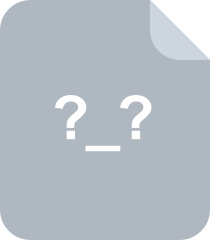

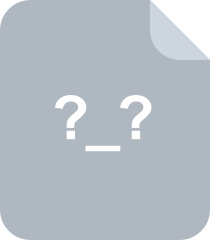

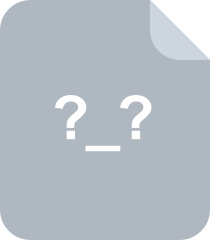
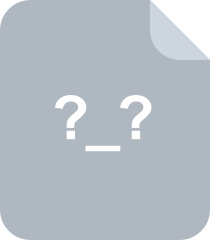
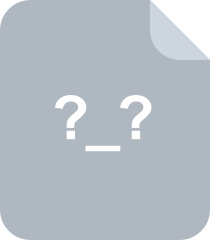
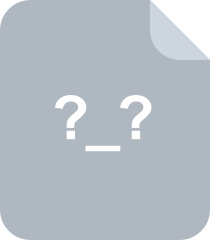

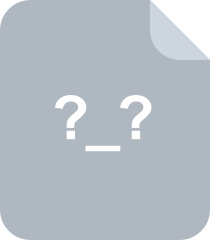
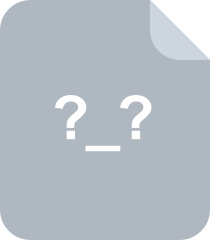

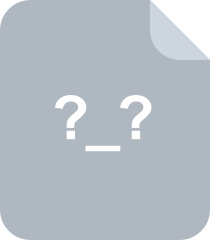
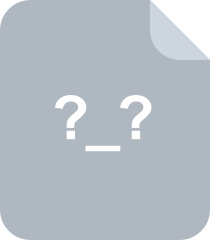
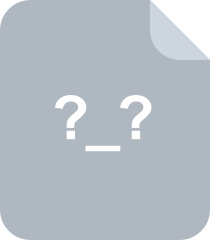
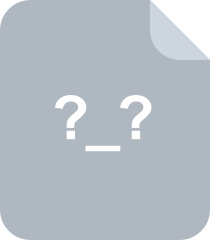
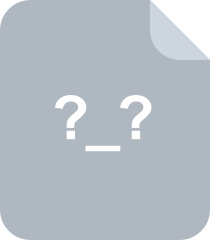
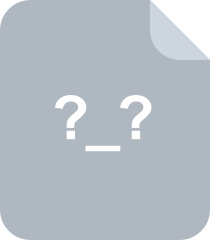
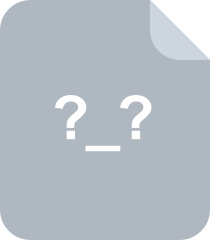
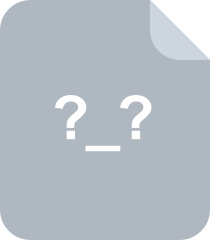
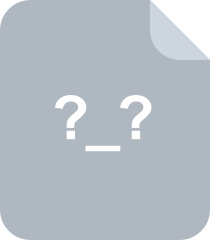
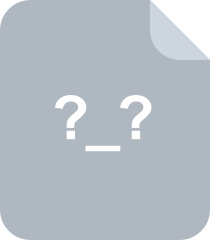
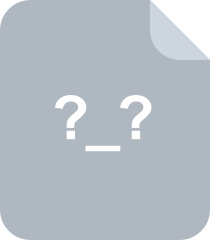
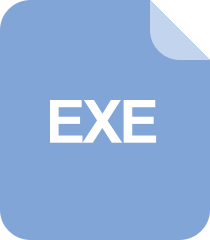
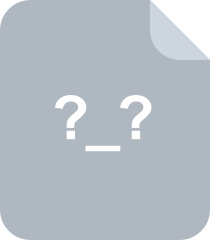
共 28 条
- 1
资源评论
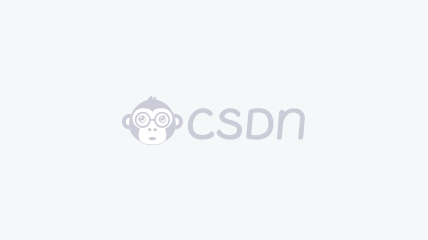
- sdsmkj2017-10-19没有源代码。。。。。
- tianyiyuli2016-11-02资源还行,就不是源码

yy763496668
- 粉丝: 1w+
- 资源: 14
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

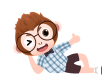
安全验证
文档复制为VIP权益,开通VIP直接复制
