package com.controller;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.*;
import javax.servlet.http.HttpServletRequest;
import com.alibaba.fastjson.JSON;
import com.utils.StringUtil;
import org.apache.commons.lang3.StringUtils;
import org.json.JSONObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.ResourceUtils;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.annotation.IgnoreAuth;
import com.baidu.aip.face.AipFace;
import com.baidu.aip.face.MatchRequest;
import com.baidu.aip.util.Base64Util;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.entity.ConfigEntity;
import com.service.CommonService;
import com.service.ConfigService;
import com.utils.BaiduUtil;
import com.utils.FileUtil;
import com.utils.R;
/**
* 通用接口
*/
@RestController
public class CommonController{
private static final Logger logger = LoggerFactory.getLogger(CommonController.class);
@Autowired
private CommonService commonService;
@Autowired
private ConfigService configService;
private static AipFace client = null;
private static String BAIDU_DITU_AK = null;
@RequestMapping("/location")
public R location(String lng,String lat) {
if(BAIDU_DITU_AK==null) {
BAIDU_DITU_AK = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "baidu_ditu_ak")).getValue();
if(BAIDU_DITU_AK==null) {
return R.error("请在配置管理中正确配置baidu_ditu_ak");
}
}
Map<String, String> map = BaiduUtil.getCityByLonLat(BAIDU_DITU_AK, lng, lat);
return R.ok().put("data", map);
}
/**
* 人脸比对
*
* @param face1 人脸1
* @param face2 人脸2
* @return
*/
@RequestMapping("/matchFace")
public R matchFace(String face1, String face2, HttpServletRequest request) {
if(client==null) {
/*String AppID = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "AppID")).getValue();*/
String APIKey = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "APIKey")).getValue();
String SecretKey = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "SecretKey")).getValue();
String token = BaiduUtil.getAuth(APIKey, SecretKey);
if(token==null) {
return R.error("请在配置管理中正确配置APIKey和SecretKey");
}
client = new AipFace(null, APIKey, SecretKey);
client.setConnectionTimeoutInMillis(2000);
client.setSocketTimeoutInMillis(60000);
}
JSONObject res = null;
try {
File file1 = new File(request.getSession().getServletContext().getRealPath("/upload")+"/"+face1);
File file2 = new File(request.getSession().getServletContext().getRealPath("/upload")+"/"+face2);
String img1 = Base64Util.encode(FileUtil.FileToByte(file1));
String img2 = Base64Util.encode(FileUtil.FileToByte(file2));
MatchRequest req1 = new MatchRequest(img1, "BASE64");
MatchRequest req2 = new MatchRequest(img2, "BASE64");
ArrayList<MatchRequest> requests = new ArrayList<MatchRequest>();
requests.add(req1);
requests.add(req2);
res = client.match(requests);
System.out.println(res.get("result"));
} catch (FileNotFoundException e) {
e.printStackTrace();
return R.error("文件不存在");
} catch (IOException e) {
e.printStackTrace();
}
return R.ok().put("data", com.alibaba.fastjson.JSONObject.parse(res.get("result").toString()));
}
/**
* 获取table表中的column列表(联动接口)
* @return
*/
@RequestMapping("/option/{tableName}/{columnName}")
@IgnoreAuth
public R getOption(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName,String level,String parent) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
if(StringUtils.isNotBlank(level)) {
params.put("level", level);
}
if(StringUtils.isNotBlank(parent)) {
params.put("parent", parent);
}
List<String> data = commonService.getOption(params);
return R.ok().put("data", data);
}
/**
* 根据table中的column获取单条记录
* @return
*/
@RequestMapping("/follow/{tableName}/{columnName}")
@IgnoreAuth
public R getFollowByOption(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName, @RequestParam String columnValue) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
params.put("columnValue", columnValue);
Map<String, Object> result = commonService.getFollowByOption(params);
return R.ok().put("data", result);
}
/**
* 修改table表的sfsh状态
* @param map
* @return
*/
@RequestMapping("/sh/{tableName}")
public R sh(@PathVariable("tableName") String tableName, @RequestBody Map<String, Object> map) {
map.put("table", tableName);
commonService.sh(map);
return R.ok();
}
/**
* 获取需要提醒的记录数
* @param tableName
* @param columnName
* @param type 1:数字 2:日期
* @param map
* @return
*/
@RequestMapping("/remind/{tableName}/{columnName}/{type}")
@IgnoreAuth
public R remindCount(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("table", tableName);
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date remindEndDate = null;
if(map.get("remindstart")!=null) {
Integer remindStart = Integer.parseInt(map.get("remindstart").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindStart);
remindStartDate = c.getTime();
map.put("remindstart", sdf.format(remindStartDate));
}
if(map.get("remindend")!=null) {
Integer remindEnd = Integer.parseInt(map.get("remindend").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindEnd);
remindEndDate = c.getTime();
map.put("remindend", sdf.format(remindEndDate));
}
}
int count = commonService.remindCount(map);
return R.ok().put("count", count);
}
/**
* 圖表统计
*/
@IgnoreAuth
@RequestMapping("/group/{tableName}")
public R group1(@PathVariable("tableName") String tableName, @RequestParam Map<String,Object> params) {
params.put("table1", tableName);
List<Map<String, Object>> result = commonService.chartBoth(params);
return R.ok().put("data", result);
}
/**
* 单列求和
*/
@RequestMapping("/cal/{tableName}/{columnName}")
@IgnoreAuth
public R cal(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
Map<String, Object> result = commonService.selectCal(params);
return R.ok().put("data", result);
}
/**
* 分组统计
*/
@RequestMapping("/group/{tableName}/{columnName}")
@IgnoreAuth
public R group(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableN
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
毕业设计javassm驾考自主学习预约平台+vue源码含教程 后台是ssm框架,后台的页面是vue,前端页面是html,数据库mysql,jdk1.8,开发工具用ecplise、myecplise、sts、idea都可以 包含:源码、数据库脚本、环境工具包、相同框架项目的安装教程(在说明文档中)
资源推荐
资源详情
资源评论
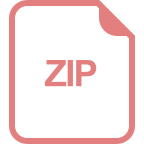
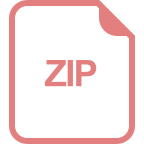
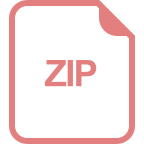
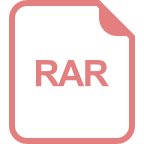
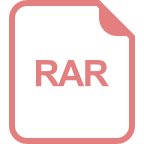
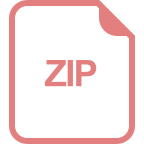
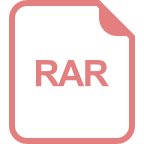
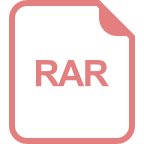
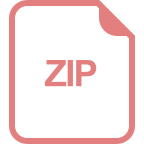
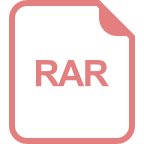
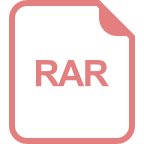
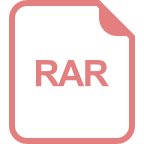
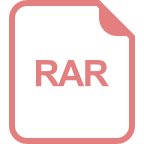
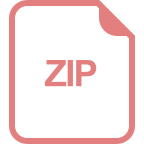
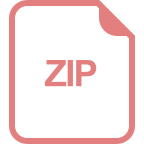
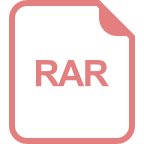
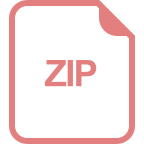
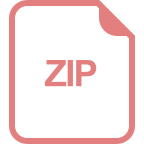
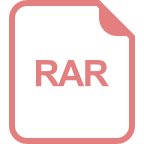
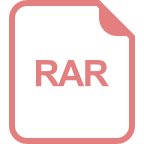
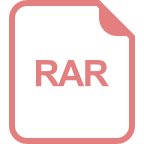
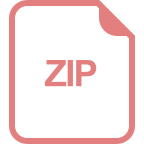
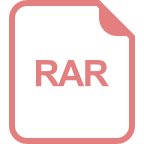
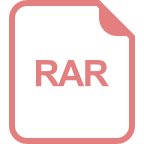
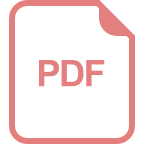
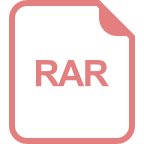
收起资源包目录

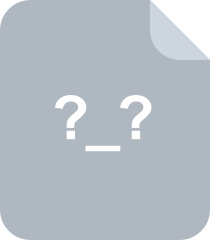
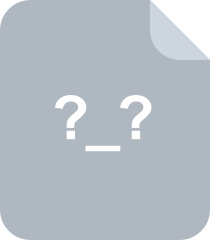
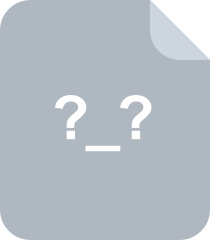
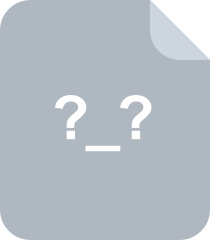
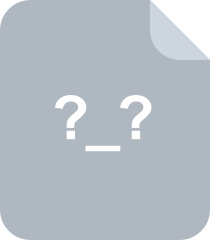
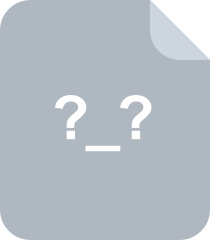
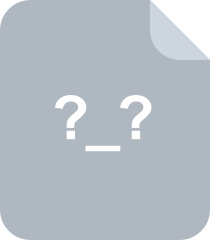
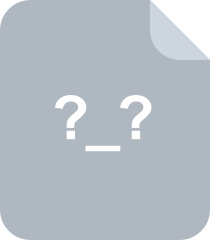
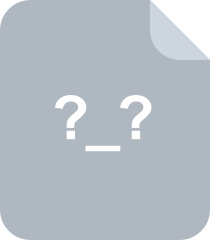
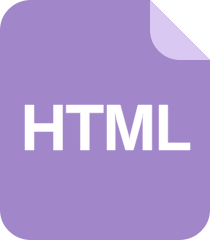
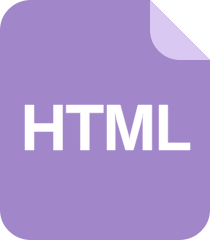
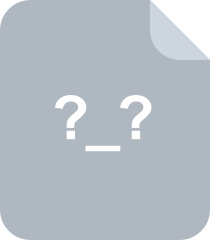
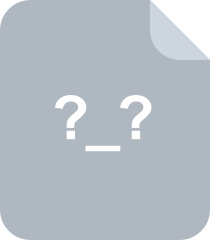
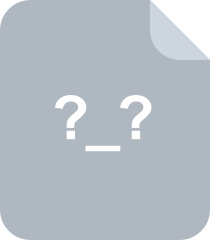
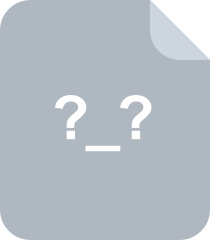
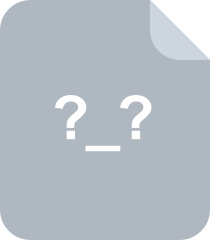
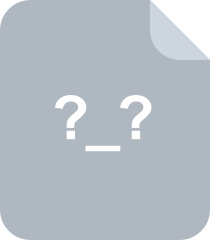
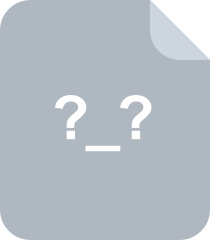
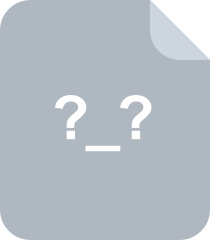
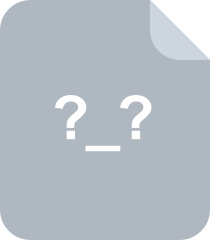
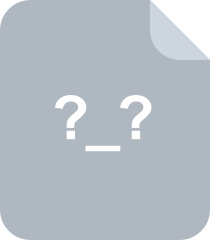
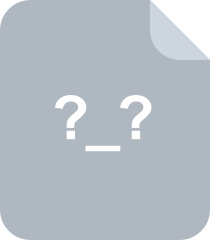
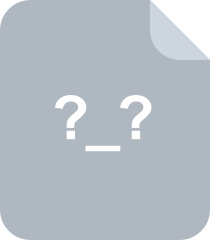
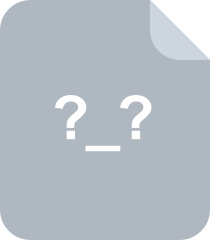
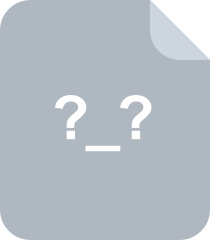
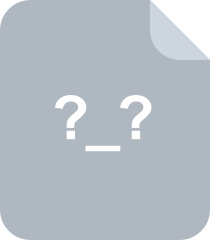
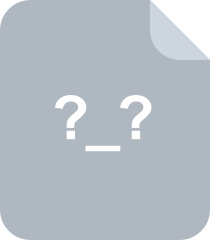
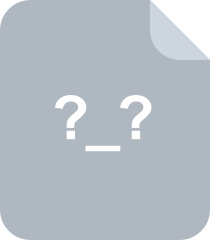
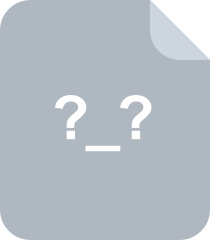
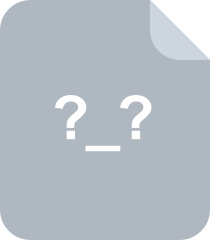
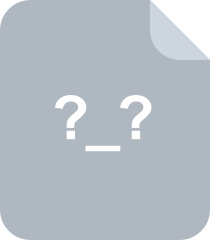
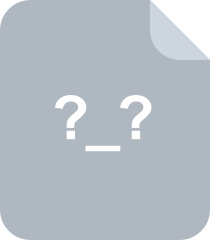
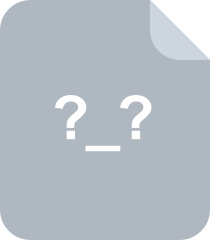
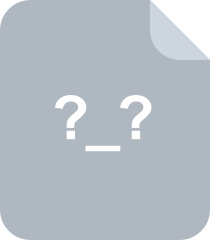
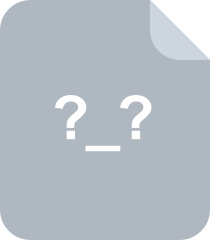
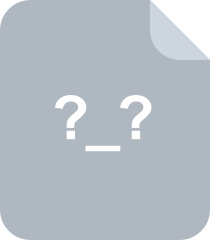
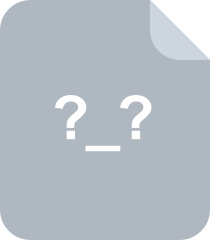
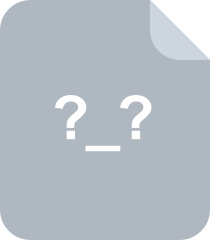
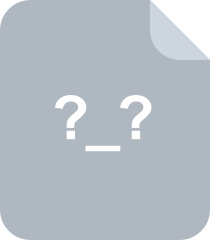
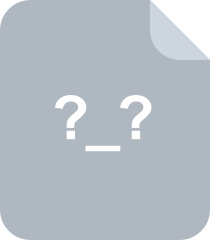
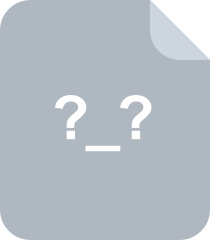
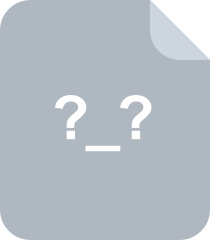
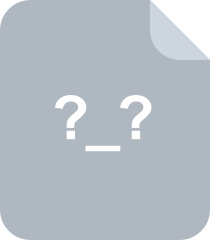
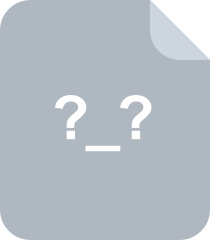
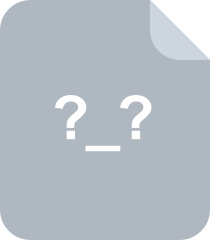
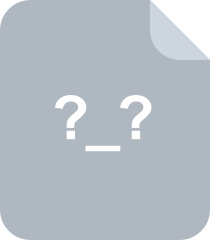
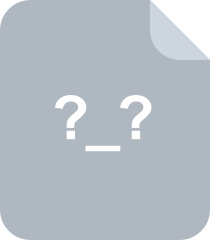
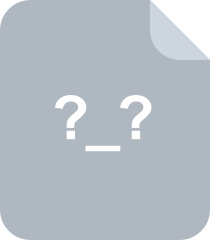
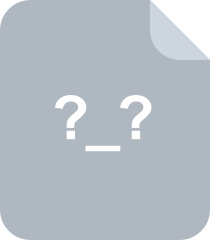
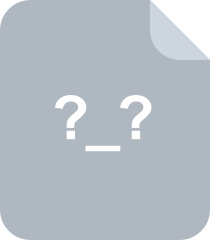
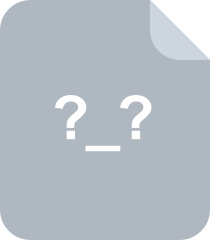
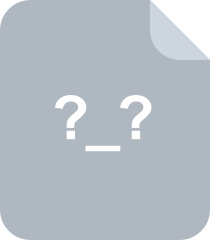
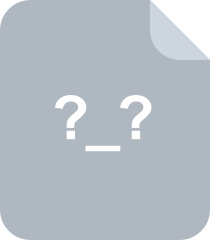
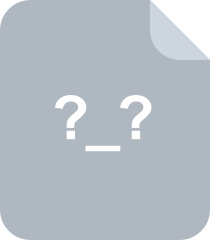
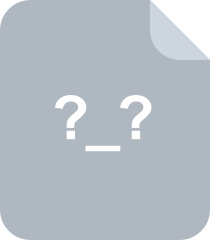
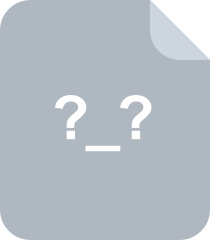
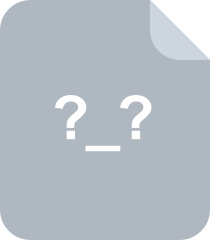
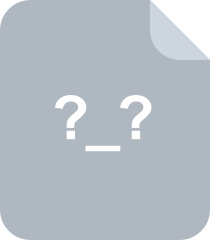
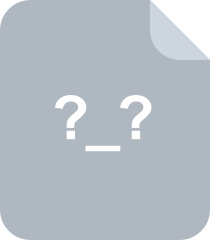
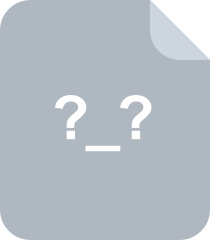
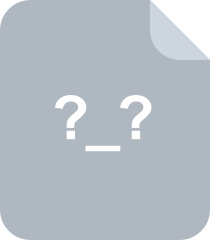
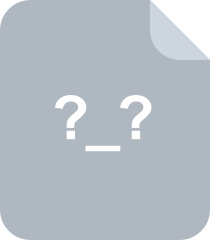
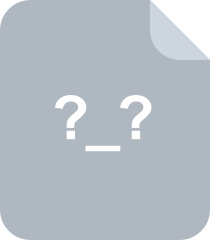
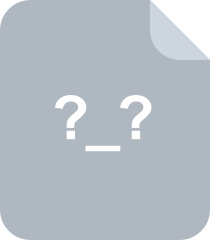
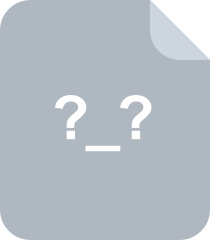
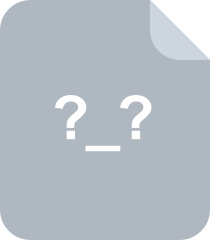
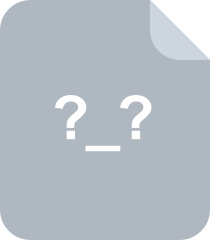
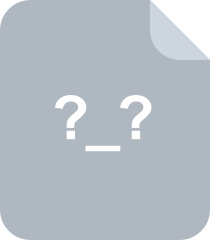
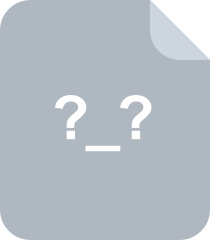
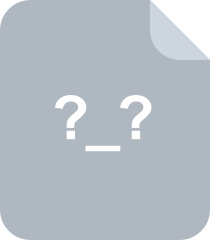
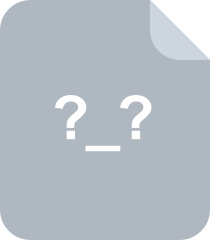
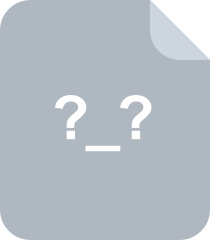
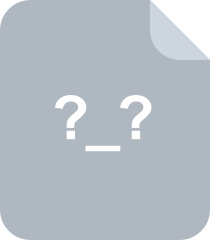
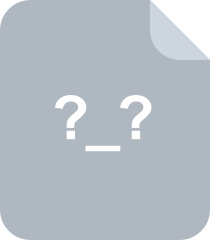
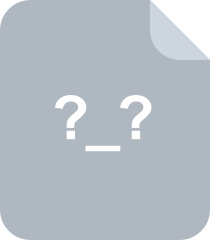
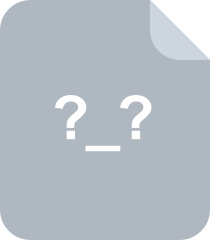
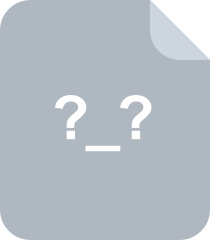
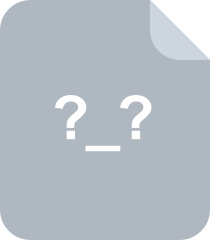
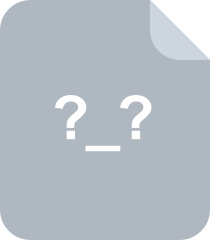
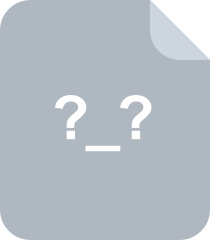
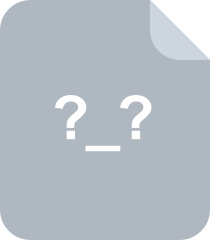
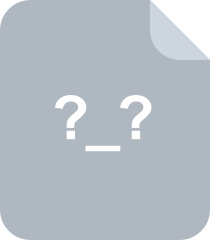
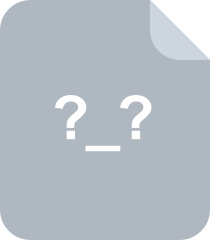
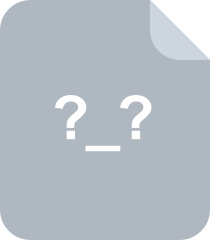
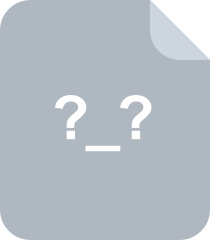
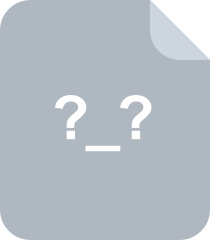
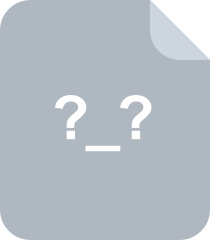
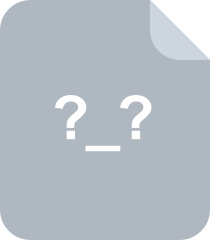
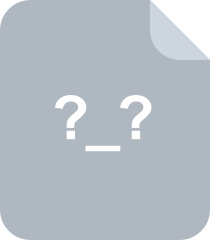
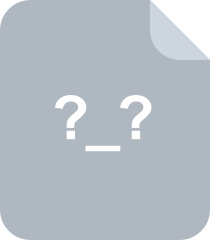
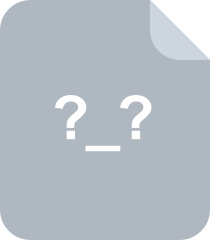
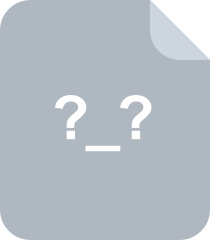
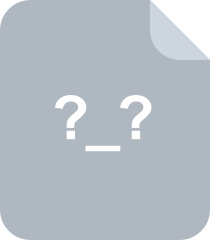
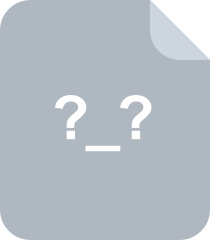
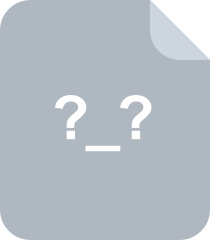
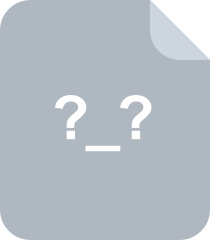
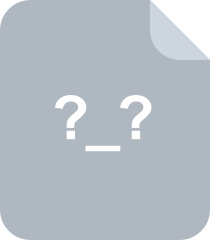
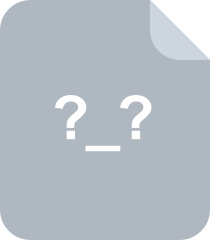
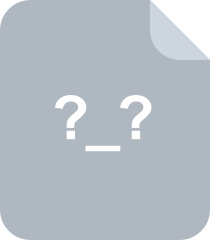
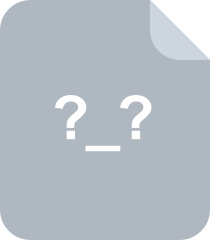
共 490 条
- 1
- 2
- 3
- 4
- 5
资源评论
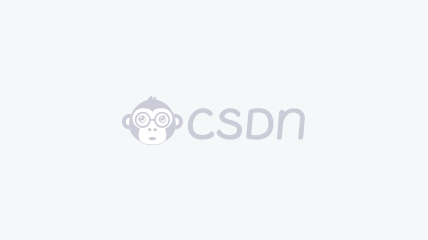

言宇程序
- 粉丝: 2413
- 资源: 5237
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

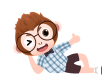
最新资源
- 本项目主要是用于微信夸夸群,之前也有很多夸夸的项目,但是效果不是很好,很容易答非所问,该项目对数据进行了优化升级 主要使用的语言为nodejs + python.zip
- 本站提出并实现了属性加密演示系统,旨在帮助初学者更好地理解属性加密技术,同时采用图文并茂的方式和通俗易懂的语言生动形象的介绍了属性加密的含义和相关理论知识 .zip
- Vue框架开发指南:从基础到高级应用
- JAVA栈(链表实现)
- 有源钳位正激功率级计算书
- 微信机器人,企业微信机器人,微信群机器人源码,微信机器人框架源码,微信发卡机器人源码,微信群聊机器人源码,微信群机器人功能菜单,易语言微信机器人源码,微信机器人源码php,微信群机器人管理系统.zip
- 废弃的易语言源码,已无力支撑下去更新 放弃,甚至有些开发一半夭折废弃.zip
- JAVA栈(链表实现)
- 将JSON数据类型一键转换为易语言自定义数据类型,喜欢的给个star吧!欢迎贡献code.zip
- 抽象代数期末试卷题目1
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


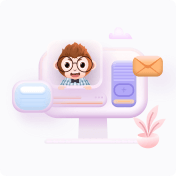
安全验证
文档复制为VIP权益,开通VIP直接复制
