/**
* @requires OpenLayers/BaseTypes/Class.js
* @requires OpenLayers/Util.js
* @requires OpenLayers/Control.js
* @requires OpenLayers/Format.js
* @requires OpenLayers/Request.js
* @requires OpenLayers/Layer/WMS.js
* @requires OpenLayers/Layer/MapServer.js
* @requires OpenLayers/Tile.js
* @requires OpenLayers/Request/XMLHttpRequest.js
* @requires OpenLayers/Layer/Vector.js
* @requires OpenLayers/Layer/Markers.js
* @requires OpenLayers/Console.js
* @requires OpenLayers/Lang.js
* @requires OpenLayers/Feature.js
* @requires OpenLayers/Layer/EventPane.js
* @requires OpenLayers/Layer/FixedZoomLevels.js
* @requires OpenLayers/Layer/SphericalMercator.js
* @requires OpenLayers/Protocol.js
* @requires OpenLayers/Format/JSON.js
* @requires OpenLayers/Format/WKT.js
* @requires OpenLayers/Format/XML.js
* @requires OpenLayers/Geometry.js
* @requires OpenLayers/Renderer/Elements.js
*/
/**
* About: Deprecated
* The deprecated.js script includes all methods, properties, and constructors
* that are not supported as part of the long-term API. If you use any of
* these, you have to explicitly include this script in your application.
*
* For example:
* (code)
* <script src="deprecated.js" type="text/javascript"></script>
* (end)
*
* You are strongly encouraged to avoid using deprecated functionality. The
* documentation here should point you to the supported alternatives.
*/
/**
* Namespace: OpenLayers.Class
*/
/**
* Property: isPrototype
* *Deprecated*. This is no longer needed and will be removed at 3.0.
*/
OpenLayers.Class.isPrototype = function () {};
/**
* APIFunction: OpenLayers.create
* *Deprecated*. Old method to create an OpenLayers style class. Use the
* <OpenLayers.Class> constructor instead.
*
* Returns:
* An OpenLayers class
*/
OpenLayers.Class.create = function() {
return function() {
if (arguments && arguments[0] != OpenLayers.Class.isPrototype) {
this.initialize.apply(this, arguments);
}
};
};
/**
* APIFunction: inherit
* *Deprecated*. Old method to inherit from one or more OpenLayers style
* classes. Use the <OpenLayers.Class> constructor instead.
*
* Parameters:
* class - One or more classes can be provided as arguments
*
* Returns:
* An object prototype
*/
OpenLayers.Class.inherit = function (P) {
var C = function() {
P.call(this);
};
var newArgs = [C].concat(Array.prototype.slice.call(arguments));
OpenLayers.inherit.apply(null, newArgs);
return C.prototype;
};
/**
* Namespace: OpenLayers.Util
*/
/**
* Function: clearArray
* *Deprecated*. This function will disappear in 3.0.
* Please use "array.length = 0" instead.
*
* Parameters:
* array - {Array}
*/
OpenLayers.Util.clearArray = function(array) {
OpenLayers.Console.warn(
OpenLayers.i18n(
"methodDeprecated", {'newMethod': 'array = []'}
)
);
array.length = 0;
};
/**
* Function: setOpacity
* *Deprecated*. This function has been deprecated. Instead, please use
* <OpenLayers.Util.modifyDOMElement>
* or
* <OpenLayers.Util.modifyAlphaImageDiv>
*
* Set the opacity of a DOM Element
* Note that for this function to work in IE, elements must "have layout"
* according to:
* http://msdn.microsoft.com/workshop/author/dhtml/reference/properties/haslayout.asp
*
* Parameters:
* element - {DOMElement} Set the opacity on this DOM element
* opacity - {Float} Opacity value (0.0 - 1.0)
*/
OpenLayers.Util.setOpacity = function(element, opacity) {
OpenLayers.Util.modifyDOMElement(element, null, null, null,
null, null, null, opacity);
};
/**
* Function: safeStopPropagation
* *Deprecated*. This function has been deprecated. Please use directly
* <OpenLayers.Event.stop> passing 'true' as the 2nd
* argument (preventDefault)
*
* Safely stop the propagation of an event *without* preventing
* the default browser action from occurring.
*
* Parameters:
* evt - {Event}
*/
OpenLayers.Util.safeStopPropagation = function(evt) {
OpenLayers.Event.stop(evt, true);
};
/**
* Function: getArgs
* *Deprecated*. Will be removed in 3.0. Please use instead
* <OpenLayers.Util.getParameters>
*
* Parameters:
* url - {String} Optional url used to extract the query string.
* If null, query string is taken from page location.
*
* Returns:
* {Object} An object of key/value pairs from the query string.
*/
OpenLayers.Util.getArgs = function(url) {
OpenLayers.Console.warn(
OpenLayers.i18n(
"methodDeprecated", {'newMethod': 'OpenLayers.Util.getParameters'}
)
);
return OpenLayers.Util.getParameters(url);
};
/**
* Maintain existing definition of $.
*
* The use of our $-method is deprecated and the mapping of
* OpenLayers.Util.getElement will eventually be removed. Do not depend on
* window.$ being defined by OpenLayers.
*/
if(typeof window.$ === "undefined") {
window.$ = OpenLayers.Util.getElement;
}
/**
* Namespace: OpenLayers.Ajax
*/
/**
* Function: OpenLayers.nullHandler
* @param {} request
*/
OpenLayers.nullHandler = function(request) {
OpenLayers.Console.userError(OpenLayers.i18n("unhandledRequest", {'statusText':request.statusText}));
};
/**
* APIFunction: OpenLayers.loadURL
* Background load a document.
* *Deprecated*. Use <OpenLayers.Request.GET> method instead.
*
* Parameters:
* uri - {String} URI of source doc
* params - {String} or {Object} GET params. Either a string in the form
* "?hello=world&foo=bar" (do not forget the leading question mark)
* or an object in the form {'hello': 'world', 'foo': 'bar}
* caller - {Object} object which gets callbacks
* onComplete - {Function} Optional callback for success. The callback
* will be called with this set to caller and will receive the request
* object as an argument. Note that if you do not specify an onComplete
* function, <OpenLayers.nullHandler> will be called (which pops up a
* user friendly error message dialog).
* onFailure - {Function} Optional callback for failure. In the event of
* a failure, the callback will be called with this set to caller and will
* receive the request object as an argument. Note that if you do not
* specify an onComplete function, <OpenLayers.nullHandler> will be called
* (which pops up a user friendly error message dialog).
*
* Returns:
* {<OpenLayers.Request.XMLHttpRequest>} The request object. To abort loading,
* call request.abort().
*/
OpenLayers.loadURL = function(uri, params, caller,
onComplete, onFailure) {
if(typeof params == 'string') {
params = OpenLayers.Util.getParameters(params);
}
var success = (onComplete) ? onComplete : OpenLayers.nullHandler;
var failure = (onFailure) ? onFailure : OpenLayers.nullHandler;
return OpenLayers.Request.GET({
url: uri, params: params,
success: success, failure: failure, scope: caller
});
};
/**
* Function: OpenLayers.parseXMLString
* Parse XML into a doc structure
*
* Parameters:
* text - {String}
*
* Returns:
* {?} Parsed AJAX Responsev
*/
OpenLayers.parseXMLString = function(text) {
//MS sucks, if the server is bad it dies
var index = text.indexOf('<');
if (index > 0) {
text = text.substring(index);
}
var ajaxResponse = OpenLayers.Util.Try(
function() {
var xmldom = new ActiveXObject('Microsoft.XMLDOM');
xmldom.loadXML(text);
return xmldom;
},
function() {
return new DOMParser().parseFromString(text, 'text/xml');
},
function() {
var req = new XMLHttpRequest();
req.open("GET", "data:" + "text/xml" +
";charset=utf-8," + encodeURIComponent(text), false);
if (req.overri
没有合适的资源?快使用搜索试试~ 我知道了~
openlayers实现本地图片作为背景,添加marker标记
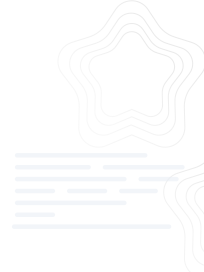
共404个文件
js:320个
png:58个
css:6个


温馨提示
刚刚使用openlayers做了一个简单的小例子,注释很全,很简单,将本地的图片作为背景,能够按照坐标添加任意个标记
资源推荐
资源详情
资源评论
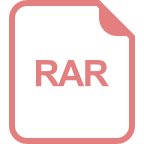
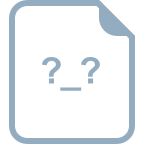
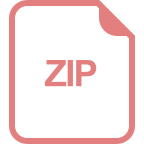
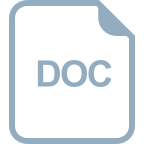
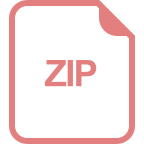
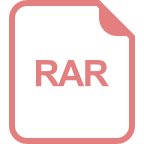
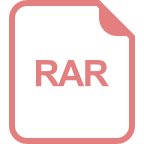
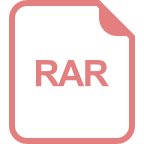
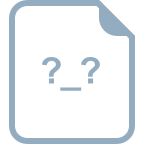
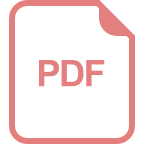
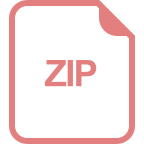
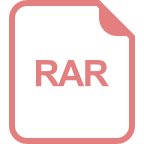
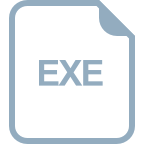
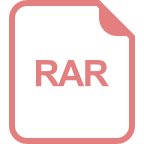
收起资源包目录

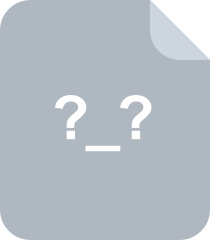
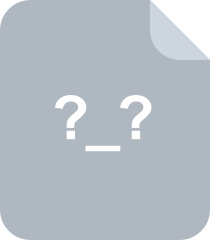
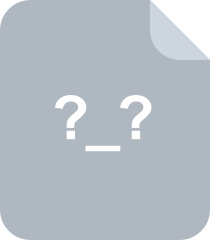
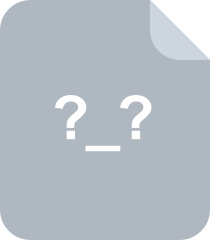
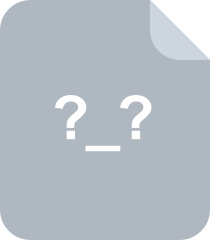
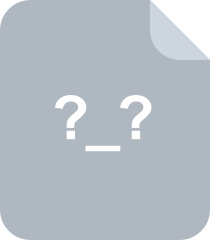
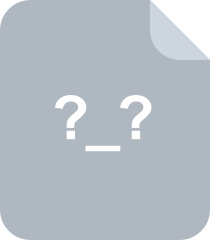
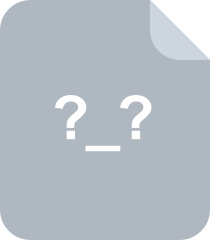
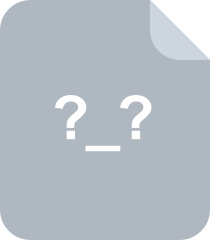
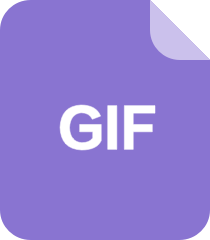
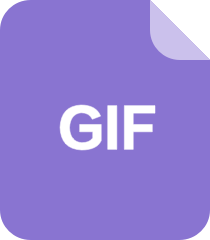
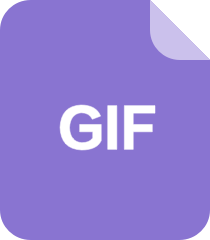
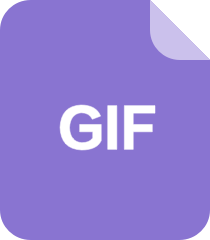
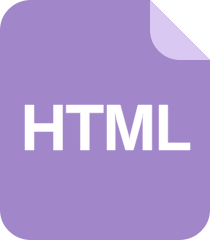
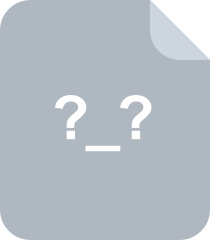
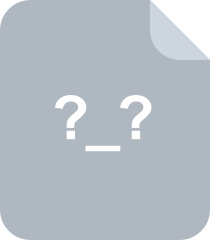
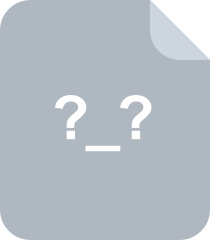
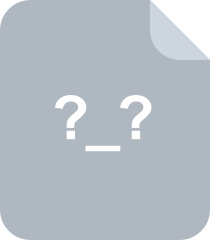
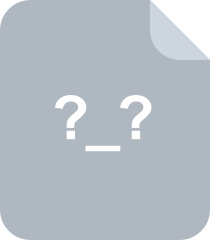
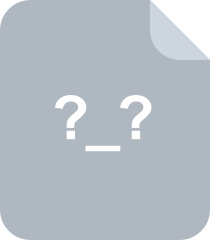
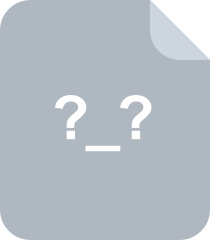
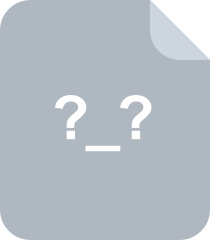
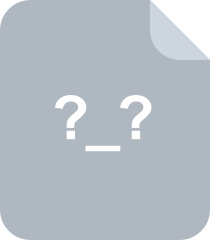
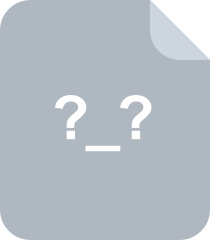
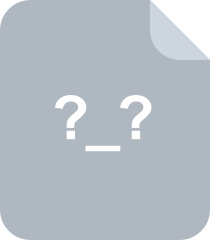
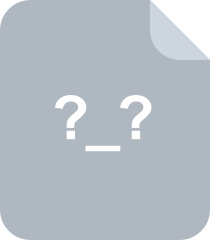
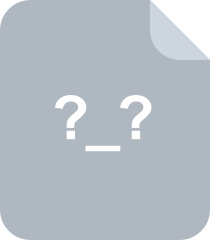
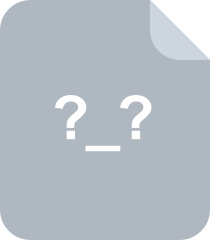
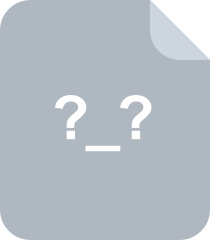
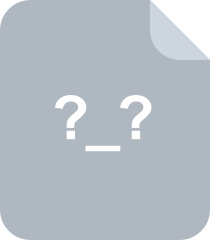
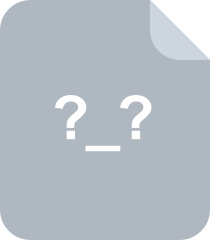
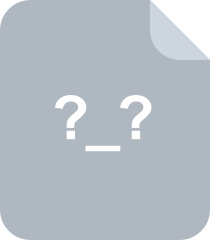
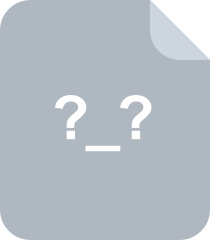
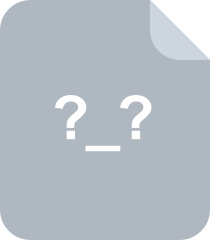
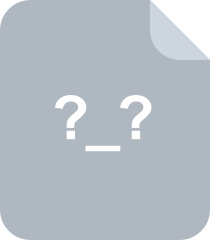
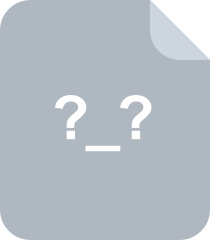
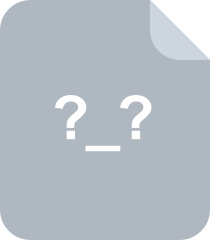
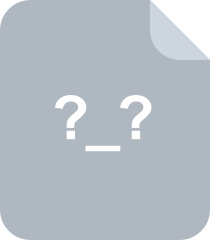
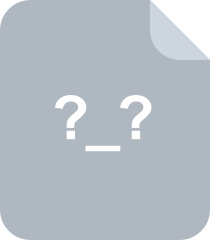
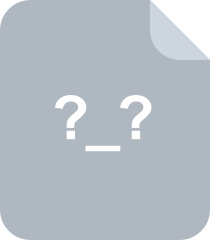
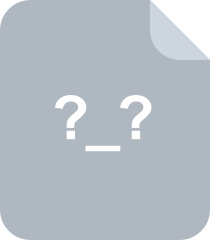
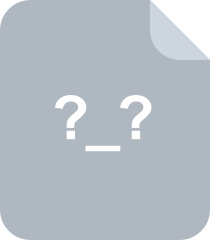
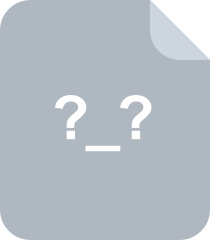
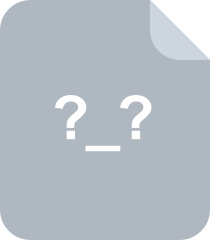
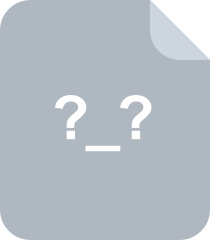
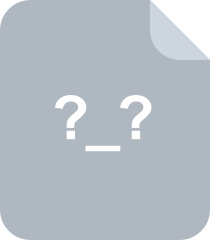
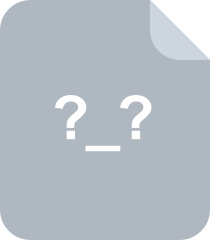
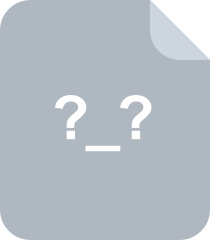
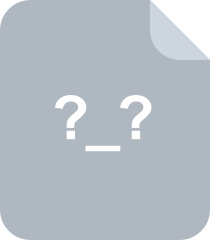
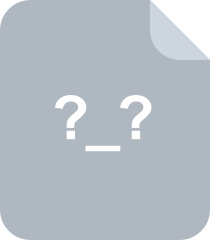
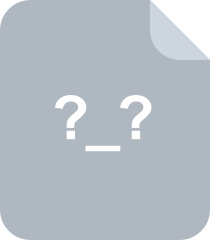
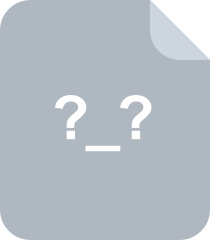
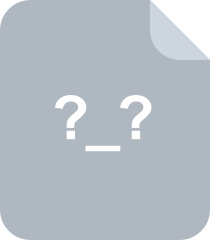
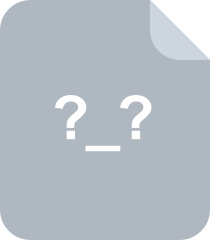
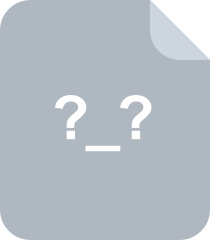
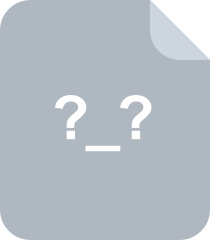
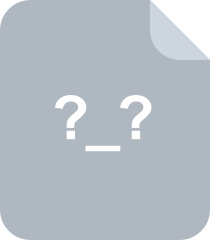
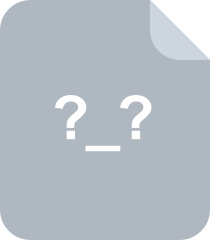
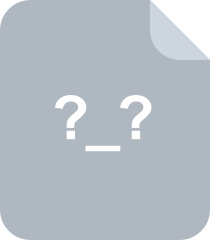
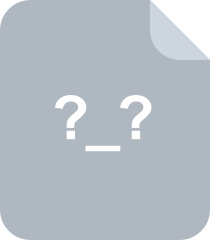
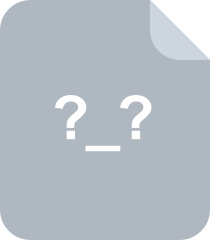
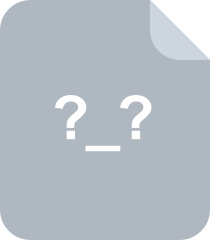
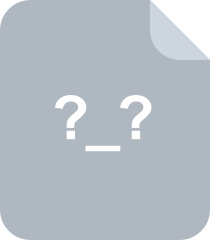
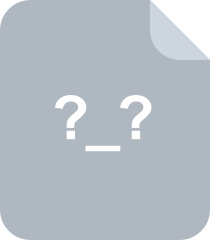
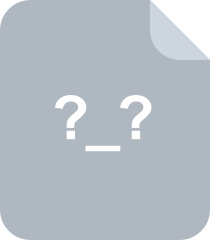
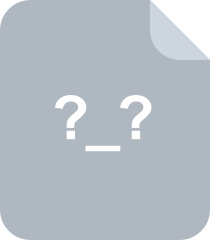
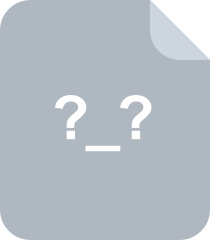
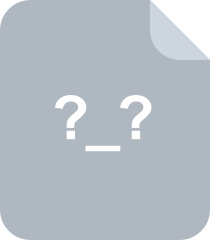
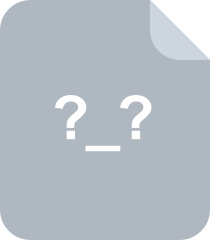
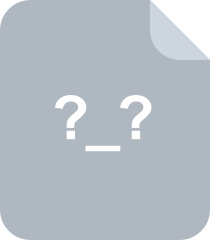
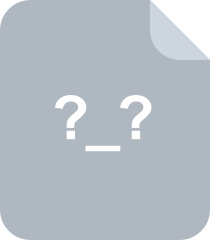
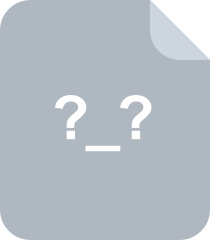
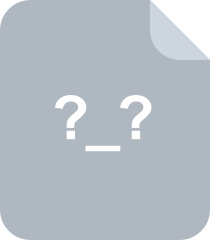
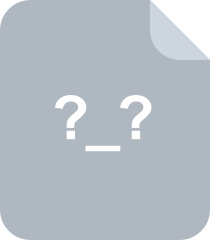
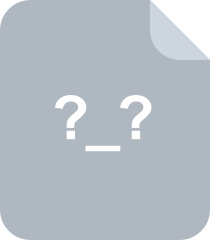
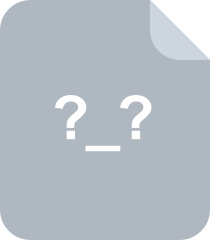
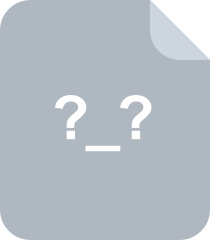
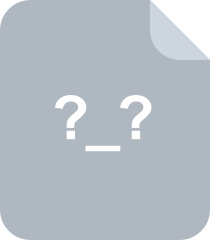
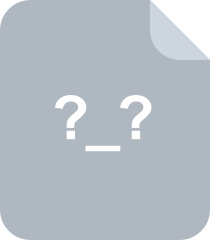
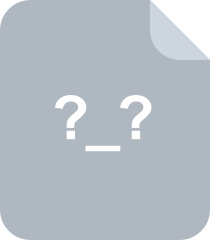
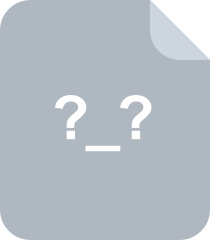
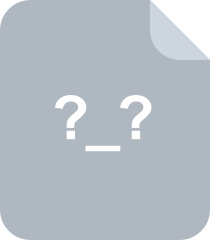
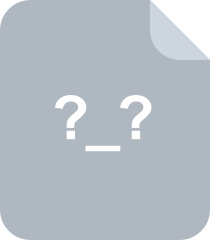
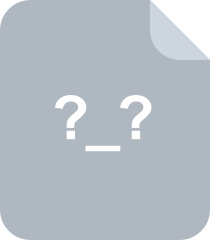
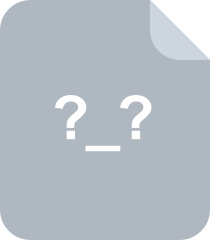
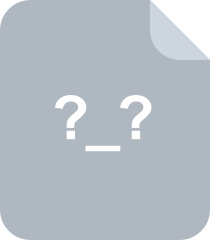
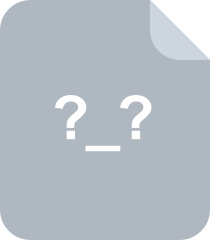
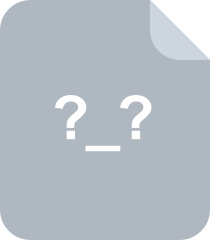
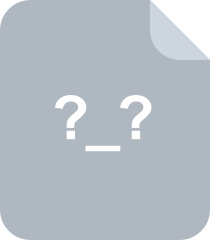
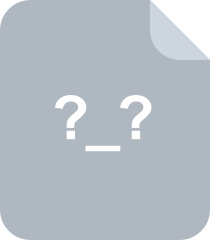
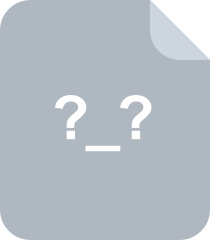
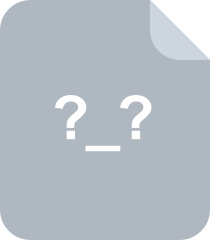
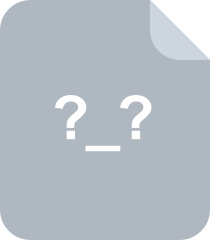
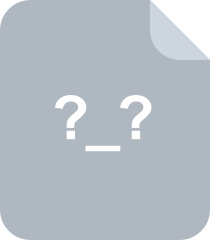
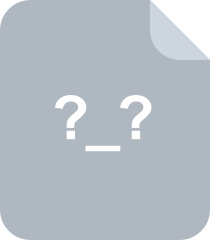
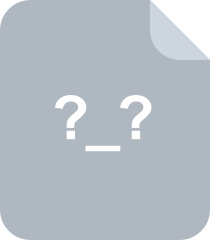
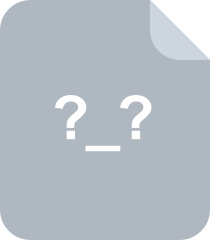
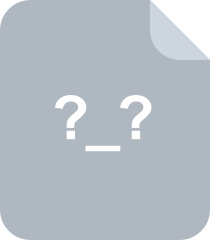
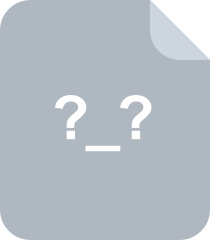
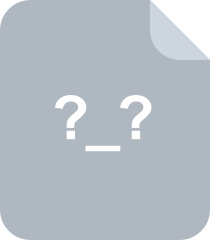
共 404 条
- 1
- 2
- 3
- 4
- 5

yy251066394
- 粉丝: 17
- 资源: 17
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

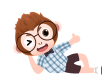
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页