<?php
// +----------------------------------------------------------------------
// | ThinkPHP
// +----------------------------------------------------------------------
// | Copyright (c) 2008 http://thinkphp.cn All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( http://www.apache.org/licenses/LICENSE-2.0 )
// +----------------------------------------------------------------------
// | Author: liu21st <liu21st@gmail.com>
// +----------------------------------------------------------------------
// $Id$
define('HAS_ONE',1);
define('BELONGS_TO',2);
define('HAS_MANY',3);
define('MANY_TO_MANY',4);
define('MUST_TO_VALIDATE',1); // 必须验证
define('EXISTS_TO_VAILIDATE',0); // 表单存在字段则验证
define('VALUE_TO_VAILIDATE',2); // 表单值不为空则验证
/**
+------------------------------------------------------------------------------
* ThinkPHP Model模型类 抽象类
* 实现了ORM和ActiveRecords模式
+------------------------------------------------------------------------------
* @category Think
* @package Think
* @subpackage Core
* @author liu21st <liu21st@gmail.com>
* @version $Id$
+------------------------------------------------------------------------------
*/
class Model extends Base implements IteratorAggregate
{
// 数据库连接对象列表
protected $_db = array();
// 当前数据库操作对象
protected $db = null;
// 数据表前缀
protected $tablePrefix = '';
// 数据表后缀
protected $tableSuffix = '';
// 模型名称
protected $name = '';
// 数据库名称
protected $dbName = '';
// 数据表名(不包含表前缀)
protected $tableName = '';
// 实际数据表名(包含表前缀)
protected $trueTableName ='';
// 字段信息
protected $fields = array();
// 字段类型信息
protected $type = array();
// 数据信息
protected $data = array();
// 查询表达式参数
protected $options = array();
// 数据列表信息
protected $dataList = array();
// 上次错误信息
protected $error = '';
// 验证错误信息
protected $validateError = array();
// 包含的聚合对象
protected $aggregation = array();
// 是否为复合对象
protected $composite = false;
// 是否为视图模型
protected $viewModel = false;
// 乐观锁
protected $optimLock = 'lock_version';
// 悲观锁
protected $pessimisticLock = false;
protected $autoSaveRelations = false; // 自动关联保存
protected $autoDelRelations = false; // 自动关联删除
protected $autoAddRelations = false; // 自动关联写入
protected $autoReadRelations = false; // 自动关联查询
protected $lazyQuery = false; // 是否启用惰性查询
// 自动写入时间戳
protected $autoCreateTimestamps = array('create_at','create_on','cTime');
protected $autoUpdateTimestamps = array('update_at','update_on','mTime');
protected $autoTimeFormat = '';
protected $blobFields = null;
protected $blobValues = null;
/**
+----------------------------------------------------------
* 架构函数
* 取得DB类的实例对象 数据表字段检查
+----------------------------------------------------------
* @access public
+----------------------------------------------------------
* @param mixed $data 要创建的数据对象内容
+----------------------------------------------------------
*/
public function __construct($data='')
{
// 模型初始化
$this->_initialize();
// 模型名称获取
$this->name = $this->getModelName();
// 如果不是复合对象进行数据库初始化操作
if(!$this->composite) {
import("Think.Db.Db");
// 获取数据库操作对象
if(!empty($this->connection)) {
// 当前模型有独立的数据库连接信息
$this->db = Db::getInstance($this->connection);
}else{
$this->db = Db::getInstance();
}
// 设置数据库的返回数据格式
$this->db->resultType = C('DATA_RESULT_TYPE');
//为获得ORACLE自增LastID而统一考虑的
$this->db->tableName = $this->parseName($this->name);
// 设置默认的数据库连接
$this->_db[0] = $this->db;
// 设置表前后缀
$this->tablePrefix = $this->tablePrefix?$this->tablePrefix:C('DB_PREFIX');
$this->tableSuffix = $this->tableSuffix?$this->tableSuffix:C('DB_SUFFIX');
// 数据表字段检测
$this->_checkTableInfo();
}
// 如果有data数据进行实例化,则创建数据对象
if(!empty($data)) {
$this->create($data);
}
}
/**
+----------------------------------------------------------
* 取得模型实例对象
+----------------------------------------------------------
* @static
* @access public
+----------------------------------------------------------
* @return mixed 返回数据模型实例
+----------------------------------------------------------
*/
public static function getInstance()
{
return get_instance_of(__CLASS__);
}
/**
+----------------------------------------------------------
* 设置数据对象的值 (魔术方法)
+----------------------------------------------------------
* @access public
+----------------------------------------------------------
* @param string $name 名称
* @param mixed $value 值
+----------------------------------------------------------
* @return void
+----------------------------------------------------------
*/
public function __set($name,$value) {
// 设置数据对象属性
$this->data[$name] = $value;
}
/**
+----------------------------------------------------------
* 获取数据对象的值 (魔术方法)
+----------------------------------------------------------
* @access public
+----------------------------------------------------------
* @param string $name 名称
+----------------------------------------------------------
* @return mixed
+----------------------------------------------------------
*/
public function __get($name) {
if(isset($this->data[$name])) {
return $this->data[$name];
}elseif(property_exists($this,$name)){
return $this->$name;
}else{
return null;
}
}
/**
+----------------------------------------------------------
* 利用__call方法重载 实现一些特殊的Model方法 (魔术方法)
+----------------------------------------------------------
* @access public
+----------------------------------------------------------
* @param string $method 方法名称
* @param mixed $args 调用参数
+----------------------------------------------------------
* @return mixed
+----------------------------------------------------------
*/
public function __call($method,$args) {
if(strtolower(substr($method,0,5))=='getby') {
// 根据某个字段获取记录
$field = $this->parseName(substr($method,5));
if(in_array($field,$this->fields,true)) {
array_unshi
没有合适的资源?快使用搜索试试~ 我知道了~
[聊天留言]zvchat 2.1.3 Release_zvchat.zip源码PHP项目源代码下载
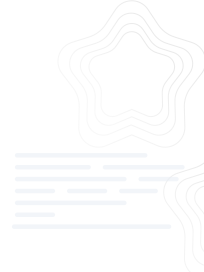
共421个文件
php:368个
dist:20个
html:8个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 100 浏览量
2022-03-15
16:34:54
上传
评论
收藏 969KB ZIP 举报
温馨提示
[聊天留言]zvchat 2.1.3 Release_zvchat.zip源码PHP项目源代码下载[聊天留言]zvchat 2.1.3 Release_zvchat.zip源码PHP项目源代码下载 1.适合个人搭建网站项目参考 2.适合学生毕业设计搭建网站参考 3.适合小公司搭建网站项目参考
资源推荐
资源详情
资源评论
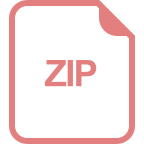
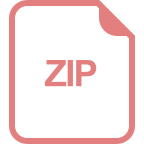
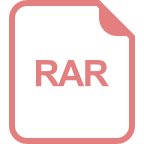
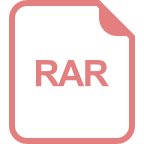
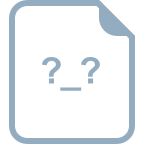
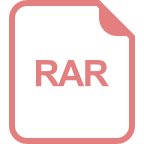
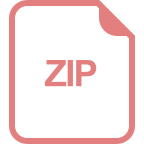
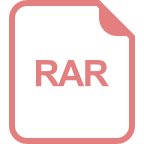
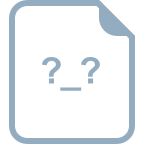
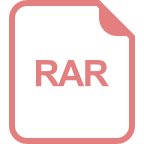
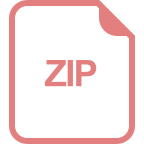
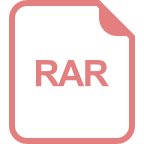
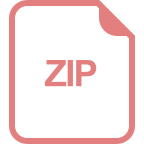
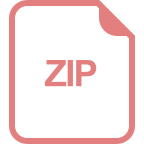
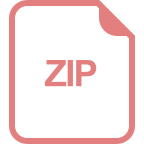
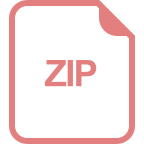
收起资源包目录

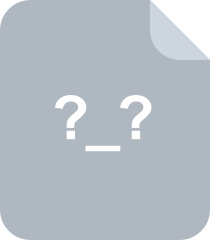
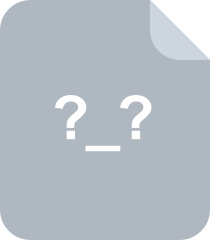
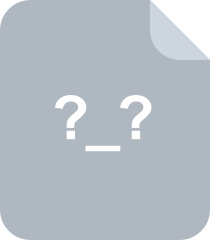
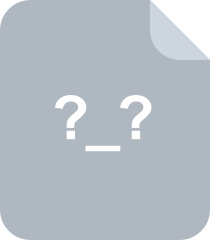
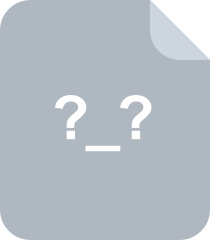
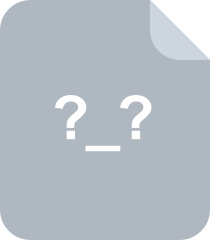
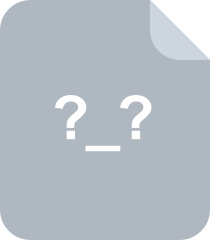
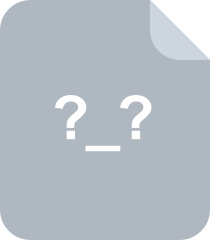
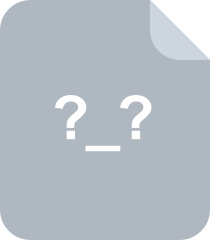
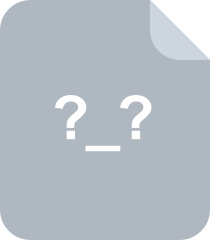
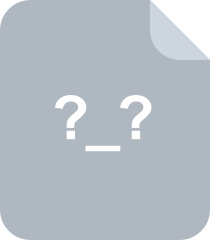
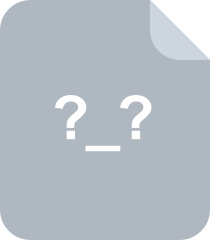
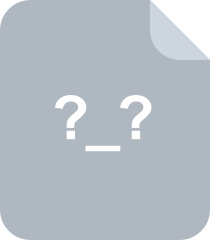
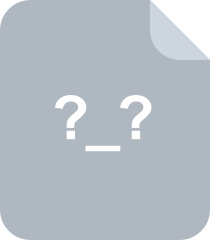
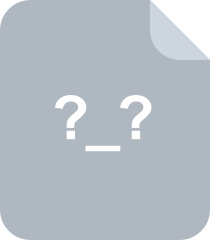
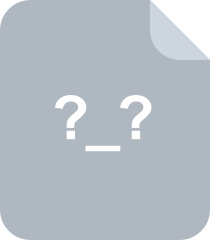
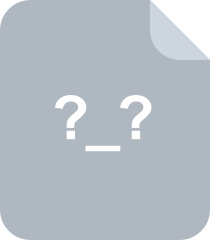
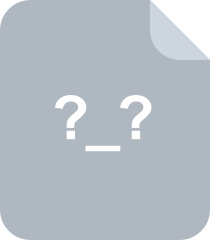
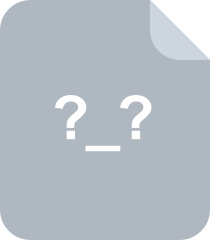
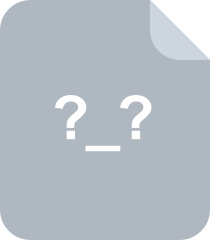
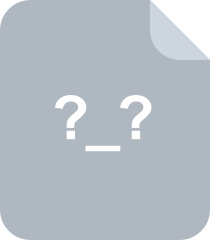
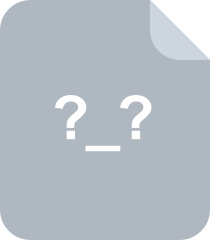
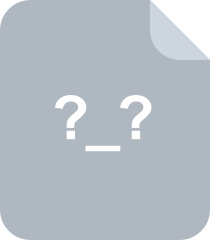
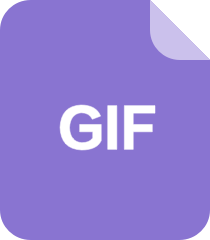
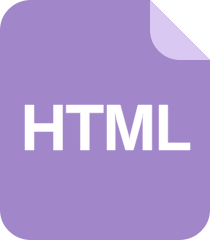
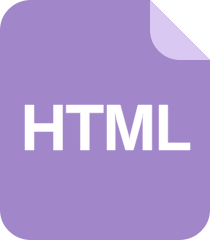
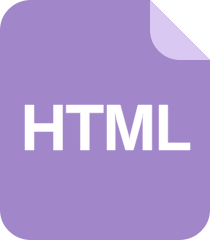
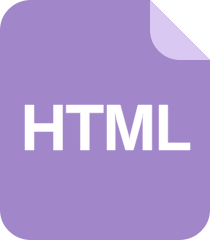
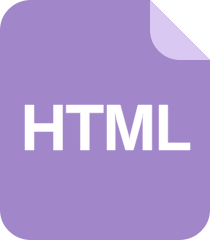
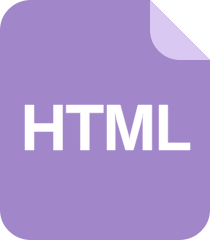
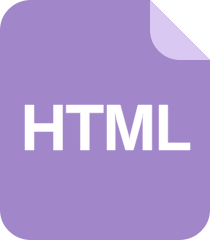
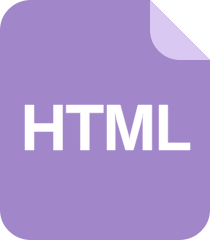
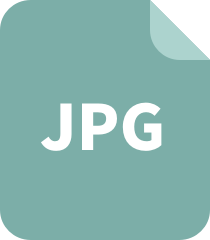
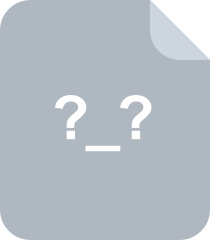
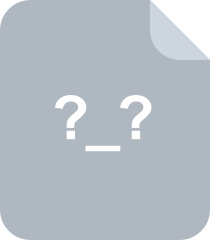
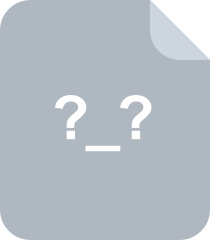
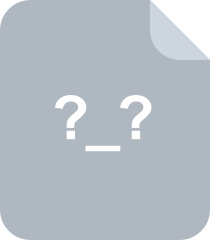
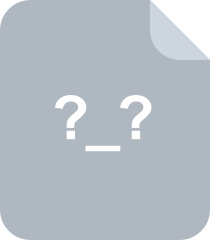
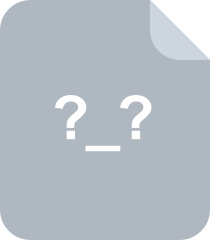
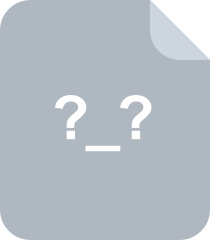
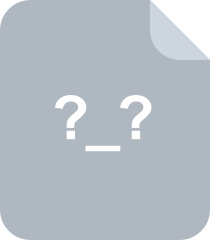
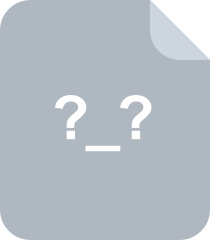
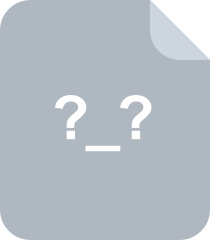
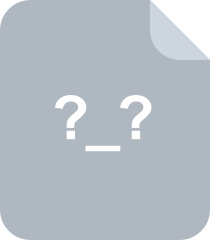
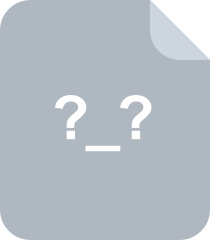
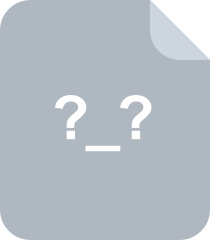
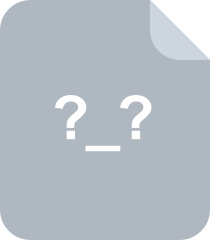
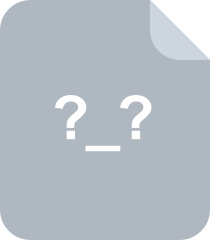
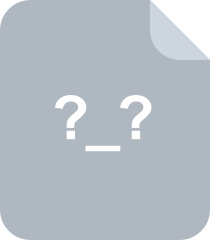
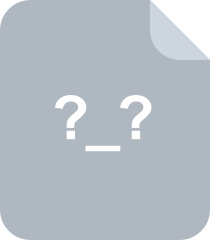
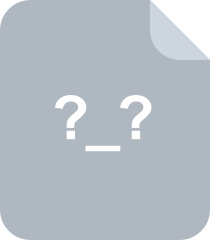
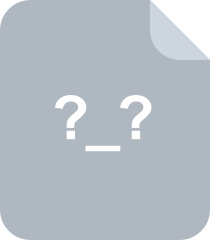
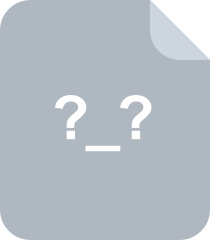
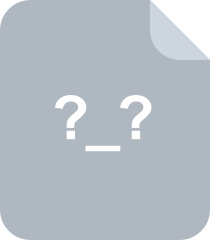
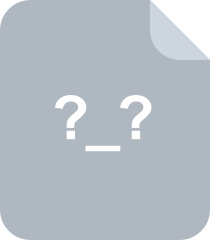
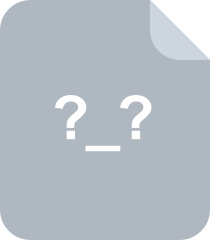
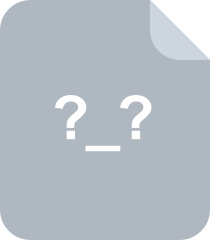
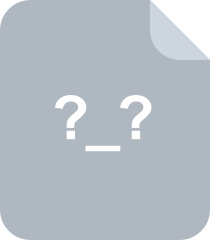
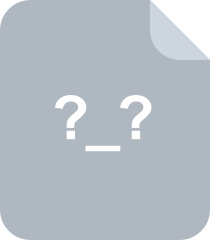
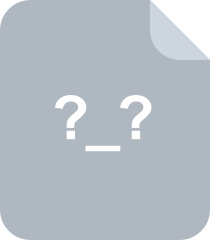
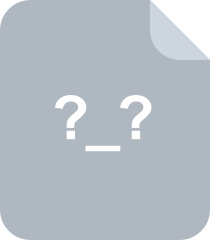
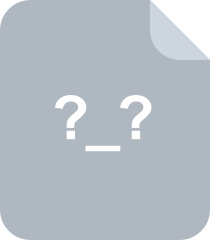
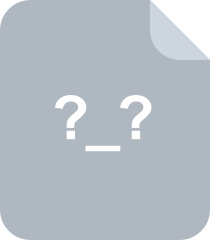
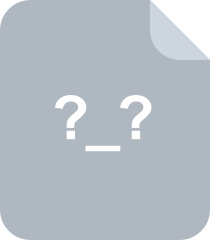
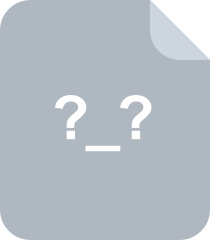
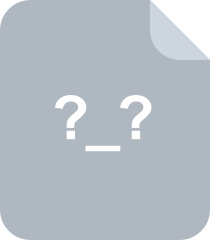
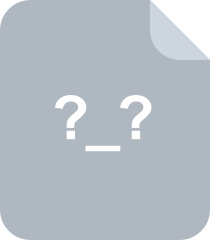
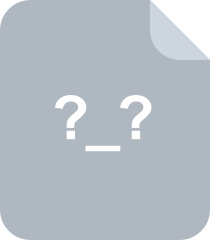
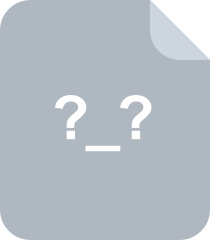
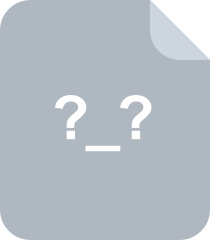
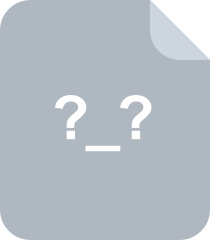
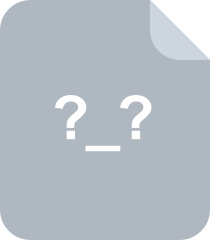
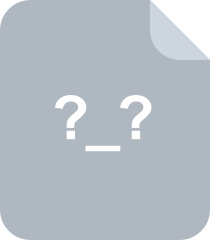
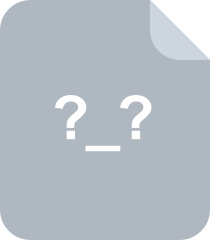
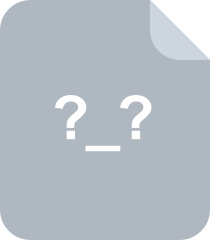
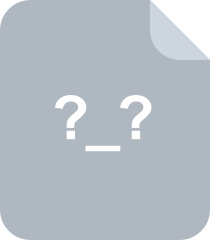
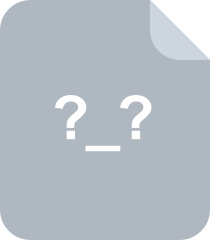
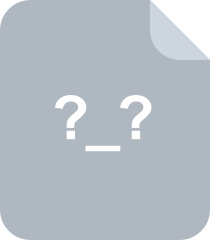
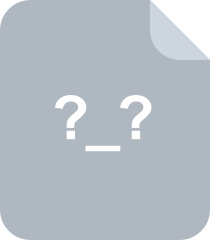
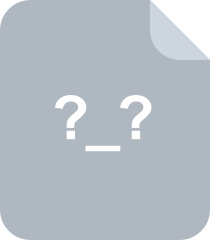
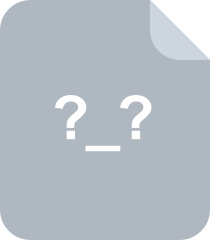
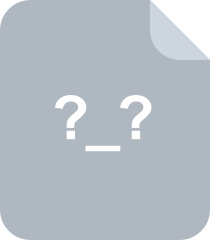
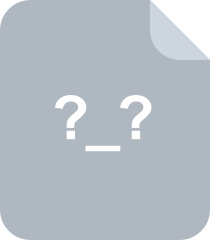
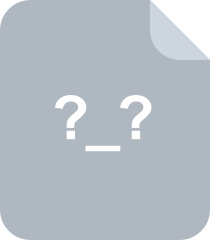
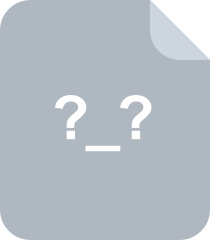
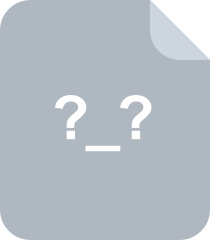
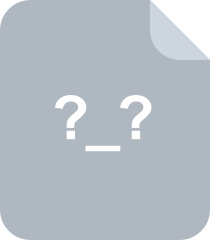
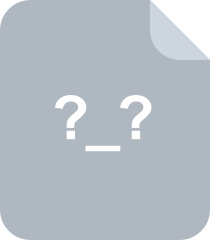
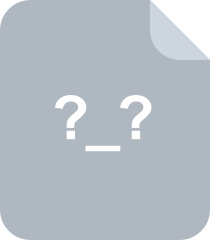
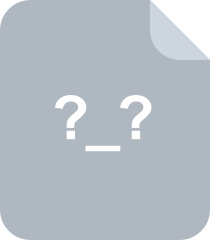
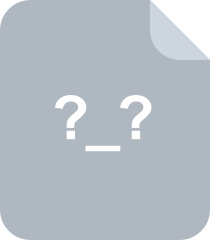
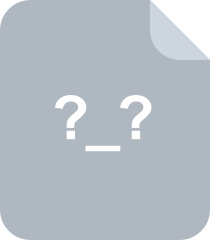
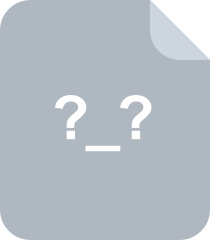
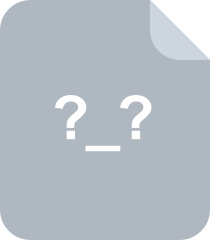
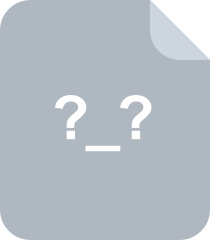
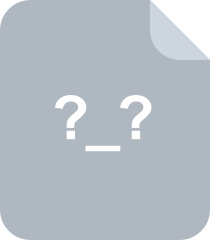
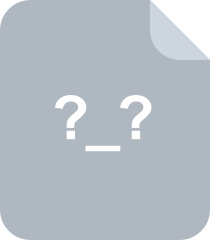
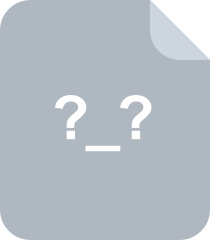
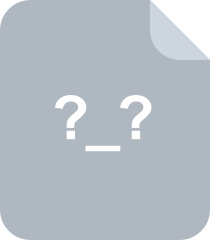
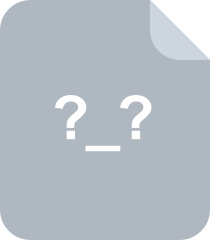
共 421 条
- 1
- 2
- 3
- 4
- 5
资源评论
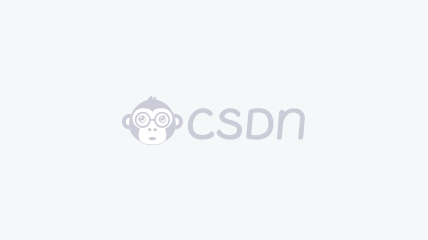

yxkfw
- 粉丝: 82
- 资源: 2万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

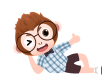
最新资源
- 几何物体检测44-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 几何物体检测43-YOLO(v5至v9)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 基于cruise的燃料电池功率跟随仿真,按照丰田氢能源车型搭建,在wltc工况下跟随效果好,最高车速175,最大爬坡30,百公里9s均已实现 1.模型通过cruise simulink联合仿真,策略
- C#源码 上位机 联合Visionpro 通用框架开发源码,已应用于多个项目,整套设备程序,可以根据需求编出来,具体Vpp功能自己编 程序包含功能 1.自动设置界面窗体个数及分布 2.照方式以命令触
- 程序名称:悬架设计计算程序 开发平台:基于matlab平台 计算内容:悬架偏频刚度挠度;螺旋弹簧,多片簧,少片簧,稳定杆,减震器的匹配计算;悬架垂向纵向侧向力学、纵倾、侧倾校核等;独立悬架杠杆比,等效
- 华为OD+真题及解析+智能驾驶
- jQuery信息提示插件
- 基于stm32的通信系统,sim800c与服务器通信,无线通信监测,远程定位,服务器通信系统,gps,sim800c,心率,温度,stm32 由STM32F103ZET6单片机核心板电路、DS18B2
- 充电器检测9-YOLO(v5至v11)、COCO、Create充电器检测9L、Paligemma、TFRecord、VOC数据集合集.rar
- 华为OD+考试真题+实现过程
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


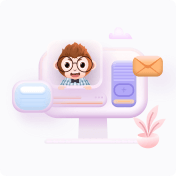
安全验证
文档复制为VIP权益,开通VIP直接复制
