package com.xdf.exams.web.action;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Set;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionForward;
import org.apache.struts.action.ActionMapping;
import com.xdf.exams.bean.Options;
import com.xdf.exams.bean.Question;
import com.xdf.exams.bean.Subject;
import com.xdf.exams.bo.BOFactory;
import com.xdf.exams.bo.IQuestionService;
import com.xdf.exams.util.Constant;
import com.xdf.exams.util.PageUtil;
import com.xdf.exams.util.Tools;
import com.xdf.exams.web.form.QuestionForm;
import com.xdf.exams.web.form.SubjectForm;
/**
* MyEclipse Struts
* Creation date: 04-05-2007
*
* XDoclet definition:
* @struts.action validate="true"
*/
public class SubjectAction extends BaseDispatchAction {
/**
* Method execute
* @param mapping
* @param form
* @param request
* @param response
* @return ActionForward
*/
public ActionForward show(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
IQuestionService ser = BOFactory.getQuestionService();
String spageno = request.getParameter("pageno");
PageUtil pu = new PageUtil(spageno,ser.findAllSubjectsnum(),Constant.PAGESIZE);
List list = ser.findAllSubjects(pu.getPageno(),pu.getPagesize());
request.setAttribute("subjectlist",list);
request.setAttribute("pageutil",pu);
return mapping.findForward("show");
}
public ActionForward add(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
this.saveToken(request);
SubjectForm sf = (SubjectForm)form;
return mapping.findForward("add");
}
public ActionForward adddo(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
SubjectForm sf = (SubjectForm)form;
ActionForward af = null;
if(this.isTokenValid(request)) {
IQuestionService ser = BOFactory.getQuestionService();
Subject s = new Subject();
s.setIntro(sf.getIntro());
s.setName(sf.getName());
s.setSdate(Tools.d2sshort(new Date()));
s.setState(sf.getState());
s.setTime(new Long(sf.getTime()));
try {
ser.addSubject(s);
request.setAttribute("message","增加科目成功");
af = mapping.findForward("show");
this.resetToken(request);
} catch (RuntimeException e) {
e.printStackTrace();
request.setAttribute("message","增加科目失败");
af = mapping.findForward("add");
}
}else {
request.setAttribute("message","请不要重复刷新");
af = mapping.findForward("show");
}
return af;
}
public ActionForward delete(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
IQuestionService ser = BOFactory.getQuestionService();
SubjectForm sf = (SubjectForm)form;
try {
Subject s = ser.findSubject(sf.getSubjectid());
ser.deleteSubject(s);
request.setAttribute("message","删除成功");
} catch (RuntimeException e) {
e.printStackTrace();
request.setAttribute("message","删除失败");
}
return mapping.findForward("show");
}
public ActionForward update(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
IQuestionService ser = BOFactory.getQuestionService();
SubjectForm sf = (SubjectForm)form;
try {
Subject s = ser.findSubject(sf.getSubjectid());
sf.setName(s.getName());
sf.setIntro(s.getIntro());
sf.setState(s.getState());
sf.setTime(s.getTime().intValue());
} catch (RuntimeException e) {
e.printStackTrace();
request.setAttribute("message","查询失败");
}
return mapping.findForward("update");
}
public ActionForward updatedo(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
IQuestionService ser = BOFactory.getQuestionService();
SubjectForm sf = (SubjectForm)form;
try {
Subject s = ser.findSubject(sf.getSubjectid());
s.setName(sf.getName());
s.setIntro(sf.getIntro());
s.setState(sf.getState());
s.setTime(new Long(sf.getTime()));
ser.updateSubject(s);
request.setAttribute("message","修改成功");
} catch (RuntimeException e) {
e.printStackTrace();
request.setAttribute("message","修改失败");
}
return mapping.findForward("show");
}
public ActionForward showquestion(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
IQuestionService ser = BOFactory.getQuestionService();
QuestionForm sf = (QuestionForm)form;
String spageno = request.getParameter("pageno");
try {
Subject s = ser.findSubject(sf.getSubjectid());
int num = ser.findQuestionnumBySubjects(sf.getSubjectid());
PageUtil pu = new PageUtil(spageno,num,Constant.PAGESIZE);
List list = ser.findQuestionBySubjects(sf.getSubjectid(),pu.getPageno(),pu.getPagesize());
request.setAttribute("questionlist",list);
request.setAttribute("pageutil",pu);
request.setAttribute("subject",s);
} catch (RuntimeException e) {
e.printStackTrace();
request.setAttribute("message","查询失败");
}
return mapping.findForward("show");
}
public ActionForward addquestion(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
QuestionForm qf= (QuestionForm)form;
IQuestionService ser = BOFactory.getQuestionService();
request.setAttribute("subject",ser.findSubject(qf.getSubjectid()));
this.saveToken(request);
return mapping.findForward("add");
}
public ActionForward addquestiondo(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
ActionForward af = null;
if(this.isTokenValid(request)) {
try {
IQuestionService ser = BOFactory.getQuestionService();
QuestionForm qf= (QuestionForm)form;
Question que = new Question();
que.setContent(qf.getContent());
que.setQtype(qf.getQtype());
String rans = "";
if(qf.getRightanswers()!=null)
for (int i=0;i<qf.getRightanswers().length;i++) {
rans += qf.getRightanswers()[i];
}
que.setRightanswer(rans);
que.setScore(qf.getScore());
que.setSdate(Tools.d2sshort(new Date()));
que.setSubject(ser.findSubject(qf.getSubjectid()));
List oplist = new ArrayList();
if(qf.getOptions()!=null)
for (int i=0;i<qf.getOptions().length;i++) {
if(qf.getOptions()[i]!=null&&!qf.getOptions()[i].equals("")) {
Options ops = new Options();
ops.setContent(qf.getOptions()[i]);
ops.setQuestion(que);
oplist.add(ops);
}
}
ser.addQuestion(que,oplist);
request.setAttribute("message","增加试题成功");
af = mapping.findForward("show");
this.resetToken(request);
} catch (RuntimeException e) {
e.printStackTrace();
request.setAttribute("message","增加试题失败");
af = mapping.findForward("add");
}
}else {
request.setAttribute("message","不要重复提交");
af = mapping.findForward("show");
}
return af;
}
public ActionForward updatequestion(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
QuestionForm qf= (QuestionForm)form;
IQuestionService ser = BOFactory.getQuestionService();
Question que = ser.findQuestion(qf.getQuestionid());
qf.setSubjectid(qf.getSubjectid());
qf.setQuestionid(que.getQuestionid());
qf.setContent(que.getContent());
qf.setQtype(que.getQtype());
qf.setScore(que.getScore());
qf.setRightanswers(Tools.tostringarray(que.getRightanswer()));
Set s = que.getOptionses();
String[] os = new String[8];
int i=0;
for (Object o:s) {
Options opt = (Options)o;
os[i]

yxkfw
- 粉丝: 82
- 资源: 2万+
最新资源
- 资源名称不得少于十一字
- PXI 429总线卡 航空总线卡 底板板+功能子卡结构 底板原理图+PCB 子卡原理图+PCB FPGA源代码(EP3C40F484) 如需要,详谈
- JDWT01 220 台,高精度称重模块,rs485通讯,支持多种通讯格式,采用g24位ad7190转模块,内码高达1000w,精度可达2w分之一 提供海为pLc标定程序 JDWT01-A 160
- 重庆大学Python课程试题解析与核心知识点汇总
- MATLAB代码:基于两阶段鲁棒优化算法的微网电源容量优化配置 关键词:容量优化配置 微网 两阶段鲁棒规划 仿真平台:MATLAB YALMIP+CPLEX 主要内容:代码主要做的是一个微网中电源
- jmeter学习笔记,基础知识,实用
- 正弦余弦指引的乌鸦搜索算法Matlab代码 1肖子雅,刘升,韩斐斐于建芳正弦余弦指引的乌鸦搜索算法研究J.计算机工程与应用,2019,55(21):52-59. 乌鸦搜索算法模拟乌鸦觅食行为对
- SVPWM算法的simulink实现 有两种,分别是只simulink的和基于s-fun函数的,附代码 默认matlab2018b
- 西门子PLC程序大型项目,siemens博途V16 V17版,配方处理程序,多个昆仑通态触摸屏配方,ScL语言,485通讯控制变频器,模拟量压力处理,多个1200cpu处理,称重数据读取
- electron rtmp桌面推流客户端
- 一个使用 JavaScript 结合 HTML 和 CSS 创建一个出租车计价器界面及实现计价功能的源码
- 串口调试助手软件,socket通信调试助手软件 很好用的两个助手软件功能强大,侦测,拦截,逆向分析串口通汛协议,是RS232 422 485串行端口的专业工貝软件
- dfajkfghdjk s12m code
- 微电网 孤岛 孤岛并网切 并网 三种模式 考虑风光储 储能环节可以根据孤岛并网模式在PQ 和VF模式两环节切 考虑蓄电池和超级电容混合储能 超级电容提供高频分量 附各个环节讲解说明 模型可塑
- 两级式光伏并网逆变器,DCDC环节采用boost电路,通过增量电导法实现光伏最大功率跟踪MPPT 逆变器采用二电平逆变器,通过双闭环控制,实现并网单位功率因数,并网电流与电网电压同相位,并网电流TH
- MATLAB simiulink永磁直驱风力发电系统, 机侧网侧均使用为SVPWM矢量控制算法,参数调节均已完成,直接运行即可,可供初学者学习 (1)采用 SVPWM 矢量控制; (2)采用转速、电
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


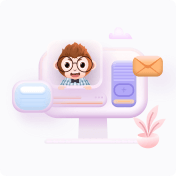