package test.service;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
import test.util.DbConnectionService;
import test.vo.BookInfo;
import test.vo.CatagoryInfo;
public class BookServiceImpl implements IBookService {
private Connection connection;
private Statement statement;
private ResultSet resultSet;
public BookServiceImpl() {
connection = DbConnectionService.getConnection();
try {
statement = connection.createStatement();
} catch (SQLException e) {
e.printStackTrace();
}
}
public Boolean addBookInfo(BookInfo bookInfo) {
Boolean boolean1 = null;
String sql = "insert into book(crono,bname,author,pdate,publisher,remark,price) values("
+ bookInfo.getCrono()
+ ",'"
+ bookInfo.getBname()
+ "','"
+ bookInfo.getAuthor()
+ "','"
+ bookInfo.getPdate()
+ "','"
+ bookInfo.getPublisher()
+ "','"
+ bookInfo.getRemark()
+ "',"
+ bookInfo.getPrice() + ")";
try {
boolean1 = statement.execute(sql);
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return boolean1;
}
public List<BookInfo> getBookListInfo() {
List<BookInfo> list = new ArrayList<BookInfo>();
String sql = "select * from book";
try {
resultSet = statement.executeQuery(sql);
while (resultSet.next()) {
list.add(new BookInfo(resultSet.getInt(1), resultSet.getInt(2),
resultSet.getString(3), resultSet.getString(4),
resultSet.getString(5), resultSet.getString(6),
resultSet.getString(7), resultSet.getFloat(8)));
}
} catch (SQLException e) {
e.printStackTrace();
}
return list;
}
public List<BookInfo> searchBooks(String bookname) {
List<BookInfo> list = new ArrayList<BookInfo>();
String sql = "select * from book where bname like '%" + bookname + "%'";
try {
resultSet = statement.executeQuery(sql);
while (resultSet.next()) {
list.add(new BookInfo(resultSet.getInt(1), resultSet.getInt(2),
resultSet.getString(3), resultSet.getString(4),
resultSet.getString(5), resultSet.getString(6),
resultSet.getString(7), resultSet.getFloat(8)));
}
} catch (SQLException e) {
e.printStackTrace();
}
return list;
}
public Boolean delBookInfo(int bookno) {
Boolean result = null;
String sql = "delete from book where bookno=" + bookno;
try {
result = statement.execute(sql);
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return result;
}
public Boolean updateBookInfo(BookInfo bookInfo) {
Boolean result = null;
String sql = "update bookinfo set crono='" + bookInfo.getCrono()
+ "', bname='" + bookInfo.getBname() + "',author='"
+ bookInfo.getAuthor() + "',pdate='" + bookInfo.getPdate()
+ "',publisher='" + bookInfo.getPublisher() + "',remark='"
+ bookInfo.getRemark() + "',price =" + bookInfo.getPrice();
try {
result = statement.execute(sql);
} catch (SQLException e) {
e.printStackTrace();
}
return result;
}
public BookInfo searchBooks(int bookno) {
return null;
}
public BookInfo getOneBookBybookID(int bookno) {
BookInfo bookInfo = null;
String sql = "select * from book where bookno=" + bookno;
try {
resultSet = statement.executeQuery(sql);
while (resultSet.next()) {
bookInfo = new BookInfo(resultSet.getInt(1), resultSet
.getInt(2), resultSet.getString(3), resultSet
.getString(4), resultSet.getString(5), resultSet
.getString(6), resultSet.getString(7), resultSet
.getFloat(8));
}
} catch (SQLException e) {
e.printStackTrace();
}
return bookInfo;
}
public List<BookInfo> showCatagoryBook(int crono) {
List<BookInfo> list = new ArrayList<BookInfo>();
String sql = "select * from book where crono=" + crono;
try {
resultSet = statement.executeQuery(sql);
while (resultSet.next()) {
list.add(new BookInfo(resultSet.getInt(1), resultSet.getInt(2),
resultSet.getString(3), resultSet.getString(4),
resultSet.getString(5), resultSet.getString(6),
resultSet.getString(7), resultSet.getFloat(8)));
}
} catch (SQLException e) {
e.printStackTrace();
}
return list;
}
}

yxkfw
- 粉丝: 81
- 资源: 2万+
最新资源
- Chrome代理 switchyOmega
- GVC-全球价值链参与地位指数,基于ICIO表,(Wang等 2017a)计算方法
- 易语言ADS指纹浏览器管理工具
- 易语言奇易模块5.3.6
- cad定制家具平面图工具-(FG)门板覆盖柜体
- asp.net 原生js代码及HTML实现多文件分片上传功能(自定义上传文件大小、文件上传类型)
- whl@pip install pyaudio ERROR: Failed building wheel for pyaudio
- Constantsfd密钥和权限集合.kt
- 基于Java的财务报销管理系统后端开发源码
- 基于Python核心技术的cola项目设计源码介绍
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


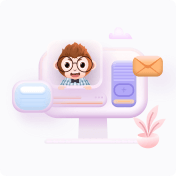