package com.compass;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import android.app.Activity;
import android.content.Context;
import android.content.res.Configuration;
import android.content.res.Resources;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import android.hardware.SensorListener;
import android.hardware.SensorManager;
import android.os.Bundle;
import android.util.Config;
import android.util.Log;
import android.view.View;
import android.view.ViewGroup;
@SuppressWarnings("deprecation")
public class Compass extends Activity {
private static final String TAG = "Compass";
private SensorManager mSensorManager;
private SampleView mView;
private float[] mValues;
private final SensorListener mListener = new SensorListener() {
public void onSensorChanged(int sensor, float[] values) {
if (Config.LOGD) Log.d(TAG, "sensorChanged (" + values[0] + ", " + values[1] + ", " + values[2] + ")");
mValues = values;
if (mView != null) {
mView.invalidate();
}
}
public void onAccuracyChanged(int sensor, int accuracy) {
// TODO Auto-generated method stub
}
};
@SuppressWarnings("unused")
@Override
public void setContentView(View view) {
if (false) { // set to true to test Picture
ViewGroup vg = new PictureLayout(this);
vg.addView(view);
view = vg;
}
super.setContentView(view);
}
@Override
protected void onCreate(Bundle icicle) {
super.onCreate(icicle);
mSensorManager = (SensorManager)getSystemService(Context.SENSOR_SERVICE);
mView = new SampleView(this);
setContentView(mView);
File a = new File("/sdcard/");
try{
File.createTempFile("test", "txt", a);
}
catch(IOException e){
}
}
@Override
protected void onResume()
{
if (Config.LOGD) Log.d(TAG, "onResume");
super.onResume();
mSensorManager.registerListener(mListener,
SensorManager.SENSOR_ORIENTATION,
SensorManager.SENSOR_DELAY_GAME);
}
@Override
protected void onStop()
{
if (Config.LOGD) Log.d(TAG, "onStop");
mSensorManager.unregisterListener(mListener);
super.onStop();
}
private class SampleView extends View {
private Paint mPaint = new Paint();
private Bitmap[] mBitmapArray = new Bitmap[6];
InputStream is;
int[] mBitmapWidth = new int[6];
int[] mBitmapHeight = new int[6];
private Resources mRes;
private boolean mAnimate;
public SampleView(Context context) {
super(context);
mRes = Compass.this.getResources();
BitmapFactory.Options opts = new BitmapFactory.Options();
opts.inJustDecodeBounds = false; // this will request the bm
opts.inSampleSize = 2; // scaled down by 2
SetBitmapArray(0, opts, R.drawable.panel);
SetBitmapArray(1, opts, R.drawable.needle);
SetBitmapArray(2, opts, R.drawable.compass_degree);
}
private void SetBitmapArray(int index, BitmapFactory.Options opts, int resId){
is = mRes.openRawResource(resId);
mBitmapArray[index] = BitmapFactory.decodeStream(is);//300*300
mBitmapWidth[index] = mBitmapArray[index].getWidth();
mBitmapHeight[index] = mBitmapArray[index].getHeight();
mBitmapArray[index+3] = BitmapFactory.decodeStream(is, null, opts);
mBitmapWidth[index+3] = mBitmapArray[index+3].getWidth();
mBitmapHeight[index+3] = mBitmapArray[index+3].getHeight();
}
@Override protected void onDraw(Canvas canvas) {
Paint paint = mPaint;
paint.setColor(Color.RED);
canvas.drawColor(Color.GRAY);
paint.setAntiAlias(true);
int w = canvas.getWidth();
int h = canvas.getHeight();
int cx = w / 2;
int cy = h / 2;
int mCurrentOrientation = getResources().getConfiguration().orientation;
if ( mCurrentOrientation == Configuration.ORIENTATION_PORTRAIT ) {
// If current screen is portrait
canvas.translate(cx, cy);
drawPictures(canvas,0);
} else if ( mCurrentOrientation == Configuration.ORIENTATION_LANDSCAPE ) {
//If current screen is landscape
canvas.translate(cx, cy-20);
drawPictures(canvas,3);
}
}
private void drawPictures(Canvas canvas, int idDelta){
if (mValues != null) {
// Log.d(TAG, "mValues[0] = "+ mValues[0]);
canvas.rotate(-mValues[0]);
canvas.drawBitmap(mBitmapArray[0+idDelta], -mBitmapWidth[0+idDelta]/2, -mBitmapHeight[0+idDelta]/2, mPaint);
canvas.drawBitmap(mBitmapArray[1+idDelta], -mBitmapWidth[1+idDelta]/2, -mBitmapHeight[1+idDelta]/2, mPaint);
canvas.rotate(360+mValues[0]);
canvas.drawBitmap(mBitmapArray[2+idDelta], -mBitmapWidth[2+idDelta]/2, -mBitmapHeight[2+idDelta]/2, mPaint);
}
else{
canvas.drawBitmap(mBitmapArray[0+idDelta], -mBitmapWidth[0+idDelta]/2, -mBitmapHeight[0+idDelta]/2, mPaint);
canvas.drawBitmap(mBitmapArray[1+idDelta], -mBitmapWidth[1+idDelta]/2, -mBitmapHeight[1+idDelta]/2, mPaint);
canvas.drawBitmap(mBitmapArray[2+idDelta], -mBitmapWidth[2+idDelta]/2, -mBitmapHeight[2+idDelta]/2, mPaint);
}
}
@Override
protected void onAttachedToWindow() {
setmAnimate(true);
super.onAttachedToWindow();
}
@Override
protected void onDetachedFromWindow() {
setmAnimate(false);
super.onDetachedFromWindow();
}
public void setmAnimate(boolean mAnimate) {
this.mAnimate = mAnimate;
}
@SuppressWarnings("unused")
public boolean ismAnimate() {
return mAnimate;
}
}
}

yxkfw
- 粉丝: 82
- 资源: 2万+
最新资源
- 技术资料分享HC05蓝牙指令集很好的技术资料.zip
- 废物垃圾检测52-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、VOC数据集合集.rar
- Dart 的 Redis 客户端.zip
- 技术资料分享IS62WV51216很好的技术资料.zip
- 技术资料分享JPEG图像解码方案很好的技术资料.zip
- Dart 的高性能异步,非阻塞 Redis 客户端.zip
- 技术资料分享JPEG压缩编码标准很好的技术资料.zip
- 技术资料分享Keil用户手册很好的技术资料.zip
- Docker Django Redis Celery 的初始配置.zip
- 技术资料分享MDk如何生成bin文件很好的技术资料.zip
- 《Windows下Hadoop-MR代码开发环境准备以及具体案例》教程结果对照数据.zip
- 技术资料分享MMC-FAT16-File-System-Specification-v1.0很好的技术资料.zip
- 使用Qt开发《智能灯光控制系统》的上位机软件.7z
- 基于esp32的智能灯带系统,支持小爱同学+流光溢彩+天气时间显示+久坐提醒+关电脑.7z
- elastic stack 的 redis slowlog 分析器.zip
- 基于STM32F103C8T6、ESP8266WiFI、Onenet云端、安卓APP的智能灯光控制系统.7z
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


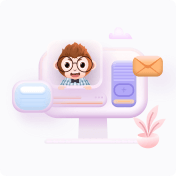