package com.kerence.mine.mineGUI;
import java.awt.Color;
import java.awt.GridLayout;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import javax.swing.BorderFactory;
import javax.swing.JPanel;
import javax.swing.border.BevelBorder;
import javax.swing.border.Border;
import javax.swing.border.EmptyBorder;
import com.kerence.mine.data_structure.MineMatrix;
import com.kerence.mine.mine_model.Strategy.MineMatrixSettable;
import com.kerence.mine.res.image.ImageIconFactory;
/**
* 扫雷矩阵面板
*
* @author Kerence
*
*/
public class JMineMatrixPanel extends JPanel
{
private JMineSweeperFrame mineSweeperFrame;
/**
* 得到一个JMineSweeperFrame的引用,这样才能对statusPanel进行操作
*
* @param mineSweeperFrame
* 一个JMineSweeperFrame对象的引用
*/
public void addMineSweeperFrame(JMineSweeperFrame mineSweeperFrame)
{
this.mineSweeperFrame = mineSweeperFrame;
}
/**
* 雷块鼠标监听器
*
* @author Kerence
*
*/
private class BlockMouseListener extends MouseAdapter
{
/**
* 鼠标移入时进行的操作
*/
@Override
public void mouseEntered(MouseEvent e)
{
currentMineBlock = ((JMineBlock) e.getSource());
int row = currentMineBlock.getRow();
int column = currentMineBlock.getColumn();
if (button1Pressed && button3Pressed)
{
setAutoProbeIcons(row, column);
} else
{
if (button1Pressed)
{// 在鼠标左键按下的条件下
if (isFlagged(row, column))
{
return;
}
if (isDigged(row, column))
{
return;
} else
{// 若没被挖则 显示空白
currentMineBlock.setBlankPressed();
return;
}
}
}
}
/**
* 鼠标移出时进行的操作
*/
@Override
public void mouseExited(MouseEvent e)
{
if (button3Pressed && button1Pressed)
{// 两键同时被按下的情况
// 显示出原图
if (currentMineBlock != null)
{
int row = currentMineBlock.getRow();
int column = currentMineBlock.getColumn();
revertAutoProbeIcons(row, column);
}
currentMineBlock = null;
return;
}
if (button3Pressed)
{// 右键被按下
currentMineBlock = null;
} else
{// 右键未被按下
int row = currentMineBlock.getRow();
int column = currentMineBlock.getColumn();
if (button1Pressed)
{// 左键按下 显示原图
if (isFlagged(row, column))
{
} else if (!isDigged(row, column))
{
if (isRabotOn() && isMine(row, column))
{
currentMineBlock.setDot();
} else
{
currentMineBlock.setBlank();
}
}
currentMineBlock = null;
return;
}
if (isDigged(row, column))
{
currentMineBlock = null;
return;
} else
{
refreshMineMatrixPanel();
}
}
currentMineBlock = null;
}
/**
* 鼠标单击时进行的操作
*/
@Override
public void mousePressed(MouseEvent e)
{
// 鼠标的操作有哪些 特别是鼠标按下的操作
if (e.getButton() == MouseEvent.BUTTON1)
{
button1Pressed = true;
} else if (e.getButton() == MouseEvent.BUTTON3)
{
button3Pressed = true;
}
// 得到被按下的块的行和列
int row = currentMineBlock.getRow();
int column = currentMineBlock.getColumn();
// 左右键都按下,则探测
if (button1Pressed && button3Pressed)
{// 两键都被按下
mineSweeperFrame.leftButtonPressedOnMineBlock();
// TODO auto probe
setAutoProbeIcons(row, column);
// refreshMineMatrixPanel();
} else if (button1Pressed && !button3Pressed)
{// 左键按下,右键未按下
mineSweeperFrame.leftButtonPressedOnMineBlock();
if (isDigged(row, column))
{
return;
} else if (isFlagged(row, column))
{// 被置旗则也不变
return;
}
jMineMatrix[row][column].setBlankPressed();
} else if (button3Pressed && !button1Pressed)
{// 右键被按下 左键未按下
// 若被挖则无反应
if (isDigged(row, column))
{
return;
}
// 未被挖则要判断是否被置旗 是否被置标记
if (isFlagged(row, column))
{// 若已放旗
if (isMarkable())
{// 可标记
setUnFlagged(row, column);// 先取消旗
setMarked(row, column);// 设为标记
} else
{// 不可标记
setUnFlagged(row, column);// 取消旗
}
} else
{// 若未放旗
if (isMarked(row, column))
{// 若被标记
setUnMarked(row, column);// 设置为未标记
} else
{
setFlagged(row, column);
}
}
mineSweeperFrame.setLEDMineCountLeft(mineMatrix.getMineCount() - mineMatrix.getFlagCount());
refreshMineMatrixPanel();
return;
}
}
/**
* 鼠标释放时进行的操作
*/
@Override
public void mouseReleased(MouseEvent e)
{// 它的源是鼠标按下时的组件。
boolean button1Released = false, button3Released = false;
if (e.getButton() == MouseEvent.BUTTON1)
{
button1Pressed = false;
button1Released = true;
} else if (e.getButton() == MouseEvent.BUTTON3)
{
button3Pressed = false;
button3Released = true;
}
// 鼠标的操作有哪些 特别是鼠标放开的操作
// 左键放开时
// 右键按下
// 这时要进行的操作是自动探测并挖雷 刷新一次界面
// 鼠标释放事件汇总 左键放开 右键放开。
if ((button1Released && button3Pressed)// 就是进行探测了。
|| (button1Pressed && button3Released))
{// 右键按下时 左键放开
// 或者左键按下时右键放开
if (currentMineBlock == null)
{
return;
}
int row = currentMineBlock.getRow();
int column = currentMineBlock.getColumn();
mineSweeperFrame.leftButtonReleasedOnMineBlock();// 对状态栏进行的操作
// 恢复图形显示
revertAutoProbeIcons(row, column);
if (!isDigged(row, column))
{// 如果没被挖过就不能自动探测
return;
}
mineMatrix.autoDetectMine(currentMineBlock.getRow(), currentMineBlock.getColumn());
if (mineMatrix.isGameOver())
{
// 找到所有雷并
// mineMatrix.gameOver();// 进行gameOver的雷阵设置
gameTerminates();
button3Pressed = false;
button1Pressed = false;
mineSweeperFrame.gameOver();
} else if (mineMatrix.isGameComplete())
{
mineMatrix.gameComplete();
gameTerminates();
button3Pressed = false;
button1Pressed = false;
mineSweeperFrame.gameComplete();
}
refreshMineMatrixPanel();// 刷新图形显示
return;
}
// 左键放开
// 挖雷 若已被挖开则不挖 刷新一次界面
else if (button1Released && !button3Pressed)
{// 右键未按下时左键放开挖雷
mineSweeperFrame.leftButtonReleasedOnMineBlock();
if (currentMineBlock == null)
{
return;
}
int row = currentMineBlock.getRow();
int column = currentMineBlock.getColumn();
if (mineMatrix.isDigged(row, column))
{// 判断是否已经被挖开
return;
} else
{// 没挖开就挖
// 还要判断是否是第一挖
if (mineMatrix.isFlagged(row, column))
{// 要先判断是否放旗。
// 是旗则不挖
return;
}
if (!mineMatrix.hasGameCommenced())
{// 如果未开局
generateRandomMatrix(row, column);// 并生成雷阵列。
mineMatrix.setGameCommenced(true);// 设置成开局
activateStatusPanel();// 通知主窗口游戏开始。只是对状态栏进行设置而已
// 而且这里的命名好像不好吧。应该换成activatestatuspanel
}
mineMatrix.digBlock(row, column);// 挖雷包括自动挖开周围的雷
// 如果是雷则自动可以挖开所有的雷。
// 判断是否挖的是雷
if (isMine(row, column))
{// 如果挖的是雷则释放所有监听器
mineMatrix.setGameOver(true);
gameTerminates(); // 释放所有雷区按钮的监听器
mineSweeperFrame.gameOver();// 通知扫雷框架对状态进行设置
} else if (mineMatrix.isGameComplete())
{
gameTerminates();
mineMatrix.gameComplete();
mineSweeperFrame.gameComplete();

yxkfw
- 粉丝: 82
- 资源: 2万+
最新资源
- 汇川H5U EtherCat总线伺服与HMI程序实战教程:轴控与气缸控制功能块详解,汇川H5U EtherCat总线伺服与HMI程序实战教程:轴控与气缸控制功能块详解,汇川H5U走EtherCat控制
- Simulink模型下电动汽车的各模块详细构建说明与功能文档:包括驾驶员、整车控制器、电机等模块,电动汽车模型的Simulink模块化设计与功能详解:涵盖驾驶员、整车控制、电机等八大模块及详细建模说明
- 西门子S1200 PID恒温恒压供冷却水系统-霍尼韦尔电动比例阀PID控制水温与变频器PID控制水压解决方案(附程序和Eplan源档图纸),西门子S1200 PID恒温恒压供冷却水系统:霍尼韦尔电动
- Comsol等离子体模型:针尖电晕放电与氩气环境下的等离子体模拟及大气压下放电过程之美,针对Comsol等离子体模型:针尖电晕放电在氩气环境下的模拟与观察 大气压下针尖电晕放电的等离子体模型研究,C
- 基于Comsol的多物理场耦合分析:熊猫光纤应力传感与固体力学光学研究,Comsol光纤应力传感分析:融合固体力学与光学模块的多物理场耦合探究,Comsol熊猫光纤应力传感分析 固体力学和光学模块多
- Halcon与C#融合下的雷赛驱动四轴运动控制贴片机系统:高效便捷,注释详尽,加密保护,轻松上手,Halcon与C#贴片机:基于雷赛驱动卡的四轴运动控制,注释详尽,易于使用,加密算法保驾护航,Halc
- MATLAB代码详解:多能互补热电联供型微网优化策略实现与注释指南,MATLAB代码详解:多能互补热电联供型微网优化策略完美实现,详细注释助您轻松理解,MATLAB 代码:多能互补热电联供型微网优化
- 基于Simulink的永磁同步电机滑模控制器与滑模观测器研究及其拓展应用探索,Simulink内置永磁同步电机滑模控制器与观测器研究及拓展应用,Simulink 内置永磁同步电机滑模控制器,滑模观测器
- 基于S7-200 PLC全自动工业洗衣机控制系统详解:梯形图程序、接线图与IO配置,基于S7-200 PLC全自动工业洗衣机智能控制系统设计与实现:梯形图程序、接线图、IO分配及组态画面全解析,基于S
- 基于PLC模拟的城轨自动售票机控制系统-S7-200与MCGS操作解析、梯形图程序及组态设计,基于PLC模拟的城轨自动售票机S7-200控制:梯形图程序详解、接线与组态画面全解析,S7-200 MC
- 三菱PLC与MCGS触摸屏在自动分拣控制系统中的组合应用:程序梯形图、接线图与组态画面详解,三菱PLC与MCGS触摸屏在自动分拣控制系统中的组合应用:程序梯形图、接线图与组态画面解析,三菱PLC程序M
- 基于PLC的S7-200复杂交通灯控制系统详解:梯形图程序、接线图与组态画面全解析,基于PLC的S7-200交通灯精准控制系统:梯形图程序解析与全套设计图示,S7-200基于PLC的复杂路交通灯控制系
- 基于PLC的4路抢答器控制系统设计与实现:梯形图程序、接线图、IO分配及组态画面详解,基于PLC的4路抢答器控制系统设计与实现:梯形图程序、接线图、IO分配及组态画面详解,基于PLC的4路抢答器控制系
- 双容水箱液位PID控制设计:P、PI、PD与PID控制方法的Simulink仿真对比研究 - 包含全面说明文档,双容水箱液位PID控制设计:Matlab Simulink平台下的P、PI、PD、PID
- 基于多时间尺度滚动优化的多能源微网双层调度模型:两阶段协同优化求解框架及策略探索,基于多时间尺度滚动优化的多能源微网双层调度策略实现与模型求解,MATLAB代码:基于多时间尺度滚动优化的多能源微网双层
- 基于PLC的S7-200组态王游泳池水处理解决方案:涵盖梯形图程序、接线图与IO分配及组态画面整合,基于PLC的S7-200组态王游泳池水处理综合解决方案:梯形图程序、接线图与组态画面全解析,S7-2
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


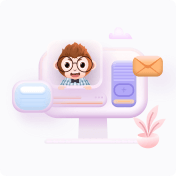