ListViewAnimations ([Play Store Demo][1])
===========
ListViewAnimations is an Open Source Android library that allows developers to easily create ListViews with animations.
Feel free to use it all you want in your Android apps provided that you cite this project and include the license in your app.
ListViewAnimations uses the [NineOldAndroids][2] library to support devices <3.0.
It also uses Roman Nurik's BETA [SwipeDismissListViewTouchListener][5] to support swipe to dismiss.
Known applications using ListViewAnimations
-----
* TreinVerkeer ([Play Store][6])
* Running Coach ([Play Store][9])
If you want your app to be listed as well please contact me via [Twitter][7] or [Google Plus][8]!
Setup
-----
* In Eclipse, just import the library as an Android library project.
* Project > Clean to generate the binaries you need, like R.java, etc.
* Then, just add ListViewAnimations as a dependency to your existing project and you're good to go!
Or:
* [Download the .jar file][4]
* Add the .jar to your project's `libs` folder.
Usage
-----
This library uses the [Decorator Pattern][3] to stack multiple `BaseAdapterDecorator`s on each other:
* Implement your own `BaseAdapter`, or reuse an existing one.
* Stack multiple `BaseAdapterDecorator`s on each other, with your `BaseAdapter` as a base.
* Set the `ListView` to your last `BaseAdapterDecorator`.
* Set your last `BaseAdapterDecorator` to the `ListView`.
Example:
-----
/* This example will stack two animations on top of eachother */
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
MyListAdapter mAdapter = new MyListAdapter(this, getItems());
SwingBottomInAnimationAdapter swingBottomInAnimationAdapter = new SwingBottomInAnimationAdapter(mAdapter);
SwingRightInAnimationAdapter swingRightInAnimationAdapter = new SwingRightInAnimationAdapter(swingBottomInAnimationAdapter);
// Or in short notation:
swingRightInAnimationAdapter =
new SwingRightInAnimationAdapter(
new SwingBottomInAnimationAdapter(
new MyListAdapter(this, getItems())));
// Assign the ListView to the AnimationAdapter and vice versa
swingRightInAnimationAdapter.setListView(getListView());
getListView().setAdapter(swingRightInAnimationAdapter);
}
private class MyListAdapter extends com.haarman.listviewanimations.ArrayAdapter<String> {
private Context mContext;
public MyListAdapter(Context context, ArrayList<String> items) {
super(items);
mContext = context;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
TextView tv = (TextView) convertView;
if (tv == null) {
tv = (TextView) LayoutInflater.from(mContext).inflate(R.layout.list_row, parent, false);
}
tv.setText(getItem(position));
return tv;
}
}
Custom AnimationAdapters
-----
Instead of using the ready-made adapters in the `.swinginadapters.prepared` package, you can also implement your own `AnimationAdapter`.
Implement one of the following classes:
* `ResourceAnimationAdapter`
* `SingleAnimationAdapter`
* `AnimationAdapter`
See the examples.
Developed By
-----
* Niek Haarman
License
-----
Copyright 2013 Niek Haarman
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
[1]: https://play.google.com/store/apps/details?id=com.haarman.listviewanimations
[2]: http://nineoldandroids.com/
[3]: http://en.wikipedia.org/wiki/Decorator_pattern
[4]: https://github.com/nhaarman/ListViewAnimations/blob/master/com.haarman.listviewanimations-1.9.jar?raw=true
[5]: https://gist.github.com/romannurik/2980593
[6]: https://play.google.com/store/apps/details?id=com.haarman.treinverkeer
[7]: https://www.twitter.com/haarmandev
[8]: https://plus.google.com/106017817931984343451
[9]: https://play.google.com/store/apps/details?id=com.niek.runningapp
没有合适的资源?快使用搜索试试~ 我知道了~
Android应用源码之listview 的各种动画效果.zip项目安卓应用源码下载
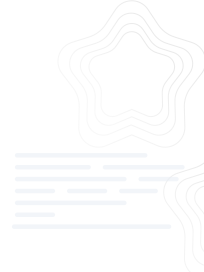
共216个文件
class:63个
java:60个
xml:32个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 189 浏览量
2022-03-08
02:48:57
上传
评论
收藏 2.8MB ZIP 举报
温馨提示
Android应用源码之listview 的各种动画效果.zip项目安卓应用源码下载Android应用源码之listview 的各种动画效果.zip项目安卓应用源码下载 1.适合学生毕业设计研究参考 2.适合个人学习研究参考 3.适合公司开发项目技术参考
资源详情
资源评论
资源推荐
收起资源包目录

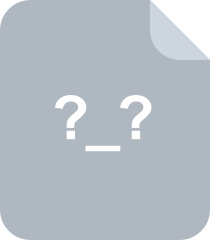
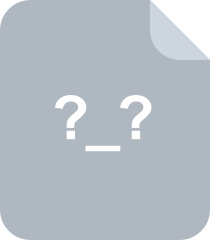
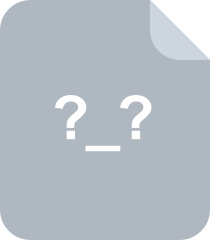
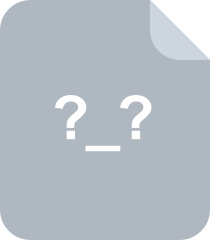
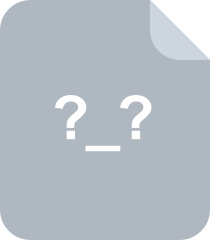
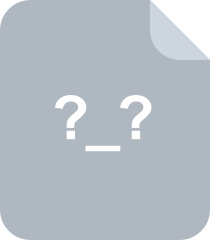
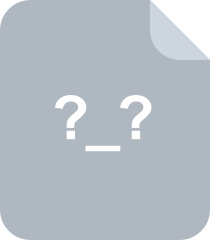
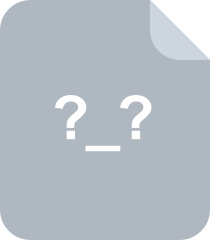
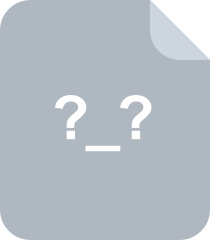
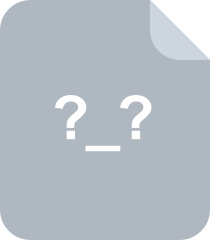
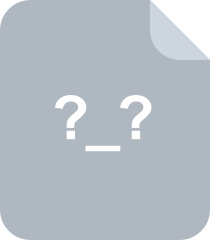
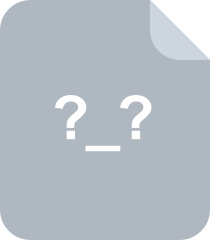
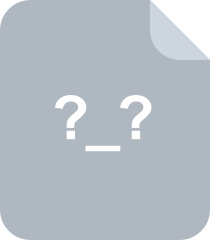
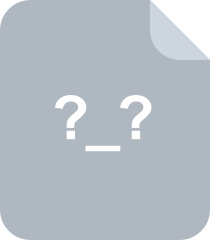
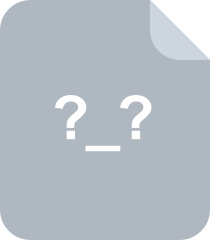
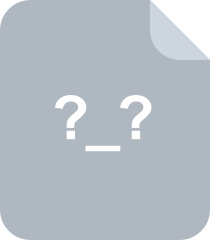
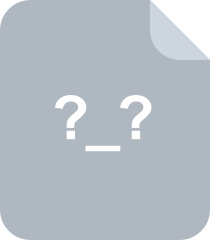
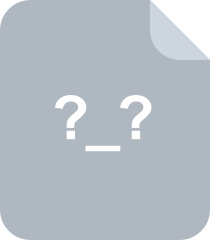
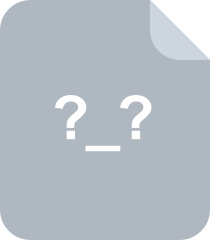
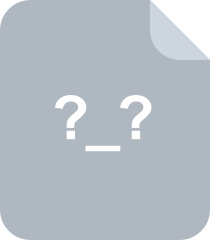
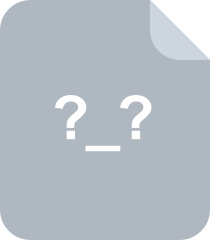
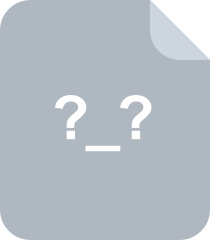
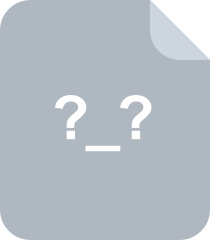
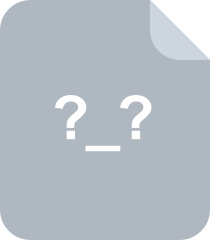
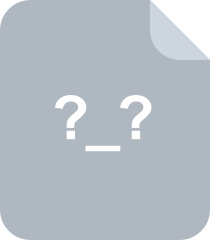
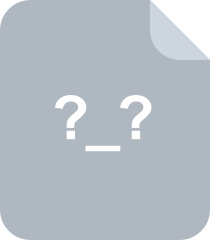
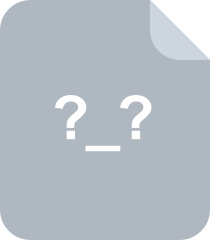
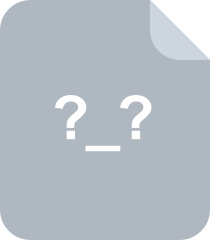
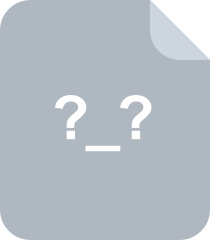
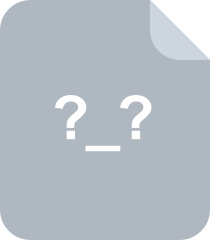
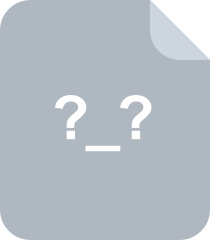
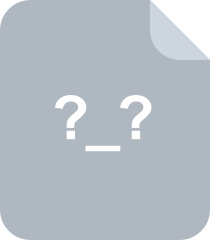
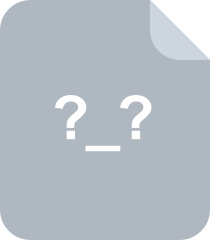
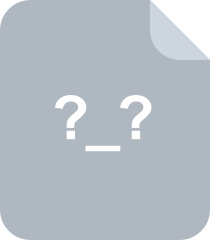
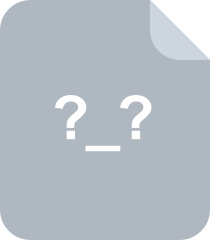
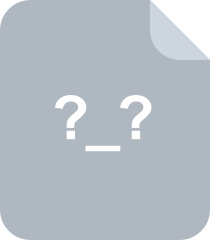
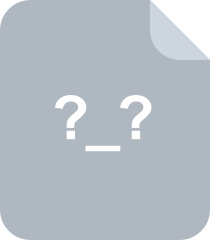
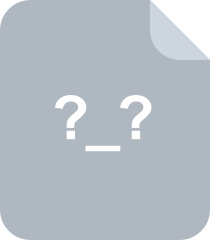
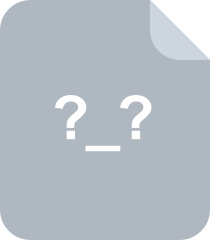
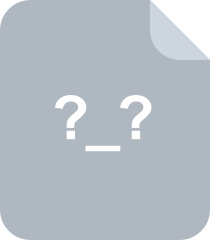
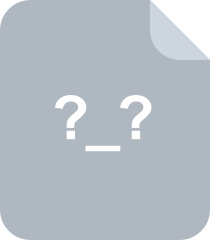
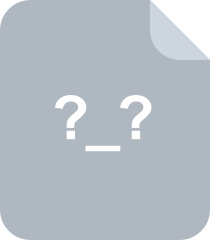
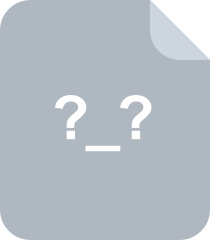
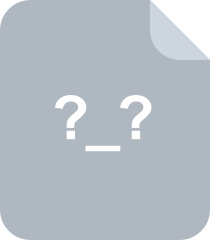
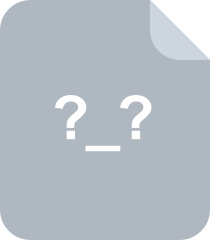
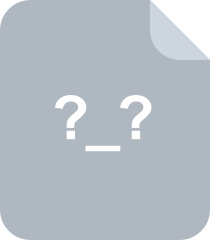
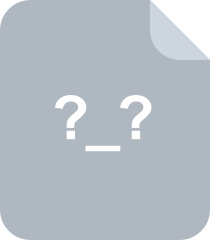
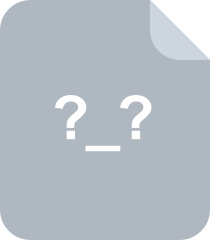
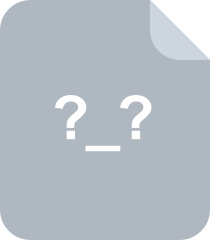
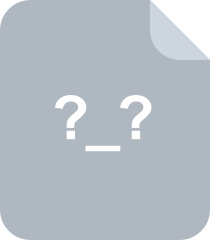
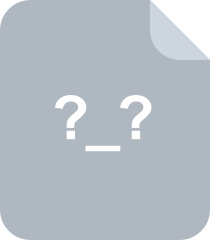
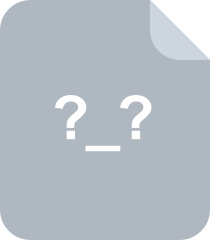
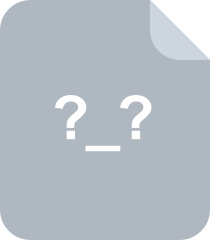
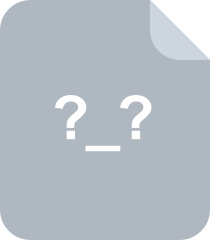
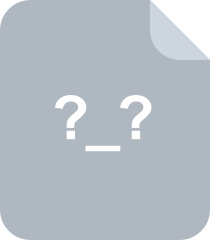
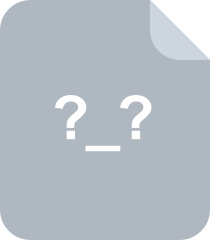
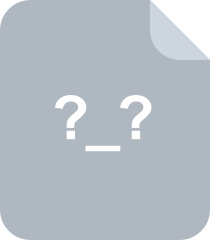
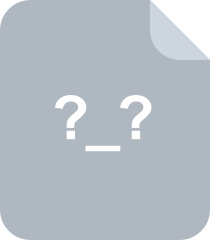
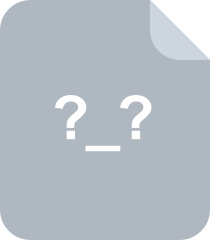
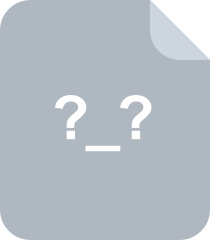
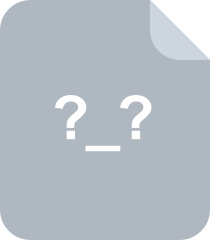
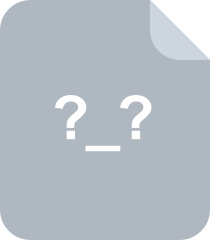
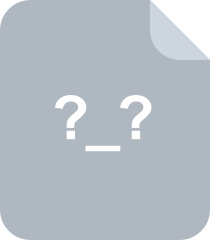
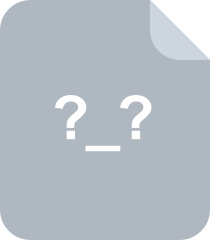
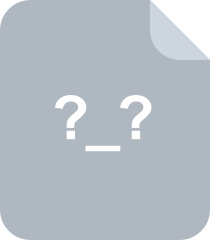
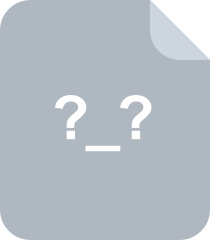
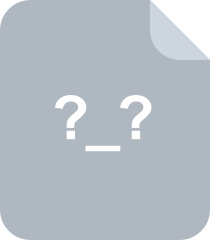
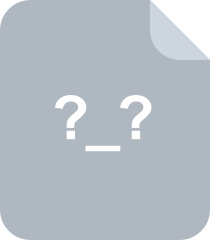
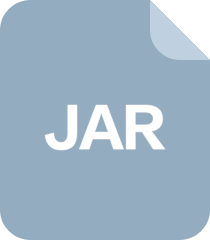
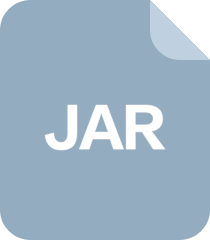
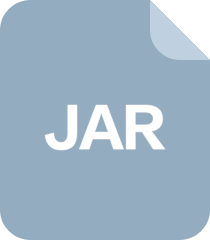
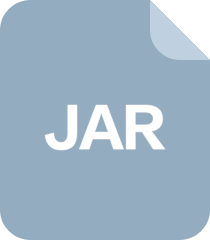
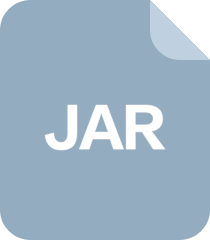
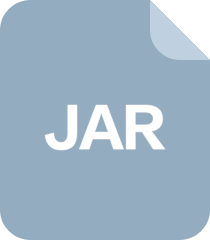
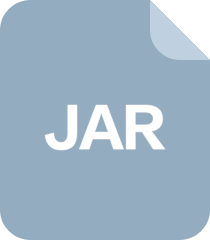
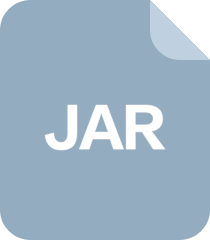
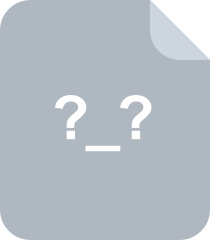
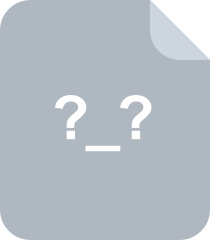
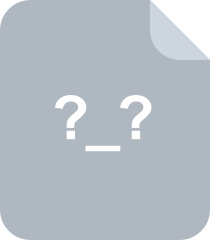
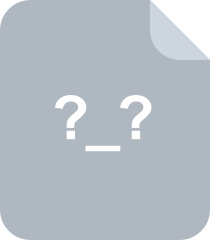
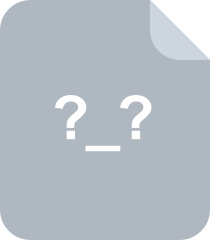
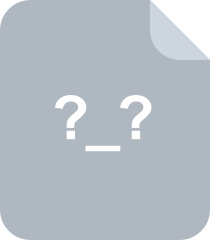
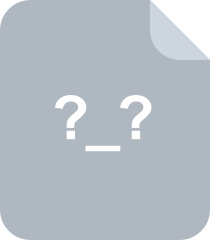
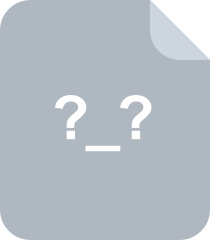
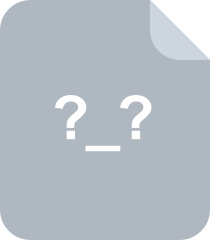
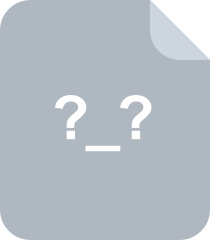
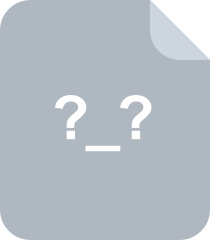
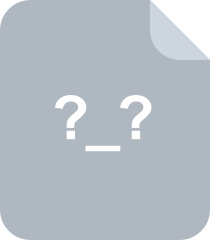
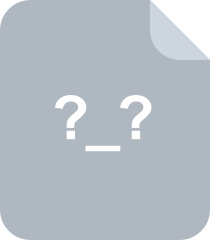
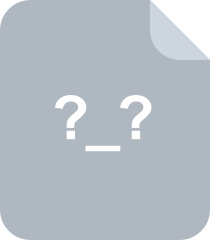
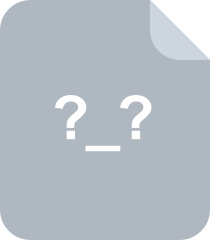
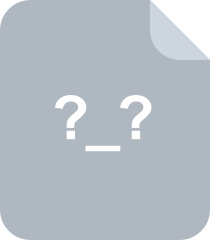
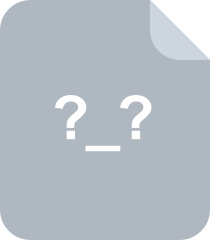
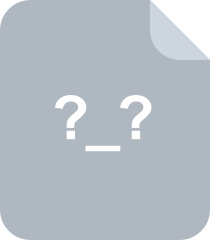
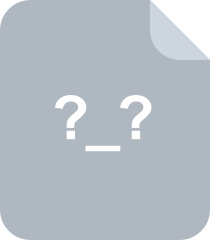
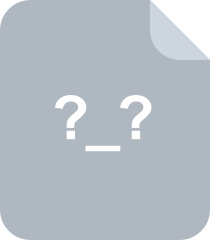
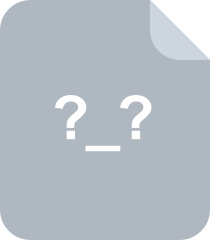
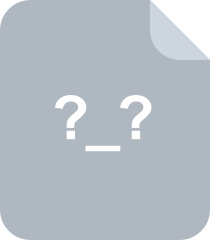
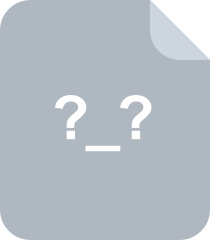
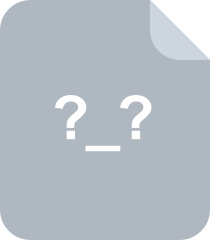
共 216 条
- 1
- 2
- 3
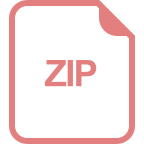
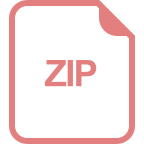
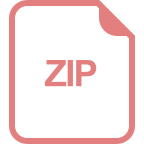
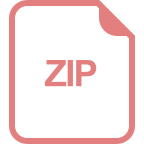
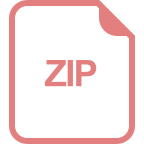
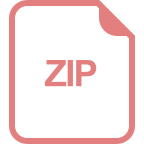
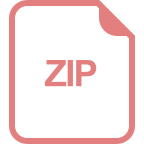
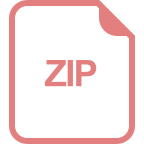
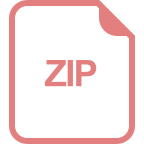
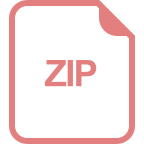
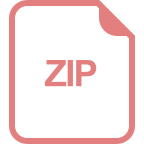
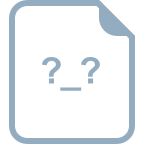
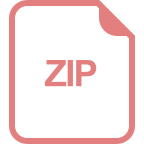
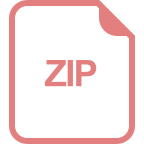
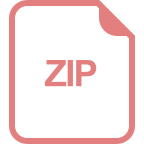

yxkfw
- 粉丝: 81
- 资源: 2万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

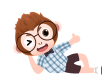
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


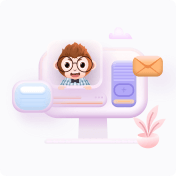
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0