/*
* Copyright (C) 2010 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.example.android.samplesync.platform;
import android.content.ContentResolver;
import android.content.ContentUris;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.net.Uri;
import android.provider.ContactsContract.Data;
import android.provider.ContactsContract.RawContacts;
import android.provider.ContactsContract.StatusUpdates;
import android.provider.ContactsContract.CommonDataKinds.Email;
import android.provider.ContactsContract.CommonDataKinds.Im;
import android.provider.ContactsContract.CommonDataKinds.Phone;
import android.provider.ContactsContract.CommonDataKinds.StructuredName;
import android.util.Log;
import com.example.android.samplesync.Constants;
import com.example.android.samplesync.R;
import com.example.android.samplesync.client.User;
import java.util.List;
/**
* Class for managing contacts sync related mOperations
*/
public class ContactManager {
/**
* Custom IM protocol used when storing status messages.
*/
public static final String CUSTOM_IM_PROTOCOL = "SampleSyncAdapter";
private static final String TAG = "ContactManager";
/**
* Synchronize raw contacts
*
* @param context The context of Authenticator Activity
* @param account The username for the account
* @param users The list of users
*/
public static synchronized void syncContacts(Context context,
String account, List<User> users) {
long userId;
long rawContactId = 0;
final ContentResolver resolver = context.getContentResolver();
final BatchOperation batchOperation =
new BatchOperation(context, resolver);
Log.d(TAG, "In SyncContacts");
for (final User user : users) {
userId = user.getUserId();
// Check to see if the contact needs to be inserted or updated
rawContactId = lookupRawContact(resolver, userId);
if (rawContactId != 0) {
if (!user.isDeleted()) {
// update contact
updateContact(context, resolver, account, user,
rawContactId, batchOperation);
} else {
// delete contact
deleteContact(context, rawContactId, batchOperation);
}
} else {
// add new contact
Log.d(TAG, "In addContact");
if (!user.isDeleted()) {
addContact(context, account, user, batchOperation);
}
}
// A sync adapter should batch operations on multiple contacts,
// because it will make a dramatic performance difference.
if (batchOperation.size() >= 50) {
batchOperation.execute();
}
}
batchOperation.execute();
}
/**
* Add a list of status messages to the contacts provider.
*
* @param context the context to use
* @param accountName the username of the logged in user
* @param statuses the list of statuses to store
*/
public static void insertStatuses(Context context, String username,
List<User.Status> list) {
final ContentValues values = new ContentValues();
final ContentResolver resolver = context.getContentResolver();
final BatchOperation batchOperation =
new BatchOperation(context, resolver);
for (final User.Status status : list) {
// Look up the user's sample SyncAdapter data row
final long userId = status.getUserId();
final long profileId = lookupProfile(resolver, userId);
// Insert the activity into the stream
if (profileId > 0) {
values.put(StatusUpdates.DATA_ID, profileId);
values.put(StatusUpdates.STATUS, status.getStatus());
values.put(StatusUpdates.PROTOCOL, Im.PROTOCOL_CUSTOM);
values.put(StatusUpdates.CUSTOM_PROTOCOL, CUSTOM_IM_PROTOCOL);
values.put(StatusUpdates.IM_ACCOUNT, username);
values.put(StatusUpdates.IM_HANDLE, status.getUserId());
values.put(StatusUpdates.STATUS_RES_PACKAGE, context
.getPackageName());
values.put(StatusUpdates.STATUS_ICON, R.drawable.icon);
values.put(StatusUpdates.STATUS_LABEL, R.string.label);
batchOperation
.add(ContactOperations.newInsertCpo(
StatusUpdates.CONTENT_URI, true).withValues(values)
.build());
// A sync adapter should batch operations on multiple contacts,
// because it will make a dramatic performance difference.
if (batchOperation.size() >= 50) {
batchOperation.execute();
}
}
}
batchOperation.execute();
}
/**
* Adds a single contact to the platform contacts provider.
*
* @param context the Authenticator Activity context
* @param accountName the account the contact belongs to
* @param user the sample SyncAdapter User object
*/
private static void addContact(Context context, String accountName,
User user, BatchOperation batchOperation) {
// Put the data in the contacts provider
final ContactOperations contactOp =
ContactOperations.createNewContact(context, user.getUserId(),
accountName, batchOperation);
contactOp.addName(user.getFirstName(), user.getLastName()).addEmail(
user.getEmail()).addPhone(user.getCellPhone(), Phone.TYPE_MOBILE)
.addPhone(user.getHomePhone(), Phone.TYPE_OTHER).addProfileAction(
user.getUserId());
}
/**
* Updates a single contact to the platform contacts provider.
*
* @param context the Authenticator Activity context
* @param resolver the ContentResolver to use
* @param accountName the account the contact belongs to
* @param user the sample SyncAdapter contact object.
* @param rawContactId the unique Id for this rawContact in contacts
* provider
*/
private static void updateContact(Context context,
ContentResolver resolver, String accountName, User user,
long rawContactId, BatchOperation batchOperation) {
Uri uri;
String cellPhone = null;
String otherPhone = null;
String email = null;
final Cursor c =
resolver.query(Data.CONTENT_URI, DataQuery.PROJECTION,
DataQuery.SELECTION,
new String[] {String.valueOf(rawContactId)}, null);
final ContactOperations contactOp =
ContactOperations.updateExistingContact(context, rawContactId,
batchOperation);
try {
while (c.moveToNext()) {
final long id = c.getLong(DataQuery.COLUMN_ID);
final String mimeType = c.getString(DataQuery.COLUMN_MIMETYPE);
uri = ContentUris.withAppendedId(Data.CONTENT_URI, id);
if (mimeType.equals(StructuredName.CONTENT_ITEM_TYPE)) {
final String lastName =
c.getString(DataQuery.COLUMN_FAMILY_NAME);

yxkfw
- 粉丝: 82
- 资源: 2万+
最新资源
- 射频电路基础复习提纲(2024年): 射频电路基本原理与应用
- 计算机二级培训ppt,培训教程课程安排格式
- 用C语言写出一个简单的圣诞树,让你的朋友们体验一下程序员的浪漫,点开即令哦!
- 空中俯视物体检测17-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- XC3568H-IPC工控主机用户手册.pdf
- java+sql server项目之科帮网计算机配件报价系统源代码.zip
- plsqldev1305x64连接数据工具
- 智能车开发案例,使用Python语言在一个文件中实现 这里我们将添加一些额外的功能,如自动驾驶模式、避障、超声波传感器读取以及通过TCP/IP网络远程控制
- 空中俯视物体检测18-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- 电赛案例,C++简单的智能家居系统,其中包含了温度监测、光照控制和报警系
- 西工大noj100题参考题解及注意事项(2024).zip
- 西工大noj100题参考题解及注意事项(2024).pdf
- java项目之仓库管理系统项目源代码.zip
- 基于python的图书管理系统demo脚本
- java项目之物流配货项目源代码.zip
- java项目之物资管理系统项目源代码.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


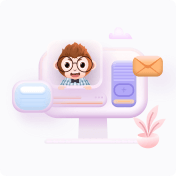