# Android Form EditText
Android form edit text is an extension of EditText that brings data validation facilities to the edittext.
# Example App
I built an example app that showcase some of the possibilities of the library.
You'll be able to find the app in the [Play Store](https://play.google.com/store/apps/details?id=com.andreabaccega.edittextformexample)
Here some screenshot of the Example app ( and the library )
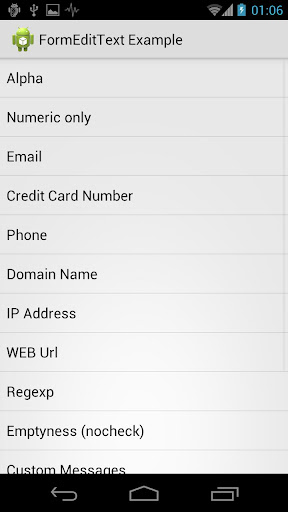 - 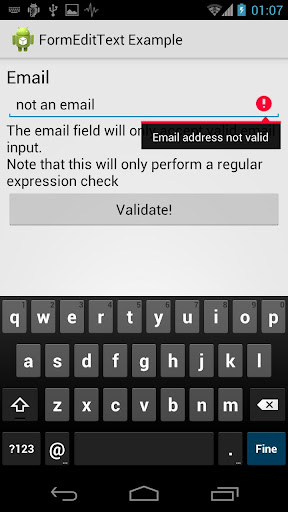
The app source code is located under this repo!
# How to include it
This library can be found in maven central repo. If you're using Android studio you can include it by writing the following in the corresponding _dependencies_ block
#### Gradle:
```groovy
dependencies {
// ...
compile 'com.andreabaccega:android-form-edittext:1.0.5'
// ...
}
```
####Maven
```xml
<dependency>
<groupId>com.andreabaccega</groupId>
<artifactId>android-form-edittext</artifactId>
<version>${com.andreabaccega.android-form-edittext-version}</version>
<type>aar</type>
<scope>provided</scope>
</dependency>
```
# How to use
In your xml import an extra namespace on the root of your layout
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:whatever="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
....
<!-- Your actual layout -->
....
</LinearLayout>
```
**Note:** It's not mandatory to use it on the root element. Also remember to change the xmlns value with your package name
Whenever you need to use the FormEditText just do the following in your xml.
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:whatever="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<!-- Some stuff -->
<com.andreabaccega.widget.FormEditText
whatever:testType="alpha"
android:id="@+id/et_firstname"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
<!-- Some other stuff -->
</LinearLayout>
```
As you noticed there is a *whatever:test* attribute setted to *alpha*. This let the FormEditText know that the data inside it should be only Alpha characters.
There are several values you can set to the test attribute:
- **regexp**: for custom regexp
- **numeric**: for an only numeric field
- **alpha**: for an alpha only field
- **alphaNumeric**: guess what?
- **personName**: checks if the entered text is a person first or last name.
- **personFullName**: checks if the entered value is a complete full name.
- **email**: checks that the field is a valid email
- **creditCard**: checks that the field contains a valid credit card using [Luhn Algorithm](http://en.wikipedia.org/wiki/Luhn_algorithm)
- **phone**: checks that the field contains a valid phone number
- **domainName**: checks that field contains a valid domain name ( always passes the test in API Level < 8 )
- **ipAddress**: checks that the field contains a valid ip address
- **webUrl**: checks that the field contains a valid url ( always passes the test in API Level < 8 )
- **date**: checks that the field is a valid date/datetime format ( if customFormat is set, checks with customFormat )
- **nocheck**: It does not check anything except the emptyness of the field.
For most of the test type values this library comes with a couple of default strings. This means that error strings ( english only ) are already available for the following test types: numeric, alpha, alphanumeric
You can customize them using the attributes
- **testErrorString** used when the field does not pass the test
- **emptyErrorString** used when the field is empty
### Example:
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:whatever="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<!-- Some stuff -->
<com.andreabaccega.widget.FormEditText
whatever:testType="alpha"
whatever:emptyErrorString="@string/your_name_cannot_be_empty"
whatever:testErrorString="@string/your_name_is_ugly"
android:id="@+id/et_firstname"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
<!-- Some other stuff -->
</LinearLayout>
```
Furthermore you can ask the FormEditText to allow the content to be *optional*.
Just use the **emptyAllowed** attribute and set it to *true*. **Note:** If you don't specify the **emptyAllowed** attribute the default value is *false*.
If you want to use **regexp** as **test** attribute value you'll need to also use the **customRegexp** attribute. Take a look in the following example:
### Example: (Email check)
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:whatever="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<!-- Some stuff -->
<com.andreabaccega.widget.FormEditText
whatever:testType="regexp"
whatever:customRegexp="^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$"
whatever:testErrorString="@string/error_emailnotvalid"
android:id="@+id/et_email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/hint_email"
android:inputType="textEmailAddress"
/>
<!-- Some other stuff -->
</LinearLayout>
```
**Note:** The library supports the email check natively using the **email** value as the **test** attribute.
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:whatever="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<!-- Some stuff -->
<com.andreabaccega.widget.FormEditText
whatever:testType="email"
android:id="@+id/et_email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/hint_email"
android:inputType="textEmailAddress"
/>
<!-- Some other stuff -->
</LinearLayout>
```
In your Java code you'll need to call a method when you want to know if the field validates.
```java
public void onClickNext(View v) {
FormEditText[] allFields = { etFirstname, etLastname, etAddress, etZipcode, etCity };
boolean allValid = true;
for (FormEditText field: allFields) {
allValid = field.testValidity() && allValid;
}
if (allValid) {
// YAY
} else {
// EditText are going to appear with an exclamation mark and an explicative message.
}
}
```
Calling *testValidity()* will cause the EditText to cycle through all the validators and call the isValid method. it will stop when one of them returns false or there are no validators left.
Furthermore *testValidity()* will also place an exclamation mark on the right of the [EditText](http://developer.android.com/reference/android/widget/EditText.html) and will call the [setError](http://developer.android.com/reference/android/widget/TextView.html#setError) method.
## Add your custom validators
You can add your custom validators runtime through the *addValidator* method. For example, let's suppouse we want to add a validator that checks that the text input is equal to the string "ciao":
```jav
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
Android Form EditText 验证输入合法性的编辑框.zip项目安卓应用源码下载Android Form EditText 验证输入合法性的编辑框.zip项目安卓应用源码下载 1.适合学生毕业设计研究参考 2.适合个人学习研究参考 3.适合公司开发项目技术参考
资源推荐
资源详情
资源评论
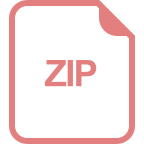
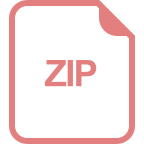
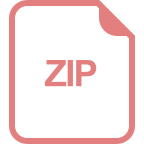
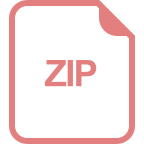
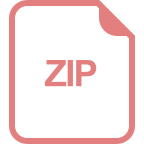
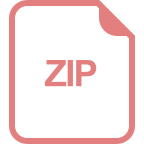
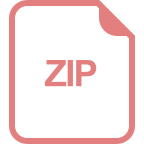
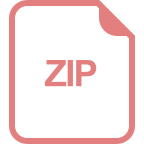
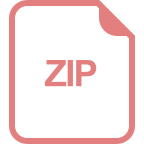
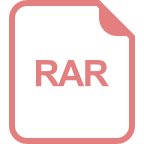
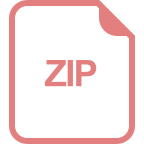
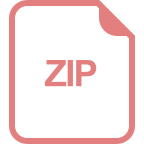
收起资源包目录




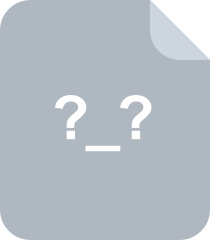
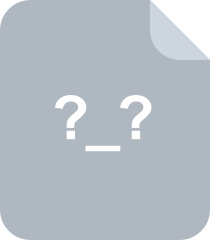
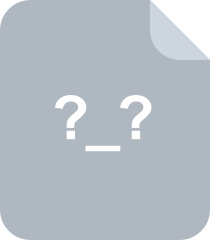

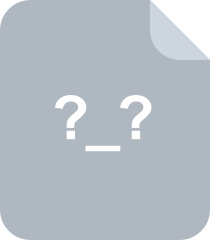


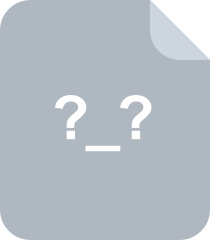

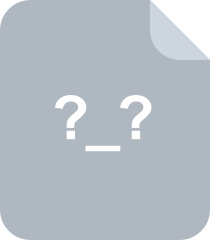

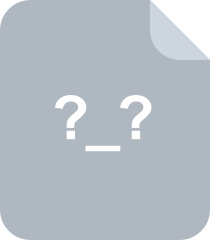

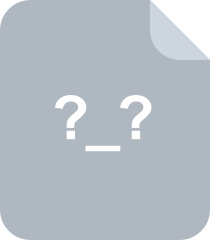

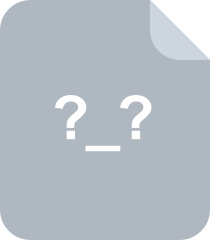

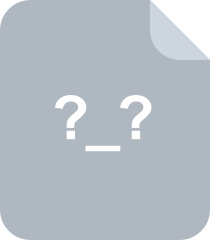
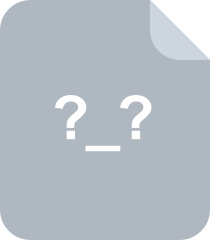
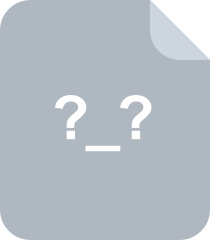




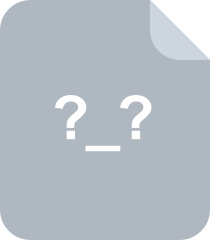
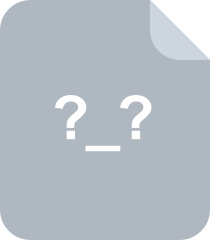
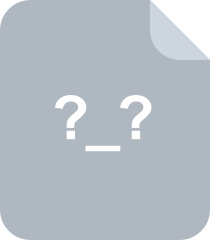
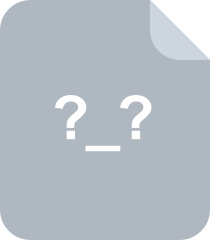

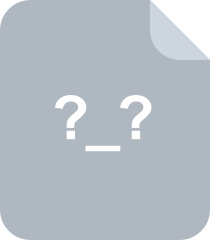
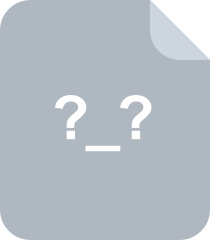
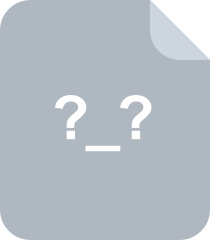
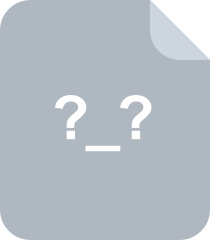
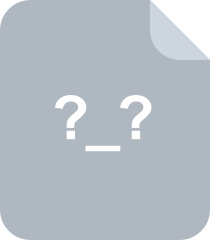
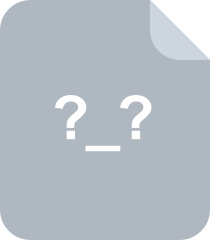
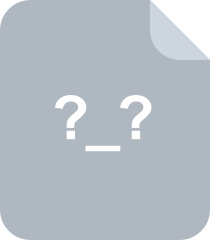
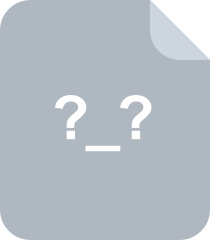
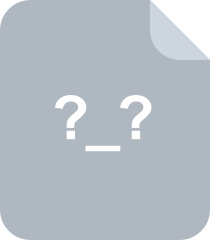
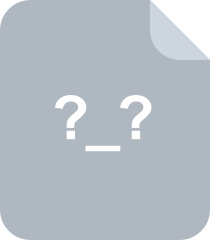
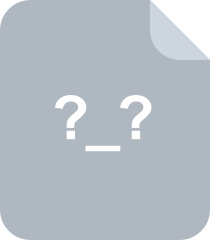
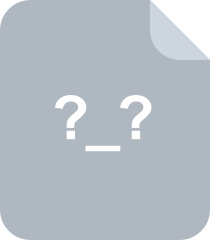
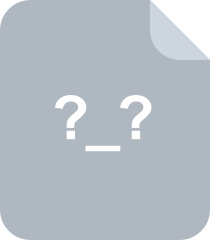
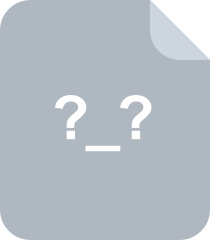
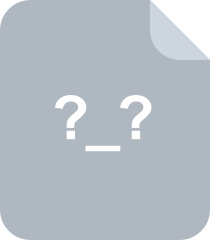
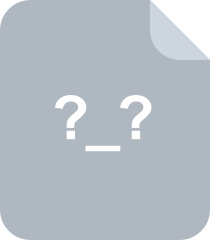
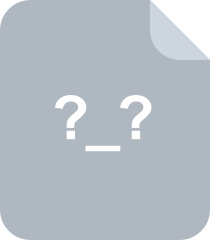
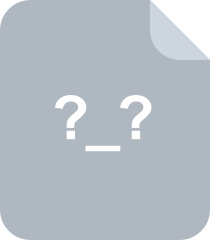
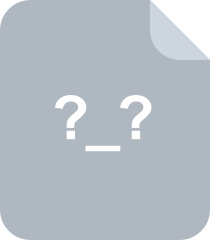
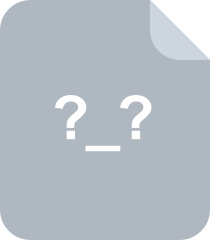
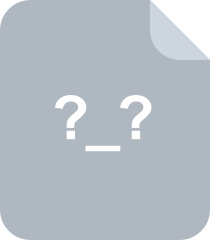
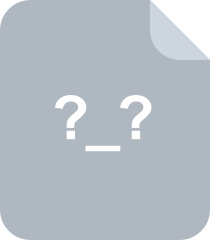
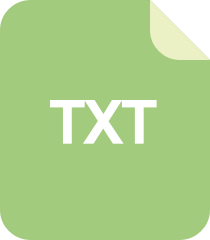
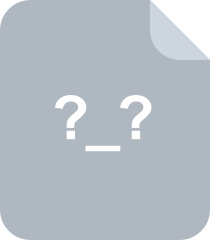
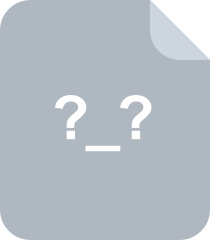

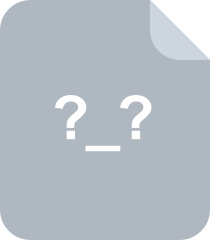
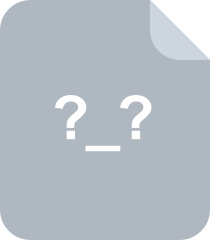
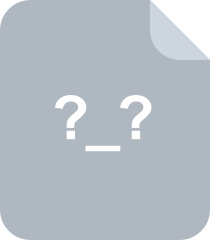
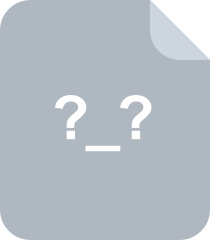

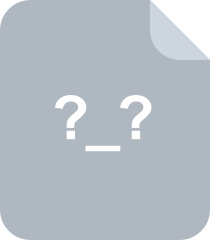


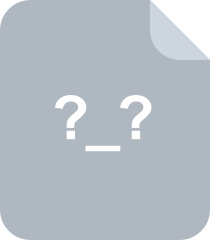
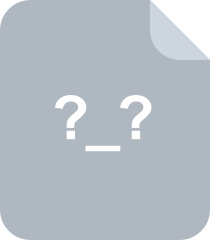
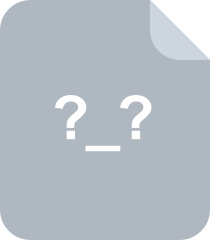
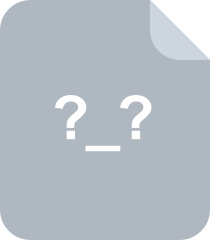
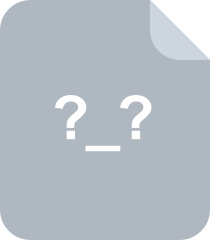
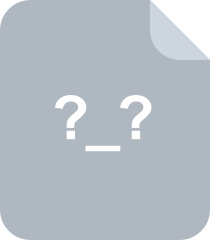
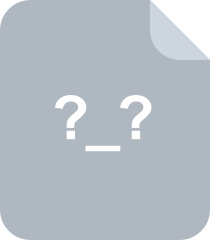
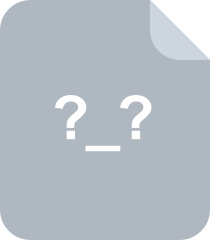
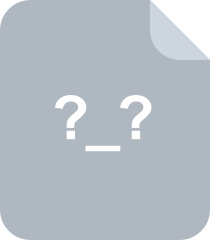
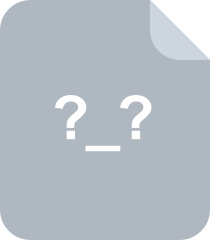
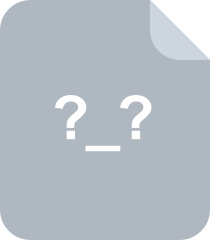
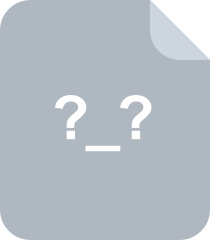
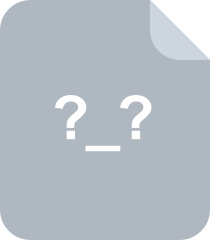
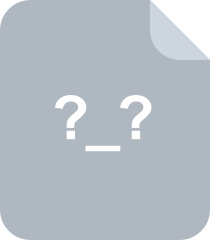
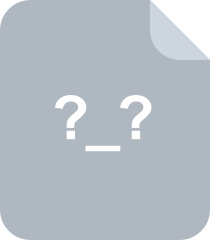
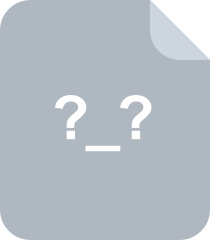
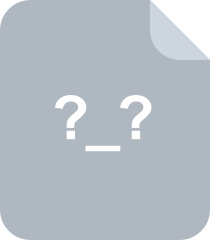
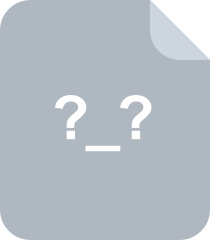
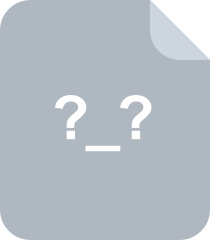

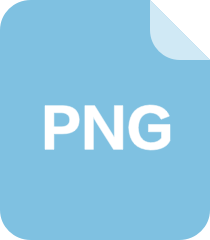

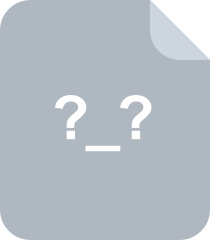

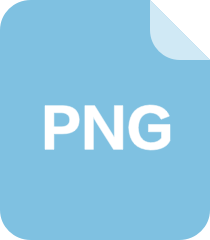

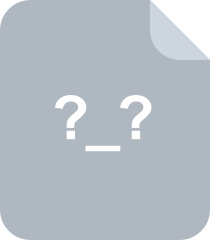

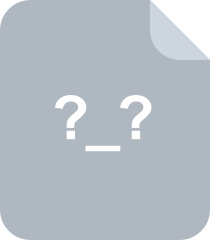

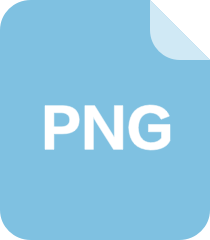

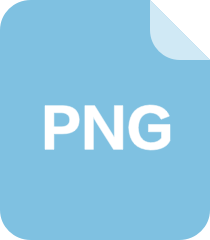

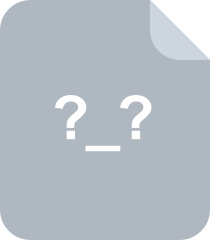
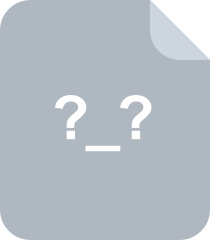
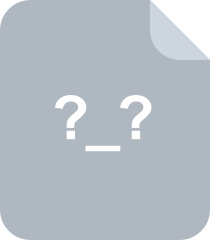




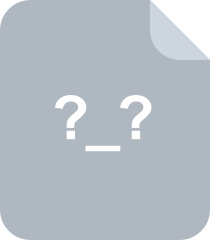
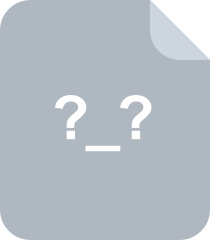
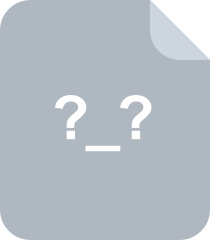

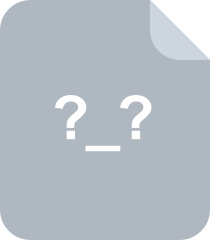
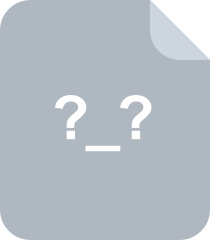
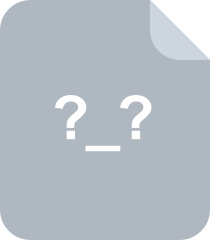
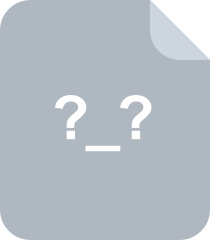
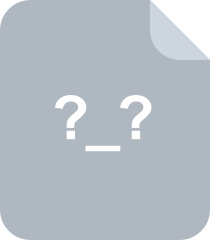
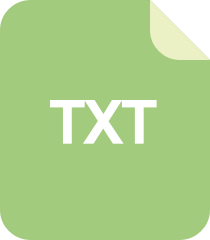
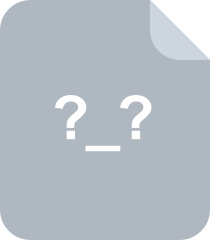

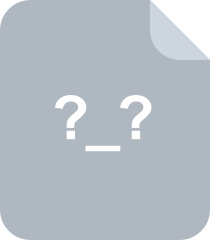
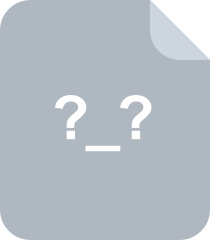
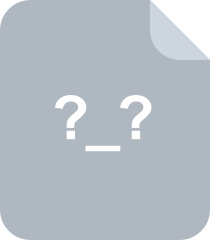
共 88 条
- 1
资源评论
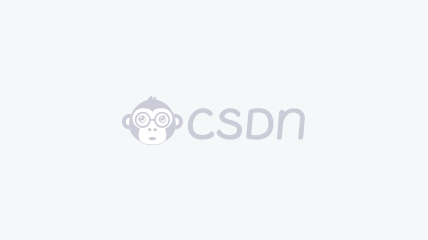

yxkfw
- 粉丝: 79
- 资源: 2万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

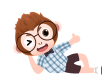
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


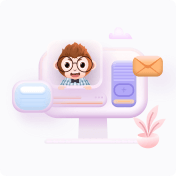
安全验证
文档复制为VIP权益,开通VIP直接复制
