/* ----------------------------------------------------------------------------
* MobileDevice.h - interface to MobileDevice.framework
* $LastChangedDate: 2007-07-09 18:59:29 -0700 (Mon, 09 Jul 2007) $
*
* Copied from http://iphonesvn.halifrag.com/svn/iPhone/
* With modifications from Allen Porter and Scott Turner
*
* ------------------------------------------------------------------------- */
#ifndef MOBILEDEVICE_H
#define MOBILEDEVICE_H
#ifdef __cplusplus
extern "C" {
#endif
#if defined(WIN32)
#include <CoreFoundation.h>
typedef unsigned int mach_error_t;
#elif defined(__APPLE__)
#include <CoreFoundation/CoreFoundation.h>
#include <mach/error.h>
#endif
/* Error codes */
#define MDERR_APPLE_MOBILE (err_system(0x3a))
#define MDERR_IPHONE (err_sub(0))
/* Apple Mobile (AM*) errors */
#define MDERR_OK ERR_SUCCESS
#define MDERR_SYSCALL (ERR_MOBILE_DEVICE | 0x01)
#define MDERR_OUT_OF_MEMORY (ERR_MOBILE_DEVICE | 0x03)
#define MDERR_QUERY_FAILED (ERR_MOBILE_DEVICE | 0x04)
#define MDERR_INVALID_ARGUMENT (ERR_MOBILE_DEVICE | 0x0b)
#define MDERR_DICT_NOT_LOADED (ERR_MOBILE_DEVICE | 0x25)
/* Apple File Connection (AFC*) errors */
#define MDERR_AFC_OUT_OF_MEMORY 0x03
/* USBMux errors */
#define MDERR_USBMUX_ARG_NULL 0x16
#define MDERR_USBMUX_FAILED 0xffffffff
/* Messages passed to device notification callbacks: passed as part of
* am_device_notification_callback_info. */
#define ADNCI_MSG_CONNECTED 1
#define ADNCI_MSG_DISCONNECTED 2
#define ADNCI_MSG_UNKNOWN 3
#define AMD_IPHONE_PRODUCT_ID 0x1290
#define AMD_IPHONE_SERIAL "3391002d9c804d105e2c8c7d94fc35b6f3d214a3"
/* Services, found in /System/Library/Lockdown/Services.plist */
#define AMSVC_AFC CFSTR("com.apple.afc")
#define AMSVC_BACKUP CFSTR("com.apple.mobilebackup")
#define AMSVC_CRASH_REPORT_COPY CFSTR("com.apple.crashreportcopy")
#define AMSVC_DEBUG_IMAGE_MOUNT CFSTR("com.apple.mobile.debug_image_mount")
#define AMSVC_NOTIFICATION_PROXY CFSTR("com.apple.mobile.notification_proxy")
#define AMSVC_PURPLE_TEST CFSTR("com.apple.purpletestr")
#define AMSVC_SOFTWARE_UPDATE CFSTR("com.apple.mobile.software_update")
#define AMSVC_SYNC CFSTR("com.apple.mobilesync")
#define AMSVC_SCREENSHOT CFSTR("com.apple.screenshotr")
#define AMSVC_SYSLOG_RELAY CFSTR("com.apple.syslog_relay")
#define AMSVC_SYSTEM_PROFILER CFSTR("com.apple.mobile.system_profiler")
typedef unsigned int afc_error_t;
typedef unsigned int usbmux_error_t;
struct am_recovery_device;
struct am_device_notification_callback_info {
struct am_device *dev; /* 0 device */
unsigned int msg; /* 4 one of ADNCI_MSG_* */
} __attribute__ ((packed));
/* The type of the device restore notification callback functions.
* TODO: change to correct type. */
typedef void (*am_restore_device_notification_callback)(struct
am_recovery_device *);
/* This is a CoreFoundation object of class AMRecoveryModeDevice. */
struct am_recovery_device {
unsigned char unknown0[8]; /* 0 */
am_restore_device_notification_callback callback; /* 8 */
void *user_info; /* 12 */
unsigned char unknown1[12]; /* 16 */
unsigned int readwrite_pipe; /* 28 */
unsigned char read_pipe; /* 32 */
unsigned char write_ctrl_pipe; /* 33 */
unsigned char read_unknown_pipe; /* 34 */
unsigned char write_file_pipe; /* 35 */
unsigned char write_input_pipe; /* 36 */
} __attribute__ ((packed));
/* A CoreFoundation object of class AMRestoreModeDevice. */
struct am_restore_device {
unsigned char unknown[32];
int port;
} __attribute__ ((packed));
/* The type of the device notification callback function. */
typedef void(*am_device_notification_callback)(struct
am_device_notification_callback_info *, void* arg);
/* The type of the _AMDDeviceAttached function.
* TODO: change to correct type. */
typedef void *amd_device_attached_callback;
/* The type of the device restore notification callback functions.
* TODO: change to correct type. */
//typedef void (*am_restore_device_notification_callback)(struct am_recovery_device *);
struct am_device {
unsigned char unknown0[16]; /* 0 - zero */
unsigned int device_id; /* 16 */
unsigned int product_id; /* 20 - set to AMD_IPHONE_PRODUCT_ID */
char *serial; /* 24 - set to AMD_IPHONE_SERIAL */
unsigned int unknown1; /* 28 */
unsigned char unknown2[4]; /* 32 */
unsigned int lockdown_conn; /* 36 */
unsigned char unknown3[8]; /* 40 */
} __attribute__ ((packed));
struct am_device_notification {
unsigned int unknown0; /* 0 */
unsigned int unknown1; /* 4 */
unsigned int unknown2; /* 8 */
am_device_notification_callback callback; /* 12 */
unsigned int unknown3; /* 16 */
} __attribute__ ((packed));
struct afc_connection {
unsigned int handle; /* 0 */
unsigned int unknown0; /* 4 */
unsigned char unknown1; /* 8 */
unsigned char padding[3]; /* 9 */
unsigned int unknown2; /* 12 */
unsigned int unknown3; /* 16 */
unsigned int unknown4; /* 20 */
unsigned int fs_block_size; /* 24 */
unsigned int sock_block_size; /* 28: always 0x3c */
unsigned int io_timeout; /* 32: from AFCConnectionOpen, usu. 0 */
void *afc_lock; /* 36 */
unsigned int context; /* 40 */
} __attribute__ ((packed));
struct afc_directory {
unsigned char unknown[0]; /* size unknown */
} __attribute__ ((packed));
struct afc_dictionary {
unsigned char unknown[0]; /* size unknown */
} __attribute__ ((packed));
typedef unsigned long long afc_file_ref;
struct usbmux_listener_1 { /* offset value in iTunes */
unsigned int unknown0; /* 0 1 */
unsigned char *unknown1; /* 4 ptr, maybe device? */
amd_device_attached_callback callback; /* 8 _AMDDeviceAttached */
unsigned int unknown3; /* 12 */
unsigned int unknown4; /* 16 */
unsigned int unknown5; /* 20 */
} __attribute__ ((packed));
struct usbmux_listener_2 {
unsigned char unknown0[4144];
} __attribute__ ((packed));
struct am_bootloader_control_packet {
unsigned char opcode; /* 0 */
unsigned char length; /* 1 */
unsigned char magic[2]; /* 2: 0x34, 0x12 */
unsigned char payload[0]; /* 4 */
} __attribute__ ((packed));
/* ----------------------------------------------------------------------------
* Public routines
* ------------------------------------------------------------------------- */
/* Registers a notification with the current run loop. The callback gets
* copied into the notification struct, as well as being registered with the
* current run loop. dn_unknown3 gets copied into unknown3 in the same.
* (Maybe dn_unknown3 is a user info parameter that gets passed as an arg to
* the callback?) unused0 and unused1 are both 0 when iTunes calls this.
* In iTunes the callback is located from $3db78e-$3dbbaf.
*
* Returns:
* MDERR_OK if successful
* MDERR_SYSCALL if CFRunLoopAddSource() failed
* MDERR_OUT_OF_MEMORY if we ran out of memory
*/
mach_error_t AMDeviceNotificationSubscribe(am_device_notification_callback
callback, unsigned int unused0, unsigned int unused1, void* //unsigned int
dn_unknown3, struct am_device_notification **notification);
/* Connects to the iPhone. Pass in the am_device structure that the
* notification
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
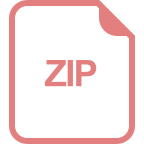
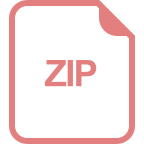
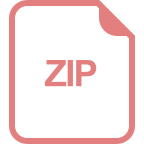
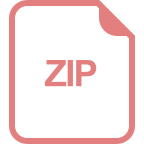
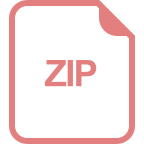
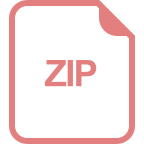
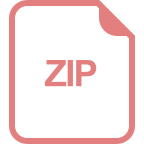
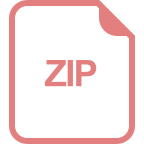
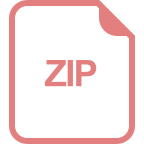
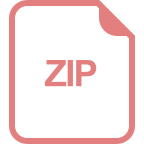
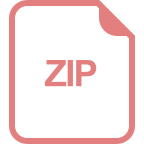
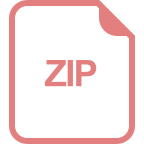
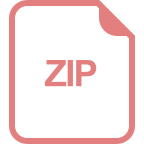
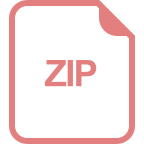
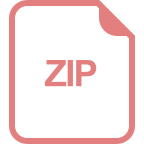
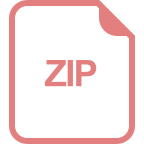
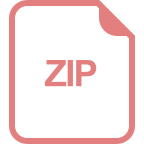
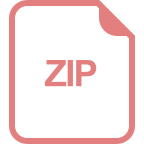
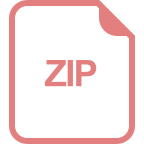
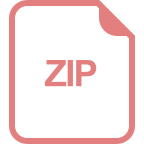
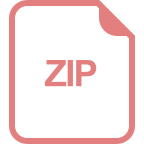
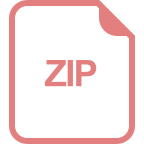
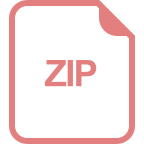
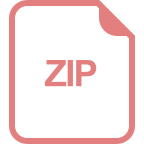
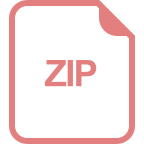
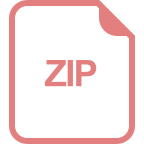
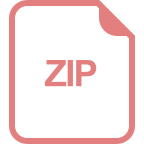
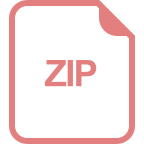
收起资源包目录


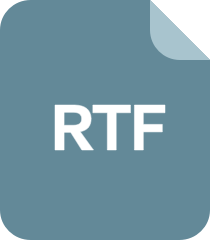
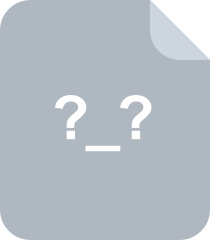


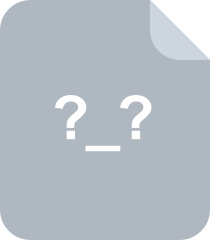

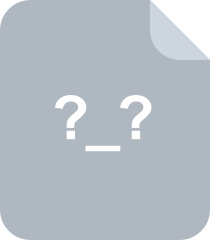

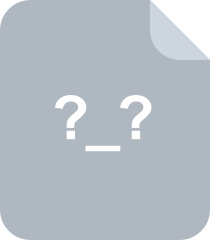
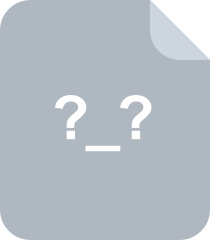
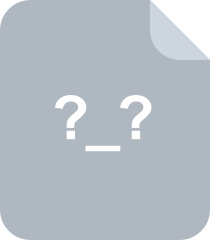
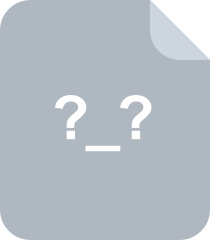
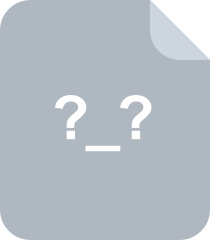

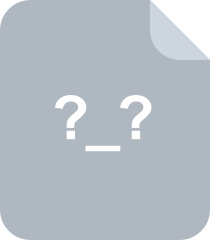

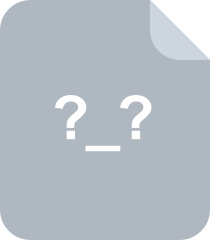

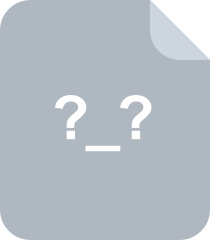
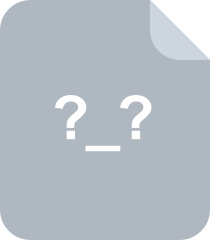
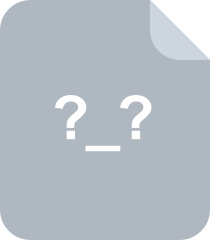
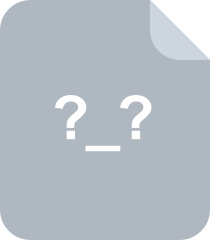
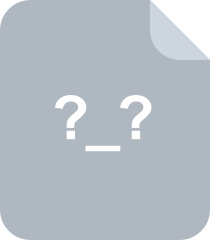

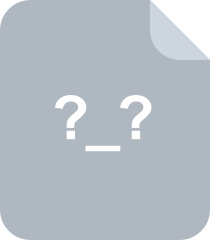
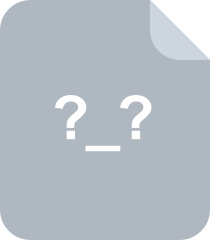
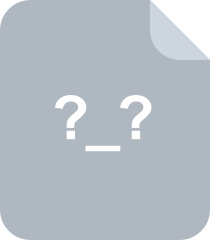
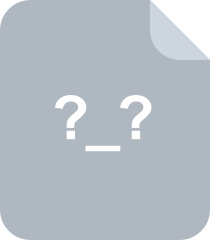
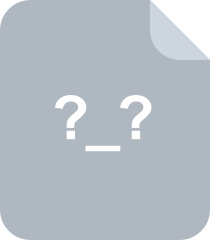
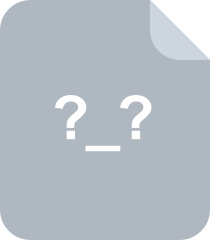
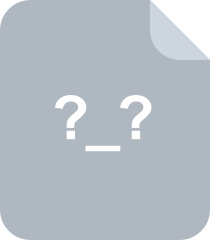
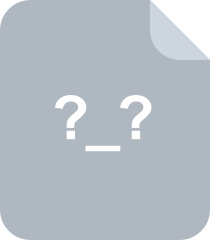
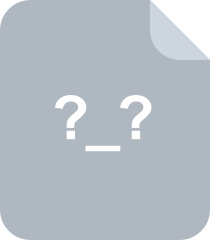
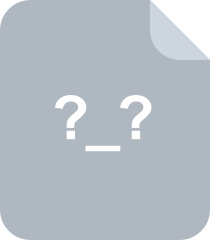
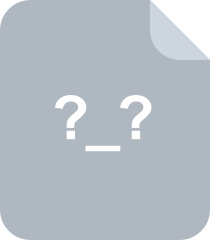
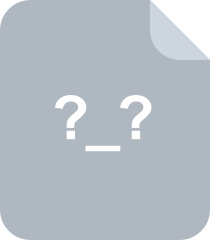
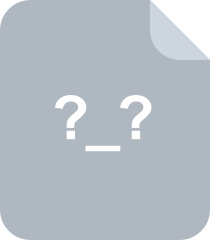
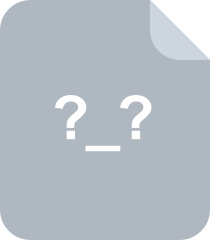
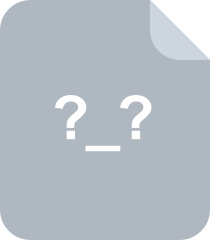
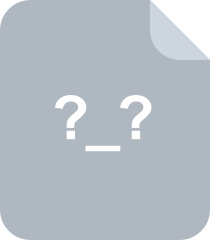
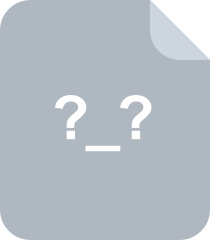
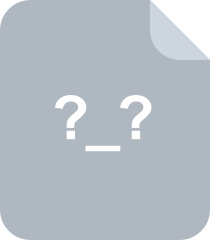
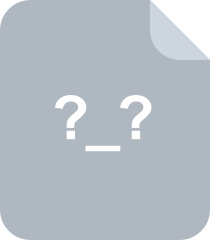
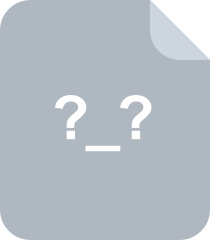
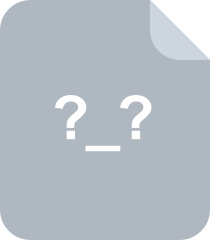
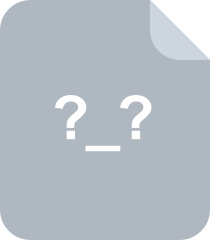
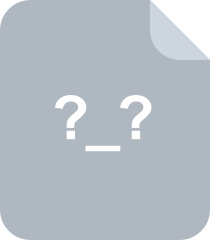
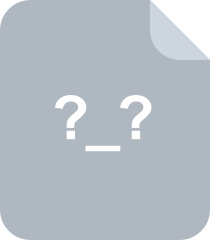
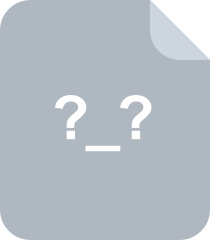
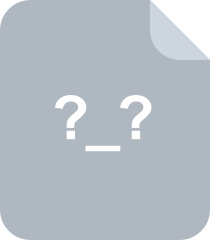
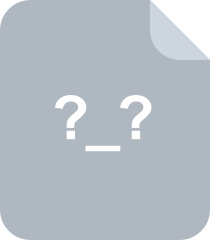
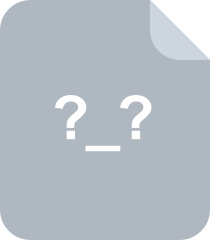

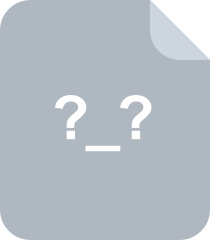
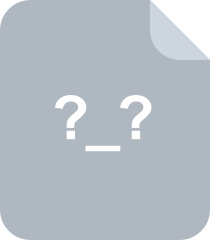
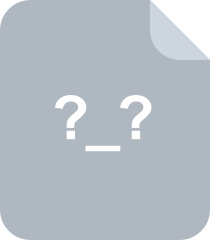

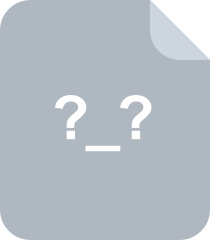

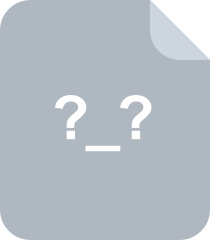

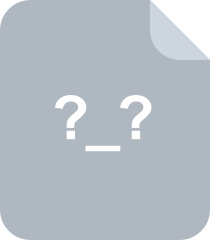
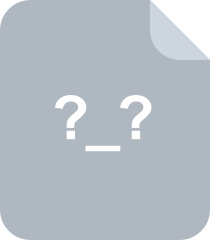
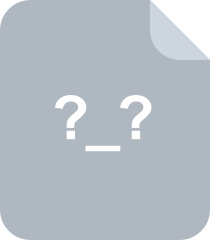
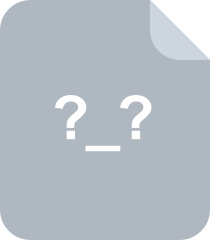
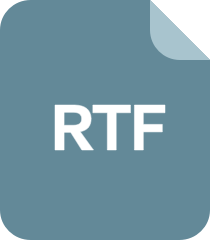
共 54 条
- 1
资源评论
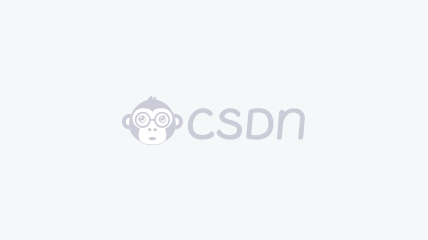

yxkfw
- 粉丝: 80
- 资源: 2万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

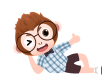
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


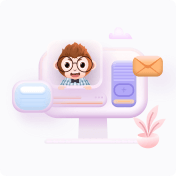
安全验证
文档复制为VIP权益,开通VIP直接复制
