package com.exam.util;
import org.apache.commons.lang.StringUtils;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.Locale;
import java.util.logging.Level;
import java.util.logging.Logger;
public class DateUtil {
public final static long ONE_DAY_SECONDS = 86400;
public final static String shortFormat = "yyyyMMdd";
public final static String longFormat = "yyyyMMddHHmmss";
public final static String concurrentFormat = "yyyyMMddHHmmssSSS";
public final static String shortConcurrentFormat = "yyMMddHHmmssSSS";
public final static String webFormat = "yyyy-MM-dd";
public final static String webMonthFormat = "yyyy-MM";
public final static String timeFormat = "HH:mm:ss";
public final static String monthFormat = "yyyyMM";
public final static String chineseDtFormat = "yyyy年MM月dd日";
public final static String chineseYMFormat = "yyyy年MM月";
public final static String newFormat = "yyyy-MM-dd HH:mm:ss";
public final static String noSecondFormat = "yyyy-MM-dd HH:mm";
public final static String MdFormat = "MM-dd";
public final static long ONE_DAY_MILL_SECONDS = 86400000;
public static DateFormat getNewDateFormat(String pattern) {
DateFormat df = new SimpleDateFormat(pattern);
df.setLenient(false);
return df;
}
public static String format(Date date, String format) {
if (date == null) {
return null;
}
return new SimpleDateFormat(format).format(date);
}
public static String format(String dateStr, String oldFormat, String newFormat) {
if (dateStr == null) {
return null;
}
String result = null;
DateFormat oldDateFormat = new SimpleDateFormat(oldFormat);
DateFormat newDateFormat = new SimpleDateFormat(newFormat);
try {
Date date = oldDateFormat.parse(dateStr);
result = newDateFormat.format(date);
} catch (ParseException e) {
e.printStackTrace();
}
return result;
}
public static Date parseDateNoTime(String sDate) {
DateFormat dateFormat = new SimpleDateFormat(shortFormat);
Date date = null;
if ((sDate == null) || (sDate.length() < shortFormat.length())) {
return null;
}
if (!StringUtils.isNumeric(sDate)) {
return null;
}
try {
date = dateFormat.parse(sDate);
} catch (ParseException e) {
e.printStackTrace();
}
return date;
}
public static Date parseDateNoTime(String sDate, String format) throws ParseException {
if (StringUtils.isBlank(format)) {
throw new ParseException("Null format. ", 0);
}
DateFormat dateFormat = new SimpleDateFormat(format);
if ((sDate == null) || (sDate.length() < format.length())) {
throw new ParseException("length too little", 0);
}
return dateFormat.parse(sDate);
}
public static Date parseDateNoTimeWithDelimit(String sDate, String delimit) throws ParseException {
sDate = sDate.replaceAll(delimit, "");
DateFormat dateFormat = new SimpleDateFormat(shortFormat);
if ((sDate == null) || (sDate.length() != shortFormat.length())) {
throw new ParseException("length not match", 0);
}
return dateFormat.parse(sDate);
}
public static Date parseDateLongFormat(String sDate) {
DateFormat dateFormat = new SimpleDateFormat(longFormat);
Date d = null;
if ((sDate != null) && (sDate.length() == longFormat.length())) {
try {
d = dateFormat.parse(sDate);
} catch (ParseException ex) {
return null;
}
}
return d;
}
public static Date parseDateNewFormat(String sDate) {
DateFormat dateFormat = new SimpleDateFormat(newFormat);
Date d = null;
dateFormat.setLenient(false);
if ((sDate != null) && (sDate.length() == newFormat.length())) {
try {
d = dateFormat.parse(sDate);
} catch (ParseException ex) {
return null;
}
}
return d;
}
public static Date parseDateNoSecondFormat(String sDate) {
DateFormat dateFormat = new SimpleDateFormat(noSecondFormat);
Date d = null;
dateFormat.setLenient(false);
if ((sDate != null) && (sDate.length() == noSecondFormat.length())) {
try {
d = dateFormat.parse(sDate);
} catch (ParseException ex) {
return null;
}
}
return d;
}
public static Date parseDateWebFormat(String sDate) {
DateFormat dateFormat = new SimpleDateFormat(webFormat);
Date d = null;
dateFormat.setLenient(false);
if ((sDate != null) && (sDate.length() == webFormat.length())) {
try {
d = dateFormat.parse(sDate);
} catch (ParseException ex) {
return null;
}
}
return d;
}
public static Date parseDateWebMonthFormat(String sDate) {
DateFormat dateFormat = new SimpleDateFormat(webMonthFormat);
Date d = null;
dateFormat.setLenient(false);
if ((sDate != null) && (sDate.length() == webMonthFormat.length())) {
try {
d = dateFormat.parse(sDate);
} catch (ParseException ex) {
return null;
}
}
return d;
}
/**
* 计算当前时间几小时之后的时间
*
* @param date
* @param hours
*
* @return
*/
public static Date addHours(Date date, long hours) {
return addMinutes(date, hours * 60);
}
/**
* 计算当前时间几分钟之后的时间
*
* @param date
* @param minutes
*
* @return
*/
public static Date addMinutes(Date date, long minutes) {
return addSeconds(date, minutes * 60);
}
/**
* @param date1
* @param secs
*
* @return
*/
public static Date addSeconds(Date date1, long secs) {
return new Date(date1.getTime() + (secs * 1000));
}
/**
* 判断输入的字符串是否为合法的小时
*
* @param hourStr
*
* @return true/false
*/
public static boolean isValidHour(String hourStr) {
if (!StringUtils.isEmpty(hourStr) && StringUtils.isNumeric(hourStr)) {
int hour = new Integer(hourStr).intValue();
if ((hour >= 0) && (hour <= 23)) {
return true;
}
}
return false;
}
/**
* 判断输入的字符串是否为合法的分或秒
*
* @param minuteStr
*
* @return true/false
*/
public static boolean isValidMinuteOrSecond(String str) {
if (!StringUtils.isEmpty(str) && StringUtils.isNumeric(str)) {
int hour = new Integer(str).intValue();
if ((hour >= 0) && (hour <= 59)) {
return true;
}
}
return false;
}
/**
* 取得新的日期
*
* @param date1
* 日期
* @param days
* 天数
*
* @return 新的日期
*/
public static Date addDays(Date date1, long days) {
Calendar cal = Calendar.getInstance();
cal.setTime(date1);
cal.add(Calendar.DATE, (int) days);
return cal.getTime();
}
public static String getTomorrowDateString(String sDate) throws ParseException {
Date aDate = parseDateNoTime(sDate);
aDate = addSeconds(aDate, ONE_DAY_SECONDS);
return getDateString(aDate);
}
public static String getTomorrowDateNewFMTString(String sDate) throws ParseException {
Date aDate = parseDateWebFormat(sDate);
aDate = addDays(aDate, 1);
return getWebDateString(aDate);
}
public static String getTomorrowDateNewFormatString(String sDate) throws ParseException {
Date aDate = parseDateNewFormat(sDate);
aDate = addDays(aDate, 1);
return getWebDateString(aDate);
}
public static String getLongDateString(Date date) {
DateFormat dateFormat = new SimpleDateFormat(longFormat);
return getDateString(date, dateFormat);
}
public static String getNewFormatDateString(Date date) {
DateFormat dateFormat = new SimpleDateFormat(newFormat);
return getDateString(date, dateFormat);
}
public static String getWebFormatDateString(Date date) {
DateFormat dateFormat = new SimpleDateFormat(webFormat);
return getDateString(date, dateFormat);
}
public static String getConcurrentFormatDateString(Date date) {
DateFormat dateFormat = new SimpleDateFormat(concurrentFormat);
return getDateString(date, dateFormat);
}
public static String getDateString(Date date, DateFormat dateFormat) {
if (date == null || dateFormat == null) {
return null;
}
return dateFormat
没有合适的资源?快使用搜索试试~ 我知道了~
Java项目:在线考试平台(java+Springboot+ssm+mysql+maven)
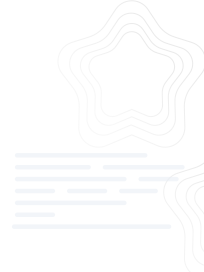
共1215个文件
png:175个
gif:162个
class:158个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
一、项目简述 功能列表 考试前台 /系统登录:学生、教师、管理员登录 /门户首页:无需认证访问 /在线考试:需认证即可查看当前考除目 /题库中心:需认证查看题库 /成绩查询:需认证查询成绩 /留言板:需认证留言 管理后台 /考:署理:发布考试,考试列表,课程管理,题库管 理,成绩管理,成绩情况 /权限管理:学院管理,班级管理,用户管理,角色管 理,资源管理 4网站管理:基础信息,友链管理,评论管理,标签管理 /系统管理:在线用户 /上传管理:云存储配置 /运维管理:数据监控 二、项目运行 环境配置: Jdk1.8 + Tomcat8.5 + mysql + Eclispe (IntelliJ IDEA,Eclispe,MyEclispe,Sts 都支持) 项目技术: JSP +SpringBoot + MyBatis + Redis+ Thymeleaf+ Druid+ JQuery + SLF4J+ Fileupload + maven等等
资源推荐
资源详情
资源评论
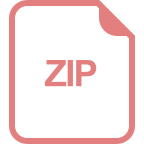
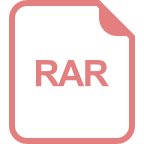
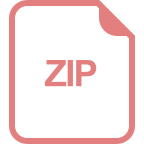
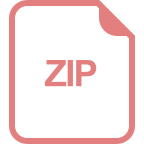
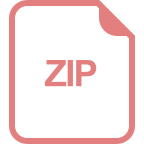
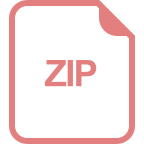
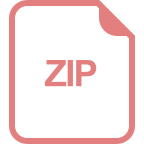
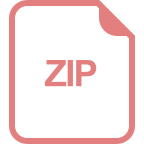
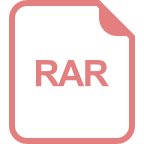
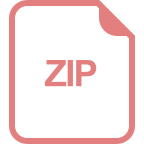
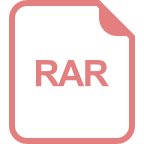
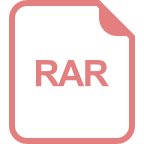
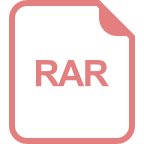
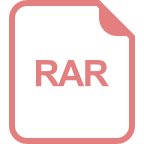
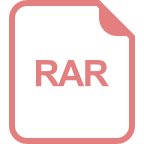
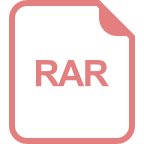
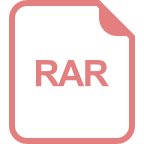
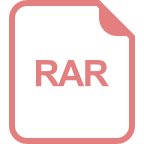
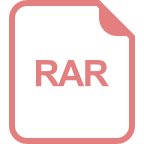
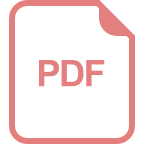
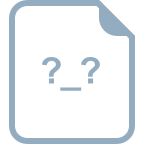
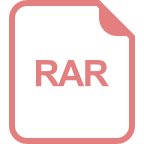
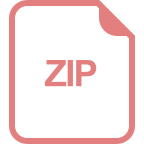
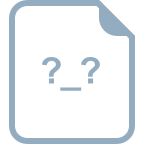
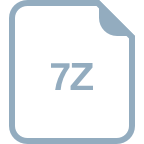
收起资源包目录

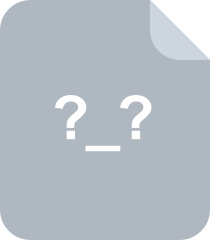
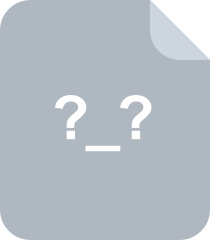
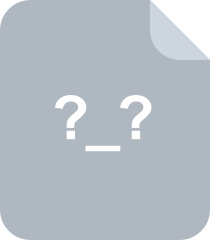
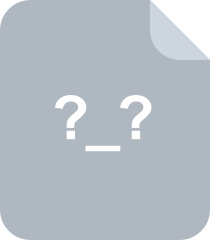
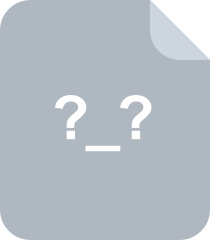
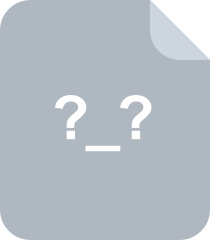
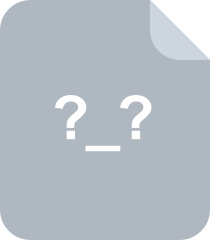
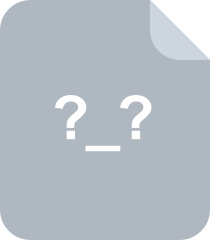
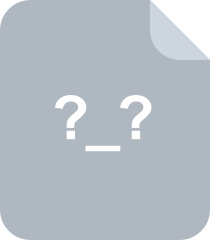
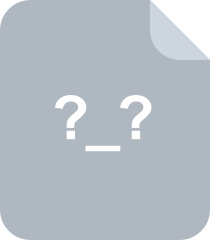
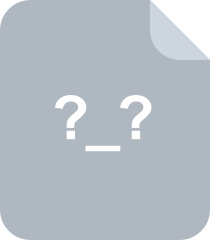
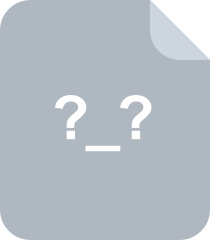
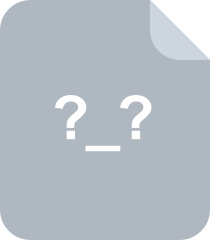
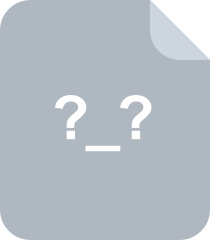
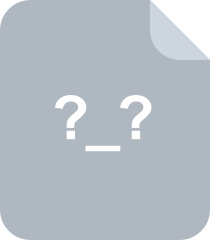
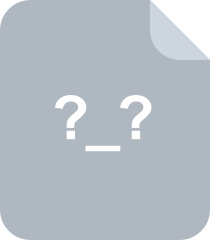
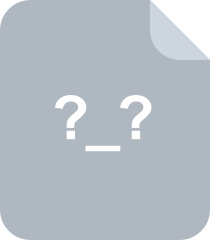
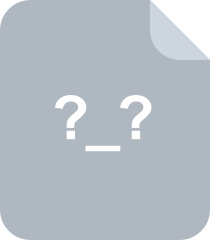
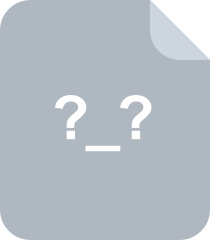
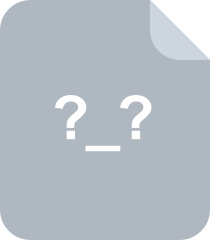
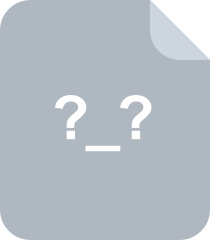
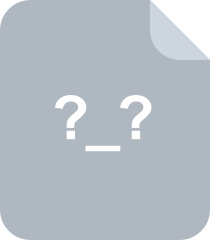
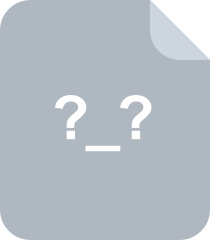
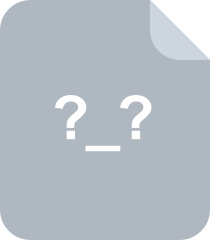
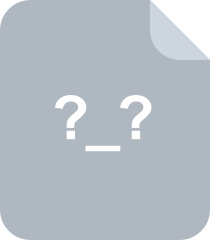
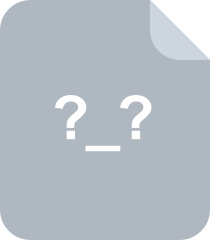
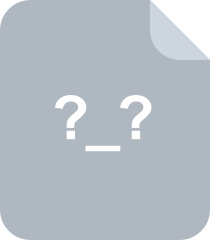
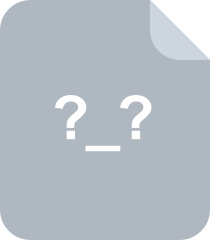
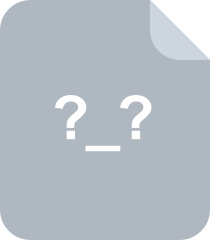
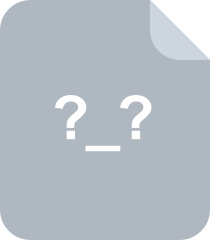
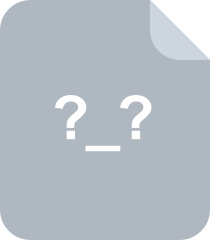
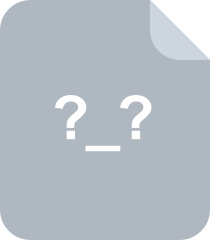
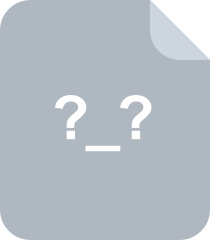
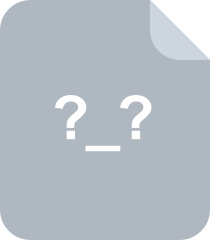
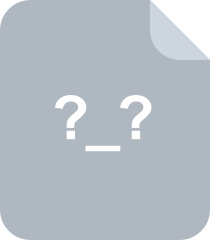
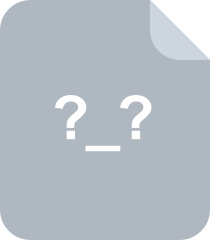
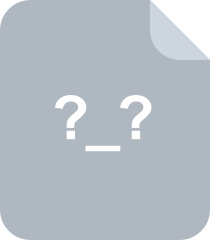
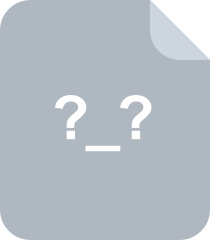
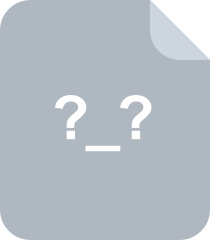
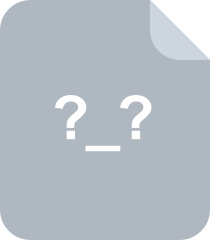
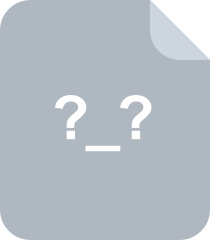
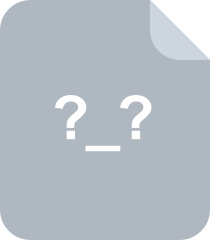
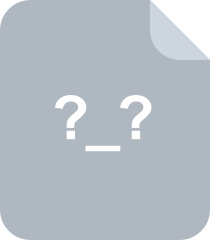
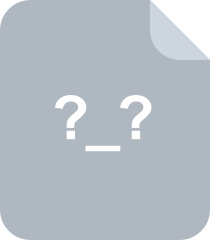
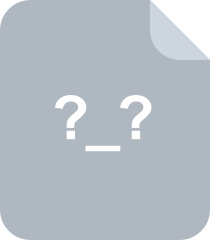
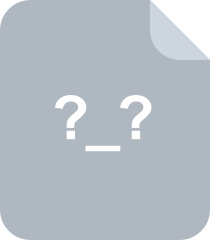
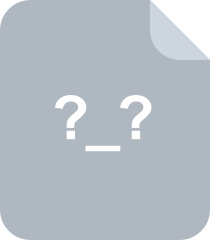
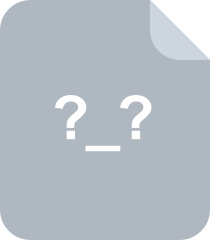
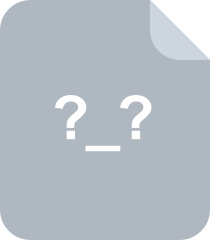
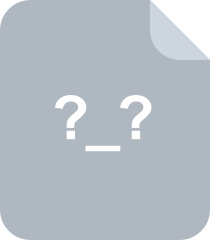
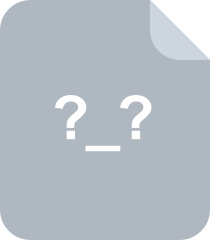
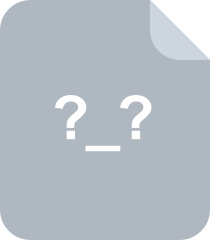
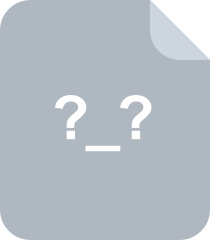
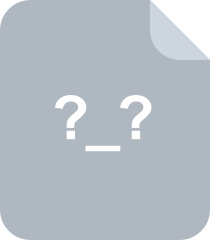
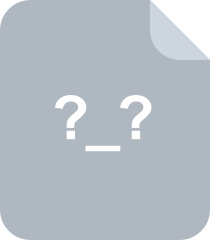
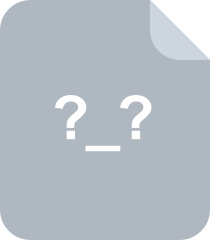
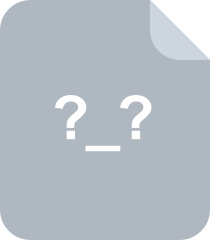
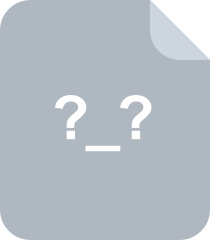
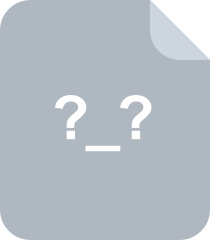
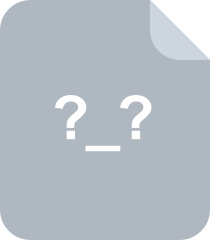
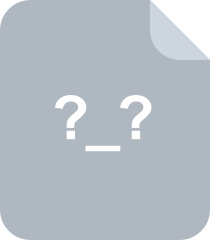
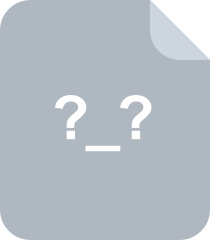
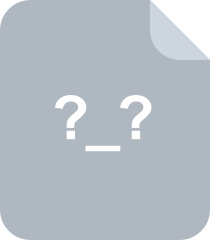
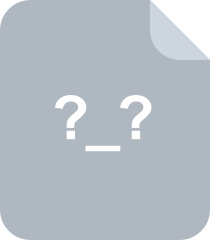
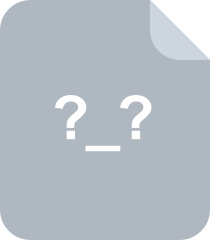
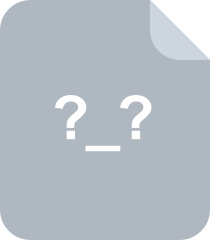
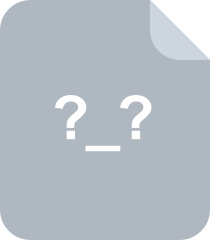
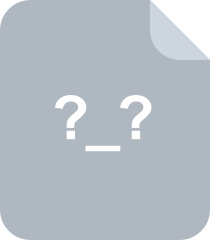
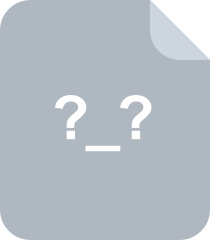
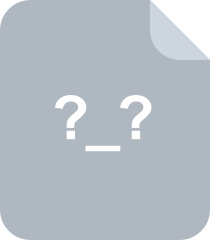
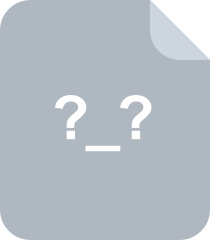
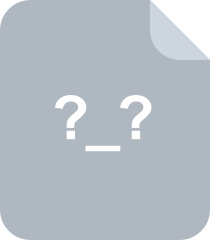
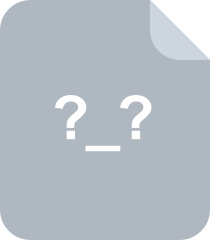
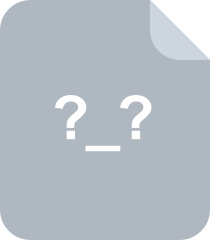
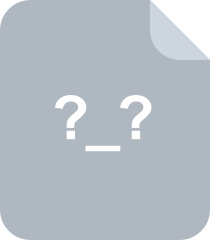
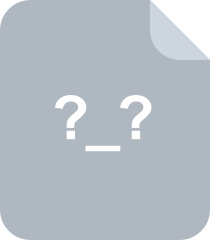
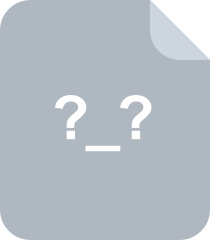
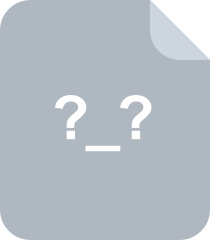
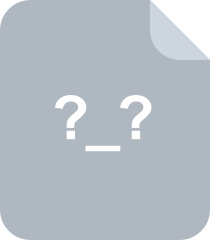
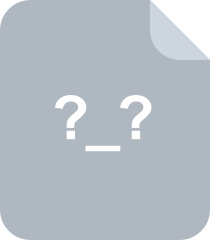
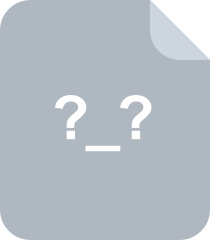
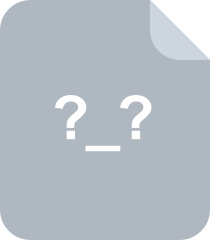
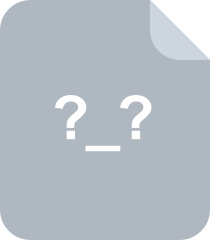
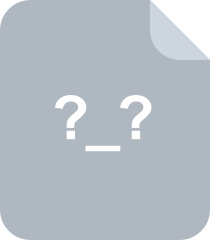
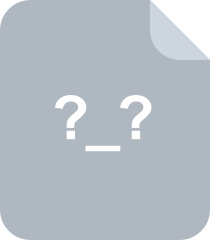
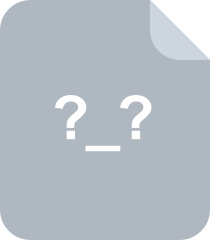
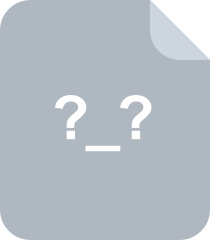
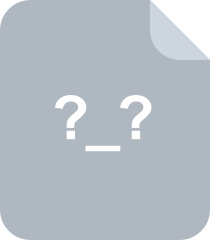
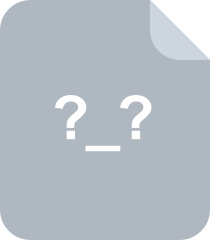
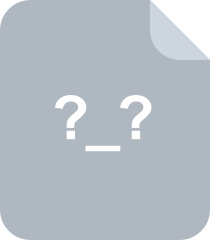
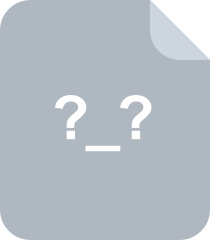
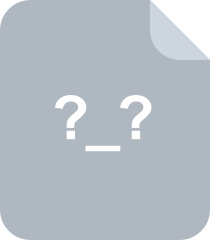
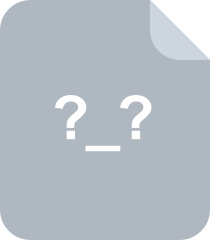
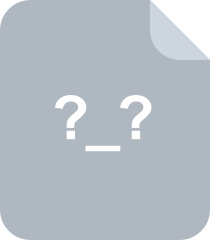
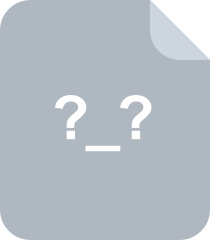
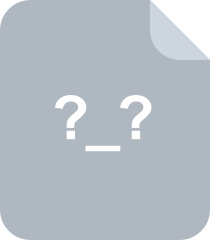
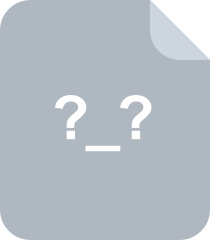
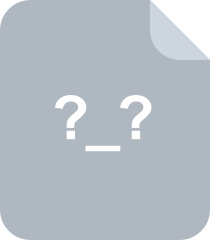
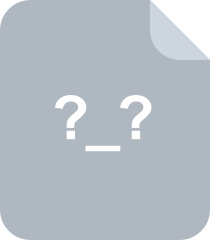
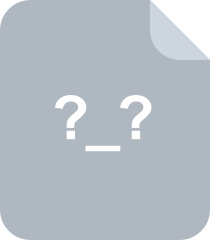
共 1215 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
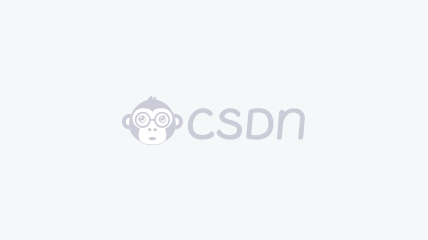
- yz_awen2022-06-19用户下载后在一定时间内未进行评价,系统默认好评。
- weixin_536061942022-06-08用户下载后在一定时间内未进行评价,系统默认好评。
- Letmegrow2021-12-21用户下载后在一定时间内未进行评价,系统默认好评。
- qq_536161372023-04-09感谢大佬分享的资源,对我启发很大,给了我新的灵感。

beyondwild
- 粉丝: 9869
- 资源: 4911
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

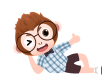
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


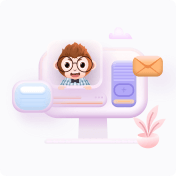
安全验证
文档复制为VIP权益,开通VIP直接复制
