/*
* GSM 07.10 Implementation with User Space Serial Ports
*
* Code heavily based on gsmMuxd written by
* Copyright (C) 2003 Tuukka Karvonen <tkarvone@iki.fi>
* Modified November 2004 by David Jander <david@protonic.nl>
* Modified January 2006 by Tuukka Karvonen <tkarvone@iki.fi>
* Modified January 2006 by Antti Haapakoski <antti.haapakoski@iki.fi>
* Modified March 2006 by Tuukka Karvonen <tkarvone@iki.fi>
* Modified October 2006 by Vasiliy Novikov <vn@hotbox.ru>
*
* Copyright (C) 2008 M. Dietrich <mdt@emdete.de>
* Modified January 2009 by Ulrik Bech Hald <ubh@ti.com>
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
#ifndef _GNU_SOURCE
#define _GNU_SOURCE
#endif
/* If compiled with the MUX_ANDROID flag this mux will be enabled to run under Android */
/**************************/
/* INCLUDES */
/**************************/
#include <errno.h>
#include <fcntl.h>
//#include <features.h>
#include <paths.h>
#ifdef MUX_ANDROID
#include <pathconf.h>
#endif
#include <signal.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/ioctl.h>
#include <sys/socket.h>
#include <sys/stat.h>
#include <sys/time.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <sys/poll.h>
#include <termios.h>
#include <time.h>
#include <unistd.h>
#include <pthread.h>
#include <stdlib.h>
//#define XINGWANG_DEBUG
#ifdef XINGWANG_DEBUG
//#include "ct_linuxframwork_log.h"
#define ct_linuxframwork_radiolog(lvl, tag, f, ...) do {{fprintf(stderr,"%d:%s(): " f "\n", __LINE__, __FUNCTION__, ##__VA_ARGS__);}}while(0)
#endif
#ifdef MUX_ANDROID //full path is required on some boards, i donot know why
int ql_system(const char * string) {
char system_bin[100];
strcpy(system_bin, "/system/bin/");
strcat(system_bin, string);
return system(system_bin);
}
#else
#define ql_system system
#endif
/**************************/
/* DEFINES */
/**************************/
/*Logging*/
#ifndef MUX_ANDROID
#include <syslog.h>
// #define LOG(lvl, f, ...) do{if(lvl<=syslog_level)syslog(lvl,"%s:%d:%s(): " f "\n", __FILE__, __LINE__, __FUNCTION__, ##__VA_ARGS__);}while(0)
#ifdef XINGWANG_DEBUG
#define DEBUG
#ifdef DEBUG
#define MUX_TAG "gsm0710"
#define LOGMUX(lvl,f,...) do {\
if (lvl <= LOG_ERR) {ct_linuxframwork_radiolog(CT_LOG_ERROR,MUX_TAG,f,##__VA_ARGS__);}\
else if (lvl <= LOG_WARNING) {ct_linuxframwork_radiolog(CT_LOG_WARN,MUX_TAG,f,##__VA_ARGS__);}\
else if (lvl <= LOG_INFO) {ct_linuxframwork_radiolog(CT_LOG_INFO,MUX_TAG,f,##__VA_ARGS__);}\
else if (lvl <= LOG_DEBUG) {ct_linuxframwork_radiolog(CT_LOG_DEBUG,MUX_TAG,f,##__VA_ARGS__);}\
else {};\
} while(0)
#define LOGMUXE(f,...) ct_linuxframwork_radiolog(CT_LOG_ERROR,MUX_TAG,f,##__VA_ARGS__)
#define LOGMUXW(f,...) ct_linuxframwork_radiolog(CT_LOG_WARN,MUX_TAG,f,##__VA_ARGS__)
#define LOGMUXD(f,...) ct_linuxframwork_radiolog(CT_LOG_DEBUG,MUX_TAG,f,##__VA_ARGS__)
#define LOGMUXI(f,...) ct_linuxframwork_radiolog(CT_LOG_INFO,MUX_TAG,f,##__VA_ARGS__)
#else
#define LOGMUX(lvl,f,...) do {} while(0)
#endif
#else
#define LOGMUX(lvl,f,...) do{if((lvl<=syslog_level) || ql_cmux_debug){\
if (logtofile){\
fprintf(muxlogfile,"%d:%s(): " f "\n", __LINE__, __FUNCTION__, ##__VA_ARGS__);\
fflush(muxlogfile);}\
else\
fprintf(stderr,"%d:%s(): " f "\n", __LINE__, __FUNCTION__, ##__VA_ARGS__);\
}\
}while(0)
#endif
#else //will enable logging using android logging framework (not to file)
#define LOG_TAG "RILC"
#include <utils/Log.h> //all Android LOG macros are defined here.
#define LOGMUX(lvl,f,...) do{if((lvl<=syslog_level) || ql_cmux_debug) {\
LOG_PRI(android_log_lvl_convert[lvl],LOG_TAG,"MUXD %d:%s(): " f, __LINE__, __FUNCTION__, ##__VA_ARGS__);}\
}while(0)
//just dummy defines since were not including syslog.h.
#define LOG_EMERG 0
#define LOG_ALERT 1
#define LOG_CRIT 2
#define LOG_ERR 3
#define LOG_WARNING 4
#define LOG_NOTICE 5
#define LOG_INFO 6
#define LOG_DEBUG 7
/* Android's log level are in opposite order of syslog.h */
int android_log_lvl_convert[8]={ANDROID_LOG_SILENT, /*8*/
ANDROID_LOG_SILENT, /*7*/
ANDROID_LOG_FATAL, /*6*/
ANDROID_LOG_ERROR,/*5*/
ANDROID_LOG_WARN,/*4*/
ANDROID_LOG_INFO,/*3*/
ANDROID_LOG_DEBUG,/*2*/
ANDROID_LOG_VERBOSE};/*1*/
#endif /*MUX_ANDROID*/
#define SYSCHECK(c) do{if((c)<0){LOGMUX(LOG_ERR,"%s %d system-error: '%s' (code: %d)", __func__, __LINE__, strerror(errno), errno);\
return -1;}\
}while(0)
/*MUX defines */
#define GSM0710_FRAME_FLAG 0xF9// basic mode flag for frame start and end
#define GSM0710_FRAME_ADV_FLAG 0x7E// advanced mode flag for frame start and end
#define GSM0710_FRAME_ADV_ESC 0x7D// advanced mode escape symbol
#define GSM0710_FRAME_ADV_ESC_COPML 0x20// advanced mode escape complement mask
#define GSM0710_FRAME_ADV_ESCAPED_SYMS { GSM0710_FRAME_ADV_FLAG, GSM0710_FRAME_ADV_ESC, 0x11, 0x91, 0x13, 0x93 }// advanced mode escaped symbols: Flag, Escape, XON and XOFF
// bits: Poll/final, Command/Response, Extension
#define GSM0710_PF 0x10//16
#define GSM0710_CR 0x02//2
#define GSM0710_EA 0x01//1
// type of frames
#define GSM0710_TYPE_SABM 0x2F//47 Set Asynchronous Balanced Mode
#define GSM0710_TYPE_UA 0x63//99 Unnumbered Acknowledgement
#define GSM0710_TYPE_DM 0x0F//15 Disconnected Mode
#define GSM0710_TYPE_DISC 0x43//67 Disconnect
#define GSM0710_TYPE_UIH 0xEF//239 Unnumbered information with header check
#define GSM0710_TYPE_UI 0x03//3 Unnumbered Acknowledgement
// control channel commands
#define GSM0710_CONTROL_PN (0x80|GSM0710_EA)//?? DLC parameter negotiation
#define GSM0710_CONTROL_CLD (0xC0|GSM0710_EA)//193 Multiplexer close down
#define GSM0710_CONTROL_PSC (0x40|GSM0710_EA)//??? Power Saving Control
#define GSM0710_CONTROL_TEST (0x20|GSM0710_EA)//33 Test Command
#define GSM0710_CONTROL_MSC (0xE0|GSM0710_EA)//225 Modem Status Command
#define GSM0710_CONTROL_NSC (0x10|GSM0710_EA)//17 Non Supported Command Response
#define GSM0710_CONTROL_RPN (0x90|GSM0710_EA)//?? Remote Port Negotiation Command
#define GSM0710_CONTROL_RLS (0x50|GSM0710_EA)//?? Remote Line Status Command
#define GSM0710_CONTROL_SNC (0xD0|GSM0710_EA)//?? Service Negotiation Command
// V.24 signals: flow control, ready to communicate, ring indicator,
// data valid
#define GSM0710_SIGNAL_FC 0x02
#define GSM0710_SIGNAL_RTC 0x04
#define GSM0710_SIGNAL_RTR 0x08
#define GSM0710_SIGNAL_IC 0x40//64
#define GSM0710_SIGNAL_DV 0x80//128
#define GSM0710_SIGNAL_DTR 0x04
#define GSM0710_SIGNAL_DSR 0x04
#define GSM0710_SIGNAL_RTS 0x08
#define GSM0710_SIGNAL_CTS 0x08
#define GSM0710_SIGNAL_DCD 0x80//128
//
#define GSM0710_COMMAND_IS(type, command) ((type & ~GSM0710_CR) == command)
#define GSM0710_FRAME_IS(type, frame) ((frame->control & ~GSM0710_PF) == type)
#ifndef min
#define min(a,b) ((a < b) ? a :b)
#endif
#define GSM0710_WRITE_RETRIES 5
#define GSM0710_MAX_CHANNELS 4
// Defines how often the modem is polled when automatic restarting is
// enabled The value is in seconds
#define GSM0710_POLLING_INTERVAL 5
#define GSM0710_BUFFER_SIZE 4096
static int write_frame(
int channel

Thor_Yu
- 粉丝: 1
- 资源: 2
最新资源
- Android 凭证交换和更新协议 - “你只需登录一次”.zip
- 2024 年 ICONIP 展会.zip
- 微信小程序毕业设计-基于SSM的电影交流小程序【代码+论文+PPT】.zip
- 微信小程序毕业设计-基于SSM的食堂线上预约点餐小程序【代码+论文+PPT】.zip
- 锐捷交换机的堆叠,一个大问题
- 微信小程序毕业设计-基于SSM的校园失物招领小程序【代码+论文+PPT】.zip
- MATLAB《结合萨克拉门托模型和遗传算法为乐安河流域建立一个水文过程预测模型》+项目源码+文档说明
- 基于人工神经网络/随机森林/LSTM的径流预测项目
- 微信小程序毕业设计-基于SSM的驾校预约小程序【代码+论文+PPT】.zip
- Aspose.Words 18.7 版本 Word转成PDF无水印
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


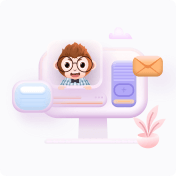