使用 JDBC 将 XML 中的数据插入到数据库
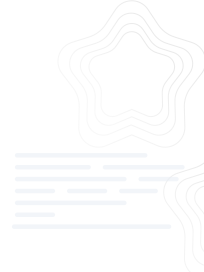

在IT行业中,数据库管理和数据处理是至关重要的环节。XML(eXtensible Markup Language)是一种广泛应用的数据交换格式,尤其在Web服务和数据存储中。JDBC(Java Database Connectivity)则是Java编程语言中用来连接和操作数据库的标准接口。本篇文章将详细讲解如何使用JDBC将XML中的数据插入到数据库。 我们需要理解XML的基本结构。XML是一种自描述的标记语言,它通过标签来定义数据元素。例如: ```xml <employees> <employee id="1"> <name>John Doe</name> <age>35</age> <position>Manager</position> </employee> <employee id="2"> <name>Jane Smith</name> <age>28</age> <position>Developer</position> </employee> </employees> ``` 上述XML文件表示了一个员工列表,其中每个`employee`标签代表一个员工,包含了`id`、`name`、`age`和`position`等信息。 接下来,我们将探讨如何使用JDBC来处理这个过程。你需要确保你的项目中已经引入了对应数据库的JDBC驱动。以MySQL为例,你需要添加以下Maven依赖: ```xml <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>8.0.23</version> </dependency> ``` 然后,你需要建立与数据库的连接: ```java import java.sql.Connection; import java.sql.DriverManager; Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/your_database", "username", "password"); ``` 为了将XML数据插入数据库,我们首先需要解析XML文件。Java中可以使用`javax.xml.parsers.DocumentBuilderFactory`和`javax.xml.parsers.DocumentBuilder`来实现: ```java import javax.xml.parsers.DocumentBuilderFactory; import javax.xml.parsers.DocumentBuilder; import org.w3c.dom.Document; import org.w3c.dom.NodeList; import org.w3c.dom.Node; import org.w3c.dom.Element; DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance(); DocumentBuilder dBuilder = dbFactory.newDocumentBuilder(); Document doc = dBuilder.parse("path_to_your_xml_file.xml"); doc.getDocumentElement().normalize(); NodeList nList = doc.getElementsByTagName("employee"); ``` 现在,我们可以遍历`nList`,将每个`employee`元素的数据插入数据库。假设我们有一个名为`employees`的表,其结构与XML文件匹配: ```sql CREATE TABLE employees ( id INT PRIMARY KEY, name VARCHAR(100), age INT, position VARCHAR(50) ); ``` 我们可以使用JDBC的`PreparedStatement`来执行插入操作,以避免SQL注入问题: ```java String sql = "INSERT INTO employees (id, name, age, position) VALUES (?, ?, ?, ?)"; try (PreparedStatement pstmt = conn.prepareStatement(sql)) { for (int i = 0; i < nList.getLength(); i++) { Node node = nList.item(i); if (node.getNodeType() == Node.ELEMENT_NODE) { Element element = (Element) node; int id = Integer.parseInt(element.getAttribute("id")); String name = element.getElementsByTagName("name").item(0).getTextContent(); int age = Integer.parseInt(element.getElementsByTagName("age").item(0).getTextContent()); String position = element.getElementsByTagName("position").item(0).getTextContent(); pstmt.setInt(1, id); pstmt.setString(2, name); pstmt.setInt(3, age); pstmt.setString(4, position); pstmt.executeUpdate(); } } } ``` 这段代码会循环遍历XML文件中的每个`employee`节点,提取出`id`、`name`、`age`和`position`,并用这些值填充`PreparedStatement`,然后执行插入操作。 别忘了关闭数据库连接: ```java conn.close(); ``` 这就是如何使用JDBC将XML文件中的数据插入到数据库的基本步骤。请注意,实际应用中可能需要处理异常,优化性能,以及考虑并发和事务管理等问题。在处理大量数据时,可能还需要批量插入或使用流式处理来提高效率。
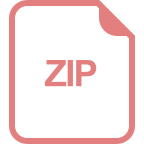
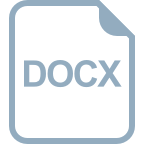
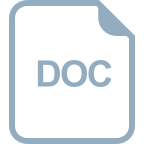
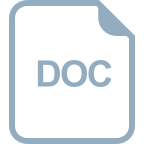
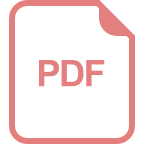
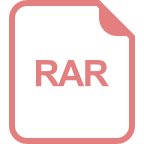
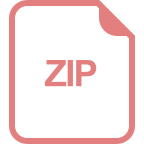
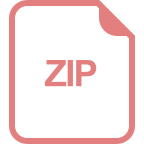
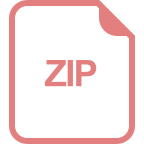
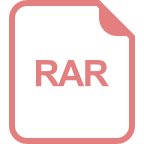
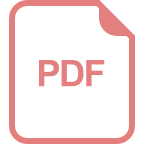
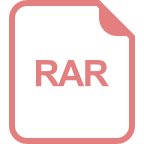
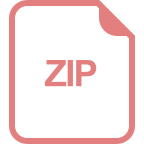
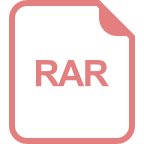
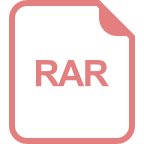

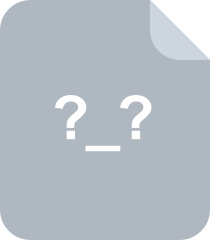
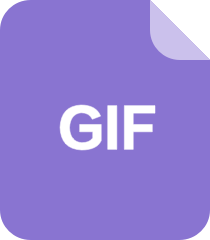
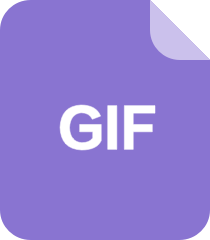
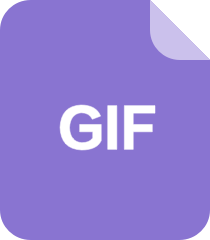
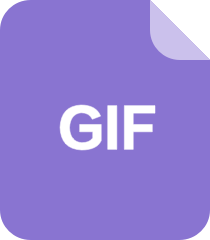
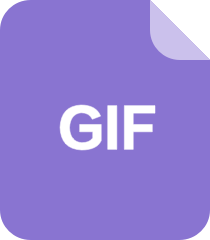
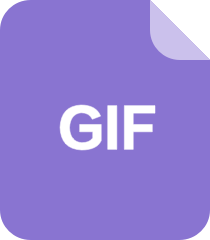
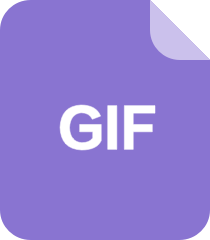
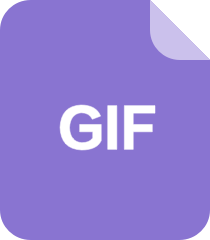
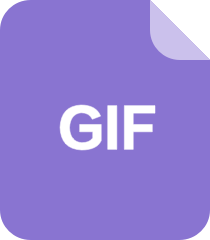
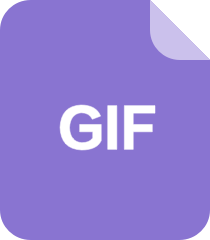
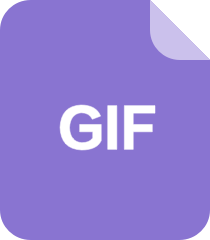
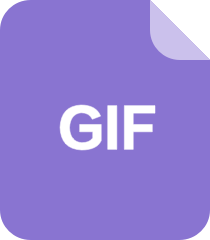
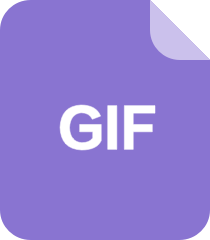
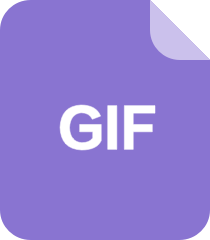
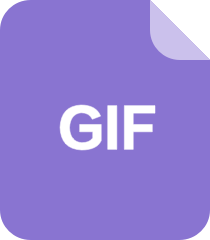
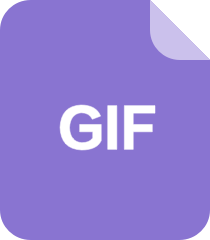
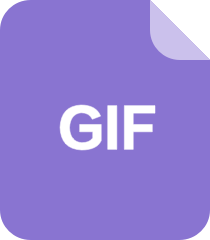
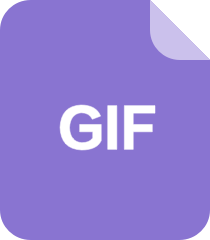
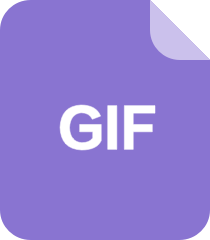
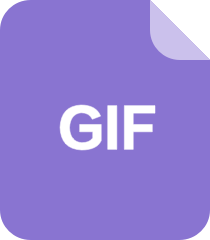
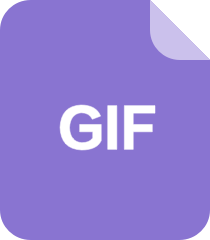
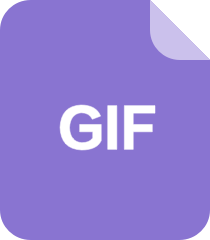
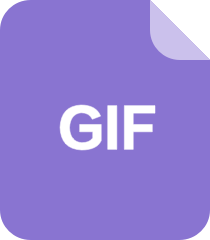
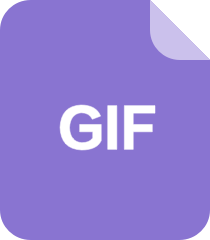
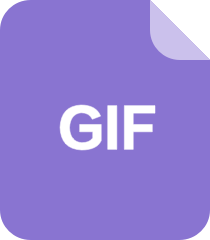
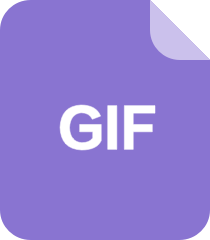
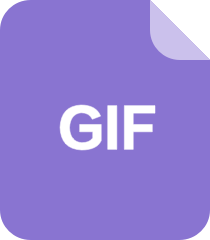
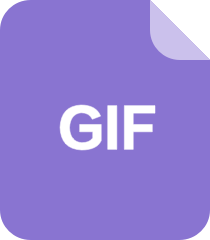
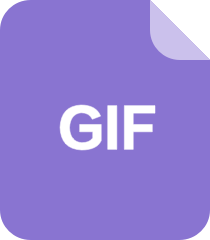
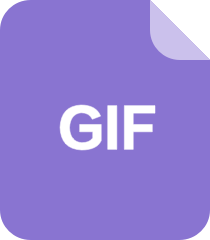
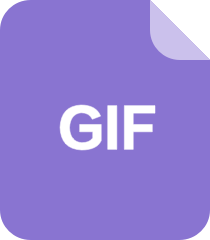
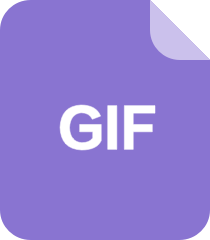
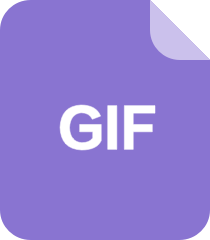
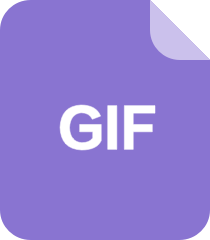
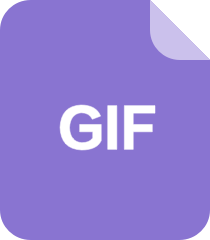
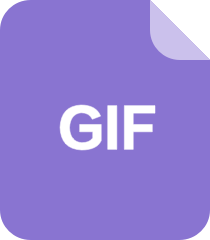
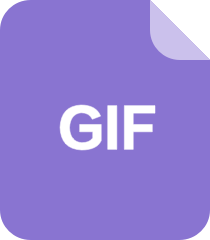
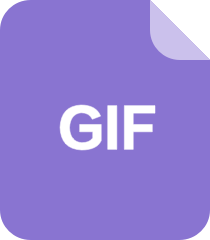
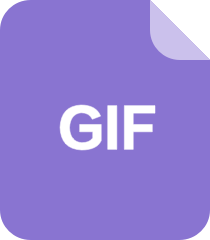
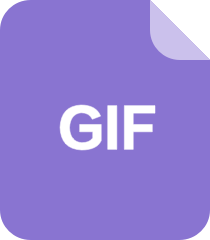
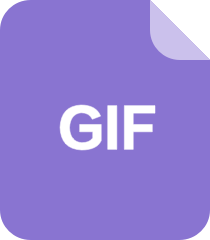
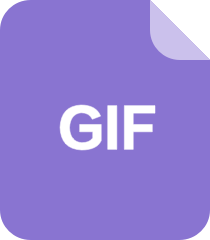
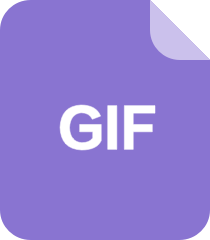
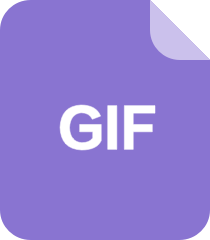
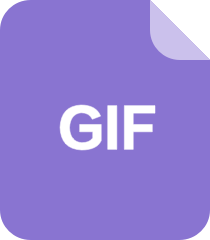
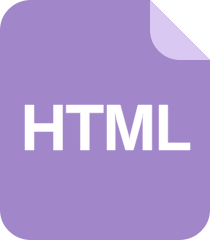
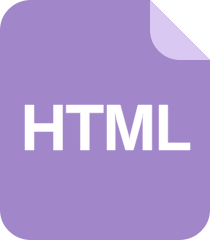
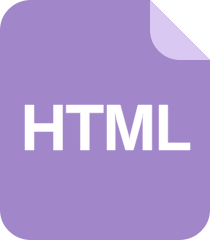
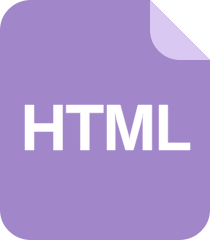
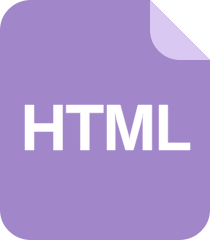
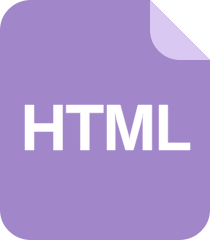
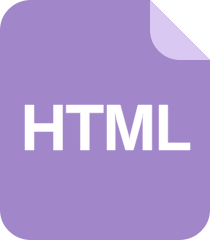
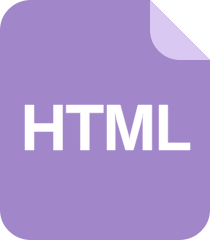
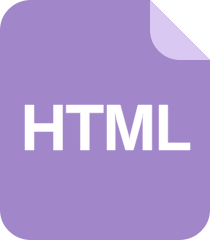
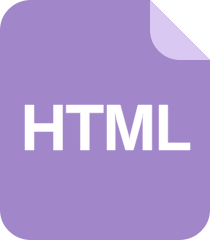
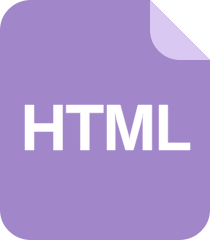
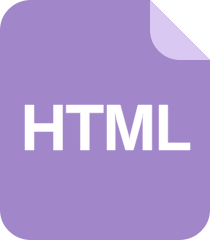
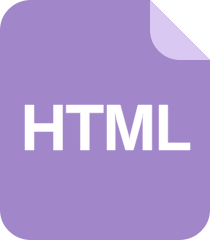
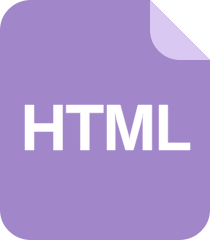
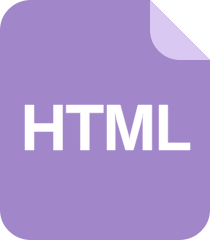
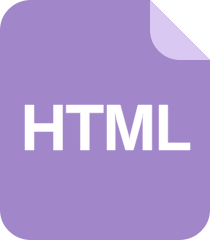
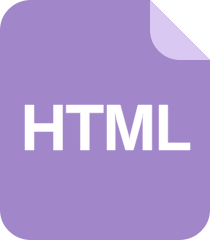
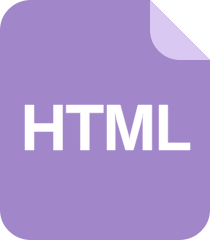
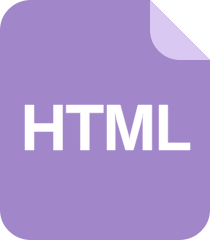
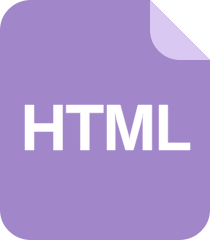
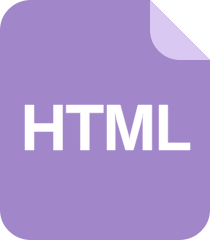
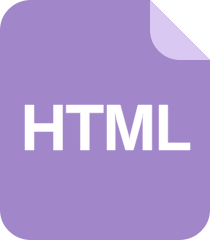
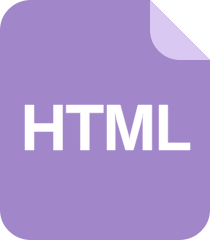
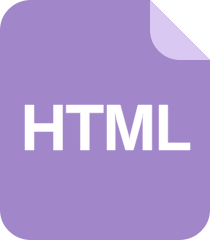
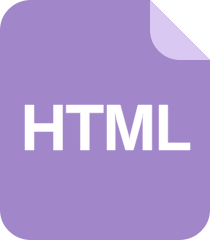
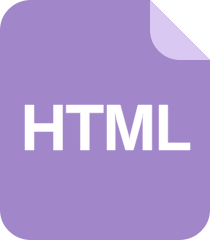
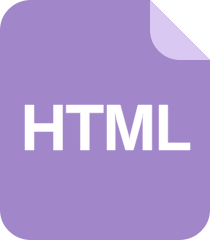
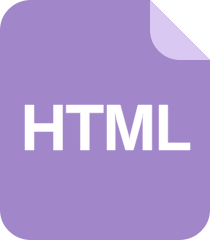
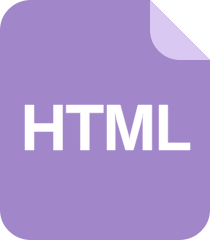
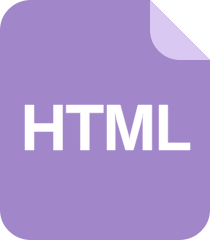
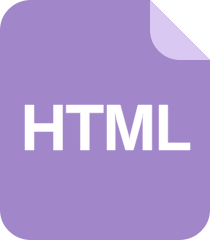
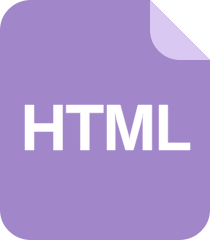
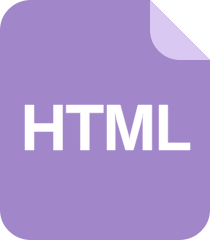
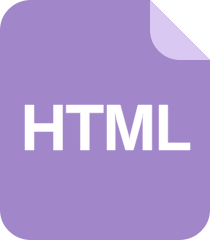
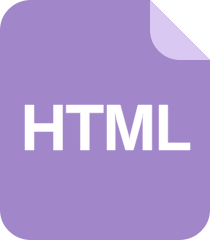
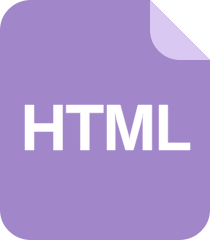
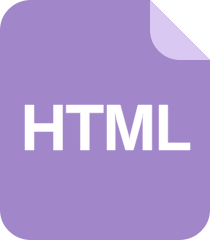
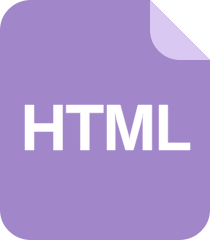
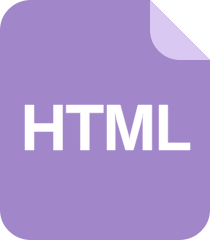
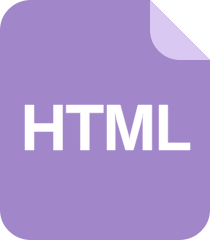
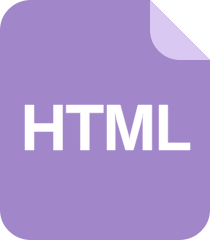
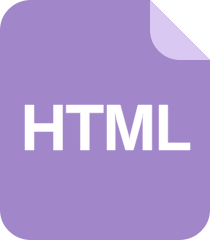
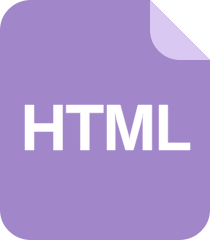
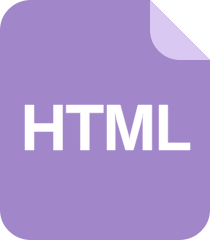
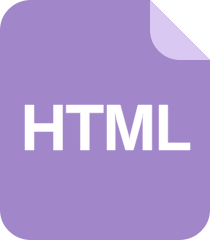
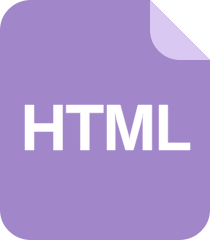
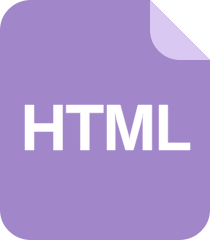
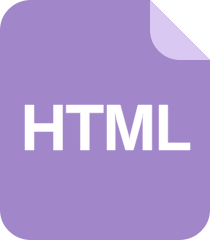
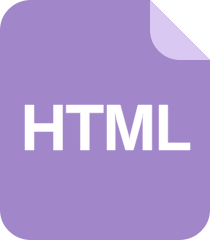
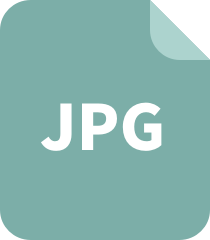
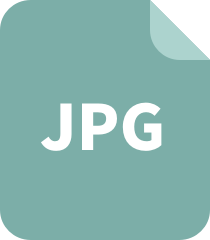
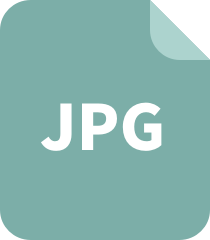
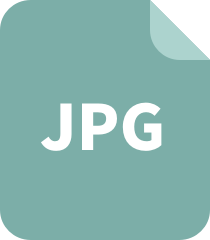
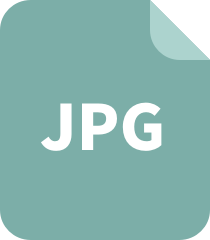
- 1
- 2
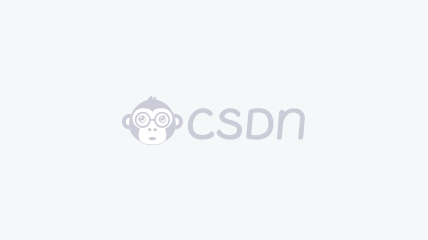

- 粉丝: 2
- 资源: 10
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

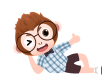
最新资源

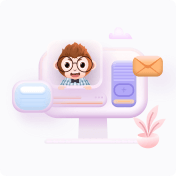
